项目编号:BS-XX-038
本系统基于springboot实现开发,主要实现国内各省的疫情数据管理及实时统计,特点是利用图形报表实现展示各省确诊人数、疑似人数、隔离人数、治愈人数,利用不同的数据展示方式来进行数据的体现,并带有后台管理功能。
Java:? jdk1.8
Mysql: mysql5.7
Tomcat:? tomcat8.5.31
开发工具:IDEA或eclipse
前台数据统计:
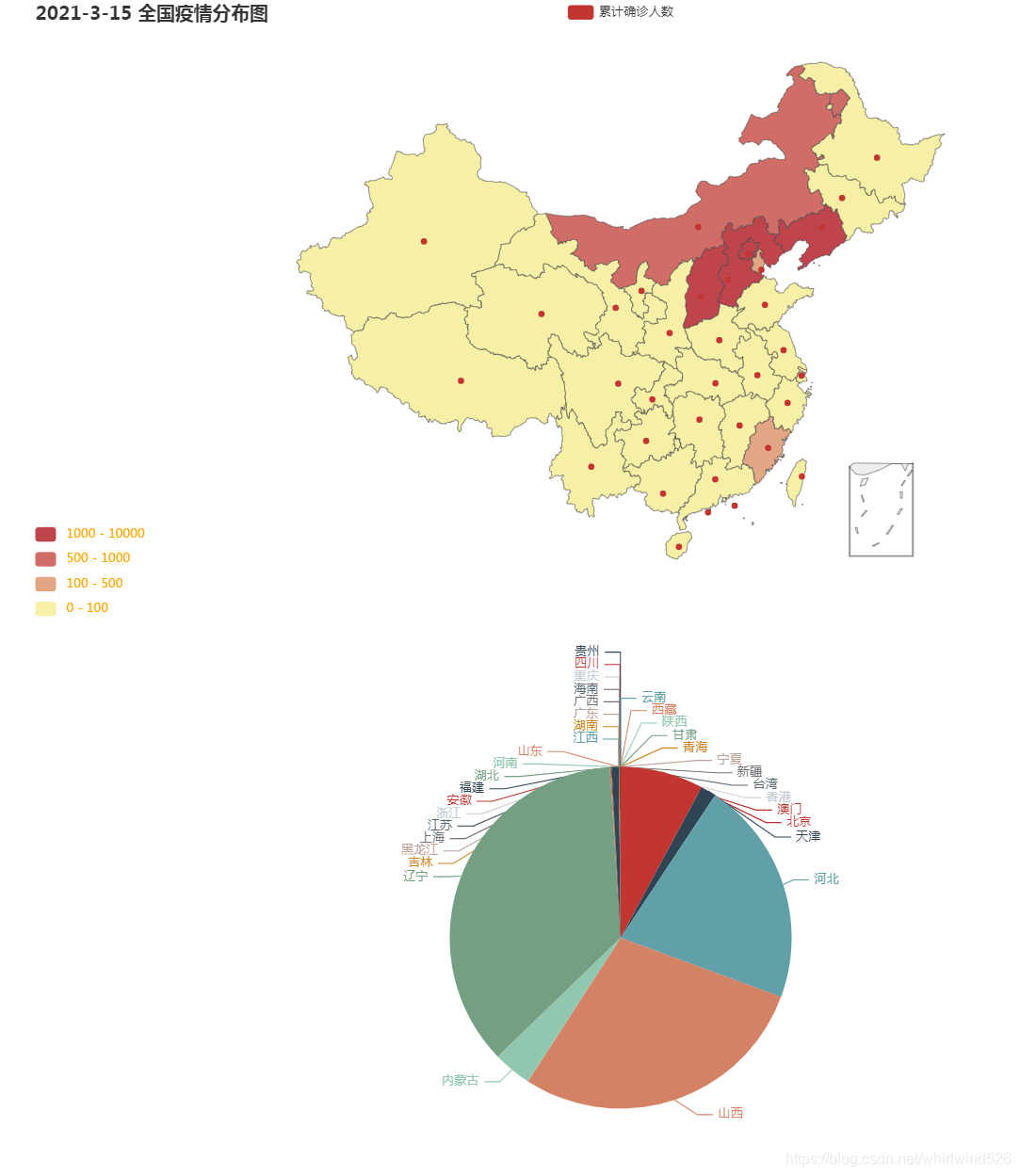
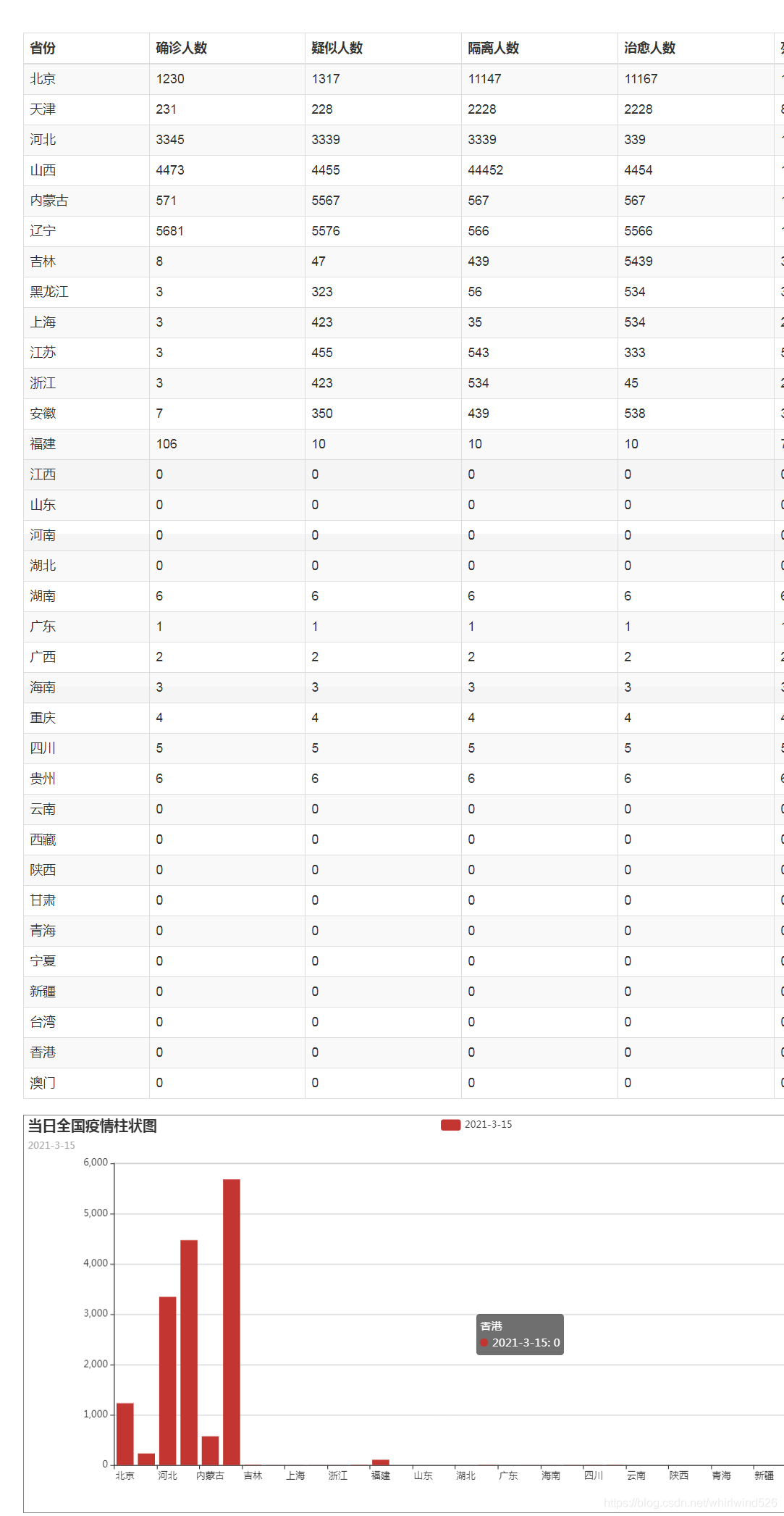
后台登陆
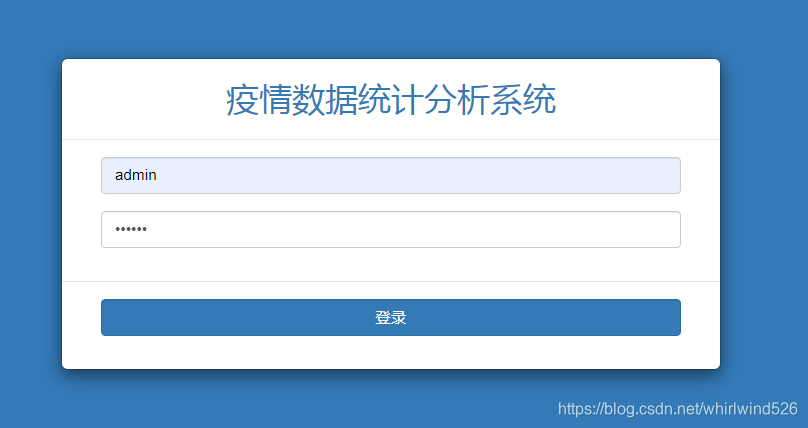
录入各省分数据:点提交后换一批省份,直接所有的省份数据录入完毕
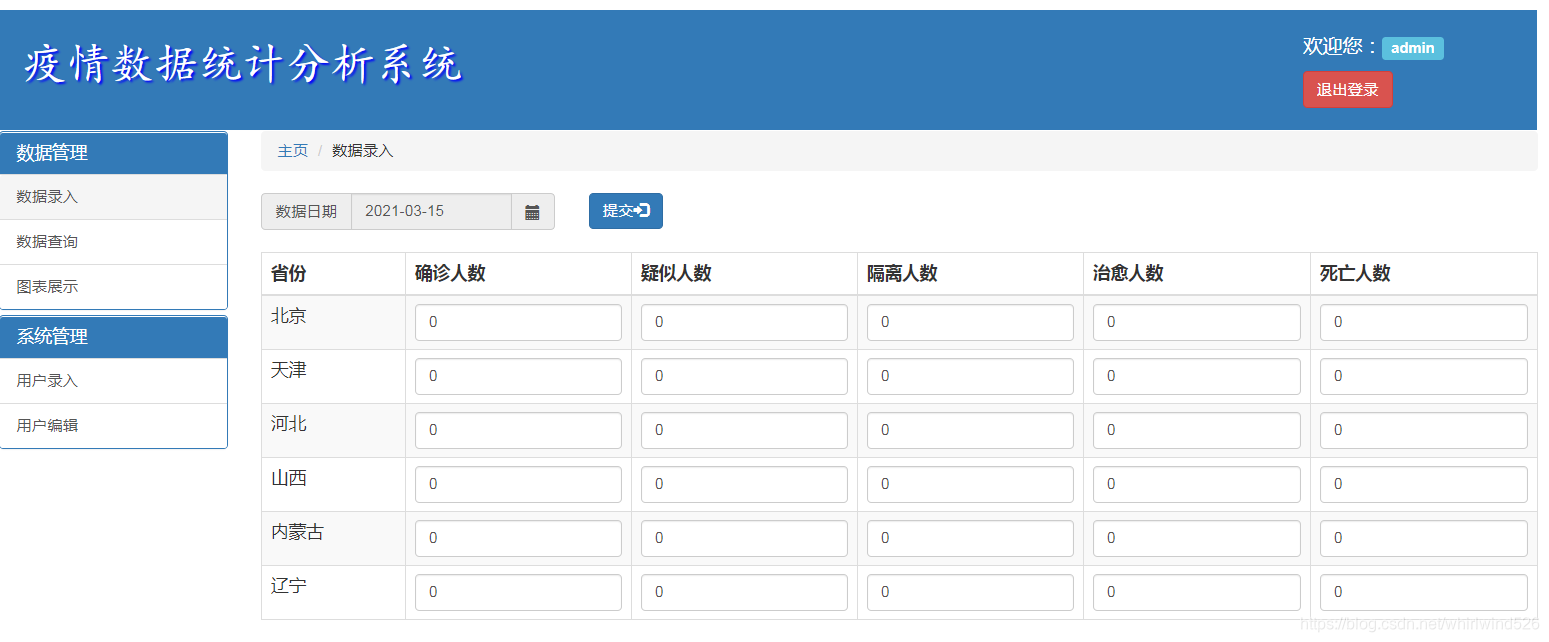
数据查询:查询今日各省数据
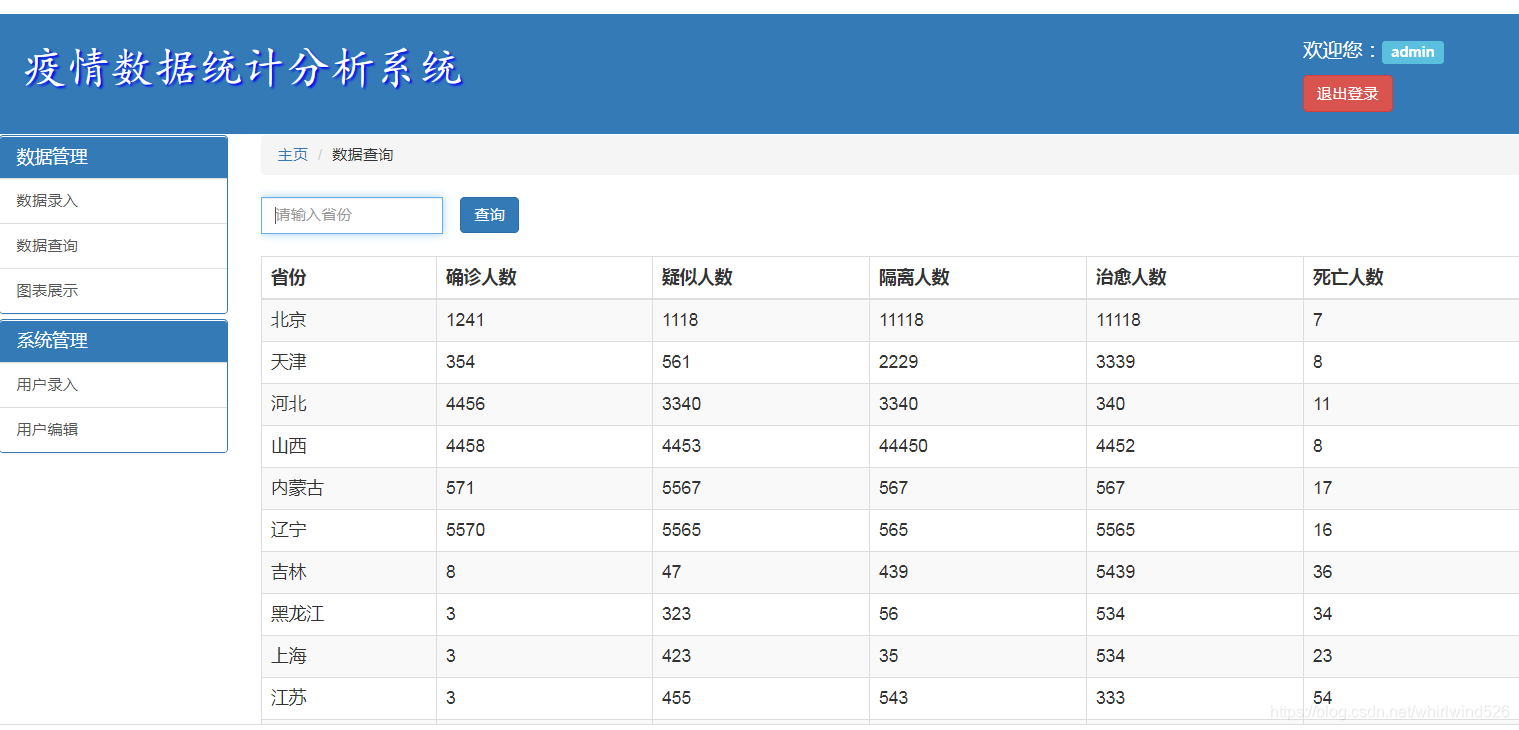
图表展示:
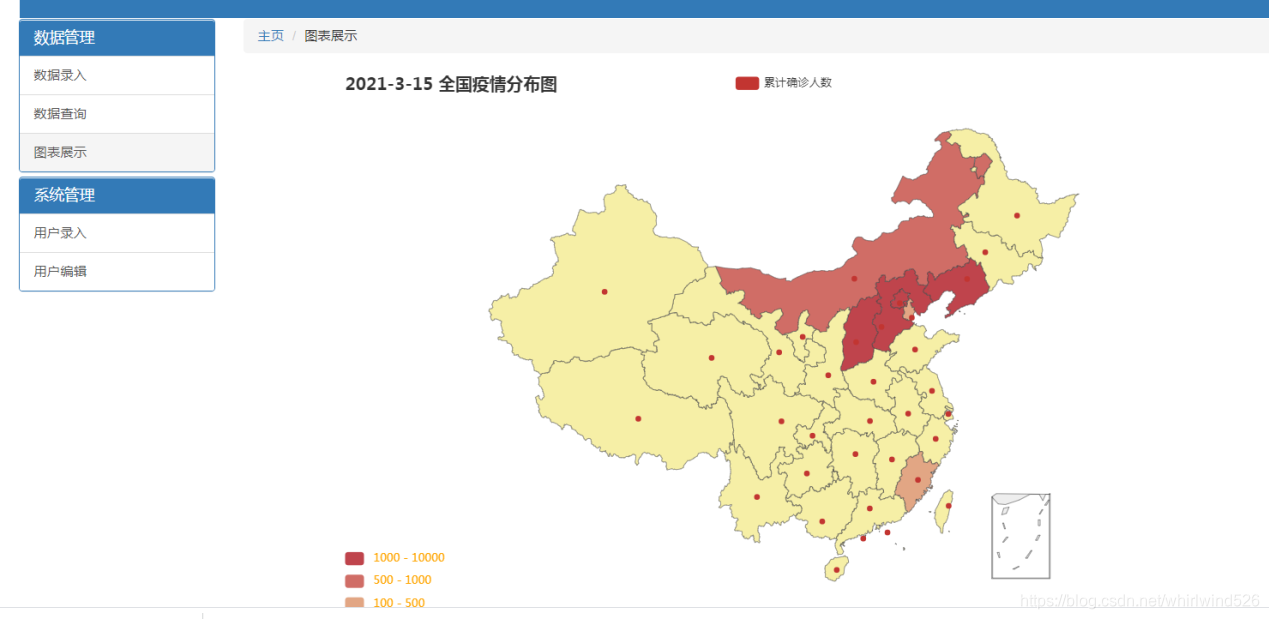
用户录入:
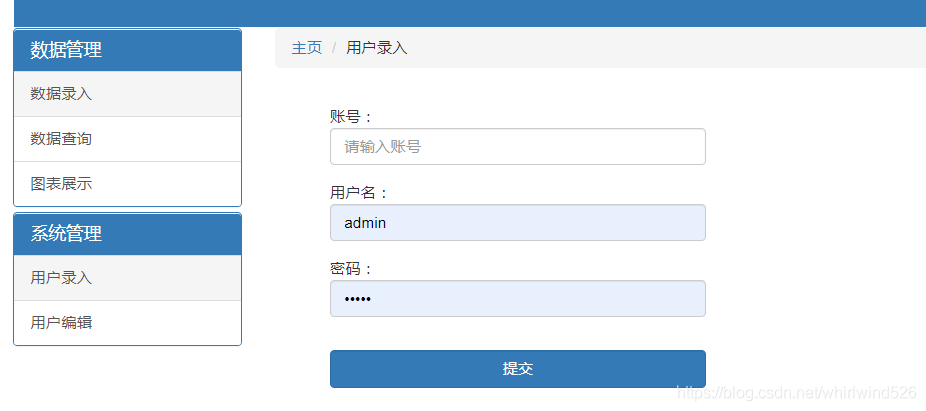
用户编辑:
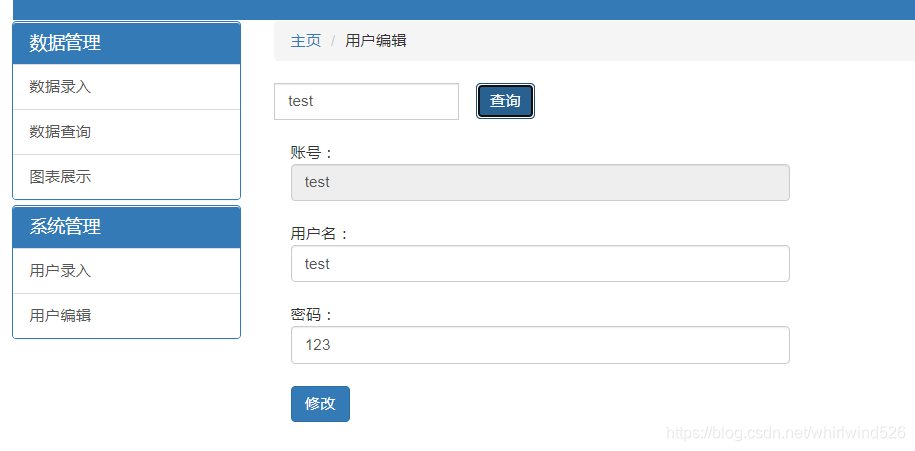
本系统很有特点,数据统计准确,比较适合做毕业设计或课程设计使用。
package com.liu.epidemic.service.impl;
import com.liu.epidemic.bean.DailyEpidemicInfo; import com.liu.epidemic.bean.EpidemicDetailInfo; import com.liu.epidemic.bean.EpidemicInfo; import com.liu.epidemic.bean.ProvinceInfo; import com.liu.epidemic.mapper.EpidemicMapper; import com.liu.epidemic.mapper.ProvinceMapper; import com.liu.epidemic.service.EpidemicService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service;
import java.util.Calendar; import java.util.Date; import java.util.GregorianCalendar; import java.util.List;
/** ?* @author znz ?* @date 2021/11/21 ?*/ @Service public class EpidemicServiceImpl implements EpidemicService { ? ? @Autowired ? ? private EpidemicMapper epidemicMapper;
? ? @Autowired ? ? private ProvinceMapper provinceMapper;
? ? @Override ? ? public List<ProvinceInfo> saveEpidemicInfos(Integer userId, DailyEpidemicInfo dailyEpidemicInfo) { ? ? ? ? String date = dailyEpidemicInfo.getDate(); ? ? ? ? List<EpidemicInfo> array = dailyEpidemicInfo.getArray(); ? ? ? ? String[] strings = date.split("-"); ? ? ? ? int year = Integer.parseInt(strings[0]); ? ? ? ? int month = Integer.parseInt(strings[1]); ? ? ? ? int day = Integer.parseInt(strings[2]); ? ? ? ? for(int i=0;i<array.size();i++){ ? ? ? ? ? ? //epidemicInfo封装页面提交过来的数据 ? ? ? ? ? ? EpidemicInfo epidemicInfo = array.get(i); ? ? ? ? ? ? epidemicInfo.setDataYear(year); ? ? ? ? ? ? epidemicInfo.setDataMonth(month); ? ? ? ? ? ? epidemicInfo.setDataDay(day); ? ? ? ? ? ? epidemicInfo.setUserId(userId); ? ? ? ? ? ? epidemicInfo.setInputDate(new Date()); //设置当前时间作为数据录入时间 ? ? ? ? ? ? //保存所有的疫情信息数据 ? ? ? ? ? ? epidemicMapper.saveEpidemicInfos(epidemicInfo); ? ? ? ? } ? ? ? ? //返回下一组 ? ? ? ? List<ProvinceInfo> noDataProvinceList = provinceMapper.findNoDataProvinceList(year, month, day); ? ? ? ? return noDataProvinceList; ? ? }
? ? @Override ? ? public List<EpidemicDetailInfo> findEpidemicInfoTotal() { ? ? ? ? GregorianCalendar calendar = new GregorianCalendar(); ? ? ? ? int year = calendar.get(Calendar.YEAR); ? ? ? ? int month = calendar.get(Calendar.MONTH)+1; ? ? ? ? int day = calendar.get(Calendar.DATE);
? ? ? ? List<EpidemicDetailInfo> epidemicInfoTotals = epidemicMapper.findEpidemicInfoTotal(year, month, day); ? ? ? ? return epidemicInfoTotals; ? ? }
? ? @Override ? ? public EpidemicDetailInfo queryEpidemicInfoByProvince(String province) { ? ? ? ? GregorianCalendar calendar = new GregorianCalendar(); ? ? ? ? int year = calendar.get(Calendar.YEAR); ? ? ? ? int month = calendar.get(Calendar.MONTH)+1; ? ? ? ? int day = calendar.get(Calendar.DATE);
? ? ? ? EpidemicDetailInfo epidemicDetailInfo = epidemicMapper.queryEpidemicInfoByProvince(year, month, day, province); ? ? ? ? return epidemicDetailInfo; ? ? } } ?
package com.liu.epidemic.service.impl;
import com.liu.epidemic.bean.ProvinceInfo; import com.liu.epidemic.mapper.ProvinceMapper; import com.liu.epidemic.service.ProvinceService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service;
import java.util.List;
/** ?* @author znz ?* @date 2021/11/21 ?*/ @Service public class ProvinceServiceImpl implements ProvinceService { ? ? @Autowired ? ? private ProvinceMapper provinceMapper;
? ? @Override ? ? public List<ProvinceInfo> noDataProvinceList(String date) { ? ? ? ? //2020-11-16 ? ? ? ? String[] strings = date.split("-"); //["2020","11","16"] ? ? ? ? int year = Integer.parseInt(strings[0]); ? ? ? ? int month = Integer.parseInt(strings[1]); ? ? ? ? int day = Integer.parseInt(strings[2]); ? ? ? ? List<ProvinceInfo> noDataProvinceList = provinceMapper.findNoDataProvinceList(year, month, day); ? ? ? ? return noDataProvinceList; ? ? } } ?
package com.liu.epidemic.service.impl;
import com.liu.epidemic.bean.UserInfo; import com.liu.epidemic.mapper.UserMapper; import com.liu.epidemic.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; import org.springframework.stereotype.Service;
/** ?* @author znz ?* @date 2021/11/21 ?* 如果要纳入spring容器的类,不属于框架的任何一层,就加@Component ?* 如果该类属于控制层,就加@Controller ?* 服务层,就加@Service ?* 持久层,就加@Repository/@Mapper ?* 以上注解可以通用 ?*/ //@Component @Service("userService") ?//将该类与实现类纳入spring容器的管理 public class UserServiceImpl implements UserService { ? ? @Autowired ? ? private UserMapper userMapper;
? ? @Override ? ? public UserInfo login(UserInfo userInfo) { ? ? ? ? UserInfo user = userMapper.login(userInfo); ? ? ? ? System.out.println("userservice"+user); ? ? ? ? return user; ? ? }
? ? @Override ? ? public void userInput(UserInfo userInfo) { ? ? ? ? userMapper.userInput(userInfo); ? ? }
? ? @Override ? ? public UserInfo queryUserByAccount(String account) { ? ? ? ? UserInfo userInfo = userMapper.queryUserByAccount(account); ? ? ? ? return userInfo; ? ? }
? ? @Override ? ? public int updateUser(UserInfo userInfo) { ? ? ? ? return userMapper.updateUser(userInfo); ? ? } } ?
|