entity类
Apple.java
package com.spring.ioc.entity;
public class Apple {
private String title;
private String color;
private String origin;
public Apple() {
System.out.println("Apple对象已经创建,"+this);
}
public Apple(String title, String color, String origin) {
System.out.println("通过带参方法创建对象,"+this);
this.title = title;
this.color = color;
this.origin = origin;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public String getOrigin() {
return origin;
}
public void setOrigin(String origin) {
this.origin = origin;
}
}
Child.java
package com.spring.ioc.entity;
import org.w3c.dom.ls.LSOutput;
public class Child{
private String name;
private Apple apple;
public Child() {
}
public Child(String name, Apple apple) {
this.name = name;
this.apple = apple;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Apple getApple() {
return apple;
}
public void setApple(Apple apple) {
this.apple = apple;
}
public void eat(){
System.out.println(name+"吃到了"+apple.getOrigin()+"种植的"+apple.getTitle());
}
}
Spring IoC容器
对象依赖注入
- 依赖注入是指运行时将容器内对象利用反射赋给其他对象的操作
- 基于setter方法注入对象
- 基于构造方法注入对象
利用setter方法注入对象
利用类的set方法实现注入
IoC容器自动利用反射机制运行时调用setXXX方法为属性赋值
<bean id="sweetApple" class="com.imooc.spring.ioc.entity.Apple">
<property name="title" value="红富士"/>
<property name="color" value="红色"/>
<property name="origin" value="欧洲"/>
</bean>
利用setter实现对象注入
利用ref注入依赖对象
<bean id="lily" class="com.imooc.spring.ioc.entity.Child">
<property name="name" value="莉莉"/>
<property name="apple" ref="sweetApple"/>
</bean>
利用构造方法注入对象
使用constructor-arg标签来完成使用类构造方法注入对象
这个方法会根据对象的个数来动态选择构造方法
<bean id="sweetApple" class="com.imooc.spring.ioc.entity.Apple">
<constructor-arg name="title" value="红富士"/>
<constructor-arg name="origin" value="欧洲"/>
<constructor-arg name="color" value="红色"/>
</bean>
注入集合对象
注入List
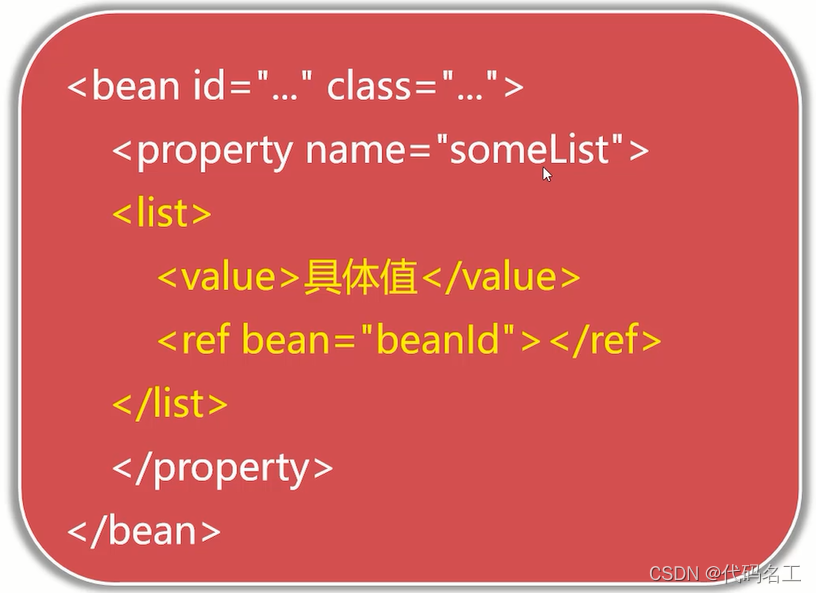
注入set
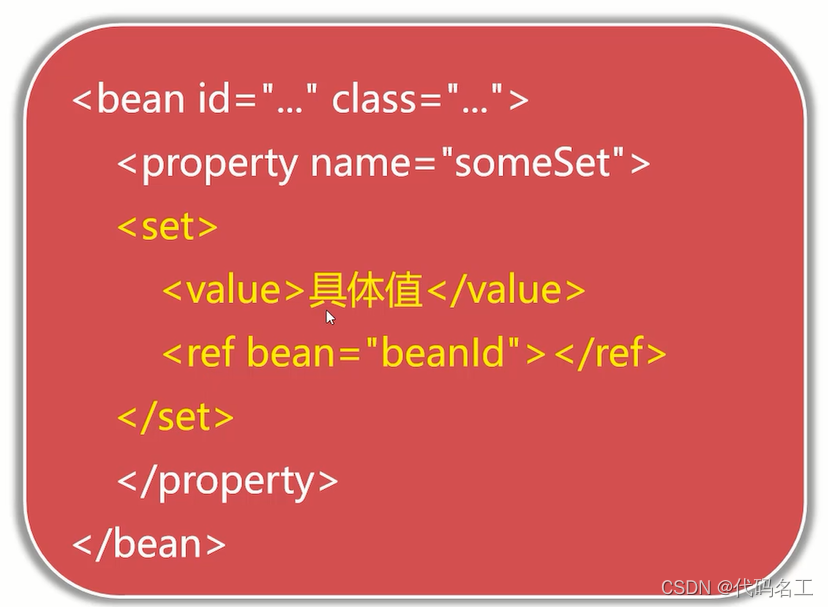
注入Map
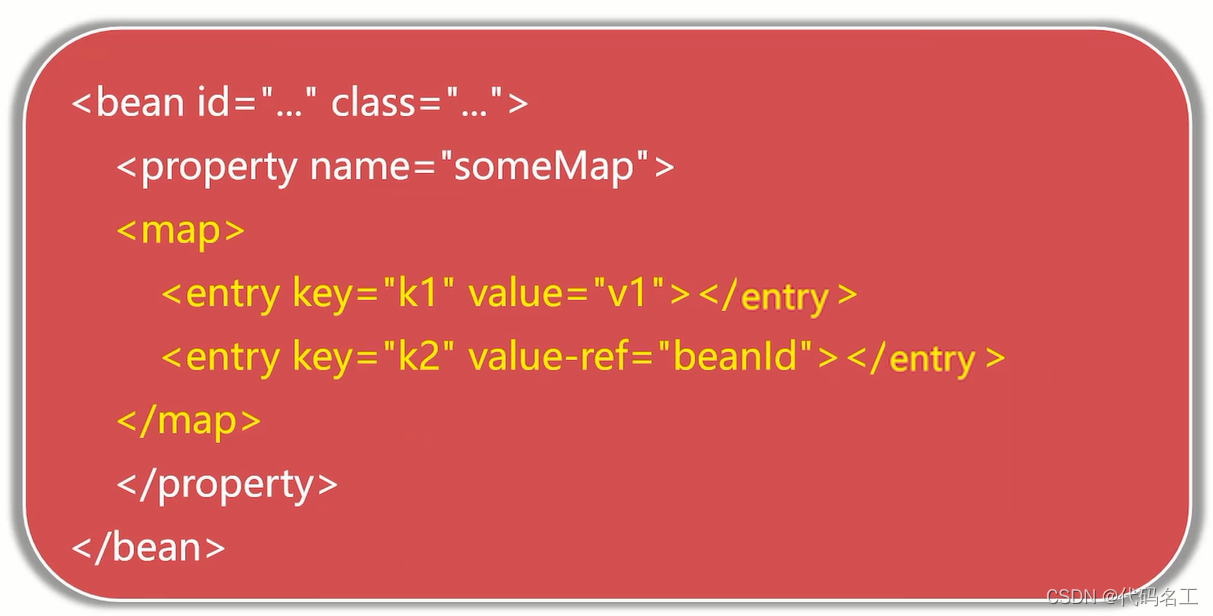
注入Properties
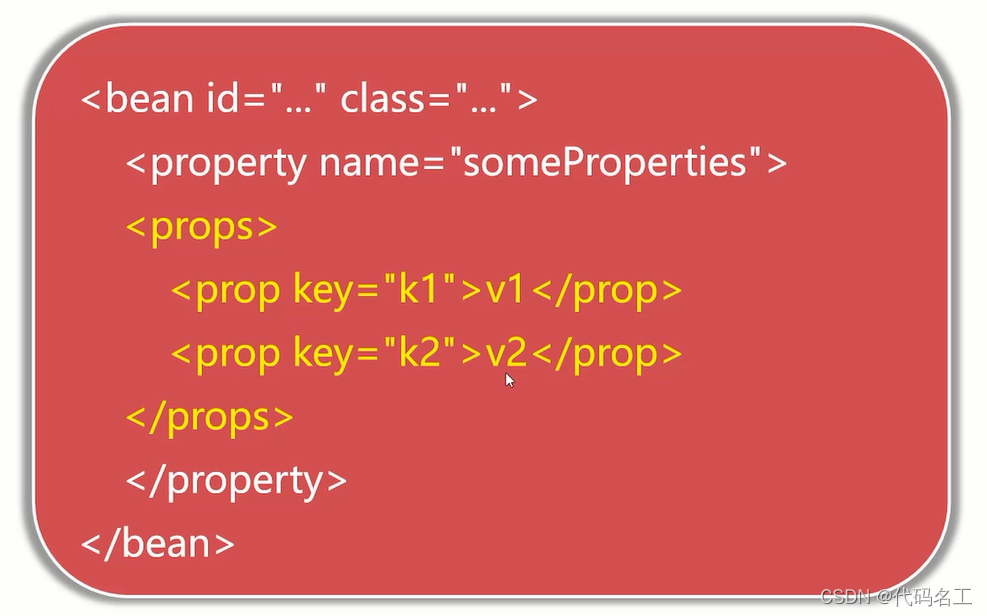
案例:
通过“公司资产配置清单”案例学习集合类型动态注入
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="c1" class="com.imooc.spring.ioc.entity.Computer">
<constructor-arg name="brand" value="联想"/>
<constructor-arg name="type" value="台式机"/>
<constructor-arg name="sn" value="8389283012"/>
<constructor-arg name="price" value="3085"/>
</bean>
<bean id="company" class="com.imooc.spring.ioc.entity.Company">
<property name="rooms">
<list>
<value>2001-总裁办</value>
<value>2003-总经理办公室</value>
<value>2010-研发部会议室</value>
</list>
</property>
<property name="computers">
<map>
<entry key="dev-88172" value-ref="c1"/>
<entry key="dev-88173">
<bean class="com.imooc.spring.ioc.entity.Computer">
<constructor-arg name="brand" value="联想"/>
<constructor-arg name="type" value="台式机"/>
<constructor-arg name="sn" value="8389283012"/>
<constructor-arg name="price" value="3085"/>
</bean>
</entry>
</map>
</property>
<property name="info">
<props>
<prop key="phone">010-12345678</prop>
<prop key="address">北京市朝阳区xx路xx大厦</prop>
<prop key="website">https://www.xxx.com</prop>
</props>
</property>
</bean>
</beans>
Company.java
package com.imooc.spring.ioc.entity;
import java.util.List;
import java.util.Map;
import java.util.Properties;
public class Company {
private List<String> rooms;
private Map<String,Computer> computers;
private Properties info;
public List<String> getRooms() {
return rooms;
}
public void setRooms(List<String> rooms) {
this.rooms = rooms;
}
public Map<String, Computer> getComputers() {
return computers;
}
public void setComputers(Map<String, Computer> computers) {
this.computers = computers;
}
public Properties getInfo() {
return info;
}
public void setInfo(Properties info) {
this.info = info;
}
@Override
public String toString() {
return "Company{" +
"rooms=" + rooms +
", computers=" + computers +
", info=" + info +
'}';
}
}
Computer.java
package com.imooc.spring.ioc.entity;
public class Computer {
private String brand;
private String type;
private String sn;
private Float price;
public Computer() {
}
public Computer(String brand, String type, String sn, Float price) {
this.brand = brand;
this.type = type;
this.sn = sn;
this.price = price;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getSn() {
return sn;
}
public void setSn(String sn) {
this.sn = sn;
}
public Float getPrice() {
return price;
}
public void setPrice(Float price) {
this.price = price;
}
@Override
public String toString() {
return "Computer{" +
"brand='" + brand + '\'' +
", type='" + type + '\'' +
", sn='" + sn + '\'' +
", price=" + price +
'}';
}
}
SpringApplication.java
package com.imooc.spring.ioc;
import com.imooc.spring.ioc.entity.Company;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class SpringApplication {
public static void main(String[] args) {
ApplicationContext context=new ClassPathXmlApplicationContext("classpath:applicationContext.xml");
Company company = context.getBean("company", Company.class);
System.out.println(company);
String website = company.getInfo().getProperty("website");
System.out.println(website);
}
}
查看容器内对象
getBeanDefinitionNames()方法获取容器内所有beanId的Id数组
getBeanDefinitionCount()方法获取容器内bean的个数
String[] names = context.getBeanDefinitionNames();
for(String name:names){
System.out.println(name);
}
int count = context.getBeanDefinitionCount();
System.out.println(count);
该方法只能获取除内部bean外的bean website = company.getInfo().getProperty(“website”); System.out.println(website); } }
## 查看容器内对象
getBeanDefinitionNames()方法获取容器内所有beanId的Id数组
getBeanDefinitionCount()方法获取容器内bean的个数
```java
//获取容器内所有beanId的Id数组
String[] names = context.getBeanDefinitionNames();
for(String name:names){
System.out.println(name);
}
//获取容器内bean的个数
int count = context.getBeanDefinitionCount();
System.out.println(count);
该方法只能获取除内部bean外的bean
|