常用类
包装类Wrapper的分类
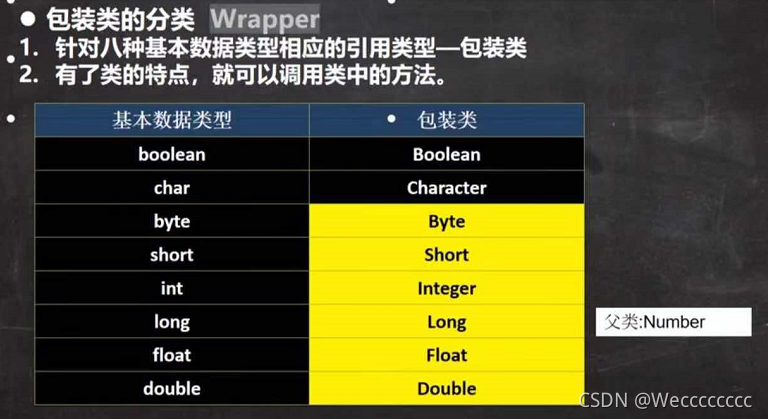
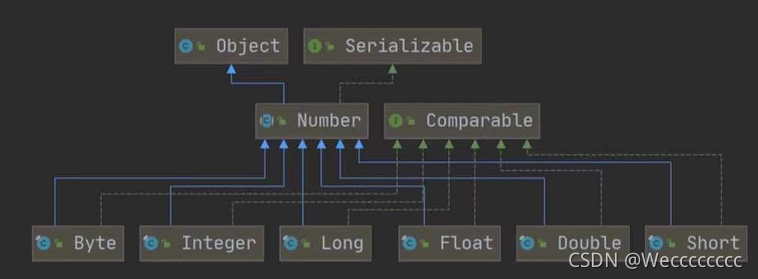
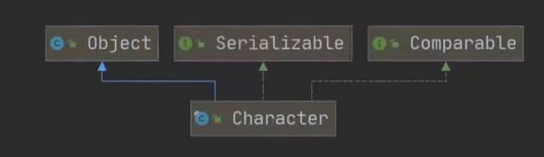
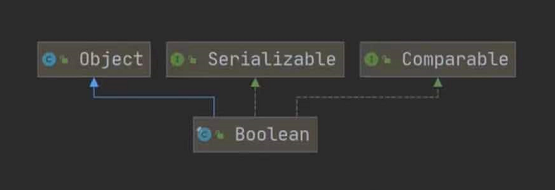
包装类和基本数据的转换
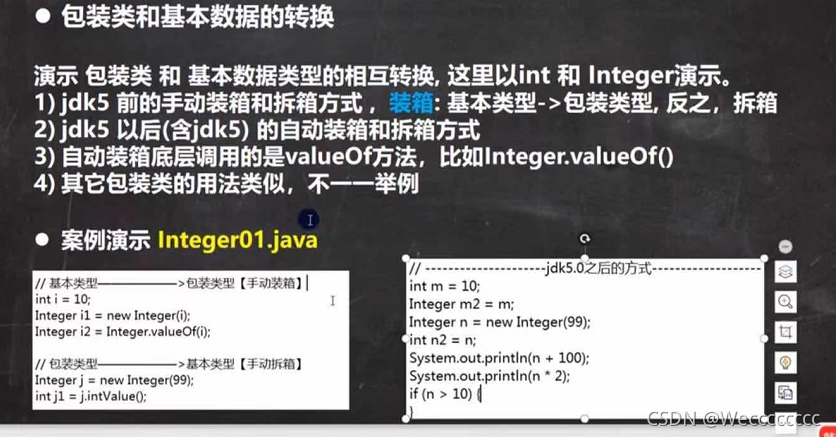
01:
package TryCatchExercise;
public class WrapperDemo {
public static void main(String[] args) {
int n1 = 100;
Integer integer = new Integer(n1);
Integer integer1 = Integer.valueOf(n1);
int i = integer.intValue();
int n2 = 200;
Integer integer2 = n2;
int n3 = integer2;
}
}
小练习
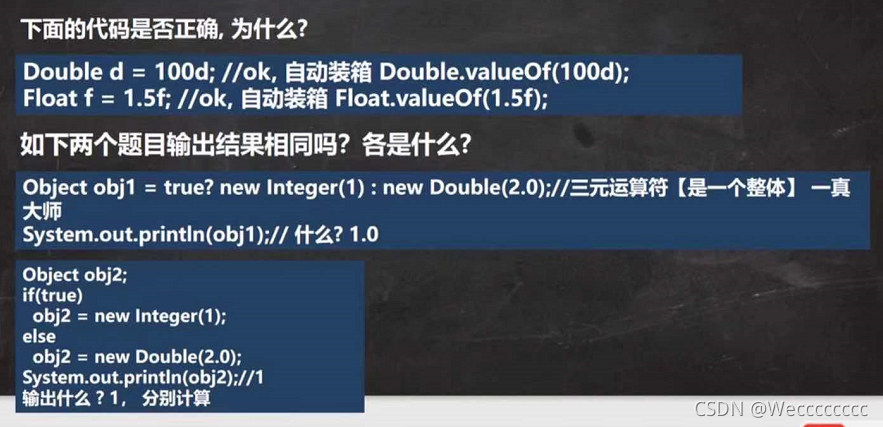
包装类型和String类型的相互转换
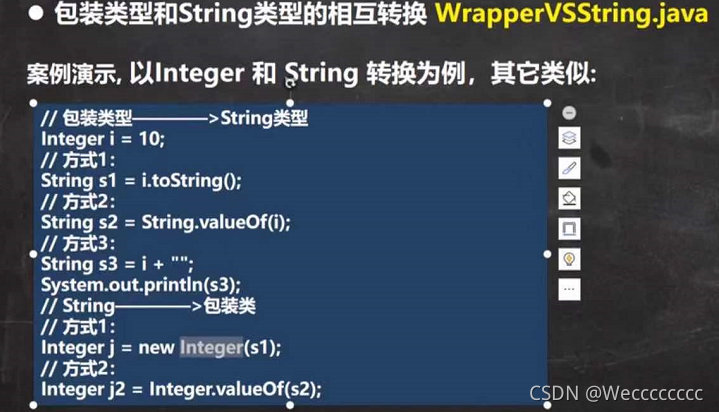
01:
package TryCatchExercise;
public class WrapperVSString {
public static void main(String[] args) {
Integer i = 100;
String str1 = i+"";
String str2 = i.toString();
String str3 = String.valueOf(i);
String str4 = "12345";
Integer i1 = Integer.parseInt(str4);
Integer integer = new Integer(str4);
}
}
Integer类和Character类的常用方法
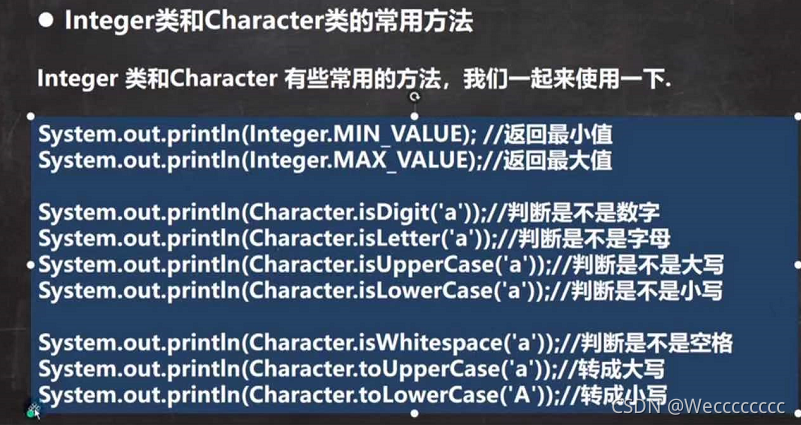
小练习
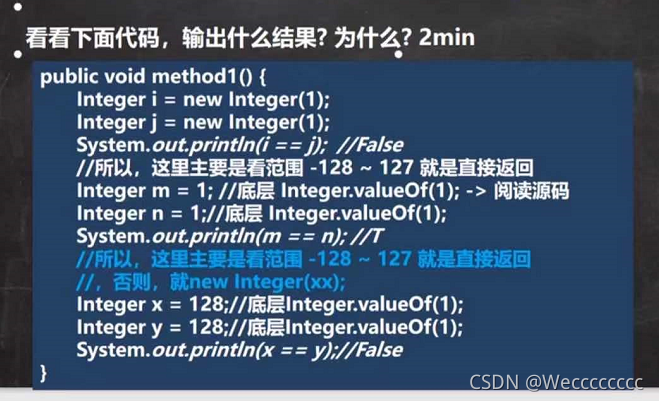
01:
package AbstractDemo01;
public class Test {
public static void main(String[] args) {
Integer i = new Integer(1);
Integer j = new Integer(1);
System.out.println(i==j);
Integer n = 1;
Integer m = 1;
System.out.println(n==m);
Integer x = 128;
Integer y = 128;
System.out.println(x==y);
}
}
02:
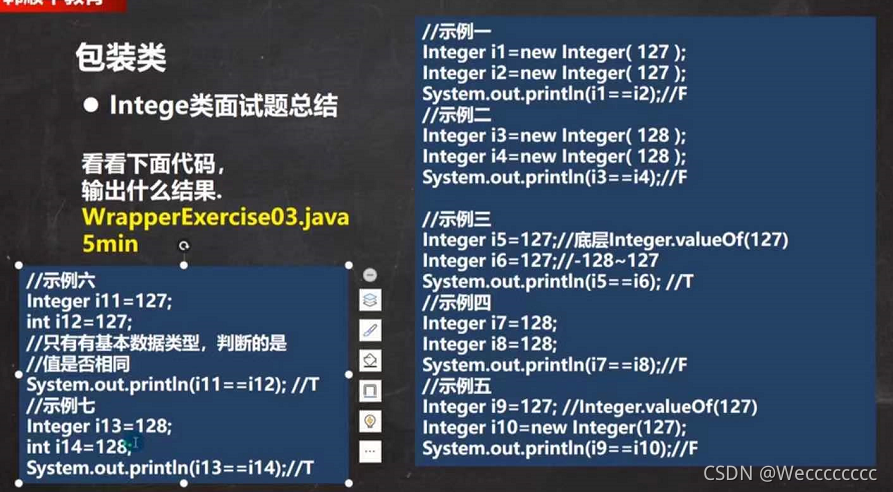
String类的理解和创建对象
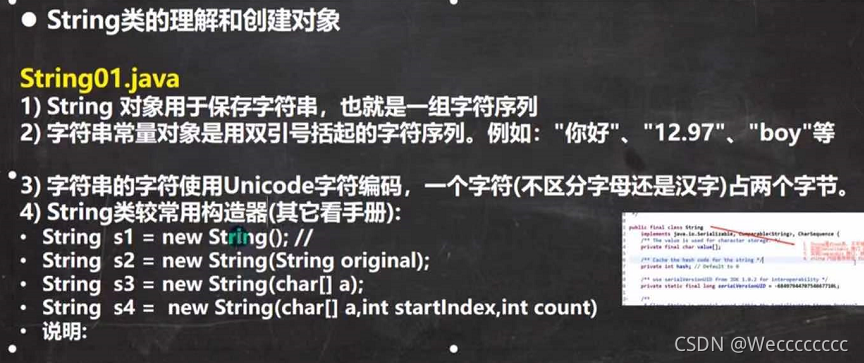
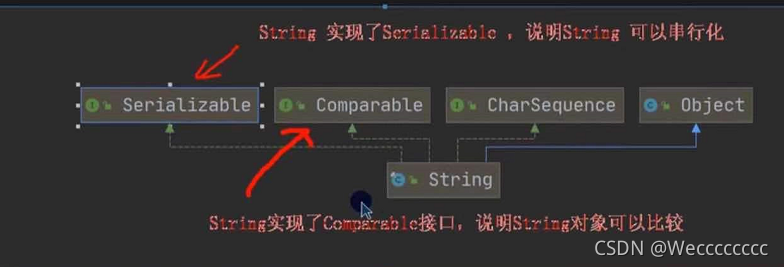
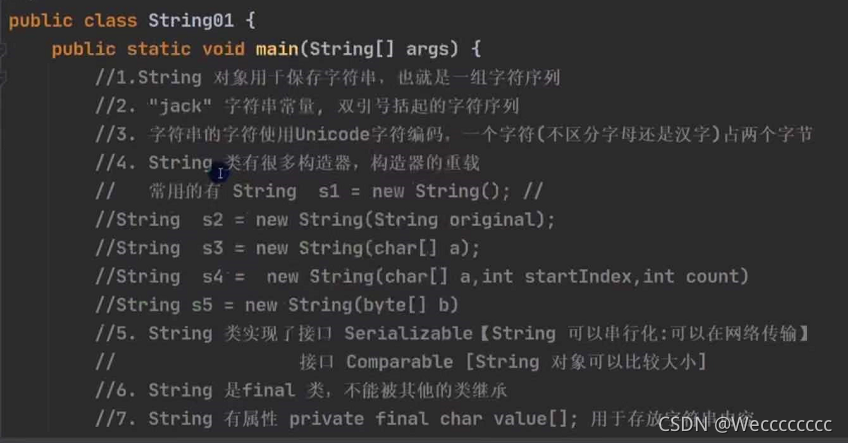
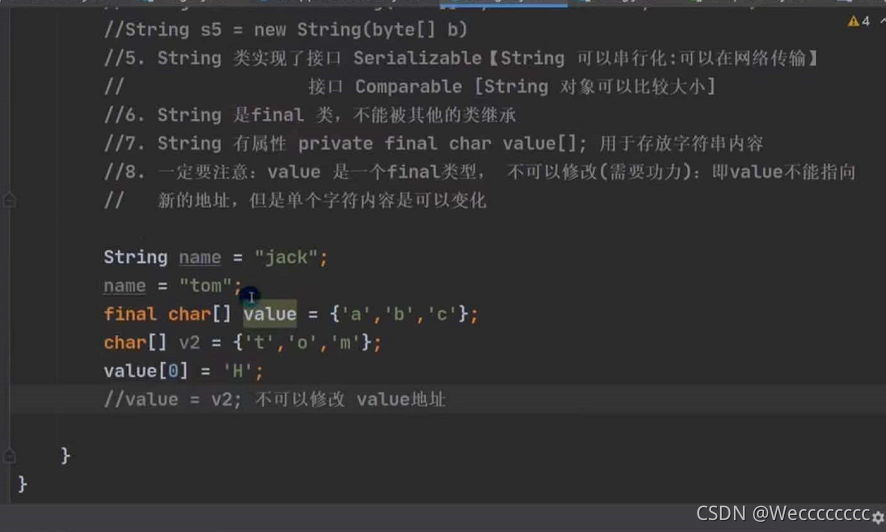
两种创建String对象的区别
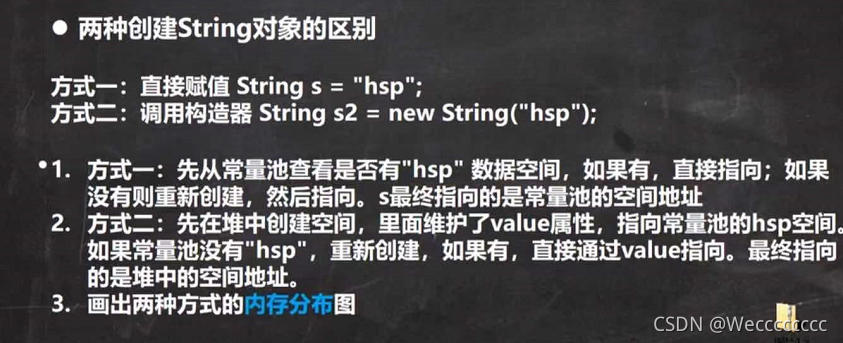 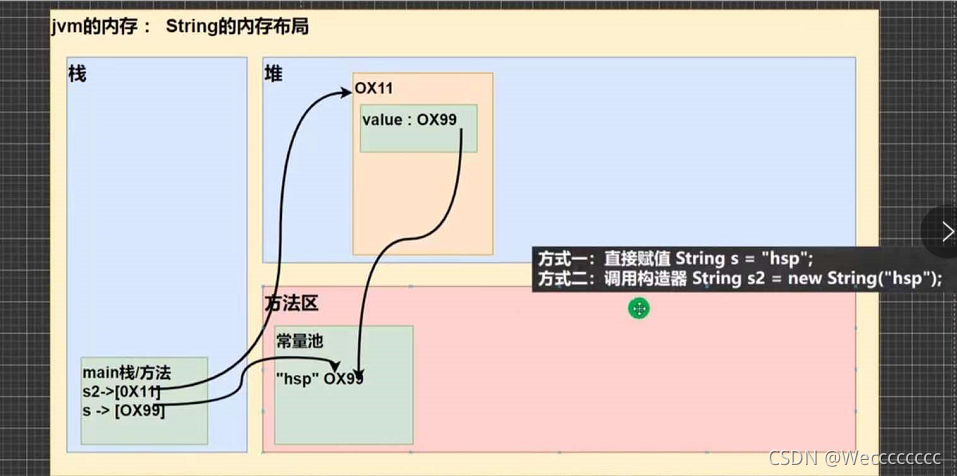
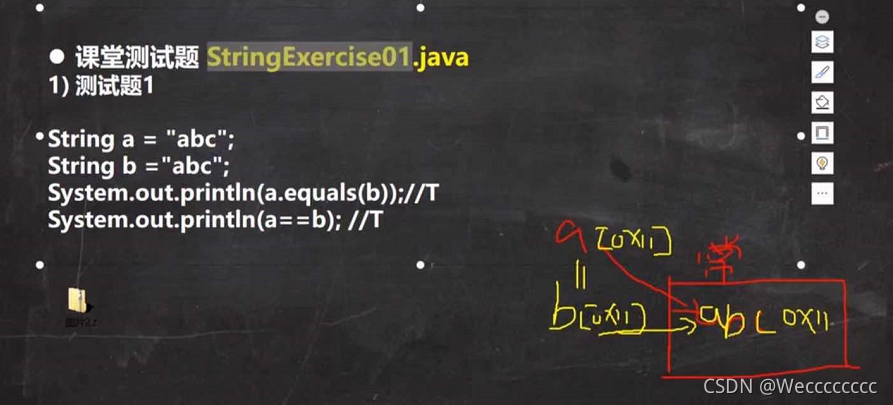 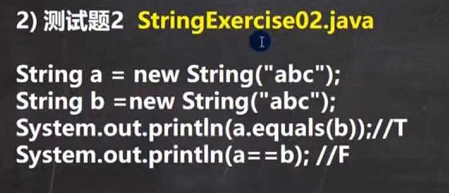
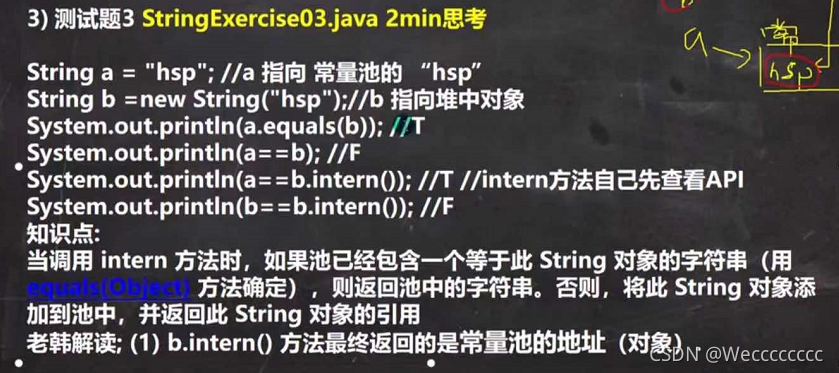
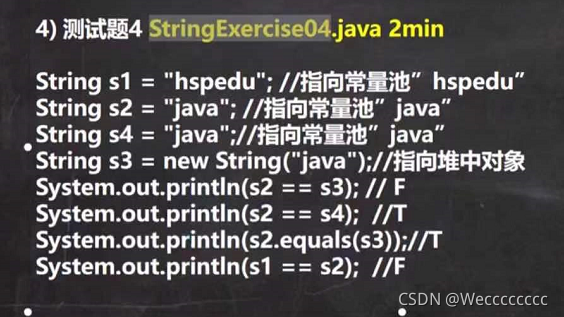
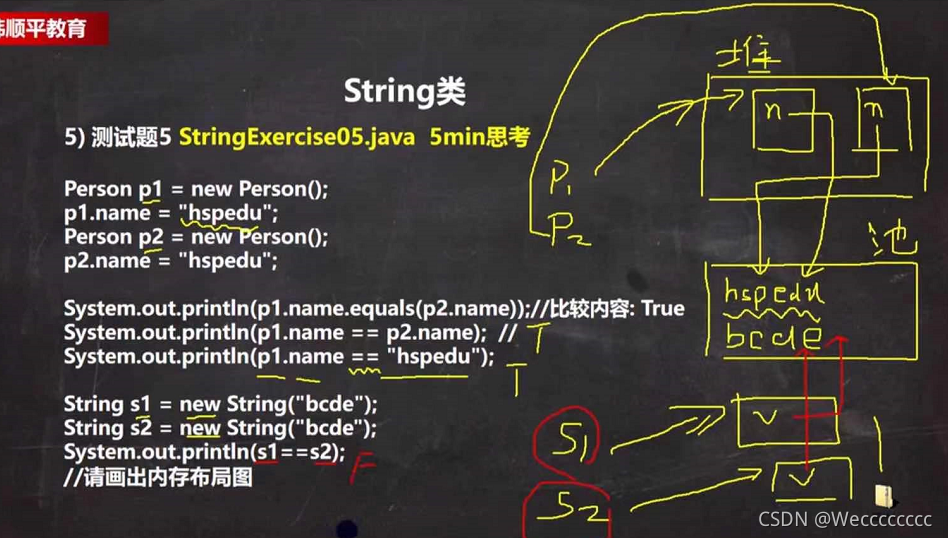
字符串的特性
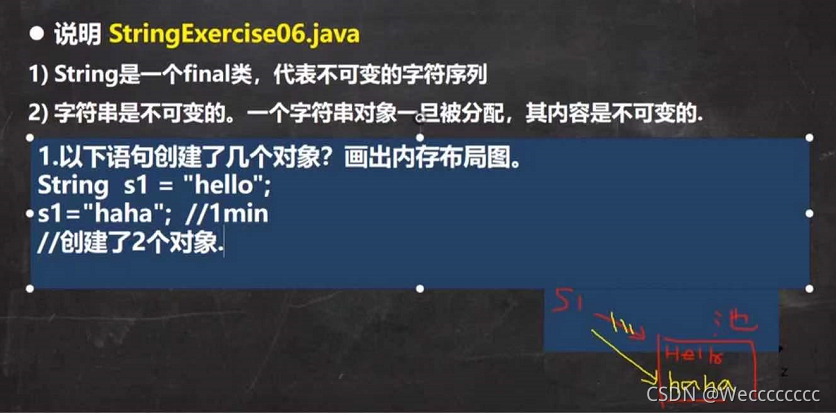
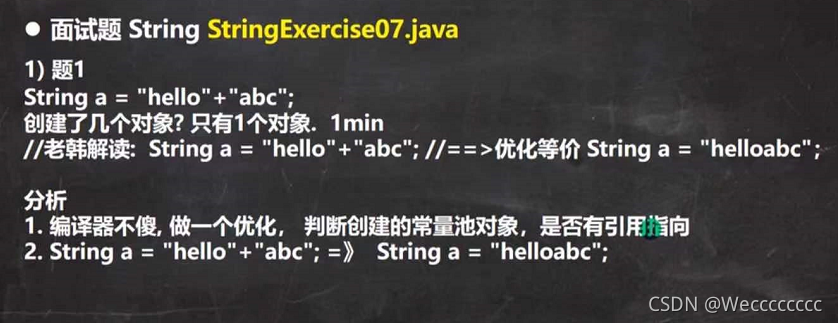
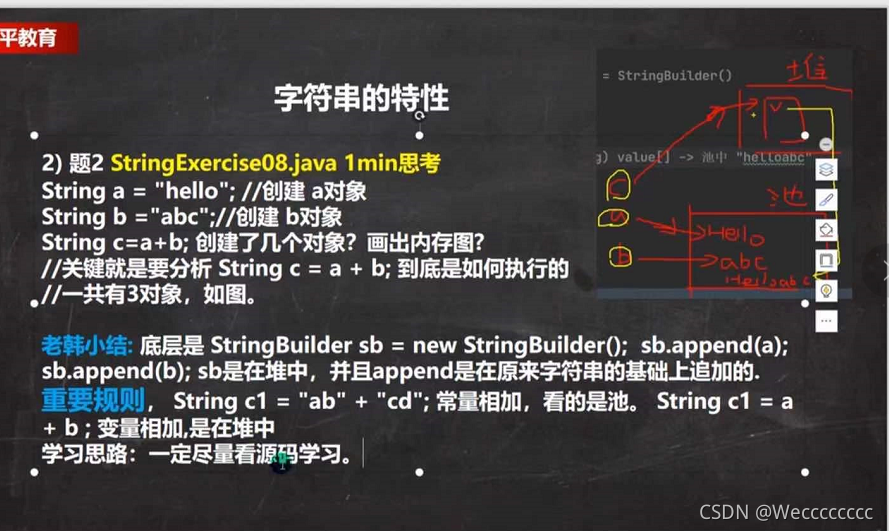
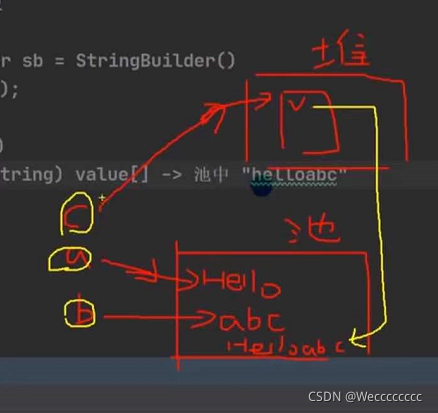
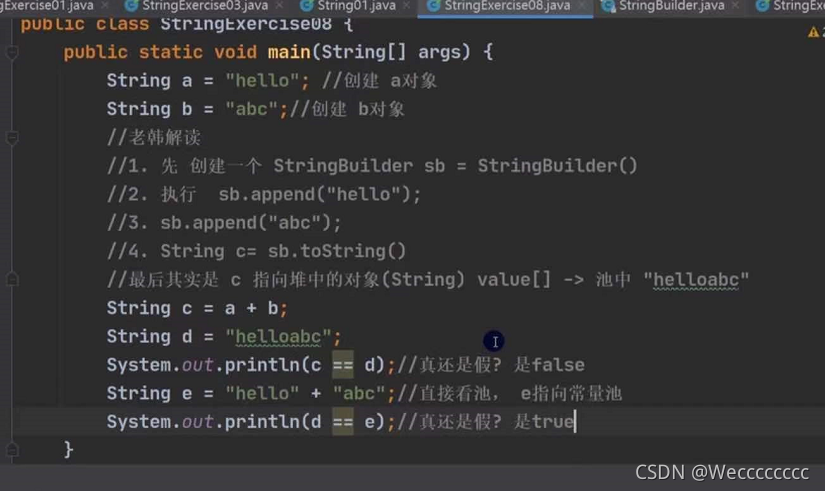
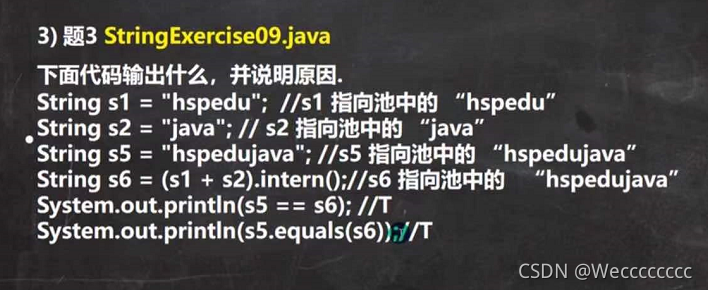
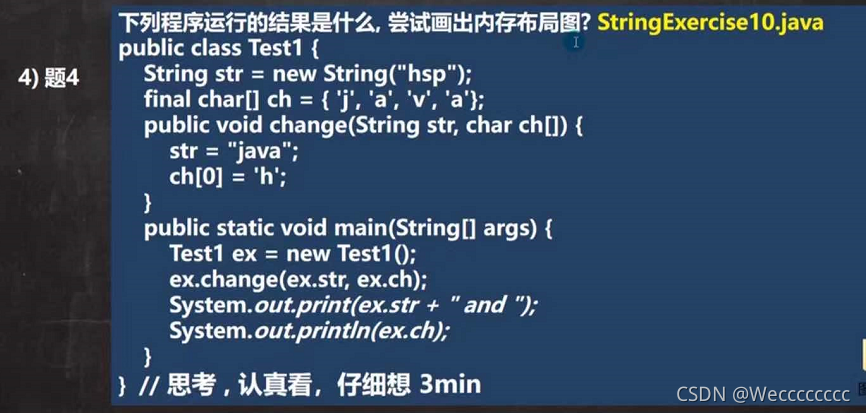 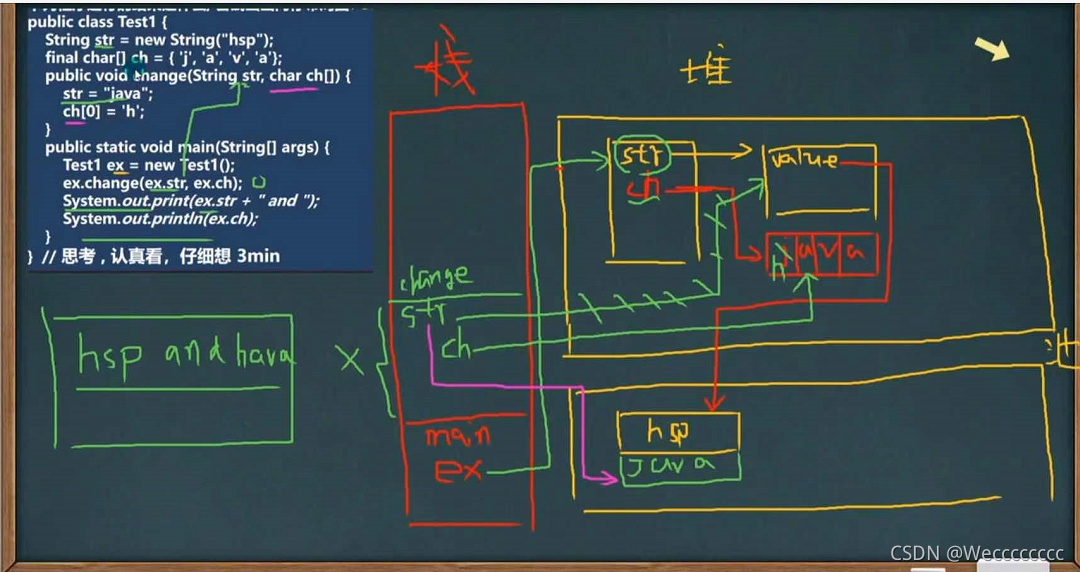
String类的常用方法
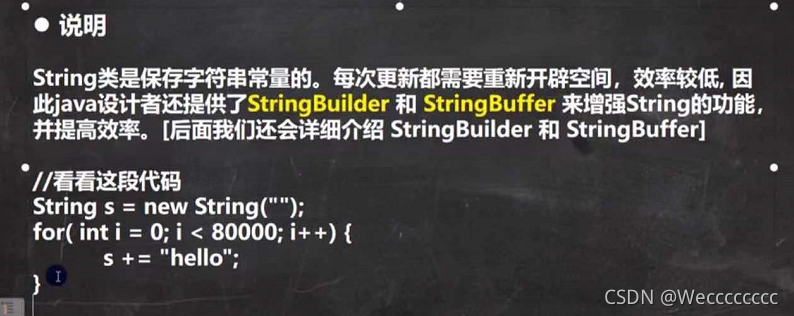 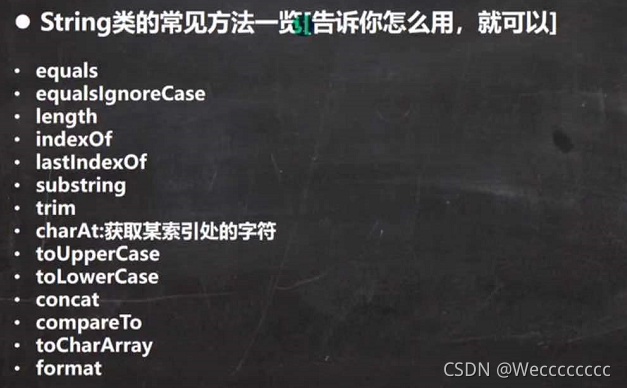
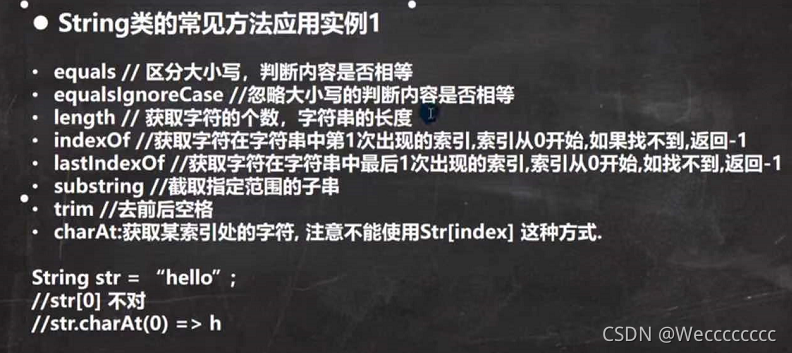
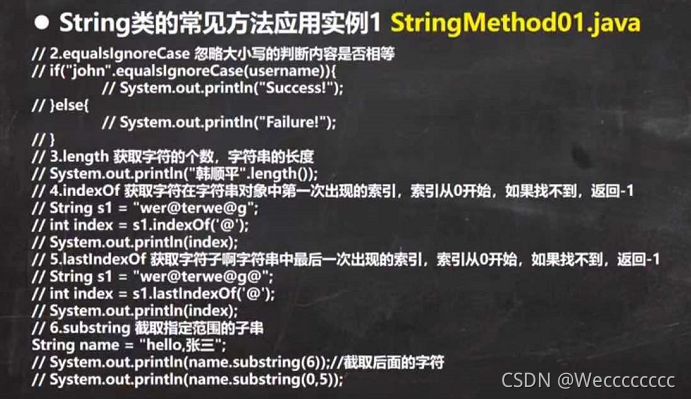
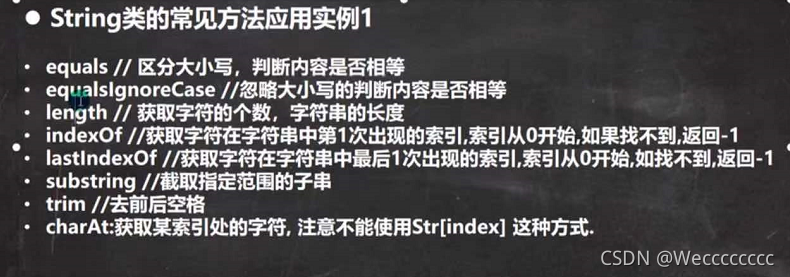
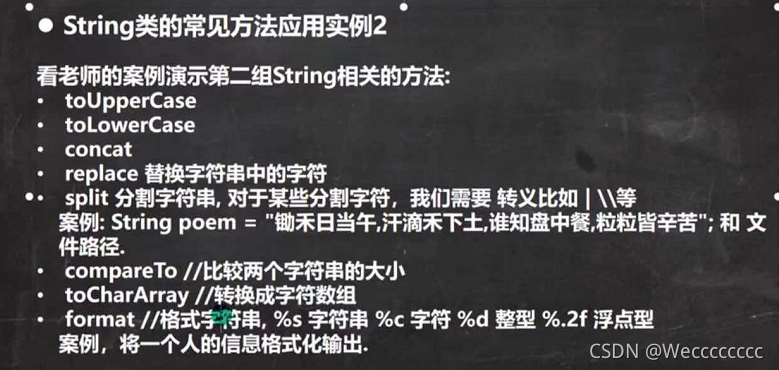
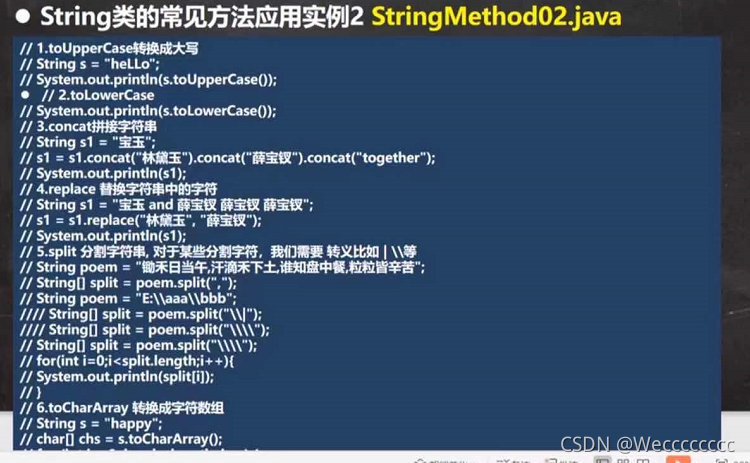
01:
package StringDemo01;
import java.util.Locale;
public class StringMethod01 {
public static void main(String[] args) {
String str1 = "hello";
String str2 = "Hello";
System.out.println(str1.equals(str2));
String username = "join";
if ("join".equalsIgnoreCase(username))
{
System.out.println("join yes");
}
else
{
System.out.println("join no");
}
String username2 = "JoIn";
if ("join".equalsIgnoreCase(username2))
{
System.out.println("join yes");
}
else
{
System.out.println("join no");
}
System.out.println("jack".length());
String s1 = "wer@terwe@g";
int idx = s1.indexOf('@');
System.out.println(idx);
s1 = "wer@asdasda@ad";
idx = s1.lastIndexOf('@');
System.out.println(idx);
String name = "jackioio";
System.out.println(name.substring(6));
System.out.println(name.substring(0,3));
String s = "hello";
System.out.println(s.toUpperCase());
System.out.println(s.toLowerCase());
String ss1 = "baoyu";
ss1 = s.concat("jack").concat("jxuad").concat("dasda");
System.out.println(ss1);
ss1 = "jack and mike jack";
ss1 = ss1.replace("jack","tom");
System.out.println(ss1);
String sss1 = "jack,miek,lll";
String[] split = sss1.split(",");
for (String v:split)
{
System.out.println(v);
}
String hhh = "hhkad\\asdas\\adas";
String[] hhhs = hhh.split("\\\\");
for (String v:hhhs)
{
System.out.println(v);
}
String h1 = "dafsfsdf";
char [] chs = h1.toCharArray();
for (int i = 0;i< h1.length();i++)
{
System.out.println(chs[i]);
}
String a = "jack";
String b = "apple";
System.out.println(b.compareTo(a));
a = "jack1";
b = "jack111";
System.out.println(b.compareTo(a));
String studentname = "john";
int age = 10;
double score = 98.3/3;
char gender = '男';
String info = "My name is"+name+"age = "+age+"score = "+score+"gender = "+gender+"I want to make eyeryone happy";
String formatStr = "my name is%s age = %d score = %.2f gender = %c ";
String info2 = String.format(formatStr,name,age,score,gender);
System.out.println(info);
System.out.println(info2);
}
}
StringBuffer类基本介绍
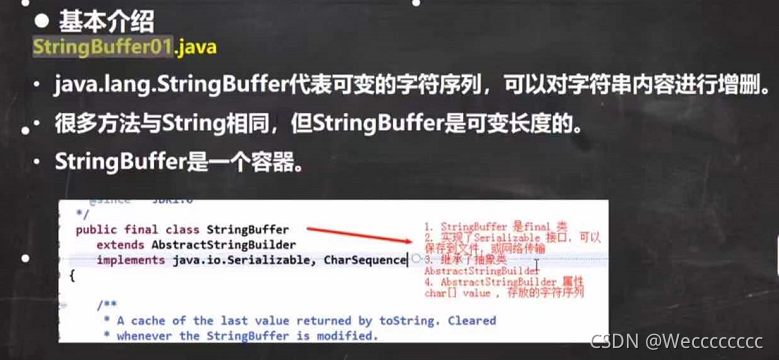
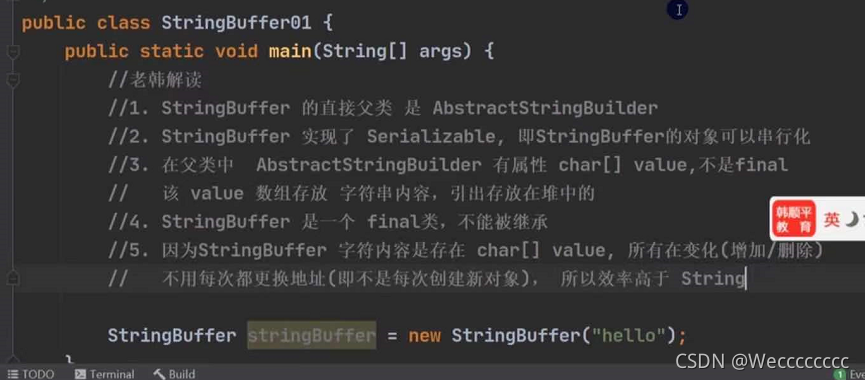
String VS StringBuffer
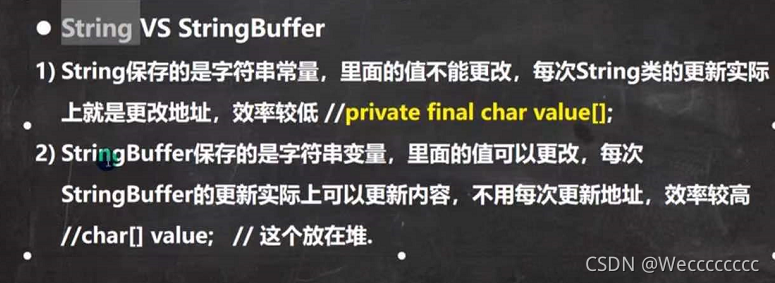
StringBuffer的构造器
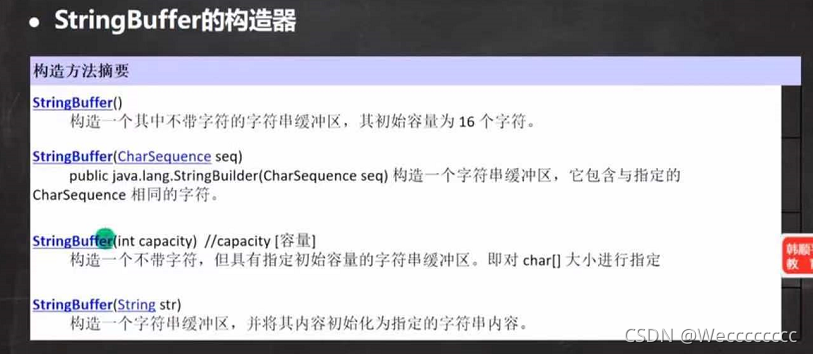
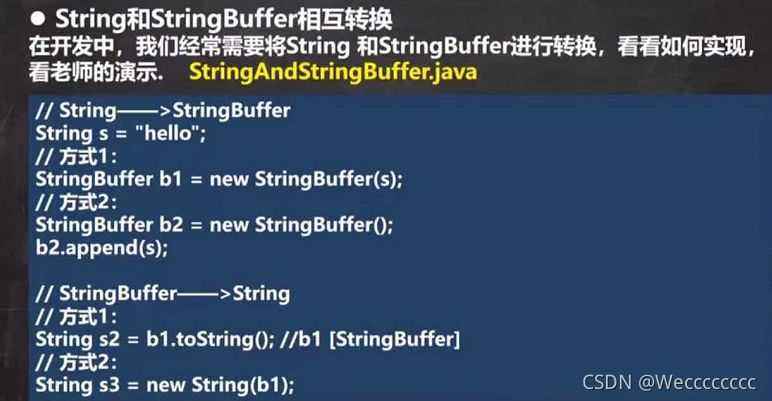
01:
package StringBufferDemo01;
public class StringBufferDemo {
public static void main(String[] args) {
StringBuffer stringBuffer = new StringBuffer();
StringBuffer stringBuffer1 = new StringBuffer(100);
String hello = new String("hello");
String str = "hello tom";
StringBuffer stringBuffer2 = new StringBuffer(str);
StringBuffer stringBuffer3 = new StringBuffer();
StringBuffer append = stringBuffer3.append(str);
StringBuffer hspedu = new StringBuffer("hspedu");
String s = hspedu.toString();
String s1 = new String(stringBuffer3);
}
}
StringBuffer类常见方法
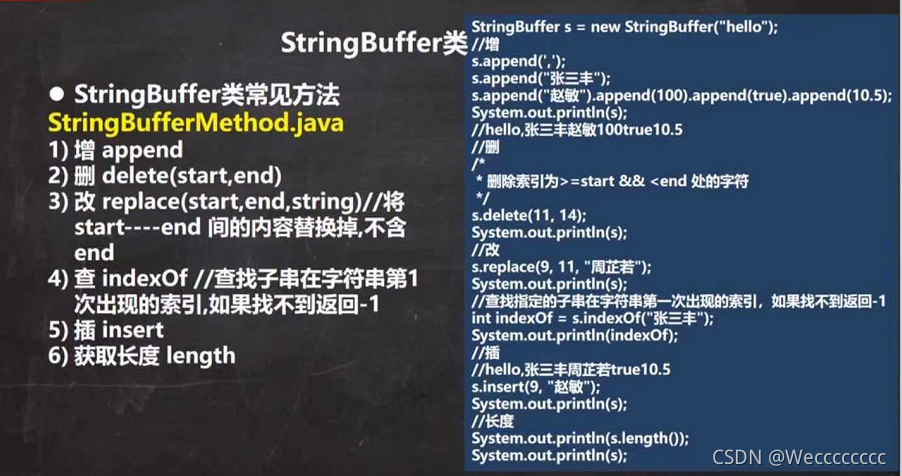
01:
package StringBufferMethod;
public class StringBufferMethod {
public static void main(String[] args) {
StringBuffer hello = new StringBuffer("hello");
hello.append(',');
hello.append("zsf");
hello.append("zhaom").append(100).append(true).append(10.5);
System.out.println(hello);
hello.delete(11,14);
System.out.println(hello);
hello.replace(9,11,"jack");
System.out.println(hello);
hello.insert(9,"mike");
System.out.println(hello);
int length = hello.length();
System.out.println(length);
}
}
小练习
01:
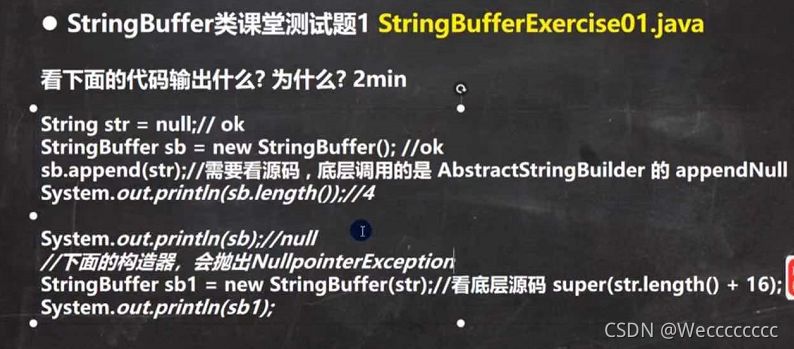
02:
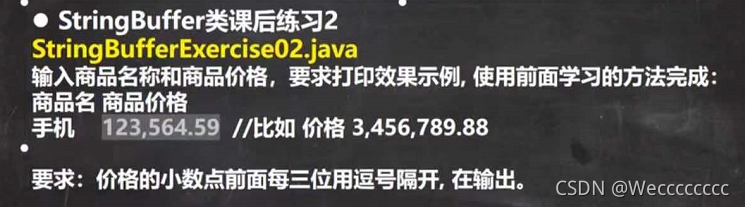
package StringBufferMethod;
public class StringBufferExercise01 {
public static void main(String[] args) {
String price = "123565467894.59";
StringBuffer sb = new StringBuffer((price));
for (int i = sb.lastIndexOf(".");i-3 >0;i-=3)
{
sb = sb.insert(i-3,",");
}
System.out.println(sb);
}
}
StringBuilder类结构剖析
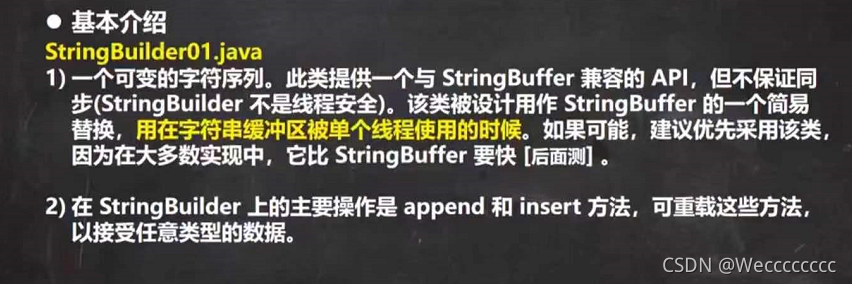
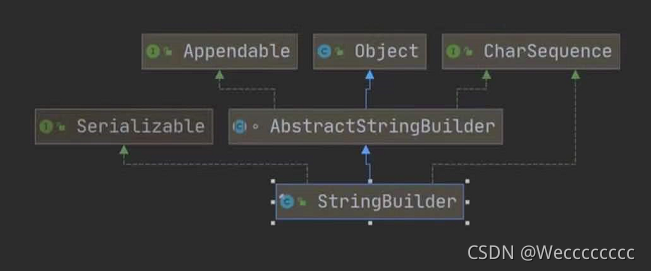
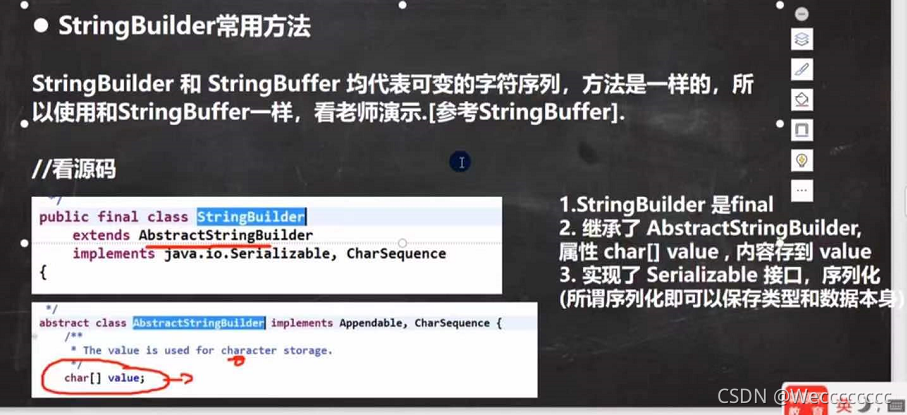
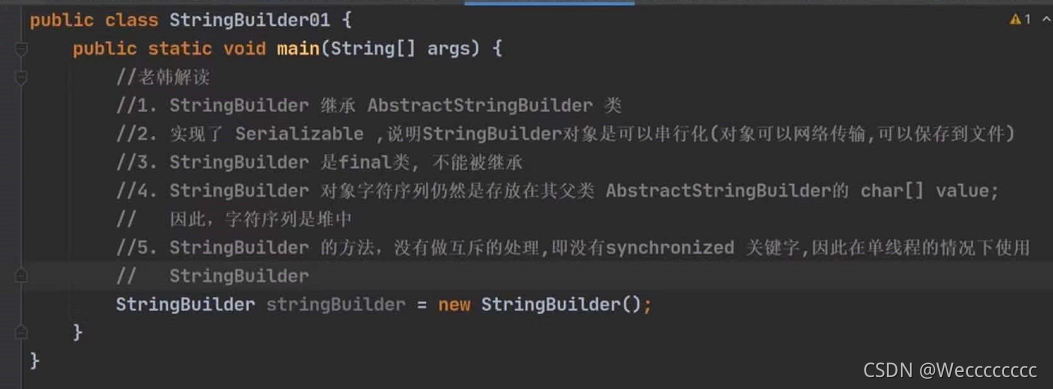
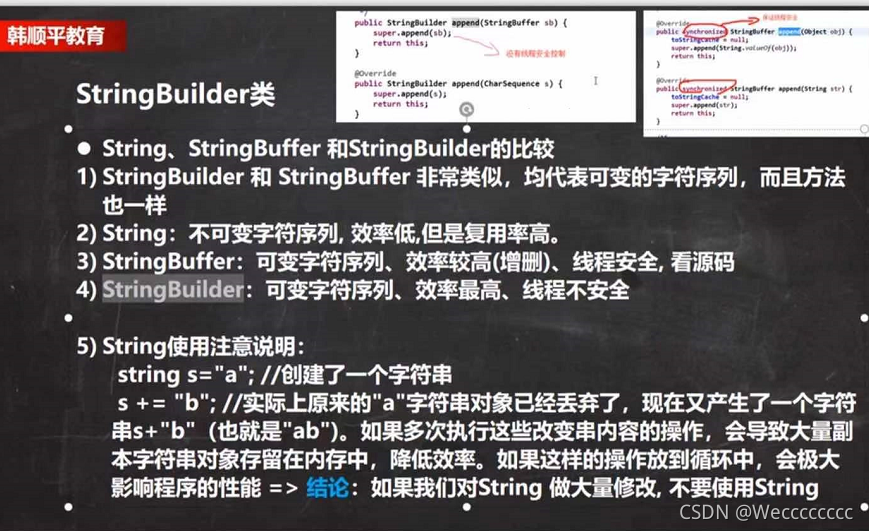
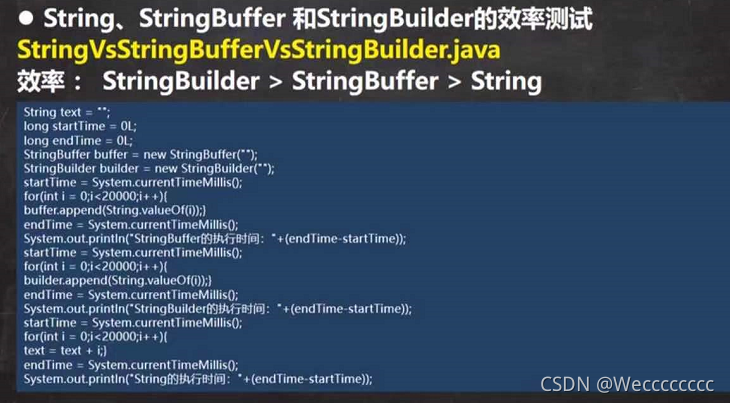
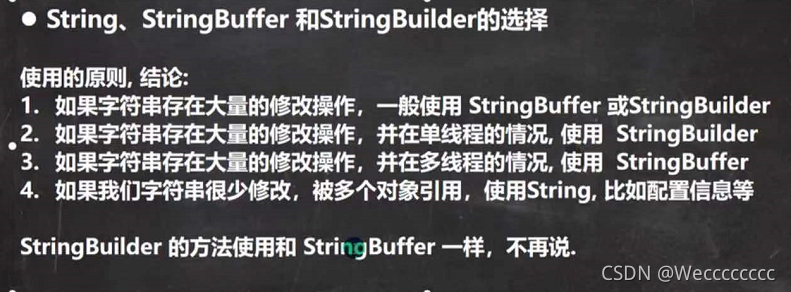
Math类
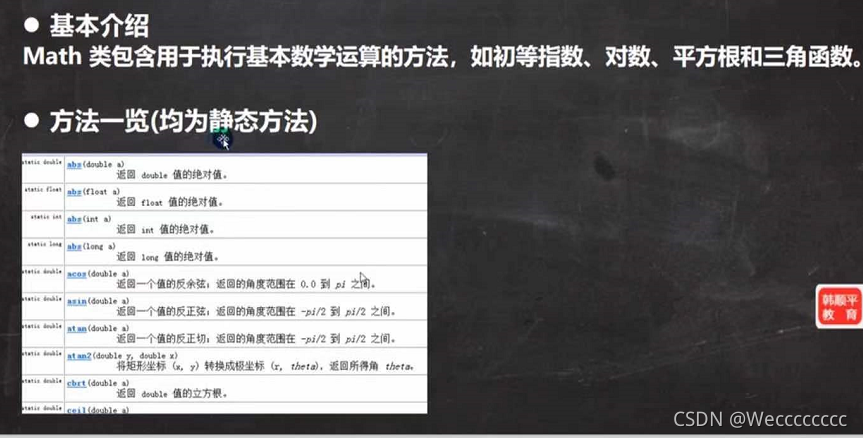
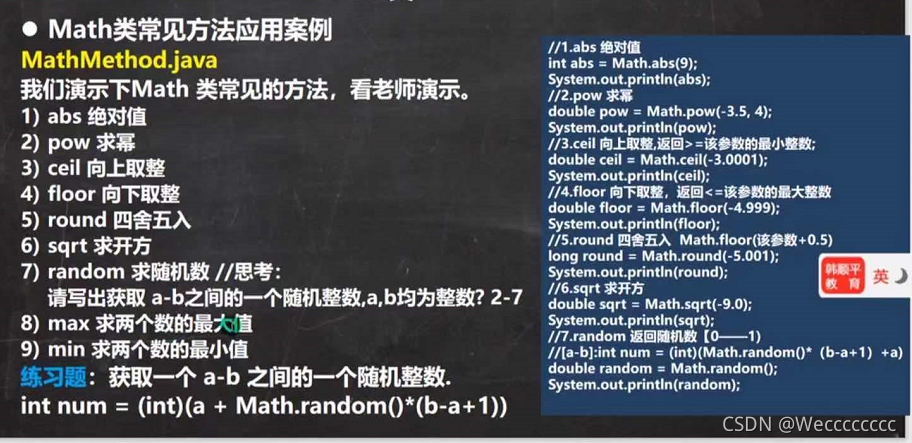
01:
package StringBufferMethod;
public class MathMethod {
public static void main(String[] args) {
int abs = Math.abs(9);
System.out.println(abs);
double pow = Math.pow(2,4);
System.out.println(pow);
double ceil = Math.ceil(-3.0001);
System.out.println(ceil);
double floor = Math.floor(-4.999);
System.out.println(floor);
long round = Math.round(-5.001);
System.out.println(round);
double sqrt = Math.sqrt(9.0);
System.out.println(sqrt);
for (int i =0;i<10;i++)
{
System.out.println(Math.random());
}
int num1 = Math.max(1,2);
System.out.println(num1);
}
}
Arrays类
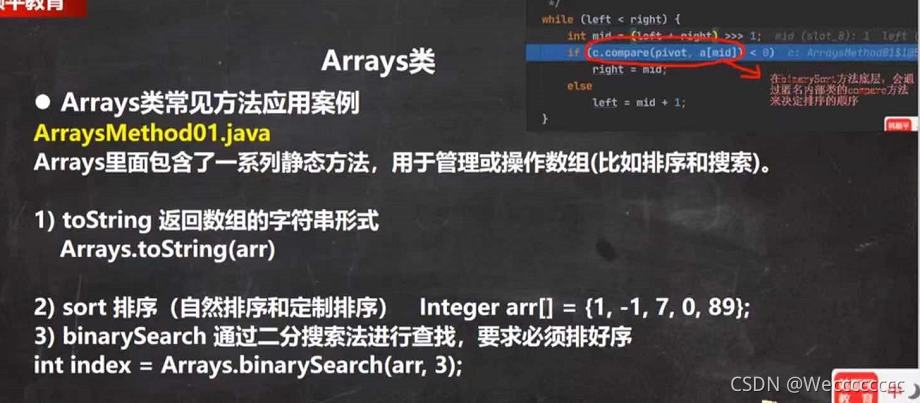
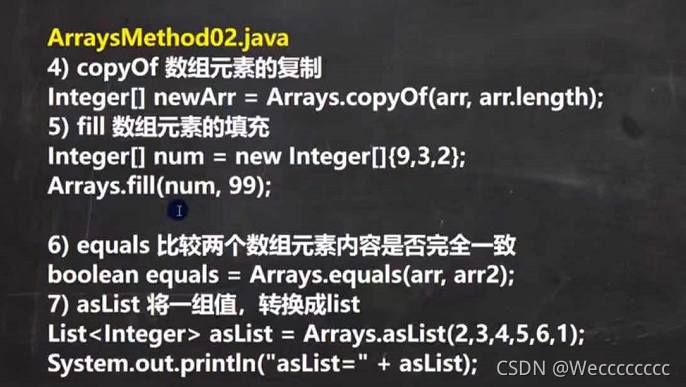
01:
package AbstractDemo01;
import java.util.Arrays;
import java.util.Comparator;
public class ArraysMethod01 {
public static void main(String[] args) {
Integer[] integers = {1,2,3};
for (int i =0;i<integers.length;i++)
{
System.out.println(integers[i]);
}
System.out.println(Arrays.toString(integers));
Integer arr[] = {1,-1,7,0,89};
Arrays.sort(arr);
System.out.println(Arrays.toString(arr));
Arrays.sort(arr, new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2-o1;
}
});
System.out.println(Arrays.toString(arr));
}
}
自己简单实现一波sort
02:
package AbstractDemo01;
import java.util.Arrays;
import java.util.Comparator;
public class ArraysSortCustom {
public static void main(String[] args) {
int[] arr = {1,-1,8,0,20};
bubble01(arr);
System.out.println(Arrays.toString(arr));
bubble02(arr, new Comparator() {
@Override
public int compare(Object o1, Object o2) {
int o11 = (Integer) o1;
int o21 = (Integer) o2;
return o21-o11;
}
});
System.out.println(Arrays.toString(arr));
}
public static void bubble01(int [] arr)
{
int tmp = 0;
for (int i = 0;i<arr.length;i++)
{
for (int j = 0;j<arr.length-1-i;j++)
{
if (arr[j] > arr[j+1])
{
tmp = arr[j+1];
arr[j+1] = arr[j];
arr[j] = tmp;
}
}
}
}
public static void bubble02(int[] arr, Comparator c)
{
int tmp = 0;
for (int i = 0;i<arr.length;i++)
{
for (int j = 0;j<arr.length-1-i;j++)
{
if (c.compare(arr[j],arr[j+1]) > 0)
{
tmp = arr[j+1];
arr[j+1] = arr[j];
arr[j] = tmp;
}
}
}
}
}
03:
import java.util.Arrays;
public class SortDemo01 {
public static void main(String[] args) {
Integer[] arr = {1,2,3,4,5,6,6786,56734,322445};
int idx = Arrays.binarySearch(arr,1);
System.out.println(idx);
int idx02 = Arrays.binarySearch(arr,92);
System.out.println(idx02);
}
}
04:
import java.util.Arrays;
public class SortDemo01 {
public static void main(String[] args) {
Integer[] arr = {1,2,3,4,5,6,6786,56734,322445};
System.out.println(Arrays.toString(arr));
System.out.println("==============================================");
Integer[] newArray = Arrays.copyOf(arr,arr.length);
System.out.println(Arrays.toString(newArray));
System.out.println("==============================================");
Integer[] newArray01 = Arrays.copyOf(arr,arr.length-1);
System.out.println(Arrays.toString(newArray01));
System.out.println("==============================================");
Integer[] newArray02 = Arrays.copyOf(arr,arr.length+1);
System.out.println(Arrays.toString(newArray02));
}
}
05:
import java.util.Arrays;
import java.util.List;
public class SortDemo01 {
public static void main(String[] args) {
Integer[] arr = new Integer[]{9,3,2};
Arrays.fill(arr,99);
System.out.println(Arrays.toString(arr));
Integer[] arr01 = {1,2,3,124,2,423,4,23};
Integer[] arr02 = {1,3,123,12421,41,3,14};
boolean equals = Arrays.equals(arr01,arr02);
System.out.println(equals);
Integer[] arr03 = {1,2,3};
Integer[] arr04 = {1,2,3};
boolean equals01 = Arrays.equals(arr03,arr04);
System.out.println(equals01);
List<Integer> asList = Arrays.asList(2,3,4,5,6,8);
System.out.println(asList);
System.out.println(asList.getClass());
}
}
小练习
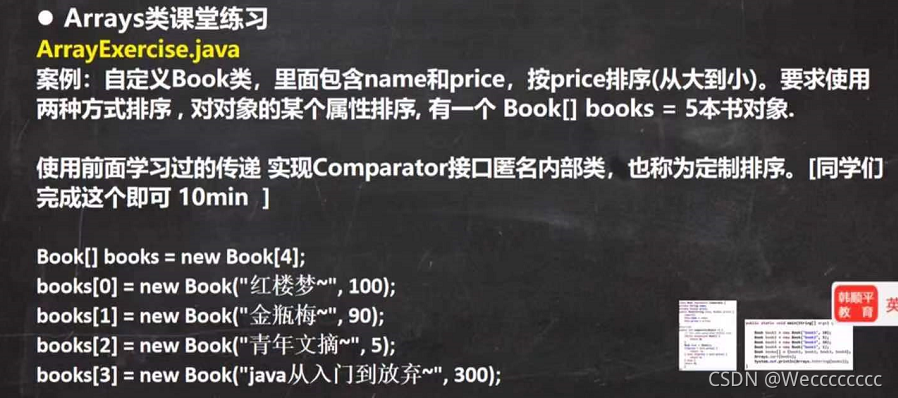
01:
package AbstractDemo01;
import java.util.Arrays;
import java.util.Comparator;
public class ArrayExercise {
public static void main(String[] args) {
Book[] books = new Book[4];
books[0] = new Book("red dream",100);
books[1] = new Book("gold new ",90);
books[2] = new Book("youth composition 20",5);
books[3] = new Book("java from enter door to give up",300);
Arrays.sort(books, new Comparator<Book>() {
@Override
public int compare(Book o1, Book o2) {
double priceVal = o2.getPrice()-o1.getPrice();
if (priceVal > 0)
{
return 1;
}
else if (priceVal < 0)
{
return -1;
}
else return 0;
}
});
System.out.println(Arrays.toString(books));
Arrays.sort(books, new Comparator<Book>() {
@Override
public int compare(Book o1, Book o2) {
double priceVal = o2.getPrice()-o1.getPrice();
if (priceVal > 0)
{
return -1;
}
else if (priceVal < 0)
{
return 1;
}
else return 0;
}
});
System.out.println(Arrays.toString(books));
Arrays.sort(books, new Comparator<Book>() {
@Override
public int compare(Book o1, Book o2) {
return o2.getName().length()-o1.getName().length();
}
});
System.out.println(Arrays.toString(books));
}
}
class Book
{
private String name;
private double price;
public Book(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", price=" + price +
'}';
}
}
System类
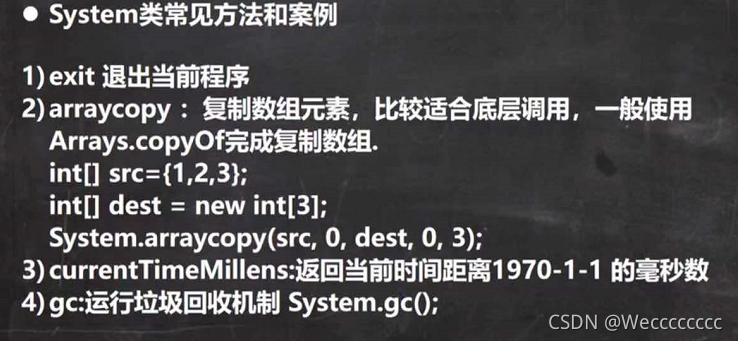
01:
package AbstractDemo01;
public class SystemTestDemo {
public static void main(String[] args) {
System.out.println("ok1");
System.exit(0);
System.out.println("ok2");
}
}
02:
package AbstractDemo01;
import java.util.Arrays;
public class SystemTestDemo {
public static void main(String[] args) {
int[] src = {1,2,3};
int[] dest = new int[3];
System.arraycopy(src,0,dest,0,src.length);
System.out.println(Arrays.toString(dest));
}
}
BigInteger和BigDecimal类
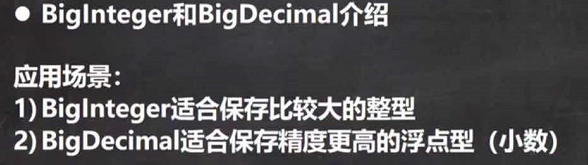
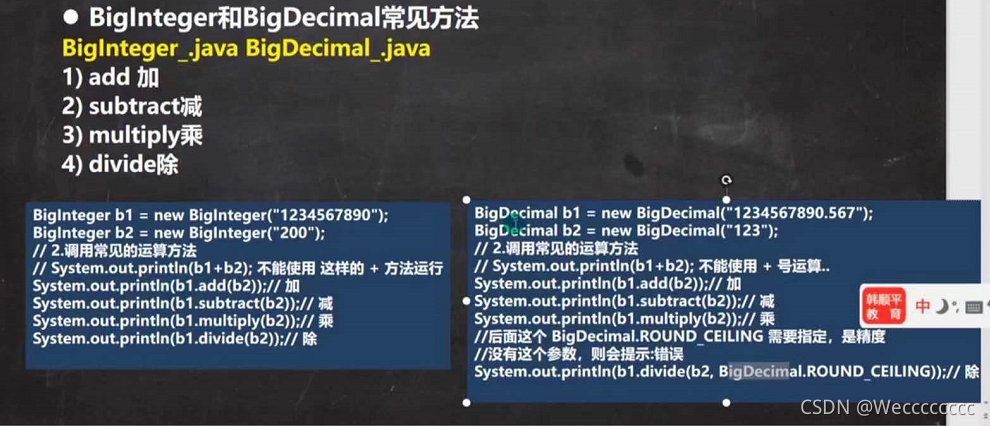
01:
package AbstractDemo01;
import java.math.BigInteger;
public class BigIntegerDemo {
public static void main(String[] args) {
BigInteger bigInteger = new BigInteger("456789556756734657894678768");
BigInteger bigInteger1 = new BigInteger("100");
System.out.println(bigInteger);
BigInteger add = bigInteger.add(bigInteger1);
System.out.println(add);
BigInteger subtract = bigInteger.subtract(bigInteger1);
System.out.println(subtract);
BigInteger multiply = bigInteger.multiply(bigInteger1);
System.out.println(multiply);
BigInteger divide = bigInteger.divide(bigInteger1);
System.out.println(divide);
}
}
02:
package BigDemo;
import java.math.BigDecimal;
public class BigDemo {
public static void main(String[] args) {
double d = 893.2134524351235424364325235;
System.out.println(d);
BigDecimal bigDecimal = new BigDecimal("893.2134524351235424364325235");
System.out.println(bigDecimal);
BigDecimal bigDecimal1 = new BigDecimal("1.1");
System.out.println(bigDecimal.add(bigDecimal1));
System.out.println(bigDecimal.subtract(bigDecimal1));
System.out.println(bigDecimal.multiply(bigDecimal1));
System.out.println(bigDecimal.divide(bigDecimal1,BigDecimal.ROUND_CEILING));
}
}
日期类
Date类
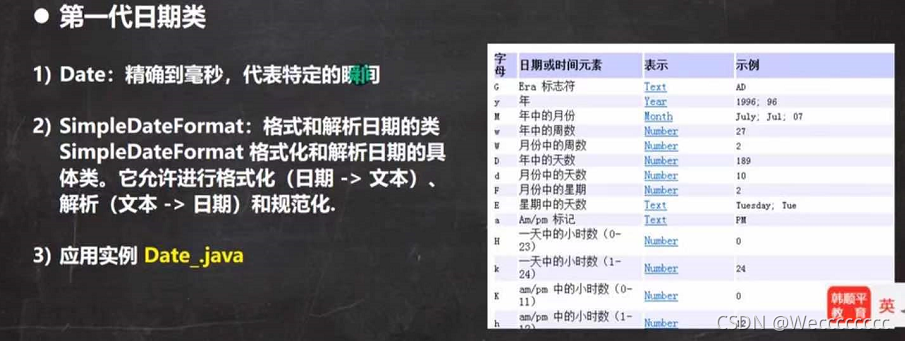 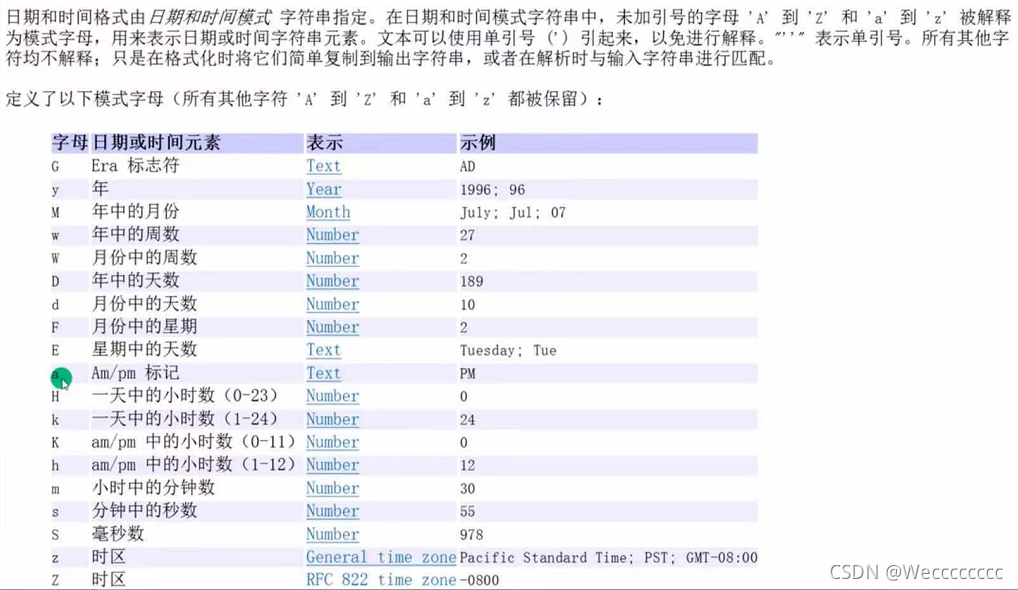
01:
package DateDemo01;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Date01 {
public static void main(String[] args) throws ParseException {
Date d1 = new Date();
System.out.println(d1);
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy年MM月dd日 hh:mm:ss E");
String format = simpleDateFormat.format(d1);
System.out.println(format);
Date date = new Date(9234567);
System.out.println(date);
String s = "1996年01月01日 10:20:30 星期一";
Date parse = simpleDateFormat.parse(s);
System.out.println(parse);
System.out.println(simpleDateFormat.format(parse));
}
}
Calendar类
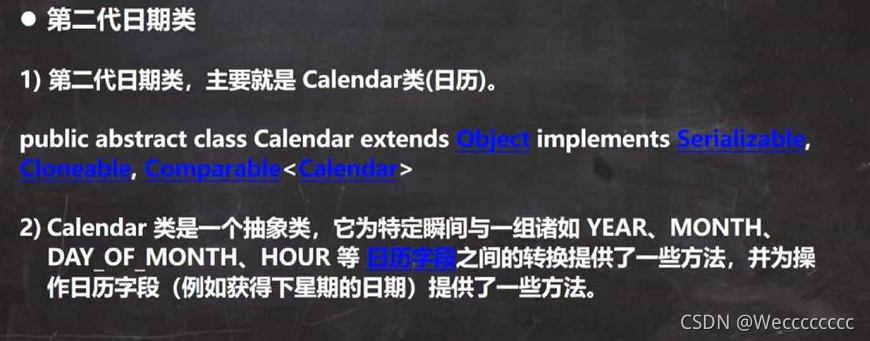
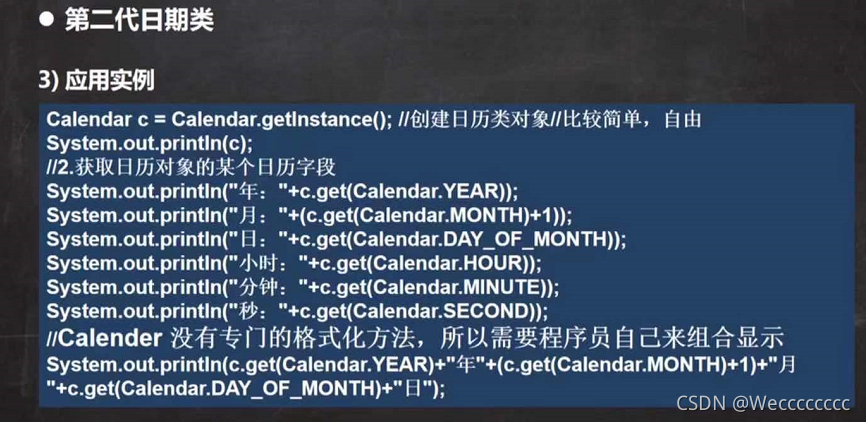
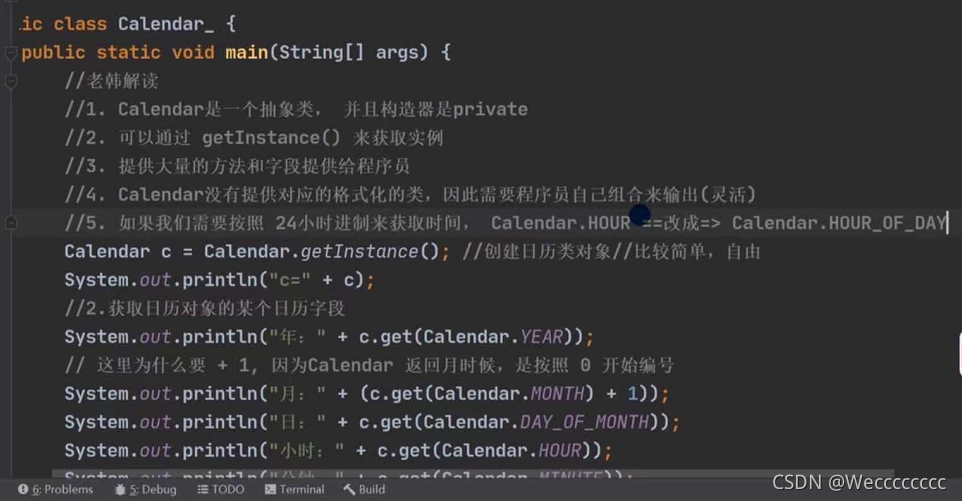
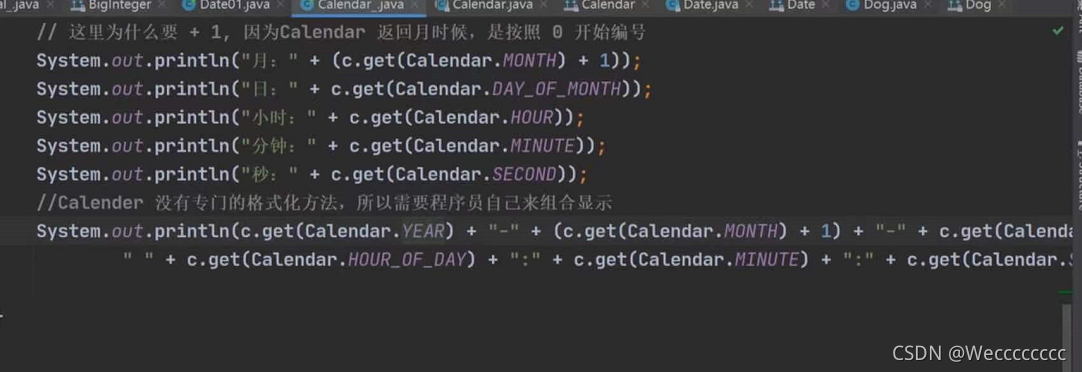
第三代日期
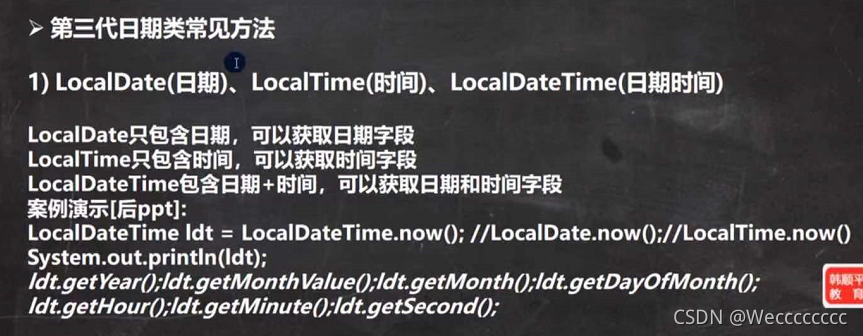
01:
package DateDemo01;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
public class LocalDateDemo01 {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
System.out.println(now);
System.out.println(now.getYear());
System.out.println(now.getMonth());
System.out.println(now.getMonthValue());
System.out.println(now.getDayOfMonth());
System.out.println(now.getHour());
System.out.println(now.getMinute());
System.out.println(now.getSecond());
LocalDate now1 = LocalDate.now();
System.out.println(now1.getYear());
LocalTime now2 = LocalTime.now();
System.out.println(now.getSecond());
}
}
DateTimeFormatter
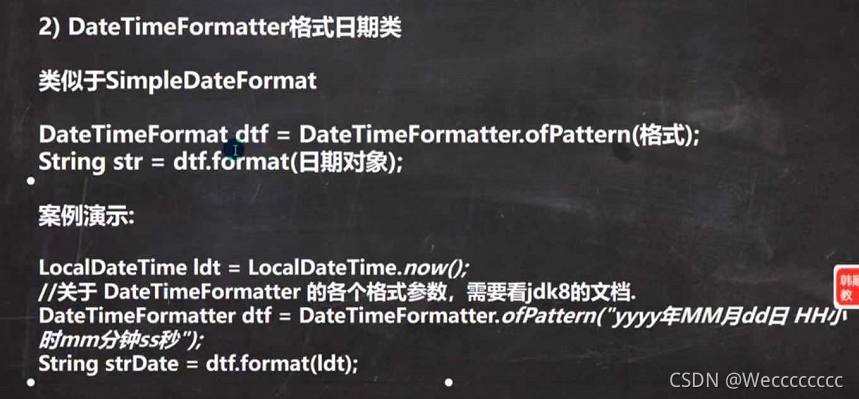
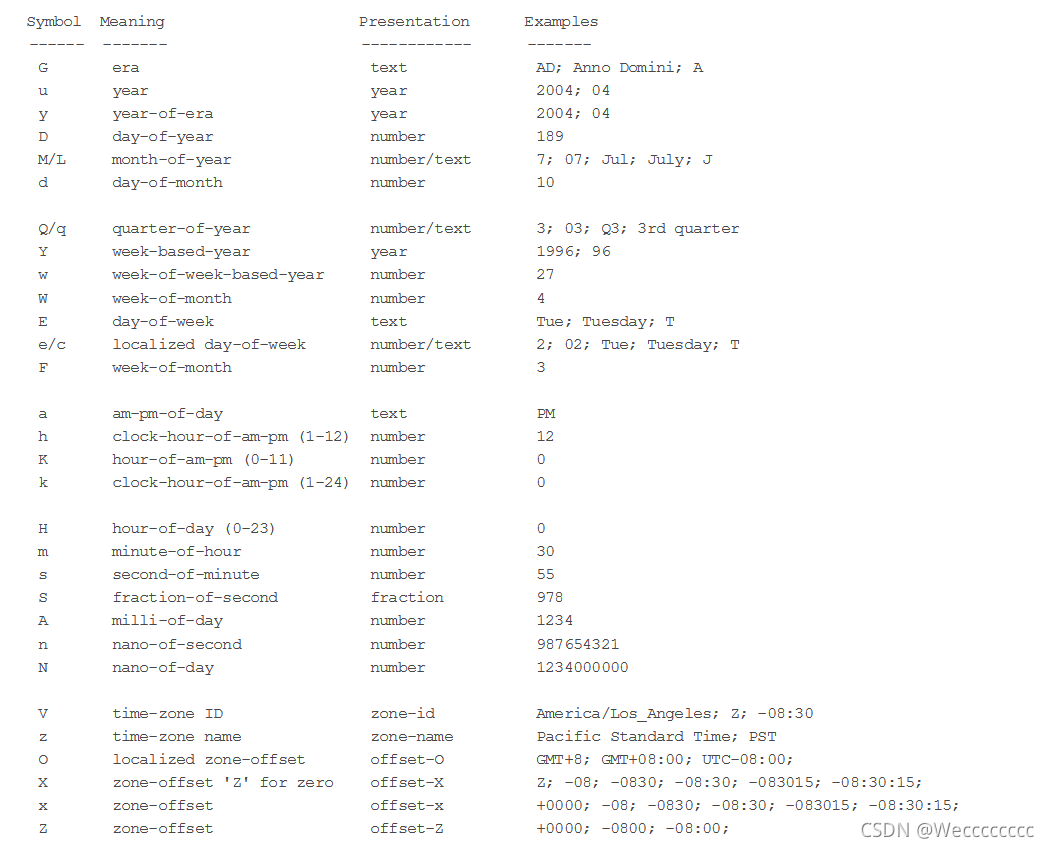
01:
package DateDemo01;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class LocalDateDemo02 {
public static void main(String[] args) {
LocalDateTime ldt = LocalDateTime.now();
System.out.println(ldt);
DateTimeFormatter dateTimeFormatter = DateTimeFormatter.ofPattern("yyyy年MM月dd日 HH小时mm分钟ss秒");
String format = dateTimeFormatter.format(ldt);
System.out.println("date = "+format);
}
}
Instant时间戳
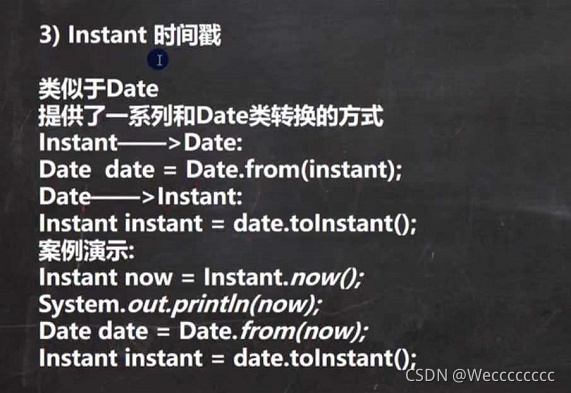
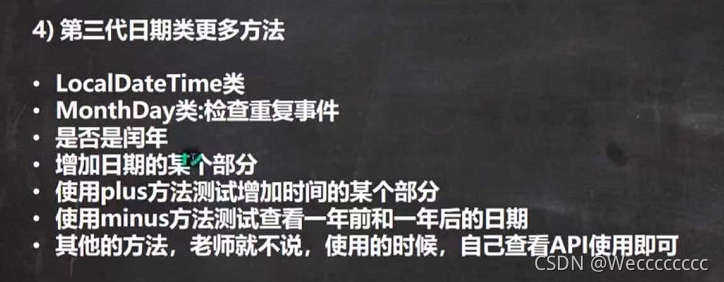
01:
package DateDemo01;
import java.time.Instant;
import java.util.Date;
public class InstantDemo01 {
public static void main(String[] args) {
Instant now = Instant.now();
System.out.println(now);
Date date = Date.from(now);
Instant instant = date.toInstant();
}
}
01:
package DateDemo01;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateDemo {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
DateTimeFormatter dateTimeFormatter = DateTimeFormatter.ofPattern("yyyy年MM月dd日 HH小时mm分钟ss秒");
LocalDateTime plus = now.plusDays(890);
System.out.println(dateTimeFormatter.format(plus));
LocalDateTime localDateTime = now.minusMinutes(3456);
System.out.println(dateTimeFormatter.format(localDateTime));
}
}
小练习

01:
package HomeWorkD01;
public class HomeWork01 {
public static void main(String[] args) {
String str = "abcdef";
try {
str = reverse(str, 1, 4);
} catch (Exception e) {
System.out.println(e.getMessage());
return;
}
System.out.println(str);
}
public static String reverse(String str,int start,int end)
{
if (!(str!=null && start >= 0 && end > start && end <str.length()))
{
throw new RuntimeException("参数不正确");
}
char[] chars = str.toCharArray();
char temp = ' ';
for (int i = start,j = end;i<j;i++,j--)
{
temp = chars[i];
chars[i] = chars[j];
chars[j] = temp;
}
return new String(chars);
}
}
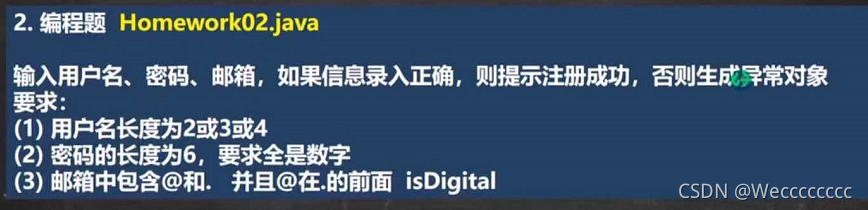
02:
package HomeWorkD01;
public class HomeWork02 {
public static void main(String[] args) {
String name = "tom";
String pwd = "123456";
String email = "tom@shouhu.com";
try {
userRegister(name,pwd,email);
System.out.println("OK");
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
public static void userRegister(String name,String pwd,String email)
{
if (!(name!=null && pwd !=null && email !=null))
{
throw new RuntimeException("Don't NULL");
}
int userLength = name.length();
if (!(userLength >= 2 && userLength <= 4))
{
throw new RuntimeException("username length should be between 2 to 4");
}
if (!(pwd.length()==6 && isDigital(pwd)))
{
throw new RuntimeException("Your password length should be equals to 6");
}
int i = email.indexOf('@');
int j = email.indexOf('.');
if ((i > 0 && j > i))
{
throw new RuntimeException("Error");
}
}
public static boolean isDigital(String str)
{
char[] chars = str.toCharArray();
for (int i = 0;i<chars.length;i++)
{
if (chars[i] < '0' || chars[i] > '9')
{
return false;
}
}
return true;
}
}

03:
package HomeWorkD01;
import java.util.Locale;
public class HomeWork03 {
public static void main(String[] args) {
String name = "Han shun Ping";
printName(name);
}
public static void printName(String str)
{
if (str==null)
{
System.out.println("str don't null");
return;
}
String[] names = str.split(" ");
if (names.length!=3)
{
System.out.println("input format is error");
return ;
}
String format = String.format("%s,%s.%c", names[2], names[0], names[1].toUpperCase().charAt(0));
System.out.println(format);
}
}

04:
package HomeWorkD01;
public class HomeWork04 {
public static void main(String[] args) {
String str = "absaf 678787 asda HUKJANJKDjkj";
countStr(str);
}
public static void countStr(String str)
{
if (str==null)
{
System.out.println("input error");
return ;
}
int numCnt = 0;
int lowerCnt = 0;
int upperCnt = 0;
int otherCnt = 0;
int strlen = str.length();
for (int i = 0;i<strlen;i++)
{
if (str.charAt(i) >= '0' && str.charAt(i) <='9')
{
numCnt++;
}
else if (str.charAt(i)>='a' && str.charAt(i) <='z')
{
lowerCnt++;
}
else if (str.charAt(i) >= 'A' && str.charAt(i) <='Z')
{
upperCnt++;
}
else
{
otherCnt++;
}
}
System.out.println(numCnt+" "+lowerCnt+" "+upperCnt);
}
}
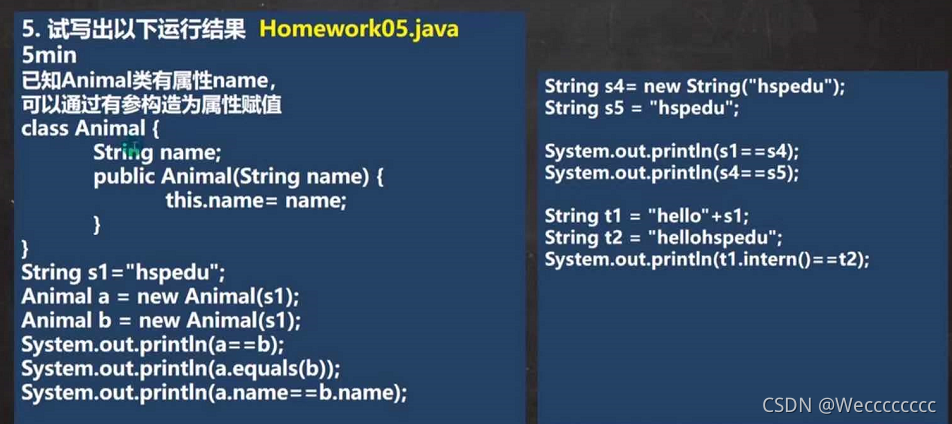
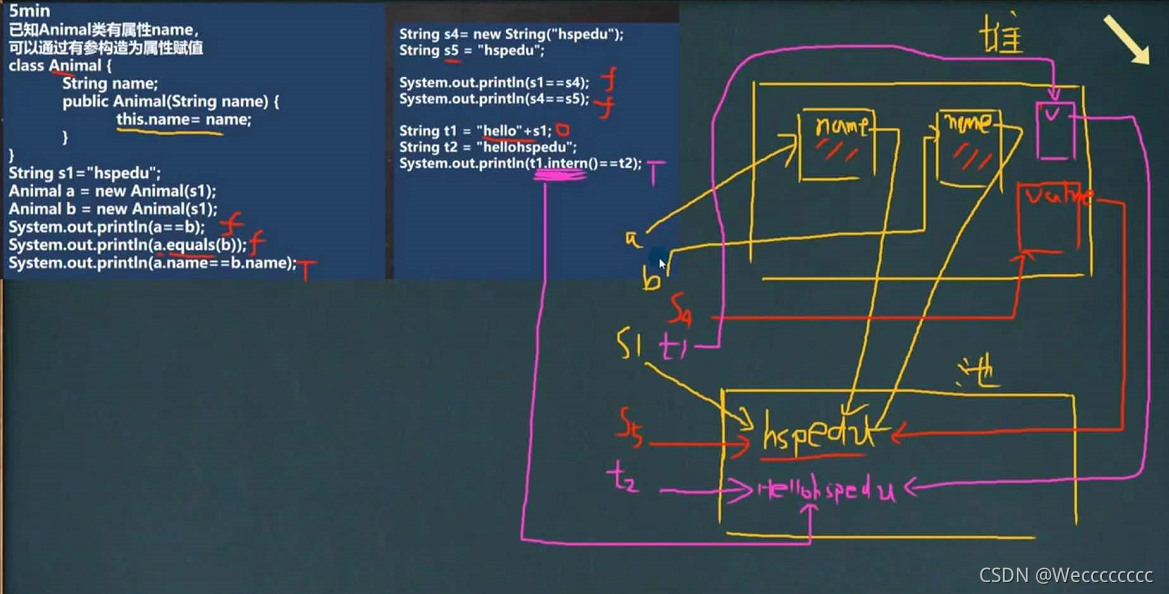
|