学习了面向对象之后想写个小的案例来练习一下,接下来分享的这个小案例充分利用了面向对象的知识,封装和继承,话不多说直接上~(最后有代码总解析)
第一步创建一个父类--披萨类,然后把父类进行封装:
//父类:Pizza类
public class Pizza {
private String name;
private int size;
private double price;
public Pizza() {
}
public Pizza(String name, int size, double price) {
this.name = name;
this.size = size;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getSize() {
return size;
}
public void setSize(int size) {
this.size = size;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String show(){
return "披萨名称:" + name + "\n披萨大小:"+ size +"寸\n披萨价格:" + price+"元\n";
}
}
第二步创建一个子类水果披萨类,同样进行封装:
//子类水果披萨类
public class FruitsPizza extends Pizza{
private String Material;
@Override
public String show() {
return super.show() + "披萨材料:" + Material;
}
public FruitsPizza() {
}
public FruitsPizza(String name, int size, double price, String material) {
super(name, size, price);
Material = material;
}
public String getMaterial() {
return Material;
}
public void setMaterial(String material) {
Material = material;
}
}
第三步创建一个子类培根披萨类,也进行封装:
//子类,培根披萨类
public class BaconPizza extends Pizza{
private double g;
@Override
public String show() {
return super.show()+"披萨重量:"+g+"克";
}
public BaconPizza() {
}
public BaconPizza(String name, int size, double price, double g) {
super(name, size, price);
this.g = g;
}
public double getG() {
return g;
}
public void setG(double g) {
this.g = g;
}
}
第四步创建一个测试类:
import java.util.Scanner;//披萨测试类
public class Test {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请选择要买的披萨种类:(1.培根披萨 || 2.水果披萨)");
int choice = sc.nextInt();
switch (choice){
case 1:{
System.out.println("请输入购买尺寸:");
int size = sc.nextInt();
System.out.println("请输入购买价格:");
double price = sc.nextDouble();
System.out.println("请输入培根的克数:");
double g = sc.nextDouble();
BaconPizza B1 = new BaconPizza("培根披萨",size,price,g);
System.out.println(B1.show());
}break;
case 2:{
System.out.println("请输入加入的材料:");
String material = sc.next();
System.out.println("请输入购买尺寸:");
int size = sc.nextInt();
System.out.println("请输入购买价格:");
double price = sc.nextDouble();
FruitsPizza F1 = new FruitsPizza("水果披萨",size,price,material);
System.out.println(F1.show());
} break;
default:
System.out.println("输入有误,已退出......");
}
}
}
总结:一共是四个类。
父类有三个属性,分别是:name(披萨种类的名字)、size(披萨尺寸)、price(披萨价格)
父类还有一个show()方法,输出披萨的名称、大小、价格。
子类的水果披萨继承了父类披萨,有父类的三个属性,同时有自己的特有属性Material(水果披萨所需材料),同时重写了父类的show()方法,还加入了自己的属性输出(披萨材料)
子类培根披萨类继承了父类披萨,也有父类的三个属性,有自己的特有属性g(克数),也重写了父类的show()方法,加入了自己属性的输出。
让我们来看一下运行的效果吧!
?先选择培根披萨:
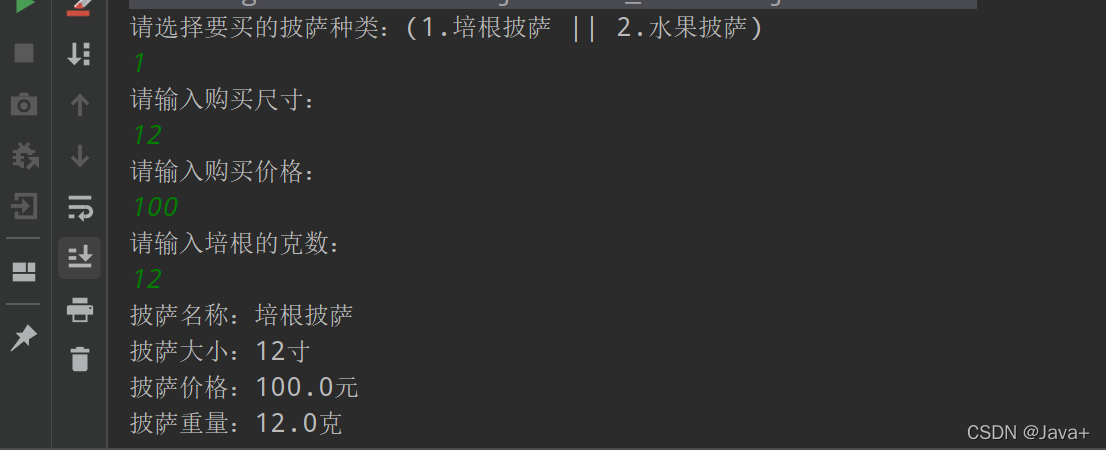
?在选择水果披萨:
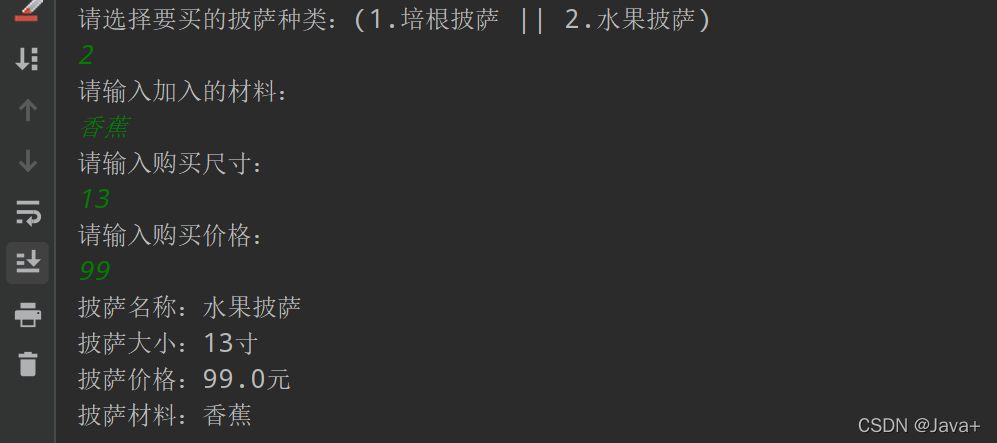
?想掌握面向对象,先把这个小案例敲20++次,然后去领悟这其中的奥秘!
|