目录
1.常见Java包
2.Object类
3.包装类
4.字符串类
5.Math类
6.日期类
1.常见Java包
(1)java.lang
Java语言包,包含String、StringBuffer、Integer、Math、System。
任何类中,该包中的类都会被自动导入。
(2)java.util
包含一些实用的工具类(包含list、calendar、date等类)
(3)java.io
提供多种输入/输出功能的类
(4)java.net
提供网络应用功能的类
2.Object类
- Object是Java语言中唯一一个没有父类的类。
- Object类是所有类的超类:一个类可以不是Object类的直接子类,但一定是Object类的子类,Java中的每一个类都是从Object扩展来的。
?
Object类中定义的方法
(1)public boolean equals(Object obj)
比较两个对象引用的值是否相等(比较哈希地址)
①equals()方法和==运算符的区别
- 默认情况下(即没有被重写时)equals()只能比较引用类型,"=="既能比较引用类型又能比较基本类型。
- equals()方法从Object类继承,即比较对象引用的值。
- 一般都被子类方法覆盖,不再比较引用的值
(2)public int hashCode()
返回十进制整数,唯一标识一个对象
(3)public String toString()
返回类名@hashcode
(4)例子
package test;
public class Test {
public static void main(String[] args) {
Object o1 = new Object();
Object o2 = new Object();
System.out.println("o1=o2?" + o1.equals(o2));//false;比较对象引用的值,所以不相等
System.out.println(o1 == o2);//false;比较对象引用的值
System.out.println(o1);//java.lang.Object@15db9742
System.out.println(o1.hashCode());//366712642;返回该对象的十进制哈希码
System.out.println(Integer.toHexString(o1.hashCode()));//15db9742;十进制转16进制
System.out.println(o1.toString());//java.lang.Object@15db9742
}
}
3.包装类
基本数据类型不是对象层次结构的组成部分。有时需要像处理对象一样处理这些基本数据类型,可通过相应的“包装类”来将其“包装”后使用。 (1)基本数据类型和对应包装类
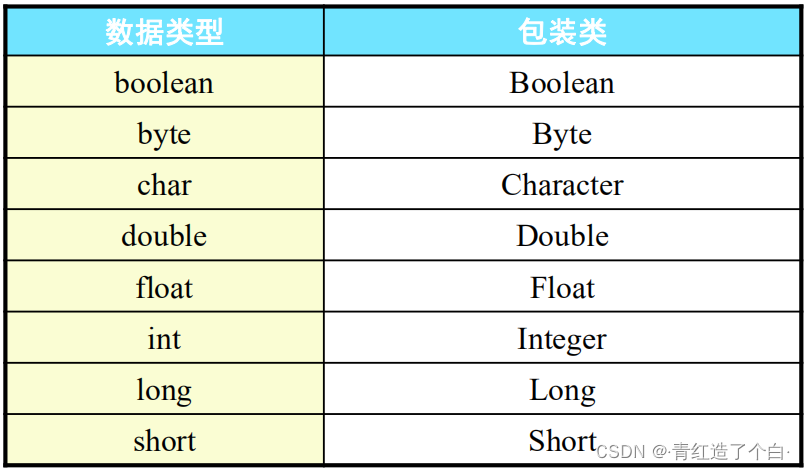
?(2)整型最大值、最小值
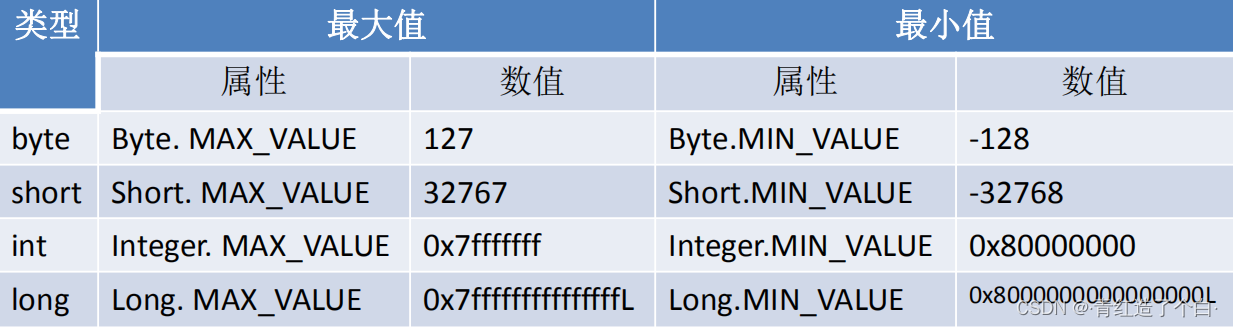
(3)使用Character对字符判断
?(4)字符串与基本数据类型、包装类型转换图
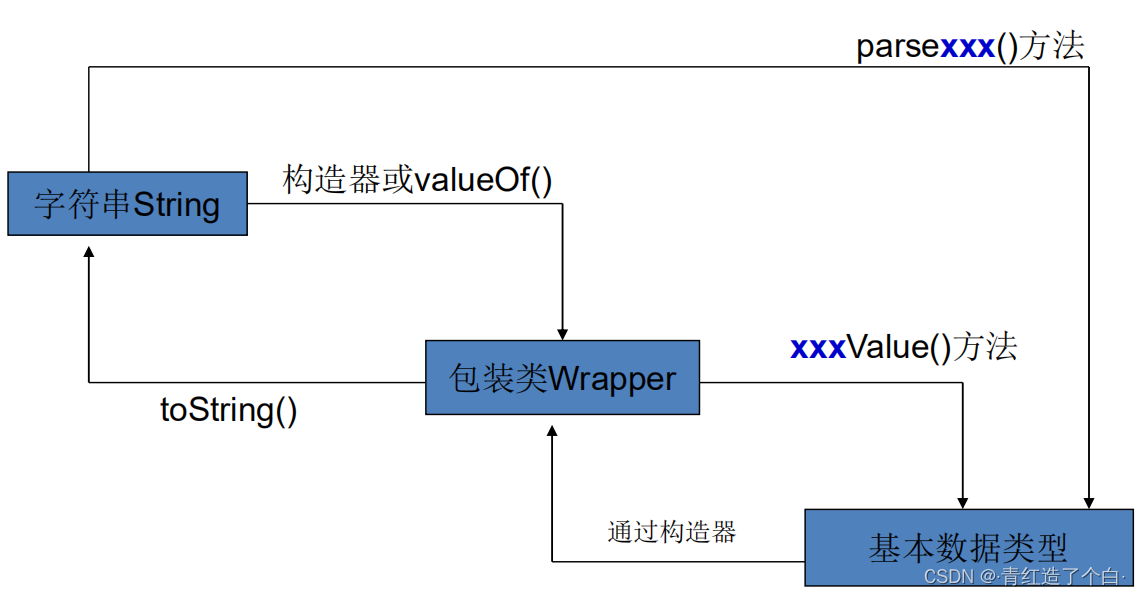
?(5)自动装箱和自动拆箱
①自动装箱:基本类型转换为对应的包装类
②自动拆箱:包装类转换为对应的基本类型
?(6)例子
public class Test {
public static void main(String[] args) {
String string = "Hello World 123456!";
char[] array = string.toCharArray();
for (char c : array) {
if(Character.isDigit(c)){//数字
System.out.println(c + "是数字");
}
else if(Character.isLetter(c)){//英文
System.out.println(c + "是英文");
}
else{//其他字符
System.out.println(c + "是其他字符");
}
}
int number = 10;
//int 转换为 Integer
Integer num = new Integer(number);
// Integer 转换为 int
int value = num.intValue();
//int 转为 String
String str = value + "";
//Integer 转为 String
str = num.toString();
//自动装箱:基本类型转换为对应的包装类
int nn = 12;
Integer n = nn;
//自动拆箱:包装类转换为对应的基本类型
int it = n;
}
}
4.字符串类
字符串是我们在编程中最常使用的一种数据类型,它的表现形式可以分为两种:
(1)String
①创建String的两种方式
?两种方式的区别:
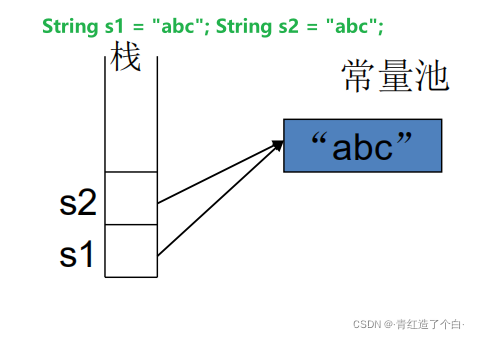
?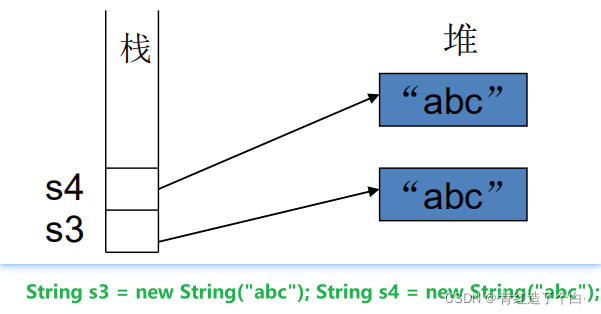
?②String类常用方法
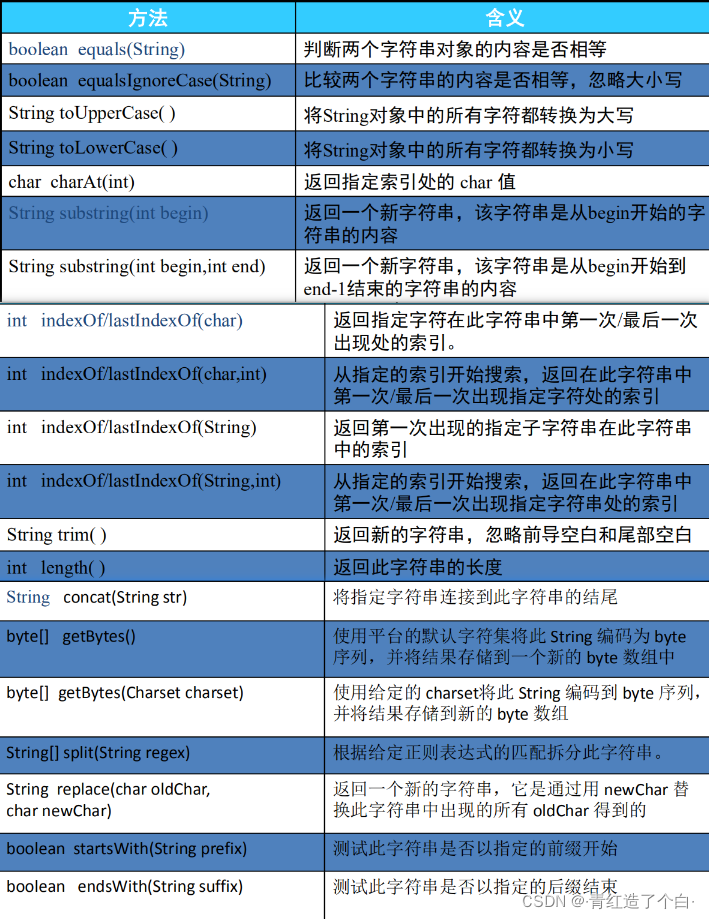
package com.lqh.pratice;
public class Test {
public static void main(String[] args) {
String str1 = "Abc";//静态方式
String str2 = new String("aBC");//动态方式
System.out.println(str1.equals("123"));//true
System.out.println("Abc".equalsIgnoreCase(str2));//true
System.out.println(str1.toUpperCase());//ABC
System.out.println(str1.toLowerCase());//abc
System.out.println(str1.charAt(1));//b
System.out.println("132456".substring(2));//2456
System.out.println("123456".substring(2, 3));//3
System.out.println("1221".indexOf("1") + "\t" + "1221".lastIndexOf("1"));//0 3
System.out.println(" AGB ".trim());//AGB
System.out.println(str1.length());//3
System.out.println(str1.concat(str2));//AbcaBC
System.out.println("@123@jl".replace('@', '-'));//-123-jl
System.out.println("123".startsWith("1"));//true
System.out.println("123".endsWith("3"));//true
}
}
(2)StringBuffer?
①创建StringBuffer对象:
- 构造一个不带字符的字符串缓冲区,默认初始容量为:16
StringBuffer 变量名 = new StringBuffer () ;
- 构造一个指定初始容量为capacity的字符串缓冲区
StringBuffer 变量名 = new StringBuffer (int capacity ) ;
StringBuffer 变量名 = new StringBuffer (String value) ;?
②StringBuffer常用方法
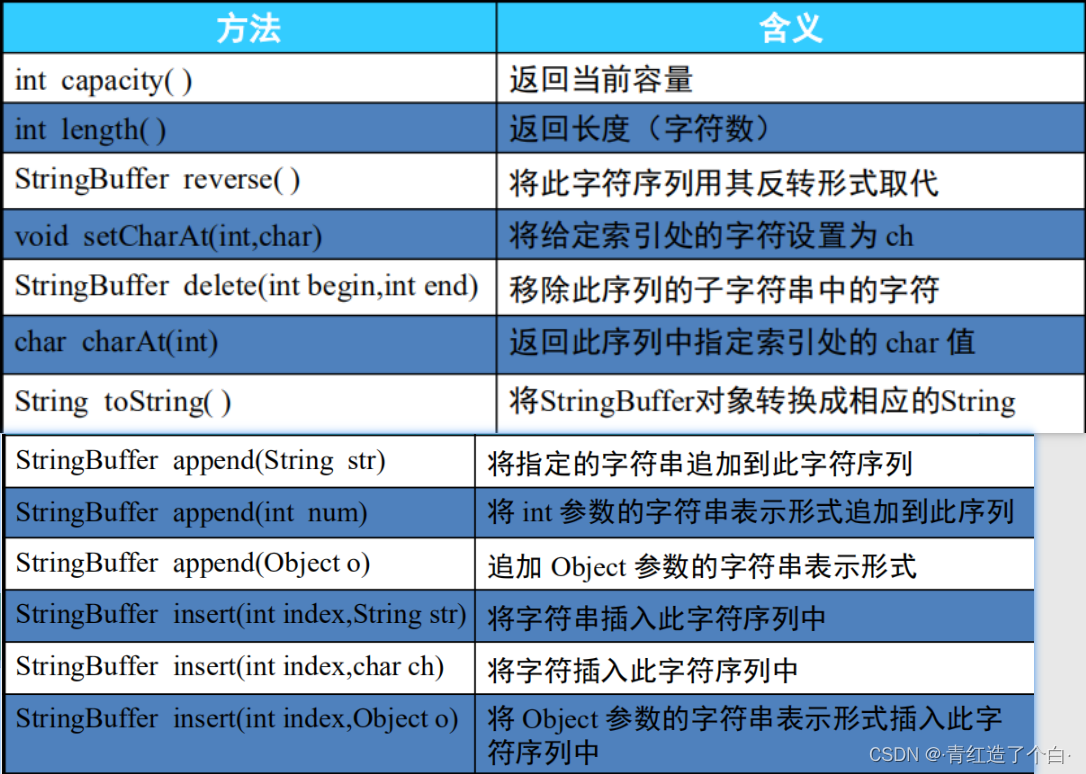
③例子
package com.lqh.pratice;
public class Test {
public static void main(String[] args) {
StringBuffer sb1 = new StringBuffer();//默认初始容量16
StringBuffer sb2 = new StringBuffer(20);//指定初始容量的字符串缓存区
StringBuffer sb3 = new StringBuffer("hello world");//指定字符串内容的字符串缓冲区
System.out.println(sb3.capacity());//27
System.out.println(sb3.length());//11
System.out.println(sb3.reverse());//dlrow olleh
sb3.setCharAt(2, 'l');
System.out.println(sb3.toString());//dllow olleh
sb3.append("123");
System.out.println(sb3);//dllow olleh123
sb3.insert(5, "hello world");
System.out.println(sb3);//dllowhello world olleh123
}
}
(3)String和StringBuffer之间的区别
- String类对象中的内容初始化不可以改变
- StringBuffer类对象中的内容可以改变
?(4)StringBuffer与StringBuilder的比较
- StringBuffer类是线程安全的;
- StringBuilder类是线程不安全的。
- StringBuffer在JDK1.0中就有,而StringBuilder是在JDK5.0后才出现的。
- StringBuilder的一些方法实现要比StringBuffer快些。
5.Math类
?Math类提供了大量用于数学运算的方法
(1)Math类中的常量
- public static final double PI
- public static final double E
(2) Math类中的常用方法
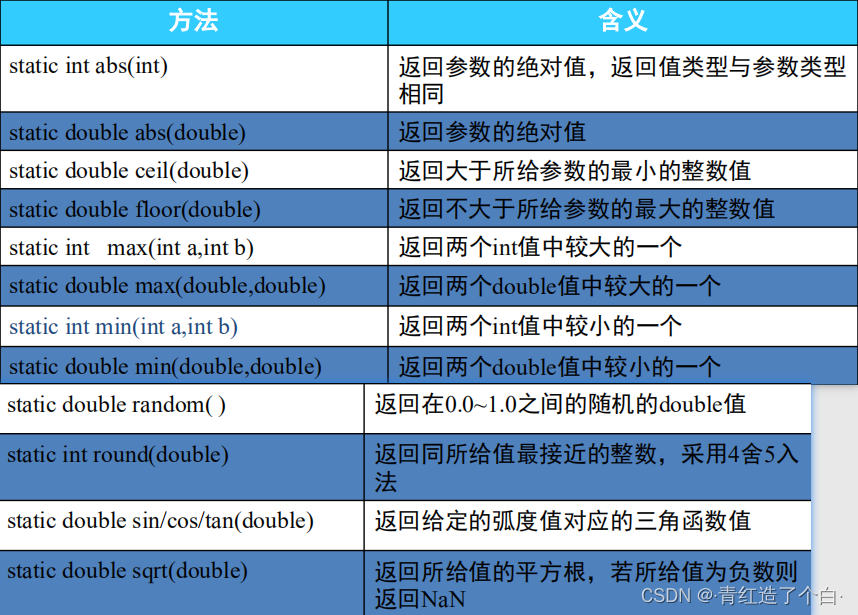
(3)例子
package test;
public class Test {
public static void main(String[] args) {
System.out.println(Math.abs(-5.5));//5.5
System.out.println(Math.ceil(45.6));//46.0
System.out.println(Math.floor(45.5));//45.0
System.out.println(Math.max(15.5, 25.5));//25.5
System.out.println(Math.min(15.5, 25.5));//15.5
System.out.println(Math.random());//0.8653846718829892
System.out.println(Math.round(45.5));//46
}
}
6.日期类
(1)Date类
常用方法:
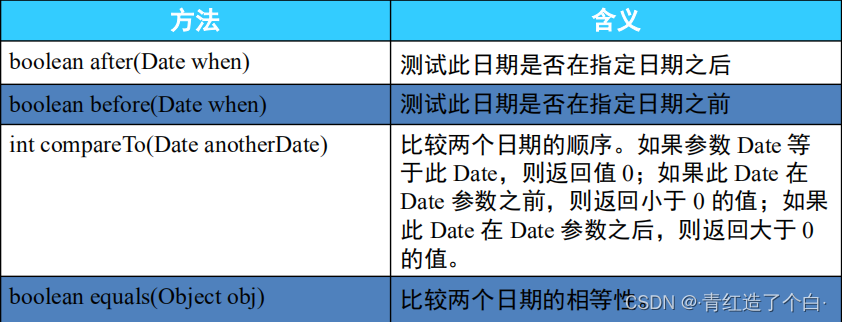
例子:
package test;
import java.util.Date;
public class Test {
public static void main(String[] args) {
Date date = new Date();
System.out.println(date);//Fri Jan 14 15:42:42 CST 2022
Date date1 = new Date(2021, 9, 4);
Date date2 = new Date(2021, 9, 3);
Date date3 = new Date(2021, 9, 4);
System.out.println(date1.after(date2));//true
System.out.println(date1.before(date2));//false
System.out.println(date1.compareTo(date2));//1
System.out.println(date1.equals(date3));//true
}
}
(2)Calendar类
常用属性
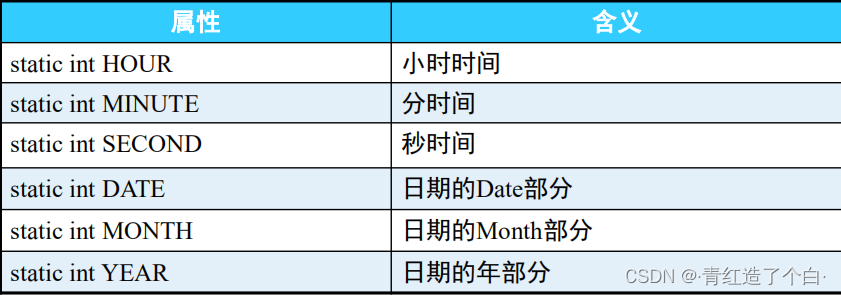
?例子:
package test;
import java.util.Calendar;
public class Test {
public static void main(String[] args) {
Calendar calendar = Calendar.getInstance();
System.out.println(calendar.get(Calendar.HOUR));//3
System.out.println(calendar.get(Calendar.MINUTE));//52
System.out.println(calendar.get(Calendar.SECOND));//10
System.out.println(calendar.get(Calendar.DATE));//14
System.out.println(calendar.get(Calendar.MONTH));//0
System.out.println(calendar.get(Calendar.YEAR));//2022
}
}
(3)SimpleDateFormat类
①获取SimpleDateFormat的实例
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
②将日期格式成指定的字符串
sdf.format(new Date());??
③将格式化的字符串转换成日期对象
sdf.parse(“2011-07-16”);?
注意:现在我们更应该多使用 Calendar 类实现日期和时间字段之间转换,使用 DateFormat 类来格式化和分析日期字符串;Date 中的相应方法已废弃
例子:
package test;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Test {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
//日期 -> 字符串
String string = sdf.format(new Date());
System.out.println(string);
//字符串 -> 日期
Date date = null;
try {
date = sdf.parse("1999-09-04 05:15:00");
} catch (ParseException e) {
e.printStackTrace();
}
System.out.println(date);//Sat Sep 04 05:15:00 CST 1999
}
}
|