新建文件file.html
用jquery需要引入路径
<script src="js/jquery-2.1.0.js"></script>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<script src="view/js/jquery-2.1.0.js"></script> <!-- 需要写全路径 -->
<body>
<form id="form">
<input type="file" name="uplaod">
<button onclick="tijioa()">提交</button>
</form>
</body>
<script type="text/javascript">
function tijiao(){
//创建formData对象 form0表示的是获取第一个form表单
var formData = new FormData($("form")[0]);
$.ajax({
type:"post",
url:"ssh_sign0506/dict!upload",
data:formData,
processData : false,
contentType : false,
dataType:"json",
success : function(data) {
}
});
}
</script>
</html>
在DictController类中添加文件上传的方法
上服务器中取一个请求路径:先获取服务器下的路径——》在工程下创建一个upload文件夹
通过接收到的文件——》在服务器下创建文件文件名:uploadFileName
?复制,把传过来的文件复制到dest上:
在后台写三行就能完成文件的上传
给这两个属性加get、set方法
//在WebContent下新建一个upload的文件夹,获取其在服务器的绝对磁盘路径
String path = ServletActionContext.getServletContext().getRealPath("/upload");
//创建一个服务器端的文件
File dest = new File(path,uploadFileName);
//完成文件上传的操作
FileUtils.copyFile(upload, dest); //将upload文件复制到路径下 有异常先抛出
需要有两个属性:uploadFileName、upload
private File upload;//定义一个File ,变量名要与jsp中的input标签的name一致
private String uploadFileName;//上传文件的名称
这里的private File upload;要和file.html中<input type="file" name="upload">?里的name一致
现在一个基本的文件上传功能就做完了。
在pojo包下创建一个ExcelEntity.java文件,
ExcelEntity.java中的属性就是需要读取表格的字段
package com.hxci.pojo;
import com.alibaba.excel.annotation.ExcelProperty;
import com.alibaba.excel.metadata.BaseRowModel;
public class ExcelEntity extends BaseRowModel {
private String id;
@ExcelProperty(index = 1 , value = "开课院系部")
private String yuanxi;
@ExcelProperty(index = 2 , value = "开课专业")
private String zhuanye;
@ExcelProperty(index = 3 , value = "课程代码")
private String daima;
@ExcelProperty(index = 4 , value = "课程名称")
private String mingcheng;
@ExcelProperty(index = 5 , value = "考试、考查")
private String xingzhi;
@ExcelProperty(index = 6 , value = "考核形式")
private String xingshi;
@ExcelProperty(index = 7 , value = "开课班级")
private String banji;
@ExcelProperty(index = 8 , value = "人数")
private String renshu;
@ExcelProperty(index = 9 , value = "任课教师")
private String jiaoshi;
@ExcelProperty(index = 10 , value = "职称")
private String zhicheng;
@ExcelProperty(index = 11 , value = "专兼职")
private String zhuanjian;
@ExcelProperty(index = 12 , value = "周学时")
private String xueshi;
@ExcelProperty(index = 13 , value = "教学周数")
private String zhoushu;
@ExcelProperty(index = 14 , value = "总学时")
private String zonngxs;
@ExcelProperty(index = 15 , value = "备注")
private String beizhu;
public String getYuanxi() {
return yuanxi;
}
public void setYuanxi(String yuanxi) {
this.yuanxi = yuanxi;
}
public String getZhuanye() {
return zhuanye;
}
public void setZhuanye(String zhuanye) {
this.zhuanye = zhuanye;
}
public String getDaima() {
return daima;
}
public void setDaima(String daima) {
this.daima = daima;
}
public String getMingcheng() {
return mingcheng;
}
public void setMingcheng(String mingcheng) {
this.mingcheng = mingcheng;
}
public String getXingzhi() {
return xingzhi;
}
public void setXingzhi(String xingzhi) {
this.xingzhi = xingzhi;
}
public String getXingshi() {
return xingshi;
}
public void setXingshi(String xingshi) {
this.xingshi = xingshi;
}
public String getBanji() {
return banji;
}
public void setBanji(String banji) {
this.banji = banji;
}
public String getRenshu() {
return renshu;
}
public void setRenshu(String renshu) {
this.renshu = renshu;
}
public String getJiaoshi() {
return jiaoshi;
}
public void setJiaoshi(String jiaoshi) {
this.jiaoshi = jiaoshi;
}
public String getZhicheng() {
return zhicheng;
}
public void setZhicheng(String zhicheng) {
this.zhicheng = zhicheng;
}
public String getZhuanjian() {
return zhuanjian;
}
public void setZhuanjian(String zhuanjian) {
this.zhuanjian = zhuanjian;
}
public String getXueshi() {
return xueshi;
}
public void setXueshi(String xueshi) {
this.xueshi = xueshi;
}
public String getZhoushu() {
return zhoushu;
}
public void setZhoushu(String zhoushu) {
this.zhoushu = zhoushu;
}
public String getZonngxs() {
return zonngxs;
}
public void setZonngxs(String zonngxs) {
this.zonngxs = zonngxs;
}
public String getBeizhu() {
return beizhu;
}
public void setBeizhu(String beizhu) {
this.beizhu = beizhu;
}
@Override
public String toString() {
return "ExcelEntity [id=" + id + ", yuanxi=" + yuanxi
+ ", zhuanye=" + zhuanye + ", daima=" + daima + ", mingcheng="
+ mingcheng + ", xingzhi=" + xingzhi + ", xingshi=" + xingshi
+ ", banji=" + banji + ", renshu=" + renshu + ", jiaoshi="
+ jiaoshi + ", zhicheng=" + zhicheng + ", zhuanjian="
+ zhuanjian + ", xueshi=" + xueshi + ", zhoushu=" + zhoushu
+ ", zonngxs=" + zonngxs + ", beizhu=" + beizhu + "]";
}
}
在util包下
package com.hxci.util;
import com.alibaba.excel.EasyExcelFactory;
import com.alibaba.excel.ExcelReader;
import com.alibaba.excel.ExcelWriter;
import com.alibaba.excel.event.AnalysisEventListener;
import com.alibaba.excel.metadata.BaseRowModel;
import com.alibaba.excel.metadata.Sheet;
import com.alibaba.excel.read.metadata.ReadSheet;
import com.alibaba.excel.support.ExcelTypeEnum;
import com.hxci.pojo.ExcelEntity;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
public class ExcelUtils {
/**
* @param is 导入文件输入流
* @param clazz Excel实体映射类
* @return
*/
public static Boolean readExcel(InputStream is, Class clazz){
BufferedInputStream bis = null;
try {
bis = new BufferedInputStream(is);
// 解析每行结果在listener中处理
AnalysisEventListener listener = new ExcelListener();
ExcelReader excelReader = EasyExcelFactory.getReader(bis, listener);
//sheets代表怎样获取表格中的sheet页
List<ReadSheet> sheets = excelReader.excelExecutor().sheetList();
//3代表从第三行往下读 循环读
for (ReadSheet sheet : sheets) {
excelReader.read(new Sheet(sheet.getSheetNo(), 3, clazz));
}
} catch (Exception e) {
e.printStackTrace();
return false;
} finally {
if (bis != null) {
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return true;
}
/**
*
* @param os 文件输出流
* @param clazz Excel实体映射类
* @param data 导出数据
* @return
*/
public static Boolean writeExcel(OutputStream os, Class clazz, List<? extends BaseRowModel> data){
BufferedOutputStream bos= null;
try {
bos = new BufferedOutputStream(os);
ExcelWriter writer = new ExcelWriter(bos, ExcelTypeEnum.XLSX);
//写第一个sheet, sheet1 数据全是List<String> 无模型映射关系
Sheet sheet1 = new Sheet(1, 0,clazz);
writer.write(data, sheet1);
writer.finish();
} catch (Exception e) {
e.printStackTrace();
return false;
} finally {
if (bos != null) {
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return true;
}
public static void main(String[] args) {
//1.读Excel
FileInputStream fis = null;
try {
fis = new FileInputStream("D:\\12.xlsx");
Boolean flag = ExcelUtils.readExcel(fis, ExcelEntity.class);
System.out.println("导入是否成功:"+flag);
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
if (fis != null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//2.读Excel
FileOutputStream fos = null;
try {
fos = new FileOutputStream("D:\\export.xlsx");
//FileOutputStream fos, Class clazz, List<? extends BaseRowModel> data
List<ExcelEntity> list = new ArrayList<>();
for (int i = 0; i < 5; i++){
ExcelEntity excelEntity = new ExcelEntity();
excelEntity.setJiaoshi("我是名字"+i);
list.add(excelEntity);
}
Boolean flag = ExcelUtils.writeExcel(fos,ExcelEntity.class,list);
System.out.println("导出是否成功:"+flag);
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
if (fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
在util包下需要有一个读取表格的方法继承后重写(对读取到的数据进行处理)
package com.hxci.util;
import com.alibaba.excel.context.AnalysisContext;
import com.alibaba.excel.event.AnalysisEventListener;
import com.hxci.pojo.ExcelEntity;
import java.util.ArrayList;
import java.util.List;
/** 解析监听器,
* 每解析一行会回调invoke()方法。
* 整个excel解析结束会执行doAfterAllAnalysed()方法
*
* 下面只是我写的一个样例而已,可以根据自己的逻辑修改该类。
*/
public class ExcelListener extends AnalysisEventListener {
//自定义用于暂时存储data。
//可以通过实例获取该值
private List<Object> datas = new ArrayList<Object>();
//invoke读取
public void invoke(Object object, AnalysisContext context) {
System.out.println("当前行:"+context.getCurrentRowNum());
System.out.println(object);
datas.add(object);//数据存储到list,供批量处理,或后续自己业务逻辑处理。
doSomething(object);//根据自己业务做处理
}
//doSomething读取后处理的结果如:打印,存入数据库
private void doSomething(Object object) {
ExcelEntity excel = (ExcelEntity) object;
//1、入库调用接口
}
public void doAfterAllAnalysed(AnalysisContext context) {
// datas.clear();//解析结束销毁不用的资源
}
public List<Object> getDatas() {
return datas;
}
public void setDatas(List<Object> datas) {
this.datas = datas;
}
}
把ExcelUtils中main()方法粘贴到DictController
public String upload(){
//在WebContent下新建一个upload的文件夹,获取其在服务器的绝对磁盘路径
String path = ServletActionContext.getServletContext().getRealPath("/upload");
//创建一个服务器端的文件
File dest = new File(path,uploadFileName);
try {
FileUtils.copyFile(upload, dest);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} //将upload文件复制到路径下
//1.读Excel
FileInputStream fis = null;
try {
fis = new FileInputStream("D:\biao\12.xlsx");
Boolean flag = ExcelUtils.readExcel(fis, ExcelEntity.class);
System.out.println("导入是否成功:"+flag);
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
if (fis != null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return null;
}
?修改蓝色和黄色保持一致
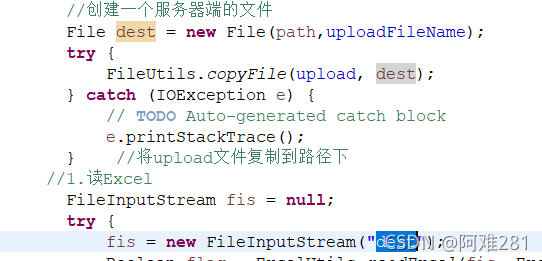
?
|