一、关于Object类中的 wait 和 notify 方法
- wait 和 notify 方法不是线程对象的方法,是java中任何一个java对象都拥有的方法,因为这个是Object类中自带的方法。
- wait() 和 notify() 方法的作用:
Object o = new Object();
- o.wait(): 让正在o对象上活动的线程进入无期限等待状态,直到被唤醒为止。
- o.notify(): 唤醒正在o对象上等待的线程。
—— notifyAll()方法:这个方法是唤醒o对象上处于等待的所有线程。 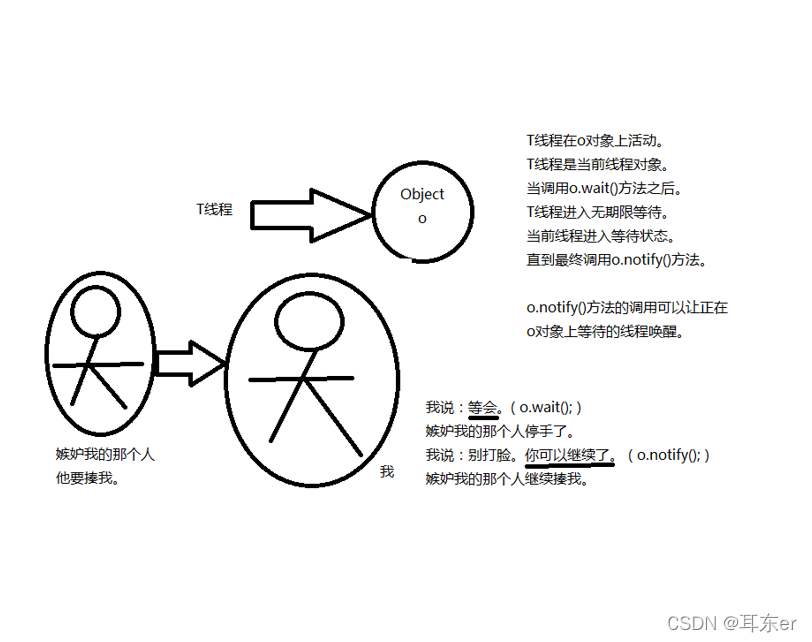
二、生产者和消费者模式
- 使用 wait() 和 notify() 方法实现“生产者和消费者模式”。
- 什么是“生产者和消费者模式”?
—— 生产线程负责生产,消费线程负责消费。生产线程和消费线程要达到均衡。 注:这是一种特殊的业务需求,在这种特殊的情况下需要使用到 wait() 和 notify() 方法。 - wait和notify方法不是线程对象的方法,是普通java对象都有的方法。
- wait方法和notify方法建立在线程同步的基础之上。因为多线程要同时操作同一个对象。有线程安全问题。
- wait 和 notify 方法的作用:
- wait方法作用: o.wait()让正在o对象上活动的线程t进入等待状态,并且释放掉t线程之前占有的o对象的锁。
- notify方法作用: o.notify()让正在o对象上等待的线程唤醒,只是通知,不会释放o对象上之前占有的锁。
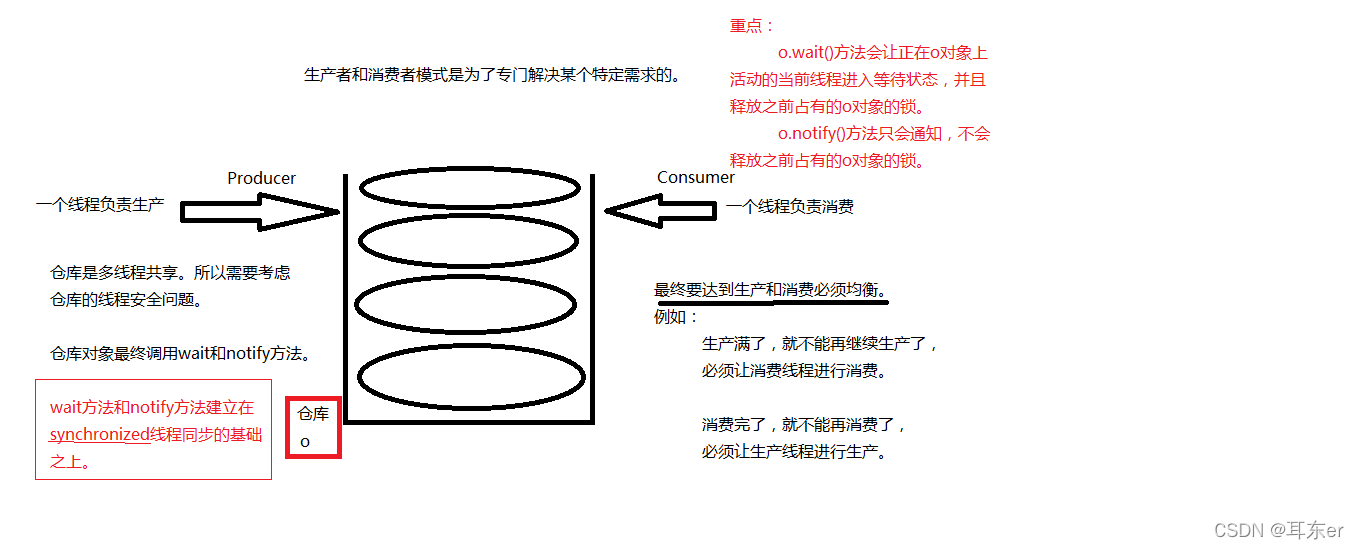
- 模拟生产者和消费者模式
public class ThreadTest07 {
public static void main(String[] args) {
List list = new ArrayList();
Thread t1 = new Thread(new Produce(list),"生产者线程");
Thread t2 = new Thread(new Consume(list),"消费者线程");
t1.start();
t2.start();
}
}
class Produce implements Runnable {
private List list;
public Produce(List list) {
this.list = list;
}
@Override
public void run() {
while (true) {
synchronized (list) {
if (list.size() > 0) {
try {
list.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
Object o = new Object();
list.add(o);
System.out.println(Thread.currentThread().getName() + "--->" + o);
list.notifyAll();
}
}
}
}
class Consume implements Runnable {
private List list;
public Consume(List list) {
this.list = list;
}
@Override
public void run() {
while (true) {
synchronized (list) {
if (list.size() == 0) {
try {
list.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
Object o = list.remove(0);
System.out.println(Thread.currentThread().getName() + "--->" + o);
list.notifyAll();
}
}
}
}
|