this关键字
内容1
package com.bjpowernode.javase.test001;
public class Customer {
String name;
public Customer() {
}
public void shopping() {
System.out.println(this.name + "在购物!");
}
public static void doSome() {
}
public static void doOther() {
Customer c = new Customer();
System.out.println(c.name);
}
}
package com.bjpowernode.javase.test001;
public class CustomerTest {
public static void main(String[] args) {
Customer c1 = new Customer();
c1.name = "zhangsan";
c1.shopping();
Customer c2 = new Customer();
c2.name = "lisi";
c2.shopping();
Customer.doSome();
Customer.doOther();
}
}
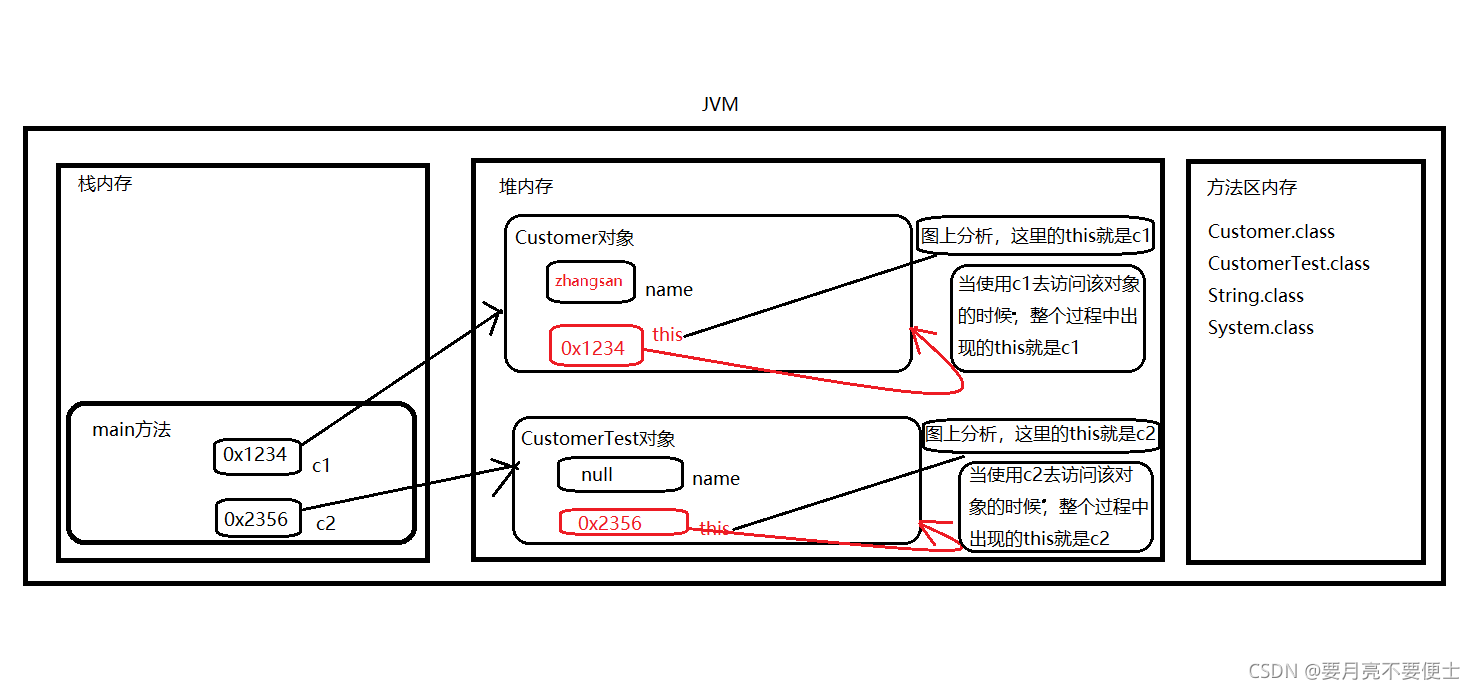
内容2
package com.bjpowernode.javase.test002;
public class ThisTest {
int num = 10;
public static void main(String[] args) {
ThisTest tt = new ThisTest();
System.out.println(tt.num);
}
}
内容3
package com.bjpowernode.javase.test003;
public class ThisTest {
public static void main(String[] args) {
ThisTest.doSome();
doSome();
ThisTest tt = new ThisTest();
tt.doOther();
tt.run();
}
public static void doSome() {
System.out.println("do Some!");
}
public void doOther() {
System.out.println("do Other!");
}
public void run() {
System.out.println("run execute!");
doOther();
this.doOther();
}
}
package com.bjpowernode.javase.test003;
public class ThisTest2 {
String name;
public void doSome() {
}
public static void main(String[] args) {
ThisTest2 tt = new ThisTest2();
tt.doSome();
System.out.println(tt.name);
}
}
内容4
package com.bjpowernode.javase.test004;
public class User {
private int id;
private String name;
public User() {
}
public User(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
package com.bjpowernode.javase.test004;
public class UserTest {
public static void main(String[] args) {
User u1 = new User(100,"zhangsan");
System.out.println(u1.getId());
System.out.println(u1.getName());
u1.setId(200);
u1.setName("lisi");
System.out.println(u1.getId());
System.out.println(u1.getName());
}
}
内容5
package com.bjpowernode.javase.test005;
public class Date {
private int year;
private int month;
private int day;
public Date(int year, int month, int day) {
this.year = year;
this.month = month;
this.day = day;
}
public Date() {
this(1970,1,1);
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
public void print() {
System.out.println(this.year + "年" + this.month + "月" + this.day + "日");
}
}
package com.bjpowernode.javase.test005;
public class DateTest {
public static void main(String[] args) {
Date time1 = new Date();
time1.print();
Date time2 = new Date(2008,9,1);
time2.print();
}
}
内容6
package com.bjpowernode.javase.test006;
public class Test {
int i = 10;
public static void doSome() {
System.out.println("do Some!");
}
public void doOther() {
System.out.println("do Other!");
}
public static void method1() {
Test.doSome();
doSome();
Test t = new Test();
t.doOther();
System.out.println(t.i);
}
public void method2() {
Test.doSome();
doSome();
this.doOther();
doOther();
System.out.println(this.i);
System.out.println(i);
}
public static void main(String[] args) {
Test.method1();
method1();
Test t = new Test();
t.method2();
}
}
内容7
package com.bjpowernode.javase.test007;
public class Test {
public static void main(String[] args) {
Test.doSome();
doSome();
Test t = new Test();
t.doSome();
t = null;
t.doSome();
}
public static void doSome() {
System.out.println("do Some!");
}
}
|