Java 集合框架
Java Collection Framework ,又被称为容器
container ,是定义在
java.util 包下的一组接口
interfaces 和其实现类
classes 。其主要表现为将多个元素
element
置于一个单元中,用于对这些元素进行快速、便捷的存储
store 、检索
retrieve 、管理
manipulate ,即平时我们俗称的
增删查改
CRUD 。
一、类和接口总览
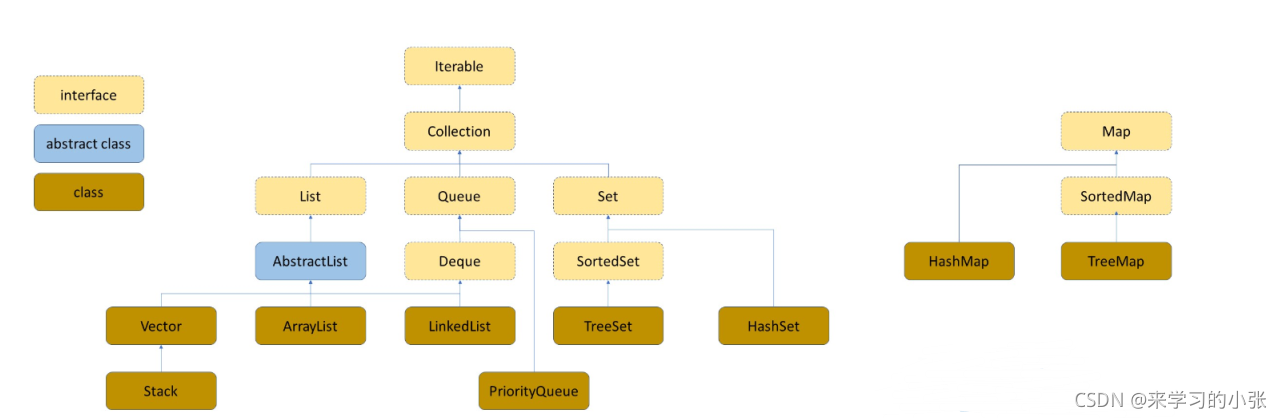 上图中最左边部分从上往下依次为:接口、抽象类、具体的实现类。
中间部分: Iterable :迭代器。相当于for-each() 。 Collection :用来存储管理一组对象 objects ,这些对象一般被成为元素elements 。
Set : 元素不能重复,背后隐含着查找/搜索的语义。SortedSet : 一组有序的不能重复的元素。List : 线性结构。Queue : 队列。Deque : 双端队列。
右边部分:
Map : 键值对 Key-Value-Pair ,背后隐含着查找/搜索的语义。SortedMap : 一组有序的键值对。
二、Collection接口常用方法说明
2.1将元素放到集合中add
boolean add(E e)
示例:
public static void main(String[] args) {
Collection<String> collection = new ArrayList<String>();
collection.add("hello");
Collection<Integer> collection2 = new ArrayList<Integer>();
collection2.add(1);
collection2.add(2);
collection2.add(3);
}
2.2 删除集合中的所有元素clear()
void clear()
2.3判断集合是否没有任何元素isEmpty()
boolean isEmpty()
2.4 删除集合中元素remove()
boolean remove(Object e)
2.5 判断集合中元素个数size()
int size()
2.6 返回一个装有所有集合中元素的数组
Object[] toArray()
示例:
public static void main(String[] args) {
Collection<String> collection = new ArrayList<String>();
collection.add("hello");
collection.add("world");
System.out.println(collection);
System.out.println(collection.size());
Object[] objects = collection.toArray();
System.out.println(Arrays.toString(objects));
}
三、Map接口常用方法说明
3.1 根据指定的k查找对应的v(get)
方式一:
V get(Object k)
方式二:
V getOrDefault(Object k, V defaultValue)
3.2 将指定的 k-v 放入Map(put)
V put(K key, V value)
3.3 判断是否包含key(containskey)
boolean containskey(object key)
3.4 判断是否包含value(containsvalue)
boolean containsvalue(object value)
3.5 判断是否为空 isEmpty()
boolean isEmpty()
3.6 返回键值对的数量size()
int size()
示例:
public static void main(String[] args) {
Map<String,String> map = new HashMap<>();
map.put("你是","you are");
map.put("及时雨","松江");
String ret = map.get("你是");
String ret2 = map.getOrDefault("你是","谁");
System.out.println(ret);
System.out.println(ret2);
boolean flg = map.containsKey("你是");
System.out.println(flg);
boolean flg2 = map.containsValue("宋江");
System.out.println(flg2);
boolean flg3 = map.isEmpty();
System.out.println(flg3);
System.out.println(map.size());
}
3.7 将所有键值对返回entrySet()
Set<Map.Entry<K, V>> entrySet()
示例:
public static void main(String[] args) {
Map<String,String> map = new HashMap<>();
map.put("你是","you are");
map.put("及时雨","宋江");
System.out.println(map);
System.out.println("=============");
Set<Map.Entry<String,String>> entrySet = map.entrySet();
for (Map.Entry<String,String> entry:entrySet) {
System.out.println("key:"+entry.getKey()+" value:"+entry.getValue());
}
}
以上。
|