标题因项目需要导出word报告,就研究了一下java生成word,网上的文章无非就是那几种方式,最简单的还是使用Freemarker的模板导出word,下面简单介绍使用方法:
Freemarker介绍:
FreeMarker是一款模板引擎: 即一种基于模板和要改变的数据, 并用来生成输出文本(HTML网页、电子邮件、配置文件、源代码等)的通用工具。 它不是面向最终用户的,而是一个Java类库,是一款程序员可以嵌入他们所开发产品的组件。 FreeMarker是免费的,基于Apache许可证2.0版本发布。其模板编写为FreeMarker Template Language(FTL),属于简单、专用的语言。需要准备数据在真实编程语言中来显示,比如数据库查询和业务运算, 之后模板显示已经准备好的数据。在模板中,主要用于如何展现数据, 而在模板之外注意于要展示什么数据 [1] 。 ------百度百科
我们利用Freemarker导出的本质也是通过组合好数据然后往模板里面对应的位置填充数据实现。
首先导入Freemarker的依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
# 版本的话还是用这个不容易出问题:
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.30</version>
</dependency>
制作ftl模板文件 word2003之后支持了xml格式,所以我们需要用word导出一份xml的文件,具体操作如下: 1.先预设好自己需要导出的格式,然后将需要输出的值用${}去占位,如果需要导出图片的话,要放一张图片去占位。 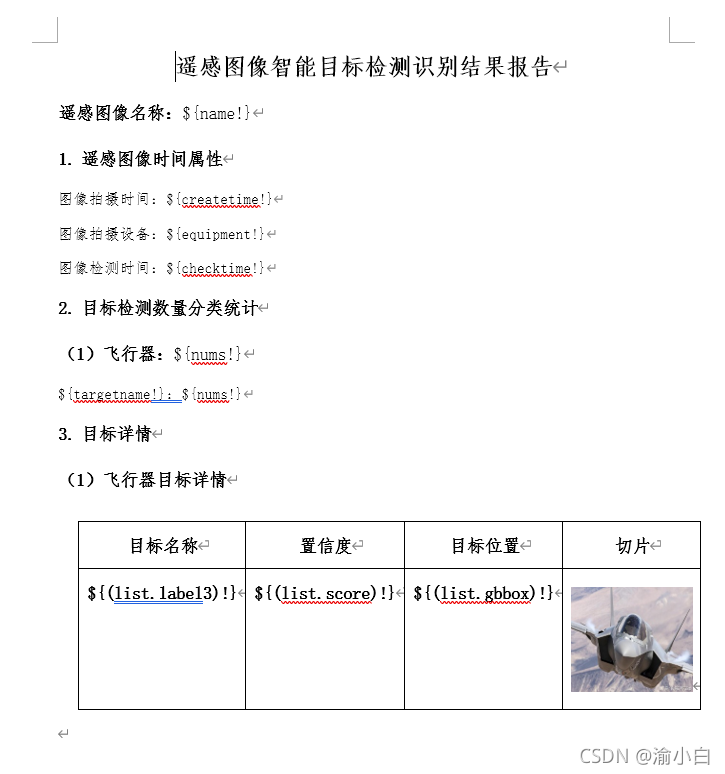 2.添加好占位符之后,将该Word文档另存为XML格式的文件 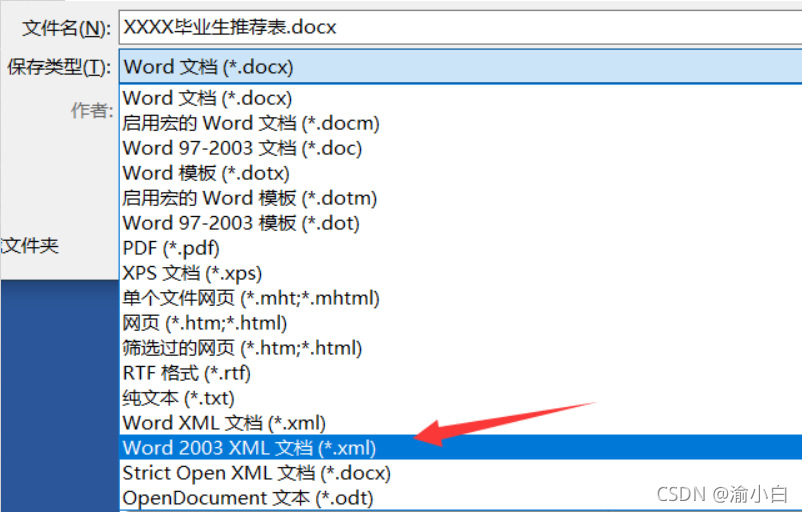
修改一下文件名称,保存 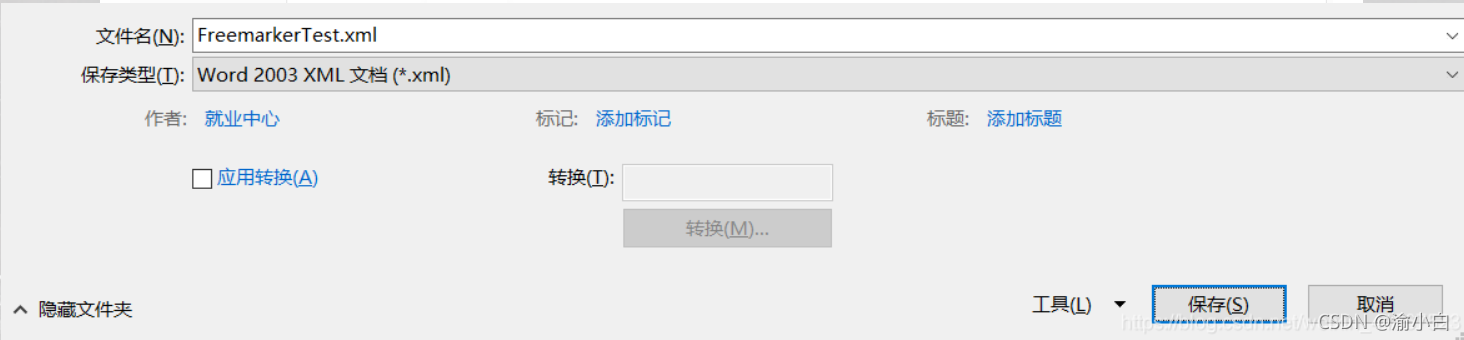 即得到一个XML文件 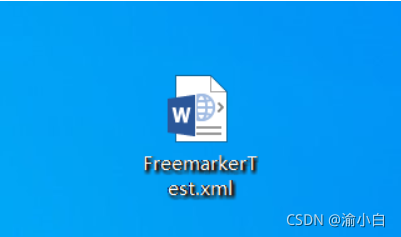 3.将xml文件放进idea,修改后缀名为ftl,使用格式化快捷键Ctrl+Alt+L,将内容格式化,这里转换成xml之后会将占位符分开例如这样: 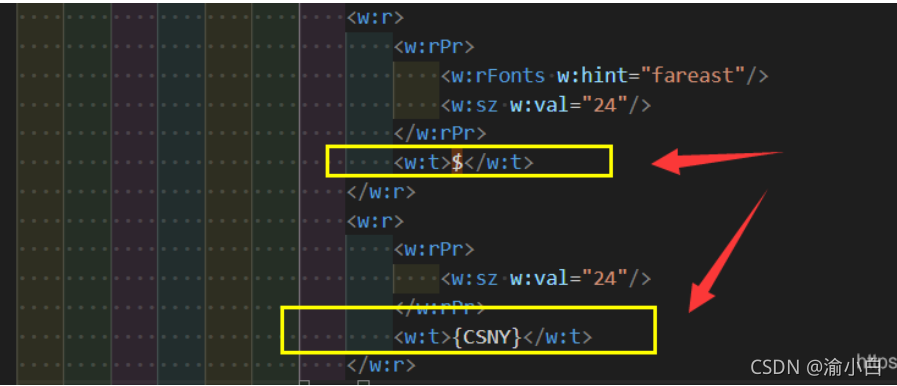 需要将中间的多出来的部分删除掉,如果数据填充时为空会报错,解决方法可以在占位符里面加一个!,例如:"${name!}",如果是用集合点属性名的话需要用()包起来:"${(list.name)!}" 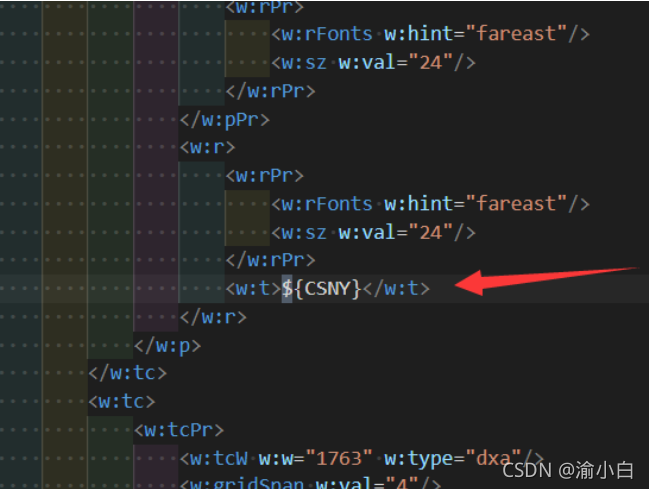 4.填充的图片转换成xml后会转换成base64的编码,需要把编码换成占位符: 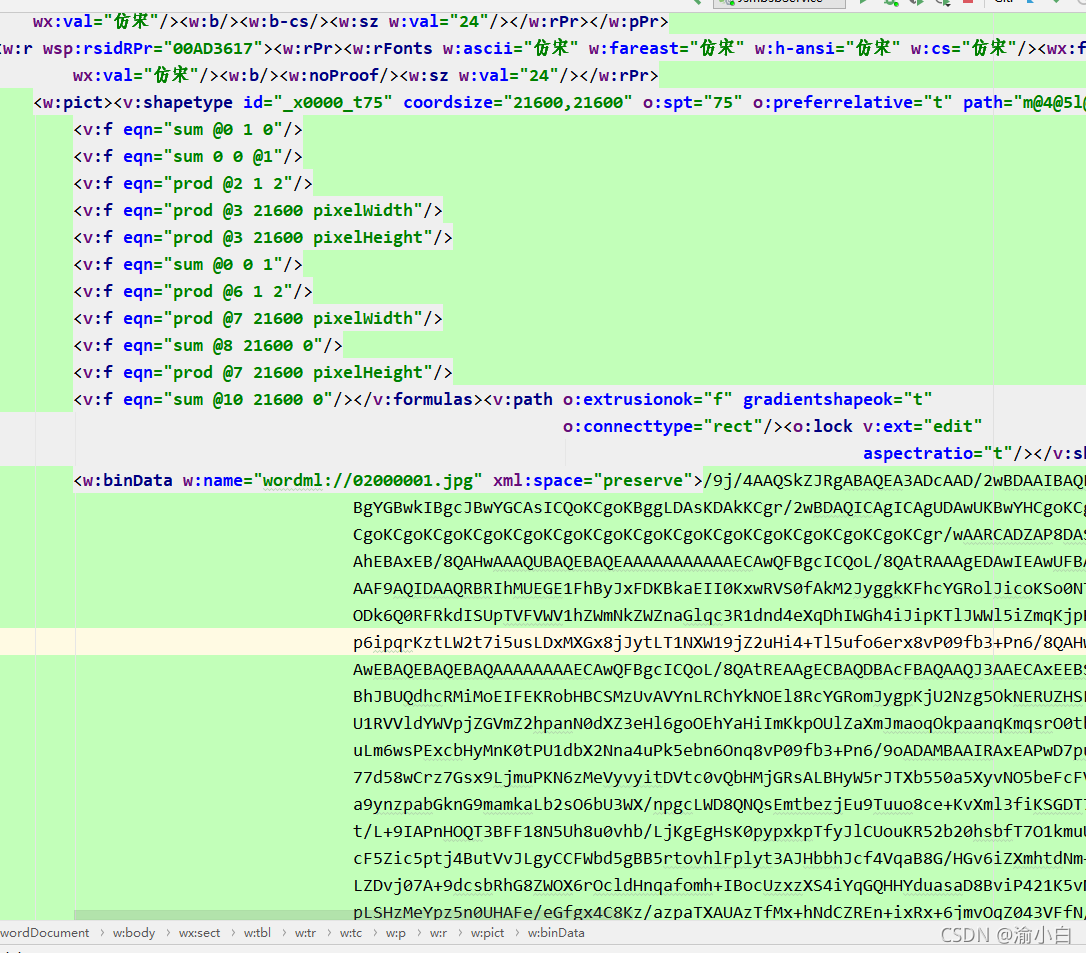 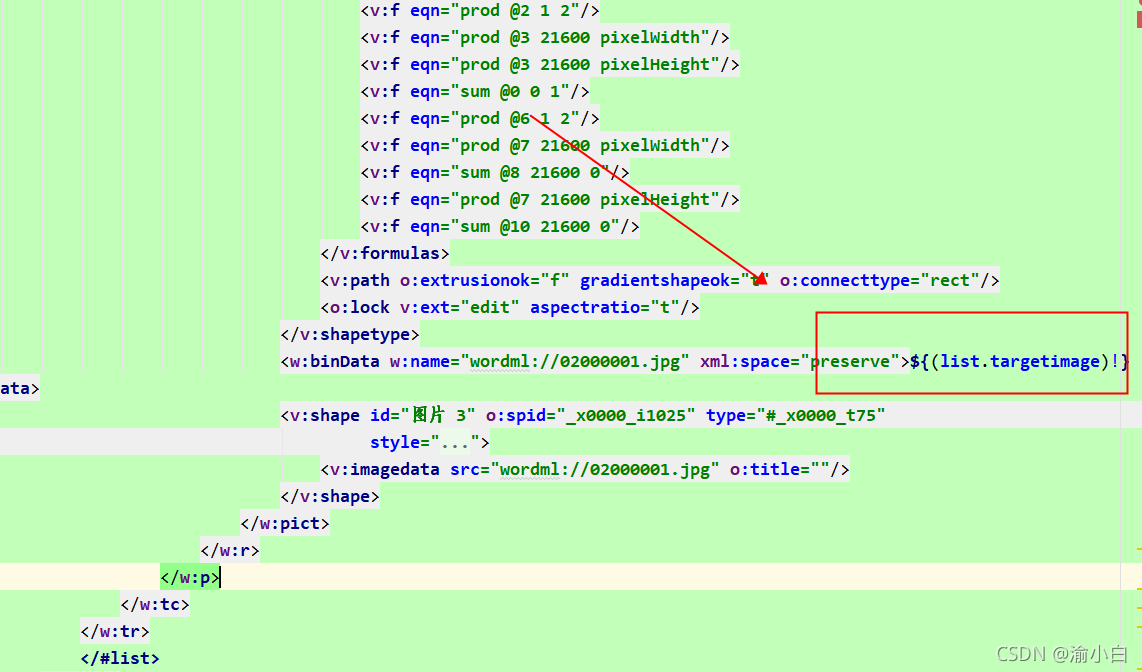
5.需要导出表格的时候需要循环填充数据,这里就需要添加<#list><#list>,标签位置如何找,可以通过你的表格的最后一个表格头的名称找到表格结尾,我的表格结尾是"切片",那我就可以去xml中搜索切片两个字,找到后例如这样:
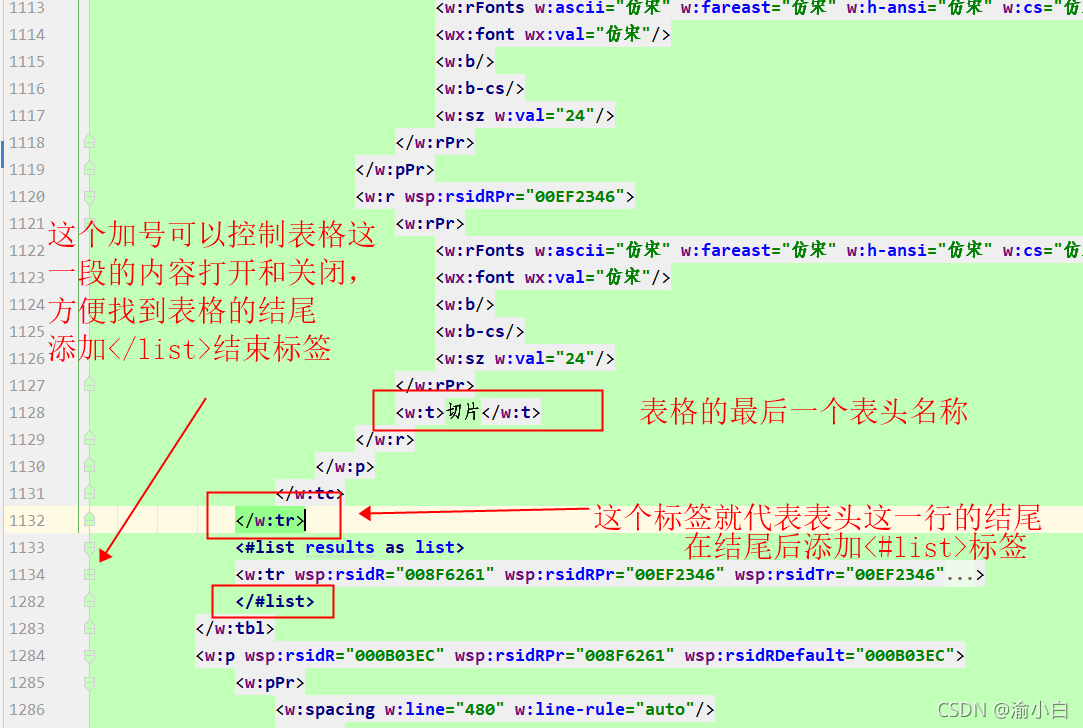 6.将文件放到resource目录下,这样方便项目打包后仍然可以找到模板,也可以将模板放在磁盘中,写代码时写成绝对路径,不过还是建议放在resource下。
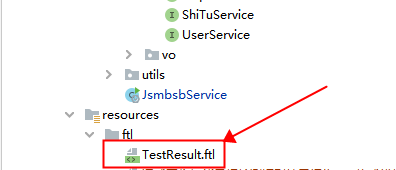 7.代码层:编写你需要存放数据的实体类,这里就不展示了;编写控制层;编写实现层:
控制层:
public Object downTestReport(@RequestParam(value = "taskid") String taskid, HttpServletResponse response) throws IOException {
String path = remoteDetectionResultService.downTestReport(taskid);
BufferedOutputStream out = null;
try {
File file = new File(path);
if (file.isFile() && file.exists()) {
String fileName = "遥感检测报告.pdf";
BufferedInputStream fis = new BufferedInputStream(new FileInputStream(path));
byte[] buffer = new byte[fis.available()];
fis.read(buffer);
fis.close();
response.setContentType("application/octet-stream;charset=utf-8");
response.setHeader("Content-disposition", "attachment;filename="
+ new String(fileName.getBytes("UTF-8"), "ISO-8859-1"));
response.setCharacterEncoding("UTF-8");
out = new BufferedOutputStream(response.getOutputStream(), 3 * 1024);
out.write(buffer);
out.flush();
out.close();
} else {
response.setStatus(0);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (out != null) {
out.close();
}
}
return response;
}
实现层:这里有一点需要说明,导出的word只能是.doc的后缀,不能是.docx,否则打不开
@Override
public String downTestReport(String taskid) {
Map<String,Object> dataMap = new HashMap<String,Object>();
Configuration configuration = new Configuration();
configuration.setDefaultEncoding("utf-8");
try {
configuration.setClassForTemplateLoading(this.getClass(), "/ftl");
Template template = configuration.getTemplate("TestResult.ftl");
QueryWrapper<RemoteDetectionResult> wrapper = new QueryWrapper<>();
wrapper.eq("taskid", taskid);
List<RemoteDetectionResult> remoteDetectionResults = remoteDetectionResultMapper.selectList(wrapper);
RemoteSensingImage image = remoteImageMapper.selectByTaskid(taskid);
dataMap.put("name",image.getName());
dataMap.put("createtime",image.getFilmingtime());
dataMap.put("equipment",image.getFilmingequipment());
dataMap.put("checktime",remoteDetectionResults.get(0).getCreatetime());
dataMap.put("nums",remoteDetectionResults.size());
dataMap.put("targetname",remoteDetectionResults.get(0).getLabel3());
for (RemoteDetectionResult remote : remoteDetectionResults) {
String path = "F:/Desktop"+remote.getPath();
String imageStrFromPath = FileUtil.getImageStrFromPath(path);
remote.setTargetimage(imageStrFromPath);
}
dataMap.put("results",remoteDetectionResults);
String doc = createDoc(dataMap, template);
return doc;
} catch (IOException | TemplateException e) {
e.printStackTrace();
}
return null;
}
public static String createDoc(Map<String, Object> dataMap, Template template)
throws TemplateException, IOException {
File outFile = new File("F:/Desktop/tif/report.doc");
Writer out = null;
try {
out = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(outFile),"utf-8"));
template.process(dataMap,out);
out.close();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(out != null){
out.close();
}
}
return "F:/Desktop/tif/report.doc";
}
代码部分就是这些了,相对来说这种方式还是很简单的,如果没有看懂的话可以参考下面的的博客,写的非常详细:https://blog.csdn.net/weixin_41367523/article/details/106944130?spm=1001.2014.3001.5506
|