通道(Channel)基本介绍
- NIO 的通道类似于流,但有些区别:
- 通道可以同时进行读写,而流只能读或者只能写
- 通道可以实现异步读写数据
- 通道可以从缓冲读数据,也可以写数据到缓冲
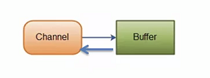
- BIO中的stream是单向的,例如FileInputStream对象只能进行读取数据的操作,而NIO中的通道(Channel)是双向的,可以读操作,也可以写操作
- Channel在NIO中是一个接口
public interface Channel extends Closeable{} - 常见的Channel类有:FileChannel、DatagramChannel、ServerSocketChannel和SocketChannel
- FileChannel用于文件的数据读写
DatagramChannel用于UDP的数据读写 ServerSocketChannel和SocketChannel用于TCP的数据读写 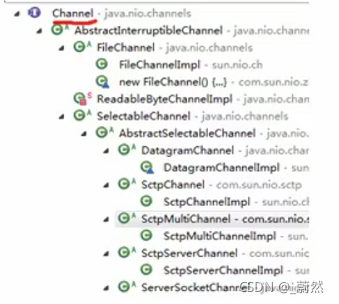
FileChannel类
FileChannel主要用来对本地文件进行IO操作,常见的方法有
- public abstract int read(ByteBuffer dst) ,从通道读取数据并放到缓冲区重
- public abstract int write(ByteBuffer src),把缓冲区的数据写到通道中
- public abstract long transferFrom(ReadableByteChannel src, long position, long count),从目标通道中复制数据到当前通道
- public abstract long transferTo(long position, long count,WritableByteChannel target),把数据从当前通道复制给目标通道
应用实例1-本地文件写数据
使用前面学到的ByteBuffer(缓冲)和(通道),将字符串写入file01.txt中
package com.example.demo.netty;
import java.io.FileOutputStream;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class NioFileChannel01 {
public static void main(String[] args) throws Exception {
String str = "hello 世界";
FileOutputStream fos = new FileOutputStream("D:/file01.txt");
FileChannel fileChannel = fos.getChannel();
ByteBuffer byteBuffer = ByteBuffer.allocate(1024);
byteBuffer.put(str.getBytes());
byteBuffer.flip();
fileChannel.write(byteBuffer);
fos.close();
}
}
应用实例2-本地文件读数据
使用前面学到的ByteBuffer(缓冲)和(通道),将file01.txt中的数据读入到程序,并显示在控制台屏幕
package com.example.demo.netty;
import java.io.FileInputStream;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class NioFileChannel02 {
public static void main(String[] args) throws Exception {
FileInputStream fis = new FileInputStream("D:/file01.txt");
FileChannel fileChannel = fis.getChannel();
ByteBuffer byteBuffer = ByteBuffer.allocate((int) fileChannel.size());
fileChannel.read(byteBuffer);
System.out.println(new String(byteBuffer.array()));
fis.close();
}
}
应用实例3-使用一个Buffer完成文件的读取
使用FileChannel(通道)和方法read write,完成文件的拷贝
package com.example.demo.netty;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class NioFileChannel03 {
public static void main(String[] args) throws Exception {
FileInputStream fileInputStream = new FileInputStream("1.txt");
FileChannel fileChannel01 = fileInputStream.getChannel();
FileOutputStream fileOutputStream = new FileOutputStream("2.txt");
FileChannel fileChannel02 = fileOutputStream.getChannel();
ByteBuffer byteBuffer = ByteBuffer.allocate(512);
while (true) {
byteBuffer.clear();
int read = fileChannel01.read(byteBuffer);
if (read == -1) {
break;
}
byteBuffer.flip();
fileChannel02.write(byteBuffer);
}
}
}
应用实例4-拷贝文件
使用FileChannel(通道)和方法transferFrom,完成文件的拷贝
package com.example.demo.netty;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.nio.channels.FileChannel;
public class NioFileChannel04 {
public static void main(String[] args) throws Exception {
FileInputStream fileInputStream = new FileInputStream("a.png");
FileOutputStream fileOutputStream = new FileOutputStream("b.png");
FileChannel sourceCh = fileInputStream.getChannel();
FileChannel destCh = fileOutputStream.getChannel();
destCh.transferFrom(sourceCh, 0, sourceCh.size());
sourceCh.close();
destCh.close();
fileInputStream.close();
fileOutputStream.close();
}
}
|