1.File
File 是文件或者文件夹
1.1常用方法
File file = new File("C:\\Users\\Administrator\\Desktop\\aa");
System.out.println(file.canExecute()+"\t"+
file.canRead()+"\t"+file.canWrite());
System.out.println(file.getAbsoluteFile());
System.out.println(file.getAbsolutePath());
System.out.println(file.getPath());
System.out.println(file.getParent());
System.out.println(file.getParentFile());
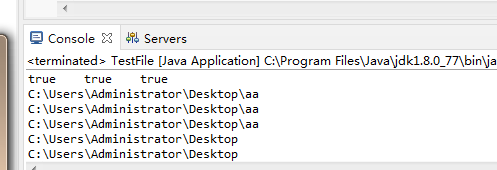 mkdir()创建指定目录 mkdirs() 创建指定目录,包含必须但不存在的父目录; isDirectory() 判断是否是一个文件夹 exists()判断文件是否存在; isFile()判断是否是一个文件; length()返回该路径名所代表的文件的长度; list() 返回一个字符串数组,这些字符串是此路径名表示的目录中的文件和目录的名字。 返回的是String的数组; listFiles();返回的是File数组;是此路径名表示的目录中的文件和文件夹;
public static void main(String[] args) {
File file = new File("C:\\Users\\Administrator\\Desktop");
String[] list1 = file.list();
for(String list:list1) {
System.out.println(list);
}
}
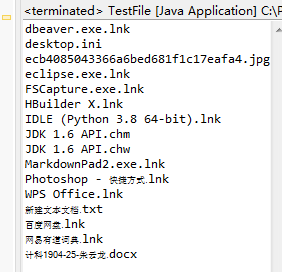
File file = new File("C:\\Users\\Administrator\\Desktop");
File[] list2 = file.listFiles();
for(File list:list2) {
System.out.println(list);
}
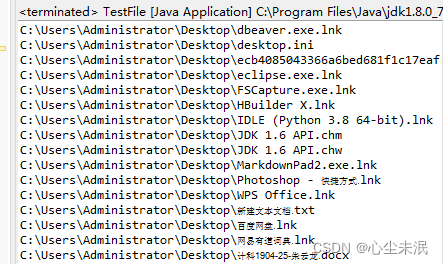
当所给路径不是文件夹,创建文件夹,若存在删除文件夹,
package com.openlab.pp;
import java.io.File;
import java.io.IOException;
public class TestFile {
public static void main(String[] args) {
File file = new File("C:\\Users\\Administrator\\Desktop\\aa");
if(!file.exists()) {
file.mkdirs();
}else{
file.delete();
System.out.println("删除成功");
}
}
}
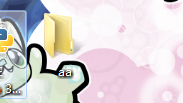 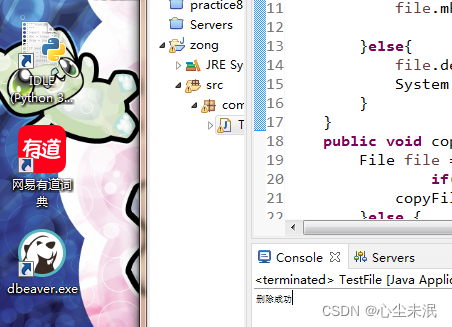 判断所给是否为文件,若不是,判断父目录是否存在,不存在则创建,再创建文件,若是文件 则删除文件;
File file = new File("C:\\Users\\Administrator\\Desktop\\aa\\1.txt");
if(!file.isFile()) {
File parents = new File(file.getParent());
if(!parents.exists()) {
file.mkdirs();
System.out.println("目录创建成功");
}
if(!file.exists()) {
try {
file.createNewFile();
System.out.println(file.getName()+"创建成功");
} catch (IOException e) {
e.printStackTrace();
}
}
}else {
file.delete();
System.out.println("删除成功");
}
注意:要想删除父目录,如果目录下还有子目录或是子文件,时不能删除的,只有它是空的才能被删除掉;
1.2将文件夹下的所有文件打印输出
public class TestFile {
public static void main(String[] args) {
String file = "C:\\Users\\Administrator\\Desktop";
copyFile(file);
}
public static void copyFile(String path) {
File file = new File(path);
File[] files = file.listFiles();
for(File list : files) {
if(list.isDirectory()) {
copyFile(list.getAbsolutePath());
}else{
System.out.println(list.getName());
}
}
}
}
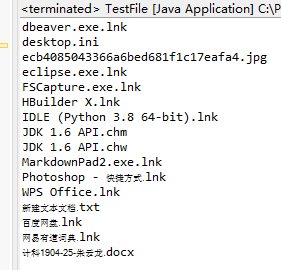
2.I/O流
2.1原理
I/O是input/output的缩写,I /O技术是用于处理设备之间的数据传输。例如读写文件,网络通讯等等。在Java程序中,对于数据的输入输出都是以流的方式进行操作的。Java.io包下提供了各种流的类与接口,可以获取不同种类的数据,通过标准的方法输入输出数据。 输入input:读取外部数据到内存中; 输出output:将程序(内存)中的数据输出到磁盘或光盘中。 根据不同的站位,流的方向有所不同,我们是站在程序的方向进行判断。 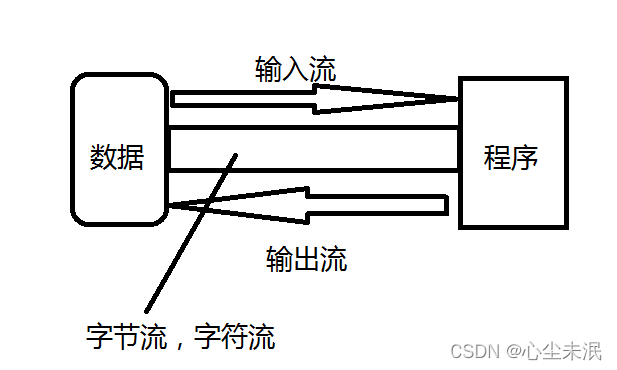
2.2流的分类
按流的操作单位分 | 按照流的流向分 | 按照流的角色分 |
---|
字节流 | 输入流 | 节点流 | 字符流 | 输出流 | 处理流 |
2.3流的体系结构划分
2.3.1节点流 / 缓冲流
基类 | 节点流 | 缓冲流 |
---|
InputStream | FileInputStream | BufferedInputStream | OutputStream | FileOutputStream | BufferedInputStream | Reader | FileReader | BufferedReader | Writer | FileWriter | BufferedWriter |
对文件进行读取
package com.openlab.pp;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import org.junit.jupiter.api.Test;
public class TestRead {
public void read01() {
File file = new File("E:\\EclipseWorkSpace\\zong\\src\\1.txt");
FileReader reader = null;
try {
reader = new FileReader(file);
int data;
while((data = reader.read()) != -1) {
System.out.print((char) data);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(reader != null) {
try {
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void read02() {
File file = new File("E:\\EclipseWorkSpace\\zong\\src\\1.txt");
FileReader reader = null;
try {
reader = new FileReader(file);
char[] buf = new char[5];
int len;
while((len = reader.read(buf)) != -1) {
System.out.print(new String(buf));
System.out.print(new String(buf,0,len));
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(reader != null) {
try {
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
|