手写Jwt加密、解密
- Jwt的本质
- 基于Base64的编码
Base64(是一种编码方式)不是加密和解密,主要是编码和解码 基于64个可打印字符来表示二进制数据 - 例如,对于某个生成Jwt可以通过Jwt官网进行解码
Jwt官网 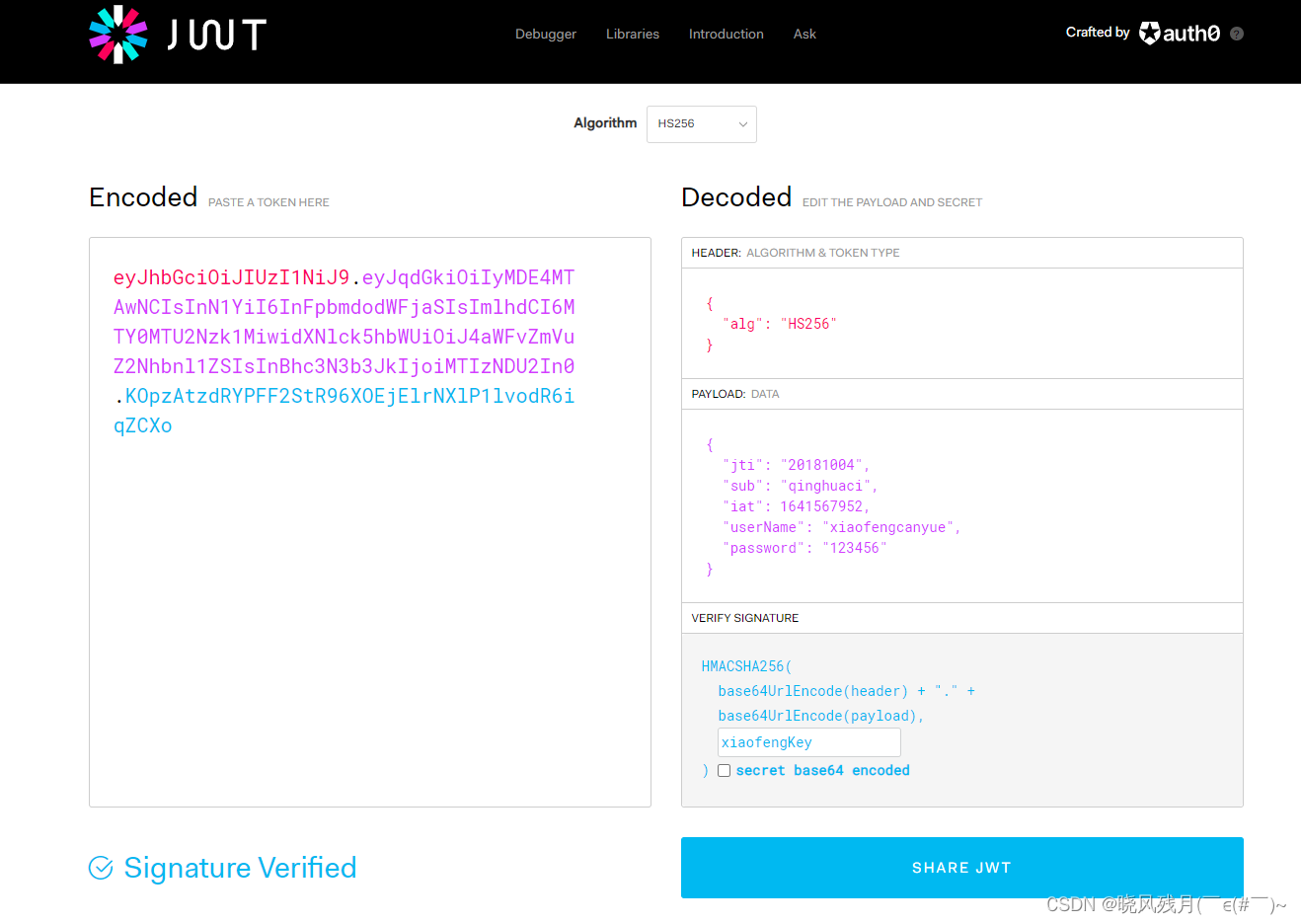 再用base64解码网站进行解码 base64编码解码网站
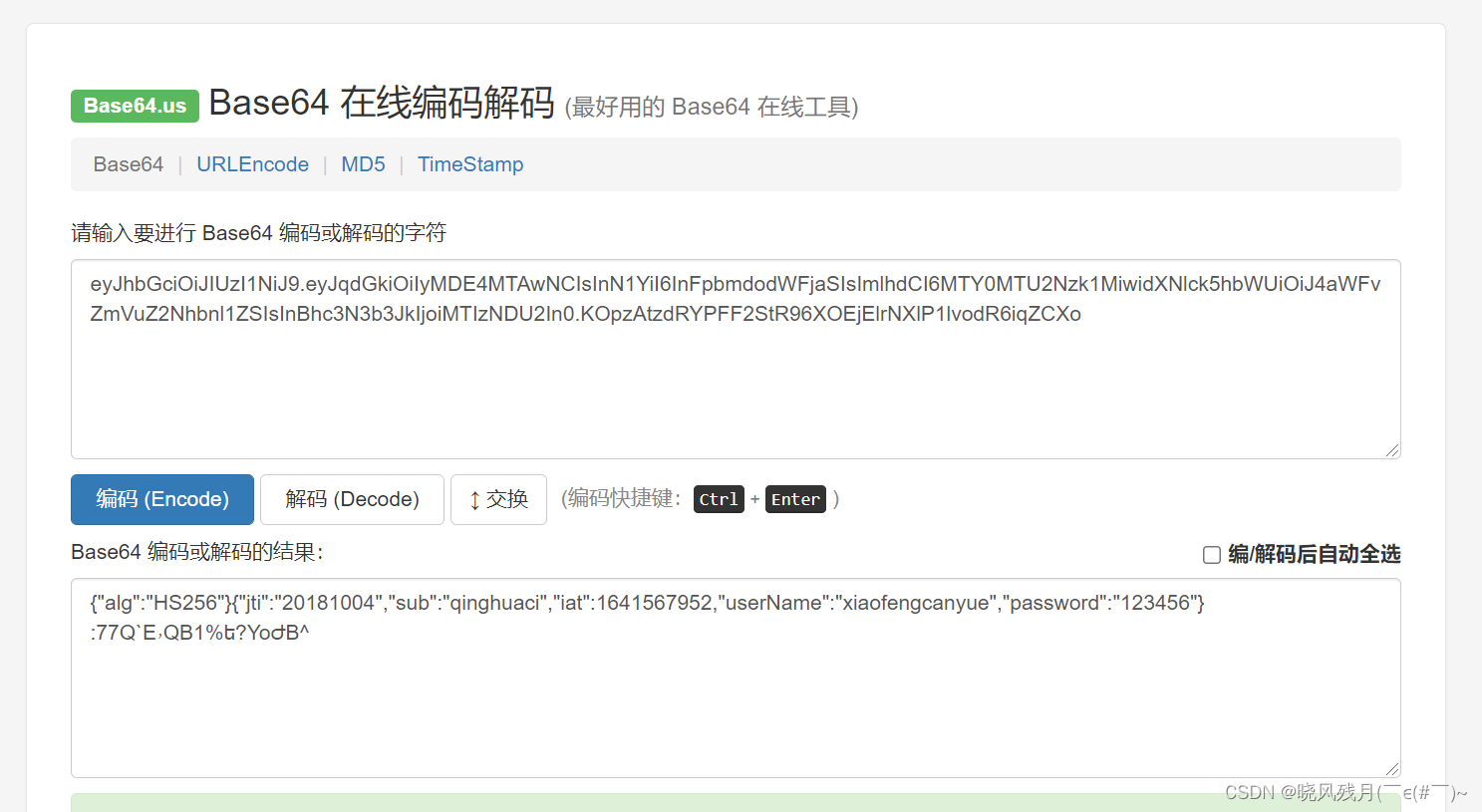 所以对数据进行base64编码即可完成Jwt数据段的编码
- 对于key的编码
使用md5 简单举例
import org.apache.commons.codec.digest.DigestUtils;
public class Jwt4MD5Demo {
public static void main(String[] args) {
String userName = "chunhuaqueyuedongxuefeng";
String sign = DigestUtils.md5Hex(userName);
System.out.println(DigestUtils.md5Hex("chunhuaqueyuedongxuefeng").equals(sign));
System.out.println(DigestUtils.md5Hex("ghghgyuedongxuefeng").equals(sign));
}
}
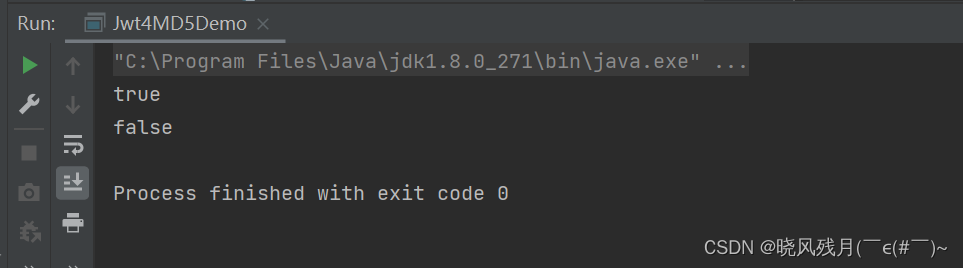
- Jwt手写
import java.io.UnsupportedEncodingException;
import java.lang.String;
import com.alibaba.fastjson.JSONObject;
import org.apache.commons.codec.digest.DigestUtils;
import java.util.Base64;
public class Jwt3HandingWriting {
public static void main(String[] args) throws UnsupportedEncodingException {
JSONObject header = new JSONObject();
header.put( "Alg", "HS256");
JSONObject payLoad = new JSONObject();
payLoad.put( "userName" , "xiaofengcanyue");
payLoad.put( "userAge" , "21");
payLoad.put( "userId" , "20181004");
payLoad.put("rd",System.currentTimeMillis());
String jwtHeader = Base64.getEncoder().encodeToString(header.toJSONString().getBytes());
String jwtPayLoad = Base64.getEncoder().encodeToString(payLoad.toJSONString().getBytes());
String signKey = "xfcyKey";
String sign = DigestUtils.md5Hex(payLoad.toJSONString()+signKey);
String jwt = jwtHeader + "." + jwtPayLoad + "." + sign;
System.out.println(jwt);
String payLoadStr = new String(Base64.getDecoder().decode(jwt.split("\\.")[1].getBytes()),"UTF-8");
String jwtSign = jwt.split("\\.")[2];
System.out.println(DigestUtils.md5Hex(payLoadStr+ signKey).equals(jwtSign));
}
}
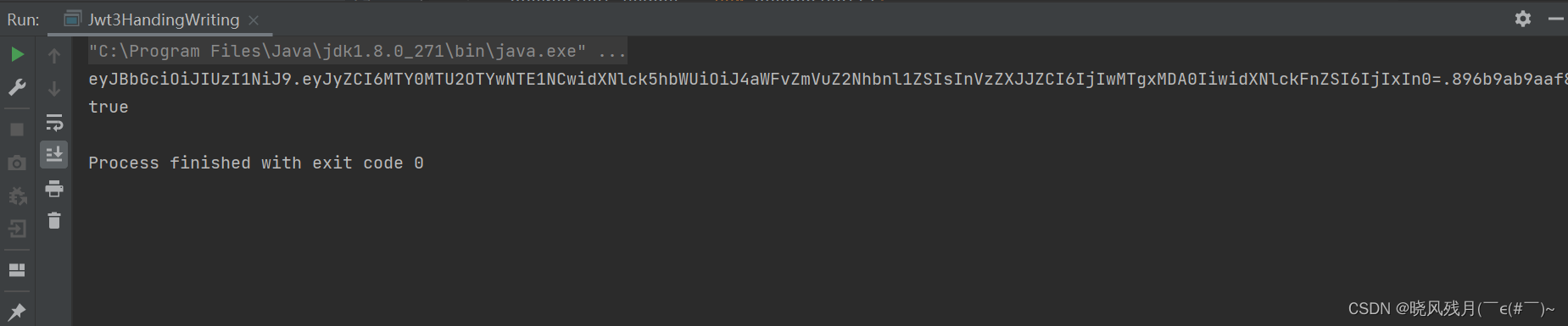
|