B站黑马视频
泛型
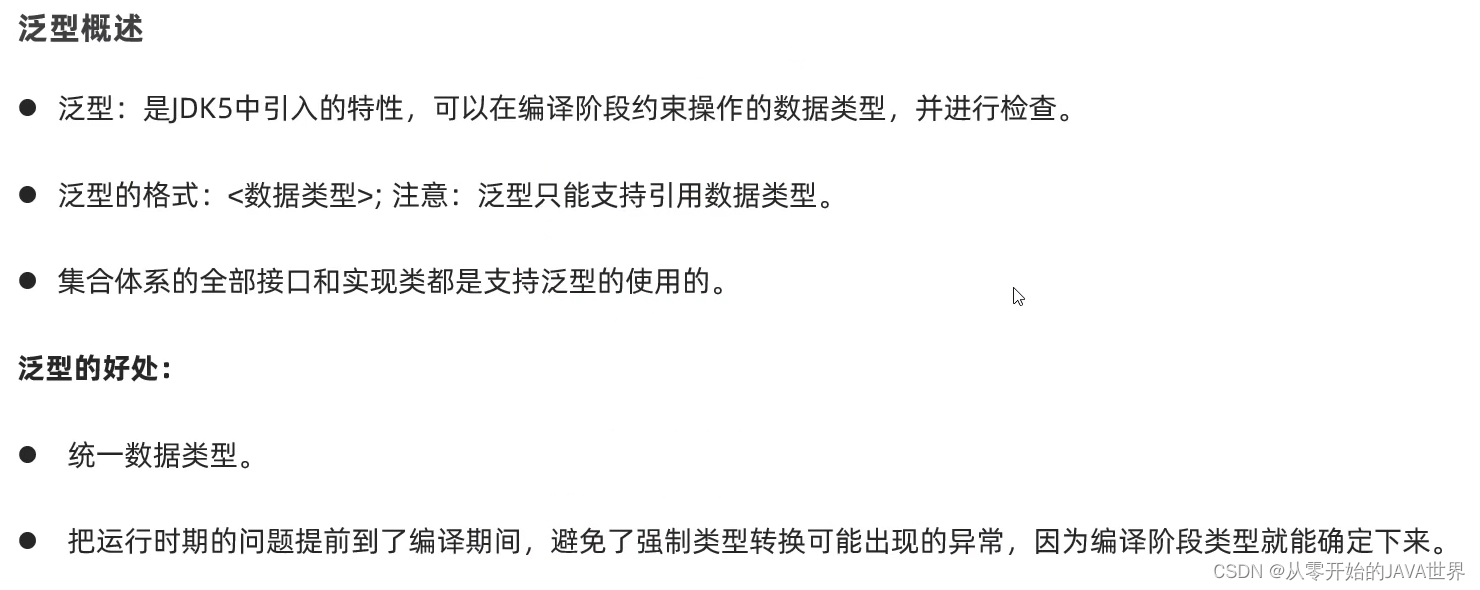
泛型类
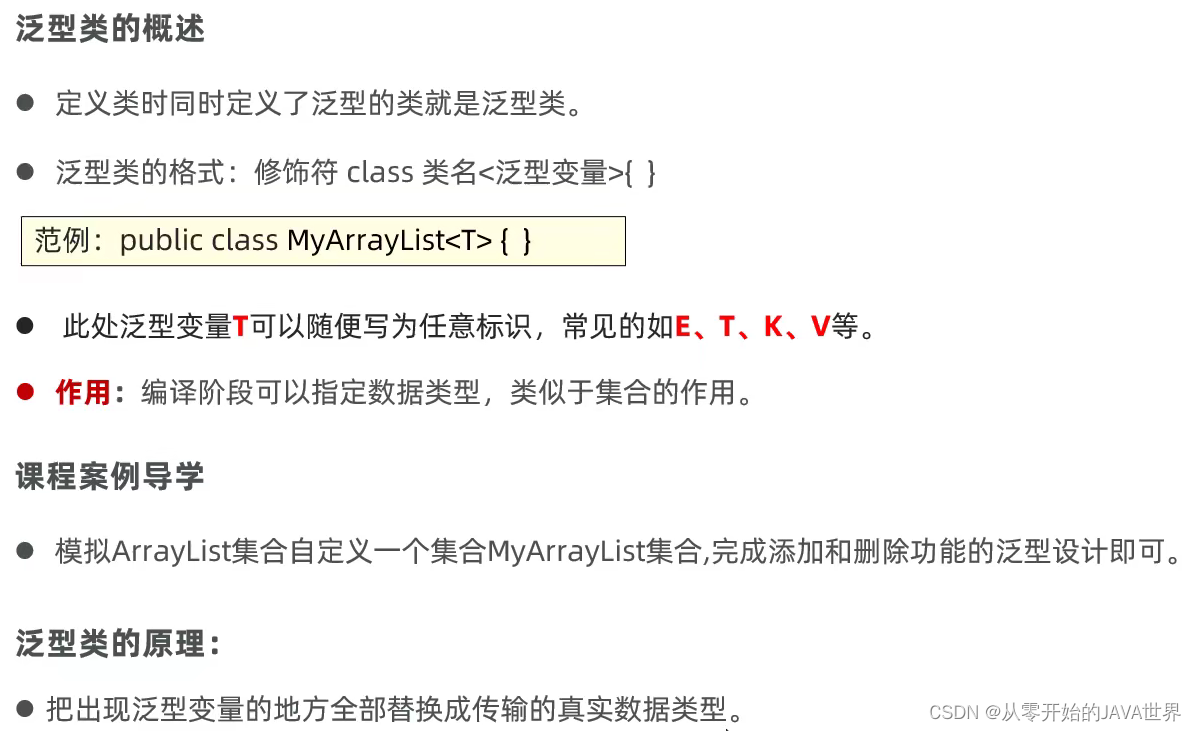
package genericity;
public class MyArrayList<E>{
public void add(E e){
}
public void remove(E e){
}
}
package genericity;
public class GenericityDemo01 {
public static void main(String[] args) {
MyArrayList<String> list = new MyArrayList<>();
}
}
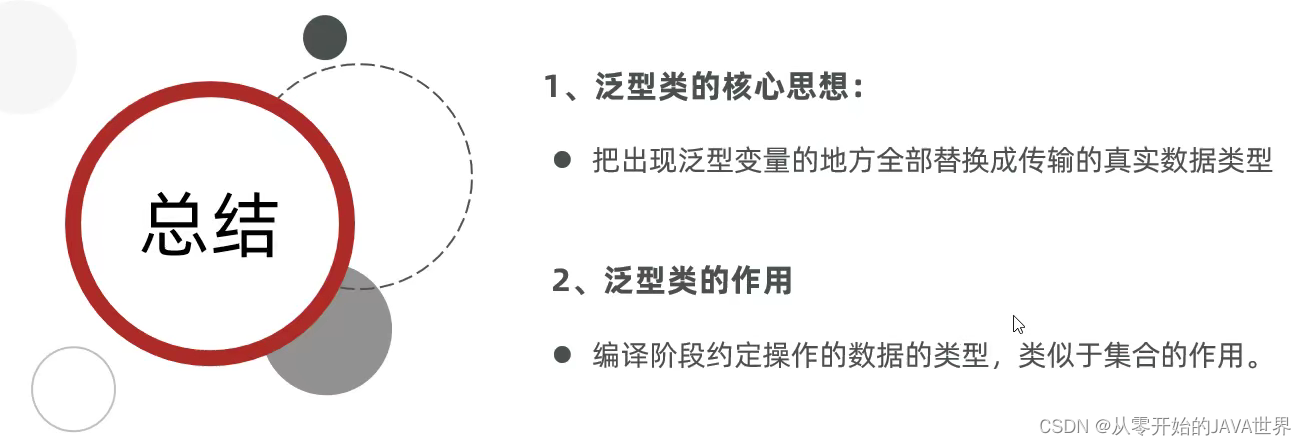
泛型方法
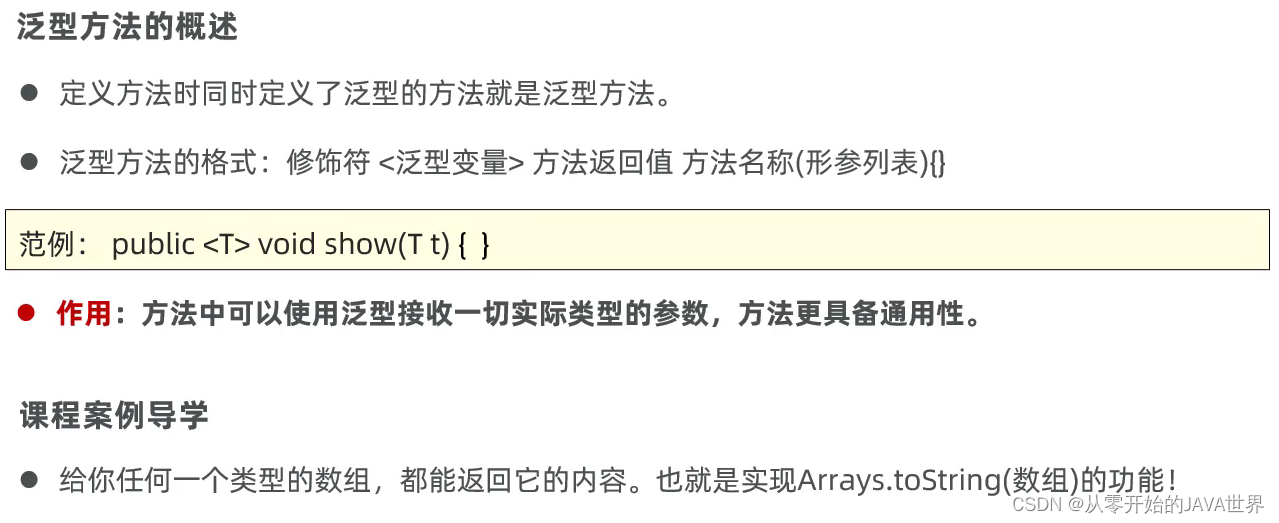
package genericity;
public class GenericityDemo01 {
public static void main(String[] args) {
printArray(new String[]{"a","b"});
}
public static <T> void printArray(T[] t){
StringBuilder sb = new StringBuilder();
if(t != null){
sb.append("[");
for (int i = 0; i < t.length; i++) {
sb.append(i==t.length-1?t[i]:t[i]+",");
}
sb.append("]");
System.out.println(sb);
}else{
System.out.println(t);
}
}
}
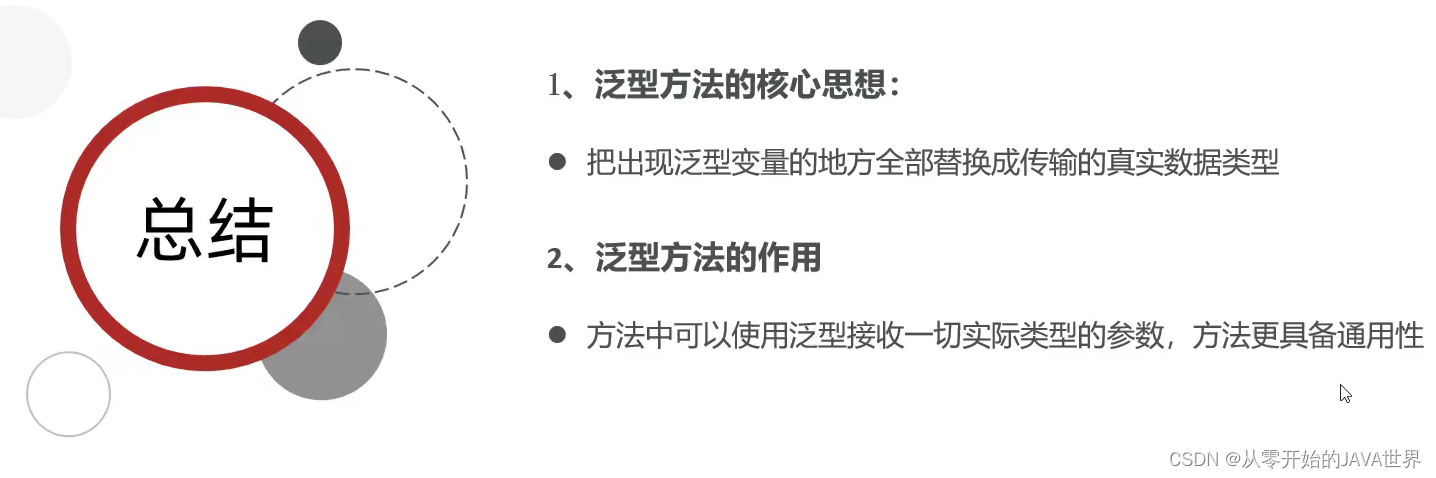
泛型接口
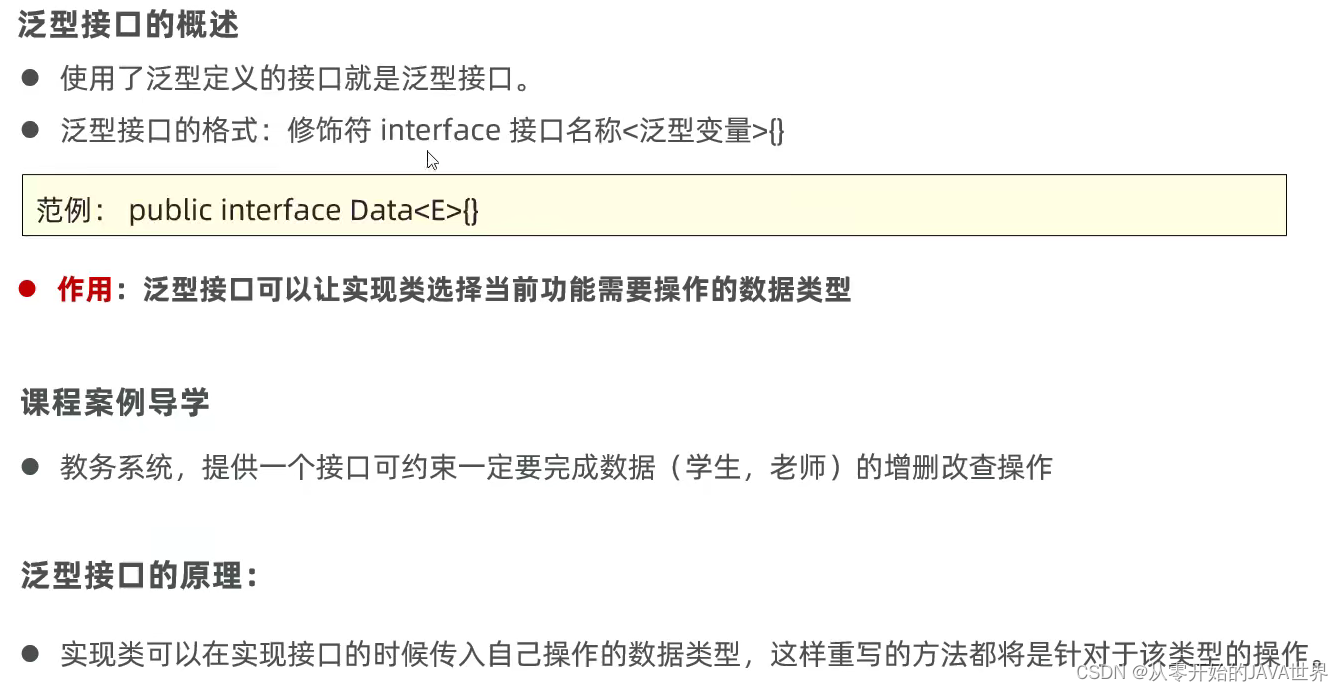
package genericity;
public class Student {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
package genericity;
public interface Date<T> {
void add(T t);
void delete(T t);
void update(T t);
T queryById(int id);
}
package genericity;
public class StudentDate implements Date<Student>{
@Override
public void add(Student student) {
}
@Override
public void delete(Student student) {
}
@Override
public void update(Student student) {
}
@Override
public Student queryById(int id) {
return null;
}
}
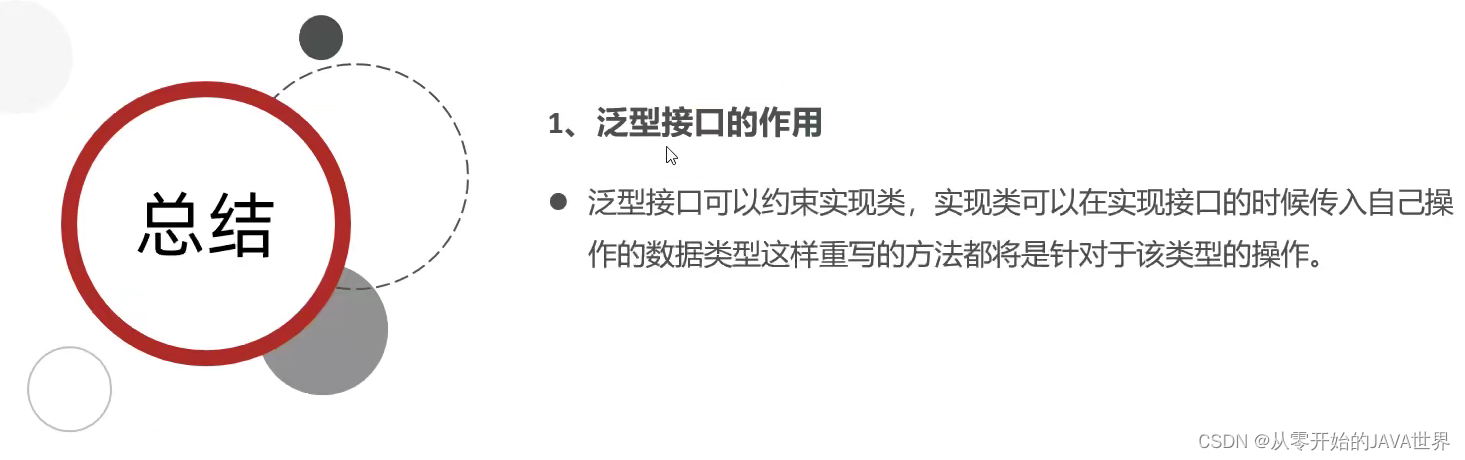
泛型通配符、上下限
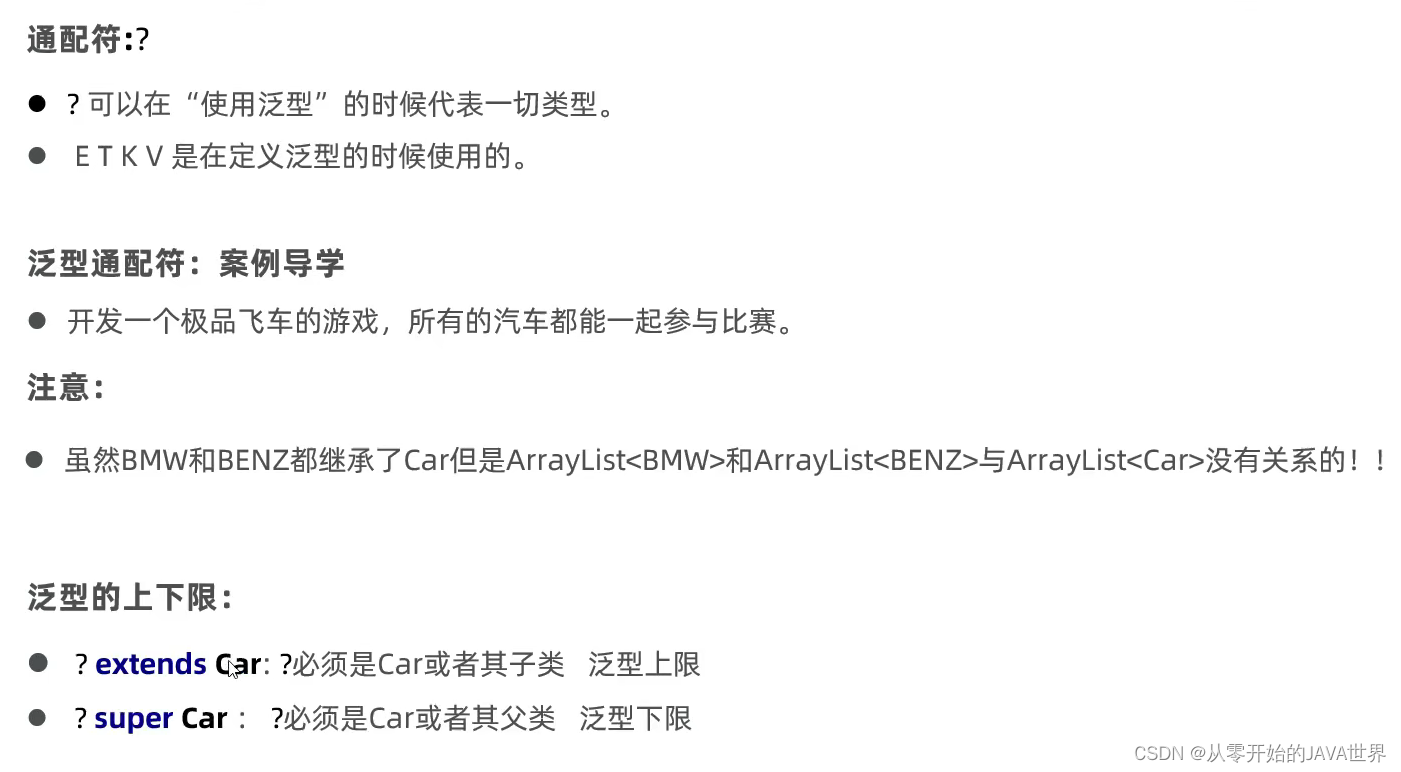
package genericity;
import java.util.ArrayList;
public class GenericityDemo {
public static void main(String[] args) {
ArrayList<BENZ> benzs = new ArrayList<>();
benzs.add(new BENZ());
go(benzs);
ArrayList<BMW> bmws = new ArrayList<>();
bmws.add(new BMW());
go(benzs);
}
public static void go(ArrayList<? extends Car> car){
}
}
class Car{}
class BENZ extends Car{}
class BMW extends Car{}
|