mapper类:
package com.mybatis.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.mybatis.pojo.Order;
import com.mybatis.pojo.OrderExample;
public interface OrderMapper {
int countByExample(OrderExample example);
int deleteByExample(OrderExample example);
int deleteByPrimaryKey(Integer id);
int insert(Order record);
int insertSelective(Order record);
List<Order> selectByExample(OrderExample example);
Order selectByPrimaryKey(Integer id);
int updateByExampleSelective(@Param("record") Order record, @Param("example") OrderExample example);
int updateByExample(@Param("record") Order record, @Param("example") OrderExample example);
int updateByPrimaryKeySelective(Order record);
int updateByPrimaryKey(Order record);
List<Order> getOrderUserMap();
}
package com.mybatis.mapper;
import com.mybatis.pojo.User;
import com.mybatis.pojo.UserExample;
import java.util.List;
import org.apache.ibatis.annotations.Param;
public interface UserMapper {
int countByExample(UserExample example);
int deleteByExample(UserExample example);
int deleteByPrimaryKey(Integer id);
int insert(User record);
int insertSelective(User record);
List<User> selectByExample(UserExample example);
User selectByPrimaryKey(Integer id);
int updateByExampleSelective(@Param("record") User record, @Param("example") UserExample example);
int updateByExample(@Param("record") User record, @Param("example") UserExample example);
int updateByPrimaryKeySelective(User record);
int updateByPrimaryKey(User record);
List<User> getUserOrderMap();
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.mybatis.mapper.OrderMapper" >
<resultMap id="BaseResultMap" type="com.mybatis.pojo.Order" >
<id column="id" property="id" jdbcType="INTEGER" />
<result column="user_id" property="userId" jdbcType="INTEGER" />
<result column="number" property="number" jdbcType="VARCHAR" />
<result column="createtime" property="createtime" jdbcType="TIMESTAMP" />
<result column="note" property="note" jdbcType="VARCHAR" />
</resultMap>
<sql id="Example_Where_Clause" >
<where >
<foreach collection="oredCriteria" item="criteria" separator="or" >
<if test="criteria.valid" >
<trim prefix="(" suffix=")" prefixOverrides="and" >
<foreach collection="criteria.criteria" item="criterion" >
<choose >
<when test="criterion.noValue" >
and ${criterion.condition}
</when>
<when test="criterion.singleValue" >
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue" >
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue" >
and ${criterion.condition}
<foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," >
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Update_By_Example_Where_Clause" >
<where >
<foreach collection="example.oredCriteria" item="criteria" separator="or" >
<if test="criteria.valid" >
<trim prefix="(" suffix=")" prefixOverrides="and" >
<foreach collection="criteria.criteria" item="criterion" >
<choose >
<when test="criterion.noValue" >
and ${criterion.condition}
</when>
<when test="criterion.singleValue" >
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue" >
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue" >
and ${criterion.condition}
<foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," >
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Base_Column_List" >
id, user_id, number, createtime, note
</sql>
<select id="selectByExample" resultMap="BaseResultMap" parameterType="com.mybatis.pojo.OrderExample" >
select
<if test="distinct" >
distinct
</if>
<include refid="Base_Column_List" />
from order
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
<if test="orderByClause != null" >
order by ${orderByClause}
</if>
</select>
<select id="selectByPrimaryKey" resultMap="BaseResultMap" parameterType="java.lang.Integer" >
select
<include refid="Base_Column_List" />
from order
where id = #{id,jdbcType=INTEGER}
</select>
<delete id="deleteByPrimaryKey" parameterType="java.lang.Integer" >
delete from order
where id = #{id,jdbcType=INTEGER}
</delete>
<delete id="deleteByExample" parameterType="com.mybatis.pojo.OrderExample" >
delete from order
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
</delete>
<insert id="insert" parameterType="com.mybatis.pojo.Order" >
insert into order (id, user_id, number,
createtime, note)
values (#{id,jdbcType=INTEGER}, #{userId,jdbcType=INTEGER}, #{number,jdbcType=VARCHAR},
#{createtime,jdbcType=TIMESTAMP}, #{note,jdbcType=VARCHAR})
</insert>
<insert id="insertSelective" parameterType="com.mybatis.pojo.Order" >
insert into order
<trim prefix="(" suffix=")" suffixOverrides="," >
<if test="id != null" >
id,
</if>
<if test="userId != null" >
user_id,
</if>
<if test="number != null" >
number,
</if>
<if test="createtime != null" >
createtime,
</if>
<if test="note != null" >
note,
</if>
</trim>
<trim prefix="values (" suffix=")" suffixOverrides="," >
<if test="id != null" >
#{id,jdbcType=INTEGER},
</if>
<if test="userId != null" >
#{userId,jdbcType=INTEGER},
</if>
<if test="number != null" >
#{number,jdbcType=VARCHAR},
</if>
<if test="createtime != null" >
#{createtime,jdbcType=TIMESTAMP},
</if>
<if test="note != null" >
#{note,jdbcType=VARCHAR},
</if>
</trim>
</insert>
<select id="countByExample" parameterType="com.mybatis.pojo.OrderExample" resultType="java.lang.Integer" >
select count(*) from order
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
</select>
<update id="updateByExampleSelective" parameterType="map" >
update order
<set >
<if test="record.id != null" >
id = #{record.id,jdbcType=INTEGER},
</if>
<if test="record.userId != null" >
user_id = #{record.userId,jdbcType=INTEGER},
</if>
<if test="record.number != null" >
number = #{record.number,jdbcType=VARCHAR},
</if>
<if test="record.createtime != null" >
createtime = #{record.createtime,jdbcType=TIMESTAMP},
</if>
<if test="record.note != null" >
note = #{record.note,jdbcType=VARCHAR},
</if>
</set>
<if test="_parameter != null" >
<include refid="Update_By_Example_Where_Clause" />
</if>
</update>
<update id="updateByExample" parameterType="map" >
update order
set id = #{record.id,jdbcType=INTEGER},
user_id = #{record.userId,jdbcType=INTEGER},
number = #{record.number,jdbcType=VARCHAR},
createtime = #{record.createtime,jdbcType=TIMESTAMP},
note = #{record.note,jdbcType=VARCHAR}
<if test="_parameter != null" >
<include refid="Update_By_Example_Where_Clause" />
</if>
</update>
<update id="updateByPrimaryKeySelective" parameterType="com.mybatis.pojo.Order" >
update order
<set >
<if test="userId != null" >
user_id = #{userId,jdbcType=INTEGER},
</if>
<if test="number != null" >
number = #{number,jdbcType=VARCHAR},
</if>
<if test="createtime != null" >
createtime = #{createtime,jdbcType=TIMESTAMP},
</if>
<if test="note != null" >
note = #{note,jdbcType=VARCHAR},
</if>
</set>
where id = #{id,jdbcType=INTEGER}
</update>
<update id="updateByPrimaryKey" parameterType="com.mybatis.pojo.Order" >
update order
set user_id = #{userId,jdbcType=INTEGER},
number = #{number,jdbcType=VARCHAR},
createtime = #{createtime,jdbcType=TIMESTAMP},
note = #{note,jdbcType=VARCHAR}
where id = #{id,jdbcType=INTEGER}
</update>
<!-- 自编写代码 -->
<resultMap type="order" id="order_user_map">
<!-- <id>用于映射主键 -->
<id property="id" column="id"/>
<!-- 普通字段用<result>映射 -->
<result property="userId" column="user_id"/>
<result property="number" column="number"/>
<result property="createtime" column="createtime"/>
<result property="note" column="note"/>
<!-- association用于配置一对一关系
property:order里面的User属性
javaType:user的数据类型,支持别名
-->
<association property="user" javaType="com.mybatis.pojo.User">
<id property="id" column="user_id"/>
<result property="username" column="username"/>
<result property="address" column="address"/>
<result property="birthday" column="birthday"/>
<result property="sex" column="sex"/>
</association>
</resultMap>
<!-- 一对一关联查询:resultMap使用 -->
<select id="getOrderUserMap" resultMap="order_user_map">
SELECT
o.`id`,
o.`user_id`,
o.`number`,
o.`createtime`,
o.`note`,
u.username,
u.address,
u.birthday,
u.sex
FROM
`order` o
LEFT JOIN `user` u
ON u.id = o.user_id
</select>
</mapper>
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.mybatis.mapper.UserMapper" >
<resultMap id="BaseResultMap" type="com.mybatis.pojo.User" >
<id column="id" property="id" jdbcType="INTEGER" />
<result column="username" property="username" jdbcType="VARCHAR" />
<result column="birthday" property="birthday" jdbcType="DATE" />
<result column="sex" property="sex" jdbcType="CHAR" />
<result column="address" property="address" jdbcType="VARCHAR" />
</resultMap>
<sql id="Example_Where_Clause" >
<where >
<foreach collection="oredCriteria" item="criteria" separator="or" >
<if test="criteria.valid" >
<trim prefix="(" suffix=")" prefixOverrides="and" >
<foreach collection="criteria.criteria" item="criterion" >
<choose >
<when test="criterion.noValue" >
and ${criterion.condition}
</when>
<when test="criterion.singleValue" >
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue" >
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue" >
and ${criterion.condition}
<foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," >
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Update_By_Example_Where_Clause" >
<where >
<foreach collection="example.oredCriteria" item="criteria" separator="or" >
<if test="criteria.valid" >
<trim prefix="(" suffix=")" prefixOverrides="and" >
<foreach collection="criteria.criteria" item="criterion" >
<choose >
<when test="criterion.noValue" >
and ${criterion.condition}
</when>
<when test="criterion.singleValue" >
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue" >
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue" >
and ${criterion.condition}
<foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," >
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Base_Column_List" >
id, username, birthday, sex, address
</sql>
<select id="selectByExample" resultMap="BaseResultMap" parameterType="com.mybatis.pojo.UserExample" >
select
<if test="distinct" >
distinct
</if>
<include refid="Base_Column_List" />
from user
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
<if test="orderByClause != null" >
order by ${orderByClause}
</if>
</select>
<select id="selectByPrimaryKey" resultMap="BaseResultMap" parameterType="java.lang.Integer" >
select
<include refid="Base_Column_List" />
from user
where id = #{id,jdbcType=INTEGER}
</select>
<delete id="deleteByPrimaryKey" parameterType="java.lang.Integer" >
delete from user
where id = #{id,jdbcType=INTEGER}
</delete>
<delete id="deleteByExample" parameterType="com.mybatis.pojo.UserExample" >
delete from user
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
</delete>
<insert id="insert" parameterType="com.mybatis.pojo.User" >
insert into user (id, username, birthday,
sex, address)
values (#{id,jdbcType=INTEGER}, #{username,jdbcType=VARCHAR}, #{birthday,jdbcType=DATE},
#{sex,jdbcType=CHAR}, #{address,jdbcType=VARCHAR})
</insert>
<insert id="insertSelective" parameterType="com.mybatis.pojo.User" >
insert into user
<trim prefix="(" suffix=")" suffixOverrides="," >
<if test="id != null" >
id,
</if>
<if test="username != null" >
username,
</if>
<if test="birthday != null" >
birthday,
</if>
<if test="sex != null" >
sex,
</if>
<if test="address != null" >
address,
</if>
</trim>
<trim prefix="values (" suffix=")" suffixOverrides="," >
<if test="id != null" >
#{id,jdbcType=INTEGER},
</if>
<if test="username != null" >
#{username,jdbcType=VARCHAR},
</if>
<if test="birthday != null" >
#{birthday,jdbcType=DATE},
</if>
<if test="sex != null" >
#{sex,jdbcType=CHAR},
</if>
<if test="address != null" >
#{address,jdbcType=VARCHAR},
</if>
</trim>
</insert>
<select id="countByExample" parameterType="com.mybatis.pojo.UserExample" resultType="java.lang.Integer" >
select count(*) from user
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
</select>
<update id="updateByExampleSelective" parameterType="map" >
update user
<set >
<if test="record.id != null" >
id = #{record.id,jdbcType=INTEGER},
</if>
<if test="record.username != null" >
username = #{record.username,jdbcType=VARCHAR},
</if>
<if test="record.birthday != null" >
birthday = #{record.birthday,jdbcType=DATE},
</if>
<if test="record.sex != null" >
sex = #{record.sex,jdbcType=CHAR},
</if>
<if test="record.address != null" >
address = #{record.address,jdbcType=VARCHAR},
</if>
</set>
<if test="_parameter != null" >
<include refid="Update_By_Example_Where_Clause" />
</if>
</update>
<update id="updateByExample" parameterType="map" >
update user
set id = #{record.id,jdbcType=INTEGER},
username = #{record.username,jdbcType=VARCHAR},
birthday = #{record.birthday,jdbcType=DATE},
sex = #{record.sex,jdbcType=CHAR},
address = #{record.address,jdbcType=VARCHAR}
<if test="_parameter != null" >
<include refid="Update_By_Example_Where_Clause" />
</if>
</update>
<update id="updateByPrimaryKeySelective" parameterType="com.mybatis.pojo.User" >
update user
<set >
<if test="username != null" >
username = #{username,jdbcType=VARCHAR},
</if>
<if test="birthday != null" >
birthday = #{birthday,jdbcType=DATE},
</if>
<if test="sex != null" >
sex = #{sex,jdbcType=CHAR},
</if>
<if test="address != null" >
address = #{address,jdbcType=VARCHAR},
</if>
</set>
where id = #{id,jdbcType=INTEGER}
</update>
<update id="updateByPrimaryKey" parameterType="com.mybatis.pojo.User" >
update user
set username = #{username,jdbcType=VARCHAR},
birthday = #{birthday,jdbcType=DATE},
sex = #{sex,jdbcType=CHAR},
address = #{address,jdbcType=VARCHAR}
where id = #{id,jdbcType=INTEGER}
</update>
<!-- 自己编写 -->
<resultMap type="user" id="user_order_map">
<id property="id" column="id" />
<result property="username" column="username" />
<result property="address" column="address" />
<result property="birthday" column="birthday" />
<result property="sex" column="sex" />
<!-- collection用于配置一对多关联
property:User当中Order的属性
ofType:orders的数据类型,支持别名
-->
<collection property="orders" ofType="com.mybatis.pojo.Order">
<!-- <id>用于映射主键 -->
<id property="id" column="oid"/>
<!-- 普通字段用<result>映射 -->
<result property="userId" column="id"/>
<result property="number" column="number"/>
<result property="createtime" column="createtime"/>
<result property="note" column="note"/>
</collection>
</resultMap>
<select id="getUserOrderMap" resultMap="user_order_map">
SELECT
u.`id`,
u.`username`,
u.`birthday`,
u.`sex`,
u.`address`,
o.`id` oid,
o.`number`,
o.`createtime`,
o.`note`
FROM
`user` u
LEFT JOIN `order` o
ON o.`user_id` = u.`id`
</select>
</mapper>
pojo类:
package com.mybatis.pojo;
import java.util.Date;
import com.mybatis.pojo.User;
public class Order {
@Override
public String toString() {
return "Order [id=" + id + ", userId=" + userId + ", number=" + number + ", createtime=" + createtime
+ ", note=" + note + ", user=" + user + "]";
}
private Integer id;
private Integer userId;
private String number;
private Date createtime;
private String note;
private User user;
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getUserId() {
return userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number == null ? null : number.trim();
}
public Date getCreatetime() {
return createtime;
}
public void setCreatetime(Date createtime) {
this.createtime = createtime;
}
public String getNote() {
return note;
}
public void setNote(String note) {
this.note = note == null ? null : note.trim();
}
}
package com.mybatis.pojo;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class OrderExample {
protected String orderByClause;
protected boolean distinct;
protected List<Criteria> oredCriteria;
public OrderExample() {
oredCriteria = new ArrayList<Criteria>();
}
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
public String getOrderByClause() {
return orderByClause;
}
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
public boolean isDistinct() {
return distinct;
}
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andIdIsNull() {
addCriterion("id is null");
return (Criteria) this;
}
public Criteria andIdIsNotNull() {
addCriterion("id is not null");
return (Criteria) this;
}
public Criteria andIdEqualTo(Integer value) {
addCriterion("id =", value, "id");
return (Criteria) this;
}
public Criteria andIdNotEqualTo(Integer value) {
addCriterion("id <>", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThan(Integer value) {
addCriterion("id >", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThanOrEqualTo(Integer value) {
addCriterion("id >=", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThan(Integer value) {
addCriterion("id <", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThanOrEqualTo(Integer value) {
addCriterion("id <=", value, "id");
return (Criteria) this;
}
public Criteria andIdIn(List<Integer> values) {
addCriterion("id in", values, "id");
return (Criteria) this;
}
public Criteria andIdNotIn(List<Integer> values) {
addCriterion("id not in", values, "id");
return (Criteria) this;
}
public Criteria andIdBetween(Integer value1, Integer value2) {
addCriterion("id between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andIdNotBetween(Integer value1, Integer value2) {
addCriterion("id not between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andUserIdIsNull() {
addCriterion("user_id is null");
return (Criteria) this;
}
public Criteria andUserIdIsNotNull() {
addCriterion("user_id is not null");
return (Criteria) this;
}
public Criteria andUserIdEqualTo(Integer value) {
addCriterion("user_id =", value, "userId");
return (Criteria) this;
}
public Criteria andUserIdNotEqualTo(Integer value) {
addCriterion("user_id <>", value, "userId");
return (Criteria) this;
}
public Criteria andUserIdGreaterThan(Integer value) {
addCriterion("user_id >", value, "userId");
return (Criteria) this;
}
public Criteria andUserIdGreaterThanOrEqualTo(Integer value) {
addCriterion("user_id >=", value, "userId");
return (Criteria) this;
}
public Criteria andUserIdLessThan(Integer value) {
addCriterion("user_id <", value, "userId");
return (Criteria) this;
}
public Criteria andUserIdLessThanOrEqualTo(Integer value) {
addCriterion("user_id <=", value, "userId");
return (Criteria) this;
}
public Criteria andUserIdIn(List<Integer> values) {
addCriterion("user_id in", values, "userId");
return (Criteria) this;
}
public Criteria andUserIdNotIn(List<Integer> values) {
addCriterion("user_id not in", values, "userId");
return (Criteria) this;
}
public Criteria andUserIdBetween(Integer value1, Integer value2) {
addCriterion("user_id between", value1, value2, "userId");
return (Criteria) this;
}
public Criteria andUserIdNotBetween(Integer value1, Integer value2) {
addCriterion("user_id not between", value1, value2, "userId");
return (Criteria) this;
}
public Criteria andNumberIsNull() {
addCriterion("number is null");
return (Criteria) this;
}
public Criteria andNumberIsNotNull() {
addCriterion("number is not null");
return (Criteria) this;
}
public Criteria andNumberEqualTo(String value) {
addCriterion("number =", value, "number");
return (Criteria) this;
}
public Criteria andNumberNotEqualTo(String value) {
addCriterion("number <>", value, "number");
return (Criteria) this;
}
public Criteria andNumberGreaterThan(String value) {
addCriterion("number >", value, "number");
return (Criteria) this;
}
public Criteria andNumberGreaterThanOrEqualTo(String value) {
addCriterion("number >=", value, "number");
return (Criteria) this;
}
public Criteria andNumberLessThan(String value) {
addCriterion("number <", value, "number");
return (Criteria) this;
}
public Criteria andNumberLessThanOrEqualTo(String value) {
addCriterion("number <=", value, "number");
return (Criteria) this;
}
public Criteria andNumberLike(String value) {
addCriterion("number like", value, "number");
return (Criteria) this;
}
public Criteria andNumberNotLike(String value) {
addCriterion("number not like", value, "number");
return (Criteria) this;
}
public Criteria andNumberIn(List<String> values) {
addCriterion("number in", values, "number");
return (Criteria) this;
}
public Criteria andNumberNotIn(List<String> values) {
addCriterion("number not in", values, "number");
return (Criteria) this;
}
public Criteria andNumberBetween(String value1, String value2) {
addCriterion("number between", value1, value2, "number");
return (Criteria) this;
}
public Criteria andNumberNotBetween(String value1, String value2) {
addCriterion("number not between", value1, value2, "number");
return (Criteria) this;
}
public Criteria andCreatetimeIsNull() {
addCriterion("createtime is null");
return (Criteria) this;
}
public Criteria andCreatetimeIsNotNull() {
addCriterion("createtime is not null");
return (Criteria) this;
}
public Criteria andCreatetimeEqualTo(Date value) {
addCriterion("createtime =", value, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeNotEqualTo(Date value) {
addCriterion("createtime <>", value, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeGreaterThan(Date value) {
addCriterion("createtime >", value, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeGreaterThanOrEqualTo(Date value) {
addCriterion("createtime >=", value, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeLessThan(Date value) {
addCriterion("createtime <", value, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeLessThanOrEqualTo(Date value) {
addCriterion("createtime <=", value, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeIn(List<Date> values) {
addCriterion("createtime in", values, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeNotIn(List<Date> values) {
addCriterion("createtime not in", values, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeBetween(Date value1, Date value2) {
addCriterion("createtime between", value1, value2, "createtime");
return (Criteria) this;
}
public Criteria andCreatetimeNotBetween(Date value1, Date value2) {
addCriterion("createtime not between", value1, value2, "createtime");
return (Criteria) this;
}
public Criteria andNoteIsNull() {
addCriterion("note is null");
return (Criteria) this;
}
public Criteria andNoteIsNotNull() {
addCriterion("note is not null");
return (Criteria) this;
}
public Criteria andNoteEqualTo(String value) {
addCriterion("note =", value, "note");
return (Criteria) this;
}
public Criteria andNoteNotEqualTo(String value) {
addCriterion("note <>", value, "note");
return (Criteria) this;
}
public Criteria andNoteGreaterThan(String value) {
addCriterion("note >", value, "note");
return (Criteria) this;
}
public Criteria andNoteGreaterThanOrEqualTo(String value) {
addCriterion("note >=", value, "note");
return (Criteria) this;
}
public Criteria andNoteLessThan(String value) {
addCriterion("note <", value, "note");
return (Criteria) this;
}
public Criteria andNoteLessThanOrEqualTo(String value) {
addCriterion("note <=", value, "note");
return (Criteria) this;
}
public Criteria andNoteLike(String value) {
addCriterion("note like", value, "note");
return (Criteria) this;
}
public Criteria andNoteNotLike(String value) {
addCriterion("note not like", value, "note");
return (Criteria) this;
}
public Criteria andNoteIn(List<String> values) {
addCriterion("note in", values, "note");
return (Criteria) this;
}
public Criteria andNoteNotIn(List<String> values) {
addCriterion("note not in", values, "note");
return (Criteria) this;
}
public Criteria andNoteBetween(String value1, String value2) {
addCriterion("note between", value1, value2, "note");
return (Criteria) this;
}
public Criteria andNoteNotBetween(String value1, String value2) {
addCriterion("note not between", value1, value2, "note");
return (Criteria) this;
}
}
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}
package com.mybatis.pojo;
import java.util.Date;
import java.util.List;
import com.mybatis.pojo.Order;
public class User {
private Integer id;
private String username;
private Date birthday;
private String sex;
private String address;
private List<Order> orders;
public List<Order> getOrders() {
return orders;
}
public void setOrders(List<Order> orders) {
this.orders = orders;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username == null ? null : username.trim();
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex == null ? null : sex.trim();
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address == null ? null : address.trim();
}
@Override
public String toString() {
return "User [id=" + id + ", username=" + username + ", birthday=" + birthday + ", sex=" + sex + ", address="
+ address + "]";
}
}
package com.mybatis.pojo;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
public class UserExample {
protected String orderByClause;
protected boolean distinct;
protected List<Criteria> oredCriteria;
public UserExample() {
oredCriteria = new ArrayList<Criteria>();
}
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
public String getOrderByClause() {
return orderByClause;
}
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
public boolean isDistinct() {
return distinct;
}
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
protected void addCriterionForJDBCDate(String condition, Date value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
addCriterion(condition, new java.sql.Date(value.getTime()), property);
}
protected void addCriterionForJDBCDate(String condition, List<Date> values, String property) {
if (values == null || values.size() == 0) {
throw new RuntimeException("Value list for " + property + " cannot be null or empty");
}
List<java.sql.Date> dateList = new ArrayList<java.sql.Date>();
Iterator<Date> iter = values.iterator();
while (iter.hasNext()) {
dateList.add(new java.sql.Date(iter.next().getTime()));
}
addCriterion(condition, dateList, property);
}
protected void addCriterionForJDBCDate(String condition, Date value1, Date value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
addCriterion(condition, new java.sql.Date(value1.getTime()), new java.sql.Date(value2.getTime()), property);
}
public Criteria andIdIsNull() {
addCriterion("id is null");
return (Criteria) this;
}
public Criteria andIdIsNotNull() {
addCriterion("id is not null");
return (Criteria) this;
}
public Criteria andIdEqualTo(Integer value) {
addCriterion("id =", value, "id");
return (Criteria) this;
}
public Criteria andIdNotEqualTo(Integer value) {
addCriterion("id <>", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThan(Integer value) {
addCriterion("id >", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThanOrEqualTo(Integer value) {
addCriterion("id >=", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThan(Integer value) {
addCriterion("id <", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThanOrEqualTo(Integer value) {
addCriterion("id <=", value, "id");
return (Criteria) this;
}
public Criteria andIdIn(List<Integer> values) {
addCriterion("id in", values, "id");
return (Criteria) this;
}
public Criteria andIdNotIn(List<Integer> values) {
addCriterion("id not in", values, "id");
return (Criteria) this;
}
public Criteria andIdBetween(Integer value1, Integer value2) {
addCriterion("id between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andIdNotBetween(Integer value1, Integer value2) {
addCriterion("id not between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andUsernameIsNull() {
addCriterion("username is null");
return (Criteria) this;
}
public Criteria andUsernameIsNotNull() {
addCriterion("username is not null");
return (Criteria) this;
}
public Criteria andUsernameEqualTo(String value) {
addCriterion("username =", value, "username");
return (Criteria) this;
}
public Criteria andUsernameNotEqualTo(String value) {
addCriterion("username <>", value, "username");
return (Criteria) this;
}
public Criteria andUsernameGreaterThan(String value) {
addCriterion("username >", value, "username");
return (Criteria) this;
}
public Criteria andUsernameGreaterThanOrEqualTo(String value) {
addCriterion("username >=", value, "username");
return (Criteria) this;
}
public Criteria andUsernameLessThan(String value) {
addCriterion("username <", value, "username");
return (Criteria) this;
}
public Criteria andUsernameLessThanOrEqualTo(String value) {
addCriterion("username <=", value, "username");
return (Criteria) this;
}
public Criteria andUsernameLike(String value) {
addCriterion("username like", value, "username");
return (Criteria) this;
}
public Criteria andUsernameNotLike(String value) {
addCriterion("username not like", value, "username");
return (Criteria) this;
}
public Criteria andUsernameIn(List<String> values) {
addCriterion("username in", values, "username");
return (Criteria) this;
}
public Criteria andUsernameNotIn(List<String> values) {
addCriterion("username not in", values, "username");
return (Criteria) this;
}
public Criteria andUsernameBetween(String value1, String value2) {
addCriterion("username between", value1, value2, "username");
return (Criteria) this;
}
public Criteria andUsernameNotBetween(String value1, String value2) {
addCriterion("username not between", value1, value2, "username");
return (Criteria) this;
}
public Criteria andBirthdayIsNull() {
addCriterion("birthday is null");
return (Criteria) this;
}
public Criteria andBirthdayIsNotNull() {
addCriterion("birthday is not null");
return (Criteria) this;
}
public Criteria andBirthdayEqualTo(Date value) {
addCriterionForJDBCDate("birthday =", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayNotEqualTo(Date value) {
addCriterionForJDBCDate("birthday <>", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayGreaterThan(Date value) {
addCriterionForJDBCDate("birthday >", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayGreaterThanOrEqualTo(Date value) {
addCriterionForJDBCDate("birthday >=", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayLessThan(Date value) {
addCriterionForJDBCDate("birthday <", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayLessThanOrEqualTo(Date value) {
addCriterionForJDBCDate("birthday <=", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayIn(List<Date> values) {
addCriterionForJDBCDate("birthday in", values, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayNotIn(List<Date> values) {
addCriterionForJDBCDate("birthday not in", values, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayBetween(Date value1, Date value2) {
addCriterionForJDBCDate("birthday between", value1, value2, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayNotBetween(Date value1, Date value2) {
addCriterionForJDBCDate("birthday not between", value1, value2, "birthday");
return (Criteria) this;
}
public Criteria andSexIsNull() {
addCriterion("sex is null");
return (Criteria) this;
}
public Criteria andSexIsNotNull() {
addCriterion("sex is not null");
return (Criteria) this;
}
public Criteria andSexEqualTo(String value) {
addCriterion("sex =", value, "sex");
return (Criteria) this;
}
public Criteria andSexNotEqualTo(String value) {
addCriterion("sex <>", value, "sex");
return (Criteria) this;
}
public Criteria andSexGreaterThan(String value) {
addCriterion("sex >", value, "sex");
return (Criteria) this;
}
public Criteria andSexGreaterThanOrEqualTo(String value) {
addCriterion("sex >=", value, "sex");
return (Criteria) this;
}
public Criteria andSexLessThan(String value) {
addCriterion("sex <", value, "sex");
return (Criteria) this;
}
public Criteria andSexLessThanOrEqualTo(String value) {
addCriterion("sex <=", value, "sex");
return (Criteria) this;
}
public Criteria andSexLike(String value) {
addCriterion("sex like", value, "sex");
return (Criteria) this;
}
public Criteria andSexNotLike(String value) {
addCriterion("sex not like", value, "sex");
return (Criteria) this;
}
public Criteria andSexIn(List<String> values) {
addCriterion("sex in", values, "sex");
return (Criteria) this;
}
public Criteria andSexNotIn(List<String> values) {
addCriterion("sex not in", values, "sex");
return (Criteria) this;
}
public Criteria andSexBetween(String value1, String value2) {
addCriterion("sex between", value1, value2, "sex");
return (Criteria) this;
}
public Criteria andSexNotBetween(String value1, String value2) {
addCriterion("sex not between", value1, value2, "sex");
return (Criteria) this;
}
public Criteria andAddressIsNull() {
addCriterion("address is null");
return (Criteria) this;
}
public Criteria andAddressIsNotNull() {
addCriterion("address is not null");
return (Criteria) this;
}
public Criteria andAddressEqualTo(String value) {
addCriterion("address =", value, "address");
return (Criteria) this;
}
public Criteria andAddressNotEqualTo(String value) {
addCriterion("address <>", value, "address");
return (Criteria) this;
}
public Criteria andAddressGreaterThan(String value) {
addCriterion("address >", value, "address");
return (Criteria) this;
}
public Criteria andAddressGreaterThanOrEqualTo(String value) {
addCriterion("address >=", value, "address");
return (Criteria) this;
}
public Criteria andAddressLessThan(String value) {
addCriterion("address <", value, "address");
return (Criteria) this;
}
public Criteria andAddressLessThanOrEqualTo(String value) {
addCriterion("address <=", value, "address");
return (Criteria) this;
}
public Criteria andAddressLike(String value) {
addCriterion("address like", value, "address");
return (Criteria) this;
}
public Criteria andAddressNotLike(String value) {
addCriterion("address not like", value, "address");
return (Criteria) this;
}
public Criteria andAddressIn(List<String> values) {
addCriterion("address in", values, "address");
return (Criteria) this;
}
public Criteria andAddressNotIn(List<String> values) {
addCriterion("address not in", values, "address");
return (Criteria) this;
}
public Criteria andAddressBetween(String value1, String value2) {
addCriterion("address between", value1, value2, "address");
return (Criteria) this;
}
public Criteria andAddressNotBetween(String value1, String value2) {
addCriterion("address not between", value1, value2, "address");
return (Criteria) this;
}
}
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}
config类:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context" xmlns:p="http://www.springframework.org/schema/p"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http:
http:
http:
http:
<!-- 加载配置文件 -->
<context:property-placeholder location="classpath:jdbc.properties" />
<!-- 数据库连接池 -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource"
destroy-method="close">
<property name="driverClassName" value="${jdbc.driver}" />
<property name="url" value="${jdbc.url}" />
<property name="username" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
<!-- 连接池的最大数据库连接数 -->
<property name="maxActive" value="10" />
<!-- 最大空闲数 -->
<property name="maxIdle" value="5" />
</bean>
<!-- SqlSessionFactory配置 -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<!-- 加载mybatis核心配置文件 -->
<property name="configLocation" value="classpath:SqlMapConfig.xml" />
<!-- 别名包扫描 -->
<property name="typeAliasesPackage" value="com.mybatis.pojo" />
</bean>
<!-- 传统Dao配置 -->
<!-- <bean class="com.mybatis.dao.impl.UserDaoImpl">
<property name="sqlSessionFactory" ref="sqlSessionFactory" />
</bean> -->
<!-- 动态代理配置方式:第一种 -->
<!-- <bean id="baseMapper" class="org.mybatis.spring.mapper.MapperFactoryBean" abstract="true" lazy-init="true">
<property name="sqlSessionFactory" ref="sqlSessionFactory" />
</bean> -->
<!-- 配置一个接口 -->
<!-- <bean parent="baseMapper">
<property name="mapperInterface" value="com.mybatis.mapper.UserMapper" />
</bean> -->
<!-- 动态代理,第二种方式:包扫描(推荐): -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<!-- basePackage多个包用","分隔 -->
<!-- <property name="basePackage" value="com.mybatis.mapper" /> -->
<property name="basePackage" value="com.mybatis.mapper" />
</bean>
</beans>
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql:
jdbc.username=root
jdbc.password=root
# Global logging configuration
log4j.rootLogger=DEBUG, stdout
# Console output...
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%5p [%t] - %m%n
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<mappers>
<package name="com.mybatis.mapper"/>
</mappers>
</configuration>
测试类:
package com.mybatis.test;
import static org.junit.Assert.fail;
import java.util.List;
import org.junit.Before;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.mybatis.mapper.OrderMapper;
import com.mybatis.mapper.UserMapper;
import com.mybatis.pojo.Order;
import com.mybatis.pojo.User;
import com.mybatis.pojo.UserExample;
import com.mybatis.pojo.UserExample.Criteria;
public class UserMapperGTest {
private ApplicationContext applicationContext;
@Before
public void init() {
applicationContext = new ClassPathXmlApplicationContext("classpath:applicationContext.xml");
}
@Test
public void testInsertSelective() {
UserMapper userMapper = applicationContext.getBean(UserMapper.class);
User user = new User();
user.setUsername("诸葛亮2");
user.setAddress("深圳黑马");
user.setSex("1");
userMapper.insertSelective(user);
}
@Test
public void testInsert() {
UserMapper userMapper = applicationContext.getBean(UserMapper.class);
User user = new User();
user.setUsername("王一");
user.setAddress("深圳");
user.setSex("1");
user.setBirthday(null);
userMapper.insertSelective(user);
}
@Test
public void testSelectByExample() {
UserMapper userMapper = applicationContext.getBean(UserMapper.class);
UserExample example = new UserExample();
Criteria criteria = example.createCriteria();
criteria.andUsernameLike("%张%");
criteria.andSexEqualTo("1");
List<User> list = userMapper.selectByExample(example);
for (User user : list) {
System.out.println(user); } }
@Test
public void testSelectByPrimaryKey() {
UserMapper userMapper = applicationContext.getBean(UserMapper.class);
User user = userMapper.selectByPrimaryKey(10);
System.out.println(user);
}
@Test
public void testUpdateByPrimaryKeySelective() {
fail("Not yet implemented");
}
@Test
public void testGetUserOrderMap() {
UserMapper userMapper = applicationContext.getBean(UserMapper.class);
List<User> list = userMapper.getUserOrderMap();
for (User user2 : list) {
System.out.println(user2);
for (Order order : user2.getOrders()) {
if(order.getId() != null){
System.out.println(" 此用户下的订单有:" + order);
} } }
}
@Test
public void testGetOrderUserMap() {
OrderMapper orderMapper = applicationContext.getBean(OrderMapper.class);
List<Order> list = orderMapper.getOrderUserMap();
for (Order order : list) {
System.out.println(order);
System.out.println(" 此订单的用户为:" + order.getUser()); } }}
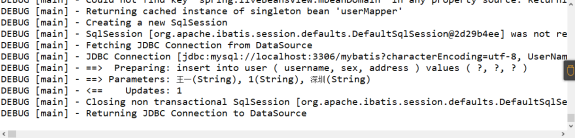 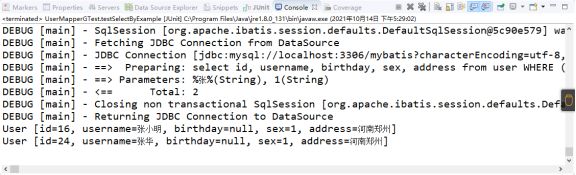 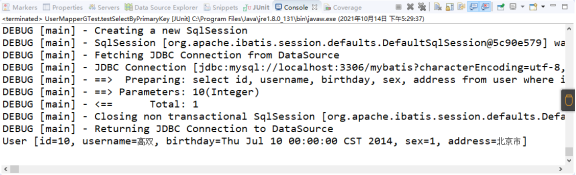 实验心得:、 通过今天试验了解到实际的开发中,对数据库的操作常常会涉及到多张表,这在面向对象中就涉及到了对象与对象之间的关联关系。针对多表之间的操作,MyBatis提供了关联映射,通过关联映射就可以很好的处理对象与对象之间的关联关系。在关系型数据库中,多表之间存在着三种关联关系,分别为一对一、一对多和多对多,掌握MyBatis中一对多关联映射案例代码的编写,掌握 MyBatis 与 Spring 框架的整合开发。 为我以后学习与工作增加经验,使我有了增加学好这么技术的信心。同时也感谢老师对我帮助。
|