一、题目描述(简述)
功能主要分为 四个部分: 1、用户的登录、注册 2、名片管理:增删改查名片,删除分为彻底删除或者放入到回收站中 3、回收站:对删除的名片进行还原或者删除 4、管理员:可以对所有名片和所有用户进行管理
二、使用技术
主要使用 jsp,css,js做的页面(做的很丑确实) java写代码 使用了 jdbc 连接数据库,servlet处理用户请求和响应,jsp前后台交互。 使用了mvc三层架构。
三、代码演示
1、java代码
结构: 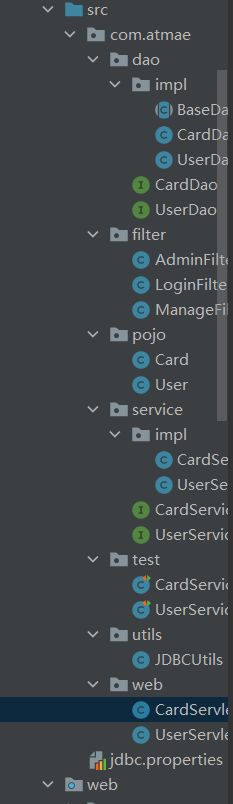
2、页面
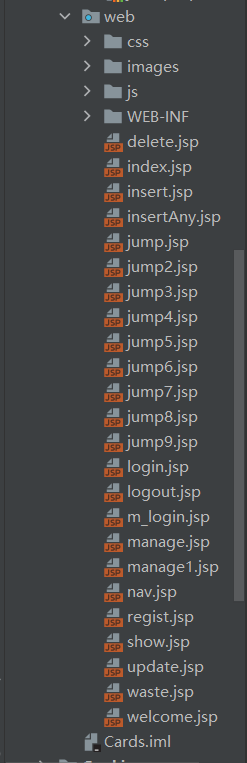
3、核心代码
package com.atmae.web;
import com.atmae.pojo.Card;
import com.atmae.pojo.User;
import com.atmae.service.CardService;
import com.atmae.service.UserService;
import com.atmae.service.impl.CardServiceImpl;
import com.atmae.service.impl.UserServiceImpl;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.nio.charset.StandardCharsets;
import java.util.List;
@WebServlet("/cardServlet")
public class CardServlet extends HttpServlet {
private CardService cardService = new CardServiceImpl();
private UserService userService = new UserServiceImpl();
protected void showAny(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
List<Card> cards = cardService.show();
req.setAttribute("cards", cards);
req.getRequestDispatcher("manage1.jsp").forward(req, resp);
}
protected void show(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String id = req.getParameter("id");
int id0 = Integer.parseInt(id);
Card card = cardService.showCardById(id0);
if (card == null) {
req.setAttribute("error", "未找到此id!");
} else {
req.setAttribute("card", card);
}
req.getRequestDispatcher("show.jsp").forward(req, resp);
}
protected void showDelete(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
HttpSession session = req.getSession();
User user = (User) session.getAttribute("user");
String username = user.getUsername();
List<Card> cards = cardService.showCardForDelete(username);
if (cards != null) {
req.setAttribute("cards", cards);
} else {
req.setAttribute("error", "回收站为空");
}
req.getRequestDispatcher("waste.jsp").forward(req, resp);
}
protected void showDeleteAdmin(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
List<Card> cards = cardService.showDeleteAdmin();
if (cards != null) {
req.setAttribute("cards", cards);
} else {
req.setAttribute("error", "回收站为空");
}
req.getRequestDispatcher("waste.jsp").forward(req, resp);
}
protected void showAll(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
HttpSession session = req.getSession();
User user = (User) session.getAttribute("user");
String username = user.getUsername();
List<Card> cards = cardService.showCards(username);
if (cards != null) {
req.setAttribute("cards", cards);
req.setAttribute("mulity", "mulity");
} else {
req.setAttribute("error", "当前用户没有名片");
}
req.getRequestDispatcher("show.jsp").forward(req, resp);
}
protected void updateName(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String name = req.getParameter("name");
name = new String(name.getBytes("ISO-8859-1"), "UTF-8");
String id = req.getParameter("id");
HttpSession session = req.getSession();
User user = (User) session.getAttribute("user");
int id0 = Integer.parseInt(id);
if (user.getUsername().equals(cardService.showCNameByID(id0)) && cardService.updateName(name, id0)) {
req.getRequestDispatcher("jump3.jsp").forward(req, resp);
} else {
req.setAttribute("error", "未找到此id号");
req.getRequestDispatcher("update.jsp").forward(req, resp);
}
}
protected void updateAccount(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String account = req.getParameter("account");
String id = req.getParameter("id");
HttpSession session = req.getSession();
User user = (User) session.getAttribute("user");
int id0 = Integer.parseInt(id);
if (user.getUsername().equals(cardService.showCNameByID(id0)) && cardService.updateAccount(account, id0)) {
req.getRequestDispatcher("jump3.jsp").forward(req, resp);
} else {
req.setAttribute("error", "未找到此id号");
req.getRequestDispatcher("update.jsp").forward(req, resp);
}
}
protected void delete(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String name = req.getParameter("name");
String prem = req.getParameter("prem");
int prem0 = Integer.parseInt(prem);
name = new String(name.getBytes("ISO-8859-1"), "UTF-8");
HttpSession session = req.getSession();
User user = (User) session.getAttribute("user");
if (prem0 == 1) {
if (user.getUsername().equals(cardService.showCNameByName(name)) && cardService.deleteRealByName(name) != -1) {
req.getRequestDispatcher("jump2.jsp").forward(req, resp);
} else {
req.setAttribute("error", "未找到此用户名");
req.getRequestDispatcher("delete.jsp").forward(req, resp);
}
} else {
if (user.getUsername().equals(cardService.showCNameByName(name)) && cardService.deleteCardByName(name)) {
req.getRequestDispatcher("jump2.jsp").forward(req, resp);
} else {
req.setAttribute("error", "未找到此用户名");
req.getRequestDispatcher("delete.jsp").forward(req, resp);
}
}
}
protected void deleteCard(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String id = req.getParameter("id");
String prem = req.getParameter("prem");
int prem0 = Integer.parseInt(prem);
int id0 = Integer.parseInt(id);
if (prem0 == 1) {
cardService.deleteCardByID(id0);
req.getRequestDispatcher("jump7.jsp").forward(req, resp);
} else {
cardService.deleteRealById(id0);
req.getRequestDispatcher("jump6.jsp").forward(req, resp);
}
}
protected void permanent(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String id = req.getParameter("id");
int id0 = Integer.parseInt(id);
cardService.deleteWaste(id0);
req.getRequestDispatcher("jump5.jsp").forward(req, resp);
}
protected void restore(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String id = req.getParameter("id");
int id0 = Integer.parseInt(id);
cardService.returnWaste(id0);
req.getRequestDispatcher("jump4.jsp").forward(req, resp);
}
protected void insert(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String name = req.getParameter("name");
String gender = req.getParameter("gender");
String account = req.getParameter("account");
String password = req.getParameter("password");
String email = req.getParameter("email");
HttpSession session = req.getSession();
User user = (User) session.getAttribute("user");
String username = user.getUsername();
name = new String(name.getBytes("ISO-8859-1"), "UTF-8");
gender = new String(gender.getBytes("ISO-8859-1"), "UTF-8");
Card card = new Card(name, gender, account, password, email, username);
cardService.insert(card);
req.getRequestDispatcher("jump.jsp").forward(req, resp);
}
protected void insertAny(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String name = req.getParameter("name");
String gender = req.getParameter("gender");
String account = req.getParameter("account");
String password = req.getParameter("password");
String email = req.getParameter("email");
String found = req.getParameter("found");
name = new String(name.getBytes("ISO-8859-1"), "UTF-8");
gender = new String(gender.getBytes("ISO-8859-1"), "UTF-8");
found = new String(found.getBytes("ISO-8859-1"), "UTF-8");
boolean flag = false;
List<User> users = userService.show();
for (User user : users) {
if (found.equals(user.getUsername())) {
flag = true;
break;
}
}
if (!flag) {
req.setAttribute("msg", "没有此用户,清重新输入");
req.getRequestDispatcher("insertAny.jsp").forward(req, resp);
} else {
cardService.insert(new Card(name, gender, account, password, email, found));
req.getRequestDispatcher("jump8.jsp").forward(req, resp);
}
}
protected void showUser(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
List<User> users = userService.show();
if (users == null) {
req.setAttribute("error", "当前没有用户信息");
req.getRequestDispatcher("manage1.jsp");
} else {
req.setAttribute("usersM", users);
req.getRequestDispatcher("manage1.jsp").forward(req, resp);
}
}
protected void deleteUser(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String name = req.getParameter("name");
name=new String(name.getBytes("ISO-8859-1"), "UTF-8");
userService.deleteUser(name);
cardService.deleteRealByUserName(name);
req.getRequestDispatcher("jump9.jsp").forward(req,resp);
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String action = req.getParameter("action");
try {
Method method = this.getClass().getDeclaredMethod(action, HttpServletRequest.class, HttpServletResponse.class);
method.invoke(this, req, resp);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String action = req.getParameter("action");
try {
Method method = this.getClass().getDeclaredMethod(action, HttpServletRequest.class, HttpServletResponse.class);
method.invoke(this, req, resp);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
}
}
}
四、功能演示
1、注册功能
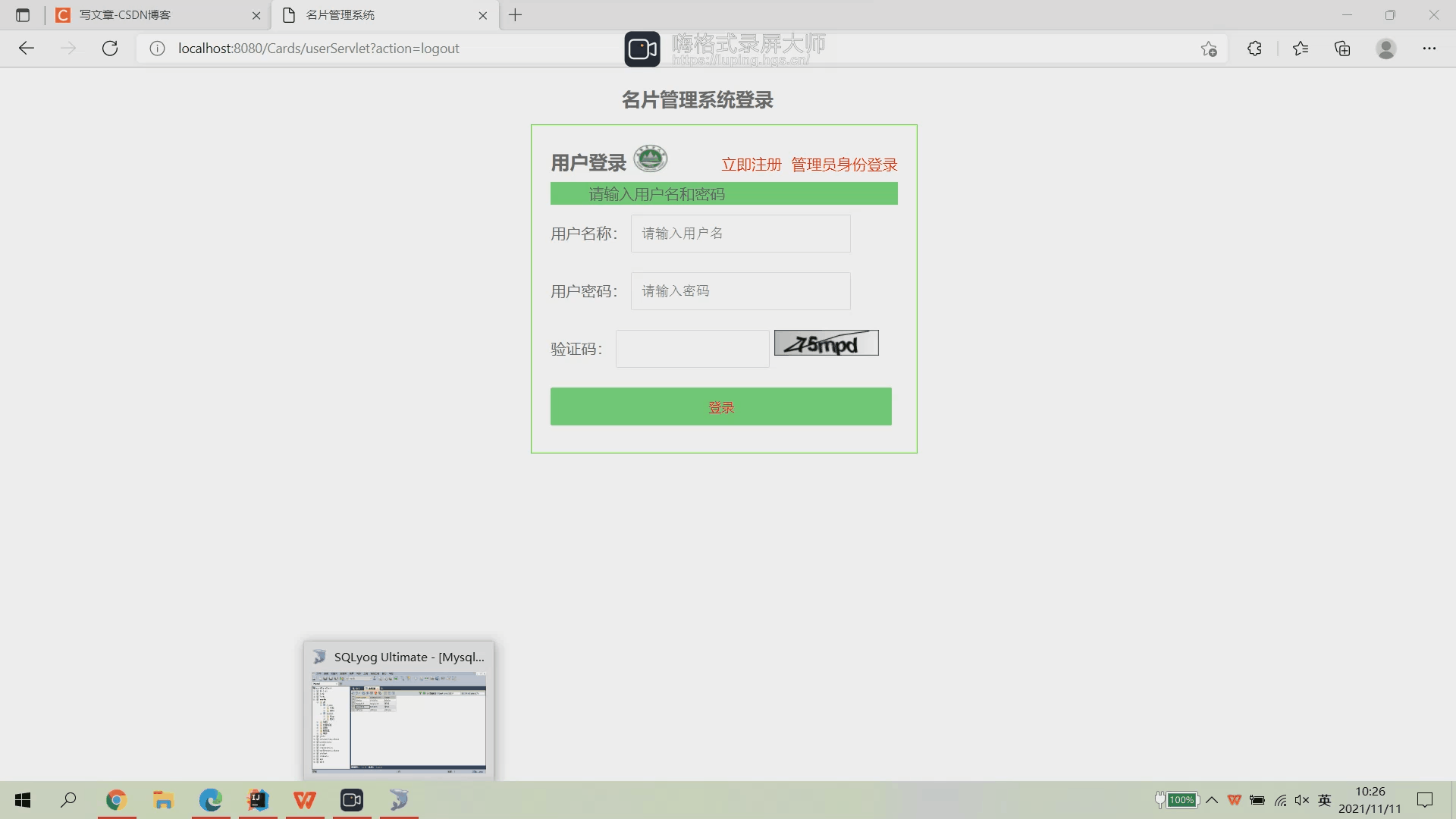
2、登录
普通用户+管理员登录
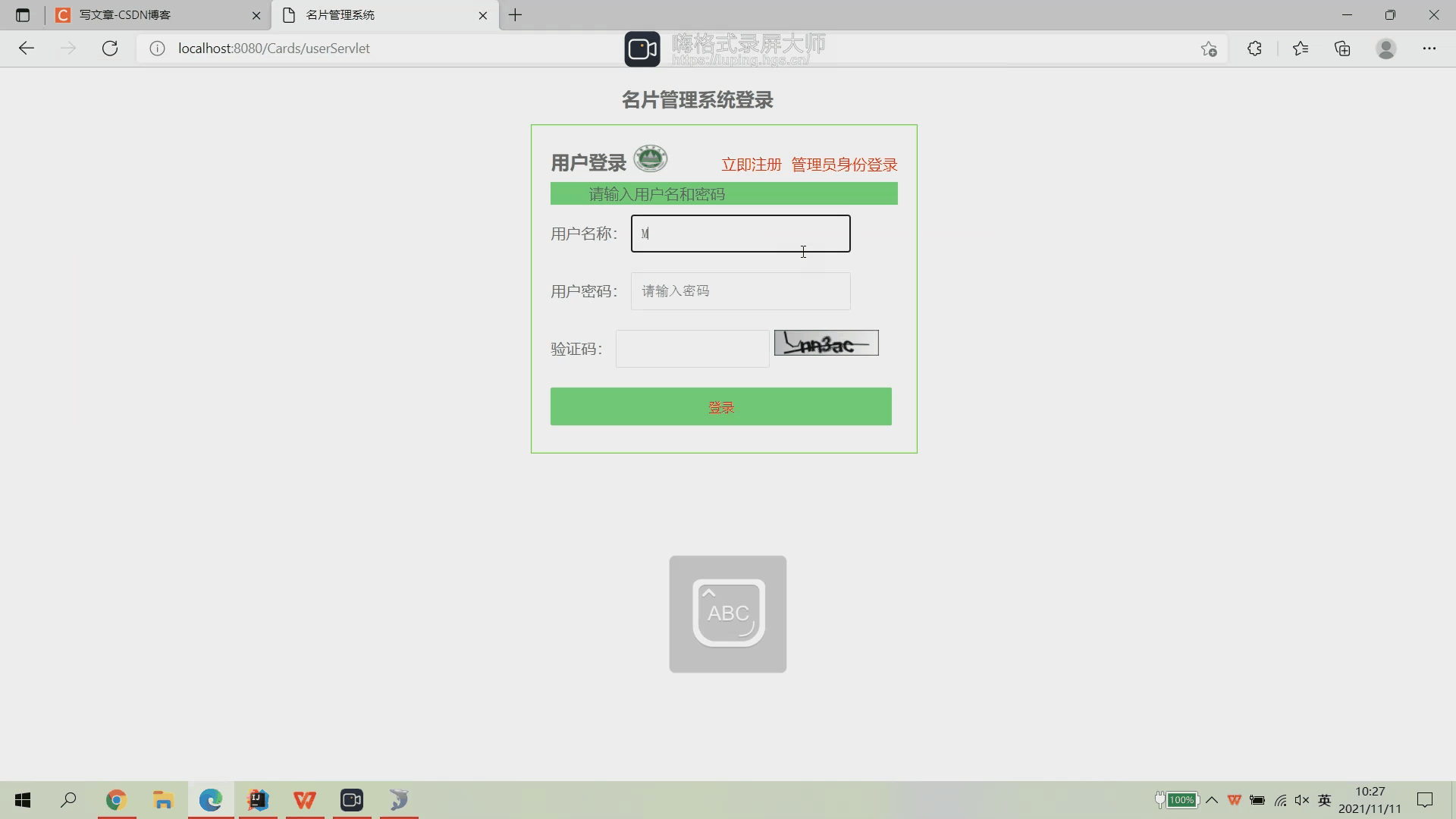
3、添加功能
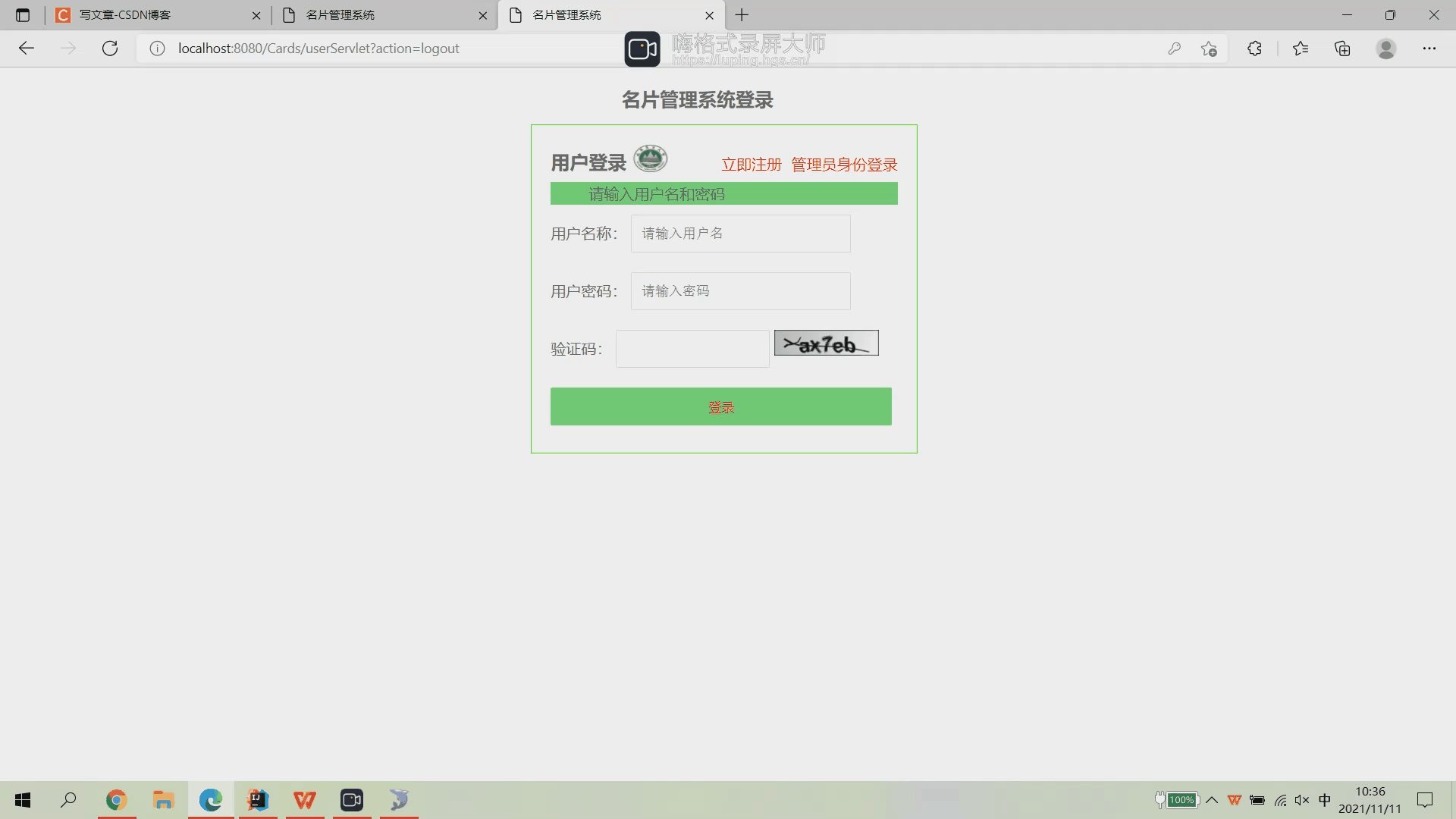
4、删除用户 显示 回收站功能
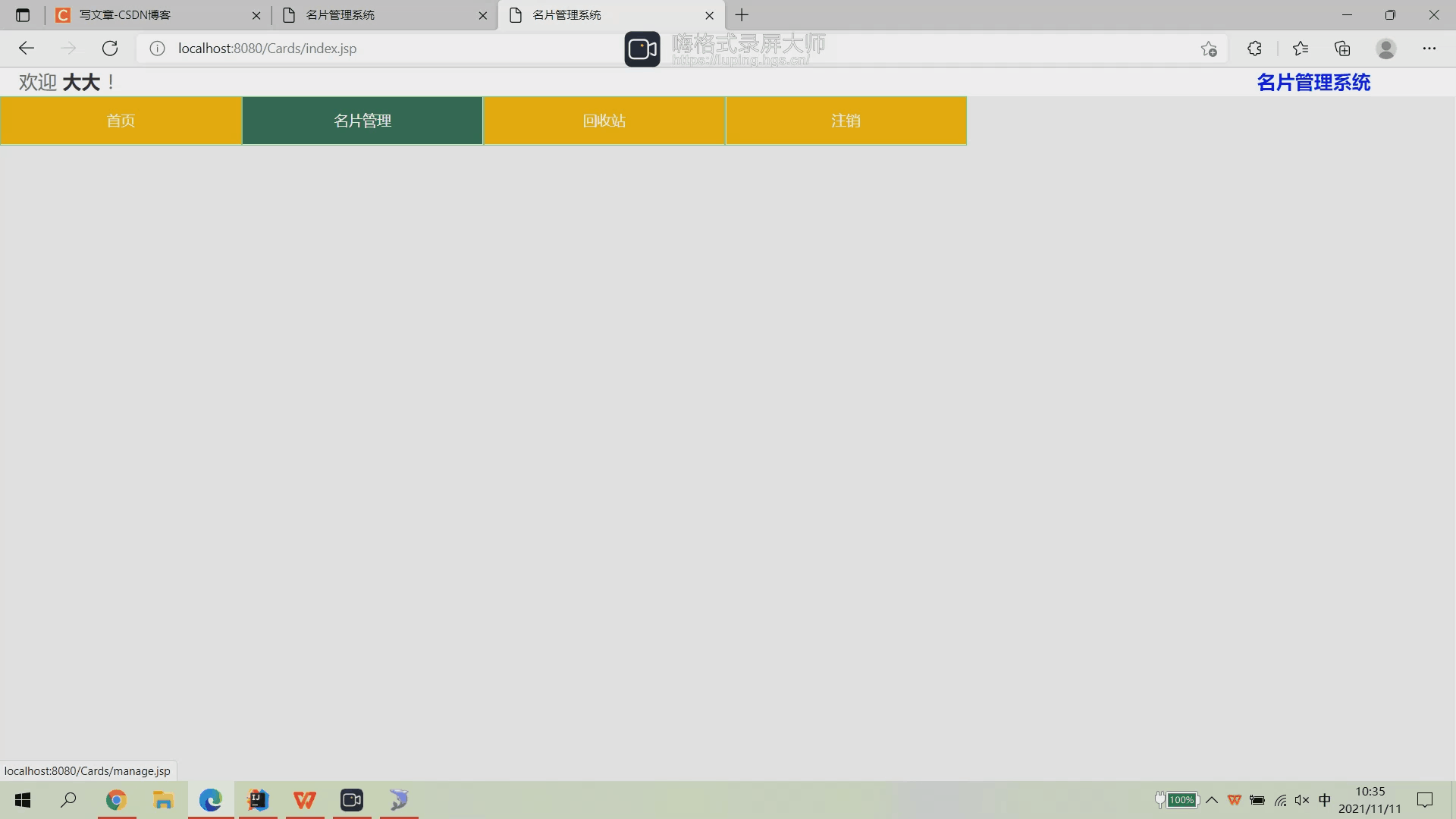
|