内容列表: 快速开始一个MyBatis 基本CURD的操作 MyBatis内部对象分析 使用Dao对象
入门案例
搭建MyBatis开发环境,实现第一个案例。
使用MyBatis准备
下载MyBatis
https://github.com/mybatis/mybatis-3/releases
搭建MyBatis开发环境
创建mysql数据库和表
数据库名 ssm ;表名 student 。  创建数据库
CREATE DATABASE `ssm`CHARACTER SET utf8 COLLATE utf8_general_ci;
创建数据表
CREATE TABLE `student` (
`id` int(11) NOT NULL ,
`name` varchar(255) DEFAULT NULL,
`email` varchar(255) DEFAULT NULL,
`age` int(11) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
手动插入一条数据,方便后续的查询操作 
创建maven工程
创建一个空项目
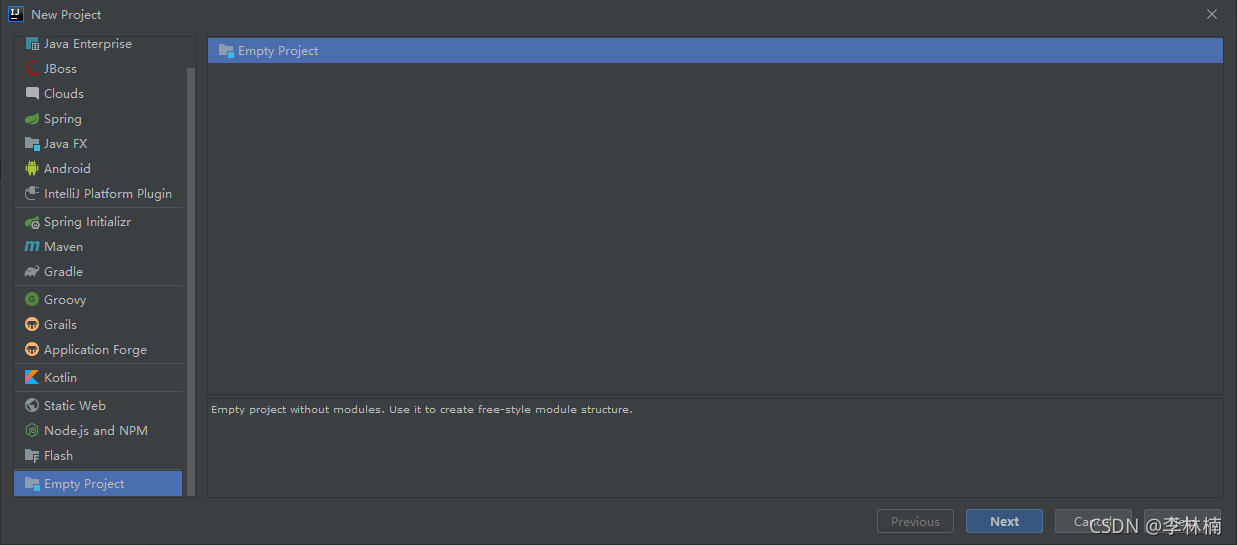 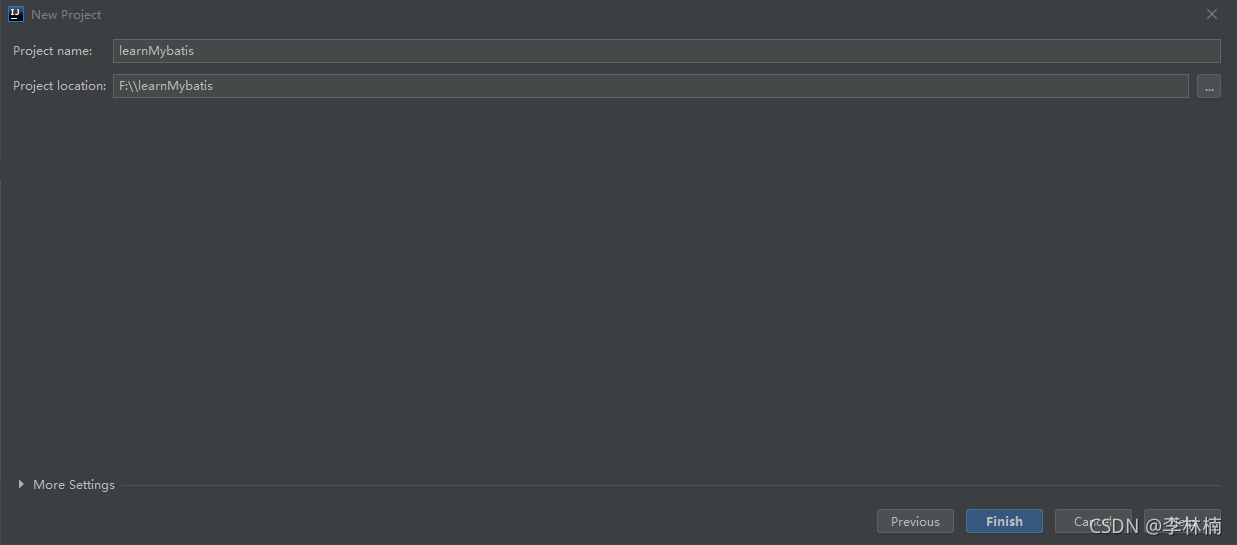
检查设置环境
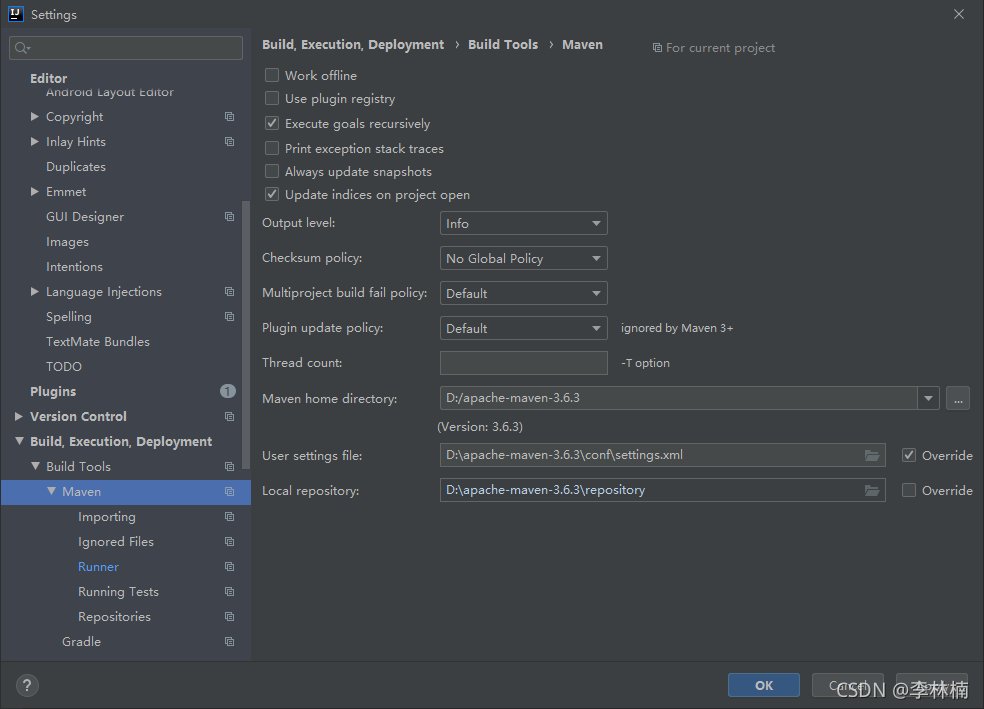 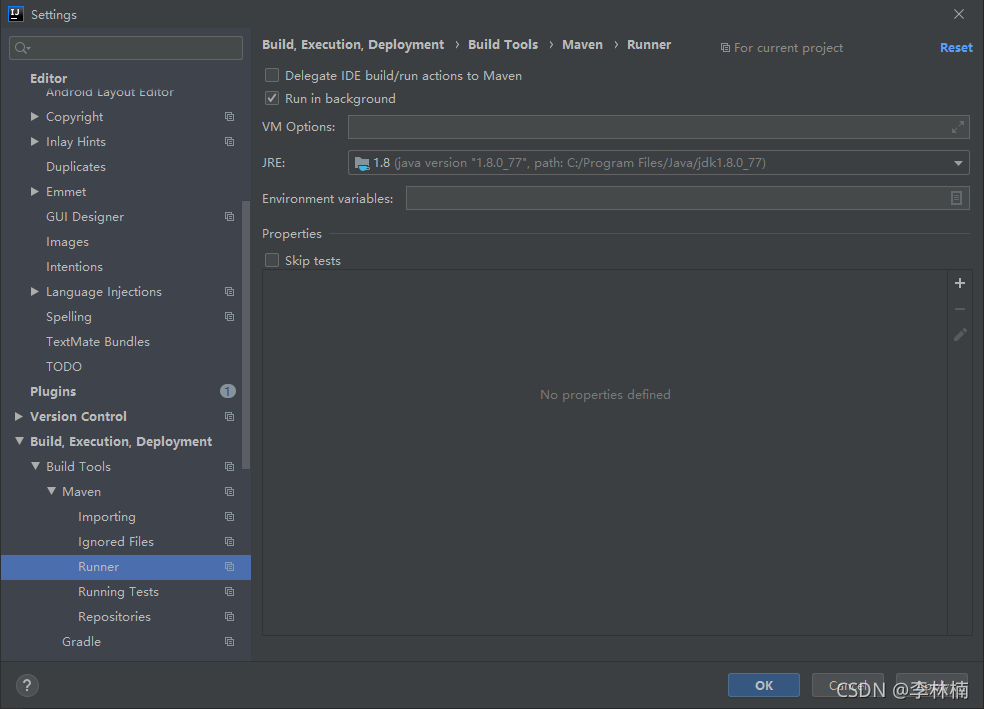
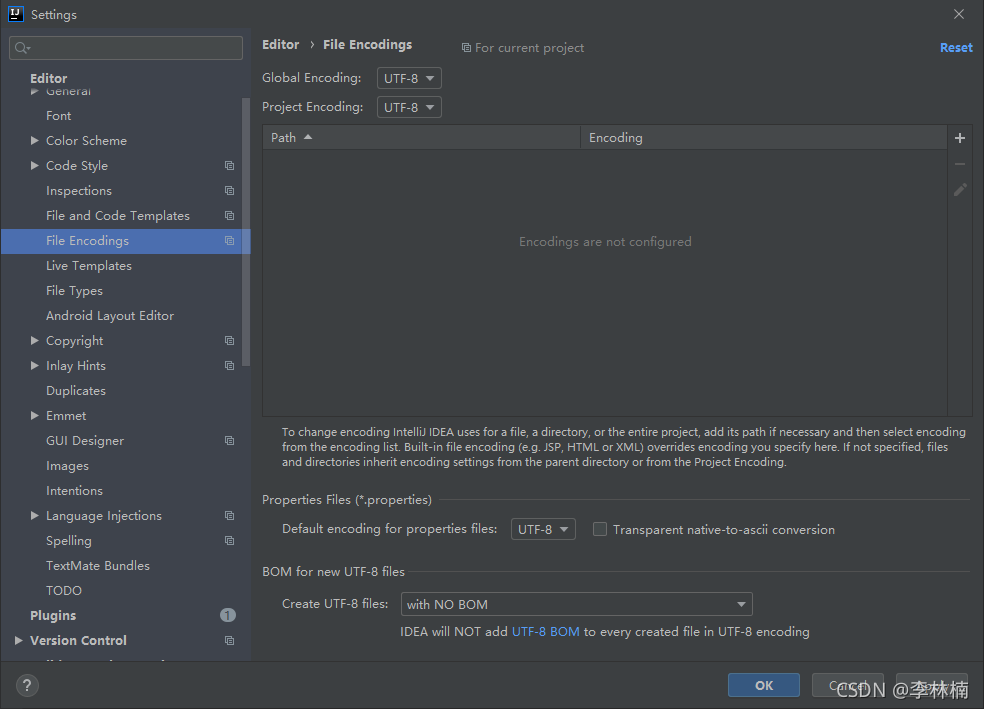
创建模块
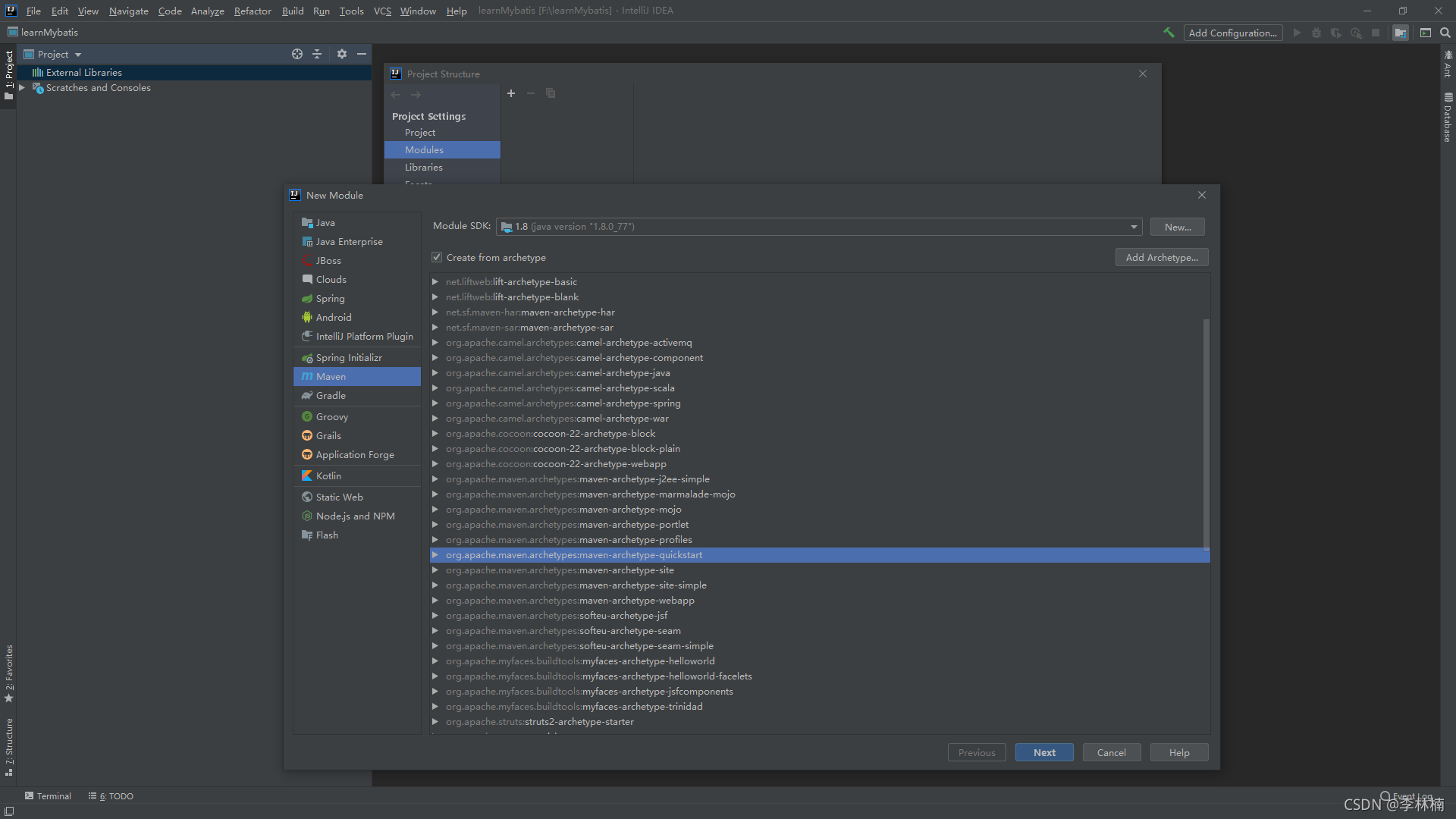 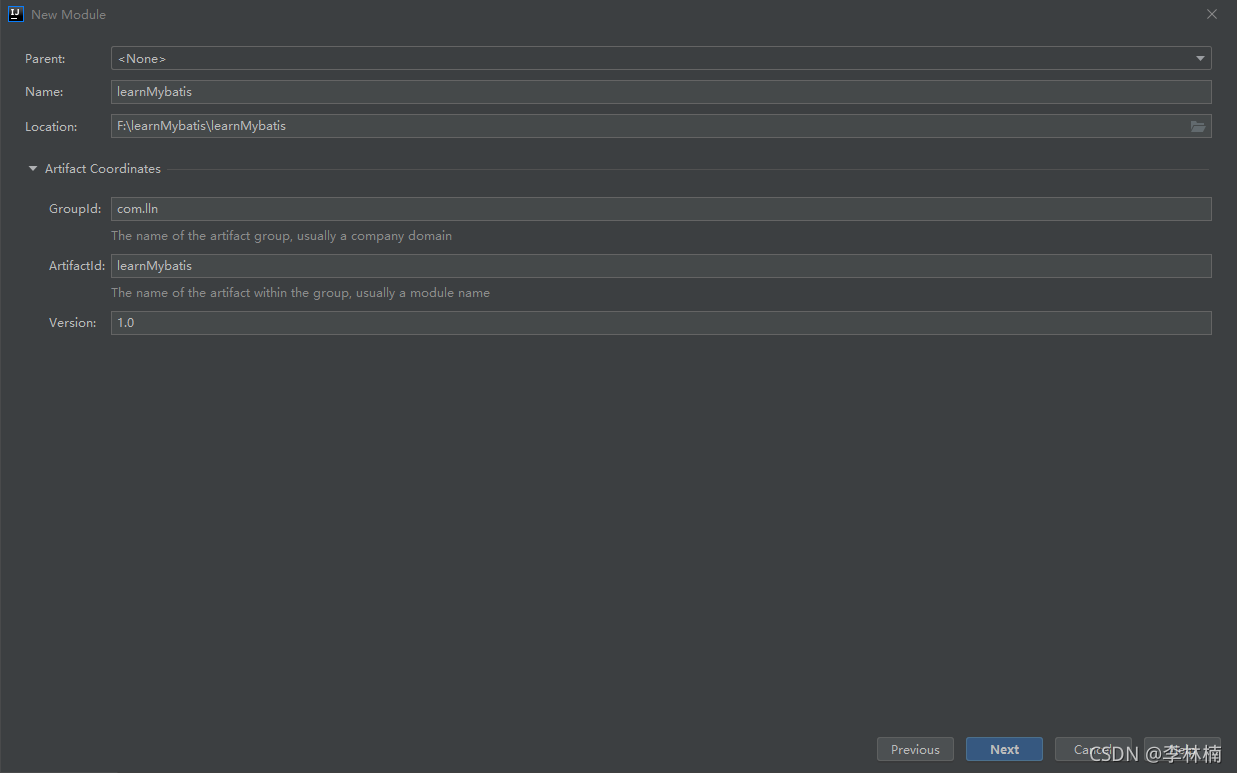 设置JDK和语言级别 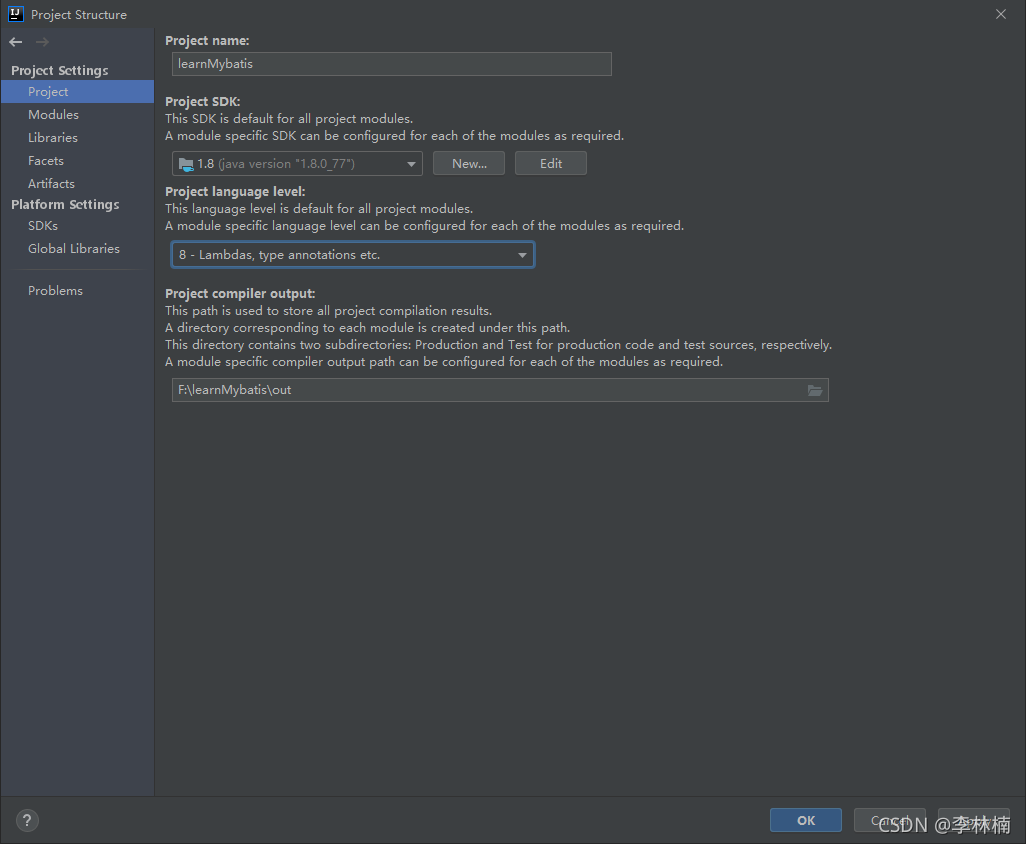
删除默认创建的App类文件
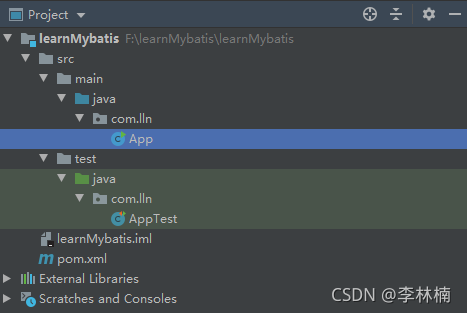
修改pom.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<!
<groupId>com.lln</groupId>
<artifactId>learnMybatis</artifactId>
<version>1.0</version>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<!
<dependencies>
<!
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.1</version>
</dependency>
<!
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.9</version>
</dependency>
<!
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<!
<resources>
<resource>
<directory>src/main/java</directory><!
<includes><!
<include>***.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
<!
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
创建Student实体类
package com.lln.vo;
public class Student {
private Integer id;
private String name;
private String email;
private Integer age;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "学生实体的信息:{" +
"id=" + id +
", name='" + name + '\'' +
", email='" + email + '\'' +
", age=" + age +
'}';
}
}
编写 Dao 接口 StudentDao 类
package com.lln.dao;
import com.lln.vo.Student;
public interface StudentDao {
Student selectStudentById(Integer id);
}
编写 Dao 接口 Mapper 映射文件
创建mapper文件,写sql语句,文件名:StudentDao.xml 目前与StudentDao接口类在同一目录下
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!
namespace:必须有值,自定义的唯一字符串
推荐使用:dao 接口的全限定名称
<mapper namespace="com.lln.dao.StudentDao">
<!
<select>: 表示查询数据, 标签中必须是 select 语句
id: 要执行的sql 语句的唯一标识,自定义名称,推荐使用 dao 接口中方法名称
resultType: 告诉mybatis,执行sql语句后,把数据赋值给哪个类型的java对象,即查询语句的返回结果数据类型,使用全限定类名
<select id="selectStudentById" resultType="com.lln.vo.Student">
<!
select id,name,email,age
from student
where id=1
<!
</select>
</mapper>
<!
1.约束文件
约束文件作用:定义和限制当前文件中可以使用的标签和属性,以及标签出现的顺序。
2.mapper是根标签
namespace:命名空间,必须有值,不能为空,唯一值。
推荐使用Dao接口的全限定名称。
作用:参与识别sql语句。
3.在mapper里面可以写<insert>,<update>,<delete>,<select>等标签
<insert>里面是insert语句,表示执行的是insert操作
创建 MyBatis 主配置文件
项目 src/main 下创建 resources 资源目录,设置 resources 目录名为 resources 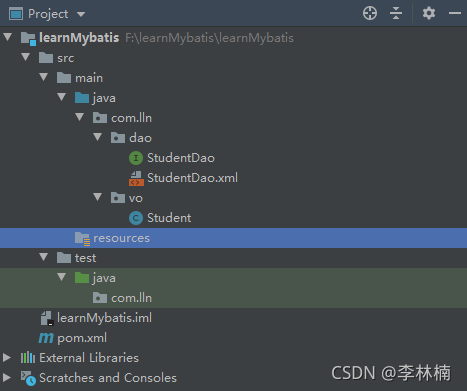 在resources目录下创建主配置文件(xml文件),名称为 mybatis.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!
<environments default="mysql">
<!
<environment id="mysql">
<!
<transactionManager type="JDBC"/>
<!
<dataSource type="POOLED">
<!
<!
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/ssm?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<!
指定其他mapper文件的位置
目的是找到其他文件中的sql语句
<mappers>
<!
使用mapper的resource属性指定mapper文件的路径
告诉 mybatis 要执行的 sql 语句的位置
使用注意:
resources="mapper文件的路径,使用/分割路径"
一个mapper resource 指定一个 mapper文件
<mapper resource="com/lln/dao/StudentDao.xml"/>
</mappers>
</configuration>
HTML字符实体
HTML中的预留字符必须被替换为字符实体。
创建测试类 MybatisTest
package com.lln;
import com.lln.vo.Student;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
public class MybatisTest {
@Test
public void testSelectStudentById() throws IOException {
String config = "mybatis.xml";
InputStream inputStream = Resources.getResourceAsStream(config);
SqlSessionFactory factory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession session = factory.openSession();
String sqlId = "com.lln.dao.StudentDao.selectStudentById";
Student student = session.selectOne(sqlId);
System.out.println("使用mybatis根据id查询一个学生:"+student);
session.close();
}
}
输出结果:
使用mybatis根据id查询一个学生:学生实体的信息:{id=1, name='张三', email='123@163.com', age=18}
初次使用占位符
修改sql语句
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!
namespace:必须有值,自定义的唯一字符串
推荐使用:dao 接口的全限定名称
<mapper namespace="com.lln.dao.StudentDao">
<!
<select>: 表示查询数据, 标签中必须是 select 语句
id: 要执行的sql 语句的唯一标识,自定义名称,推荐使用 dao 接口中方法名称
resultType: 告诉mybatis,执行sql语句后,把数据赋值给哪个类型的java对象,即查询语句的返回结果数据类型,使用全限定类名
<select id="selectStudentById" resultType="com.lln.vo.Student">
<!
select id,name,email,age
from student
where id=
<!
<!
</select>
</mapper>
<!
1.约束文件
约束文件作用:定义和限制当前文件中可以使用的标签和属性,以及标签出现的顺序。
2.mapper是根标签
namespace:命名空间,必须有值,不能为空,唯一值。
推荐使用Dao接口的全限定名称。
作用:参与识别sql语句。
3.在mapper里面可以写<insert>,<update>,<delete>,<select>等标签
<insert>里面是insert语句,表示执行的是insert操作
修改测试语句
package com.lln;
import com.lln.vo.Student;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
public class MybatisTest {
@Test
public void testSelectStudentById() throws IOException {
String config = "mybatis.xml";
InputStream inputStream = Resources.getResourceAsStream(config);
SqlSessionFactory factory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession session = factory.openSession();
String sqlId = "com.lln.dao.StudentDao.selectStudentById";
Student student = session.selectOne(sqlId,1);
System.out.println("使用mybatis根据id查询一个学生:"+student);
session.close();
}
}
配置日志功能
mybatis.xml主配置文件中添加setting设置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!
<settings>
<setting name="logImpl" value="STDOUT_LOGGING" />
</settings>
<!
<environments default="mysql">
<!
<environment id="mysql">
<!
<transactionManager type="JDBC"/>
<!
type: POOLED 使用数据库的连接池
<dataSource type="POOLED">
<!
<!
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/ssm?useUnicode=true&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<!
指定其他mapper文件的位置
目的是找到其他文件中的sql语句
<mappers>
<!
使用mapper的resource属性指定mapper文件的路径
告诉 mybatis 要执行的 sql 语句的位置
使用注意:
resources="mapper文件的路径,使用/分割路径"
一个mapper resource 指定一个 mapper文件
<mapper resource="com/lln/dao/StudentDao.xml"/>
</mappers>
</configuration>
再次执行查询测试,输出结果:
Logging initialized using 'class org.apache.ibatis.logging.stdout.StdOutImpl' adapter.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
Opening JDBC Connection
Created connection 1131316523.
Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@436e852b]
==> Preparing: select id,name,email,age from student where id=?
==> Parameters: 1(Integer)
<== Columns: id, name, email, age
<== Row: 1, 张三, 123@163.com, 18
<== Total: 1
使用mybatis根据id查询一个学生:学生实体的信息:{id=1, name='张三', email='123@163.com', age=18}
Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@436e852b]
Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@436e852b]
Returned connection 1131316523 to pool.
Process finished with exit code 0
提交事务
1.自动提交:当sql语句执行完毕后,自动提交事务,数据库更新操作直接保存到数据库。
2.手动提交:在需要提交事务的位置,执行方法,提交事务或者回滚事务。
3.mybatis默认执行sql语句是手动提交事务,在insert,update,delete后需要提交事务
添加数据
接口类添加接口:
package com.lln.dao;
import com.lln.vo.Student;
public interface StudentDao {
Student selectStudentById(Integer id);
int insertStudent(Student student);
}
添加sql语句(mapper映射文件)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!
namespace:必须有值,自定义的唯一字符串
推荐使用:dao 接口的全限定名称
<mapper namespace="com.lln.dao.StudentDao">
<!
<select>: 表示查询数据, 标签中必须是 select 语句
id: 要执行的sql 语句的唯一标识,自定义名称,推荐使用 dao 接口中方法名称
resultType: 告诉mybatis,执行sql语句后,把数据赋值给哪个类型的java对象,即查询语句的返回结果数据类型,使用全限定类名
<select id="selectStudentById" resultType="com.lln.vo.Student">
<!
select id,name,email,age
from student
where id=
<!
</select>
<!
<insert id="insertStudent">
insert into student values(2,"李四","456@163.com",26)
</insert>
</mapper>
<!
1.约束文件
约束文件作用:定义和限制当前文件中可以使用的标签和属性,以及标签出现的顺序。
2.mapper是根标签
namespace:命名空间,必须有值,不能为空,唯一值。
推荐使用Dao接口的全限定名称。
作用:参与识别sql语句。
3.在mapper里面可以写<insert>,<update>,<delete>,<select>等标签
<insert>里面是insert语句,表示执行的是insert操作
测试类:
package com.lln;
import com.lln.vo.Student;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
public class MybatisTest {
@Test
public void testSelectStudentById() throws IOException {
String config = "mybatis.xml";
InputStream inputStream = Resources.getResourceAsStream(config);
SqlSessionFactory factory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession session = factory.openSession();
String sqlId = "com.lln.dao.StudentDao.selectStudentById";
Student student = session.selectOne(sqlId,1);
System.out.println("使用mybatis根据id查询一个学生:"+student);
session.close();
}
@Test
public void testInsertStudent() throws IOException {
String config = "mybatis.xml";
InputStream inputStream = Resources.getResourceAsStream(config);
SqlSessionFactory factory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession session = factory.openSession();
String sqlId = "com.lln.dao.StudentDao.insertStudent";
int rows = session.insert(sqlId);
session.commit();
System.out.println("使用mybatis添加一个学生,影响行数rows="+rows);
session.close();
}
}
更新数据库查看添加结果:添加成功! 
使用占位符添加数据
修改sql语句(mapper映射文件:StudentDao.xml)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!
namespace:必须有值,自定义的唯一字符串
推荐使用:dao 接口的全限定名称
<mapper namespace="com.lln.dao.StudentDao">
<!
<select>: 表示查询数据, 标签中必须是 select 语句
id: 要执行的sql 语句的唯一标识,自定义名称,推荐使用 dao 接口中方法名称
resultType: 告诉mybatis,执行sql语句后,把数据赋值给哪个类型的java对象,即查询语句的返回结果数据类型,使用全限定类名
<select id="selectStudentById" resultType="com.lln.vo.Student">
<!
select id,name,email,age
from student
where id=
<!
</select>
<!
<!
如果传给mybatis的是一个对象,使用
属性名放到
<insert id="insertStudent">
insert into student values(
</insert>
</mapper>
<!
1.约束文件
约束文件作用:定义和限制当前文件中可以使用的标签和属性,以及标签出现的顺序。
2.mapper是根标签
namespace:命名空间,必须有值,不能为空,唯一值。
推荐使用Dao接口的全限定名称。
作用:参与识别sql语句。
3.在mapper里面可以写<insert>,<update>,<delete>,<select>等标签
<insert>里面是insert语句,表示执行的是insert操作
修改测试类
package com.lln;
import com.lln.vo.Student;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
public class MybatisTest {
@Test
public void testSelectStudentById() throws IOException {
String config = "mybatis.xml";
InputStream inputStream = Resources.getResourceAsStream(config);
SqlSessionFactory factory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession session = factory.openSession();
String sqlId = "com.lln.dao.StudentDao.selectStudentById";
Student student = session.selectOne(sqlId,1);
System.out.println("使用mybatis根据id查询一个学生:"+student);
session.close();
}
@Test
public void testInsertStudent() throws IOException {
String config = "mybatis.xml";
InputStream inputStream = Resources.getResourceAsStream(config);
SqlSessionFactory factory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession session = factory.openSession();
String sqlId = "com.lln.dao.StudentDao.insertStudent";
Student student = new Student();
student.setId(3);
student.setName("王五");
student.setEmail("789@163.com");
student.setAge(30);
int rows = session.insert(sqlId,student);
session.commit();
System.out.println("使用mybatis添加一个学生,影响行数rows="+rows);
session.close();
}
}
运行结果:
Logging initialized using 'class org.apache.ibatis.logging.stdout.StdOutImpl' adapter.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
Opening JDBC Connection
Created connection 205962452.
Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@c46bcd4]
==> Preparing: insert into student values(?,?,?,?)
==> Parameters: 3(Integer), 王五(String), 789@163.com(String), 30(Integer)
<== Updates: 1
Committing JDBC Connection [com.mysql.jdbc.JDBC4Connection@c46bcd4]
使用mybatis添加一个学生,影响行数rows=1
Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@c46bcd4]
Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@c46bcd4]
Returned connection 205962452 to pool.
更新数据库查看添加结果:添加成功! 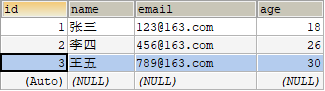
|