项目目录
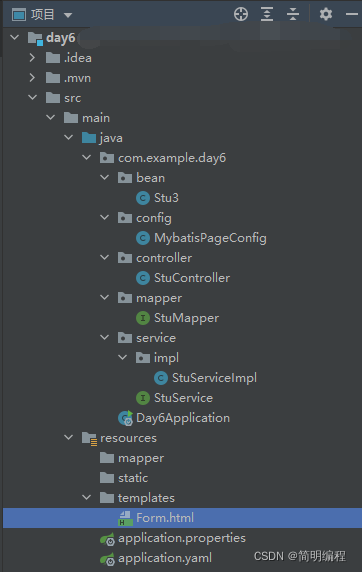
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.2</version>
<relativePath/>
</parent>
<groupId>com.example</groupId>
<artifactId>day6</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>day6</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.1</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.16</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.8</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
核心配置
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.16</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.8</version>
</dependency>
实体类
package com.example.day6.bean;
import com.baomidou.mybatisplus.annotation.TableName;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
@TableName("stu3")
public class Stu3 {
private int id;
private String name;
private int age;
private String sex;
private int math;
}
@TableName注解
用于指定实体类对应的表名
@TableName("stu3")
分页控制配置类
package com.example.day6.config;
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.extension.plugins.MybatisPlusInterceptor;
import com.baomidou.mybatisplus.extension.plugins.inner.PaginationInnerInterceptor;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MybatisPageConfig {
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor() {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
PaginationInnerInterceptor paginationInnerInterceptor = new PaginationInnerInterceptor(DbType.H2);
paginationInnerInterceptor.setMaxLimit(50L);
interceptor.addInnerInterceptor(paginationInnerInterceptor);
return interceptor;
}
}
文档
https://baomidou.com/pages/8f40ae/
Mapper
package com.example.day6.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.example.day6.bean.Stu3;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface StuMapper extends BaseMapper<Stu3> {
}
Service接口
package com.example.day6.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.example.day6.bean.Stu3;
public interface StuService extends IService<Stu3> {
}
Service实现类
package com.example.day6.service.impl;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.example.day6.bean.Stu3;
import com.example.day6.mapper.StuMapper;
import com.example.day6.service.StuService;
import org.springframework.stereotype.Service;
@Service
public class StuServiceImpl extends ServiceImpl<StuMapper,Stu3> implements StuService {
}
Controller
package com.example.day6.controller;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.example.day6.bean.Stu3;
import com.example.day6.service.StuService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import java.util.List;
@Controller
public class StuController {
@Autowired
StuService service;
@GetMapping("/form")
public String stu_table(@RequestParam(value = "png",defaultValue = "1") Integer png,Model model){
List<Stu3> list = service.list();
Page<Stu3> page = new Page<Stu3>(png,2);
Page<Stu3> resultPage = service.page(page, null);
long current = resultPage.getCurrent();
long pages = resultPage.getPages();
long total = resultPage.getTotal();
List<Stu3> records = resultPage.getRecords();
model.addAttribute("page",page);
return "Form";
}
@GetMapping("/stu/delete/{id}")
public String deleteStu(@PathVariable("id") Long id,
@RequestParam(value = "png",defaultValue = "1") Integer png,
RedirectAttributes attributes){
service.removeById(id);
attributes.addAttribute("png",png);
return "redirect:/form";
}
}
Form.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
td{
height: 30px;
width: 120px;
}
#pageNum{
height: 40px;
width: 40px;
border-radius: 50%;
background-color: silver;
line-height: 40px;
text-align: center;
}
#pageNum a{
display: inline-block;
height: 100%;
width: 100%;
}
</style>
</head>
<body>
<h1>Form 表单</h1>
<table>
<thead>
<tr>
<td>id</td>
<td>name</td>
<td>age</td>
<td>sex</td>
<td>math</td>
<td>删除</td>
</tr>
</thead>
<tbody>
<tr th:each="stu,index:${page.records}">
<td th:text="${stu.id}"></td>
<td th:text="${stu.name}"></td>
<td th:text="${stu.age}"></td>
<td th:text="${stu.sex}"></td>
<td th:text="${stu.math}"></td>
<td>
<a th:href="@{/stu/delete/{id}(id=${stu.id},png=${page.current})}">删除</a>
</td>
</tr>
</tbody>
</table>
<div>当前:[[${page.current}]],共计[[${page.pages}]]页</div>
<div>共计:[[${page.total}]]条记录</div>
<div>
<ul>
<li id="pageNum" th:each="pageNum:${#numbers.sequence(1,page.pages)}">
<a th:href="@{/form(png=${pageNum})}">
[[${pageNum}]]
</a>
</li>
</ul>
</div>
</body>
</html>
展示
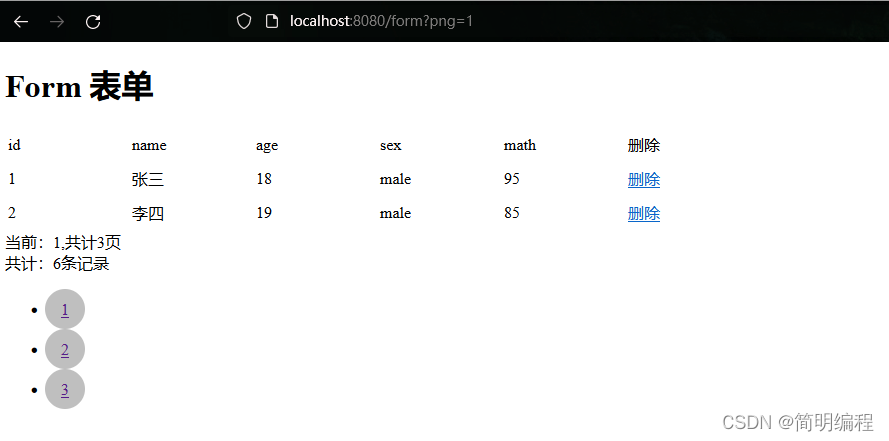
|