参考:https://blog.csdn.net/qq_43470725/article/details/119750430
https://blog.csdn.net/qq_43470725/article/details/121969868
pom:
<!-- servlet依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
</dependency>
<!-- tomcat的支持-->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
<scope>provided</scope>
</dependency>
yml:
mvc:
view:
prefix: /WEB-INF/jsp/
suffix: .jsp
thymeleaf:
cache: false
enabled: false
jsp:
<%@ page import="java.io.File" %>
<%@ page import="org.apache.commons.fileupload.disk.DiskFileItemFactory" %>
<%@ page import="org.apache.commons.fileupload.servlet.ServletFileUpload" %>
<%@ page import="org.apache.commons.fileupload.FileItem" %>
<%@ page import="java.text.SimpleDateFormat" %>
<%@ page import="java.util.*" %><%--
Created by IntelliJ IDEA.
User: Administrator
Date: 2021/8/17
Time: 10:23
To change this template use File | Settings | File Templates.
--%>
<%--<%@ page contentType="text/html;charset=UTF-8" language="java" %>--%>
<%--<html>--%>
<%--<head>--%>
<%-- <title>Title</title>--%>
<%--</head>--%>
<%--<body>--%>
<%--</body>--%>
<%--</html>--%>
<%--语法:https://www.w3cschool.cn/jsp/jsp-syntax.html
1、脚本程序: 可以包含任意量的Java语句、变量、方法或表达式,只要它们在脚本语言中是有效的:<% 代码片段 %>
或者,您也可以编写与其等价的XML语句
<jsp:scriptlet>
代码片段
</jsp:scriptlet>
2、任何文本、HTML标签、JSP元素必须写在脚本程序的外面
3、JSP声明 :用来声明变量和方法
一个声明语句可以声明一个或多个变量、方法,供后面的Java代码使用。在JSP文件中,您必须先声明这些变量和方法然后才能使用它们。
JSP声明的语法格式: <%! declaration; [ declaration; ]+ ... %>
<%! int i = 0; %>
<%! int a, b, c; %>
<%! Circle a = new Circle(2.0); %>
4、JSP表达式
一个JSP表达式中包含的脚本语言表达式,先被转化成String,然后插入到表达式出现的地方。
由于表达式的值会被转化成String,所以您可以在一个文本行中使用表达式而不用去管它是否是HTML标签。
表达式元素中可以包含任何符合Java语言规范的表达式,但是不能使用分号来结束表达式。
JSP表达式的语法格式:
<%= 表达式 %>
5、JSP隐含对象
JSP支持九个自动定义的变量,江湖人称隐含对象。这九个隐含对象的简介见下表:
request HttpServletRequest类的实例
response HttpServletResponse类的实例
out PrintWriter类的实例,用于把结果输出至网页上
session HttpSession类的实例
application ServletContext类的实例,与应用上下文有关
config ServletConfig类的实例
pageContext PageContext类的实例,提供对JSP页面所有对象以及命名空间的访问
page 类似于Java类中的this关键字
Exception Exception类的对象,代表发生错误的JSP页面中对应的异常对象
6、控制流语句
JSP提供对Java语言的全面支持。您可以在JSP程序中使用Java API甚至建立Java代码块,包括判断语句和循环语句等等:
判断语句:eg:
<%! int day = 3; %>
<html>
<head>
<title>IF...ELSE Example</title>
</head>
<body>
<% if (day == 1 | day == 7) { %>
<p> Today is weekend</p>
<% } else { %>
<p> Today is not weekend</p>
<% } %>
</body>
</html>
--%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%--设置cookie--%>
<%
Cookie firstName = new Cookie("first_name", "123");
Cookie lastName = new Cookie("last_name","456");
firstName.setMaxAge(60*60*24);
lastName.setMaxAge(60*60*24);
response.addCookie( firstName );
response.addCookie( lastName );
%>
<html>
<head>
<title>life.jsp</title>
</head>
<body>
<%!
private int initVar=0;
private int serviceVar=0;
private int destroyVar=0;
%>
<%!
public void jspInit(){
initVar++;
System.out.println("jspInit(): JSP被初始化了"+initVar+"次");
}
public void jspDestroy(){
destroyVar++;
System.out.println("jspDestroy(): JSP被销毁了"+destroyVar+"次");
}
%>
<%
serviceVar++;
System.out.println("_jspService(): JSP共响应了"+serviceVar+"次请求");
String content1="初始化次数 : "+initVar;
String content2="响应客户请求次数 : "+serviceVar;
String content3="销毁次数 : "+destroyVar;
%>
<h1>w3cschool教程 JSP 测试实例</h1>
<p><%=content1 %></p>
<p><%=content2 %></p>
<p><%=content3 %></p>
<ul>
<li><p><b>First Name:</b>
<%= request.getParameter("first_name")%>
</p></li>
<li><p><b>Last Name:</b>
<%= request.getParameter("last_name")%>
</p></li>
</ul>
<form action="JspTest01.jsp" method="POST">
First Name: <input type="text" name="first_name">
<br />
Last Name: <input type="text" name="last_name" />
<input type="submit" value="Submit" />
</form>
<%--获取cookie--%>
<%
Cookie cookie = null;
Cookie[] cookies = null;
cookies = request.getCookies();
if( cookies != null ){
out.println("<h2> Found Cookies Name and Value</h2>");
for (int i = 0; i < cookies.length; i++){
cookie = cookies[i];
out.print("Name : " + cookie.getName( ) + ", ");
out.print("Value: " + cookie.getValue( )+" <br/>");
}
}else{
out.println("<h2>No cookies founds</h2>");
}
%>
<%--删除cookie--%>
<%
cookies = request.getCookies();
if( cookies != null ){
out.println("<h2> Found Cookies Name and Value</h2>");
for (int i = 0; i < cookies.length; i++){
cookie = cookies[i];
if((cookie.getName( )).compareTo("first_name") == 0 ){
cookie.setMaxAge(0);
response.addCookie(cookie);
out.print("Deleted cookie: " +
cookie.getName( ) + "<br/>");
}
out.print("Name : " + cookie.getName( ) + ", ");
out.print("Value: " + cookie.getValue( )+" <br/>");
}
}else{
out.println(
"<h2>No cookies founds</h2>");
}
%>
<%--session--%>
<%
Date createTime = new Date(session.getCreationTime());
Date lastAccessTime = new Date(session.getLastAccessedTime());
String title = "Welcome Back to my website";
Integer visitCount = new Integer(0);
String visitCountKey = new String("visitCount");
String userIDKey = new String("userID");
String userID = new String("ABCD");
if (session.isNew()){
title = "Welcome to my website";
session.setAttribute(userIDKey, userID);
session.setAttribute(visitCountKey, visitCount);
}
visitCount = (Integer)session.getAttribute(visitCountKey);
visitCount = visitCount + 1;
userID = (String)session.getAttribute(userIDKey);
session.setAttribute(visitCountKey, visitCount);
%>
<table border="1" align="center">
<tr bgcolor="#949494">
<th>Session info</th>
<th>Value</th>
</tr>
<tr>
<td>id</td>
<td><% out.print( session.getId()); %></td>
</tr>
<tr>
<td>Creation Time</td>
<td><% out.print(createTime); %></td>
</tr>
<tr>
<td>Time of Last Access</td>
<td><% out.print(lastAccessTime); %></td>
</tr>
<tr>
<td>User ID</td>
<td><% out.print(userID); %></td>
</tr>
<tr>
<td>Number of visits</td>
<td><% out.print(visitCount); %></td>
</tr>
</table>
<%--日期: https://www.w3cschool.cn/jsp/jsp-handling-date.html --%>
<% Date date = new Date(); out.print( "<h2 align=\"center\">" +date.toString()+"</h2>");
%>
<%
Date dNow = new Date( );
SimpleDateFormat ft = new SimpleDateFormat ("E yyyy.MM.dd 'at' hh:mm:ss a zzz");
out.print( "<h2 align=\"center\">" + ft.format(dNow) + "</h2>");
%>
<%--页面重定向--%>
<%--<form action="001" method="POST">--%>
<form action="001" method="GET">
isSuccess: <input type="text" name="isSuccess">
<br />
<input type="submit" value="Submit" />
</form>
<%
out.print(request.getParameter("isSuccess"));
String isSuccess = request.getParameter("isSuccess");
if("1".equals(isSuccess)){
String site = new String("http://www.w3cschool.cn");
response.setStatus(response.SC_MOVED_TEMPORARILY);
response.setHeader("Location", site);
}
%>
<%--JSP 点击量统计--%>
<%
Integer hitsCount = (Integer)application.getAttribute("hitCounter");
if( hitsCount ==null || hitsCount == 0 ){
out.println("Welcome to my website!"+"<br/>");
hitsCount = 1;
}else{
out.println("Welcome back to my website!"+"<br/>");
hitsCount += 1;
}
application.setAttribute("hitCounter", hitsCount);
out.print("Total number of visits:"+hitsCount +"<br/>");
%>
<%--JSP 自动刷新--%>
<%
response.setIntHeader("Refresh", 60*24);
Calendar calendar = new GregorianCalendar();
String am_pm;
int hour = calendar.get(Calendar.HOUR);
int minute = calendar.get(Calendar.MINUTE);
int second = calendar.get(Calendar.SECOND);
if(calendar.get(Calendar.AM_PM) == 0)
am_pm = "AM";
else
am_pm = "PM";
String CT = hour+":"+ minute +":"+ second +" "+ am_pm;
out.println("Crrent Time: " + CT + "\n");
%>
</body>
</html>
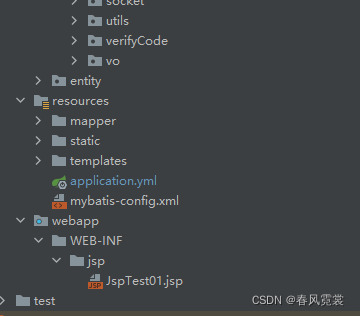
controller:
package com.example.dtest.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/JSP")
public class JspTestController {
@GetMapping("/001")
public String myVueTest(){
return "JspTest01";
}
}
测试:
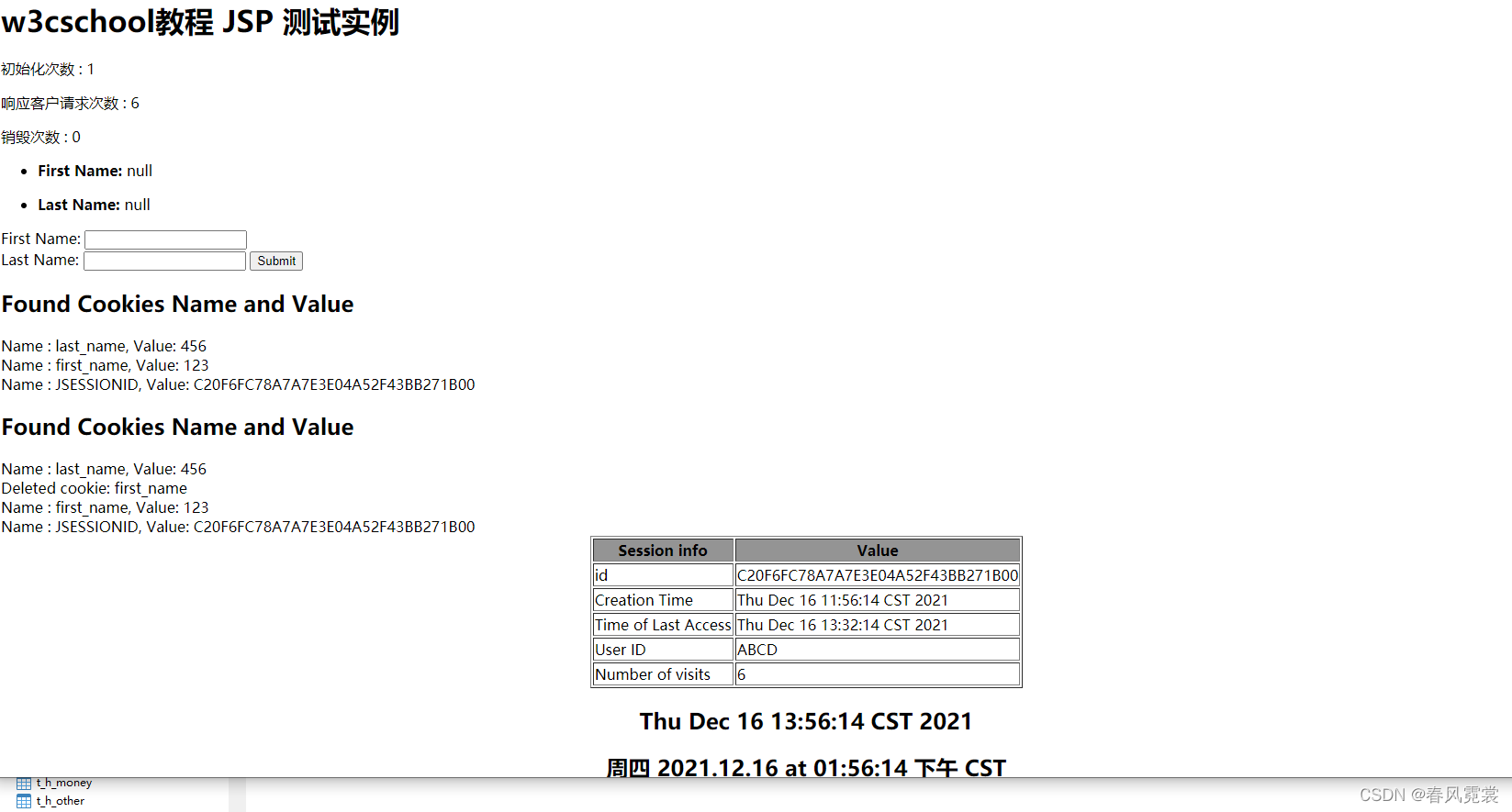
|