libhv 作为一个高性能异步网络库,想要快速入门,莫过于动手实现一个tinyhttpd (即微型HTTP 服务)。 libhv examples目录下的 tinyhttpd.c 源代码包括注释在内不到400行,麻雀虽小,五脏俱全,代码绝对是工业级水准,可直接用于生产环境,不是和著名 Tinyhttpd 一样的玩具。 通过这个示例你将掌握事件循环 、TCP服务 、定时器 、拆包 、HTTP协议 等核心功能。
废话不多说,先上代码:
#include "hv.h"
#include "hloop.h"
#define HTTP_KEEPALIVE_TIMEOUT 60000
#define HTTP_HEAD_MAX_LENGTH 1024
#define HTML_TAG_BEGIN "<html><body><center><h1>"
#define HTML_TAG_END "</h1></center></body></html>"
#define HTTP_OK "OK"
#define NOT_FOUND "Not Found"
#define NOT_IMPLEMENTED "Not Implemented"
#define TEXT_PLAIN "text/plain"
#define TEXT_HTML "text/html"
typedef enum {
s_begin,
s_first_line,
s_request_line = s_first_line,
s_status_line = s_first_line,
s_head,
s_head_end,
s_body,
s_end
} http_state_e;
typedef struct {
int major_version;
int minor_version;
union {
struct {
char method[32];
char path[256];
};
struct {
int status_code;
char status_message[64];
};
};
int content_length;
char content_type[64];
unsigned keepalive: 1;
const char* body;
} http_msg_t;
typedef struct {
hio_t* io;
http_state_e state;
http_msg_t request;
http_msg_t response;
} http_conn_t;
static char s_date[32] = {0};
static void update_date(htimer_t* timer) {
uint64_t now = hloop_now(hevent_loop(timer));
gmtime_fmt(now, s_date);
}
static int http_response_dump(http_msg_t* msg, char* buf, int len) {
int offset = 0;
offset += snprintf(buf + offset, len - offset, "HTTP/%d.%d %d %s\r\n", msg->major_version, msg->minor_version, msg->status_code, msg->status_message);
offset += snprintf(buf + offset, len - offset, "Server: libhv/%s\r\n", hv_version());
if (msg->content_length > 0) {
offset += snprintf(buf + offset, len - offset, "Content-Length: %d\r\n", msg->content_length);
}
if (*msg->content_type) {
offset += snprintf(buf + offset, len - offset, "Content-Type: %s\r\n", msg->content_type);
}
if (*s_date) {
offset += snprintf(buf + offset, len - offset, "Date: %s\r\n", s_date);
}
offset += snprintf(buf + offset, len - offset, "\r\n");
if (msg->body && msg->content_length > 0) {
memcpy(buf + offset, msg->body, msg->content_length);
offset += msg->content_length;
}
return offset;
}
static int http_reply(http_conn_t* conn,
int status_code, const char* status_message,
const char* content_type,
const char* body, int body_len) {
char stackbuf[HTTP_HEAD_MAX_LENGTH + 1024] = {0};
char* buf = stackbuf;
int buflen = sizeof(stackbuf);
http_msg_t* req = &conn->request;
http_msg_t* resp = &conn->response;
resp->major_version = req->major_version;
resp->minor_version = req->minor_version;
resp->status_code = status_code;
if (status_message) strcpy(resp->status_message, status_message);
if (content_type) strcpy(resp->content_type, content_type);
if (body) {
if (body_len <= 0) body_len = strlen(body);
resp->content_length = body_len;
resp->body = body;
}
if (resp->content_length > buflen - HTTP_HEAD_MAX_LENGTH) {
HV_ALLOC(buf, HTTP_HEAD_MAX_LENGTH + resp->content_length);
}
int msglen = http_response_dump(resp, buf, buflen);
int nwrite = hio_write(conn->io, buf, msglen);
if (buf != stackbuf) HV_FREE(buf);
return nwrite < 0 ? nwrite : msglen;
}
static int http_serve_file(http_conn_t* conn) {
http_msg_t* req = &conn->request;
http_msg_t* resp = &conn->response;
const char* filepath = req->path + 1;
if (*filepath == '\0') {
filepath = "index.html";
}
FILE* fp = fopen(filepath, "rb");
if (!fp) {
http_reply(conn, 404, NOT_FOUND, TEXT_HTML, HTML_TAG_BEGIN NOT_FOUND HTML_TAG_END, 0);
return 404;
}
char buf[4096] = {0};
size_t filesize = hv_filesize(filepath);
resp->content_length = filesize;
const char* suffix = hv_suffixname(filepath);
const char* content_type = NULL;
if (strcmp(suffix, "html") == 0) {
content_type = TEXT_HTML;
} else {
}
int nwrite = http_reply(conn, 200, "OK", content_type, NULL, 0);
if (nwrite < 0) return nwrite;
int nread = 0;
while (1) {
nread = fread(buf, 1, sizeof(buf), fp);
if (nread <= 0) break;
nwrite = hio_write(conn->io, buf, nread);
if (nwrite < 0) return nwrite;
if (nwrite == 0) {
hv_delay(10);
}
}
fclose(fp);
return 200;
}
static bool parse_http_request_line(http_conn_t* conn, char* buf, int len) {
http_msg_t* req = &conn->request;
sscanf(buf, "%s %s HTTP/%d.%d", req->method, req->path, &req->major_version, &req->minor_version);
if (req->major_version != 1) return false;
return true;
}
static bool parse_http_head(http_conn_t* conn, char* buf, int len) {
http_msg_t* req = &conn->request;
const char* key = buf;
const char* val = buf;
char* delim = strchr(buf, ':');
if (!delim) return false;
*delim = '\0';
val = delim + 1;
while (*val == ' ') ++val;
if (stricmp(key, "Content-Length") == 0) {
req->content_length = atoi(val);
} else if (stricmp(key, "Content-Type") == 0) {
strcpy(req->content_type, val);
} else if (stricmp(key, "Connection") == 0) {
if (stricmp(val, "keep-alive") == 0) {
req->keepalive = 1;
} else {
req->keepalive = 0;
}
} else {
}
return true;
}
static int on_request(http_conn_t* conn) {
http_msg_t* req = &conn->request;
if (strcmp(req->method, "GET") == 0) {
if (strcmp(req->path, "/ping") == 0) {
http_reply(conn, 200, "OK", TEXT_PLAIN, "pong", 4);
return 200;
} else {
}
return http_serve_file(conn);
} else if (strcmp(req->method, "POST") == 0) {
if (strcmp(req->path, "/echo") == 0) {
http_reply(conn, 200, "OK", req->content_type, req->body, req->content_length);
return 200;
} else {
}
} else {
}
http_reply(conn, 501, NOT_IMPLEMENTED, TEXT_HTML, HTML_TAG_BEGIN NOT_IMPLEMENTED HTML_TAG_END, 0);
return 501;
}
static void on_close(hio_t* io) {
http_conn_t* conn = (http_conn_t*)hevent_userdata(io);
if (conn) {
HV_FREE(conn);
hevent_set_userdata(io, NULL);
}
}
static void on_recv(hio_t* io, void* buf, int readbytes) {
char* str = (char*)buf;
http_conn_t* conn = (http_conn_t*)hevent_userdata(io);
http_msg_t* req = &conn->request;
switch (conn->state) {
case s_begin:
conn->state = s_first_line;
case s_first_line:
if (readbytes < 2) {
fprintf(stderr, "Not match \r\n!");
hio_close(io);
return;
}
str[readbytes - 2] = '\0';
if (parse_http_request_line(conn, str, readbytes - 2) == false) {
fprintf(stderr, "Failed to parse http request line:\n%s\n", str);
hio_close(io);
return;
}
conn->state = s_head;
hio_readline(io);
break;
case s_head:
if (readbytes < 2) {
fprintf(stderr, "Not match \r\n!");
hio_close(io);
return;
}
if (readbytes == 2 && str[0] == '\r' && str[1] == '\n') {
conn->state = s_head_end;
} else {
str[readbytes - 2] = '\0';
if (parse_http_head(conn, str, readbytes - 2) == false) {
fprintf(stderr, "Failed to parse http head:\n%s\n", str);
hio_close(io);
return;
}
hio_readline(io);
break;
}
case s_head_end:
if (req->content_length == 0) {
conn->state = s_end;
goto s_end;
} else {
conn->state = s_body;
hio_readbytes(io, req->content_length);
break;
}
case s_body:
req->body = str;
if (readbytes == req->content_length) {
conn->state = s_end;
} else {
break;
}
case s_end:
s_end:
on_request(conn);
if (hio_is_closed(io)) return;
if (req->keepalive) {
memset(&conn->request, 0, sizeof(http_msg_t));
memset(&conn->response, 0, sizeof(http_msg_t));
conn->state = s_first_line;
hio_readline(io);
} else {
hio_close(io);
}
break;
default: break;
}
}
static void on_accept(hio_t* io) {
hio_setcb_close(io, on_close);
hio_setcb_read(io, on_recv);
hio_set_keepalive_timeout(io, HTTP_KEEPALIVE_TIMEOUT);
http_conn_t* conn = NULL;
HV_ALLOC_SIZEOF(conn);
conn->io = io;
hevent_set_userdata(io, conn);
conn->state = s_first_line;
hio_readline(io);
}
int main(int argc, char** argv) {
if (argc < 2) {
printf("Usage: %s port\n", argv[0]);
return -10;
}
const char* host = "0.0.0.0";
int port = atoi(argv[1]);
hloop_t* loop = hloop_new(0);
hio_t* listenio = hloop_create_tcp_server(loop, host, port, on_accept);
if (listenio == NULL) {
return -20;
}
printf("listenfd=%d\n", hio_fd(listenio));
htimer_add(loop, update_date, 1000, INFINITE);
hloop_run(loop);
hloop_free(&loop);
return 0;
}
编译:
git clone https://gitee.com/libhv/libhv.git
./configure
make
windows 平台编译可查看libhv教程02–编译与安装
运行:
HTTP服务端
bin/tinyhttpd 8000
HTTP客户端
bin/curl -v http://127.0.0.1:8000/
bin/curl -v http://127.0.0.1:8000/ping
bin/curl -v http://127.0.0.1:8000/echo -d "hello,world!"
HTTP压测
bin/wrk http://127.0.0.1:8000/ping
在 Github Actions 上你可以找到测试结果,单线程也可轻松达到7w QPS 。 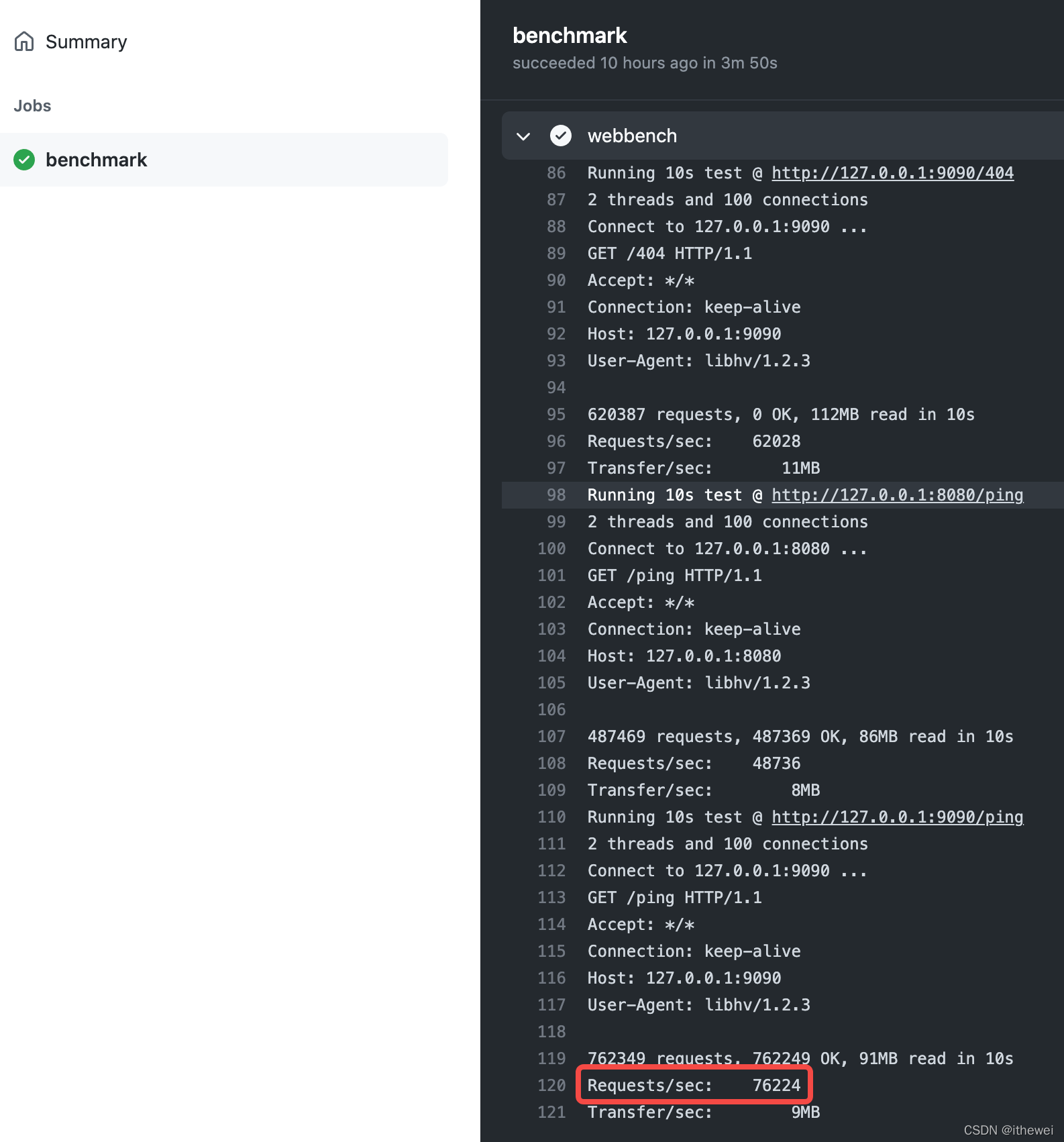
代码流程图
TCP服务
Created with Rapha?l 2.3.0
main
hloop_new
创建事件循环
hloop_create_tcp_server
创建TCP服务
hloop_run
运行事件循环
hloop_free
释放事件循环
结束
HTTP请求响应
Created with Rapha?l 2.3.0
on_accept
接收到连接
hio_readline
读取一行
on_recv
接收到数据
parse_http_request_line
解析HTTP请求行
hio_readline
读取一行
on_recv
接收到数据
parse_http_head
解析HTTP头
......
hio_readbytes
读取body
on_recv
接收到数据
on_request
接收到完整的HTTP请求
http_reply
HTTP响应
hio_write
发送数据
keepalive?
hio_close
关闭连接
on_close
连接断开
yes
no
代码解读
上面的流程图里基本展示了所有用到的核心函数,下面主要说下hio_readline 、hio_readbytes 两个用于流式读取的方法。
看过 libhv教程14–200行实现一个纯C版jsonrpc框架 这篇教程的应该知道,libhv里提供了一个设置拆包规则的函数hio_set_unpack ,支持固定包长、分隔符、头部长度字段 三种常见的拆包方式,内部会根据拆包规则处理粘包与分包,保证回调上来的是完整的一包数据。但是这个函数却并不适用于HTTP协议的拆包,HTTP协议是文本协议,看似比较简单,实际拆包却并不简单。
一个基本的HTTP POST 请求如下所示:
POST /echo HTTP/1.1
Accept: */*
Content-Length: 5
Content-Type: text/plain
Host: 127.0.0.1:8080
User-Agent: Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/63.0.3239.132 Safari/537.36
hello
HTTP头部是根据\r\n 分割,body长度则由头部的Content-Length 进行说明,以上三种拆包规则不能满足这种复合方式。所以libhv提供了hio_read_until_delim 、hio_read_until_length 来完美cover 这种情况。
hio_read_until_delim : 读取数据直到遇到指定的分隔符hio_read_until_length :读取一定长度的数据
hio_readline 、hio_readbytes 其实是宏别名,见 hloop.h 头文件里的定义:
HV_EXPORT int hio_read_until_length(hio_t* io, unsigned int len);
HV_EXPORT int hio_read_until_delim (hio_t* io, unsigned char delim);
#define hio_readline(io) hio_read_until_delim(io, '\n')
#define hio_readstring(io) hio_read_until_delim(io, '\0')
#define hio_readbytes(io, len) hio_read_until_length(io, len)
我们使用hio_readline 读取HTTP头 、hio_readbytes 读取HTTP body ,就得到了完整的一份HTTP请求 了。
当然,代码里还有很多小技巧等待你去挖掘,如用htimer_add 添加了一个定时器去每秒更新GMT 时间字符串,用于HTTP Date 头,避免每次响应都要重复生成,追求极致性能。再如,hio_set_keepalive_timeout 一行就实现了一段时间内无请求过来就自动断开连接以节省资源。
|