继学习简单的jdbc之后发现会有很多重复的代码,所以要抽离出来制作出一个jdbc工具类,并且要有一个properties文件更改jdbc的一些配置。
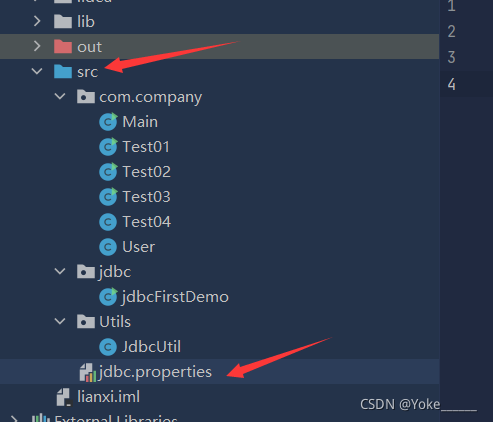
首先由一个配置文件,jdbc.properties
driver=com.mysql.jdbc.Driver
url=jdbc:mysql://localhost:3306/fisrt21?useUnicod=true&characterEncoding=utf8&useSSL=true
username=root
password=2020
?JdbcUtil类如下 ; 包含读取properties文件 和 创造connection对象并且断开连接。
package Utils;
import java.io.IOException;
import java.io.InputStream;
import java.sql.*;
import java.util.Properties;
/**
* @Author:XK
* @Date: Created in 22:33 2021/10/26
* @Description: jdbc工具类
**/
public class JdbcUtil {
private static String driver=null;
private static String url=null;
private static String username=null;
private static String password=null;
static {
try {
InputStream in = JdbcUtil.class.getClassLoader().getResourceAsStream("jdbc.properties");
Properties properties=new Properties();
properties.load(in);
//读取properties里的对应字段数据赋给变量
driver=properties.getProperty("driver");
url=properties.getProperty("url");
username=properties.getProperty("username");
password=properties.getProperty("password");
//驱动只需要加载一次
Class.forName(driver);
} catch (Exception e) {
e.printStackTrace();
}
}
//获取链接
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(url, username, password);
}
//释放连接资源
public static void relase(Connection co, Statement st, ResultSet rs){
if(rs!=null){
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (st != null) {
try {
st.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (co != null) {
try {
co.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
测试类 :
package com.company;
import Utils.JdbcUtil;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
/**
* @Author:XK
* @Date: Created in 17:22 2021/10/25
* 测试jdbc工具类
**/
public class Test02 {
public static void main(String[] args) {
Connection connection =null;
Statement statement=null;
ResultSet resultSet=null;
try {
connection = JdbcUtil.getConnection();//获得数据库对象
statement=connection.createStatement();//获得sql执行对象
String sql ="delete from class where id = 1";
//增删改都用update ,查询要用Query 由结果集来接
int i= statement.executeUpdate(sql);
if(i>0){
System.out.println("删除成功");
}
}catch (SQLException e){
e.printStackTrace();
}
}
}
简单的JDBC工具类就是如此
|