1、配置pom.xml坐标?cloud.tianai.captcha
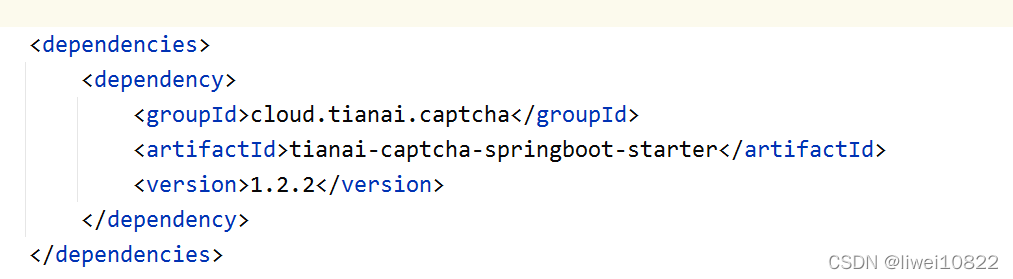
?2、配置Listener
package com.lw.utils;
import cloud.tianai.captcha.slider.SliderCaptchaApplication;
import cloud.tianai.captcha.template.slider.Resource;
import cloud.tianai.captcha.template.slider.ResourceStore;
import cloud.tianai.captcha.template.slider.SliderCaptchaResourceManager;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.context.event.ApplicationStartedEvent;
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Component;
@Component
public class CodeListener implements ApplicationListener<ApplicationStartedEvent> {
@Autowired
private SliderCaptchaApplication sliderCaptchaApplication;
@Override
public void onApplicationEvent(ApplicationStartedEvent event) {
SliderCaptchaResourceManager sliderCaptchaResourceManager = sliderCaptchaApplication.getSliderCaptchaResourceManager();
ResourceStore resourceStore = sliderCaptchaResourceManager.getResourceStore();
resourceStore.clearResources();
resourceStore.addResource(new Resource("classpath", "bgimages/a.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/b.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/c.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/d.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/e.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/g.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/h.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/i.jpg"));
resourceStore.addResource(new Resource("classpath", "bgimages/j.jpg"));
}
}
3、编写login.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="http://libs.baidu.com/jquery/2.0.0/jquery.min.js"></script>
</head>
<body>
<div class="slider">
<div class="content">
<div class="bg-img-div">
<img id="bg-img" src="" alt/>
</div>
<div class="slider-img-div">
<img id="slider-img" src="" alt/>
</div>
</div>
<div class="slider-move">
<div class="slider-move-track">
拖动滑块完成拼图
</div>
<div class="slider-move-btn"></div>
</div>
<div class="bottom">
<div class="close-btn"></div>
<div class="refresh-btn"></div>
</div>
</div>
<script>
let start = 0;
let currentCaptchaId = null;
let movePercent = 0;
const bgImgWidth = $(".slider-img-div").width();
let end = 206;
$(function () {
alert("fsfsdfsdf")
refreshCaptcha();
})
$(".slider-move-btn").mousedown((event) => {
start = event.pageX;
$(".slider-move-btn").css("background-position", "-5px 31.0092%")
window.addEventListener("mousemove", move);
window.addEventListener("mouseup", up);
});
function move(event) {
let moveX = event.pageX - start;
console.log(moveX)
if (moveX < 0) {
moveX = 0;
} else if (moveX > end) {
moveX = end;
}
// if (moveX > 0 && moveX <= end) {
$(".slider-move-btn").css("transform", "translate(" + moveX + "px, 0px)")
$(".slider-img-div").css("transform", "translate(" + moveX + "px, 0px)")
// }
movePercent = moveX / bgImgWidth;
}
function up(event) {
console.log(currentCaptchaId, movePercent, bgImgWidth);
window.removeEventListener("mousemove", move);
window.removeEventListener("mouseup", up);
valid();
}
$(".close").click(() => {
});
$(".refresh-btn").click(() => {
refreshCaptcha();
});
function valid() {
let data = {
form: "13333333333",
id: currentCaptchaId,
percentage: movePercent
};
$.get("/platform/check", {
id: currentCaptchaId,
percentage: movePercent
}, function (res) {
console.log(res);
if (res) {
alert("验证成功!!!");
}
refreshCaptcha();
});
}
function refreshCaptcha() {
$.get("/platform/code", function (data) {
reset();
currentCaptchaId = data.id;
$("#bg-img").attr("src", data.captcha.backgroundImage);
$("#slider-img").attr("src", data.captcha.sliderImage);
})
}
function reset() {
$(".slider-move-btn").css("background-position", "-5px 11.79625%")
$(".slider-move-btn").css("transform", "translate(0px, 0px)")
$(".slider-img-div").css("transform", "translate(0px, 0px)")
start = 0;
movePercent = 0;
currentCaptchaId = null;
}
</script>
</body>
</html>
4、Controller编写
@GetMapping("/code")
@ResponseBody
public CaptchaResponse<SliderCaptchaVO> genCaptcha() {
CaptchaResponse<SliderCaptchaVO> response = sliderCaptchaApplication.generateSliderCaptcha();
return response;
}
@GetMapping("/check")
@ResponseBody
public boolean checkCaptcha(@RequestParam("id") String id, @RequestParam("percentage") Float percentage) {
boolean matching = sliderCaptchaApplication.matching(id, percentage);
return matching;
}
|