一 概念
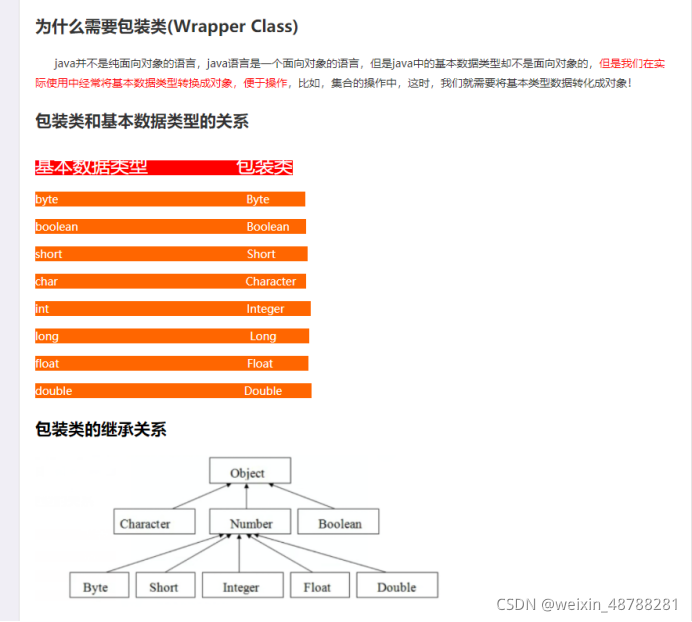
二 基本使用
例子:
public class Integer_01 {
public static void main(String[] args) {
//基本类型
byte b=10;
//转换为引用类型
Byte byte1=new Byte(b);
Integer i1 = new Integer(11);
Boolean b2 = new Boolean(false);
//方法调用传递
m1(byte1);//10 包装类都覆写了equals和toString
}
//需求:声明一个方法,该方法可以接受所有类型的值
//多态
public static void m1(Object obj){
System.out.println(obj);
// 包装类都覆写了equals和toString
}
}
2.1 Integer
2.1.1 Integer基本使用
public class Integer_02 {
public static void main(String[] args) {
System.out.println("int最大值"+Integer.MAX_VALUE);
System.out.println("int最小值"+Integer.MIN_VALUE);
System.out.println(Byte.MAX_VALUE);
System.out.println(Long.MAX_VALUE);
//创建Integer对象
//Int类型转换为Integer类型
Integer i1=new Integer(10);
// 可以直接把纯数字的字符串转换为Integer类型
Integer i2 = new Integer("10");
System.out.println(i1);//10
System.out.println(i2);//10
System.out.println(i1==i2);//false 都创建了对象,属于不同的对象,所以地址值不相等
// 覆写了equals
System.out.println(i1.equals(i2));//true
}
}
2.1.2 常用方法
public class Integer_03 {
public static void main(String[] args) {
// 1 对象 int->integer
Integer i1=new Integer(10);
//2 integer -> Int
int i2=i1.intValue();
System.out.println(i2);
//3 static int parseInt(String s); 把纯数字字符串转换为int类型
// String->int 必须是纯数字字符串,小数点也不行
int i3 = Integer.parseInt("123");
System.out.println(i3);//123
//String->double 转化为小数时,小数允许有一个小数点
double d = Double.parseDouble("3.2");
System.out.println(d);//3.2
// 将int类型的值,转换为二进制的字符串表示形式
// static String toBinaryString(int value);
String s1 = Integer.toBinaryString(12);
System.out.println(s1);//1100
// 转换为十六进制
System.out.println(Integer.toHexString(10));//a
// 转换为八进制
System.out.println(Integer.toOctalString(10));//12
// int --> Integer
Integer i4 = Integer.valueOf(10);
// String --> Integer
Integer i5 = Integer.valueOf("10");
System.out.println(i4 == i5);//true
}
}
2.1.3?Integer String int 三种类型的转换
public class Integer_04 {
public static void main(String[] args) {
// 1 int-->Integer
Integer i1=Integer.valueOf(10);
//2 Integer-->int
int i2=i1.intValue();
//3 String-->Integer
Integer i3=Integer.valueOf("22");
//4 Integer-->String
String s1=i3.toString();
System.out.println(s1);
//5 String-->int
int i4=Integer.parseInt("33");
//6 int-->String
String s2=2+"1";
System.out.println(s2);
}
}
2.1.4 自动装箱和自动拆箱
概念:
Jdk 1.5开始 新特性 ?? ? ? ? ? ? ?自动装箱: ?? ? ? ? ? ? ? ? ?把 基本类型 自动转换为 对应的包装类型 ?? ? ? ? ? ? ?自动拆箱: ?? ? ? ? ? ? ? ? ?把对应的包装类型自动转换为 基本类型 ??并且 自动装箱和自动拆箱是在编译阶段完成的
例子:
public class Integer_05 {
public static void main(String[] args) {
//装箱和拆箱
Integer i1 = Integer.valueOf(11);
int i2 = i1.intValue();
// 自动装箱和拆箱
Integer i3 = 2;
int i4 = i3;
// 此时 10 是int类型,int是没有办法转换为Object类型的
// 所以 需要把int 自动装箱为 Integer类型,然后发生多态,转换为Object类型
m1(10);
}
public static void m1(Object obj){
System.out.println(obj);
}
}
深入理解自动装箱和自动拆箱
1 都是编译时进行的操作 2 自动装箱的时候会把赋值操作 改变为?? ?Integer.valueOf(值) ? ? ? ?nteger.valueOf() 底层实现 : ? ? ? public static Integer valueOf(int i) { ? ? ? ? if (i >= IntegerCache.low && i <= IntegerCache.high) ? ? ? ? ? ? return IntegerCache.cache[i + (-IntegerCache.low)]; ? ? ? ? return new Integer(i); ? ? } ?? ? ? ? ?? ? ?? ????????IntegerCache 是Integer中的静态内部类,也就是我们说的整型常量池 ??? ?? ? ? ? ? ? ? static final int low = -128; ? ? ? ? ?? ??? ?static final int high = 127; ? ? ? ? ?? ??? ?static final Integer cache[]; ? ? ? ? ?? ?在static语句块中,对cache数组 进行初始化操作 ? ? ? ? ?? ??? ??? ? ?cache = new Integer[(high - low) + 1]; ?长度为256 ? ? ? ? ?? ??? ??? ? ?初始化数组中的数据 ? ? ? ? ?? ??? ??? ? cache[k] = new Integer(j++); ? ? ? ? ?? ??? ? 数组中数据为 -128,-127,-126.....127 ?共 256个数字,下标为0~255? ? ? ? ? ? ? ? ? ? ? ? ? ?? ??? ? 此时 整型常量池就初始化完成了,在堆内存中创建了256个对象,? ? ? ? ? ?valueOf方法中这么写的 ? ? ? ??? ??? ?// 判断 要赋值的数据值 是否在-128到127之间 ? ? ? ? ?? ?if (i >= IntegerCache.low && i <= IntegerCache.high) ? ? ? ? ?? ??? ?// 如果在这个范围内的话,之间在case数组中把对应的对象的地址拿出来,返回回去 ? ? ? ? ? ? ?? ?return IntegerCache.cache[i + (-IntegerCache.low)]; ? ? ? ? ? ? ?? ?// 如果不再这个范围内的话,就new一个新的对象,保存这个数据 ? ? ? ? ?? ?return new Integer(i);? ? ?? ? ? ? ? 所以 我们写 Integer i1 = 123; ?Integer i2 = 123; 使用 == 是相等的,因为他们指向堆内存的地址都是同一个? ? ? ? ? 反之 我们写 Integer i3 = 128; 就等于 Integer i3 = new Integer(128) , 如果使用== 肯定是不等的?
?例子:
public class Integer_06 {
public static void main(String[] args) {
// TODO Auto-generated method stub
// 自动装箱 = Integer.valueOf(123);
// 整型常量池的范围 : -128~127之间
Integer i1 = 123;
Integer i2 = 123;
System.out.println(i1 == i2); // true
i1 = new Integer(123);
i2 = new Integer(123);
System.out.println(i1 == i2); // false
// 这些写法 就等于 new Integer(128);
i1 = 128;
i2 = 128;
// false
System.out.println(i1 == i2);
String s1 = "abc";
String s2 = "abc";
System.out.println(s1 == s2);
s1 = new String("abc");
s2 = new String("abc");
System.out.println(s1 == s2);
}
}
三? Data
3.1 获取当前时间
public class Date_01 {
public static void main(String[] args) {
// TODO Auto-generated method stub
//获取当前系统时间
Date d=new Date();
System.out.println(d);
// 获取时间原点到指定毫秒数的时间
// d=new Date(1000);
// System.out.println(d);//Thu Jan 01 08:00:01 CST 1970
//时间格式化
//年 y , 月 M , 日 d , 小时 H , 分 m , 秒 s , 毫秒 S
//创建格式化对象,并指定格式
SimpleDateFormat sdf=new SimpleDateFormat("yyyy年MM月dd日 HH:mm:ss SSS");
//对时间进行格式化,返回字符串类型
String strDate=sdf.format(d);
System.out.println(strDate);//2021年10月19日 18:48:17 044
//解析字符串格式必须和解析格式一致
try {
Date d2=sdf.parse(strDate);
System.out.println(d2);
} catch (ParseException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
3.2 增强
public class Date_02 {
public static void main(String[] args) {
//获取当前时间毫秒数
long time=System.currentTimeMillis();
System.out.println(time);
//减去十分钟的毫秒数
time-=10*60*1000;
//转换为Date格式
Date d=new Date(time);
//格式化
SimpleDateFormat sdf=new SimpleDateFormat("yyyy年MM月dd日 HH:mm:ss SSS");
String strDate=sdf.format(d);
System.out.println(strDate);
}
}
3.3 System
3.3.1 概念
system代表系统,系统很多的系统属性和控制方法都在这个类中,位与java.lang包下 ?? long currentTimeMillis() : 获取当前系统时间的毫秒数 , 从1970-1-1 0:0:0 000开始 到现在的时间毫秒数 ?? 我们这个地区为东八区 ? 时间为 1970.1.1 8:00:00 000 ?? void exit(int status) : 退出虚拟机, 参数为0 表示正常退出 非0 表示异常退出,常用于图形界面,实现退出功能
例子:
public class System_01 {
public static void main(String[] args) {
//计算时间差
long startTime = System.currentTimeMillis();
String[] strs = { "a", "b", "c", "d", "e", "f", "a", "b", "c", "d" };
// String temp = "";
// for (int i = 0; i < strs.length; i++) {
// temp += strs[i] + ",";
// }
StringBuilder sb = new StringBuilder();
for (int i = 0; i < strs.length; i++) {
sb.append(strs[i] + ",");
}
String temp = sb.toString();
System.out.println(temp);
long endTime = System.currentTimeMillis();
// 关闭JVM , 0 正常退出, 非0 异常退出, 一般用于关闭图形界面
//也就是说,后面的程序都不执行了
System.exit(0);
System.out.println("程序运行时间为:"+(endTime-startTime)+"毫秒");
}
}
?四 Math
4.1 概念
Math: 提供科学计算和基本的数学操作,常用方法都是静态的,使用类名直接调用即可 ?在java.lang下面,使用不需要导包
4.2 基本使用
public class Math_01 {
public static void main(String[] args) {
//abs 绝对值
System.out.println(Math.abs(-100));
//ceil 向上取整
System.out.println(Math.ceil(3.6));
//floor 向下取整
System.out.println(Math.floor(3.6));
//max 比较谁大
System.out.println(Math.max(1, 2));
//min 比较谁小
System.out.println(Math.min(1, 2));
//sqrt 平方根
System.out.println(Math.sqrt(16));
//cbrt 立方根
System.out.println(Math.cbrt(8));
System.out.println("========================");
//随机数:获取一个大于等于0且小于1的数
System.out.println(Math.random());
//随机范围[最小值最大值]: 向下取整( 随机数*(最大-最小 +1) + 最小)
//eg: 随机取一个在[10,20]范围里的数
System.out.println(Math.floor(Math.random()*10+10));
// 四舍五入 : 四舍六入五留双, 小数大于0.5 就进位,小于0.5就舍弃,如果是0.5整 , 取偶数
// 2.50001 : 3 , 3.50000 : 4 , 2.50000 : 2
System.out.println(Math.rint(2.5001));
// 2的3次方
System.out.println(Math.pow(2, 3));
}
}
五 BigInteger
特点:精度极高
基本使用:
public class BigInteger_01 {
public static void main(String[] args) {
//参数是字符串
BigInteger v0=new BigInteger("11");
System.out.println(v0);
//参数是数值
BigDecimal v1=new BigDecimal(1000);
System.out.println(v1);
BigDecimal v2 = new BigDecimal(111);
// +
BigDecimal v3=v1.add(v2);
System.out.println(v3);//1111
// -
v3 = v1.subtract(v2);
System.out.println(v3);//889
// *
v3 = v1.multiply(v2);
System.out.println(v3);//111000
// /
// v3=v1.divide(v2);
v3 = v1.divide(v2, 2, BigDecimal.ROUND_HALF_UP);//9.01
// 原来JAVA中如果用BigDecimal做除法的时候一定要在divide方法中传递第二个参数,定义精确到小数点后几位,否则在不整除的情况下,结果是无限循环小数时,就会抛出以上异常。
// 解决方法:
//
// v3 = v1.divide(v2, 2, BigDecimal.ROUND_HALF_UP);
System.out.println(v3);
// // %
// v3 = v1.remainder(v2);
// System.out.println(v3);
System.out.println(Long.MAX_VALUE);
BigDecimal sum = new BigDecimal(1);
for (int i = 1; i <=100; i++) {
sum = sum.multiply(new BigDecimal(i));
}
System.out.println(sum);
}
}
六 random(随机数)
1 Math类中方法
//随机数:获取一个大于等于0且小于1的数
?? ?System.out.println(Math.random());
?? ?//随机范围[最小值最大值]: 向下取整( 随机数*(最大-最小 +1) + 最小)
?? ?//eg: 随机取一个在[10,20]范围里的数
?? ?System.out.println(Math.floor(Math.random()*10+10));
2 Random生成器
public class Random_01 {
public static void main(String[] args) {
// 创建随机数生成器
Random r = new Random();
// 大于等于0 且小于10的整数
int result = r.nextInt(10);
System.out.println(result);
// 生成 10~20
// nextInt(最大值 - 最小值 +1) + 最小值
result = r.nextInt(11) + 10;
System.out.println(result);
// 生成a~z
result = r.nextInt(26);
System.out.println(result);
char c = (char) (result + 97);
System.out.println(c);
}
}
?
|