常用组件介绍
标签名称 | 标签作用 | 标签说明 | 备注 | JFrame | 类似于 Windows 系统中窗口形式的界面,是所有Swing运行的基础 | JFrame():构造一个初始时不可见的新窗体 | 构造时,该类必须继承JFrame类,用new来创建新的JFrame | JFrame(String title):创建一个具有 title 指定标题的不可见新窗体 | JPanel | JPanel是容纳其他小组件的,即JFrame之下,千万小组件之上 | JPanel():使用默认的布局管理器创建新面板,默认的布局管理器为 FlowLayout | 如果包含多个JPanel一般只会显示最后一个定义的JPanel,如果想要在一个JFrame中显示多个JPanel,可以建立一个JPanel包含子JPanel | JLable | 是一个装载文字或者图像的标签 | JLabel label=new JLabel("在JPanel上的标签"); | JTextField | 允许编辑的单行文本,可以用在账号输入等等 | JTextField textField=new JTextField; | JPasswordField | 用于输入密码的单行框,显示成*号 | JPasswordField passoWord=new?JPasswordField; | JButton | 按钮标签 | JButton login=new JButton("登录"); | JRadioButton | 单选按钮标签 | JRadioButton radioBtn01 = new JRadioButton("男"); | 创建单选按钮不仅仅只创建一个按钮,还需要创建一个按钮组,说明其为单选按钮,具体例子 | JCheckBox? | 复选框 | JCheckBox checkBox01 = new JCheckBox("菠萝"); | JTextArea | 多行文本区域 | final JTextArea textArea = new JTextArea(10,10); 设置10行10列的文本区域 | JComboBox | 下拉列表框 | String[] listData = new String[]{"香蕉", "雪梨", "苹果", "荔枝"}; JComboBox<String> comboBox = new JComboBox<String>(listData); | JFileChooser | 文件选取器 | JFileChooser fileChooser = new JFileChooser(); | JColorChooser | 颜色选取器 | Color color = JColorChooser.showDialog(jf, "选取颜色", null); | JMenuBar | 菜单栏 | | JToolBar | 工具栏 | | JDialog、JOptionPane | 对话框 | JOptionPane.showMessageDialog(null,"需要展示的信息");
|
代码示例说明组件建立顺序
import javax.swing.*;
import java.awt.*;
/**第一步建立注册类,并且要继承JFrame类*/
class FunctionRegister extends JFrame {
/**接下来分别定义JPanel,JLabel等标签,建议将定义放到public FunctionRegister之外,后面学到事件监听和多个页面调用可以更加方便*/
JPanel panel1;
JLabel label1, label2, label3, label4, label5;
JButton registerButton1, exitButton1;
JTextField label2Field, label5Field;
JPasswordField passwordField3, passwordField4;
public FunctionRegister() {
//可以认为给window窗口定义大标题,即整个框架的标题
JFrame frame=new JFrame("天地银行注册系统");
//整个框架的大小,即JFrame的大小
frame.setSize(400, 300);
//设置窗口出现的位置,为null则出现在屏幕正中央
this.setLocationRelativeTo(null);
//设置框架的关闭方式
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//系统分配JPanel,JLabel等
panel1 = new JPanel();
//定义一个"欢迎您注册天地银行"的内容
label1 = new JLabel("欢迎您注册天地银行");
label2 = new JLabel("请输入您的用户名");
//定义用户名输入区域,并且定义其长度
label2Field = new JTextField(16);
label3 = new JLabel("请输入您的密码");
//定义一个密码输入区域
passwordField3 = new JPasswordField(16);
label4 = new JLabel("请再次确认您的密码");
passwordField4 = new JPasswordField(16);
label5 = new JLabel("请输入您的身份证号");
label5Field = new JTextField(18);
//定义一个名字为注册的按钮
registerButton1 = new JButton("注册");
exitButton1 = new JButton("返回");
//首先将小组件加入到面板JPanel,一定要注意增加的顺序
panel1.add(label1);
panel1.add(label2);
panel1.add(label2Field);
panel1.add(label3);
panel1.add(passwordField3);
panel1.add(label4);
panel1.add(passwordField4);
panel1.add(label5);
panel1.add(label5Field);
panel1.add(registerButton1);
panel1.add(exitButton1);
//将面板加入到框架JFrame中,this表示JFrame,也可以不定义JFrame,用this.add(panel)d的方法
frame.add(panel1);
//将面板设置为可见!!!如果是false看不见的
frame.setVisible(true);
}
}
public class Test {
public static void main(String[]args){
//实现方法,new一个注册面板
new FunctionRegister();
}
}
效果展示:
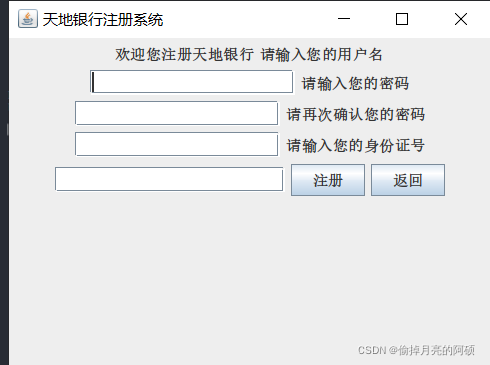
?复选框,单选等代码示例
import javax.swing.*;
import java.awt.*;
public class Test {
public static void main(String[] args) throws AWTException {
JFrame jf = new JFrame("单选按钮窗口测试");
jf.setSize(200, 200);
jf.setLocationRelativeTo(null);
jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
// 创建两个单选按钮
JRadioButton radioBtn01 = new JRadioButton("男");
JRadioButton radioBtn02 = new JRadioButton("女");
// 创建按钮组,把两个单选按钮添加到该组!!很重要!!不然你会发现你的按钮中男女两个都可以同时是选中黑色状态,可以将下面三行代码注释体验一下
ButtonGroup btnGroup = new ButtonGroup();
btnGroup.add(radioBtn01);
btnGroup.add(radioBtn02);
// 设置第一个单选按钮是默认选中状态
radioBtn01.setSelected(true);
//定义复选框
JCheckBox checkBox01 = new JCheckBox("菠萝");
JCheckBox checkBox02 = new JCheckBox("香蕉");
JCheckBox checkBox03 = new JCheckBox("雪梨");
JCheckBox checkBox04 = new JCheckBox("荔枝");
JCheckBox checkBox05 = new JCheckBox("橘子");
//创建下拉表
String[] listData = new String[]{"香蕉", "雪梨", "苹果", "荔枝"};
JComboBox<String> comboBox = new JComboBox<String>(listData);
//将按钮加入到面板中
panel.add(radioBtn01);
panel.add(radioBtn02);
panel.add(checkBox01);
panel.add(checkBox02);
panel.add(checkBox03);
panel.add(checkBox04);
panel.add(checkBox05);
panel.add(comboBox);
jf.add(panel);
jf.setVisible(true);
}
}
效果展示:
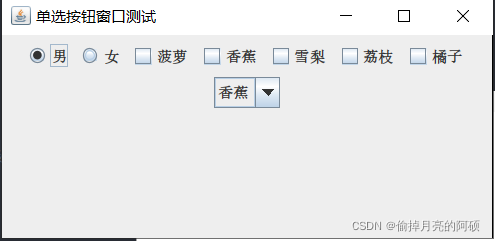
?文件选择器,颜色选择器等效果实现
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.logging.FileHandler;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.logging.SimpleFormatter;
public class TextEditorFrame<EditName> extends JFrame {
File file=null;
/**默认颜色为黑色*/
Color color=Color.black;
TextDAL dal=null;
TextEditorFrame(TextDAL dal){
this.dal=dal;
//文本部分
initTextPane();
//菜单部分
initMenu();
//对话框部分
initAboutDialog();
//工具条部分
initToolBar();
}
void initTextPane(){//将文本框放入又滚动对象,并加入到Frame中
getContentPane().add(new JScrollPane(text));
}
JTextPane text=new JTextPane();
/**文件选择框*/
JFileChooser fileChooser=new JFileChooser();
/**颜色选择对话框*/
JColorChooser colorChooser=new JColorChooser();
/**关于对话框*/
JDialog about=new JDialog();
/**菜单*/
JMenuBar menuBar=new JMenuBar();
/**一级菜单包括以下三个选项*/
JMenu[] menus=new JMenu[]{new JMenu("文件"),new JMenu("编辑"),new JMenu("帮助")};
/**设置菜单的子选项*/
JMenuItem[][] menuItem =new JMenuItem[][]{{
new JMenuItem("新建文件"),
new JMenuItem("打开文件"),
new JMenuItem("保存文件"),
new JMenuItem("退出文件")},
{new JMenuItem("复制文件"),
new JMenuItem("剪切文件"),
new JMenuItem("粘贴文件"),
new JMenuItem("颜色")},
{new JMenuItem("关于")}
};
/**初始化菜单,将的三个一级菜单选项加入到menuBar中,并且给每一个子菜单添加监听事件*/
void initMenu(){
for(int i=0;i<menus.length;i++) {
menuBar.add(menus[i]);
for (int j = 0; j < menuItem[i].length; j++) {
menus[i].add(menuItem[i][j]);
menuItem[i][j].addActionListener(action);
}
}
//将menuBar加入到面板中
this.setJMenuBar(menuBar);
}
/**定义action监听事件*/
ActionListener action=new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JMenuItem mi = (JMenuItem) e.getSource();
String id = mi.getText();
if (id.equals("新建文件")) {text.setText("");file = null;}
else if (id.equals("打开文件")) {
if (file != null) {fileChooser.setSelectedFile(file);}
int returnVal = fileChooser.showOpenDialog(TextEditorFrame.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
file = fileChooser.getSelectedFile();
openFile();
}}
else if (id.equals("保存文件")) {
if (file != null) {
fileChooser.setSelectedFile(file);
}
int returnVal = fileChooser.showOpenDialog(TextEditorFrame.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
file = fileChooser.getSelectedFile();
saveFile();
}}
else if (id.equals("退出文件")) {System.exit(0);}
else if (id.equals("剪切文件")) {text.cut();}
else if (id.equals("复制文件")) {text.copy();}
else if (id.equals("粘贴文件")) {text.paste();}
else if (id.equals("颜色")) {
color = JColorChooser.showDialog(TextEditorFrame.this, "", color);
text.setForeground(color);}
else if (id.equals("关于")) {
about.setSize(100, 50);
about.setVisible(true);
}
}
};
/**定义保存文件的函数*/
void saveFile(){
String content=text.getText();
dal.save(file,content);
}
/**定义打开文件的函数*/
void openFile(){
String content=dal.read(file);text.setText(content);
}
void initAboutDialog(){
about.getContentPane().add(new JLabel("<html><body>简单的文本编辑器1.0<br>CSDN作者:偷掉月亮的阿硕<br>本片文章代码改编自Java程序设计唐大仕老师的代码,如果侵犯您的权益请联系<body></html>"));
//更改窗口为模式对话框
about.setModal(true);
about.setSize(100,50);
}
/**定义工具栏,并且可以添加相应的图片*/
JToolBar toolBar=new JToolBar();
JButton[] buttons=new JButton[]{
new JButton("",new ImageIcon("copy.jpg")),
new JButton("",new ImageIcon("cut.jpg")),
new JButton("",new ImageIcon("paste.jpg"))
};
/**定义工具按钮的相关功能,加入到组件中*/
void initToolBar(){
for (int i=0;i<buttons.length;i++){
toolBar.add(buttons[i]);
}
buttons[0].setToolTipText("复制文件");
buttons[0].addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
text.copy();
}
});
buttons[1].setToolTipText("剪切文件");
buttons[1].addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
text.cut();
}
});
buttons[2].setToolTipText("粘贴文件");
buttons[2].addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
text.paste();
}
});
this.getContentPane().add(toolBar,BorderLayout.NORTH);
//当且仅当鼠标悬停在该按钮时,才会显示出该按钮的边框
toolBar.setRollover(true);
}
}
class TextEditorApp2{
public static void main(String[] args){
//分发线程,保证不会发生死锁
javax.swing.SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
TextDAL dal=new FileTextDAL();
TextEditorFrame f=new TextEditorFrame(dal);
f.setTitle("简单的编辑器");
f.setSize(800,600);
f.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
f.setVisible(true);
}
});
}
}
interface TextDAL{
String read(File file);
void save(File file,String text);
}
/**logger是一个日志文件,记录相应的操作*/
class FileTextDAL implements TextDAL{
@Override
public String read(File file) {
logger.log(Level.INFO,"read","reab..."+file.getPath());
try{
FileReader fr=new FileReader(file);
int len=(int)file.length();
char[] buffer=new char[len];
fr.read(buffer,0,len);
fr.close();
return new String(buffer);
}catch (Exception e){
e.printStackTrace();
logger.log(Level.SEVERE,null,e);
}
return "";
}
@Override
public void save(File file, String text) {
logger.log(Level.INFO,"save","save..."+file.getPath());
try{
FileWriter fw=new FileWriter(file);
fw.write(text);
fw.close();
}catch (Exception ex){
ex.printStackTrace();
logger.log(Level.SEVERE,null,ex);
}
}
Logger logger= Logger.getLogger(FileTextDAL.class.getName());
{
try{
FileHandler handler=new FileHandler("TextEditorApp2.log");
handler.setFormatter(new SimpleFormatter());
logger.addHandler(handler);
}catch (IOException ex){}
}
}
效果展示: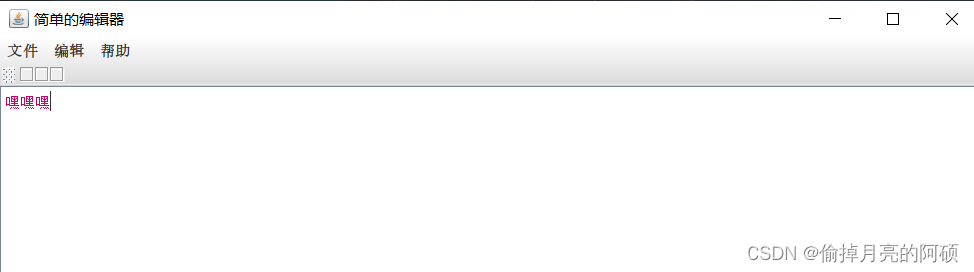
?
说明:
这个界面不整齐啊?? 答:看出来了,这就是继续学习排布的重要性,要不肯定会被弄死,后面大家可能会建立多个JPanel来管理布局,这个仅仅做个新手展示!!!
可以实现对输入的判断吗?? ? 当然可以!!!不过为了代码简洁一点,删掉了😏,我后面再发😏😏😏😏😏。
|