springboot集成sentinel和nacos
- 导入依赖
<!-- nacos注册中心-->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<!-- nacos配置中心-->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId>
</dependency>
<!--需要加入此依赖才会读取bootstrap配置文件-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bootstrap</artifactId>
<version>3.0.3</version>
</dependency>
<!-- 集成sentinel 版本是1.8.0,根据自己的版本而定,不一样可能会有bug-->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-alibaba-sentinel-gateway</artifactId>
</dependency>
- 添加配置
spring:
application:
name: gateway
sentinel:
transport:
dashboard: localhost:9000
port: 8719 #应用与Sentinel控制台交互的端口
# 应用与Sentinel控制台的心跳间隔时间
heartbeat-interval-ms: 10000 #默认是10s
# 此配置的客户端IP将被注册到 Sentinel Server 端
client-ip: localhost
#是否提前触发 Sentinel 初始化
eager: true
filter:
enabled: false
scg:
fallback:
mode: response # 响应模式 response、redirect
response-status: 444 # 响应状态码
response-body: 服务器炸了!让它先缓缓哈 # 响应信息
content-type: application/json
order: -100
redirect: /errorPage.html # 响应模式为 'redirect' 模式对应的重定向 URL
- 修改sentinel控制台源码
下载地址 https://github.com/alibaba/Sentinel
修改sentinel-dashboard的源码
将此路径下的文件全部复制到下下面的路径下 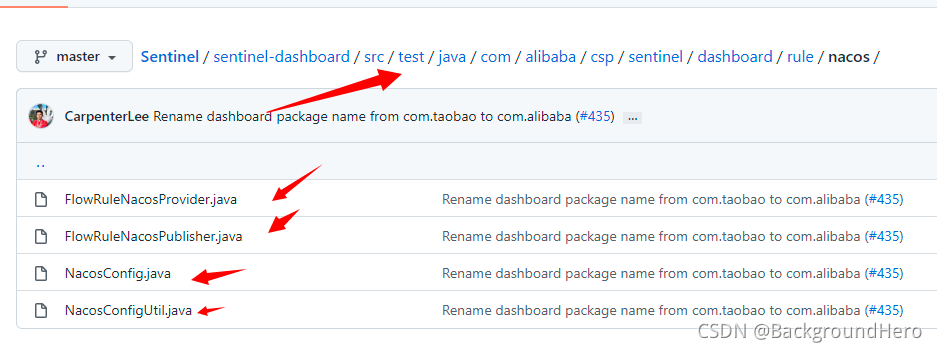
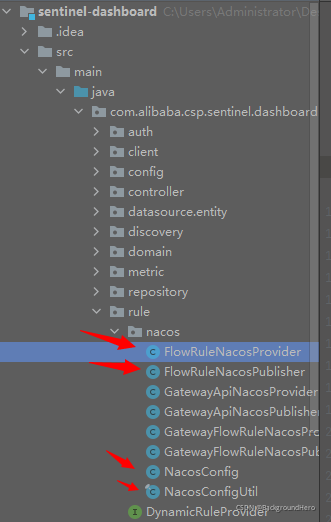 修改NacosConfig,换成自己的要拉取的nacos缓存 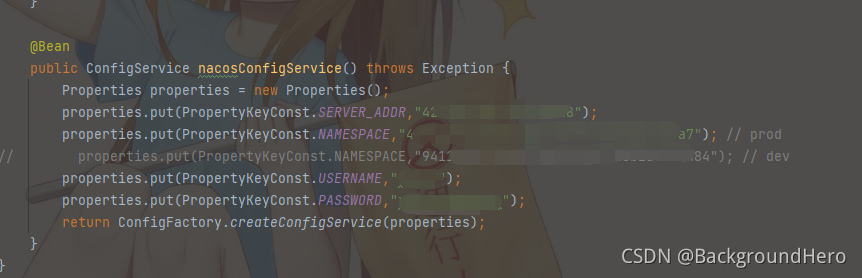
修改NacosConfigUtil 默认配置文件的分组在SENTINEL_GROUP,根据自己的修改就行 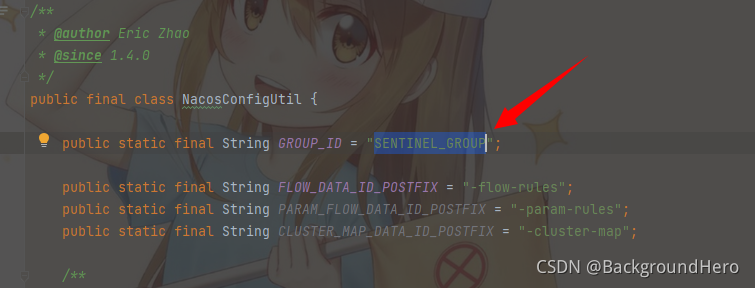
剩下四个文件就是我们要自己创建的,用来从nacos推拉配置
/**
* @author xiaohong
* @version 1.0
* @date 2021/11/17 0017 11:22
* @description 从nacos拉取网关api分组配置规则
*/
@Component
public class GatewayApiNacosProvider {
@Autowired
private ConfigService configService;
/**
* 从nacos拉取配置
* @param appName
* @return
* @throws Exception
*/
public List<ApiDefinitionEntity> fetchApis(String appName) throws Exception {
// 随便取得名字,用来保存对应配置,记得先在nacos里创建好,初始值为[]
String rules = configService.getConfig("sentinel-gateway-gw-api-group-rules.json",
NacosConfigUtil.GROUP_ID, 3000);
if (StringUtil.isEmpty(rules)) {
return new ArrayList<>();
}
List<ApiDefinitionEntity> list = new ArrayList<>();
List result = JSON.parseObject(rules, List.class);
if (result != null && result.size() > 0){
for (Object o : result) {
ApiDefinitionEntity apiDefinitionEntity = JSON.toJavaObject((JSON) o, ApiDefinitionEntity.class);
list.add(apiDefinitionEntity);
}
}
return list;
}
}
/**
* @author xiaohong
* @version 1.0
* @date 2021/11/17 0017 11:29
* @description 网关api分组规则推送到nacos
*/
@Component
public class GatewayApiNacosPublisher {
@Autowired
private ConfigService configService;
public Boolean modifyApis(String app, List<ApiDefinitionEntity> rules) {
AssertUtil.notEmpty(app, "app name cannot be empty");
if (rules == null) {
return false;
}
try {
// 随便取得名字,用来保存对应配置,记得先在nacos里创建好,初始值为[]
configService.publishConfig("sentinel-gateway-gw-api-group-rules.json",
NacosConfigUtil.GROUP_ID, JSON.toJSONString(rules));
return true;
} catch (NacosException e) {
e.printStackTrace();
return false;
}
}
}
- GatewayFlowRuleNacosProvider
/**
* @author xiaohong
* @version 1.0
* @date 2021/11/17 0017 11:37
* @description 使用nacos拉取网关流控规则
*/
@Component
public class GatewayFlowRuleNacosProvider {
@Autowired
private ConfigService configService;
/**
* 从nacos拉取配置
* @param appName
* @return
* @throws Exception
*/
public List<GatewayFlowRuleEntity> fetchGatewayFlowRules(String appName) throws Exception {
// 随便取得名字,用来保存对应配置,记得先在nacos里创建好,初始值为[]
String rules = configService.getConfig("sentinel-gateway-gw-flow-rules.json",
NacosConfigUtil.GROUP_ID, 3000);
if (StringUtil.isEmpty(rules)) {
return new ArrayList<>();
}
List<GatewayFlowRuleEntity> list = new ArrayList<>();
List result = JSON.parseObject(rules, List.class);
if (result != null && result.size() > 0){
for (Object o : result) {
GatewayFlowRuleEntity gatewayFlowRuleEntity = JSON.toJavaObject((JSON) o, GatewayFlowRuleEntity.class);
list.add(gatewayFlowRuleEntity);
}
}
return list;
}
}
- GatewayFlowRuleNacosPublisher
/**
* @author xiaohong
* @version 1.0
* @date 2021/11/17 0017 11:38
* @description 使用nacos推送网关流控规则
*/
@Component
public class GatewayFlowRuleNacosPublisher {
@Autowired
private ConfigService configService;
public Boolean modifyGatewayFlowRules(String app, List<GatewayFlowRuleEntity> rules) {
AssertUtil.notEmpty(app, "app name cannot be empty");
if (rules == null) {
return false;
}
try {
// 随便取得名字,用来保存对应配置,记得先在nacos里创建好,初始值为[]
configService.publishConfig("sentinel-gateway-gw-flow-rules.json",
NacosConfigUtil.GROUP_ID, JSON.toJSONString(rules));
return true;
} catch (NacosException e) {
e.printStackTrace();
return false;
}
}
}
然后用我们自己写的方法替换掉原来的方法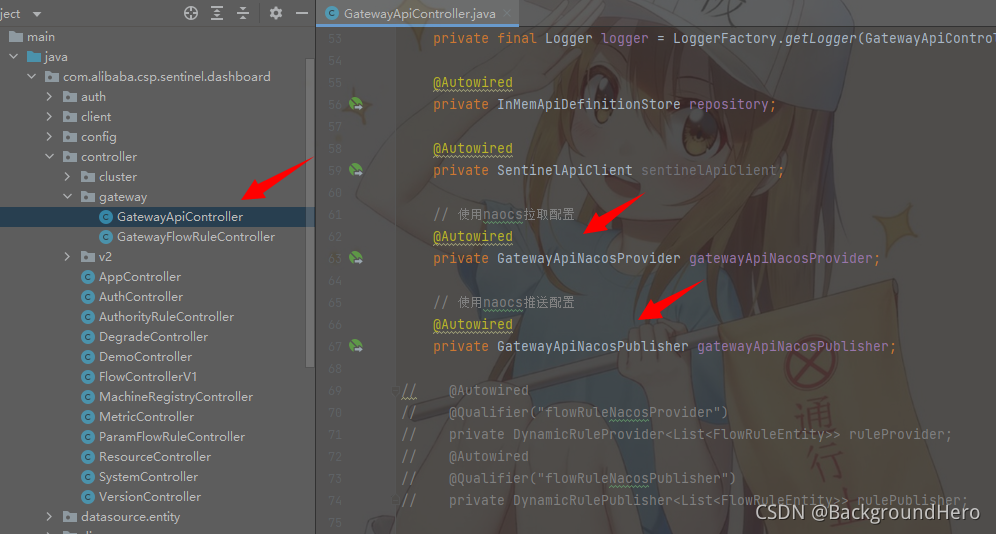   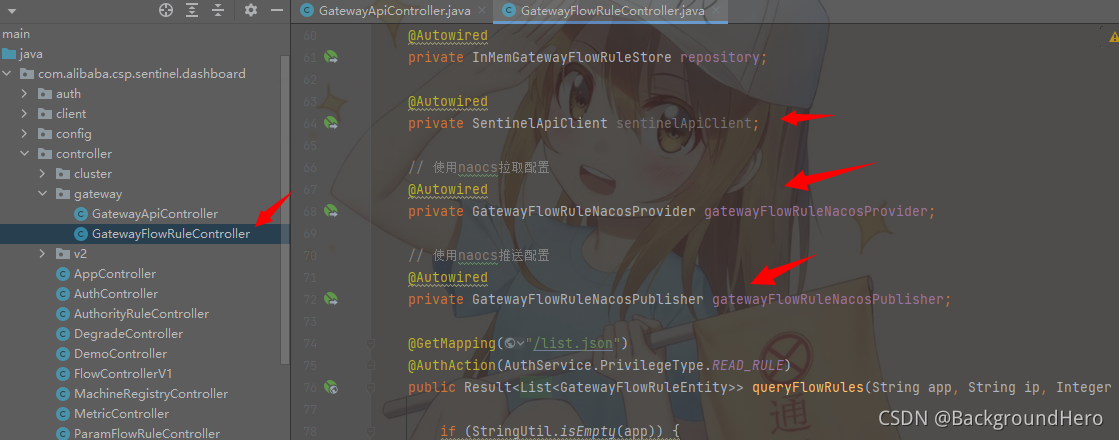  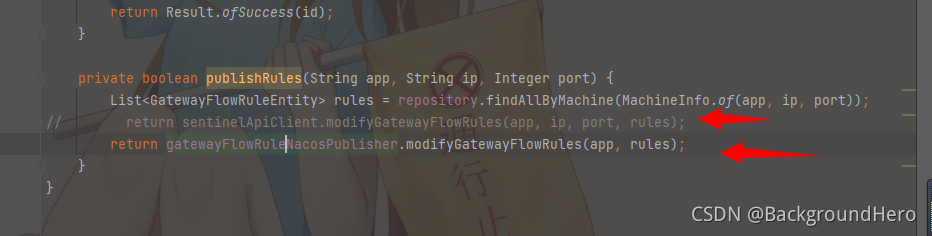 nacos创建配置文件 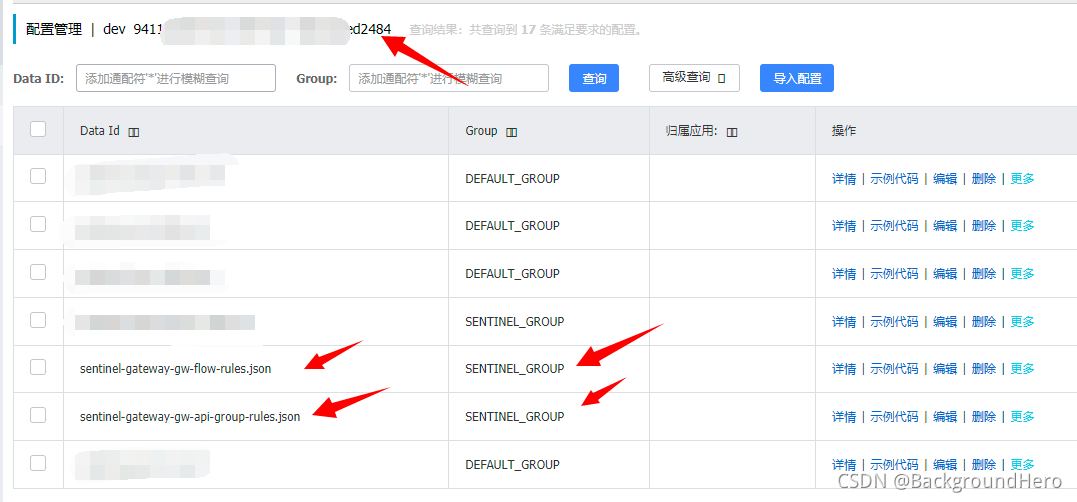 这里的配置记得要和自己在NacosConfig和NacosConfigUtil配的一样,名字也要和那四个文件里取的匹配,初始值都是空数组[]
测试
运行sentinel-dashboard 启动前在配置文件里加段配置,配置sentinel启动的端口号,与gateway里的配置对应上
server.port=9000
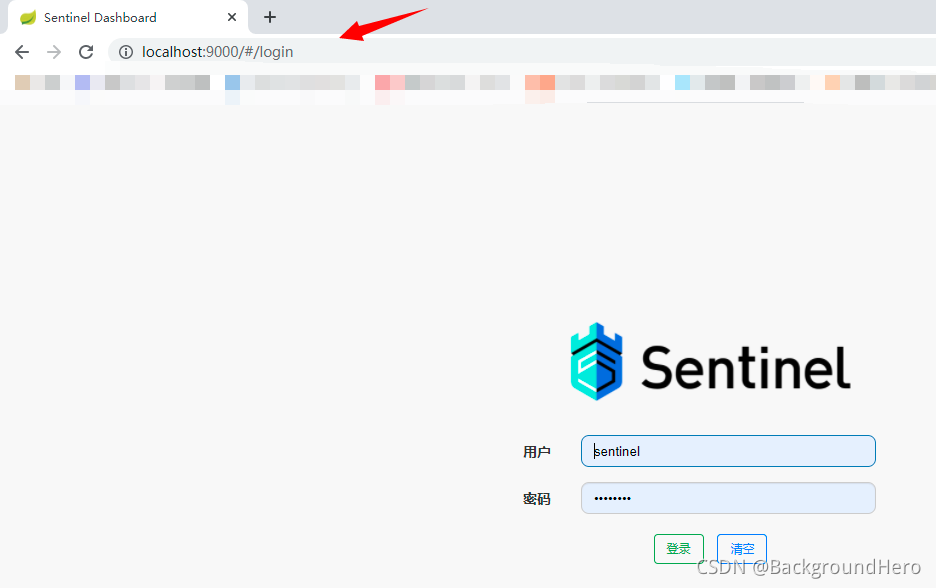
启动gateway 在启动参数里加入-Dcsp.sentinel.app.type=1 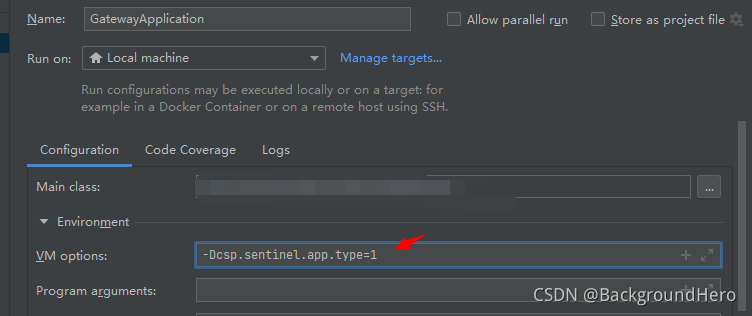 告诉sentinel表明是gateway模式
登录sentinel,默认用户名密码是sentinel-sentinel 也可以自己在配置文件处修改 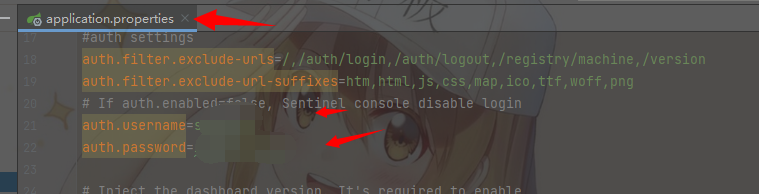
登录进去可以看到监听到的网关服务 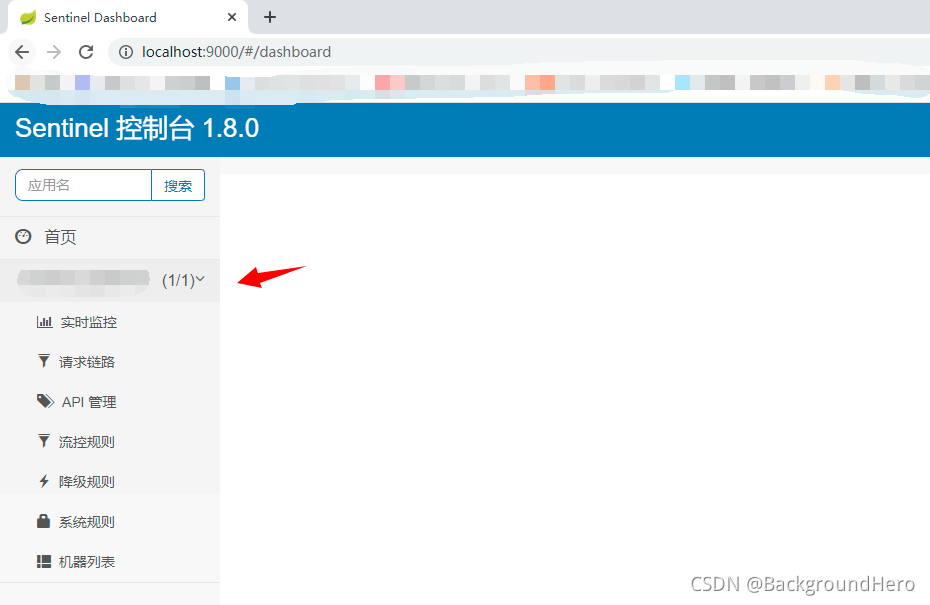 当前配置都为空 
创建一个试试 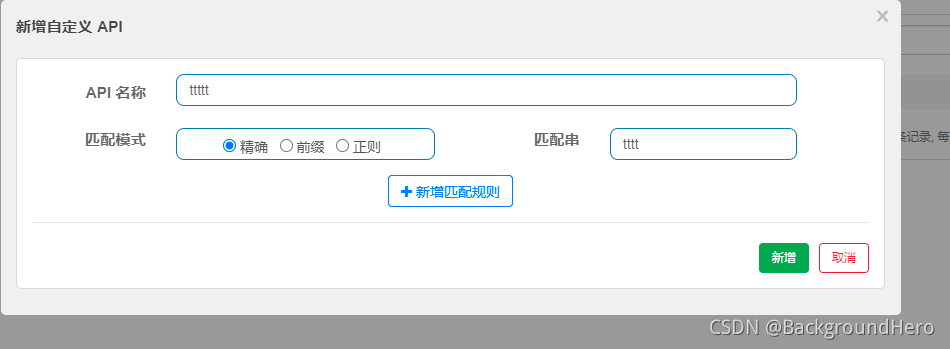  去nacos看看  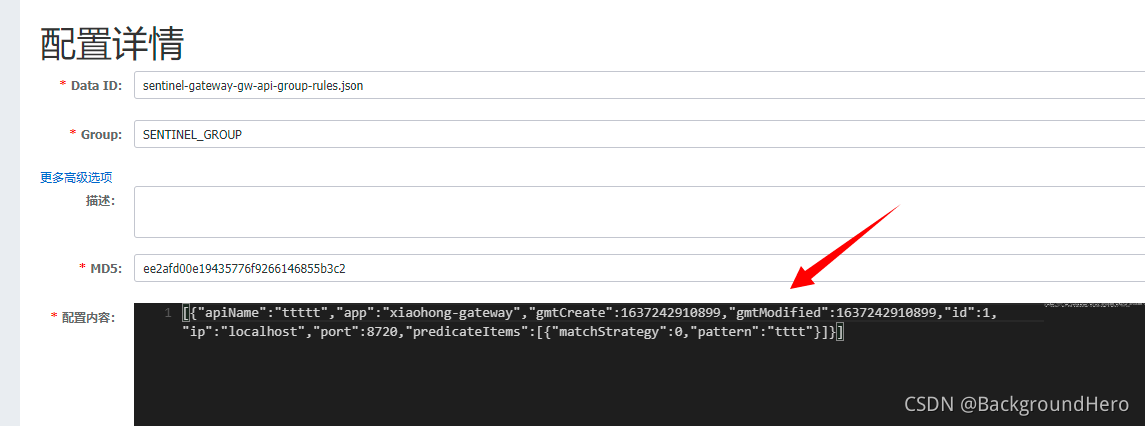 可以看到配置已经写进来了 修改试试 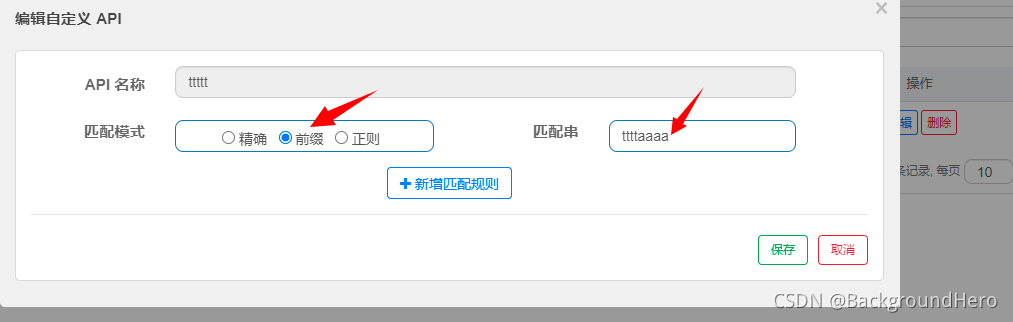 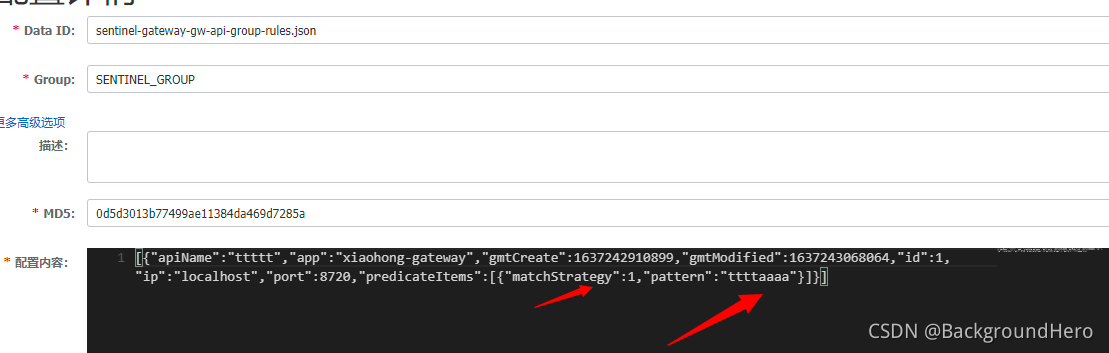 nacos也同步修改了
此刻也就可以将修改好的sentinel-dashboard打包了 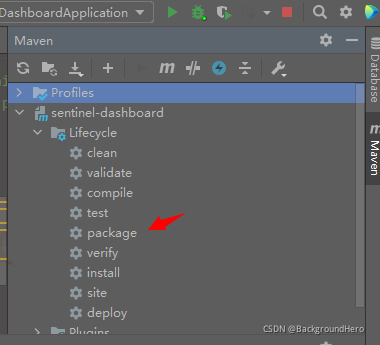
当然 为避免每次切换环境都需要修改源码,可以将sentinel也注册到nacos管理,把nacos作为配置中心,把一些动态修改的配置放到nacos中 这里就不演示了 掉头发了 睡觉了
原创不易,求个赞不过分吧~
|