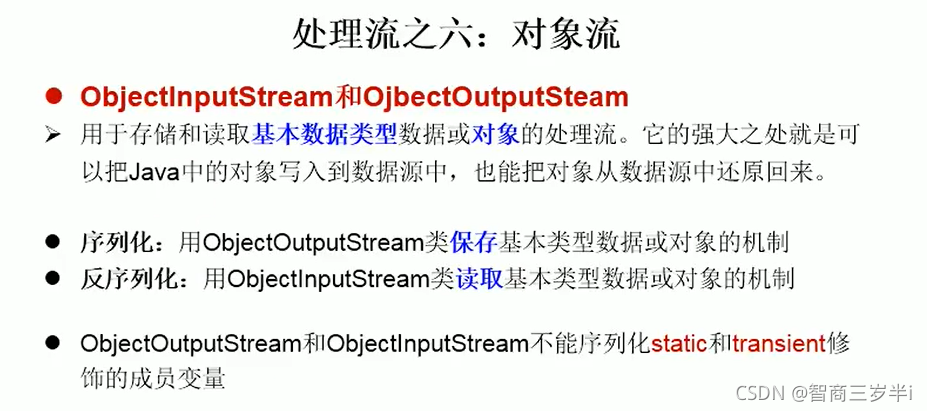
一.序列化
序列化:用ObjectOutputStream类保存基本类型数据或对象的机制 反序列化:用ObjectlnputStream类读取基本类型数据或对象的机制
对象序列化机制:把内存中的Java对象转换成平台无关的二进制流,从而把这种二进制流持久地保存在磁盘上,或通过网络将这种二进制流进行网络传输。 反序列化机制:当其它程序获取了这种二进制流,就可以恢复成原来的Java对象。 某个类的对象实现序列化:① 实现Serializable接口 ② 提供一个全局常量:private static final long serialVersionUID ③ 类中所有的属性可序列化(基本数据类型默认可序列化) 注意:被static和transient修饰的成员变量无法实例化。
@Test
public void test01(){
ObjectOutputStream oos = null;
try {
oos = new ObjectOutputStream(new FileOutputStream("E:\\java\\javaSenior\\Day_10\\data.dat"));
oos.writeObject(new String("我爱编程,我爱Java"));
oos.flush();
oos.writeObject(new Person("小王",20));
oos.flush();
oos.writeObject(new Person("小吕",18,new Account(5000)));
oos.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(oos!=null){
try {
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void test02(){
ObjectInputStream ois = null;
try {
ois = new ObjectInputStream(new FileInputStream("E:\\java\\javaSenior\\Day_10\\data.dat"));
Object o = ois.readObject();
String str = (String) o;
Person p = (Person)ois.readObject();
Person p1 = (Person)ois.readObject();
System.out.println(str);
System.out.println(p);
System.out.println(p1);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
if(ois!=null){
try {
ois.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}

二.RandomAccessFile(随机访问流)
 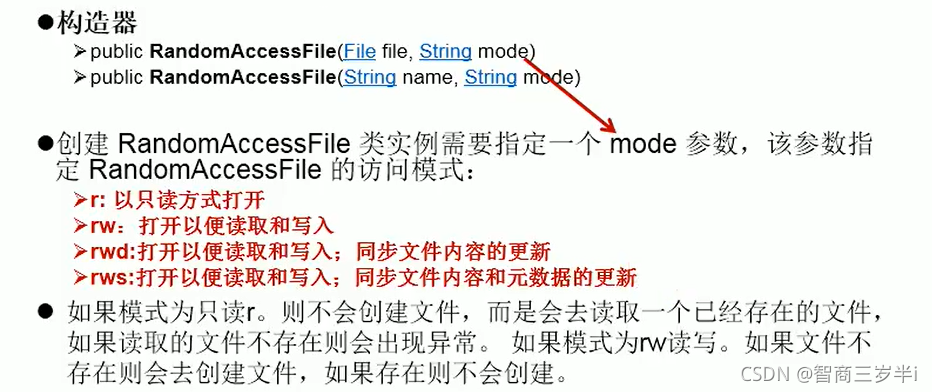 该类的实例既可以作为输入流,也可作为输出流。 作为输出流时,若文件不存在,则执行过程中自己创建,若存在,则从头开始逐个覆盖(文本文件可以查看验证)。 1.使用RandomAccessFile实现文件的复制
@Test
public void test01(){
RandomAccessFile raf1 = null;
RandomAccessFile raf2 = null;
try {
raf1 = new RandomAccessFile(new File("E:\\java\\javaSenior\\Day_10\\src\\壁纸.jpg"),"r");
raf2 = new RandomAccessFile(new File("E:\\java\\javaSenior\\Day_10\\src\\壁纸1.jpg"), "rw");
byte[] buffer = new byte[1024];
int len ;
while ((len=raf1.read(buffer))!=-1){
raf2.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(raf1!=null){
try {
raf1.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(raf2!=null){
try {
raf2.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
覆盖前:  覆盖后:  2.使用RandomAccessFile实现数据的插入: 
@Test
public void test03(){
RandomAccessFile raf = null;
try {
raf = new RandomAccessFile(new File("E:\\java\\javaSenior\\Day_10\\hello.txt"),"rw");
raf.seek(3);
StringBuilder builder = new StringBuilder((int) new File("E:\\java\\javaSenior\\Day_10\\hello.txt").length());
byte[] buffer = new byte[1024];
int len ;
while ((len=raf.read(buffer))!=-1){
builder.append(new String(buffer,0,len));
}
raf.seek(3);
raf.write("xyz".getBytes());
raf.write(builder.toString().getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
if(raf!=null){
try {
raf.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
插入前:  插入后:
三.网络编程
1.网络编程目的:直接或间接地通过网络协议与其他计算机实现数据交换,进行通信。 2.网络编程中主要有两个问题: ① 使用IP或端口号唯一标识Internet上的计算机 ② 使用TCP/IP网络传输协议进行可靠高效地进行数据传输 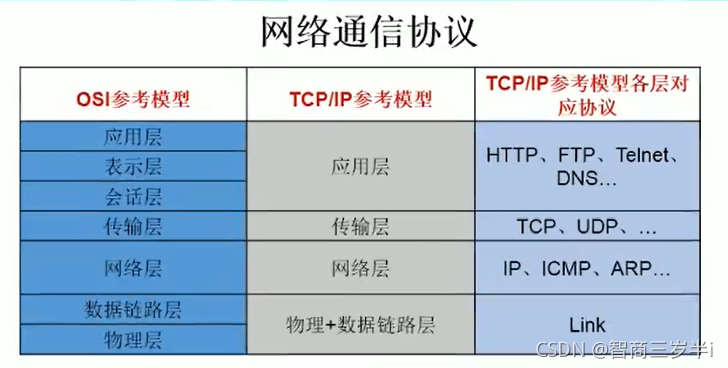 3.在Java中使用InetAddress类表示ip。 实例化InetAddress类及两个常用方法
public class NetTest {
public static void main(String[] args) {
try {
InetAddress address = InetAddress.getByName("127.0.0.1");
System.out.println(address);
InetAddress address1 = InetAddress.getByName("www.baidu.com");
System.out.println(address1);
InetAddress address2 = InetAddress.getLocalHost();
System.out.println(address2);
System.out.println(address2.getHostName());
System.out.println(address2.getHostAddress());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
4.端口号标识正在计算机上运行的唯一进程(程序),被规定为16位的整数0-65535,端口号和地址的组合得出一个网络套接字:socket 5.网络通信协议 传输层协议中有重要的协议:传输控制协议TCP、用户数据报协议UDP TCP/IP及其两个主要协议:传输控制协议(TCP)和网络互联协议(IP)
TCP协议:(类似打电话) 使用TCP协议前,须先建立TCP连接,形成传输数据通道传输前采用“三次握手”方式,点对点通信,是可靠的 TCP协议进行通信的两个应用进程:客户端、服务端。 在连接中可进行大数据量的传输 传输完毕,需释放已建立的连接,效率低 UDP协议:(类似发短信、电报) 将数据、源、目的封装成数据包,不需要建立连接 每个数据报的大小限制在64K内 发送不管对方是否准备好,接收方收到也不确认,故是不可靠的 可以广播发送 发送数据结束时无需释放资源,开销小,速度快
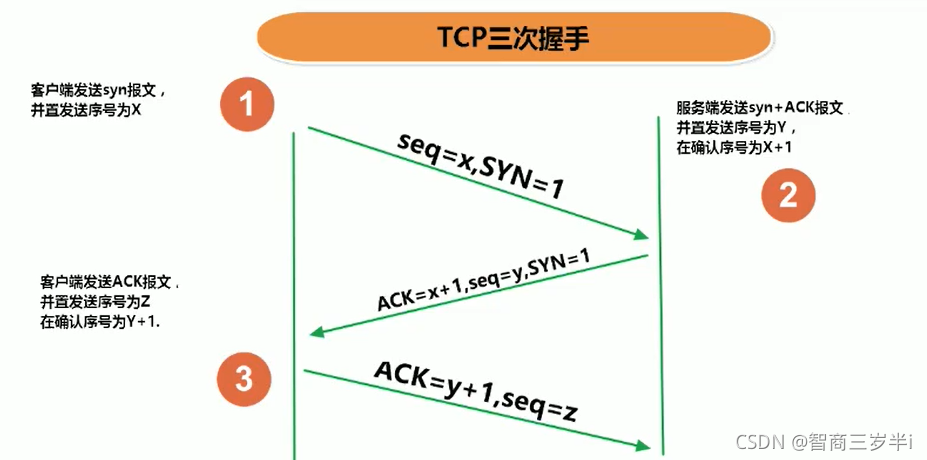 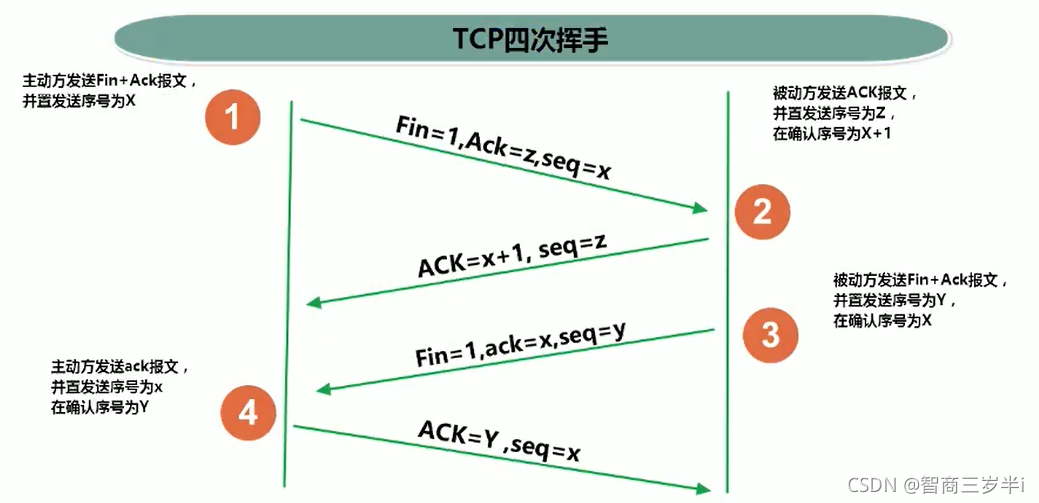 ① TCP网络编程练习: 客户端发送内容给服务端,服务端将内容打印到控制台上。
@Test
public void client(){
OutputStream os = null;
try {
InetAddress address = InetAddress.getByName("127.0.0.1");
Socket socket = new Socket(address, 8899);
os = socket.getOutputStream();
os.write("你好,我是客户端...".getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void server(){
ServerSocket ss = null;
ByteArrayOutputStream baos = null;
InputStream is = null;
Socket socket = null;
try {
ss = new ServerSocket(8899);
socket = ss.accept();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[10];
int len;
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
System.out.println("收到来自:"+socket.getInetAddress().getHostAddress()+"发送的消息...");
} catch (IOException e) {
e.printStackTrace();
} finally {
if(ss!=null){
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(baos!=null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
② TCP网络编程练习: 客户端发送文件给服务端,服务端将文件保存在本地。
@Test
public void client(){
Socket socket = null;
OutputStream os = null;
FileInputStream fis = null;
try {
socket = new Socket(InetAddress.getByName("127.0.0.1"), 8899);
os = socket.getOutputStream();
fis = new FileInputStream(new File("E:\\java\\javaSenior\\Day_10\\src\\壁纸.jpg"));
byte[] buffer = new byte[1024];
int len ;
while ((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis!=null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void server(){
ServerSocket ss =null;
Socket socket = null;
InputStream is = null;
FileOutputStream fos = null;
try {
ss = new ServerSocket(8899);
socket = ss.accept();
is = socket.getInputStream();
fos = new FileOutputStream(new File("E:\\java\\javaSenior\\Day_10\\src\\壁纸2.jpg"));
byte[] buffer = new byte[1024];
int len;
while((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
System.out.println("收到来自:"+socket.getInetAddress().getHostName()+"发送的文件...");
} catch (IOException e) {
e.printStackTrace();
} finally {
if(ss!=null){
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos!=null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
③ TCP网络编程练习: 从客户端发送文件给服务端,服务端保存到本地。返回“发送成功”给客户端,并关闭相应的连接。
@Test
public void client(){
Socket socket = null;
OutputStream os = null;
FileInputStream fis = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
socket = new Socket(InetAddress.getByName("127.0.0.1"), 8899);
os = socket.getOutputStream();
fis = new FileInputStream(new File("E:\\java\\javaSenior\\Day_10\\src\\壁纸.jpg"));
byte[] buffer = new byte[1024];
int len ;
while ((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
socket.shutdownOutput();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer1 = new byte[20];
int len1;
while ((len1=is.read(buffer1))!=-1){
baos.write(buffer1,0,len1);
}
System.out.println(baos.toString());
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis!=null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(baos!=null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void server(){
ServerSocket ss =null;
Socket socket = null;
InputStream is = null;
FileOutputStream fos = null;
OutputStream os = null;
try {
ss = new ServerSocket(8899);
socket = ss.accept();
is = socket.getInputStream();
fos = new FileOutputStream(new File("E:\\java\\javaSenior\\Day_10\\src\\壁纸3.jpg"));
byte[] buffer = new byte[1024];
int len;
while((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
os = socket.getOutputStream();
os.write("你好,图片已收到...".getBytes());
System.out.println("收到来自:"+socket.getInetAddress().getHostName()+"发送的文件...");
} catch (IOException e) {
e.printStackTrace();
} finally {
if(ss!=null){
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos!=null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
 
|