前言
为了能更好的理解Spring IoC中的依赖注入(DI),下面将会用代码实例,逐步分析DI如何在SpringBoot中运用的。
例如:人类(Person)会利用一些动物(Animal)去完成一些工作,比如狗(Dog)来看门,猫(Cat)来抓老师,鹦鹉(Parrot)来迎客…于是做一些事情就依赖于那些动物。
简单依赖注入的实例
我们先准备一些接口和实现类
public interface Person {
public void service();
public void setAnimal(Animal animal);
}
public interface Animal {
public void use();
}
@Component
public class BussninessPerson implements Person{
@Autowired
private Animal dog = null;
@Override
public void service() {
this.dog.use();
}
@Override
public void setAnimal(Animal animal) {
this.dog =animal;
}
}
@Component
public class Dog implements Animal{
@Override
public void use() {
System.out.println("狗【"+Dog.class.getSimpleName()+"】是看门用的");
}
}
对于人和动物的接口实现类
@Component
public class BussninessPerson implements Person{
@Autowired
private Animal dog = null;
@Override
public void service() {
this.dog.use();
}
@Override
public void setAnimal(Animal animal) {
this.dog =animal;
}
}
@Component
public class Dog implements Animal{
@Override
public void use() {
System.out.println("狗【"+Dog.class.getSimpleName()+"】是看门用的");
}
}
此处要注意注解@Autowired ,这是Spring中最常用的注解,十分重要的,也是本文主要讨论的一个注解之一,它会根据属性的类型(by type)找到对应的bean进行注入。
这里的Dog类是动物的一致,所以Spring的IoC容器会把Dog实例注入BussinessPerson中。
除此之外还要准备AppConfig.java
@Configuration
@ComponentScan("com.weirdo")
public class AppConfig {
}
配置AppConfig.java 的目的是替换xml配置文件,@Configuration注解是用于定义配置类
@Configuation 等价于<Beans></Beans>
@ComponentScan 等价于<context:component-scan base-package="com.weirdo"/>
测试类
@SpringBootTest
class SpringbootHolaApplicationTests {
public static void main(String[] args) {
ApplicationContext ctx =
new AnnotationConfigApplicationContext(AppConfig.class);
Person person = (Person) ctx.getBean(BussninessPerson.class);
person.service();
}
}
测试类的写法也和我们之前学过的XML的配置文件的测试写法近似,此处不做过多的解释。
输出结果如下: 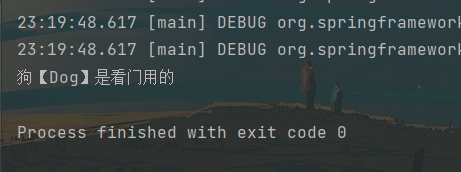 测试是成功的,这个时候 Spring IoC 容器己经通过注解@Autowired 成功地将 Dog注入到BussinessPerson 实例中 这只是一个比较简单的例子 ,我们还要进行探究@Autowired 。
@Autowired注解给谁注入?
我们知道@Autowired 的注入机制最基本的一条就是根据类型(by type),如果我们追溯源码就会知道IoC容器的顶级接口是BeanFactory,进而了解IoC容器是通过getBean方法获取Bean的,而getBean方法又支持根据类型(by type)和根据名字(by name)。
我们回到上面的简单注入例子中,我们再创建一个猫类。
@Component
public class Cat implements Animal {
@Override
public void use() {
System.out.println("猫【"+Cat.class.getSimpleName()+"】是抓老鼠用的");
}
}
ok,如果我们继续使用上面的BussinessPerson类,那就会出现一个麻烦, 这个类只定义了一个动物属性,而我们现在却有俩个动物,那么IoC会如何注入?简单测试一下 
从报错中不难看出,IoC容器并不知道我们需要注入什么动物,狗?猫?从而引发了错误,那@Autowired 这个注解还可以处理这问题吗?
当然可以,假定我们目前需要狗提供服务,那我们就将属性改为dog,即:
@Autowired
private Animal animal = null;
@Autowired
private Animal dog = null;
如此修改了属性名称后,再测试,IoC会告诉我们是狗再提供服务,那是因为@Autowired 提供这样的规则,首先它会根据类型找到对应的Bean,如果对应类型的Bean不是唯一的,那它就会根据属性名称和Bean名称进行匹配,如果匹配的上,就会使用该Bean,如果匹配不上就会抛出异常。
对了,@Autowired 是一个默认必须找到对应Bean的注解,如果不能确定标注的属性一定存在并且允许被标注的属性为null,那么可以这样设置
@Autowired(required = false)
private Animal dog = null;
同样的,它除了可以标注属性还可以标注方法,如:
@Override
@Autowired
public void setAnimal(Animal animal) {
this.dog =animal;
}
这样使用,它也会使用此方法再容器中找到对应的动物进行注入,甚至在方法的参数上使用,后面会谈到它。
消除歧义性——@Primary和@Quelifier
上面我们可以知道,有猫有狗的情况时候,为了让@Autowired 能够使用,我们将BussinessPerson的属性名称从animal修改为了dog。显然是以恶搞憋屈的做法,好好的动物,却被我们定义成了狗。注入失败的根本原因就是按类型(by type)查找,正如动物可以有多种类型,这种情况就会造成IoC容器注入的困扰,我们可以将这样的问题成为歧义性。知道这个原因后,我们就可以先从@Primary 上入手。
@Primary 注解,顾名思义,它是一个修改优先权的注解,有猫有狗的情况下,假设这次我们需要猫,只需要在猫的类定义上加入@Primary
@Component
@Primary
public class Cat implements Animal {
@Override
public void use() {
System.out.println("猫【"+Cat.class.getSimpleName()+"】是抓老鼠用的");
}
}
这里的@Primary 就相当于告诉IoC容器,当发现多个同样类型的Bean时候,优先使用我进行注入。
这时候就会有另一个问题参数,如果@Primary 也使用在多个类上,无论猫还是狗都带上了@Primary ,IoC容器又是无法区分使用哪个Bean来注入了,此时,我们有第二个注解Quelifier 就可以出场了,它的配置项value需要一个字符串去定义,它将和@Primary 组合在一起,通过类型和名称一起找到Bean
@Autowired
@Qualifier("dog")
private Animal animal = null;
这样声明后,IoC将会以类型和名称去寻找对应的Bean注入。
带有参数的构造方法类的装配
上面,我们谈到的所有情况就默认于,不带参数的构造方法下实现依赖注入。
当然我们也可以带参数来进行依赖注入,不过上面的方法都不能在使用了,比如@Autowired 注解可以对构造方法的参数进行注入。
@Component
public class BussinessPerson implements Person{
private Animal animal = null;
public BussinessPerson (@Autowired @Qualifier("dog") Animal animal){
this.animal=animal;
}
@Override
public void service() {
this.animal.use();
}
@Override
public void setAnimal( Animal animal) {
this.animal =animal;
}
}
可以看到,代码中取消了@Autowired 对属性和方法的标注,这里使用@Qualifier 是为了避免歧义性。
@Resource
@Resource 也可以进行依赖注入,但与前面俩种Spring提供的注解不同的是,@Resource 是JDK中自带的一个注解。
注解 | 说明 |
---|
@Resource | 默认通过名称注入 ,如名称无找到则通过类型注入 | @Autowired | 默认通过类型注入,如存在多个类型则通过名称注入 |
@Component(value = "bc")
public class BlackCat implements Animal{
@Override
public void use() {
}
}
@Component(value = "wc")
public class WhiteCat implements Animal{
@Override
public void use() {
}
}
@Resource(name = "wc")
private Animal animal = null;
|