1、反射的作用
(1)在运行时判断对象所属的类(java.lang.reflect.Class类)
(2)在运行时获取类的对象(java.lang.reflect.Constructor类)
(3)获取修改对象的属性(java.lang.reflect.Field类)
? (4) 调用对象的方法(java.lang.reflect.Method类)
2、java.lang.reflect.Class
(1)每一个类都有一个唯一的Class对象用于描述类的信息,一个类只有一个Class对象,但是可以有多个实例对象
(2)类的Class对象是JVM创建的是唯一的,其构造函数是私有函数,无法手动创建。
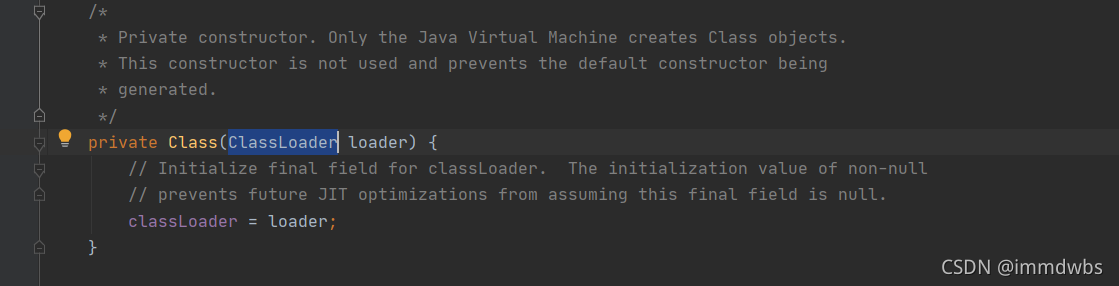
(3)获取Class对象的三种方法
a、通过Class.forName(包名+类名);
b、通过 类的实例对象的getClass()方法;
c、通过类名.class;
package reflect;
public class ClazzTest {
public static void main(String[] args) throws ClassNotFoundException {
ClazzTest clazzTest=new ClazzTest();
//(1)通过 Class.forName()
Class calzz1=Class.forName("reflect.ClazzTest");
//(2)通过 类的实例对象的getClass()方法
Class calzz2=clazzTest.getClass();
//(3)通过 类名.class
Class calzz3=ClazzTest.class;
//运行返回True
System.out.println(calzz1==calzz2&&calzz2==calzz3);
}
}
3、java.lang.reflect.Constructor
(1)获取构造方法
a、getConstructors():获取所有的公有的构造方法数组
? ? ?getConstructor(Class<?>...):获取单个指定参数类型的公有构造方法

?b、getDeclaredConstructors()获取该类声明的所有(访问权限)的构造方法数组
? ? ? ? getDeclaredConstructor(Class<?>...)获取单个指定参数类型的构造方法(可为所有类型的访问权限)

?代码实例:
package reflect;
public class ConstructorDemo {
private String member;
private Integer number;
public ConstructorDemo(String s,Integer n){
this.member=s;
this.number=n;
System.out.println("调用了公有权限的构造方法");
}
protected ConstructorDemo(String s){
this.member=s;
System.out.println("调用了保护权限的构造方法");
}
private ConstructorDemo(Integer n){
this.number=n;
System.out.println("调用了私有权限的构造方法");
}
ConstructorDemo(){
System.out.println("调用了默认权限的构造方法");
}
}
package reflect;
import java.lang.reflect.Constructor;
public class ReflectTest {
public static void main(String[] args) throws ClassNotFoundException, NoSuchMethodException {
Class clazz=Class.forName("reflect.ConstructorDemo");
Constructor[] cArray1=clazz.getConstructors();
System.out.println("-----------获取所有的公有构造方法------------------");
for (Constructor c:cArray1) {
System.out.println(c);
}
System.out.println("-----------获取声明的所有访问权限的构造方法------------------");
Constructor[] cArray2=clazz.getDeclaredConstructors();
for (Constructor c:cArray2) {
System.out.println(c);
}
Constructor c1=clazz.getConstructor(String.class,Integer.class);
System.out.println("-----------获取指定参数的且为公有的构造方法------------------");
System.out.println(c1);
Constructor c2=clazz.getDeclaredConstructor(Integer.class);
System.out.println("-----------获取指定参数的构造方法(无所谓访问权限)------------------");
System.out.println(c2);
}
}
运行结果:
-----------获取所有的公有构造方法------------------
public reflect.ConstructorDemo(java.lang.String,java.lang.Integer)
-----------获取声明的所有访问权限的构造方法------------------
reflect.ConstructorDemo()
private reflect.ConstructorDemo(java.lang.Integer)
protected reflect.ConstructorDemo(java.lang.String)
public reflect.ConstructorDemo(java.lang.String,java.lang.Integer)
-----------获取指定参数的且为公有的构造方法------------------
public reflect.ConstructorDemo(java.lang.String,java.lang.Integer)
-----------获取指定参数的构造方法(无所谓访问权限)------------------
private reflect.ConstructorDemo(java.lang.Integer)
Process finished with exit code 0
(2)调用构造方法创建对象实例:
通过newInstance(构造函数对应的实参列表)来创建对象
package reflect;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
public class ReflectTest {
public static void main(String[] args) throws ClassNotFoundException, NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException {
Class clazz=Class.forName("reflect.ConstructorDemo");
Constructor c1=clazz.getConstructor(String.class,Integer.class);
System.out.println("-----------获取指定参数的且为公有的构造方法------------------");
System.out.println(c1);
c1.newInstance("aaa",3);
Constructor c2=clazz.getDeclaredConstructor(Integer.class);
//c2.setAccessible(true)
System.out.println("-----------获取私有的构造方法------------------");
System.out.println(c2);
c2.newInstance(3);
}
}
运行报错:调用私有构造函数是非法的,通过调用c2.setAccessible(true),来实现私有构造函数的访问
-----------获取指定参数的且为公有的构造方法------------------
public reflect.ConstructorDemo(java.lang.String,java.lang.Integer)
调用了公有权限的构造方法
-----------获取私有的构造方法------------------
private reflect.ConstructorDemo(java.lang.Integer)
Exception in thread "main" java.lang.IllegalAccessException: Class reflect.ReflectTest can not access a member of class reflect.ConstructorDemo with modifiers "private"
at sun.reflect.Reflection.ensureMemberAccess(Reflection.java:102)
at java.lang.reflect.AccessibleObject.slowCheckMemberAccess(AccessibleObject.java:296)
at java.lang.reflect.AccessibleObject.checkAccess(AccessibleObject.java:288)
at java.lang.reflect.Constructor.newInstance(Constructor.java:413)
at reflect.ReflectTest.main(ReflectTest.java:26)
Process finished with exit code 1
4、java.lang.reflect.Field
(1)获取成员变量
a、getFields()获取所有的公有权限的字段数组(包含继承自父类)
? ? ?getField(字段名)通过字段名获取公有权限的字段

b、getDeclaredFields()获取当前类声明的(不包含继承的)所有权限的字段数组
? ? ?getDeclaredField(字段名)通过字段名获取当前类声明的(不包含继承的)所有权限的字段

?代码实例:
package reflect;
public class FieldOriDemo {
public String fatherPublicField;
}
package reflect;
public class FieldDemo extends FieldOriDemo {
private String priField;
protected String proField;
String defFiled;
public Integer pubField;
public FieldDemo(){
}
}
package reflect;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
public class ReflectTest {
public static void main(String[] args) throws ClassNotFoundException, NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException, NoSuchFieldException {
Class clazz=Class.forName("reflect.FieldDemo");
Field[] fs1=clazz.getFields();
System.out.println("---------------获取所有的公有字段(包含继承自父类的)----------------------");
for (Field f:fs1) {
System.out.println(f);
}
Field[] fs2=clazz.getDeclaredFields();
System.out.println("---------------获取该类声明(不包含继承自父类的)的所有权限的字段----------------------");
for (Field f:fs2) {
System.out.println(f);
}
Field f1=clazz.getField("fatherPublicField");
System.out.println("---------------获取公有字段(可以是继承自父类)fatherPublicField----------------------");
System.out.println(f1);
Field f2=clazz.getDeclaredField("priField");
System.out.println("---------------获取该类声明的私有字段priField----------------------");
System.out.println(f2);
}
}
运行结果:
---------------获取所有的公有字段(包含继承自父类的)----------------------
public java.lang.Integer reflect.FieldDemo.pubField
public java.lang.String reflect.FieldOriDemo.fatherPublicField
---------------获取该类声明(不包含继承自父类的)的所有权限的字段----------------------
private java.lang.String reflect.FieldDemo.priField
protected java.lang.String reflect.FieldDemo.proField
java.lang.String reflect.FieldDemo.defFiled
public java.lang.Integer reflect.FieldDemo.pubField
---------------获取公有字段(可以是继承自父类)fatherPublicField----------------------
public java.lang.String reflect.FieldOriDemo.fatherPublicField
---------------获取该类声明的私有字段priField----------------------
private java.lang.String reflect.FieldDemo.priField
Process finished with exit code 0
(2)设置成员变量的值
通过Field.set(实例对象,成员变量的值)来完成对对象成员变量的赋值。
package reflect;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
public class ReflectTest {
public static void main(String[] args) throws ClassNotFoundException, NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException, NoSuchFieldException {
//构造方法
Constructor constructor=clazz.getConstructor();
FieldDemo fieldDemo=(FieldDemo)constructor.newInstance();
Field f1=clazz.getField("fatherPublicField");
System.out.println("---------------获取公有字段(可以是继承自父类)fatherPublicField----------------------");
System.out.println(f1);
f1.set(fieldDemo,"aaaa");
System.out.println(fieldDemo.fatherPublicField);
Field f2=clazz.getDeclaredField("priField");
System.out.println("---------------获取该类声明的私有字段priField----------------------");
System.out.println(f2);
// f2.setAccessible(true);
f2.set(fieldDemo,"aaaa");
}
}
运行结果:不能对私有的成员变量进行赋值,与构造函数一样,可以通过调用Field.setAccessible(true)来解决该问题。
---------------获取公有字段(可以是继承自父类)fatherPublicField----------------------
public java.lang.String reflect.FieldOriDemo.fatherPublicField
aaaa
---------------获取该类声明的私有字段priField----------------------
private java.lang.String reflect.FieldDemo.priField
Exception in thread "main" java.lang.IllegalAccessException: Class reflect.ReflectTest can not access a member of class reflect.FieldDemo with modifiers "private"
at sun.reflect.Reflection.ensureMemberAccess(Reflection.java:102)
at java.lang.reflect.AccessibleObject.slowCheckMemberAccess(AccessibleObject.java:296)
at java.lang.reflect.AccessibleObject.checkAccess(AccessibleObject.java:288)
at java.lang.reflect.Field.set(Field.java:761)
at reflect.ReflectTest.main(ReflectTest.java:53)
Process finished with exit code 1
5、java.lang.reflect.Method
(1)获取类的方法
a、getMethods()获取所有的公有的方法,包括继承自父类
? ? ? getMethod(方法名,参数类型...)获取对应签名的公有方法,包括继承自父类

? ? ? ? getDeclaredMethods()获取该类声明的所有权限的方法,不继承自父类
????????getDeclaredMethod(方法名,参数类型...)获取对应签名的(任意访问权限)方法,不继承自? ? ? ? ? ?父类
?
?代码实例:
package reflect;
public class MethodOriDemo {
public void show1(String a,String b){
System.out.println("father show1:"+a+" "+b);
}
}
package reflect;
public class MethodDemo extends MethodOriDemo{
public MethodDemo(){
}
public void show1(String a){
System.out.println("child show1:"+a);
}
protected void show2(String a){
System.out.println("show2:"+a);
}
void show3(String a){
System.out.println("show3:"+a);
}
private void show4(String a){
System.out.println("show4:"+a);
}
}
package reflect;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class ReflectTest {
public static void main(String[] args) throws ClassNotFoundException, NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException, NoSuchFieldException {
Class clazz=Class.forName("reflect.MethodDemo");
Method[] methods1 =clazz.getMethods();
System.out.println("---------------获取所有的公有方法(包含继承自父类的)----------------------");
for (Method method:methods1) {
System.out.println(method);
}
Method[] methods2 =clazz.getDeclaredMethods();
System.out.println("---------------获取该类声明的所有访问权限的方法(不包含继承自父类)----------------------");
for (Method method:methods2) {
System.out.println(method);
}
Method method1=clazz.getMethod("show1", String.class, String.class);
System.out.println("---------------获取继承自父类的show1方法----------------------");
System.out.println(method1);
Method method2=clazz.getDeclaredMethod("show4", String.class);
System.out.println("---------------获取该类声明的私有的show4方法----------------------");
System.out.println(method2);
}
}
运行结果:
---------------获取所有的公有方法(包含继承自父类的)----------------------
public void reflect.MethodDemo.show1(java.lang.String)
public void reflect.MethodOriDemo.show1(java.lang.String,java.lang.String)
public final void java.lang.Object.wait() throws java.lang.InterruptedException
public final void java.lang.Object.wait(long,int) throws java.lang.InterruptedException
public final native void java.lang.Object.wait(long) throws java.lang.InterruptedException
public boolean java.lang.Object.equals(java.lang.Object)
public java.lang.String java.lang.Object.toString()
public native int java.lang.Object.hashCode()
public final native java.lang.Class java.lang.Object.getClass()
public final native void java.lang.Object.notify()
public final native void java.lang.Object.notifyAll()
---------------获取该类声明的所有访问权限的方法(不包含继承自父类)----------------------
public void reflect.MethodDemo.show1(java.lang.String)
private void reflect.MethodDemo.show4(java.lang.String)
protected void reflect.MethodDemo.show2(java.lang.String)
void reflect.MethodDemo.show3(java.lang.String)
---------------获取继承自父类的show1方法----------------------
public void reflect.MethodOriDemo.show1(java.lang.String,java.lang.String)
---------------获取该类声明的私有的show4方法----------------------
private void reflect.MethodDemo.show4(java.lang.String)
Process finished with exit code 0
(2)调用对象的方法
package reflect;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class ReflectTest {
public static void main(String[] args) throws ClassNotFoundException, NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException, NoSuchFieldException {
Class clazz=Class.forName("reflect.MethodDemo");
Constructor constructor=clazz.getConstructor();
MethodDemo methodDemo=(MethodDemo)constructor.newInstance();
Method method1=clazz.getMethod("show1", String.class, String.class);
System.out.println("---------------获取继承自父类的show1方法----------------------");
System.out.println(method1);
method1.invoke(methodDemo,"aaa","bbbb");
Method method2=clazz.getDeclaredMethod("show4", String.class);
System.out.println("---------------获取该类声明的私有的show4方法----------------------");
System.out.println(method2);
// method2.setAccessible(true);
method2.invoke(methodDemo,"aaa");
}
}
运行结果:不能直接调用私有的方法,与构造函数,成员变量一样,可以通过调用Method.setAccessible(true)来解决该问题。
---------------获取继承自父类的show1方法----------------------
public void reflect.MethodOriDemo.show1(java.lang.String,java.lang.String)
father show1:aaa bbbb
---------------获取该类声明的私有的show4方法----------------------
private void reflect.MethodDemo.show4(java.lang.String)
Exception in thread "main" java.lang.IllegalAccessException: Class reflect.ReflectTest can not access a member of class reflect.MethodDemo with modifiers "private"
at sun.reflect.Reflection.ensureMemberAccess(Reflection.java:102)
at java.lang.reflect.AccessibleObject.slowCheckMemberAccess(AccessibleObject.java:296)
at java.lang.reflect.AccessibleObject.checkAccess(AccessibleObject.java:288)
at java.lang.reflect.Method.invoke(Method.java:491)
at reflect.ReflectTest.main(ReflectTest.java:77)
Process finished with exit code 1
?
|