?AOP
AOP基本概念
?AOP底层原理
- AOP底层使用动态代理
- 使用动态代理,创建接口实现类的代理对象,从而达到增强类的方法?
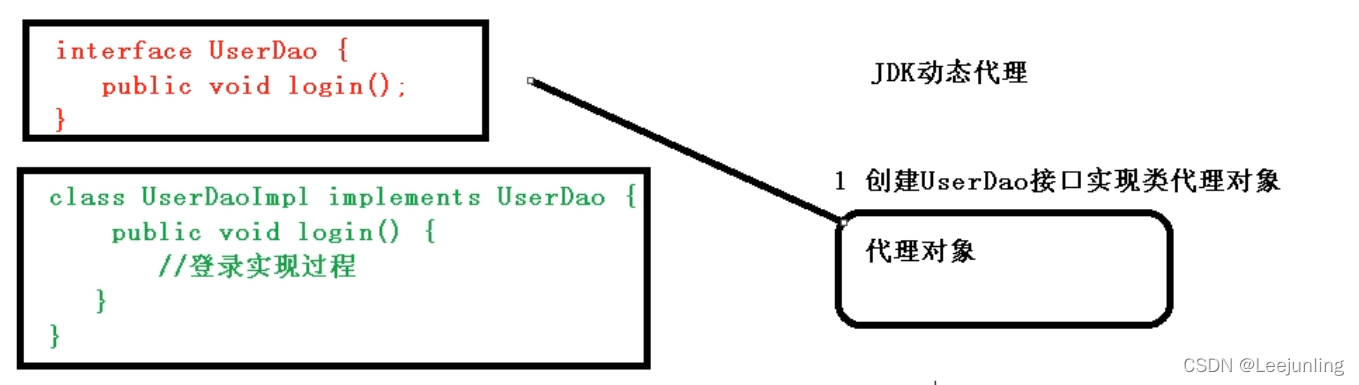
?AOP(JDK动态代理的实现)
- 使用JDK动态代理,使用proxy类中的方法创建代理对象
- newProxyInstancef(ClassLoader loader,类<?>[] interfaces,InvocationHandler h):返回指定接口的代理类的实例,该接口将方法调用分配给指定的调用处理系统
- ClassLoader:类的加载器
- Interfaces:增强方法所在类,这个类实现的接口,支持多个接口
- InvocationHandler:实现这个接口,创建代理的对象,写增强的方法
- JDK动态代理代码
- 创建接口定义方法
public interface UserDao {
public int add(int a,int b);
public String update(String id);
} -
创建接口实现类,实现方法 public class UserDaoImpl implements UserDao{
@Override
public int add(int a, int b) {
System.out.println("add执行了");
return a+b;
}
@Override
public String update(String id) {
return id;
}
} -
使用Proxy类创建接口代理对象 public class JDKProxy {
public static void main(String[] args) {
//创建接口实现类
Class[] interfaces = {UserDao.class};
UserDaoImpl userDao = new UserDaoImpl();
UserDao dao = (UserDao) Proxy.newProxyInstance(JDKProxy.class.getClassLoader(), interfaces, new UserDaoProxy(userDao));
System.out.println(dao.add(1, 2));
}
}
//创建代理对象代码
class UserDaoProxy implements InvocationHandler{
//1 把创建的是谁的代理对象,就把谁传递过来
//有参数的构造器传递
private Object obj;
public UserDaoProxy (Object obj){
this.obj = obj;
}
//增强的逻辑
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
//方法之前
System.out.println("方法执行之前...." + method.getName() + ":传递的参数..." +
Arrays.toString(args));
//被增强的方法执行
Object res = method.invoke(obj, args);
//方法之后
System.out.println("方法之后执行" + obj);
return res;
}
}
AOP术语?
- 连接点:类里哪些方法是可以被增强,哪些方法就成为连接点
- 切入点:实际被增强的方法,称为切入点
- 通知(增强):
- 实际增强的逻辑部分称为通知(增强)
- 通知有很多种类型:
- 切面:是一种动作,把通知应用到切入点过程
AOP的操作(AspectJ注解)
- 创建类,在类中定义方法
//被增强的类
public class User {
public void add(){
System.out.println("add....");
}
} -
创建增强类(编写增强逻辑的类) //增强的类
public class UserProxy {
//前置通知
public void before(){
System.out.println("before....");
}
} -
在配置文件中添加通知
-
在Spring配置文件中,开启注解扫描 <beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd">
<!-- 开启注解扫描-->
<context:component-scan base-package="com.atguigu.spring.aopanno">
</context:component-scan>
</beans> -
使用注解创建User和UserProxy对象 @Component
public class UserProxy {
@Componet
public class User{} -
在增强类中添加注解@Aspect @Component
@Aspect
public class UserProxy {
} -
在Spring配置文件中开启生成代理对象 <aop:aspectj-autoproxy> </aop:aspectj-autoproxy> 1 -
配置不同类型的通知
-
在增强类里,在作为通知方法上面添加通知l类型注解,使用切入点表达式配置 @Before(value = "execution(* com.ken.spring.aopanno.User.add(..))")
//前置通知
public void before(){} -
最终通知 @After(value = "execution(* com.ken.spring.aopanno.User.add(..))")
//最终通知
public void after(){}
-
相同切入点抽取(@Pointcut) 如果execution后的内容一致可以进行切入点抽取 @Pointcut(value = "execution(* com.ken.spring.aopanno.User.add(..))")
public void pointdemo(){
} -
有多个增强类对同一个方法增强,可以设置增强类的优先级 在增强类上添加注解@Order(数字类型值),数字类型值越小优先级越高 @Order(1)
@Before(value = "execution(* com.ken.spring.aopanno.User.add(..))")
public void before(){
System.out.println("Person before...");
}
@Order(3)
@Before(value = "execution(* com.ken.spring.aopanno.User.add(..))")
public void before(){
System.out.println("before....");
}
|