1. 线程的状态介绍
线程有以下五种状态:创建状态、就绪状态、阻塞状态、运行状态、死亡状态。
它们状态转化如下:
- 当 new 了一个线程后,线程就处于创建状态:
Thread t = new Thread(); 则 t 就处于创建状态; - 当线程调用了
start() 方法后,线程就处于就绪状态:t.start(); ,还不是运行状态; - 处于就绪状态的线程获取 CPU 资源后,被 CPU 调用,就会处于运行状态,此时,它会执行线程中的
run() 方法; - 当一个处于运行状态的线程调用了
sleep() 方法后,它就处于阻塞状态,等待再次被 CPU 调度; - 当线程的
run() 方法执行完毕后,线程就处于死亡状态了。此时,再次调用 start() 方法就会报错。
转换状态如下图: 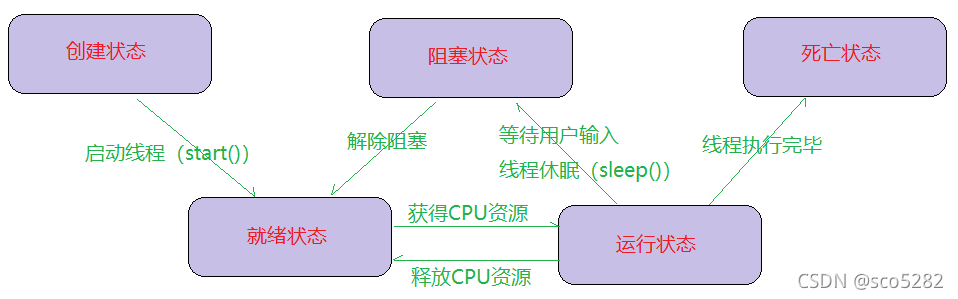
2. 线程中的方法
2.1 线程停止
停止线程时,不推荐使用 JDK 中的 stop() 、destory() 等方法,推荐使用标志位,让线程自己停下来。
public class TestStop implements Runnable{
private boolean flag = true;
@Override
public void run() {
int i = 0;
while (flag) {
System.out.println("run...thread" + i++);
}
}
public void stop() {
this.flag = false;
}
public static void main(String[] args) throws Exception {
TestStop testStop = new TestStop();
new Thread(testStop).start();
Thread.sleep(200);
for (int i = 0; i < 1000; i++) {
if (i == 900) {
testStop.stop();
System.out.println("线程停止了...");
}
}
}
}
2.2 线程休眠 sleep()
sleep(long) :表示当前线程线程阻塞的毫秒数,它会抛出 InterruptedException 异常;当睡眠时间到达后,线程就会自动处于就绪状态;sleep() 方法并不会释放对象的锁。
案例:模拟倒计时
public class TestSleep {
public static void tenDown() {
int num = 10;
while (true) {
if (num < 0) {
break;
}
try {
System.out.println(num--);
Thread.sleep(1000);
} catch (Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
tenDown();
}
}
2.3 线程礼让 yield()
yield() :礼让线程,让当前正在执行的线程暂停,但不阻塞(将线程从运行状态转换为就绪状态),但不一定成功,完全取决于 CPU。简单来说,就是让 CPU 重新调度线程。
public class TestYield {
public static void main(String[] args) {
MyYield myYield = new MyYield();
new Thread(myYield, "a").start();
new Thread(myYield, "b").start();
}
}
class MyYield implements Runnable{
@Override
public void run() {
System.out.println(Thread.currentThread().getName() + "线程开始执行");
Thread.yield();
System.out.println(Thread.currentThread().getName() + "线程执行结束");
}
}
运行结果如下:
这是线程礼让成功了。a 线程先执行,然后 b 线程又执行了 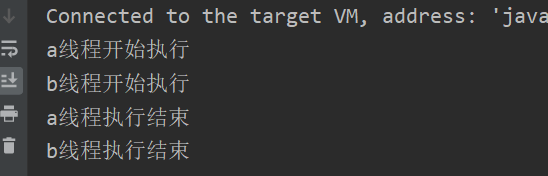
2.4 线程合并 join()
join():待此线程执行完后,再执行其它线程,其它线程处于阻塞状态。
2.5 线程状态转化
public class TestState {
public static void main(String[] args) {
Thread thread = new Thread(() -> {
for (int i = 0; i < 5; i++) {
try {
Thread.sleep(1000);
} catch (Exception e) {
e.printStackTrace();
}
}
System.out.println("/");
});
Thread.State state = thread.getState();
System.out.println(state);
thread.start();
state = thread.getState();
System.out.println(state);
while (state != Thread.State.TERMINATED) {
try {
Thread.sleep(100);
} catch (Exception e) {
e.printStackTrace();
}
state = thread.getState();
System.out.println(state);
}
}
}
3. 守护线程
线程分为:用户线程、守护线程(daemon)。虚拟机必须确保用户线程执行完毕,不用等待守护线程(后台记录操作日志、垃圾回收)执行完毕。
案例:
public class TestDaemon {
public static void main(String[] args) {
God god = new God();
You you = new You();
Thread thread = new Thread(god);
thread.setDaemon(true);
thread.start();
new Thread(you).start();
}
}
class God implements Runnable {
@Override
public void run() {
while (true) {
System.out.println("上帝一直保佑你!");
}
}
}
class You implements Runnable {
@Override
public void run() {
for (int i = 0; i < 365000; i++) {
System.out.println("你一生都开心地活着~~");
}
System.out.println("=====goodbye!world=====");
}
}
执行上述程序后,当线程 You 执行完一段时间后,main 线程也结束了,没有理会守护线程 God(它在一直运行着)。如果 God 不为守护线程,那么 main 线程将会一直执行。
|