java 控制台版五子棋
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Random;
import java.util.Scanner;
import java.util.regex.PatternSyntaxException;
public class ConsoleGobang {
public static final int BOARD_SIZE = 16;
public static String[][] stringArr;
public static Random random = new Random();
public static Scanner scanner = new Scanner(System.in);
public static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
public static int userX = 0;
public static int compX = 0;
public static int userY = 0;
public static int compY = 0;
public static int userXN = 0;
public static int compXN = 0;
public static int userDB = 0;
public static int compDB = 0;
public static void main(String[] args) throws IOException {
System.out.println("开始五子棋游戏!");
initCheckerBoard();
printCheckerBoard();
System.out.println("输入1玩家线下,输入2电脑线下,其他选项退出游戏:");
int i = scanner.nextInt();
if (i == 1) {
while (true) {
System.out.println("请输入下棋的坐标,格式为[x,y]:");
String line;
while ((line = br.readLine()) != null) {
try {
String[] split = line.split(",");
int x = Integer.parseInt(split[0]);
int y = Integer.parseInt(split[1]);
if (x > BOARD_SIZE || x < 1 || y > BOARD_SIZE || y < 1) {
System.out.println("棋盘上没有位置[" + x + "," + y + "],请输入[1~15]之间的数字:");
} else {
System.out.println("玩家下棋坐标[x=" + x + ",y=" + y + "]");
if (isHavePiece(x, y)) {
System.out.println("棋盘上该位置[" + x + "," + y + "]已经有棋子,请输入下棋的坐标,格式为[x,y]:");
} else {
stringArr[x][y] = "●";
break;
}
}
} catch (PatternSyntaxException | NumberFormatException e) {
System.out.println("输入坐标格式有误,请输入下棋的坐标,格式为[x,y]:");
}
}
computer();
printCheckerBoard();
if (isSuccess()) {
System.out.println("游戏结束");
return;
}
}
} else if (i == 2) {
while (true) {
computer();
printCheckerBoard();
System.out.println("请输入下棋的坐标,格式为[x,y]:");
String line;
while ((line = br.readLine()) != null) {
try {
String[] split = line.split(",");
int x = Integer.parseInt(split[0]);
int y = Integer.parseInt(split[1]);
if (x > BOARD_SIZE || x < 1 || y > BOARD_SIZE || y < 1) {
System.out.println("棋盘上没有位置[" + x + "," + y + "],请输入[1~15]之间的数字:");
} else {
System.out.println("玩家下棋坐标[x=" + x + ",y=" + y + "]");
if (isHavePiece(x, y)) {
System.out.println("棋盘上该位置[" + x + "," + y + "]已经有棋子,请输入下棋的坐标,格式为[x,y]:");
} else {
stringArr[x][y] = "●";
break;
}
}
} catch (PatternSyntaxException | NumberFormatException e) {
System.out.println("输入坐标格式有误,请输入下棋的坐标,格式为[x,y]:");
}
}
printCheckerBoard();
if (isSuccess()) {
System.out.println("游戏结束");
return;
}
}
} else {
System.out.println("退出");
}
}
public static void initCheckerBoard() {
stringArr = new String[BOARD_SIZE][BOARD_SIZE];
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (i == 0) {
stringArr[i][j] = String.valueOf(j);
} else if (j == 0) {
stringArr[i][j] = String.valueOf(i);
} else {
stringArr[i][j] = "?";
}
}
}
}
public static void printCheckerBoard() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
System.out.print(stringArr[i][j] + "\t");
}
System.out.println();
}
}
public static void computer() {
int i = random.nextInt(BOARD_SIZE - 1) + 1;
int j = random.nextInt(BOARD_SIZE - 1) + 1;
if (isHavePiece(i, j)) {
computer();
return;
} else {
System.out.println("电脑下棋坐标[x=" + i + ",y=" + j + "]");
stringArr[i][j] = "○";
}
}
public static boolean isHavePiece(int x, int y) {
return stringArr[x][y].equals("●") || stringArr[x][y].equals("○");
}
public static boolean isSuccess() {
for (int i = 0; i < stringArr.length; i++) {
for (int j = 0; j < stringArr[i].length; j++) {
if (stringArr[i][j].equals("●")) {
userX++;
} else {
userX = 0;
}
if (stringArr[i][j].equals("○")) {
compX++;
} else {
compX = 0;
}
if (compX >= 5 || userX >= 5) return true;
}
}
for (int i = 0; i < stringArr.length; i++) {
for (int j = 0; j < stringArr[i].length; j++) {
if (stringArr[j][i].equals("●")) {
userY++;
} else {
userY = 0;
}
if (stringArr[j][i].equals("○")) {
compY++;
} else {
compY = 0;
}
if (userY >= 5 || compY >= 5) return true;
}
}
for (int k = 0; k < BOARD_SIZE - 1; k++) {
for (int i = 0, j = k; i < BOARD_SIZE - 1 && j >= 0; i++, j--) {
if (stringArr[i][j].equals("●")) {
userXN++;
} else {
userXN = 0;
}
if (stringArr[i][j].equals("○")) {
compXN++;
} else {
compXN = 0;
}
if (userXN >= 5 || compXN >= 5) return true;
}
}
for (int i = 0, j = BOARD_SIZE - 1; i < BOARD_SIZE - 1 && j >= 0; i++, j--) {
if (stringArr[i][j].equals("●")) {
userXN++;
} else {
userXN = 0;
}
if (stringArr[i][j].equals("○")) {
compXN++;
} else {
compXN = 0;
}
if (userXN >= 5 || compXN >= 5) return true;
}
for (int k = 1; k < BOARD_SIZE; k++) {
for (int i = k, j = BOARD_SIZE - 1; i < BOARD_SIZE && j >= 0; i++, j--) {
if (stringArr[i][j].equals("●")) {
userXN++;
} else {
userXN = 0;
}
if (stringArr[i][j].equals("○")) {
compXN++;
} else {
compXN = 0;
}
if (userXN >= 5 || compXN >= 5) return true;
}
}
for (int k = 1; k < BOARD_SIZE; k++) {
for (int i = 0, j = k; i < BOARD_SIZE - 1 && j < BOARD_SIZE; i++, j++) {
if (stringArr[i][j].equals("●")) {
userDB++;
} else {
userDB = 0;
}
if (stringArr[i][j].equals("○")) {
compDB++;
} else {
compDB = 0;
}
if (userDB >= 5 || compDB >= 5) return true;
}
}
for (int i = 0, j = 0; i < BOARD_SIZE - 1 && j < BOARD_SIZE; i++, j++) {
if (stringArr[i][j].equals("●")) {
userDB++;
} else {
userDB = 0;
}
if (stringArr[i][j].equals("○")) {
compDB++;
} else {
compDB = 0;
}
if (userDB >= 5 || compDB >= 5) return true;
}
for (int k = 1; k < BOARD_SIZE; k++) {
for (int i = k, j = 0; i < BOARD_SIZE && j < BOARD_SIZE; i++, j++) {
if (stringArr[i][j].equals("●")) {
userDB++;
} else {
userDB = 0;
}
if (stringArr[i][j].equals("○")) {
compDB++;
} else {
compDB = 0;
}
if (userDB >= 5 || compDB >= 5) return true;
}
}
return false;
}
}
效果展示
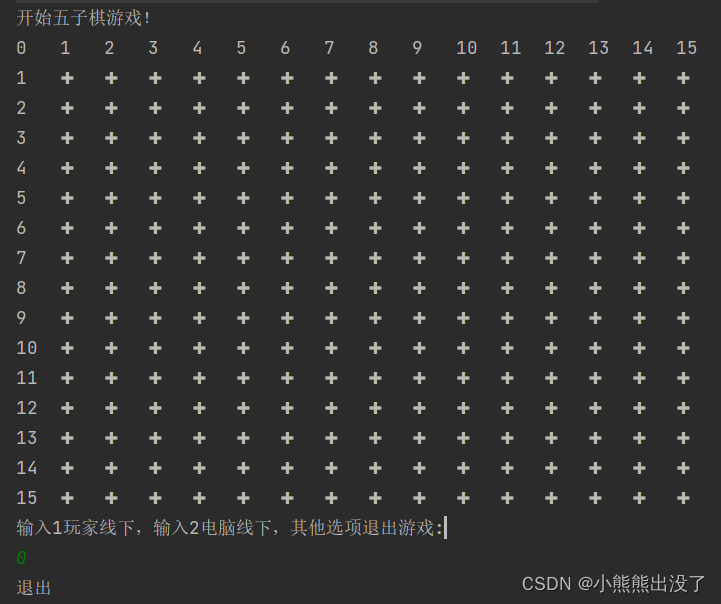 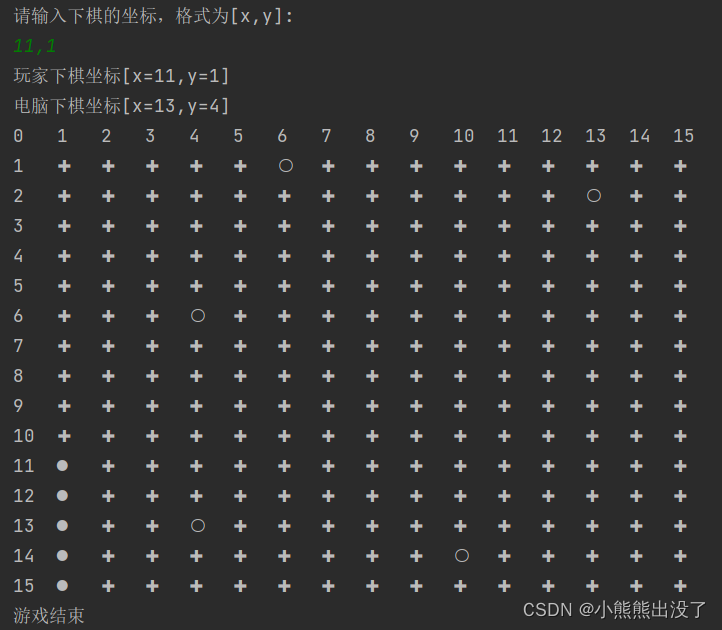
|