用java来模拟抢手气红包,多线程
在上一个模拟微信抢红包的基础上,添加多线程
代码
import java.math.BigDecimal;
import java.util.Scanner;
public class qianhoubao {
public static void main(String[] args) {
System.out.println("-----模拟微信发拼手气红包-----");
Scanner sc = new Scanner(System.in);
System.out.println("请输入所发红包的总金额");
double money= sc.nextDouble();
System.out.println("请输入群成员个数");
int people = sc.nextInt();
String[] name = new String[people];
for (int i=0;i<people;i++){
System.out.println("请输入群成员名字");
name[i] = sc.next();
}
System.out.println("请输入所发红包的个数");
int num=sc.nextInt();
if (money / num == 0.01) {
for (int i = 0; i <people; i++) {
System.out.println(name[i] + "抢到红包红包0.01元");
}
} else if (money / num < 0.01) {
System.out.println("金额过小或个数过大,不合理!,请重新输入!");
} else {
Hongbao n=new Hongbao(num,money);
System.out.println("抢红包开始,红包总共"+money+"元");
for (int i = 0; i <people; i++) {
new Thread(new People(i, n,name)).start();
}
}
}
}
class Hongbao{
int num;
double money;
public Hongbao(int num,double money){
this.num=num;
this.money=new BigDecimal(money).setScale(2,BigDecimal.ROUND_HALF_UP).doubleValue();
}
synchronized public double qiang(){
double ramoney;
if(num>1){
ramoney=new BigDecimal(Math.random()*money).setScale(2,BigDecimal.ROUND_HALF_UP).doubleValue();
money-=ramoney;
num--;
}else if (num==1){
ramoney=new BigDecimal(money).setScale(2,BigDecimal.ROUND_HALF_UP).doubleValue();
money=0;
num--;
}else {
ramoney=0;
}
return ramoney;
}
}
class People implements Runnable{
int id;
Hongbao n;
String[] name;
double idmoney;
public People(int id,Hongbao n ,String[] name){
this.id=id;
this.n=n;
this.name=name;
}
@Override
public void run() {
System.out.println(name[id]+",开始抢红包");
try{
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
idmoney=n.qiang();
if(idmoney>0){
System.out.println("恭喜,"+name[id]+"抢到"+idmoney+"元");
}else {
System.out.println("很遗憾,"+name[id]+"手速慢了,未抢到红包");
}
}
}
实验结果:
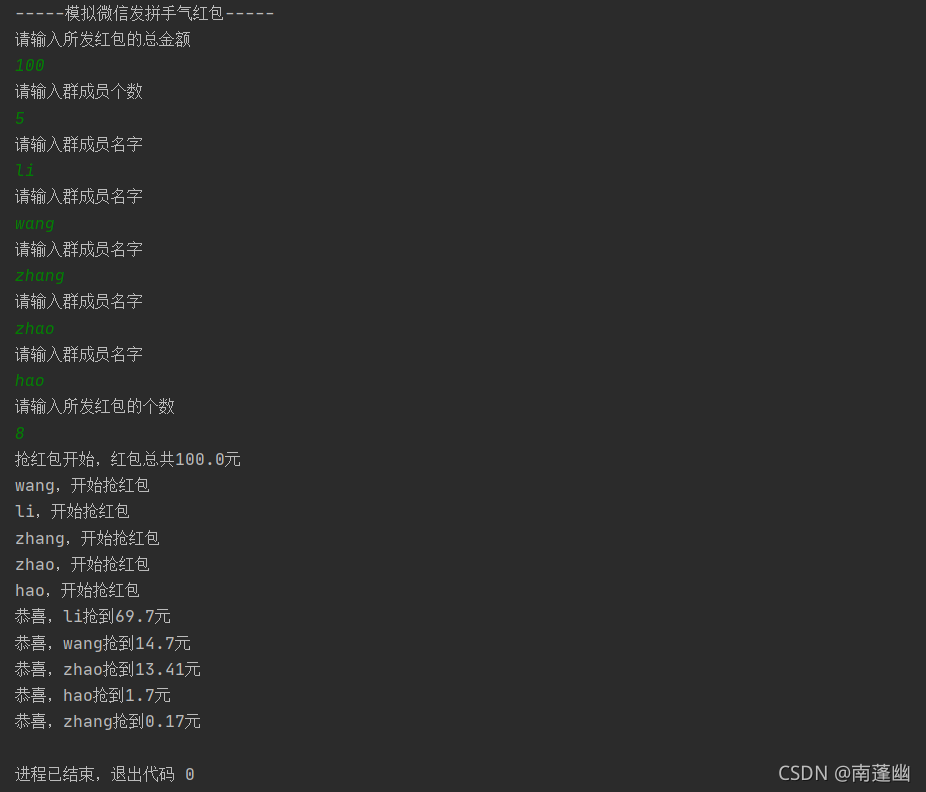
|