一、数据库连接池技术
1.数据库连接池的基本思想︰ 就是为数据库连接建立一个"缓冲池"。预先在缓冲池中放入一定数量的连接,当需要建立数据库连接时,只需从"缓冲池"中取出一个,使用完毕之后再放回去。 数据库连接池负责分配、管理和释放数据库连接,它允许应用程序重复使用一个现有的数据库连接,而不是重新建立一个。 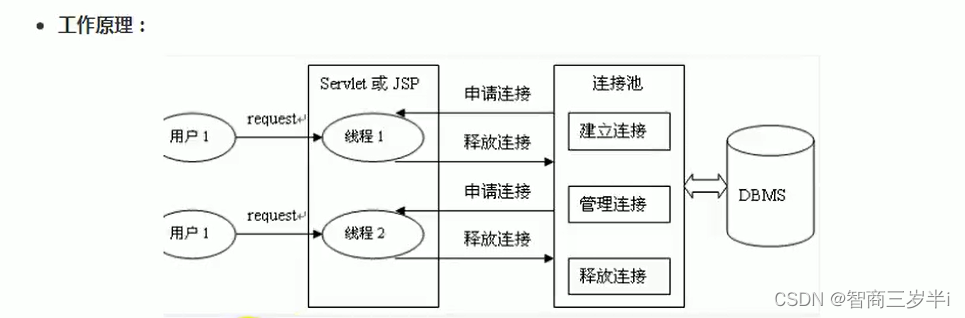 2.数据库连接池的优点 资源重用、更快的系统反应速度、新的资源分配手段、统一的连接管理,避免数据库连接泄露 3.数据库连接池的设置: 数据库连接池在初始化时将创建一定数量的数据库连接放到连接池中,这些数据库连接的数量是由最小数据库连接数来设定的。无论这些数据库连接是否被使用,连接池都将一直保证至少拥有这么多的连接数量。连接池的最大数据库连接数限定了这个连接池能占有的最大连接数,当应用程序向连接池请求的连接数超过最大连接数量时,这些请求将被加入到等待队列中。
二、多种开源的数据库连接池
JDBC的数据库连接池使用javax.sql.DataSource 来表示,DataSource只是一个接口,该接口通常由服务器(Weblogic, WebSphere,Tomcat)提供实现,也有一些开源组织提供实现︰ (1)dbcp:是Apache提供的数据库连接池。tomcat服务器自带dbcp数据库连接池。速度相对C3P0较快,但因自身存在BUG,Hibernate3已不再提供支持。 (2)c3p0:是一个开源组织提供的一个数据库连接池,速度相对较慢,稳定性较好(hibernate官方推荐使用) (3)Proxool:是sourceforge下的一个开源项目数据库连接池,有监控连接池状态的功能,稳定性较c3p0差一点 (4)BoneCP:是一个开源组织提供的数据库连接池,速度快 (5)Druid:是阿里提供的数据库连接池,据说是集DBCP、C3P0、Proxool 优点于一身的数据库连接池,但是速度不确定是否有BoneCP快
DataSource通常被称为数据源,它包含连接池和连接池管理两个部分,习惯上也经常把DataSource称为连接池,DataSource用来取代DriverManager来获取Connection,获取速度快,同时可以大幅度提高数据库访问速度。
1.c3p0数据库连接池 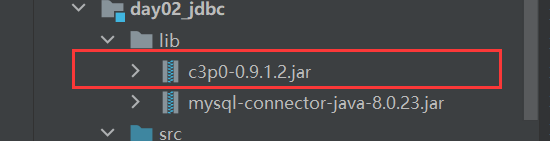
public class C3P0Test {
@Test
public void getConnectionTest() throws Exception {
ComboPooledDataSource cpds = new ComboPooledDataSource();
cpds.setDriverClass("com.mysql.cj.jdbc.Driver" );
cpds.setJdbcUrl( "jdbc:mysql://localhost:3306/test" );
cpds.setUser("root");
cpds.setPassword("12345678");
cpds.setInitialPoolSize(10);
Connection connect = cpds.getConnection();
System.out.println(connect);
DataSources.destroy(cpds);
}
@Test
public void getConnectionTest01() throws SQLException {
ComboPooledDataSource cpds = new ComboPooledDataSource("helloc3p0");
Connection connect = cpds.getConnection();
System.out.println(connect);
}
}
配置文件:c3p0-config.xml
<?xml version="1.0" encoding="utf-8" ?>
<c3p0-config>
<named-config name="helloc3p0">
<property name="driverClass">com.mysql.cj.jdbc.Driver</property>
<property name="jdbcUrl">jdbc:mysql://localhost:3306/test</property>
<property name="user">root</property>
<property name="password">12345678</property>
<property name="acquireIncrement">5</property>
<property name="initialPoolSize">10</property>
<property name="minPoolSize">10</property>
<property name="maxPoolSize">100</property>
<property name="maxStatement">50</property>
<property name="maxStatementPerConnection">2</property>
</named-config>
</c3p0-config>
连接成功: 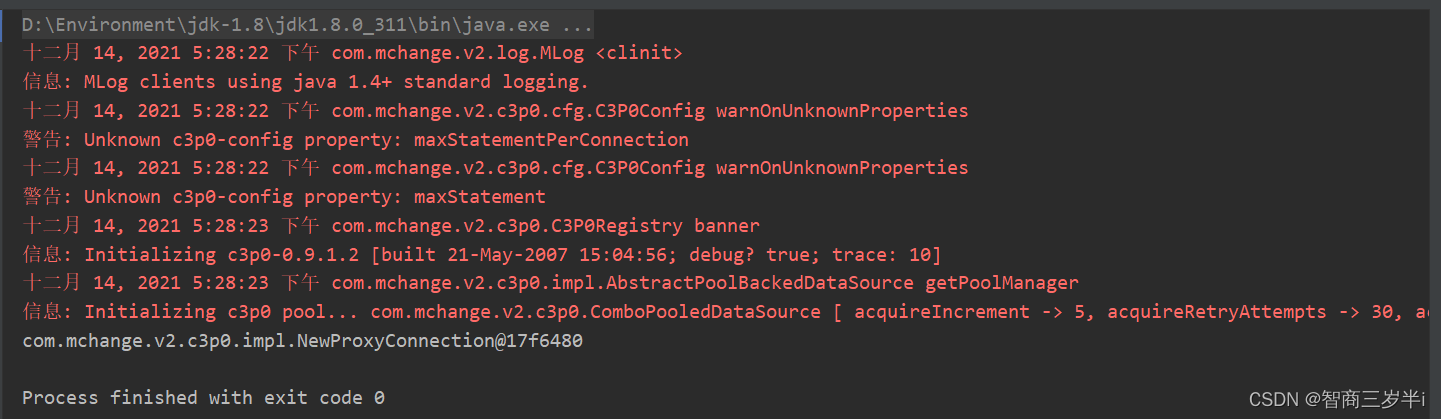 2.dbcp数据库连接池 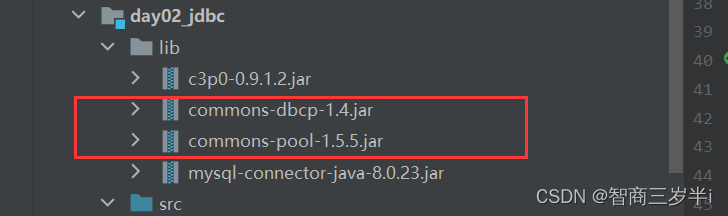
public class DbcpTest {
@Test
public void getConnectionTest() throws SQLException {
BasicDataSource dataSource = new BasicDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/test");
dataSource.setUsername("root");
dataSource.setPassword("12345678");
dataSource.setInitialSize(10);
dataSource.setMaxActive(10);
Connection connect = dataSource.getConnection();
System.out.println(connect);
}
@Test
public void getConnectionTest01() throws Exception {
Properties pros = new Properties();
FileInputStream fileInputStream = new FileInputStream(new File("E:\\java\\JDBC\\day02_jdbc\\src\\dbcp.properties"));
pros.load(fileInputStream);
DataSource dataSource = BasicDataSourceFactory.createDataSource(pros);
Connection connect = dataSource.getConnection();
System.out.println(connect);
}
}
配置文件: dbcp.properties
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/test
username=root
password=12345678
连接成功: 
|