-
假如现在有这样一张表:
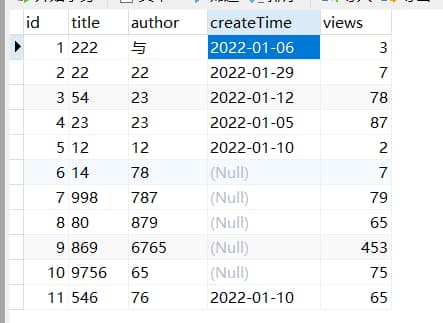
-
实体类
package com.lys.model;
import java.util.Date;
public class Blog {
private int id;
private String title;
private String author;
private Date createTime;
private int views;
public Blog() {
}
public Blog(int id, String title, String author, Date createTime, int views) {
this.id = id;
this.title = title;
this.author = author;
this.createTime = createTime;
this.views = views;
}
}
-
接口
public interface BlogDAO {
List<Blog> selectAll();
}
对应的mapper文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.lys.dao.BlogDAO">
<sql id="select_fields">
id,title,author,createTime,views
</sql>
<select id="selectAll" resultType="blog">
select
<include refid="select_fields"></include>
from blog
</select>
</mapper>
-
测试:
public class BlogTest {
private SqlSession sqlSession = MybatisUtils.getSqlSession();
@Test
public void test1(){
BlogDAO mapper = sqlSession.getMapper(BlogDAO.class);
PageHelper.startPage(2, 3);
List<Blog> blogs = mapper.selectAll();
Page<Blog> blogPage = (Page<Blog>) blogs;
System.out.println("当前页码" + blogPage.getPageNum());
System.out.println("总页数:" + blogPage.getPages());
System.out.println("页面大小:"+blogPage.getPageSize());
for (Blog blog : blogs) {
System.out.println(blog);
}
}
}
结果:
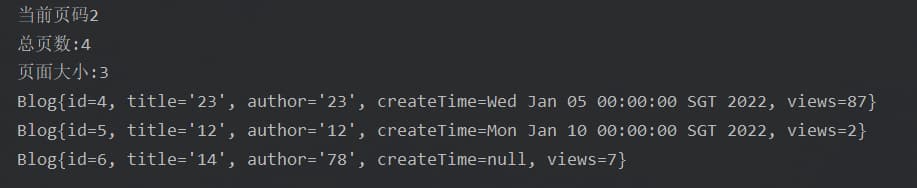
补充:Page这个类里还有好多方法属性,比如总页数,开始行,最后一行,等等,如下:
public class Page<E> extends ArrayList<E> {
private static final long serialVersionUID = 1L;
private int pageNum;
private int pageSize;
private int startRow;
private int endRow;
private long total;
private int pages;
private boolean count;
private Boolean countSignal;
private String orderBy;
private boolean orderByOnly;
private Boolean reasonable;
private Boolean pageSizeZero;
}
可以根据实际需要获取相应的分页属性值。可以尝试一下哦!
是不是很方便呢
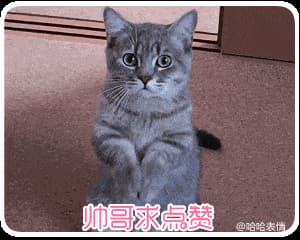