1.IO流的“首领”
? ? ? ? java.io.InputStream? 字节输入流
? ? ? ? java.io.OutputStream? 字节输出流
? ? ? ? java.io.Reader? 字符输入
? ? ? ? java.io.Writer? 字符输出
都实现了closeable,都是可以关闭的
输出流都实现了flushable,都是可以刷新的
所有流中以Stream结尾的叫做字节流
所有六中以Reader/Writer结尾的时字符流
1.2.? 16个流
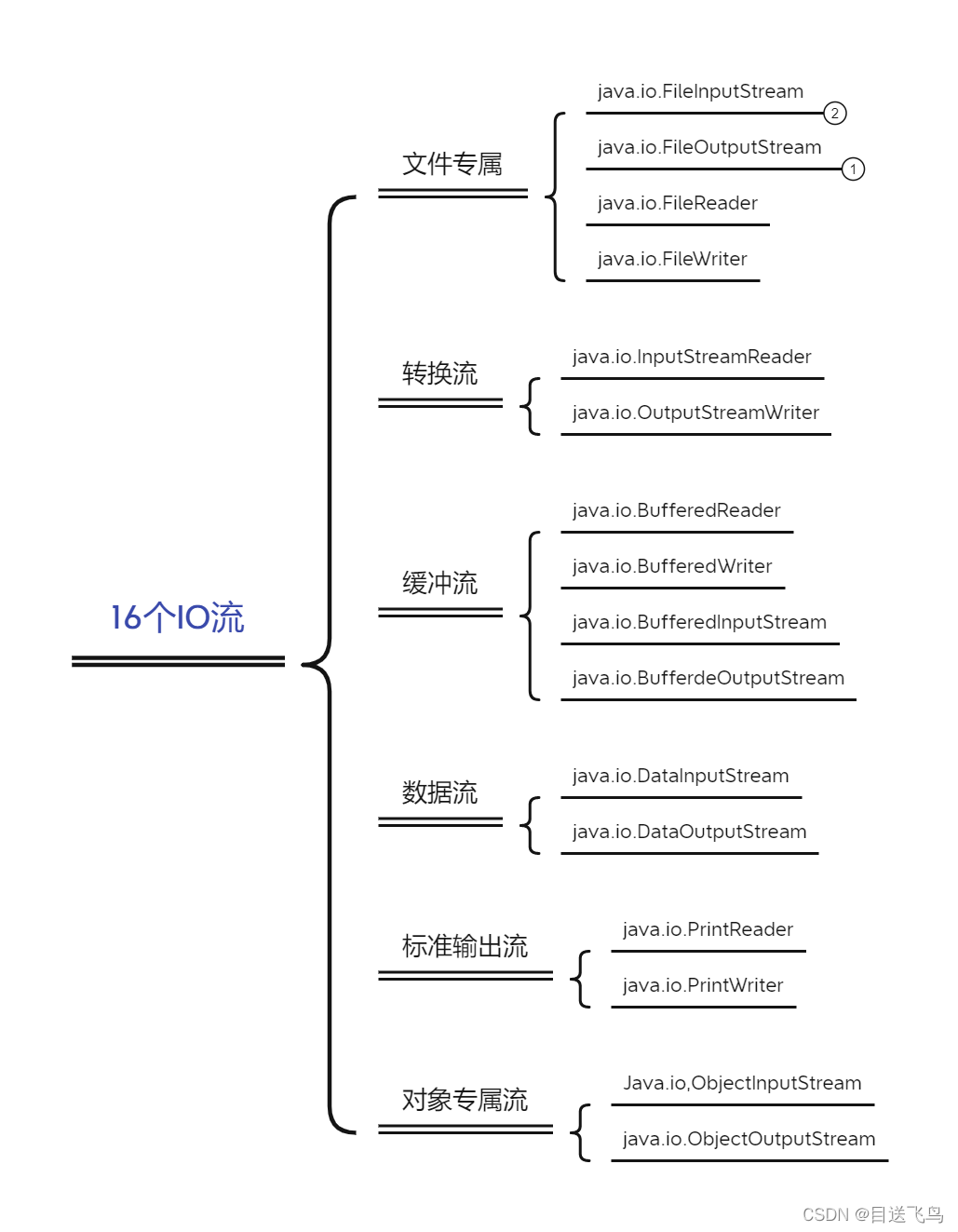
2.File类
package File;
import java.io.File;
import java.io.IOException;
/**
* java中万物皆对象
* file对象就是文件或者目录的抽象表示形式,它和流的四大家族无关,无法完成文件的读写
*
* 下面介绍常用方法
*/
public class Test {
public static void main(String[] args) throws IOException {
File file = new File("D:\\MyJava\\Temp2");
//1.判断是否存在
System.out.println(file.exists());
//2.若不存在,可用下面的两种方法以两种形式将之创建出来
File f1 = new File("D:\\MyJava\\Temp3");
if (!f1.exists()){
//以文件形式创建
//f1.createNewFile();
//以目录形式创建
//f1.mkdir();
f1.mkdirs();//以多重形式创建
}
//3.获取文件的父路径
String parentPath = file.getParent();
System.out.println(parentPath);//D:\MyJava
//4.获取绝对路径
File f2 = new File("fileTemp");
if (!f2.exists()){
f2.createNewFile();
}
System.out.println(f2.getAbsolutePath());//D:\MyJava\Temp2\fileTemp
}
}
File f1 = new File("D:\\course\\01-开课\\开学典礼.ppt");
// 获取文件名
System.out.println("文件名:" + f1.getName());
// 判断是否是一个目录
System.out.println(f1.isDirectory()); // false
// 判断是否是一个文件
System.out.println(f1.isFile()); // true
// 获取文件最后一次修改时间
long haoMiao = f1.lastModified(); // 这个毫秒是从1970年到现在的总毫秒数。
// 将总毫秒数转换成日期?????
Date time = new Date(haoMiao);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss SSS");
String strTime = sdf.format(time);
System.out.println(strTime);
// 获取文件大小
System.out.println(f1.length()); //216064字节。
// 获取当前目录下所有的子文件。
File f = new File("D:\\course\\01-开课");
File[] files = f.listFiles();
3.FileInputStream
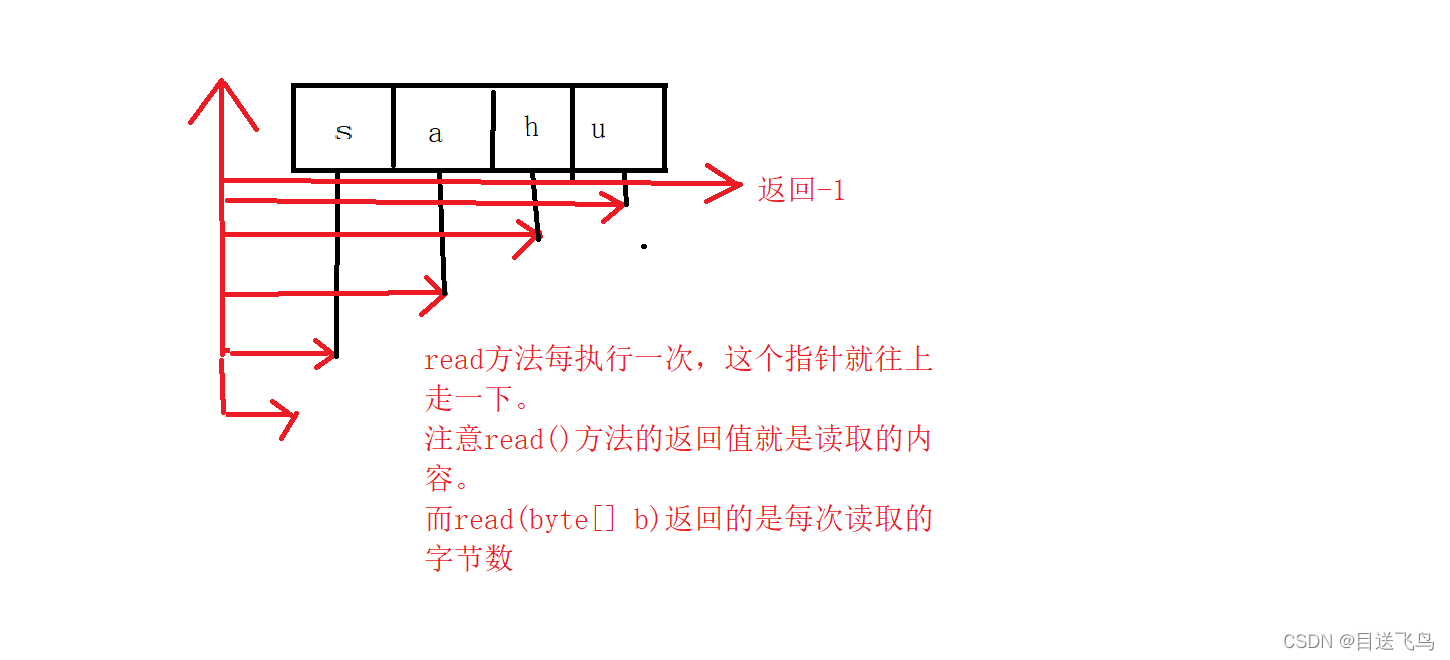
3.1read()? 返回值为读取到的数据
3.2read(byte[] b)? 返回值为读取到的字节数,而返回内容存储在byte[]数组中
3.3read(byte[] b,int off,int len)?
package FileStream.FileInputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
/**
* 使用循环改写
*/
public class Test04 {
public static void main(String[] args) {
FileInputStream fis = null;
try {
fis = new FileInputStream("temp.txt");
byte[] b = new byte[5];//一次性读取5个
int readCount = 0;
while ((readCount = fis.read(b))!=-1){
System.out.println(new String(b,0,readCount));
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fis!=null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
3.4.FileInputStream的其他几个方法
/**
* FileInputStream其他常用方法
* int available():返回流中剩余没有读到的字节数量
* long skip():跳过几个字节不读
*/
public class Test05 {
public static void main(String[] args) {
FileInputStream fis = null;
try {
fis = new FileInputStream("temp.txt");
System.out.println("该文件的总字节数为"+fis.available());//16
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fis!=null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
4.FileOutputStream
4.1FileOutputStream的声明
fos = new FileOutputStream("tempOut.txt");
//没有这个文件,会自动生成,如果有,这样写会清空原来的内容
fos = new FileOutputStream("temp.txt",true);//这样写,在源文件的基础上添加
4.2write方法
fos = new FileOutputStream("tempOut.txt",true);//这样写,在源文件的基础上添加
byte[] bytes = {97,98,99,100,101,102,103};
fos.write(bytes);
5.
|