第13章:IO流
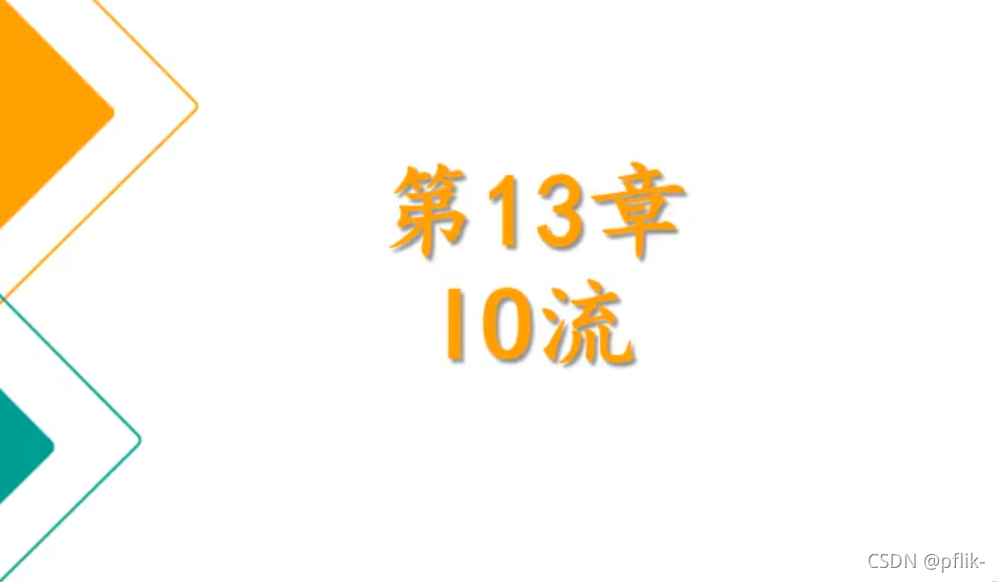
目录:
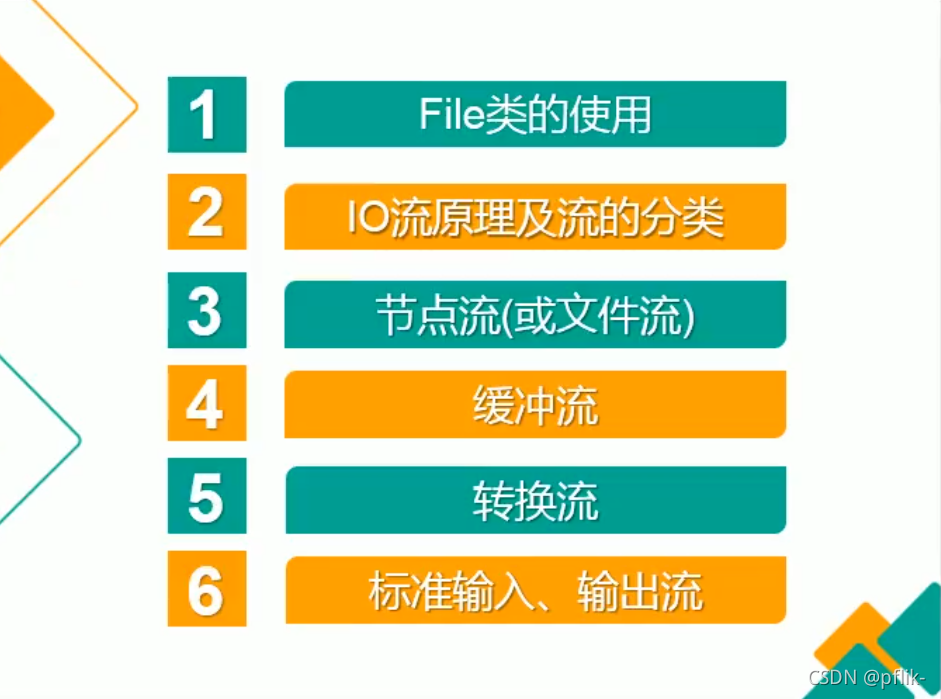 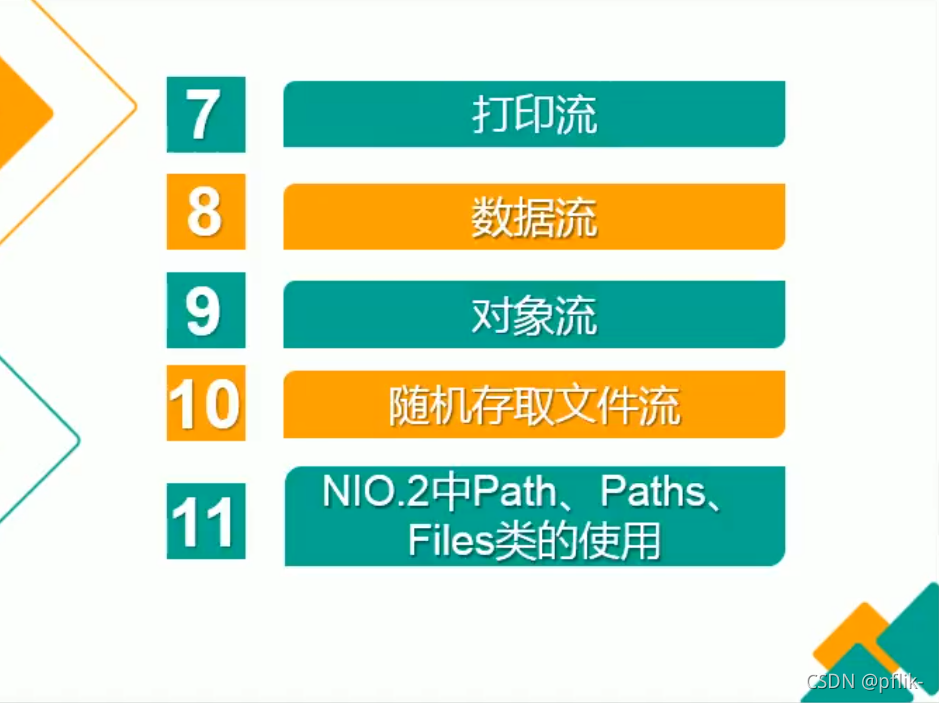
13.1、File类的使用
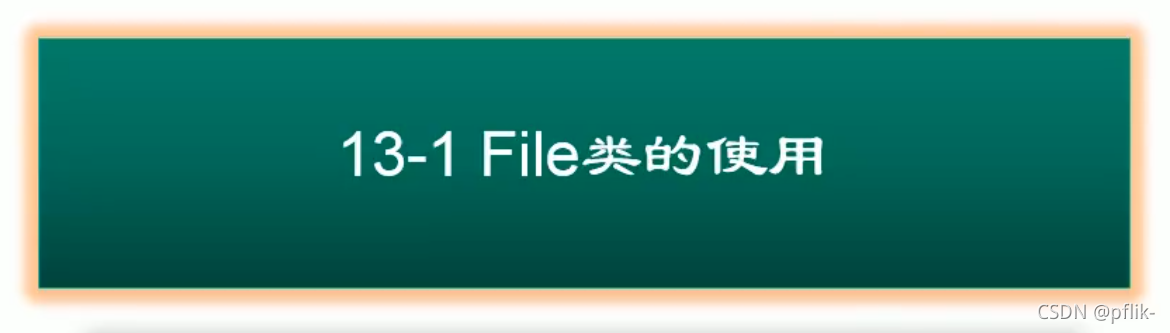 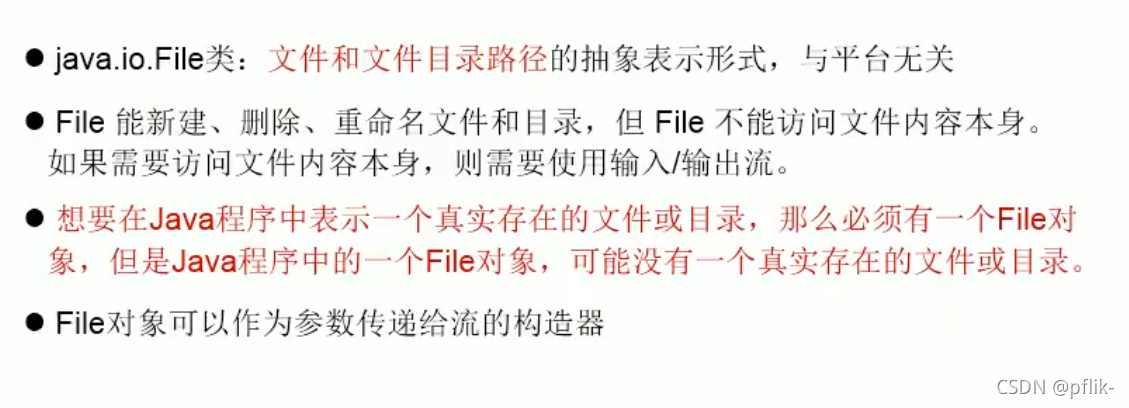 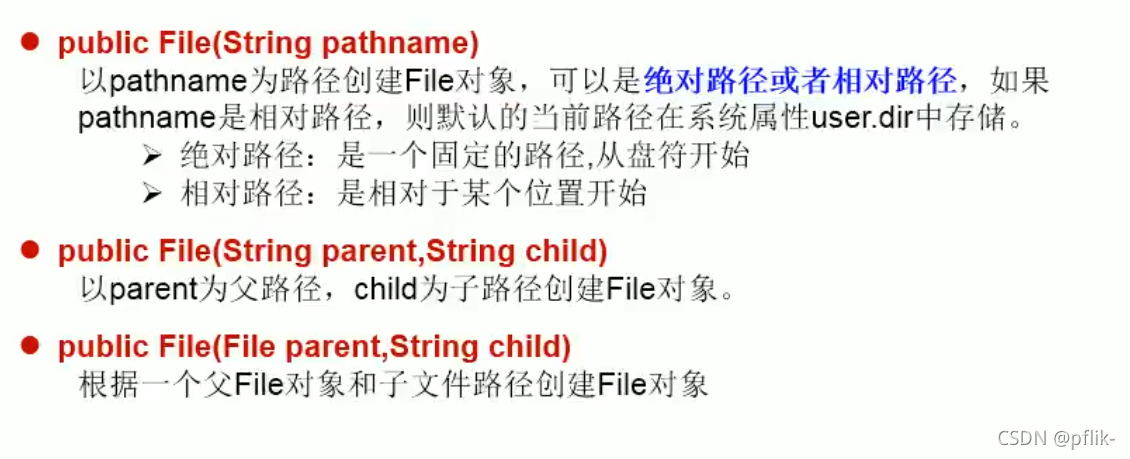 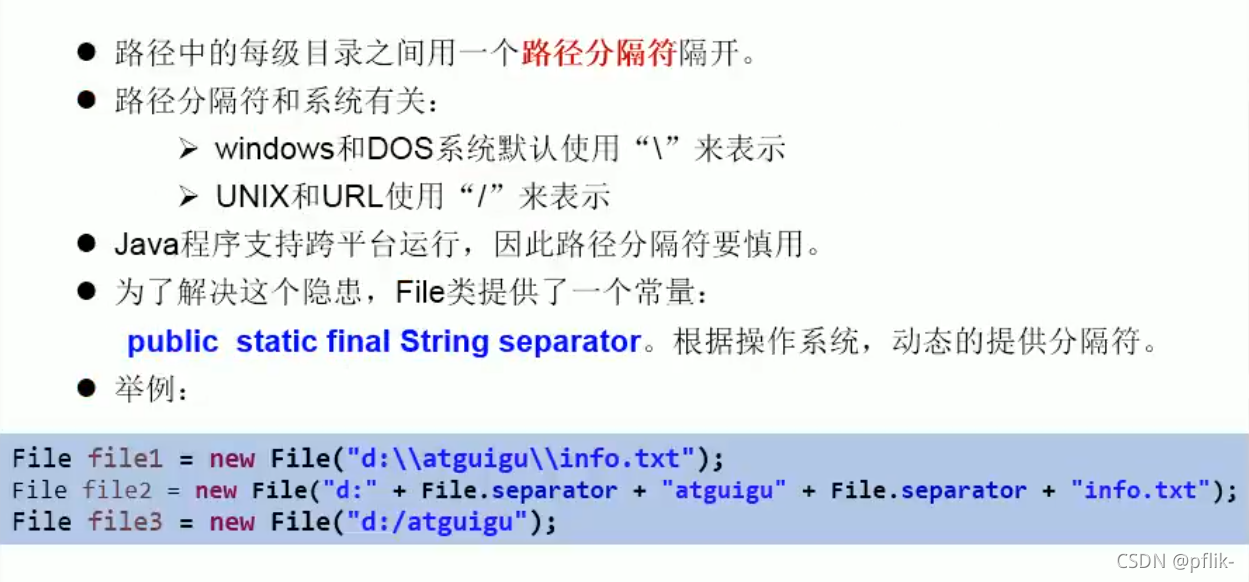 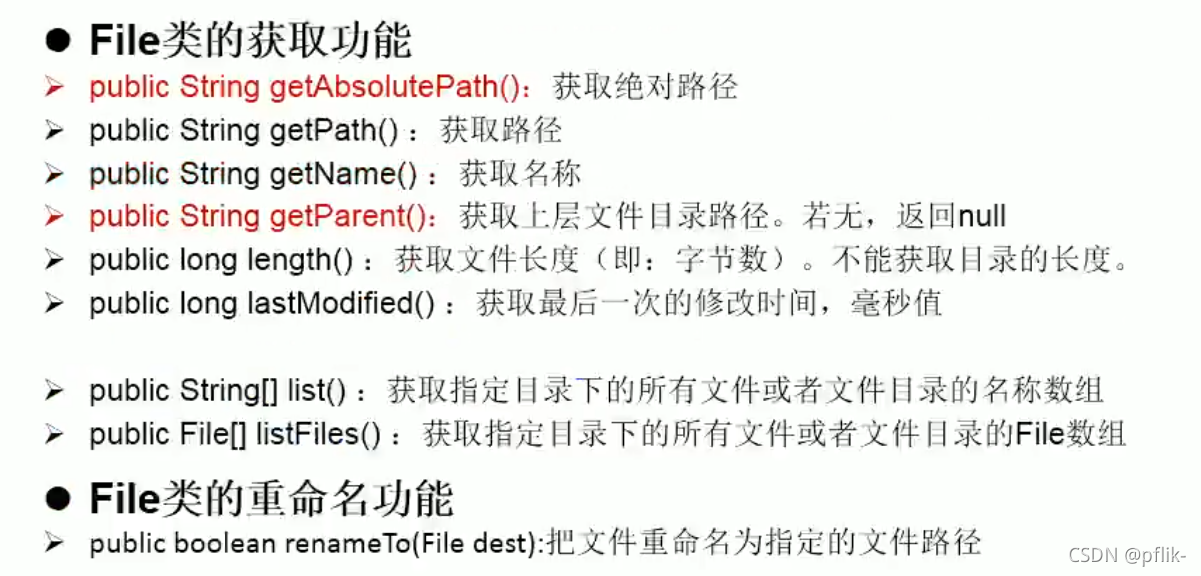 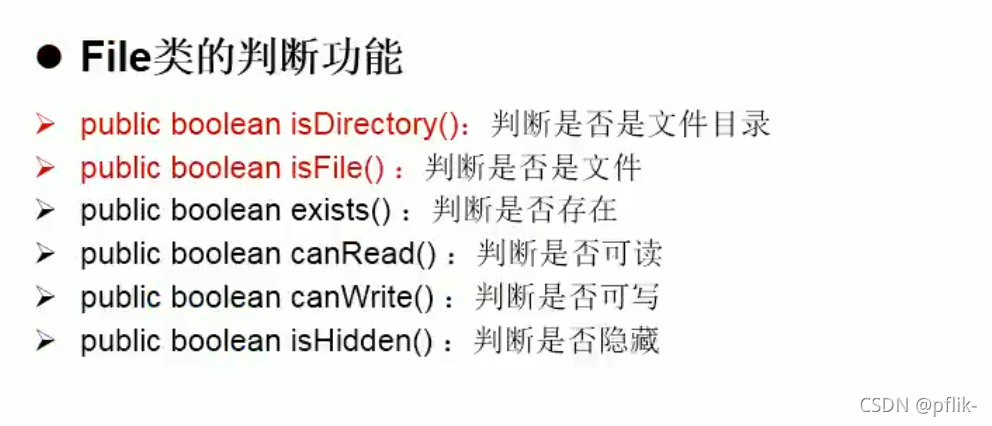 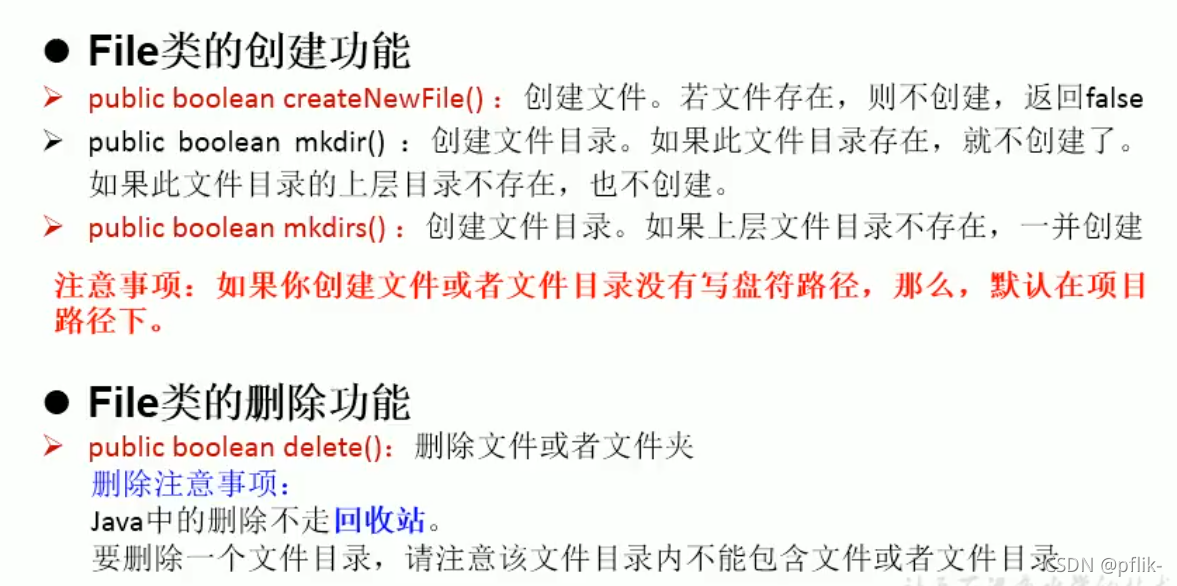 FileTest
package com.pfl.java3;
import org.junit.Test;
import java.io.File;
import java.io.IOException;
import java.util.Date;
public class FileTest {
@Test
public void test1() {
File file1 = new File("hello.txt");
File file2 = new File("D:\\Software\\Java\\WorkSpace\\workspace-idea1\\day08\\hello.txt");
System.out.println(file1);
System.out.println(file2);
File file3 = new File("D:\\Software","Java");
System.out.println(file3);
File file4 = new File(file3,"he.txt");
System.out.println(file4);
}
@Test
public void test2() {
File file1 = new File("hello.txt");
File file2 = new File("D:\\Software\\Java\\WorkSpace\\io\\hello.txt");
System.out.println(file1.getAbsolutePath());
System.out.println(file1.getPath());
System.out.println(file1.getName());
System.out.println(file1.getParent());
System.out.println(file1.length());
System.out.println(new Date(file1.lastModified()));
System.out.println("*****************");
System.out.println(file2.getAbsolutePath());
System.out.println(file2.getPath());
System.out.println(file2.getName());
System.out.println(file2.getParent());
System.out.println(file2.length());
System.out.println(file2.lastModified());
}
@Test
public void test3() {
File file = new File("D:\\Software\\Java\\WorkSpace\\workspace-idea1");
String[] list = file.list();
for(String s : list) {
System.out.println(s);
}
System.out.println("*****************************");
File[] files = file.listFiles();
for (File f : files) {
System.out.println(f);
}
}
@Test
public void test4() {
File file1 = new File("hello.txt");
File file2 = new File("D:\\Software\\Java\\WorkSpace\\io\\hi.txt");
boolean renameTo = file2.renameTo(file1);
System.out.println(renameTo);
}
@Test
public void test5() {
File file1 = new File("hello.txt");
System.out.println(file1.isDirectory());
System.out.println(file1.isFile());
System.out.println(file1.exists());
System.out.println(file1.canRead());
System.out.println(file1.canWrite());
System.out.println(file1.isHidden());
System.out.println("*************************");
file1 = new File("hello1.txt");
System.out.println(file1.isDirectory());
System.out.println(file1.isFile());
System.out.println(file1.exists());
System.out.println(file1.canRead());
System.out.println(file1.canWrite());
System.out.println(file1.isHidden());
System.out.println("*************************");
File file2 = new File("D:\\Software\\Java\\WorkSpace\\io");
System.out.println(file2.isDirectory());
System.out.println(file2.isFile());
System.out.println(file2.exists());
System.out.println(file2.canRead());
System.out.println(file2.canWrite());
System.out.println(file2.isHidden());
System.out.println("*************************");
}
@Test
public void test6() throws IOException {
File file1 = new File("hi.txt");
if(!file1.exists()) {
file1.createNewFile();
System.out.println("创建成功!");
} else {
file1.delete();
System.out.println("删除成功");
}
}
@Test
public void test7() {
File file1 = new File("D:\\Software\\Java\\WorkSpace\\io\\io1\\io3");
boolean mkdir = file1.mkdir();
if(mkdir) {
System.out.println("创建成功1");
}
File file2 = new File("D:\\Software\\Java\\WorkSpace\\io\\io1\\io4");
boolean mkdir2 = file1.mkdirs();
if(mkdir2) {
System.out.println("创建成功2");
}
File file3 = new File("D:\\Software\\Java\\WorkSpace\\io\\io1\\io3");
file3 = new File("D:\\Software\\Java\\WorkSpace\\io");
System.out.println(file3.delete());
}
}
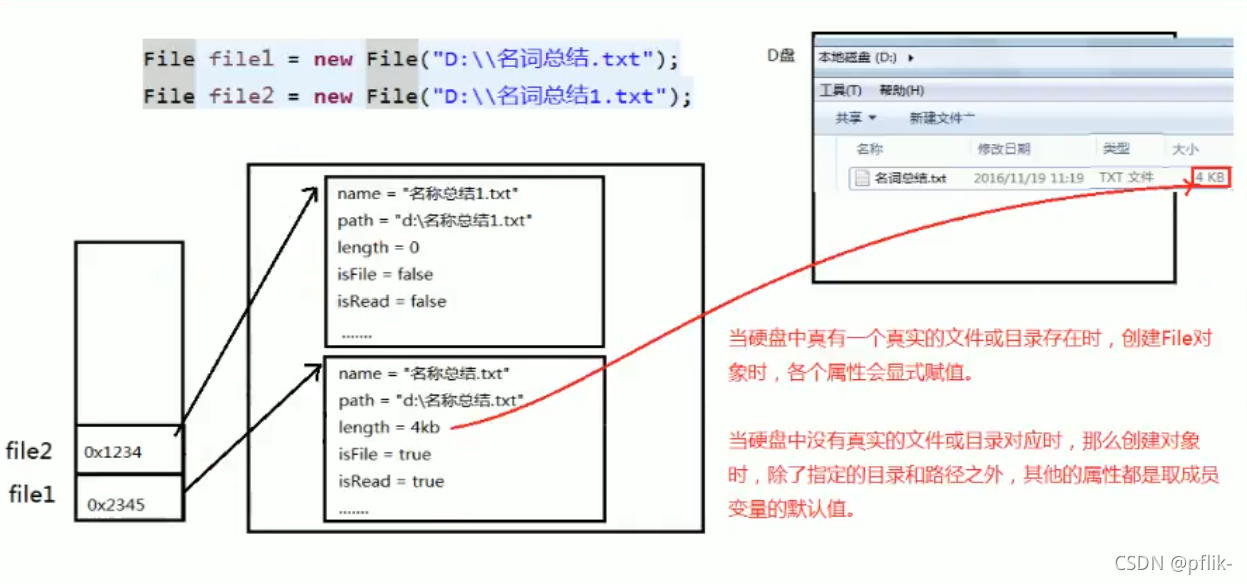
练习:
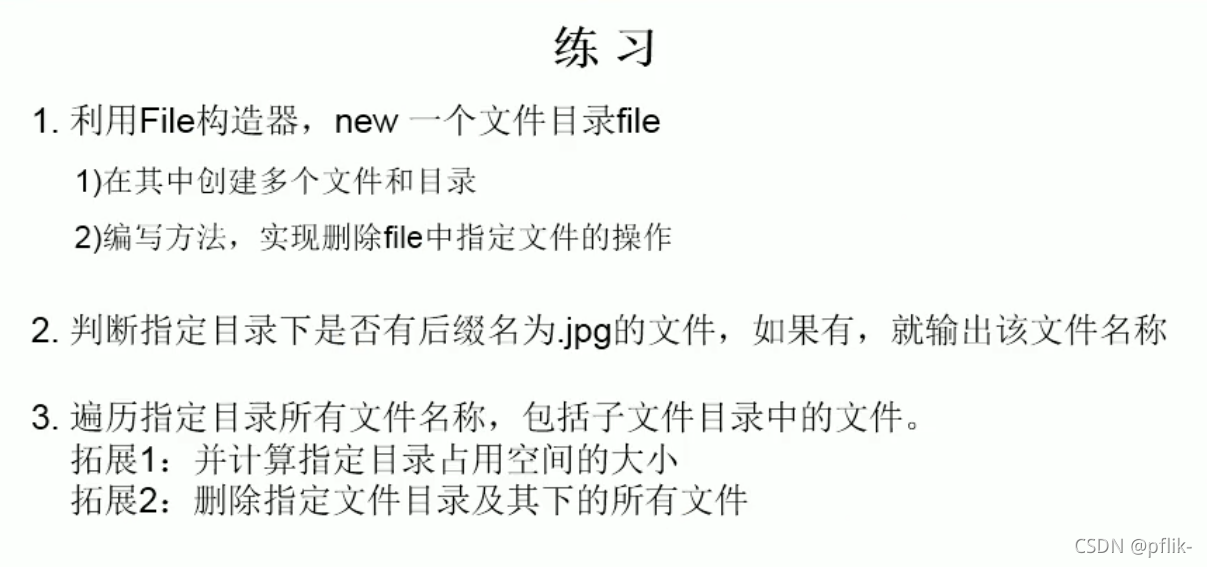 FileDemo
package com.pfl.exer2;
import org.junit.Test;
import java.io.File;
import java.io.IOException;
public class FileDemo {
@Test
public void test1() throws IOException {
File file = new File("D:\\Software\\Java\\WorkSpace\\io\\io1\\hello.txt");
File destFile = new File(file.getParent(), "haha.txt");
boolean newFile = destFile.createNewFile();
if (newFile) {
System.out.println("创建成功!");
}
}
}
FindGPJFileTest:
package com.pfl.exer2;
import org.junit.Test;
import java.io.File;
import java.io.FilenameFilter;
public class FindGPJFileTest {
@Test
public void test1() {
File srcFile = new File("D:\\Software\\Java\\WorkSpace\\io");
String[] fileNames = srcFile.list();
for (String fileName : fileNames) {
if (fileName.endsWith(".jpg")) {
System.out.println(fileName);
}
}
}
@Test
public void test2() {
File srcFile = new File("D:\\Software\\Java\\WorkSpace\\io");
File[] listFiles = srcFile.listFiles();
for (File listFile : listFiles) {
if (listFile.getName().endsWith(".jpg")) {
System.out.println(listFile.getAbsolutePath());
}
}
}
@Test
public void test3() {
File srcFile = new File("D:\\Software\\Java\\WorkSpace\\io");
File[] subFiles = srcFile.listFiles(new FilenameFilter() {
@Override
public boolean accept(File dir, String name) {
return name.endsWith(".jpg");
}
});
for (File file:subFiles) {
System.out.println(file.getAbsolutePath());
}
}
}
ListFileTest:
package com.pfl.exer2;
import java.io.File;
public class ListFilesTest {
public static void main(String[] args) {
File dir = new File("D:\\Software\\Java\\WorkSpace\\io");
printSubFile(dir);
}
public static void printSubFile(File dir) {
File[] subfiles = dir.listFiles();
for (File f : subfiles) {
if (f.isDirectory()) {
printSubFile(f);
} else {
System.out.println(f.getAbsolutePath());
}
}
}
public void listSubFiles(File file) {
if (file.isDirectory()) {
String[] all = file.list();
for (String s : all) {
System.out.println(s);
}
} else {
System.out.println(file + "是文件!");
}
}
public void listAllSubFiles(File file) {
if (file.isFile()) {
System.out.println(file);
} else {
File[] all = file.listFiles();
for (File f : all) {
listAllSubFiles(f);
}
}
}
public long getDirectorySize(File file) {
long size = 0;
if (file.isFile()) {
size += file.length();
}else {
File[] all = file.listFiles();
for (File f : all) {
size += getDirectorySize(f);
}
}
return size;
}
public void deleteDirectory (File file) {
if (file.isDirectory()) {
File[] all = file.listFiles();
for (File f : all) {
deleteDirectory(f);
}
}
file.delete();
}
}
每日一练:
1.如何遍历Map 的key集,value集, key-value集,使用上泛型。
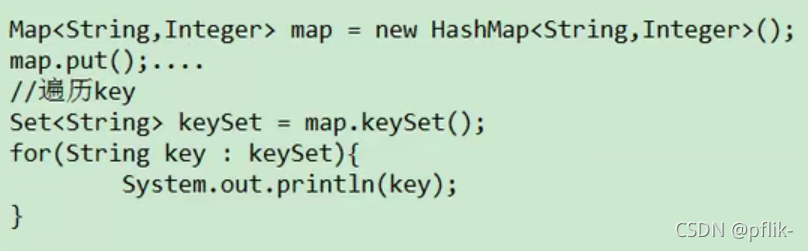 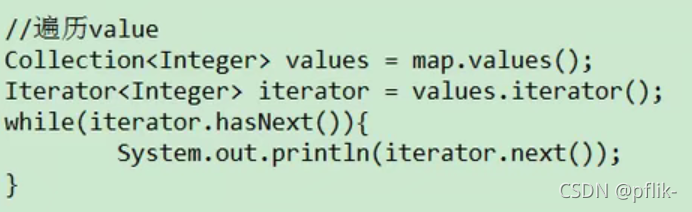 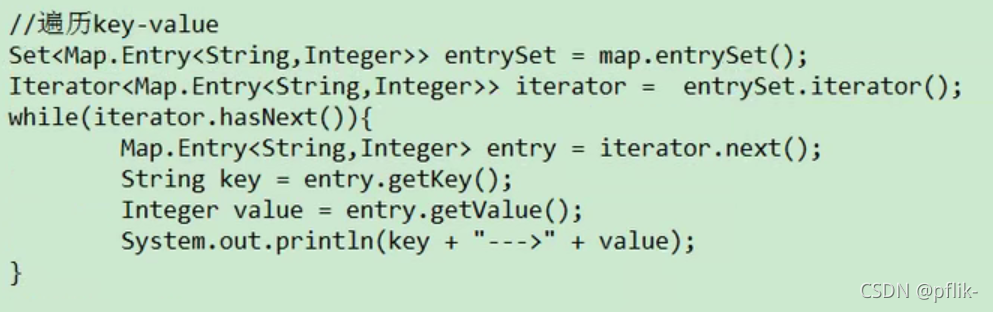
2.写出使用Iterator和增强for循环遍历List的代码,使用上泛型。
3.提供一个方法,用于遍历获取HashMap<String,String>中的所有value,并存放在List中返回。考虑上集合中泛型的使用。
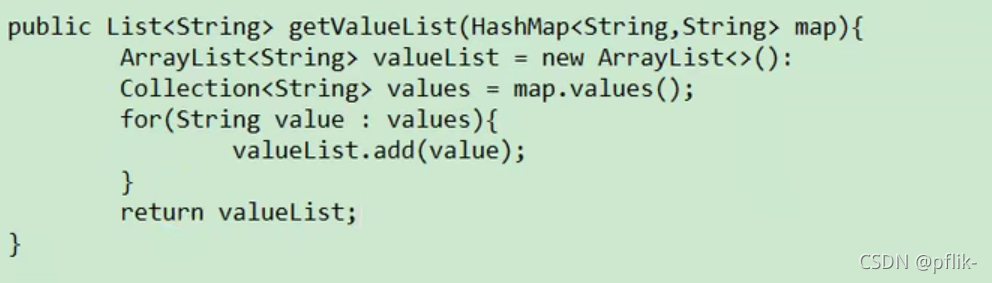
4.创建一个与a.txt文件同目录下的另外一个文件b.txt。

5.Map接口中的常用方法有哪些。
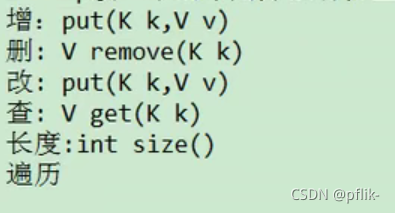
13.2、IO流原理及流的分类
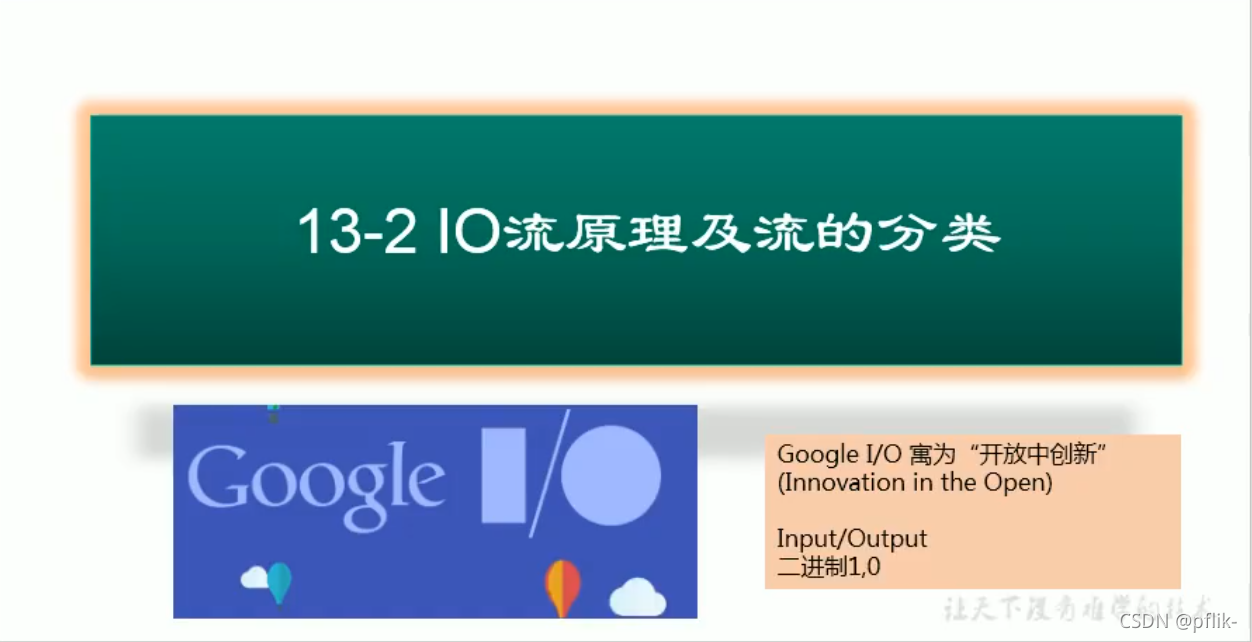 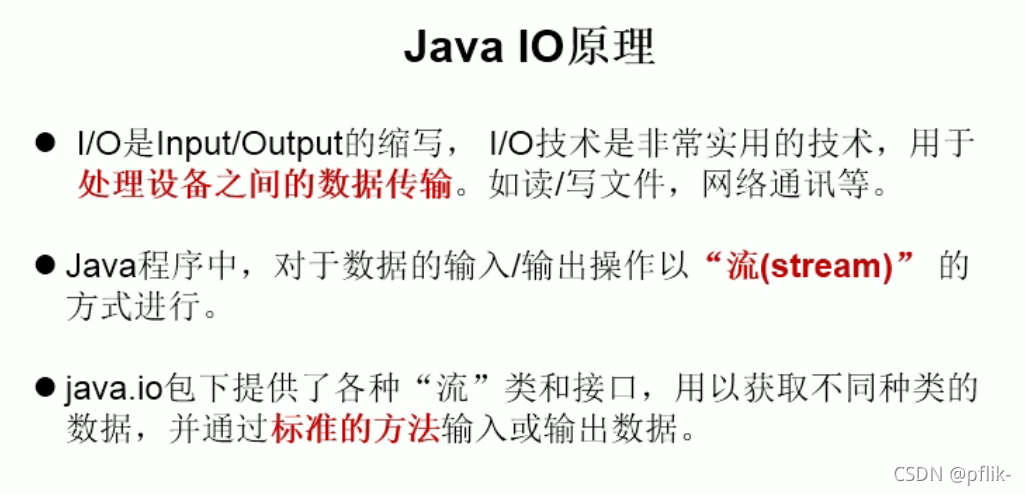 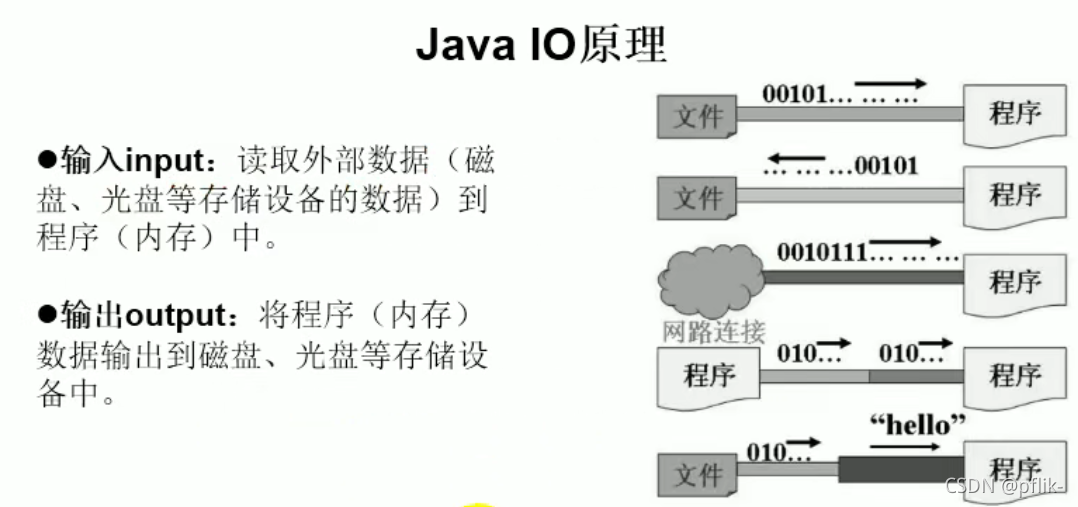 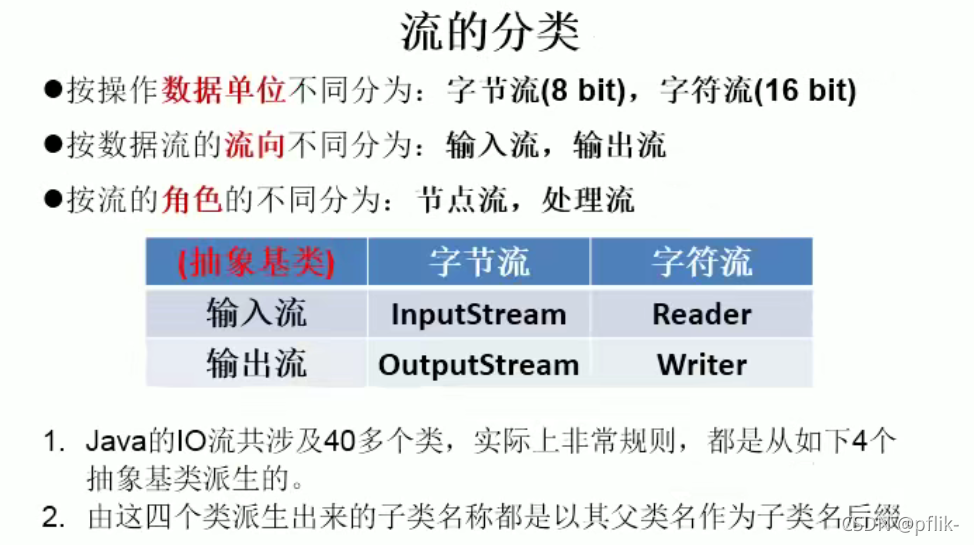 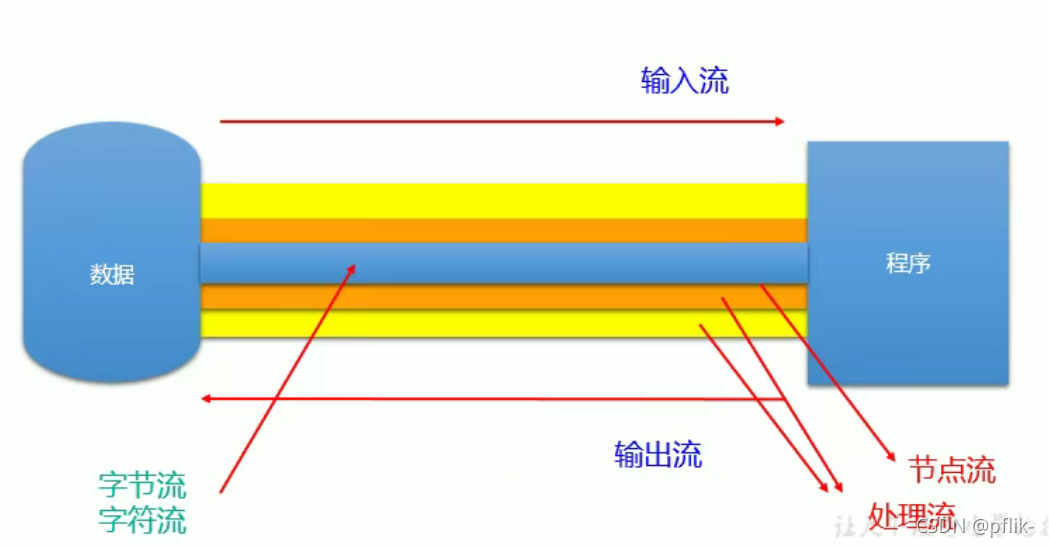 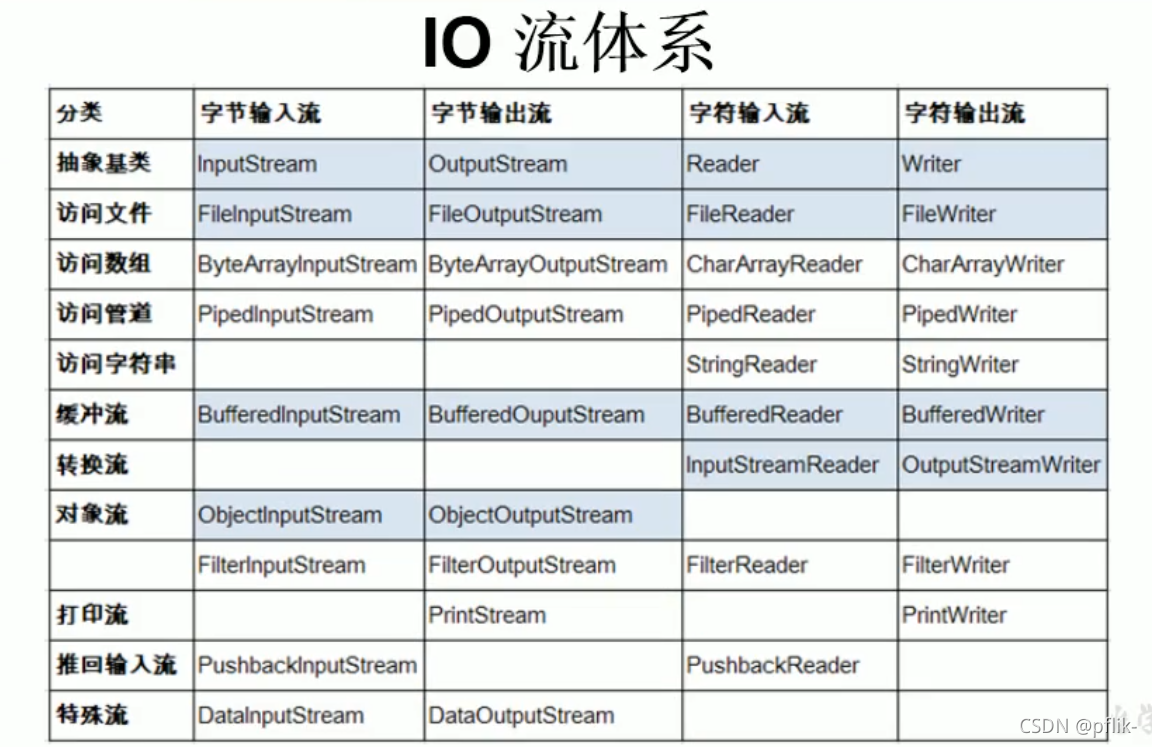 注:垃圾回收机制的关键点: 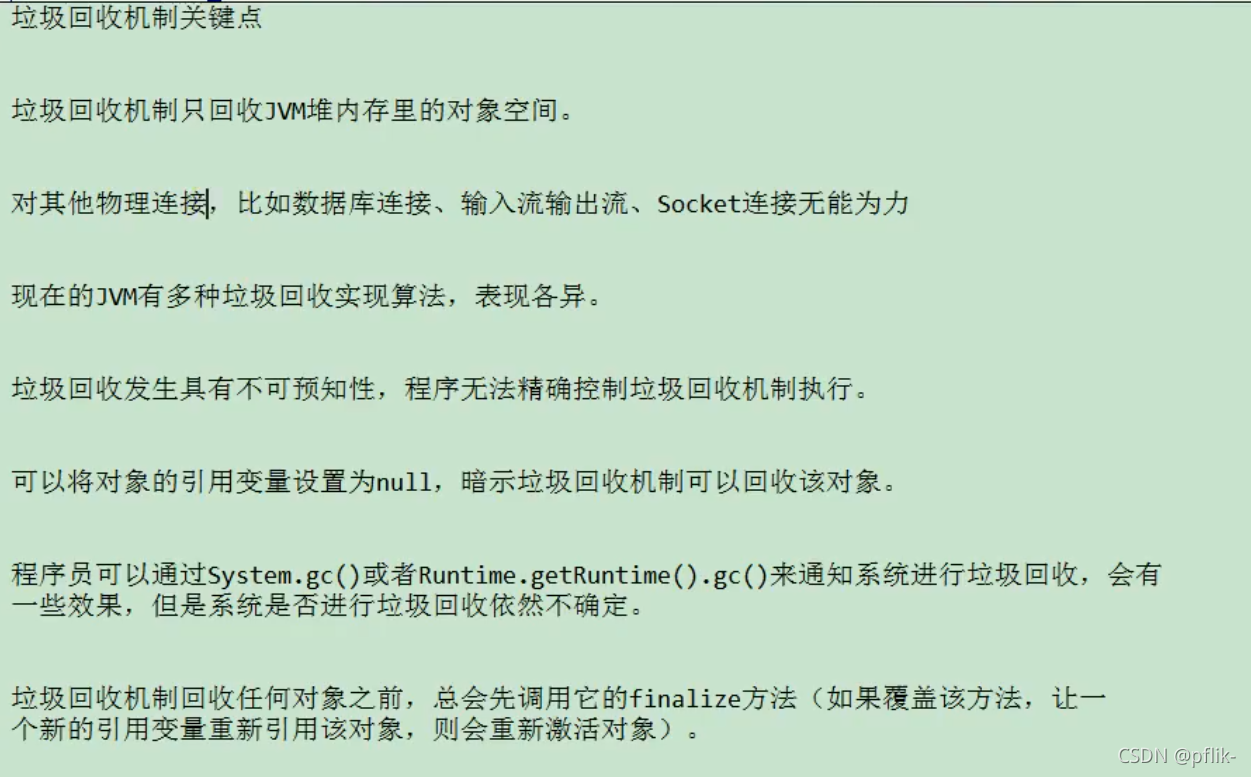 FileReaderWriterTest:
package com.pfl.java;
import org.junit.Test;
import java.io.*;
public class FileReaderWriterTest {
public static void main(String[] args) {
File file = new File("hello.txt");
System.out.println(file.getAbsolutePath());
File file1 = new File("day09\\hello.txt");
System.out.println(file1.getAbsolutePath());
}
@Test
public void testFileReader(){
FileReader fileReader = null;
try {
File file = new File("hello1.txt");
fileReader = new FileReader(file);
int data;
while((data = fileReader.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fileReader != null) {
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testFileReader1(){
FileReader fr = null;
try {
File file = new File("hello.txt");
fr = new FileReader(file);
char[] cbuf = new char[5];
int len;
while ((len = fr.read(cbuf)) != -1) {
String str = new String(cbuf, 0, len);
System.out.print(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fr != null) {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testFileWriter(){
FileWriter fw = null;
try {
File file = new File("hello1.txt");
fw = new FileWriter(file,false);
fw.write("I have a dream!\n");
fw.write("you need to have a dream\n");
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fw != null) {
try {
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testFileReaderFileWriter(){
FileReader fr = null;
FileWriter fw = null;
try {
File srcFile = new File("hello.txt");
File destFile = new File("hello2.txt");
fr = new FileReader(srcFile);
fw = new FileWriter(destFile);
char[] cbuf = new char[5];
int len;
while ((len = fr.read(cbuf)) != -1) {
fw.write(cbuf,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fr != null)
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
if(fw != null)
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
FileInputOutputStreamTest:
package com.pfl.java;
import org.junit.Test;
import java.io.*;
public class FileInputOutputStreamTest {
@Test
public void testFileInputStream(){
FileInputStream fis = null;
try {
File file = new File("hello.txt");
fis = new FileInputStream(file);
byte[] buffer = new byte[5];
int len;
while((len = fis.read(buffer)) != -1) {
String str = new String(buffer, 0, len);
System.out.print(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testFileInputOutputStream() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File srcfile = new File("浅绿色壁纸.jpg");
File destfile = new File("浅绿色壁纸2.jpg");
fis = new FileInputStream(srcfile);
fos = new FileOutputStream(destfile);
byte[] buffer = new byte[5];
int len;
while((len = fis.read(buffer)) != -1) {
fos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public void copyFile(String srcPath, String destPath) {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File srcfile = new File(srcPath);
File destfile = new File(destPath);
fis = new FileInputStream(srcfile);
fos = new FileOutputStream(destfile);
byte[] buffer = new byte[1024];
int len;
while((len = fis.read(buffer)) != -1) {
fos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testCopyFile() {
long start = System.currentTimeMillis();
String srcPath = "F:\\图片\\桌面图片\\小姐姐.mp4";
String destPath = "F:\\图片\\桌面图片\\小姐姐2.mp4";
copyFile(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println("复制操作花费的时间为:" + (end - start));
}
}
BufferTest:
package com.pfl.java;
import org.junit.Test;
import java.io.*;
public class BufferTest {
@Test
public void BufferedStreamTest() {
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
File srcFile = new File("浅绿色壁纸.jpg");
File destFile = new File("浅绿色壁纸2.jpg");
FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile);
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[10];
int len;
while((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0 ,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bis != null) {
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bos != null) {
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public void copyFileWithBuffered(String srcPath, String destPath) {
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
File srcFile = new File(srcPath);
File destFile = new File(destPath);
FileInputStream fis = new FileInputStream(srcFile);
FileOutputStream fos = new FileOutputStream(destFile);
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[10];
int len;
while((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0 ,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bis != null) {
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bos != null) {
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testCopyFileWithBuffered() {
long start = System.currentTimeMillis();
String srcPath = "D:\\life\\photo\\桌面图片\\小姐姐.mp4";
String destPath = "D:\\life\\photo\\桌面图片\\小姐姐2.mp4";
copyFileWithBuffered(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println("复制操作花费的时间为:" + (end - start));
}
@Test
public void testBufferedReaderBufferedWriter() {
BufferedReader br = null;
BufferedWriter bw = null;
try {
br = new BufferedReader(new FileReader(new File("hello.txt")));
bw = new BufferedWriter(new FileWriter(new File("hello1.txt")));
String data;
while((data = br.readLine()) != null) {
bw.write(data);
bw.newLine();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bw != null) {
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
练习:
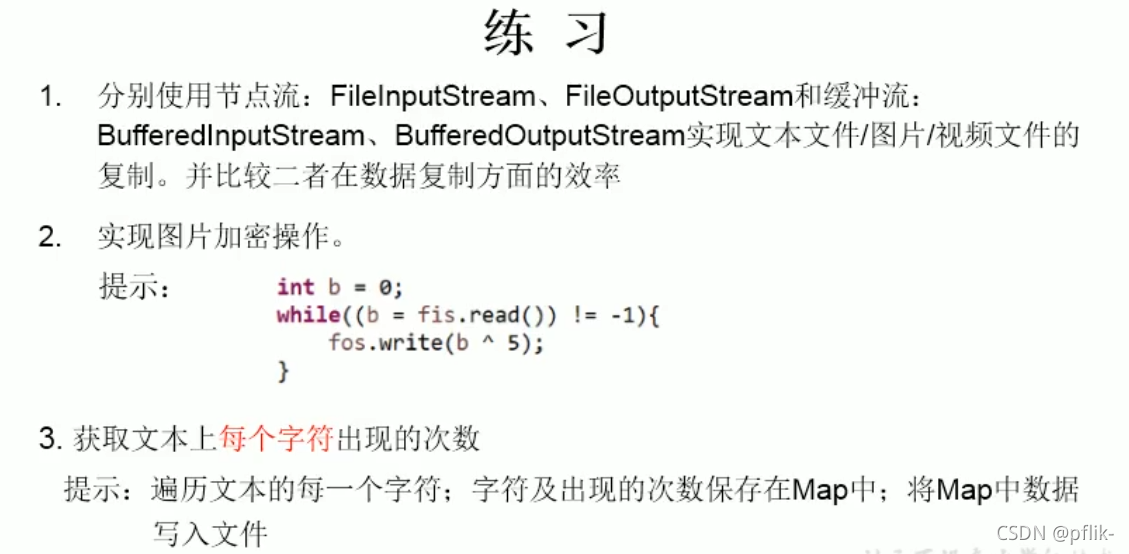 T2:
package com.pfl.exer;
import org.junit.Test;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
/**
* @author pflik-
* @create 2021-10-06 19:39
*/
public class PicTest {
//图片的加密---使用字节流---这是一个可逆的过程,还是可以继续解密的
@Test
public void test1() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
// FileInputStream fis = new FileInputStream(new File("IU.jpg"));
//其实是把字符串包装成了文件
fis = new FileInputStream(new File("IU.jpg"));
fos = new FileOutputStream("IU-secret.jpg");
byte[] buffer = new byte[20];
int len;
while((len = fis.read(buffer)) != -1) {
//对字节数据进行修改
//注意:增强for循环改的是一个临时的变量,buffer数组并没有改--一个错误的演示
// for(byte b : buffer) {
// b = (byte) (b ^ 5);
// }
//正确的--对字节数上的每个位置上的字节做了异或运算
for(int i = 0;i < len;i++) {
buffer[i] = (byte) (buffer[i] ^ 5);
}
fos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
//图片的解密---使用字节流
@Test
public void test2() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
// FileInputStream fis = new FileInputStream(new File("IU.jpg"));
//其实是把字符串包装成了文件
fis = new FileInputStream(new File("IU-secret.jpg"));
fos = new FileOutputStream("IU2.jpg");
byte[] buffer = new byte[20];
int len;
while((len = fis.read(buffer)) != -1) {
//对字节数据进行修改
//注意:增强for循环改的是一个临时的变量,buffer数组并没有改--一个错误的演示
// for(byte b : buffer) {
// b = (byte) (b ^ 5);
// }
//正确的--对字节数上的每个位置上的字节做了异或运算
for(int i = 0;i < len;i++) {
buffer[i] = (byte) (buffer[i] ^ 5);
}
fos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
T3:
package com.pfl.exer;
import org.junit.Test;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
/**
*
* 练习3:获取文本上字符出现的次数,把数据写入文件
*
* 思路:
* 1.遍历文本每一个字符
* 2.字符出现的次数存在Map中
* Map<Character, Integer> map = new HashMap<Character, Integer>();
* map.put('a', 18);
* map.put('你', 2);
* 3.把map中的数据写入文件
*
*
* @author pflik-
* @create 2021-10-06 20:07
*/
public class WordCount {
/*
说明:如果使用单元测试,文件相对路径为当前module
如果使用main()测试,文件相对路径为当前工程
*/
@Test
public void testWordCount() {
FileReader fr = null;
BufferedWriter bw = null;
try {
//1.创建map集合
Map<Character, Integer> map = new HashMap<>();
//2.遍历每一个字符,每一个字符出现的次数放到map中
fr = new FileReader("hello.txt");
int c = 0;
while((c = fr.read()) != -1) {
//int 还原 char
char ch = (char)c;
//判断char是否在map中第一次出现
if(map.get(ch) == null) {
map.put(ch, 1);
} else {
map.put(ch, map.get(ch) + 1);
}
}
//3.把map中数据存在文件count.txt
//3.1 创建Writer
bw = new BufferedWriter(new FileWriter("wordcount.txt"));
//3.2 遍历map,再写入数据
Set<Map.Entry<Character, Integer>> entrySet = map.entrySet();
for(Map.Entry<Character, Integer> entry : entrySet) {
switch (entry.getKey()) {
case ' ':
bw.write("空格=" + entry.getValue());
break;
case '\t': //\t表示tab键字符
bw.write("tab键=" + entry.getValue());
break;
case '\r': //
bw.write("回车=" + entry.getValue());
break;
case '\n': //
bw.write("换行=" + entry.getValue());
break;
default:
bw.write(entry.getKey() + "=" + entry.getValue());
break;
}
bw.newLine(); //换行
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fr != null) {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bw != null) {
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
13.5、转换流–处理流的一种
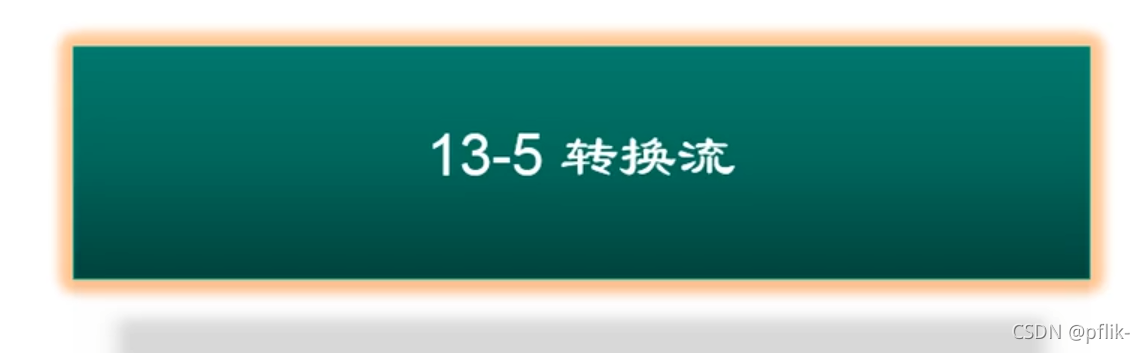 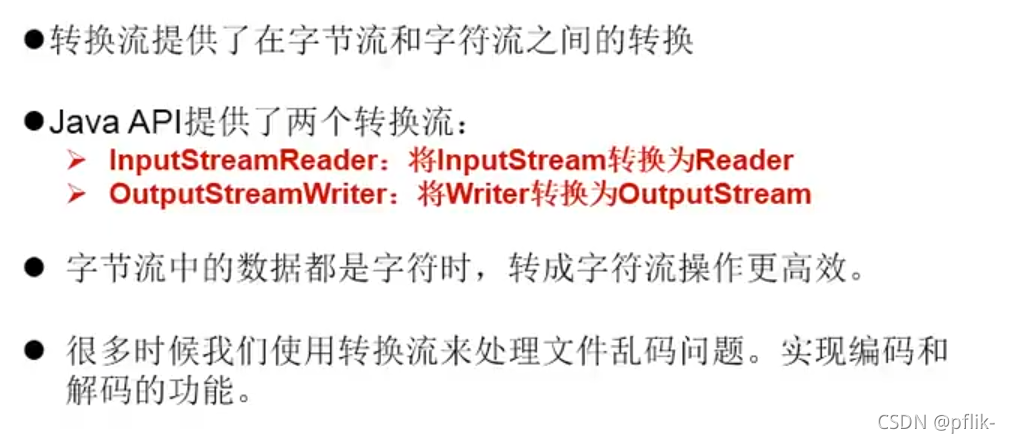 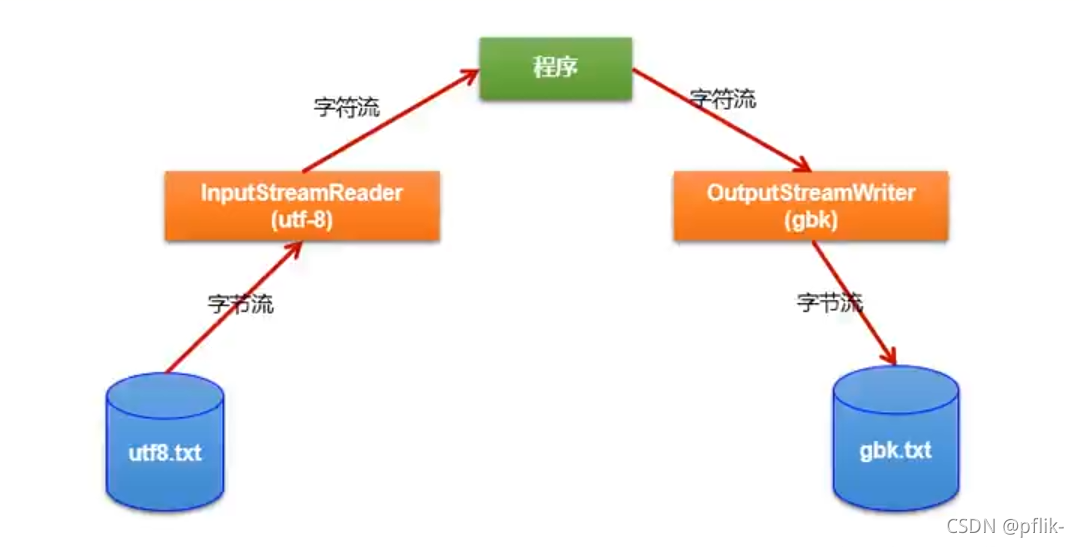 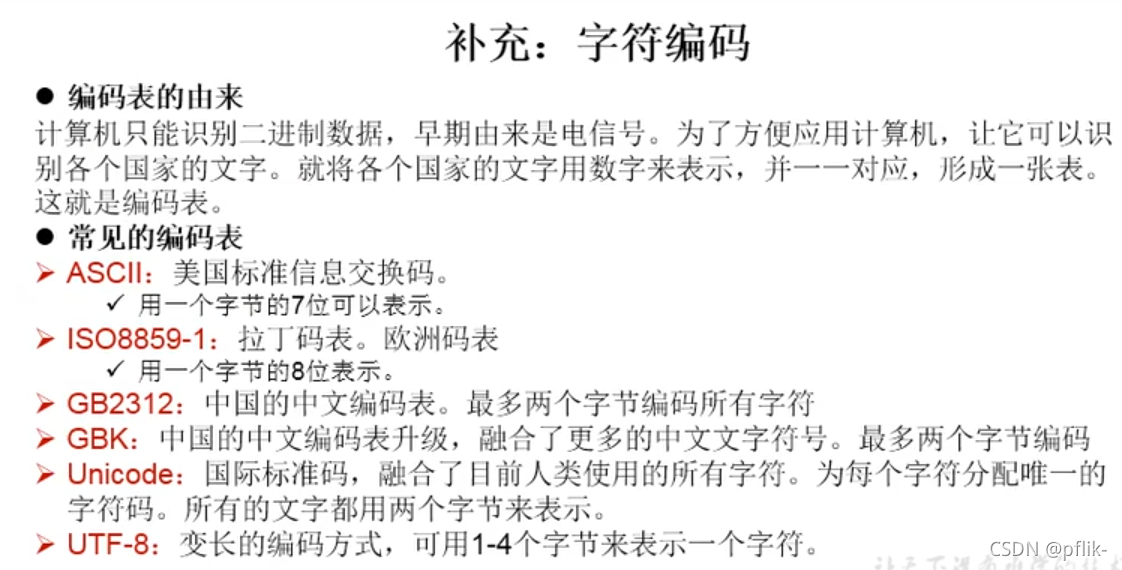 InputStreamReader:
package com.pfl.java;
import org.junit.Test;
import java.io.*;
public class InputStreamReaderTest {
@Test
public void test1() {
InputStreamReader isr = null;
try {
FileInputStream fis = new FileInputStream("hello.txt");
isr = new InputStreamReader(fis);
char[] cbuf = new char[20];
int len;
while((len = isr.read(cbuf)) != -1) {
String str = new String(cbuf,0,len);
System.out.println(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(isr != null) {
try {
isr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void test2() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File file1 = new File("hello.txt");
File file2 = new File("hello_gbk.txt");
fis = new FileInputStream(file1);
fos = new FileOutputStream(file2);
InputStreamReader isr = new InputStreamReader(fis, "utf-8");
OutputStreamWriter osw = new OutputStreamWriter(fos, "gbk");
char[] cbuf = new char[20];
int len;
while ((len = isr.read(cbuf)) != -1) {
osw.write(cbuf, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
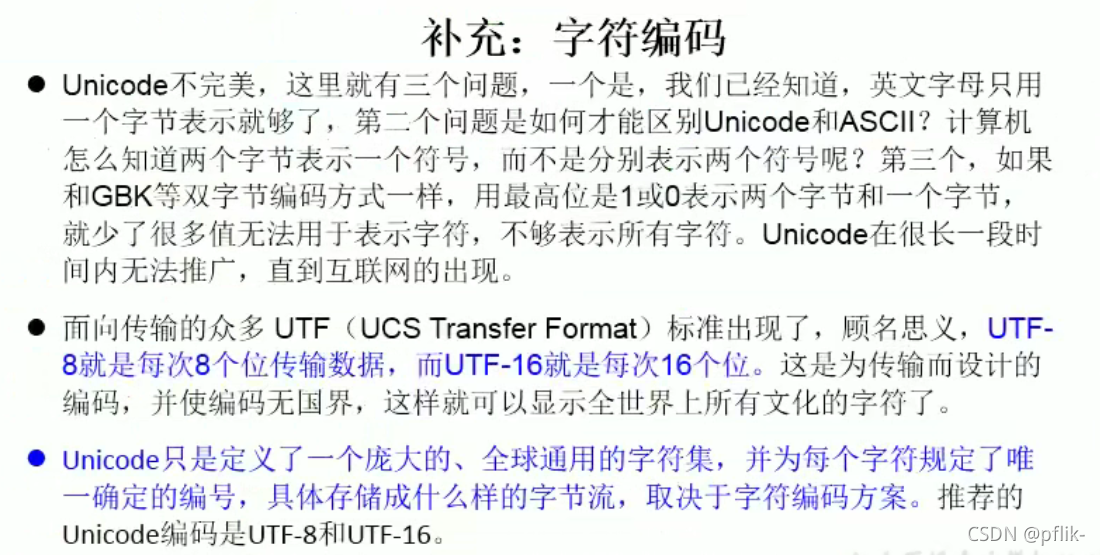 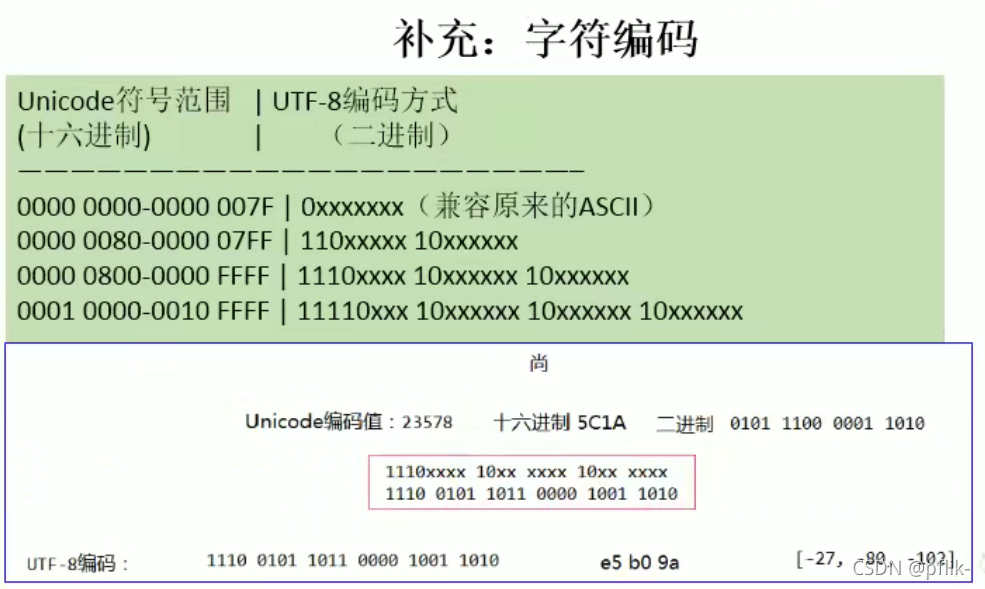 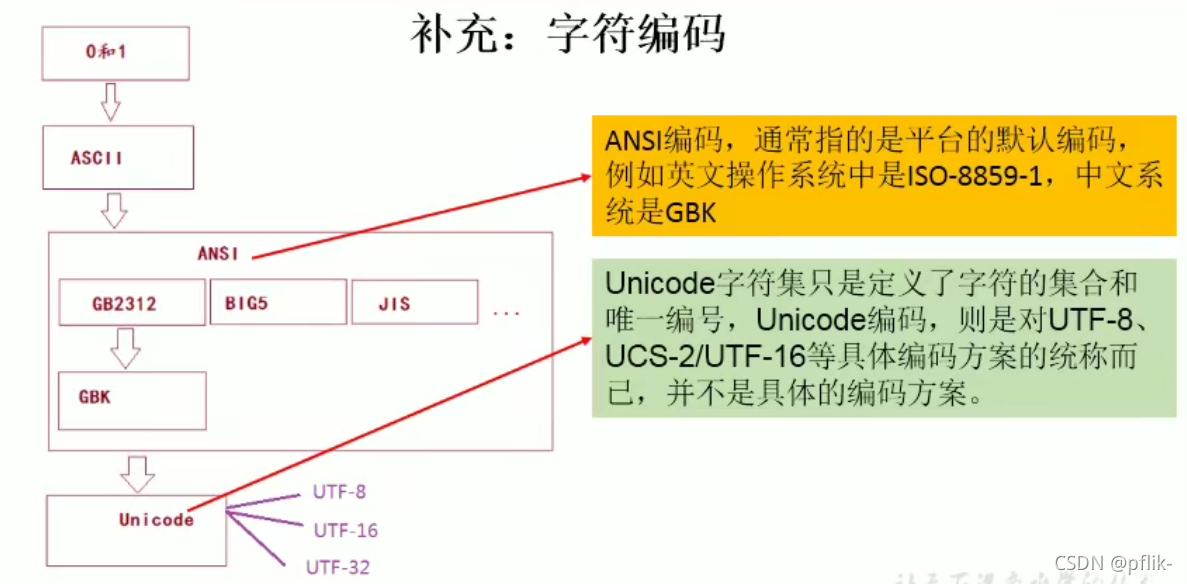
13.6、标准输入、输出流
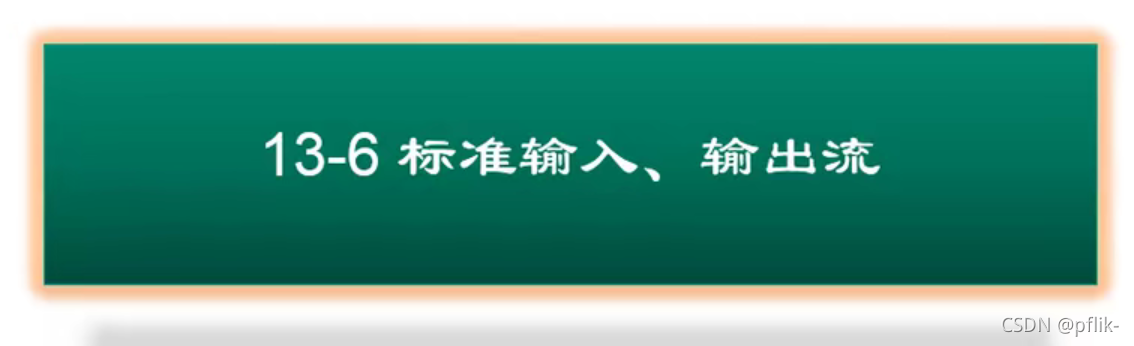 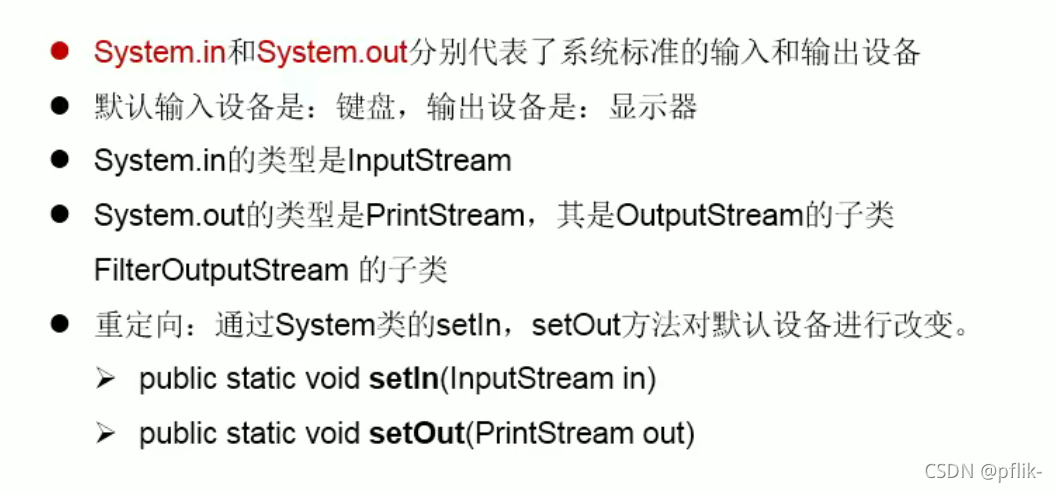 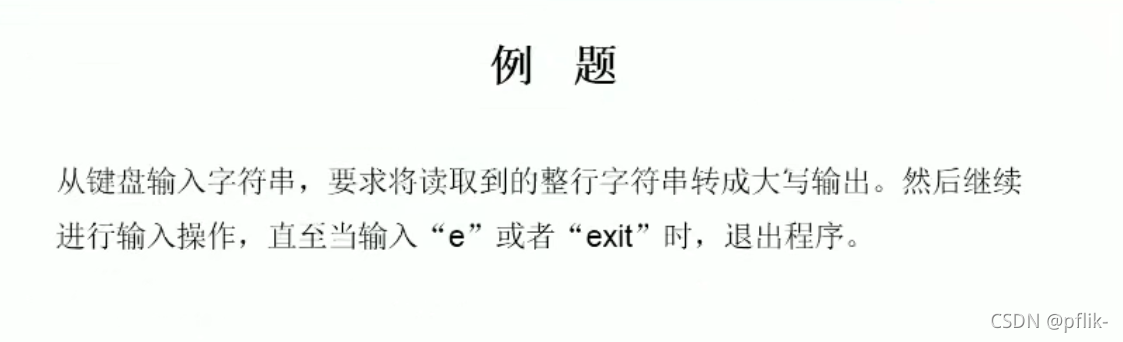 OtherStreamTest:
package com.pfl.java;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class OtherStreamTest {
public static void main(String[] args) {
BufferedReader br = null;
try {
InputStreamReader isr = new InputStreamReader(System.in);
br = new BufferedReader(isr);
while(true) {
System.out.println("请输入字符串:");
String data = br.readLine();
if("e".equalsIgnoreCase(data) || "exit".equalsIgnoreCase(data)) {
System.out.println("程序结束!");
break;
}
String upperCase = data.toUpperCase();
System.out.println(upperCase);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
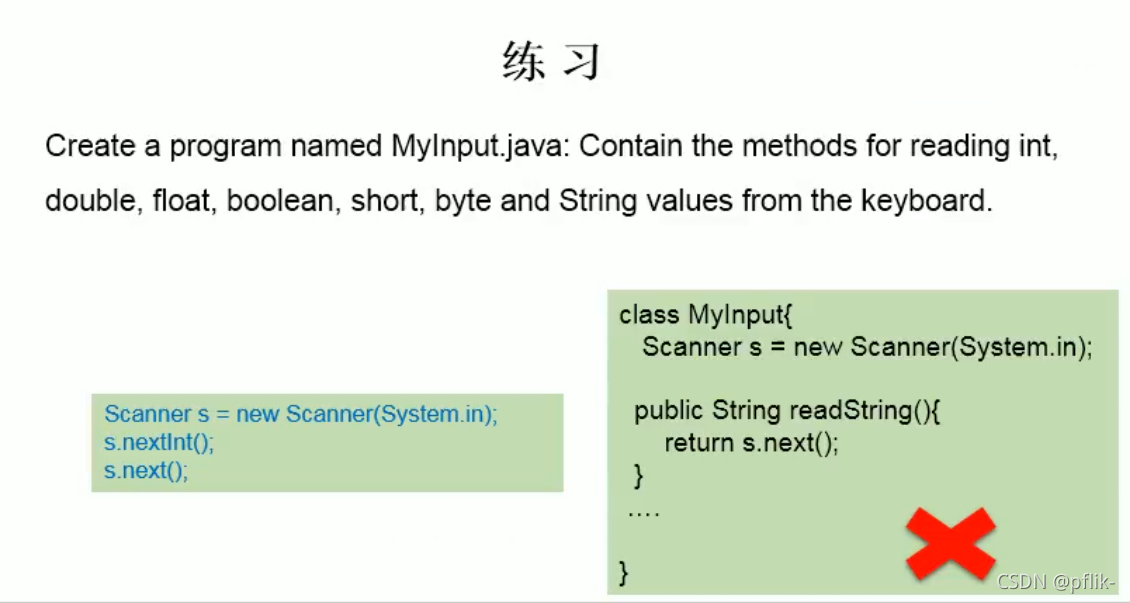 MyInput:
package com.pfl.exer;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class MyInput {
public static String readString() {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String string = "";
try {
string = br.readLine();
} catch (IOException e) {
System.out.println(e);
}
return string;
}
public static int readInt() { return Integer.parseInt(readString()); }
public static double readDouble() { return Double.parseDouble(readString()); }
public static double readByte() { return Byte.parseByte(readString()); }
public static double readShort() { return Short.parseShort(readString()); }
public static double readLong() { return Long.parseLong(readString()); }
public static double readFloat() { return Float.parseFloat(readString()); }
}
13.7、打印流
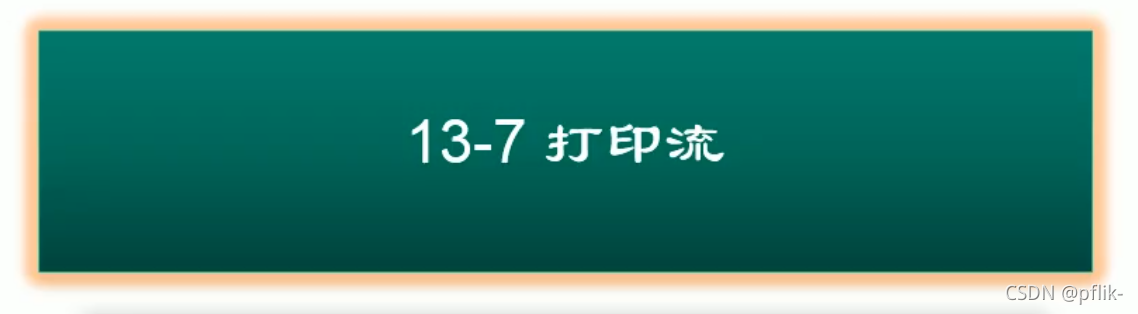 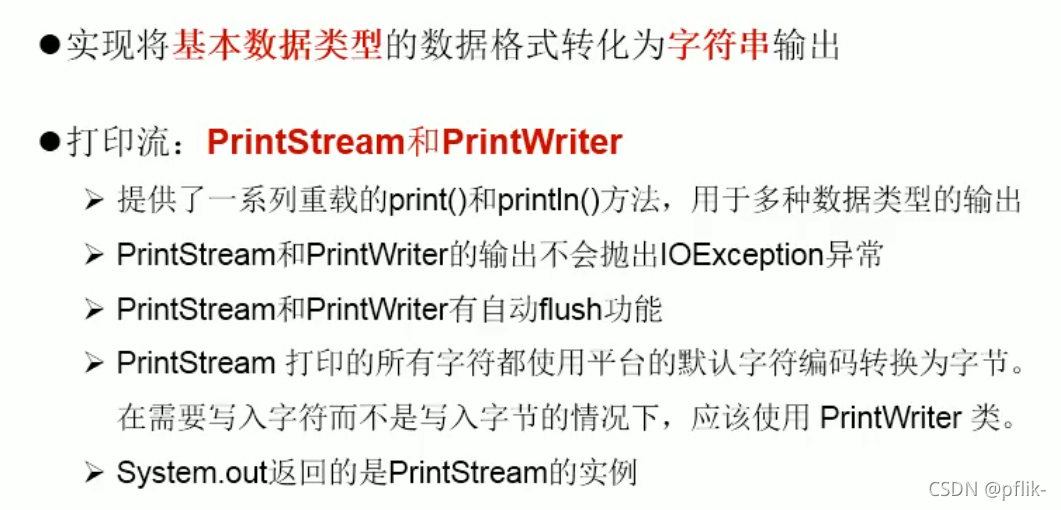 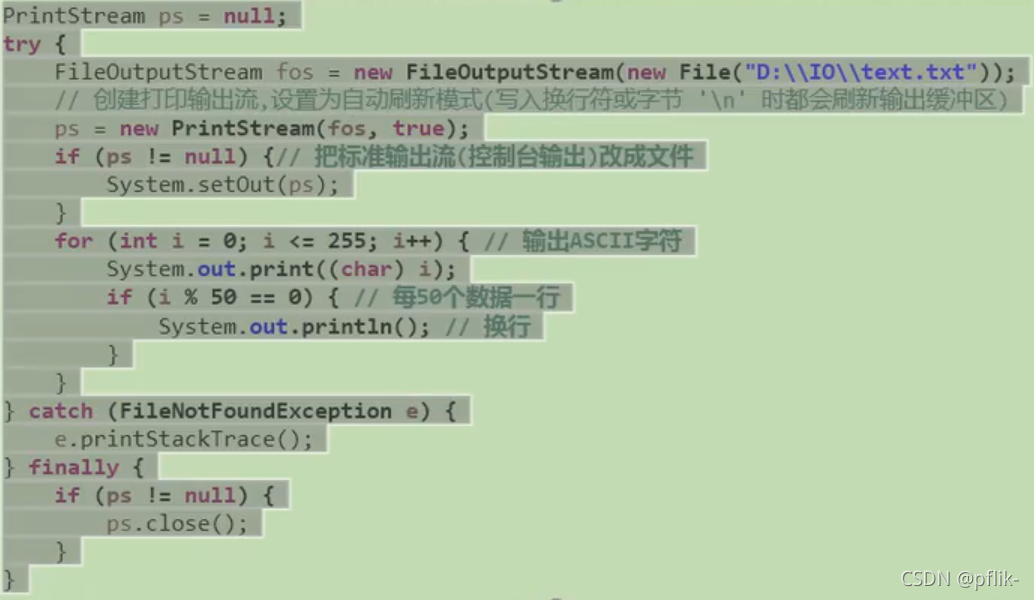
13.8、数据流
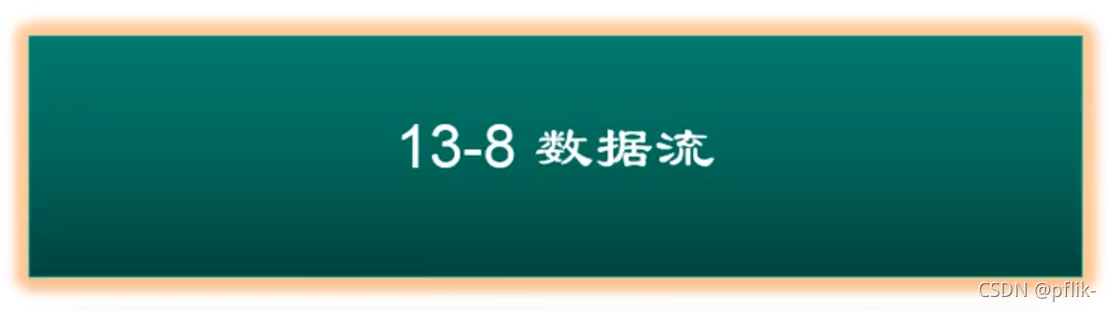 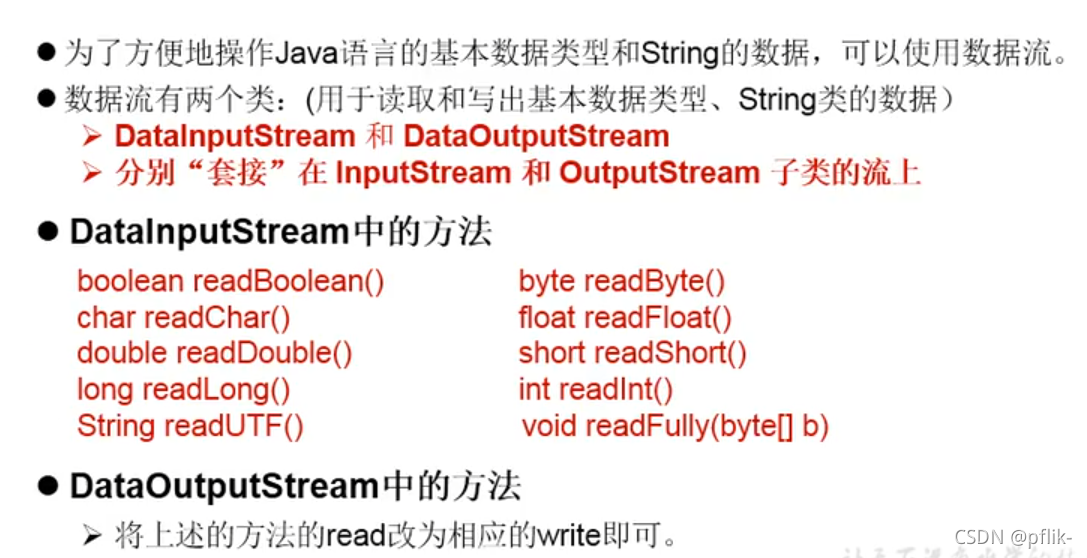
package com.pfl.java;
import org.junit.Test;
import java.io.*;
public class OtherStreamTest {
public static void main(String[] args) {
BufferedReader br = null;
try {
InputStreamReader isr = new InputStreamReader(System.in);
br = new BufferedReader(isr);
while(true) {
System.out.println("请输入字符串:");
String data = br.readLine();
if("e".equalsIgnoreCase(data) || "exit".equalsIgnoreCase(data)) {
System.out.println("程序结束!");
break;
}
String upperCase = data.toUpperCase();
System.out.println(upperCase);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void test3() {
DataOutputStream dos = null;
try {
dos = new DataOutputStream(new FileOutputStream("data.txt"));
dos.writeUTF("刘建辰");
dos.flush();
dos.writeInt(23);
dos.flush();
dos.writeBoolean(true);
dos.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(dos != null) {
try {
dos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void test4() {
DataInputStream dis = null;
try {
dis = new DataInputStream(new FileInputStream("data.txt"));
String name = dis.readUTF();
int age = dis.readInt();
boolean isMale = dis.readBoolean();
System.out.println("name = " + name);
System.out.println("age = " + age);
System.out.println("isMale = " + isMale);
} catch (IOException e) {
e.printStackTrace();
} finally {
if(dis != null) {
try {
dis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
每日一练:
1.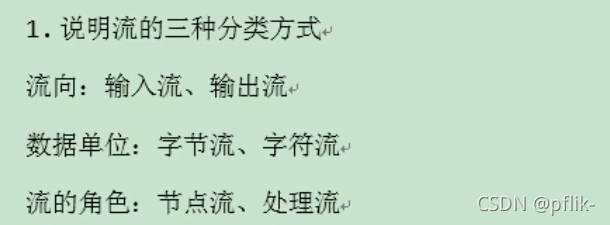 2. 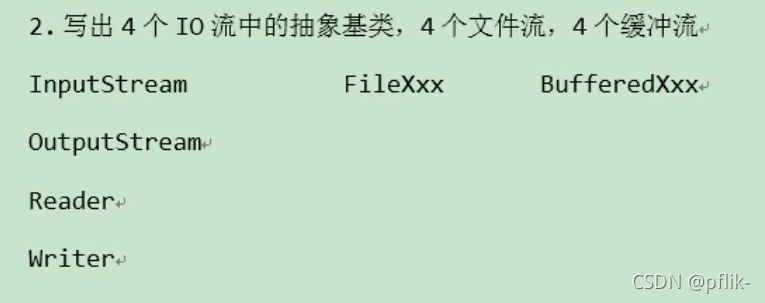 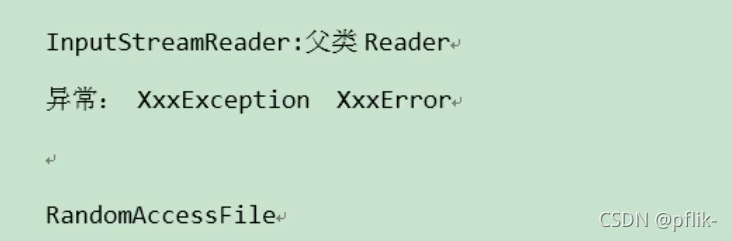 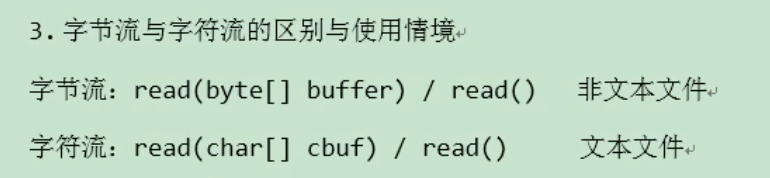 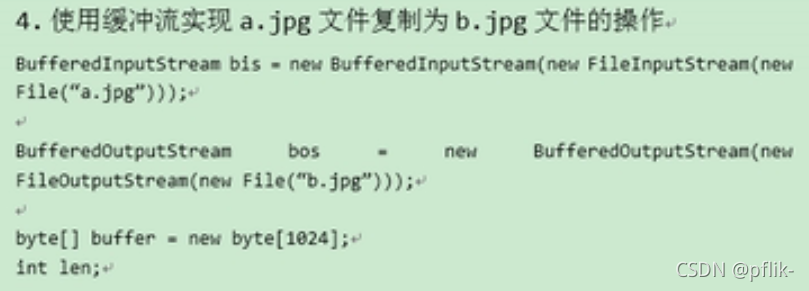 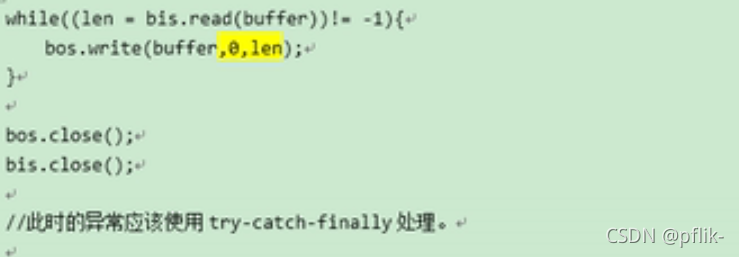 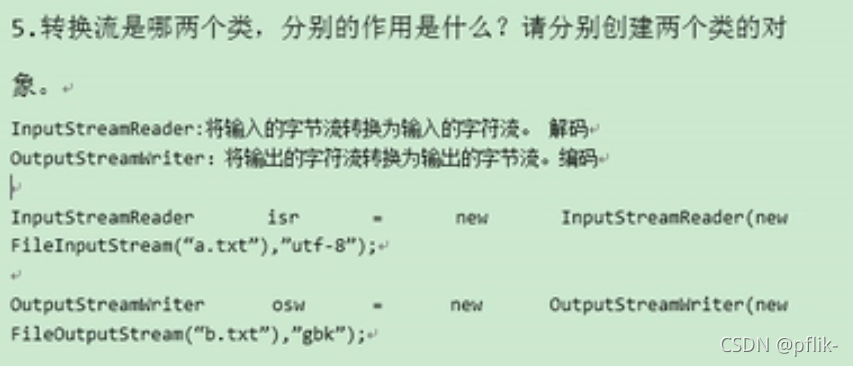
13.9、对象流
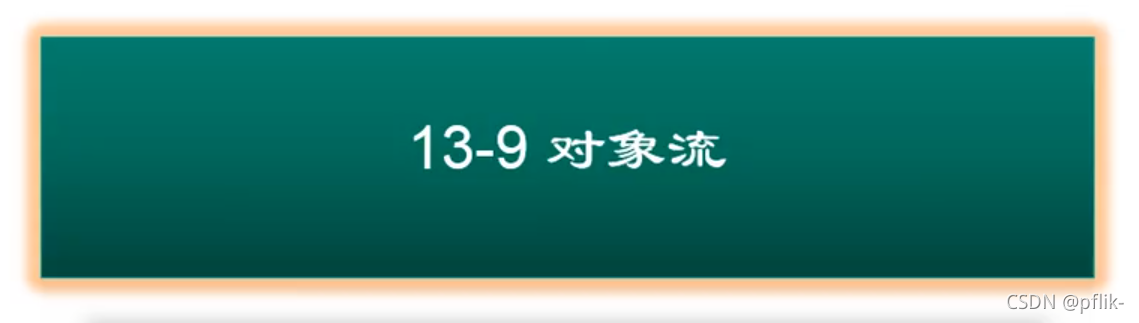 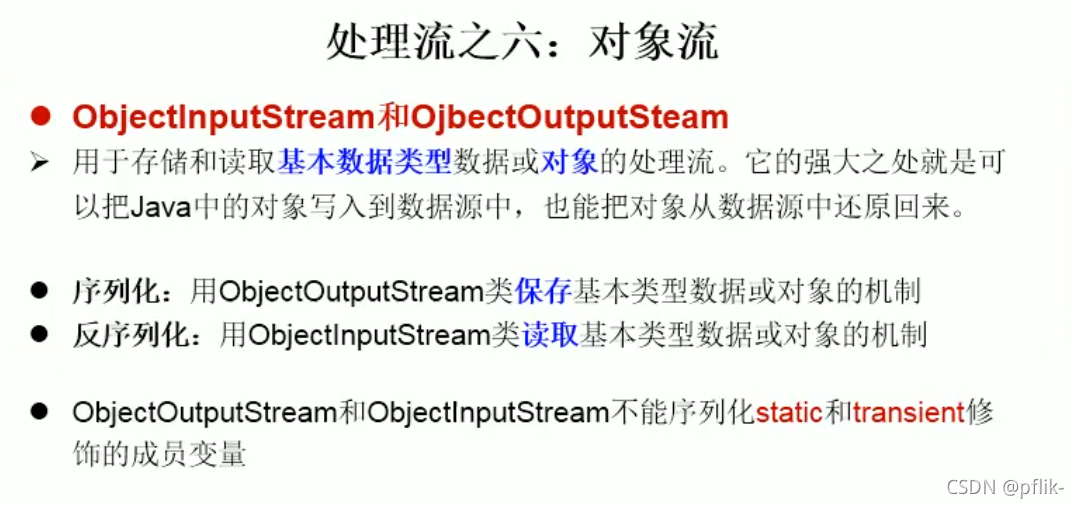 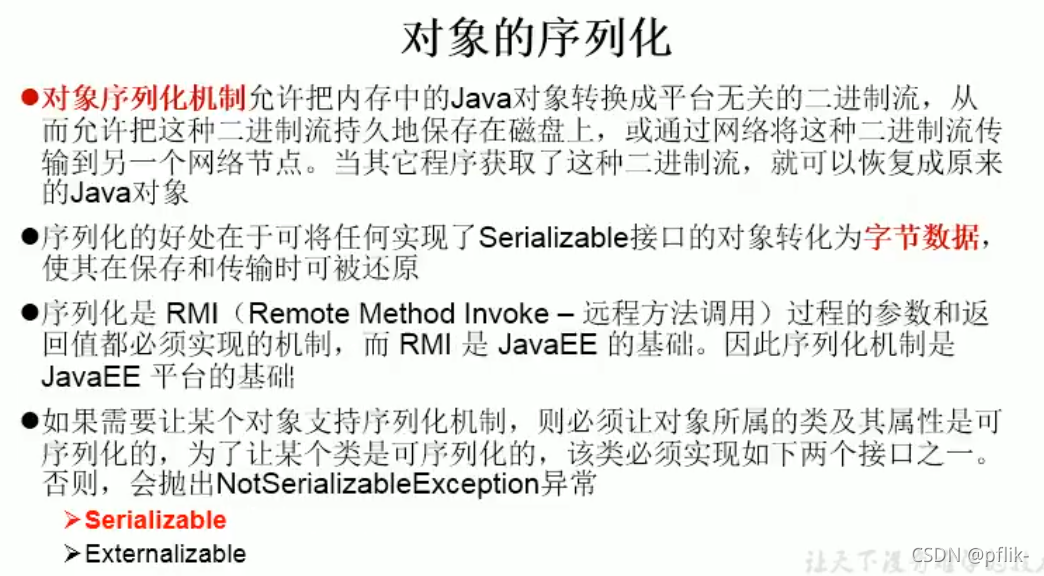 ObjectInputOutputStreamTest:
package com.pfl.java;
import org.junit.Test;
import java.io.*;
public class ObjectInputOutputStreamTest {
@Test
public void testObjectOutputStream() {
ObjectOutputStream oos = null;
try {
oos = new ObjectOutputStream(new FileOutputStream("object.dat"));
oos.writeObject(new String("我爱北京天安门"));
oos.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(oos != null) {
try {
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testObjectInputStream() {
ObjectInputStream ois = null;
try {
ois = new ObjectInputStream(new FileInputStream("object.dat"));
Object obj = ois.readObject();
String str = (String) obj;
System.out.println(str);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
if(ois != null) {
try {
ois.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
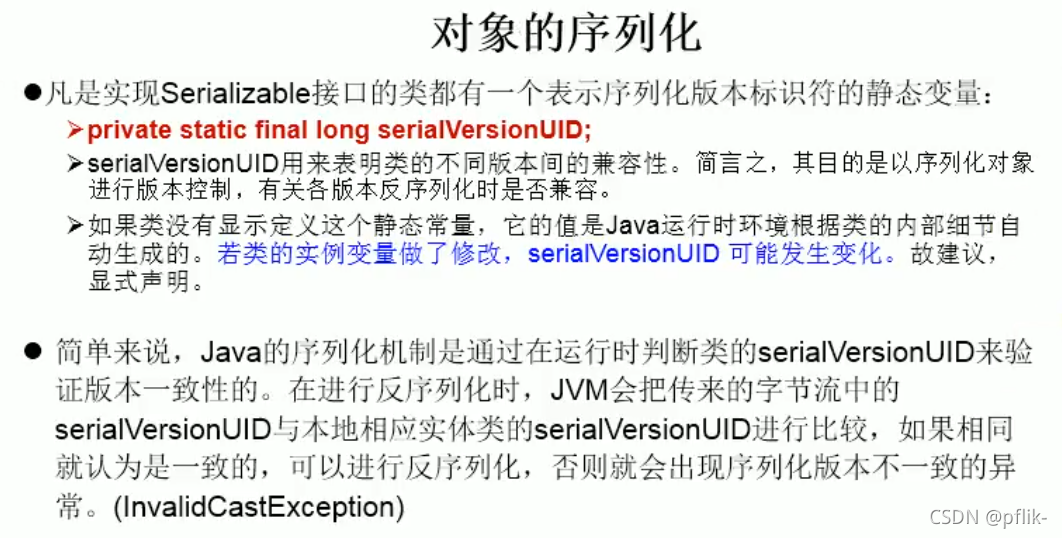 Person:
package com.pfl.java;
import java.io.Serializable;
public class Person implements Serializable {
public static final long serialVersionUID = 43242313465L;
private String name;
private int age;
private int id;
private Account acct;
public Person(String name, int age, int id, Account acct) {
this.name = name;
this.age = age;
this.id = id;
this.acct = acct;
}
public Person() {
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public Person(String name, int age, int id) {
this.name = name;
this.age = age;
this.id = id;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", id=" + id +
", acct=" + acct +
'}';
}
}
class Account implements Serializable{
public static final long serialVersionUID = 43242313465L;
private double balance;
public Account(double balance) {
this.balance = balance;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
@Override
public String toString() {
return "Account{" +
"balance=" + balance +
'}';
}
}
ObjectInputOutputStreamTest:
package com.pfl.java;
import org.junit.Test;
import java.io.*;
public class ObjectInputOutputStreamTest {
@Test
public void testObjectOutputStream() {
ObjectOutputStream oos = null;
try {
oos = new ObjectOutputStream(new FileOutputStream("object.dat"));
oos.writeObject(new String("我爱北京天安门"));
oos.flush();
oos.writeObject(new Person("王明", 23));
oos.flush();
oos.writeObject(new Person("张学良", 23, 1001, new Account(5000)));
oos.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(oos != null) {
try {
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testObjectInputStream() {
ObjectInputStream ois = null;
try {
ois = new ObjectInputStream(new FileInputStream("object.dat"));
Object obj = ois.readObject();
String str = (String) obj;
Person p = (Person) ois.readObject();
Person p1 = (Person) ois.readObject();
System.out.println(str);
System.out.println(p);
System.out.println(p1);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
if(ois != null) {
try {
ois.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
13.10、随机存取文件流
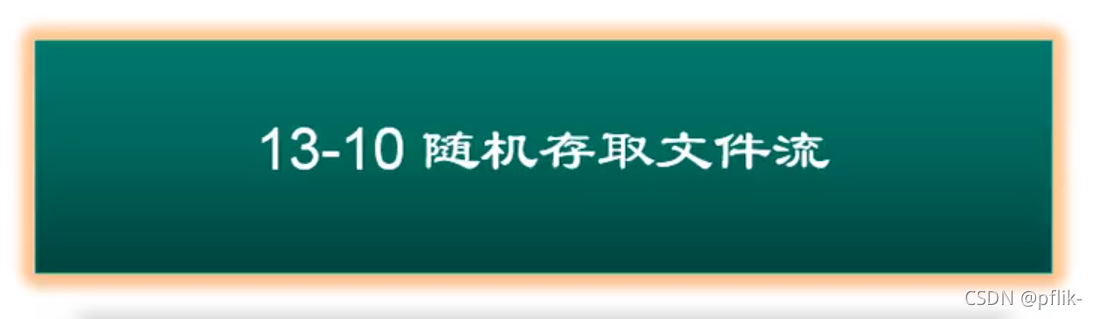 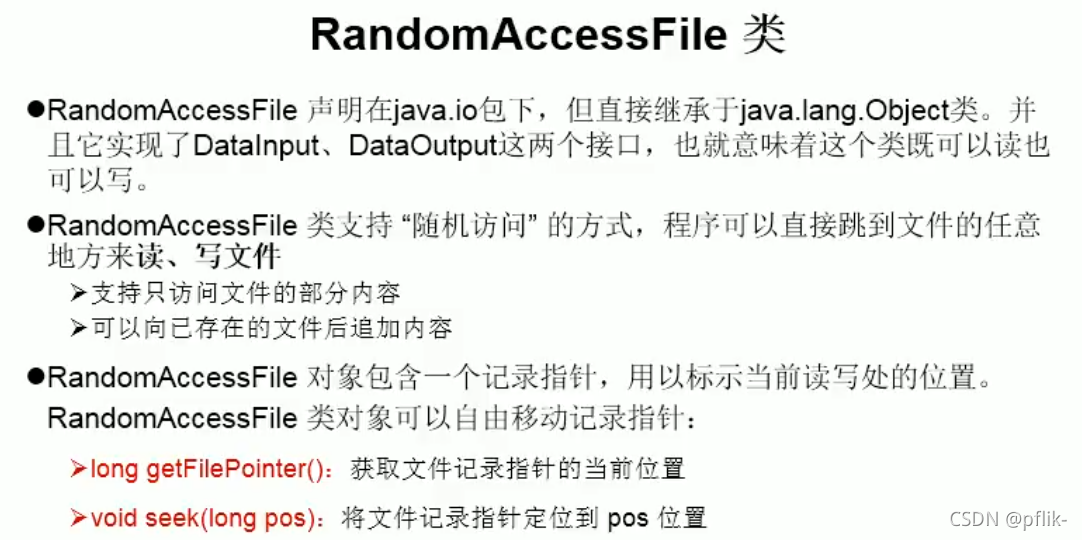 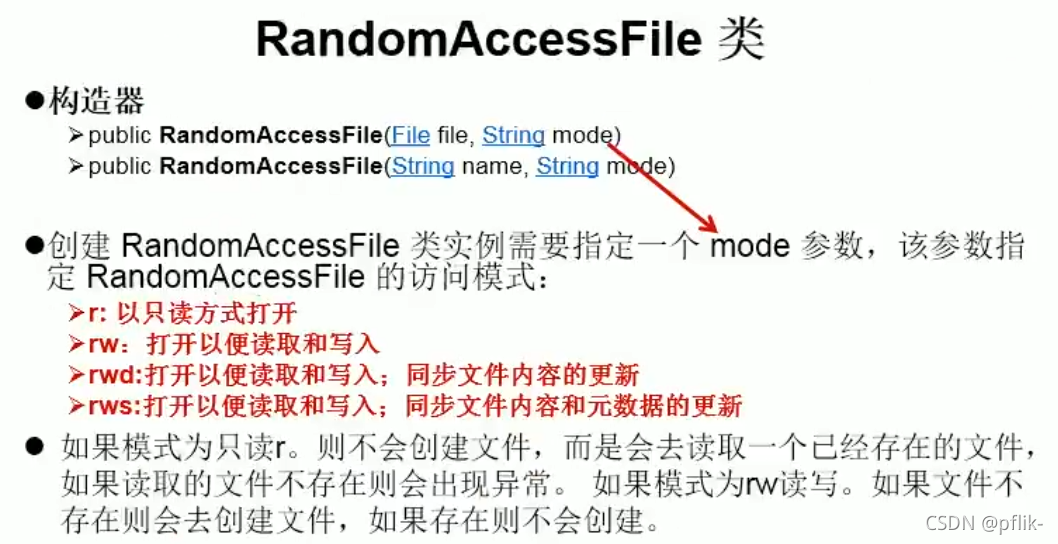 视频617:6.30–
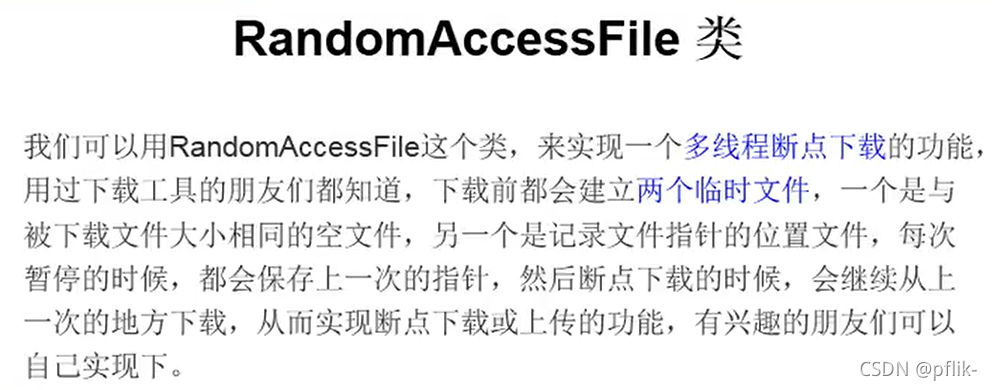 ByteArrayOutputStreamTest.java
package com.pfl.java;
import org.junit.Test;
import java.io.*;
import java.nio.Buffer;
public class ByteArrayOutputStreamTest {
@Test
public void test1() throws IOException {
FileInputStream fis = new FileInputStream("abc.txt");
String info = readStringFromInputStream(fis);
System.out.println(info);
}
private String readStringFromInputStream(FileInputStream fis) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(fis));
char[] buf = new char[10];
int len;
String str = "";
while((len = reader.read(buf)) != -1) {
str += new String(buf, 0, len);
}
return str;
}
}
13.11、NIO.2中Path、Paths、Files类的使用
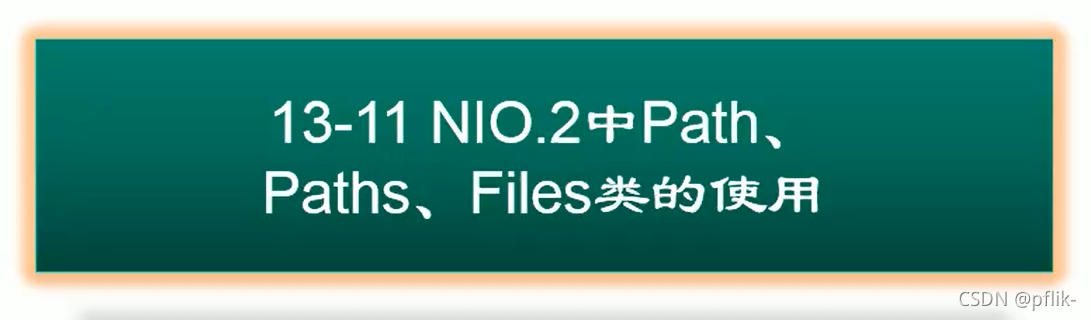 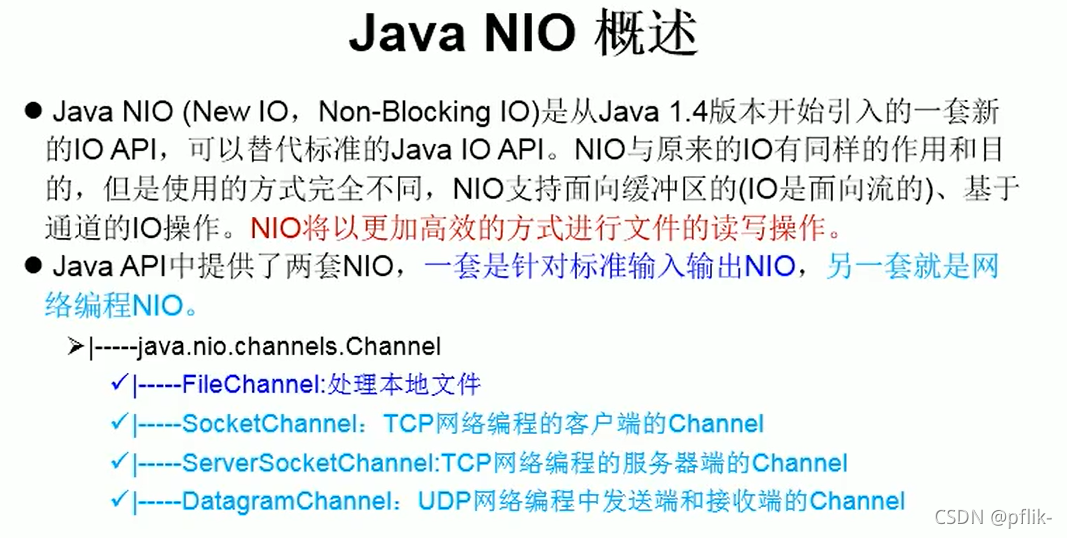 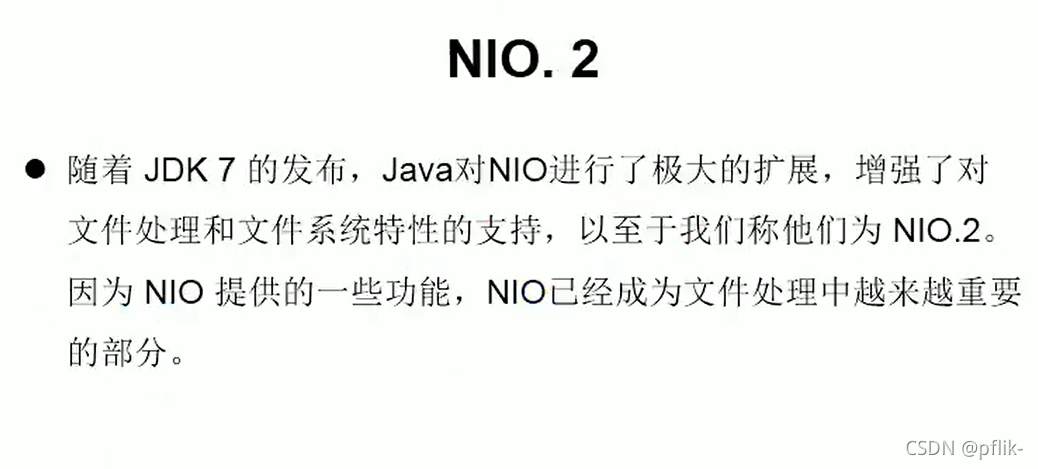 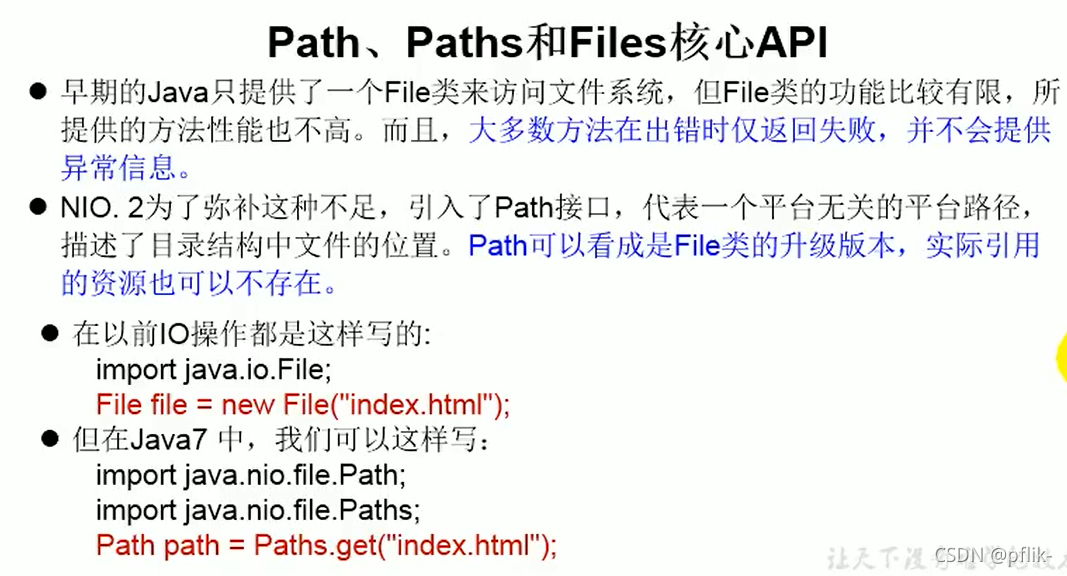 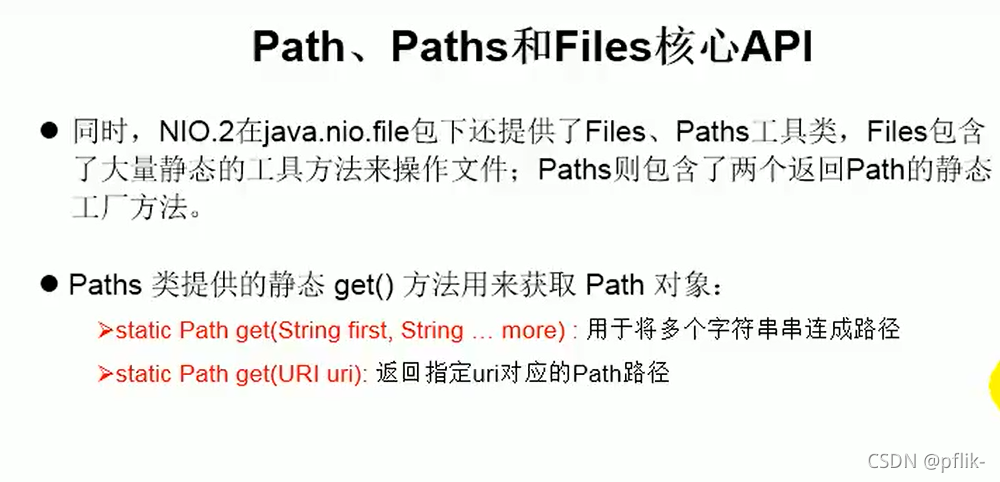 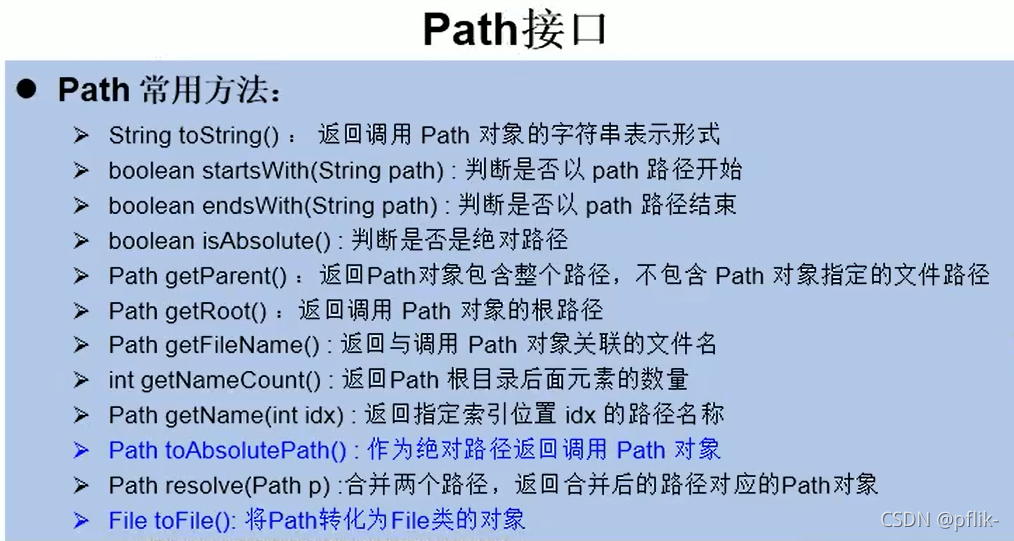 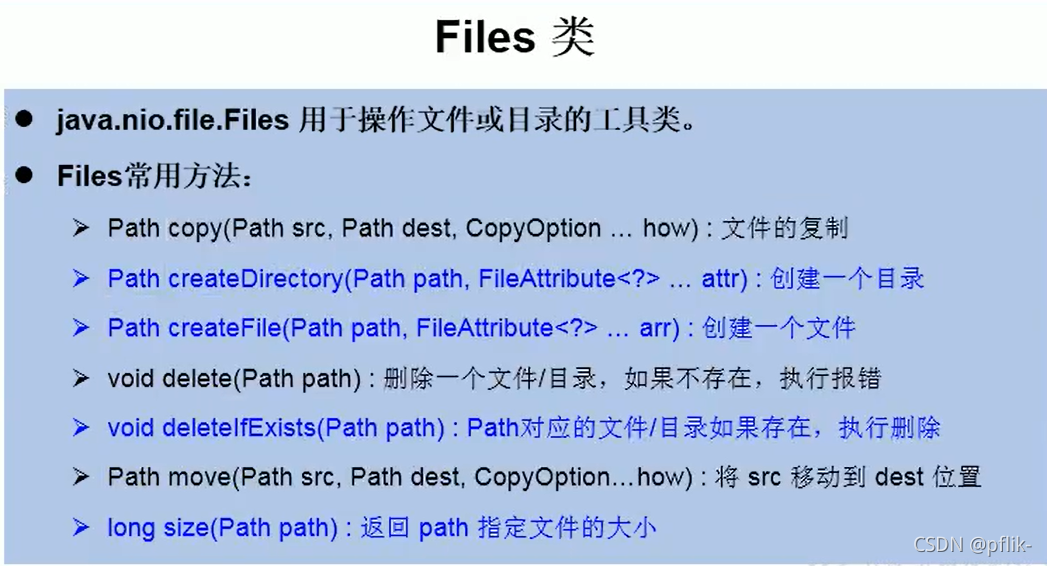 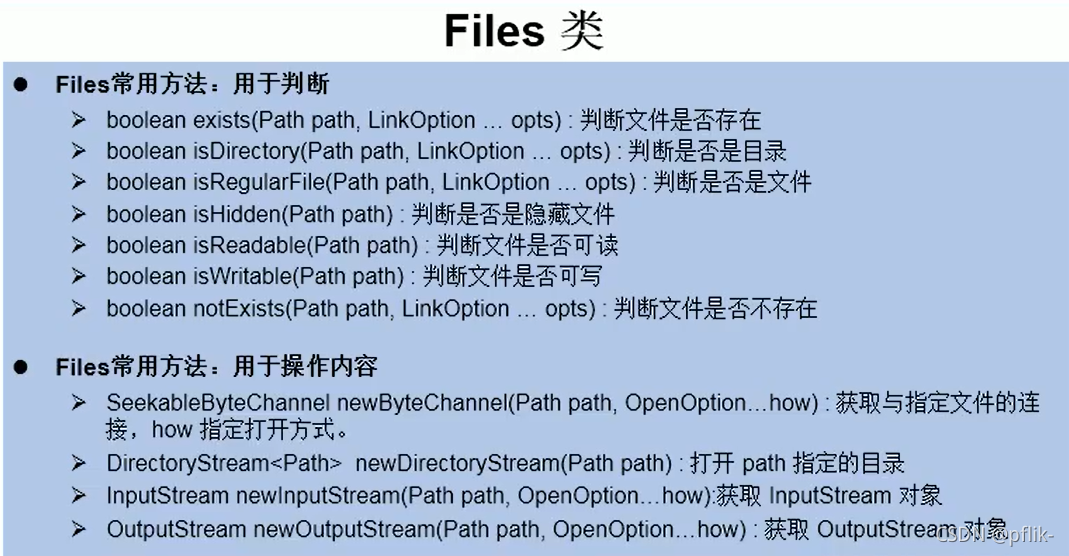
|