-
创建运行时类的对象
newInstance():内部调用了该运行时类的空参构造器,为通用方法
@Test
public void test() throws InstantiationException, IllegalAccessException {
Class<Person> c = Person.class;
Person p = c.newInstance();
}
注:要想此方法正常的创建运行时类的对象,需满足
- 运行时类必须提供空参构造器
- 空参构造器的访问权限需够用,设置为public
-
获取属性及其内部结构
@Test
public void testFields() {
Class<Person> c = Person.class;
Field[] fields = c.getFields();
for (Field f : fields) {
System.out.println(f);
}
Field[] declaredFields = c.getDeclaredFields();
for (Field f : declaredFields) {
System.out.println(f);
}
Field[] declaredFields = c.getDeclaredFields();
for (Field f : declaredFields) {
int modifiers = f.getModifiers();
System.out.print(modifiers + "\t");
System.out.print(Modifier.toString(modifiers) + "\t");
Class<?> type = f.getType();
System.out.print(type + "\t");
String fName = f.getName();
System.out.println(fName);
}
}
-
获取方法及其内部结构
经常用的是通过获取运行时类的方法,得到其注解(框架 = 注解 + 反射 + 设计模式)
@Test
public void testMethods() {
Class<Person> c = Person.class;
Method[] methods = c.getMethods();
for (Method m : methods) {
System.out.println(m);
}
Method[] methods2 = c.getDeclaredMethods();
for (Method m : methods2) {
System.out.println(m);
}
Method[] methods1 = c.getDeclaredMethods();
for (Method m : methods1) {
System.out.println();
Annotation[] annotations = m.getAnnotations();
for (Annotation a : annotations) {
System.out.println(a);
}
System.out.print(m + "\t");
System.out.print(Modifier.toString(m.getModifiers()) + "\t");
System.out.print(m.getReturnType().getName() + "\t");
System.out.print(m.getName());
System.out.print("(");
Class[] parameterTypes = m.getParameterTypes();
if (!(parameterTypes == null && parameterTypes.length == 0)) {
for (int i = 0; i < parameterTypes.length; i++) {
if (i == parameterTypes.length-1) {
System.out.print(parameterTypes[i].getName() + " args_" + i);
break;
}
System.out.print(parameterTypes[i].getName() + " args_" + i + ",");
}
}
System.out.println(")");
Class[] exceptionTypes = m.getExceptionTypes();
if (exceptionTypes.length > 0) {
System.out.print("throws ");
for (int i = 0; i < exceptionTypes.length; i++) {
if (i == exceptionTypes.length-1) {
System.out.println(exceptionTypes[i].getName());
break;
}
System.out.print(exceptionTypes[i].getName() + ",");
}
}
}
}
-
获取构造器及其内部结构
@Test
public void testConstructors() {
Class<Person> c = Person.class;
Constructor[] constructors = c.getConstructors();
for (Constructor cons : constructors) {
System.out.println(cons);
}
Constructor[] constructors1 = c.getDeclaredConstructors();
for (Constructor cons : constructors1) {
System.out.println(cons);
}
}
-
获取运行时类的父类
单继承,只有一个父类
@Test
public void testSuperClass() {
Class<Person> c = Person.class;
Class superclass = c.getSuperclass();
System.out.println(superclass);
Type genericSuperclass = c.getGenericSuperclass();
System.out.println(genericSuperclass);
ParameterizedType parameterizedType = (ParameterizedType) genericSuperclass;
Type[] actualTypeArguments = parameterizedType.getActualTypeArguments();
for (Type cl : actualTypeArguments ) {
System.out.println(cl.getTypeName());
}
}
-
获取运行时类实现的接口
@Test
public void testInterface() {
Class c = Person.class;
Class[] interfaces = c.getInterfaces();
for (Class in : interfaces) {
System.out.println(in);
}
Class[] interfaces1 = c.getSuperclass().getInterfaces();
for (Class in1 : interfaces1) {
System.out.println(in1);
}
}
-
获取运行时类所在的包
@Test
public void testPackage() {
Class c = Person.class;
Package aPackage = c.getPackage();
System.out.println(aPackage);
}
-
获取运行时类的注解
作用:通过反射获取注解后来得知该自定义类具体是要做什么
@Test
public void testAnnotation() {
Class c = Person.class;
Annotation[] annotations = c.getAnnotations();
for (Annotation a : annotations) {
System.out.println(a);
}
}
-
重要
9.1 获取父类的泛型
9.2 获取运行时类实现的接口(动态代理中会用)
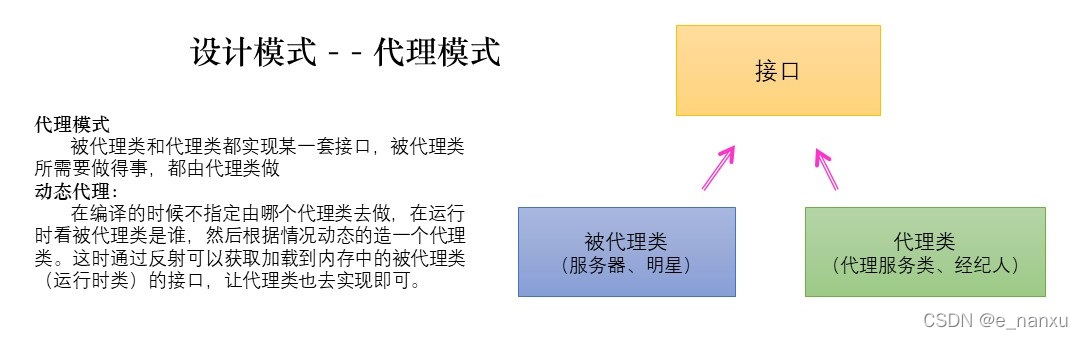
9.3 获取运行时类的注解(在框架中使用较多)