1、Sentinel
1.1、Hystrix和Sentinel的比较
1. Hystrix说明
- 需要我们程序员自己手动的搭建监控平台。
- 没有一套
web 界面可以给我们进行更细粒度化的配置流量控制、速率控制、服务熔断、服务降级。
2. Sentinel说明
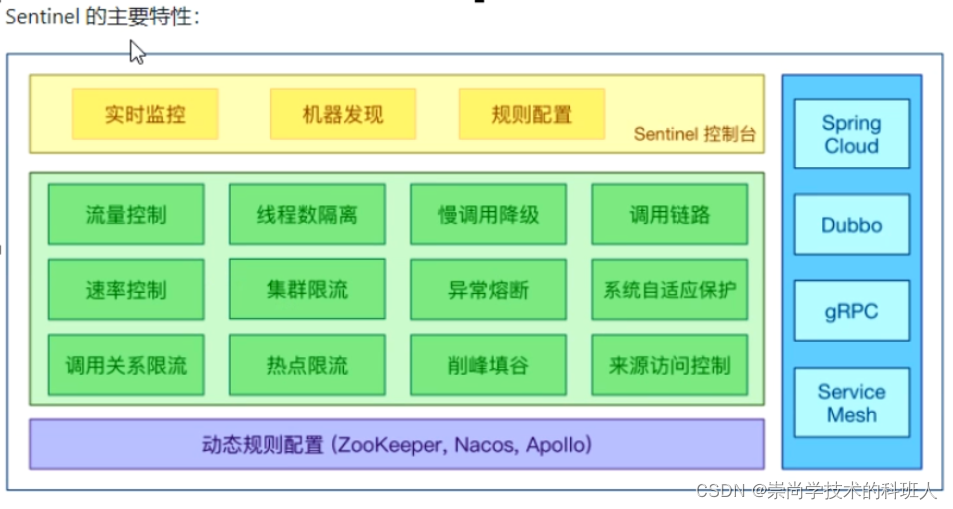
- 官方文档
- 下载地址
- 使用手册
- 它就是一个单独的组件,可以独立出来。
- 直接界面化的细粒度同一配置。
- 约定
> 配置 > 编码。 - 它可以解决服务雪崩、服务降级、服务熔断和服务限流。
1.2、安装并运行Sentinel控制台
- 核心库(
java 客户端)不依赖任何框架和库,能够运行于所有·java·运行时环境,同时对Dubbo / SpringCloud 等框架也有较好的支持。 - 控制台(
Dashboard )基于SpringBoot 开发,打包后可以直接运行,不需要额外的Tomcat 等应用容器。
java8 环境8080 端口不能被占用
java -jar sentinel-dashboard-1.8.3.jar
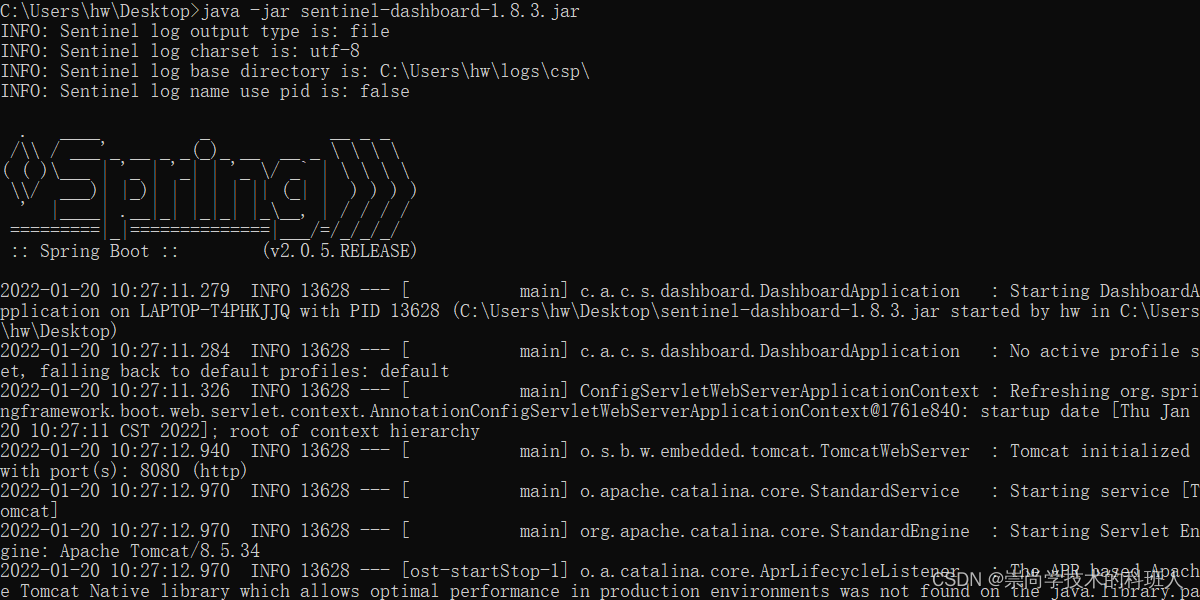
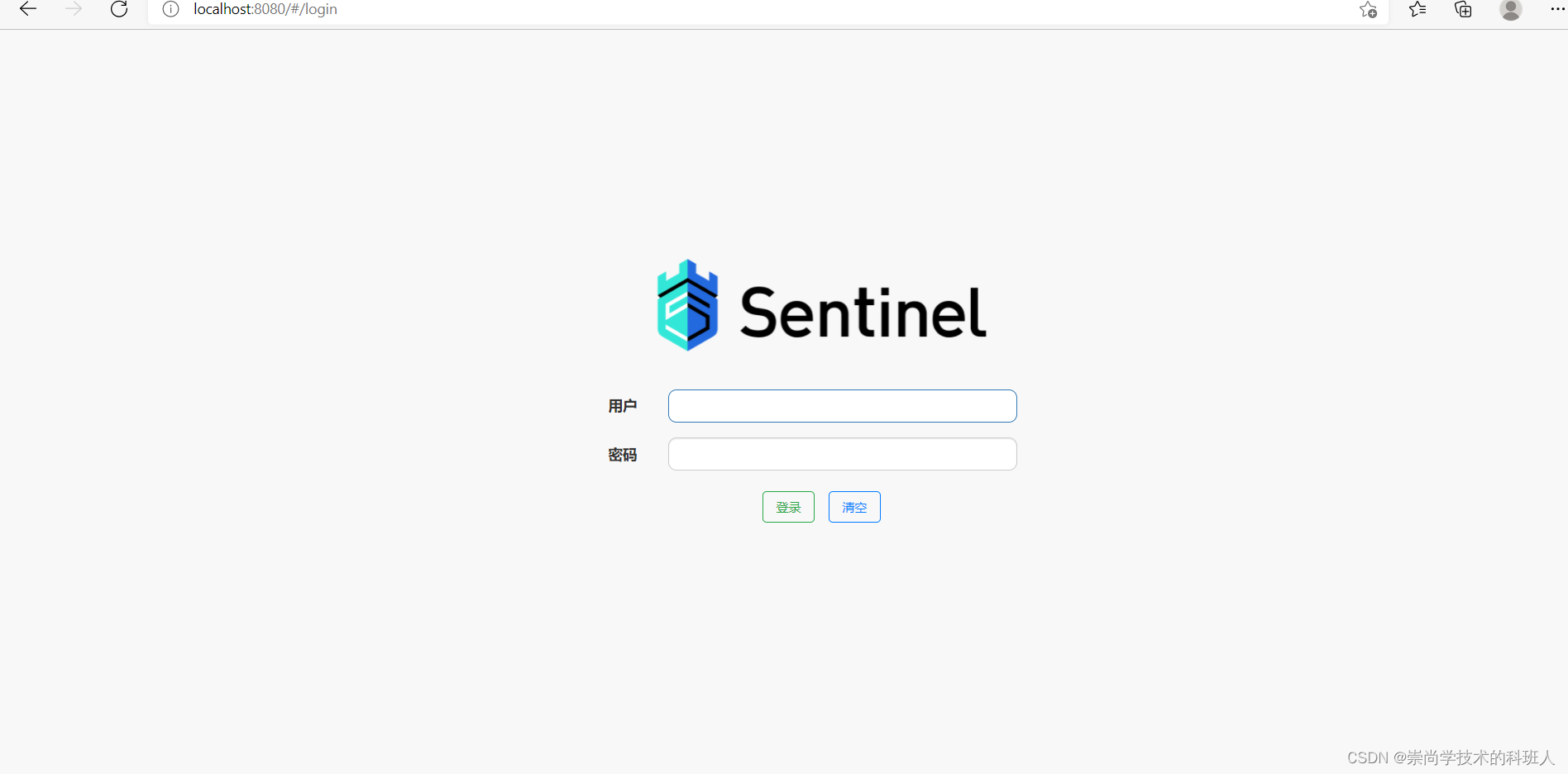
- 访问
localhost://8080 。 - 用户名和密码都是
sentinel 。
1.3、初始化监控工程
1.3.1、启动Nacos
startup.cmd
1.3.2、新建一个Module
1. 建Module
Module 的名称为cloudalibaba-sentinel-service8401 。
2. 改POM
<dependencies>
<dependency>
<groupId>com.xiao</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>${project.version}</version>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.csp</groupId>
<artifactId>sentinel-datasource-nacos</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>4.6.3</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
3. 改YML
server:
port: 8401
spring:
application:
name: cloudalibaba-sentinel-service
cloud:
nacos:
discovery:
server-addr: localhost:8848
sentinel:
transport:
dashboard: localhost:8080
port: 8719
management:
endpoints:
web:
exposure:
include: '*'
4. 主启动
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
@EnableDiscoveryClient
public class MainApp8401 {
public static void main(String[] args) {
SpringApplication.run(MainApp8401.class,args);
}
}
5. 业务类
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class FlowLimitController
{
@GetMapping("/testA")
public String testA() {
return "------testA";
}
@GetMapping("/testB")
public String testB() {
return "------testB";
}
}
6. 测试结果
(1)、访问http://localhost:8401/testA
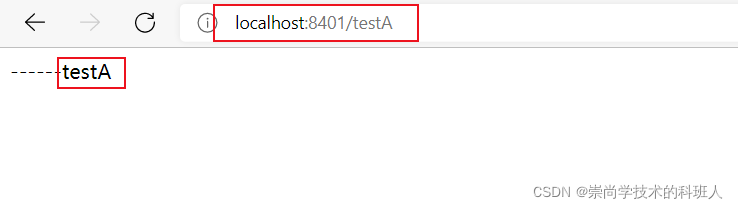
(2)、访问http://localhost:8401/testB

1.3.3、启动Sentinel进行监控
java -jar sentinel-dashboard-1.8.3.jar
- 由于
sentinel 是懒加载机制,所以是需要对所监控的对象进行访问之后才会出现监控的明细。不然不会出现对应的详细的明细。
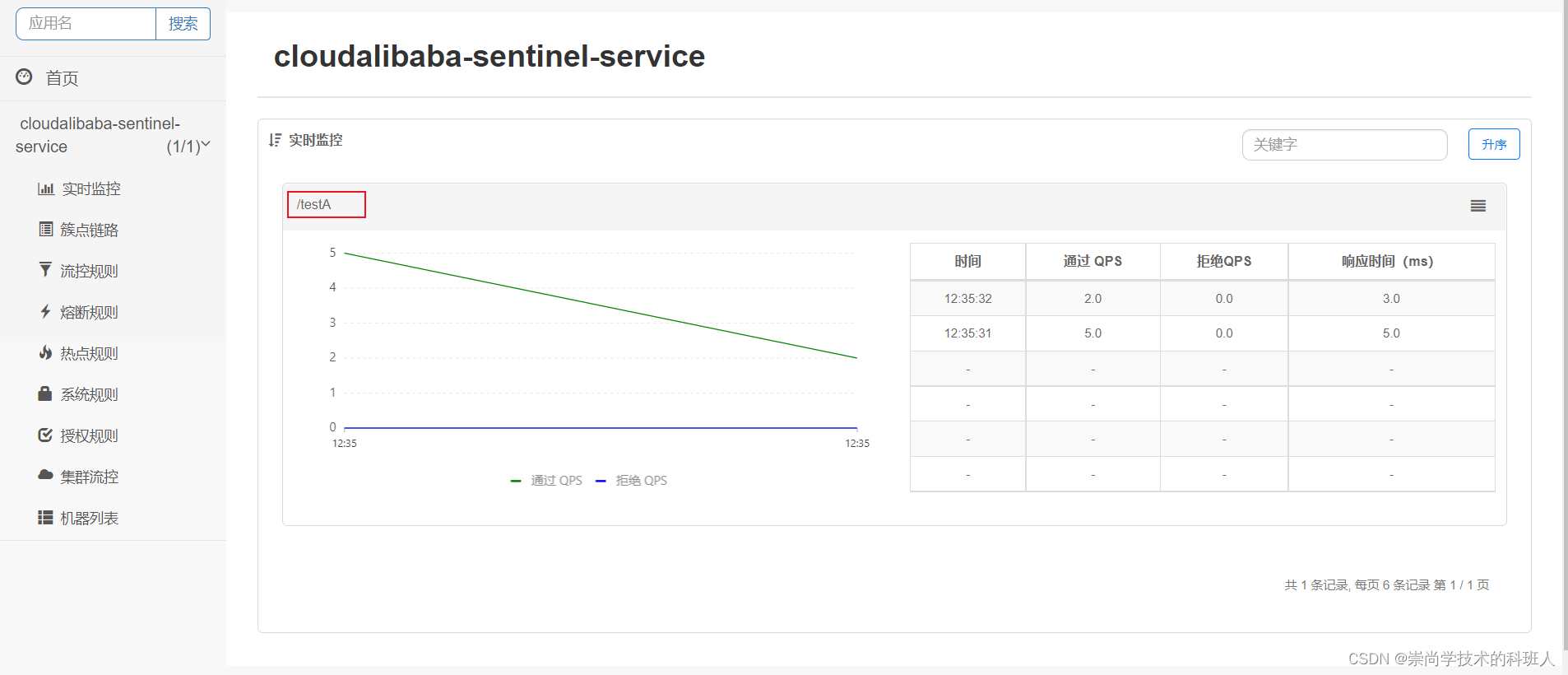
1.4、流控规则
1.4.1、基本介绍
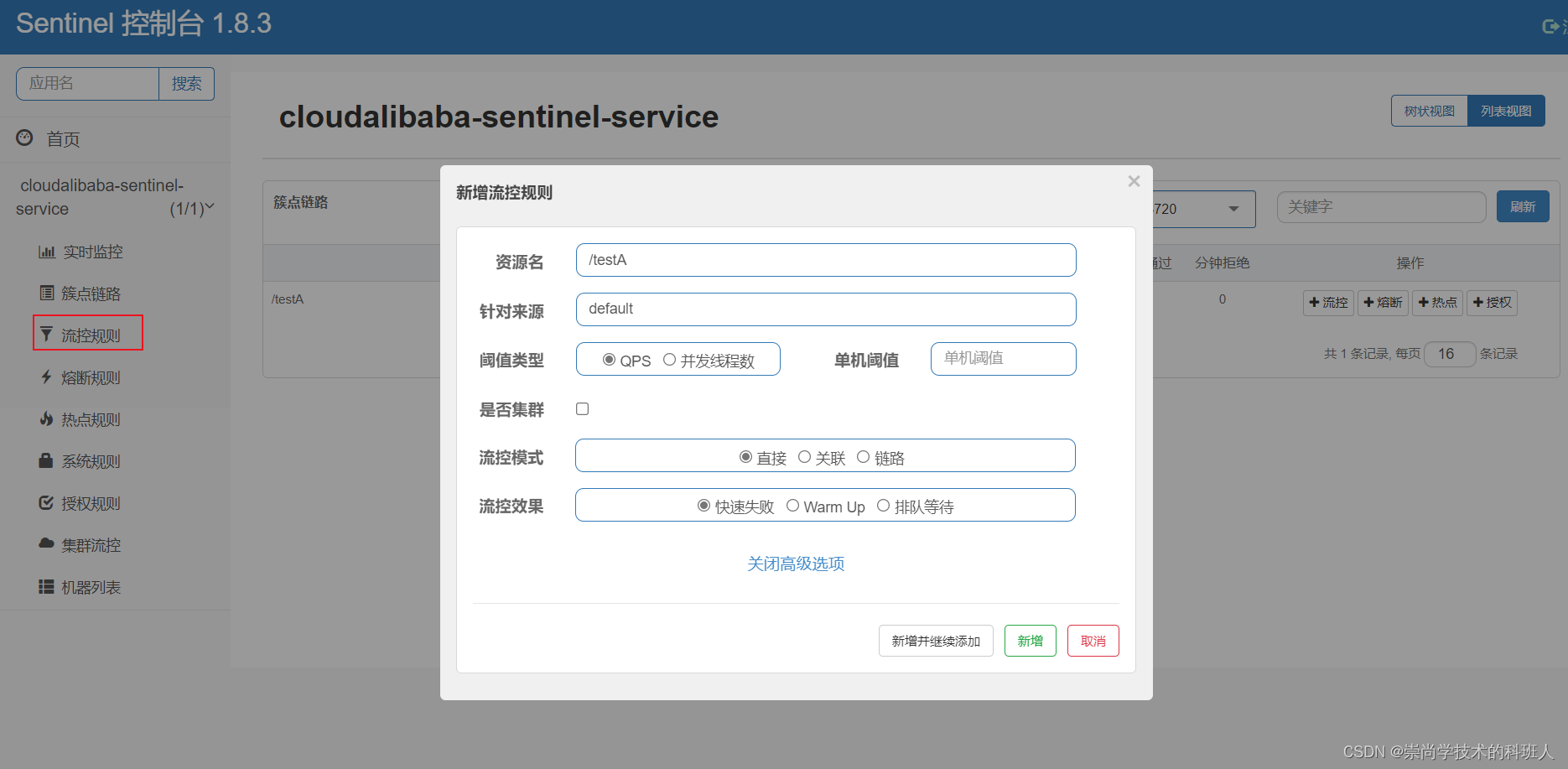
-
资源名:唯一名称,默认请求路径。 -
针对来源:Sentinel 可以针对调用者进行限流,默认是default (不区分来源)。 -
阈值类型/单机阈值
QPS (每秒钟的请求数量):当调用改API 的QPS 达到阈值的时候,进行限流。- 线程数:当调用改
API 的线程数达到阈值的时候,进行限流。 -
是否集群:不需要集群。 -
流控模式:
- 直接:
API 达到限流条件时,直接限流。 - 关联:当关联的资源达到阈值时,就限流自己。
- 链路:只记录指定链路上的流量(指定资源从入口资源进来的流量,如果达到阈值时,就进行限流)【
API 级别的针对来源】。 -
流控效果:
- 快速失败:直接失败,抛异常。
Warm up :根据codeFactor (冷家在因子,默认为3)的值,从阈值/codeFactor ,经过预热时长,才达到设置的QPS 阈值。- 排队等待:匀速排队,让请求以匀速的速度通过,阈值类型必须设置为
QPS ,否则无效。
1.4.2、流控模式
(1)、直接模式
1. QPS情况
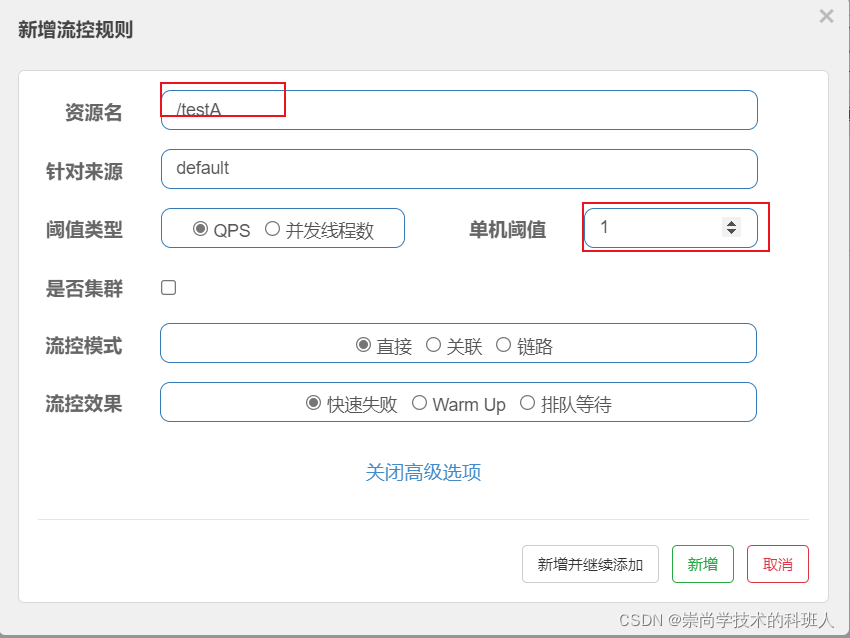
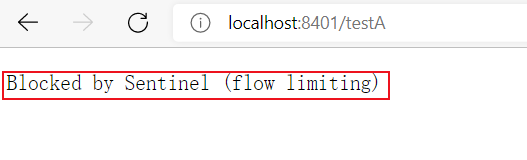
- 当我们多次点击发送这个请求的话,那么就会报默认错误。但是只是默认错误,目前我们还没有实现自定义错误,自定义错误需要我们之后设置。
2. 线程数情况
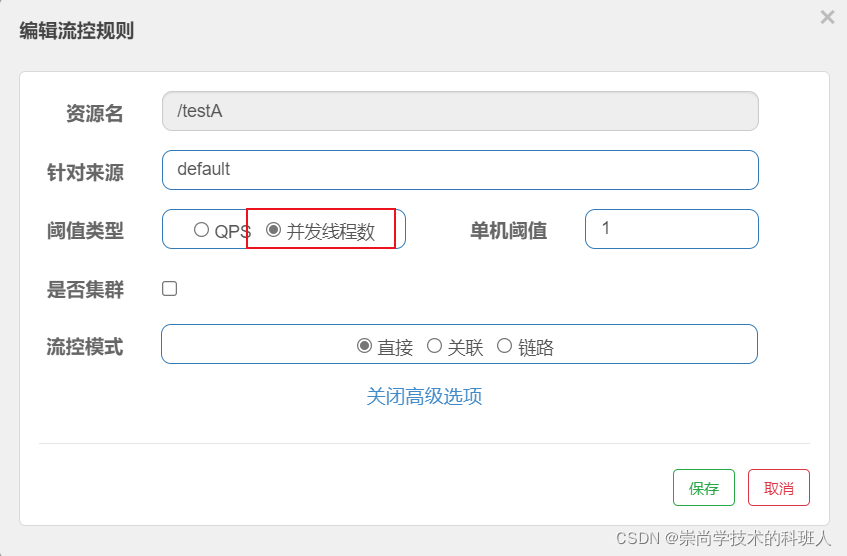
- 线程数:指的是在Sentinel内部处理对应请求的线程数量,所以它和我们所发送的请求的数量无关。
- 为了测试想要的效果,我们在处理请求的业务类上加上相应的代码让其睡眠一定的时间。
@GetMapping("/testA")
public String testA() {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "------testA";
}
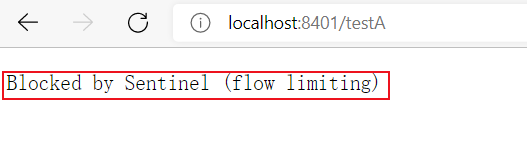
(2)、关联模式
- 当关联的资源达到阈值时,就限流自己。
- 在我们这个测试案例中,就是当与
A 关联的资源B 达到阈值后,就限流自己。
测试过程
(1)、进行Sentinel配置
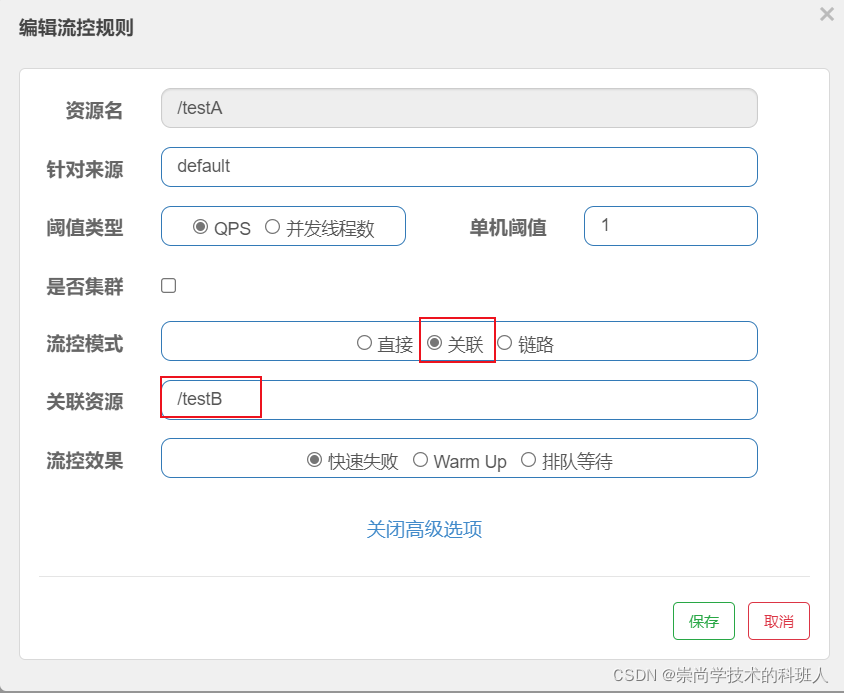
(2)、使用postman发送20个请求
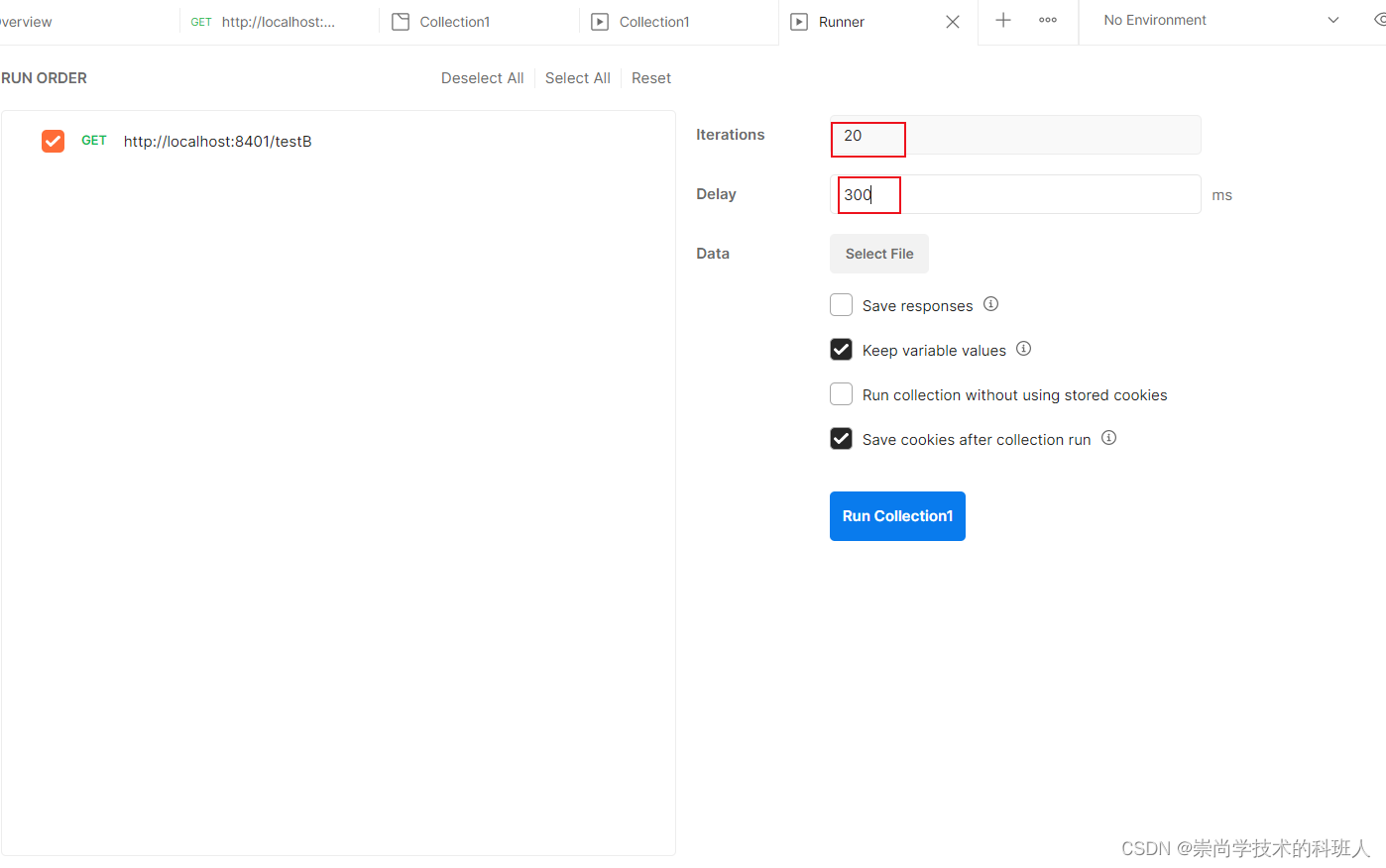
- 上述配置表示我们发送
20 个请求每隔300ms 到localhost:8401/testB 。
(3)、当我们再次访问localhost:8401/testA
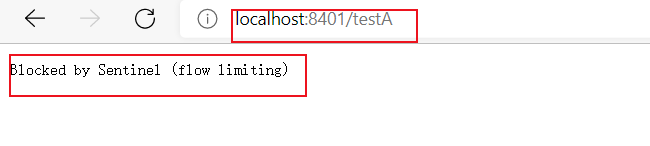
(3)、链路模式
1.4.3、流控效果
(1)、快速失败
- 直接失败,抛出异常。(
Blocked by Sentinel (flow limiting) )。
(2)、预热
- 公式:阈值除以
coldFactor (默认值为3 ),经过预热时长后才会达到阈值。默认coldFactor 为3 ,即请求QPS 从threshold/3 开始,经预热时长逐渐升至设定的QPS 阈值。
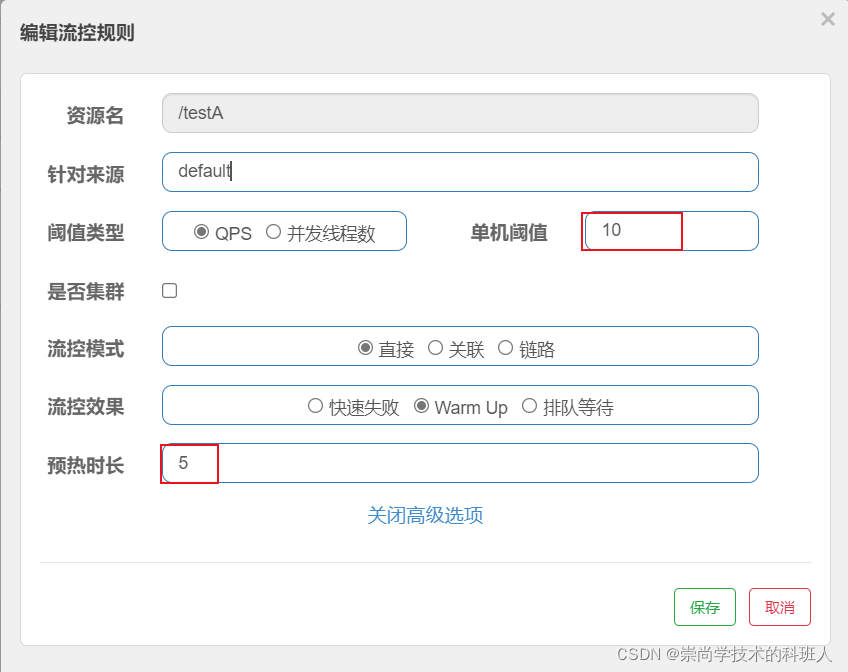
- 上述配置表示的是系统初始化的阈值为
10 / 3 约等于3 ,也就是阈值刚开始的时候为3 ;然后过了5 秒后阈值才慢慢升高恢复到10 。 应用场景 :秒杀系统在开启的瞬间,会有很多流量上来,很有可能把系统打死,预热方式就是把为了保护系统,可慢慢的把流量放进来,慢慢的把阈值增长到设置的阈值。
(3)、排队等待
- 匀速排队,阈值必须设置为
QPS 。 - 匀速排队方式会严格控制请求通过的间隔时间,也即是让请求以均匀的速度通过,对应的是漏桶算法。
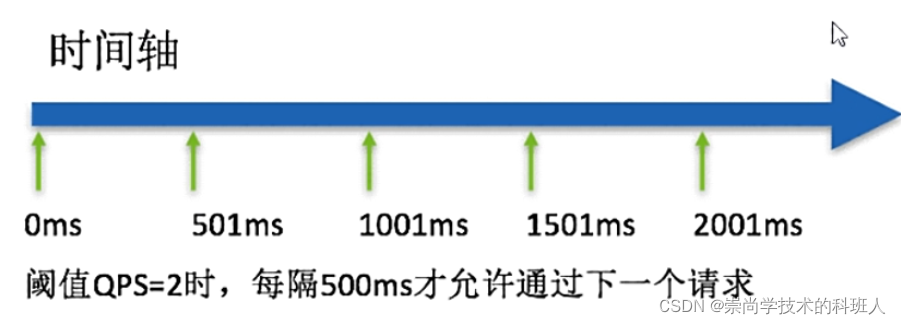
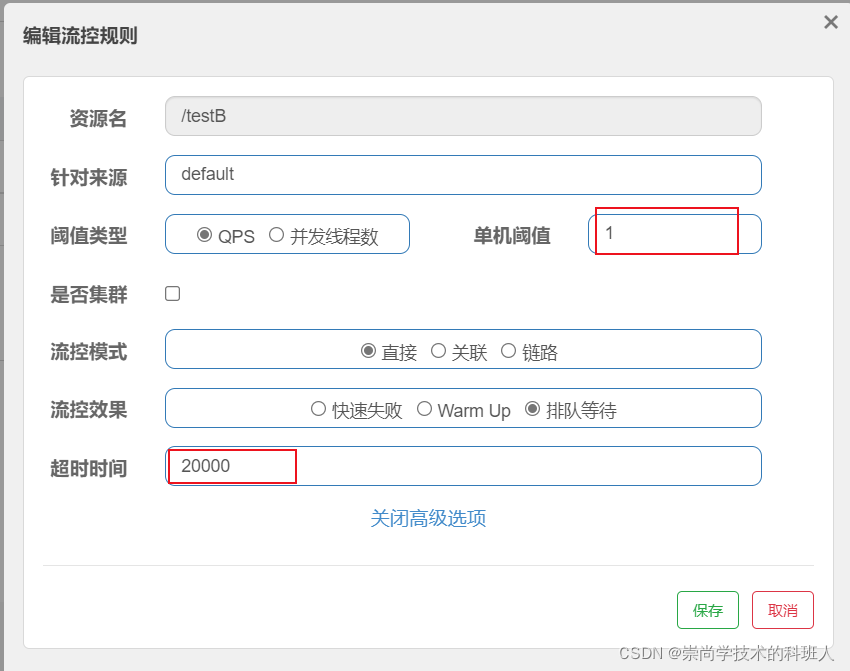
- 上图配置表示的是我们允许每秒钟
1 个请求匀速通过,超时时间为20 秒。
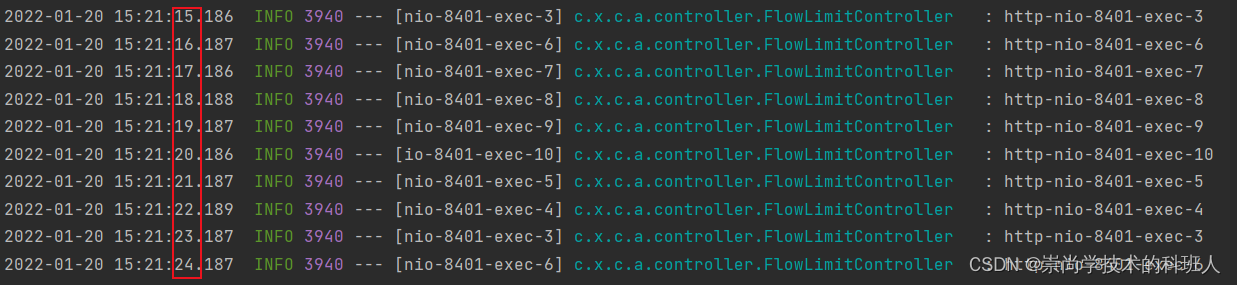
- 我们使用
postman 向对应的请求每隔500ms 总发送了10 个请求 ,可以看出都是一秒一秒的被处理了。
1.5、降级规则
1.5.1、基本介绍
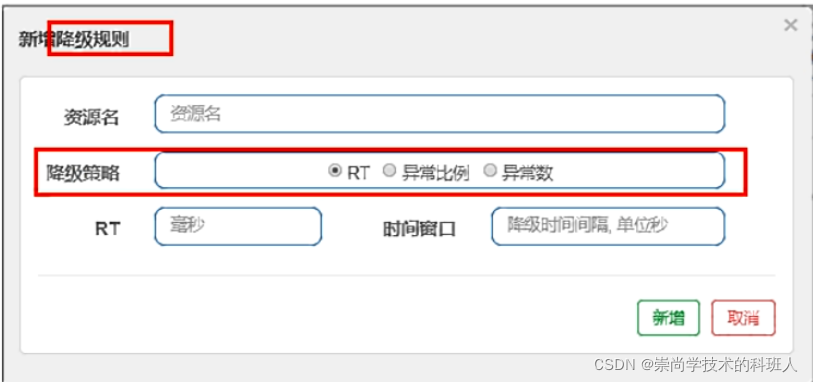
RT (平均响应时间,秒级):平均响应时间超出阈值且在时间窗口内通过的请求 >= 5 ,两个条件同时满足后触发降级,窗口期过后关闭断路器。RT 最大为4900 (更大的需要通过-Dcsp.sentinel.statistic.max.rt=XXXX 才能生效)。- 异常比例(秒级):
QPS >= 5 且异常比例(秒级统计)超过阈值时,触发降级;时间窗口结束后,关闭降级。 - 异常数(分钟级):异常数(
1 分钟统计)超过阈值时,触发降级;时间窗口结束后,关闭降级。
Sentinel 熔断降级会在调用链路中某个资源出现不稳定状态时(例如调用超时或异常比例升高),对这个资源的调用进行限制,让请求快速失败,避免影响到其他的资源而导致级联错误。当资源被降级后,在接下来的降级时间窗口之内,对该资源的调用都自动熔断(默认行为是DegradeException )。Sentinel 的断路器是没有半开状态的:半开的状态系统自动去检测是否请求有异常,没有异常就关闭断路器恢复使用,有异常则继续打开断路器不可用。
1.5.2、降级策略实战
(1)、RT
1. 处理流程 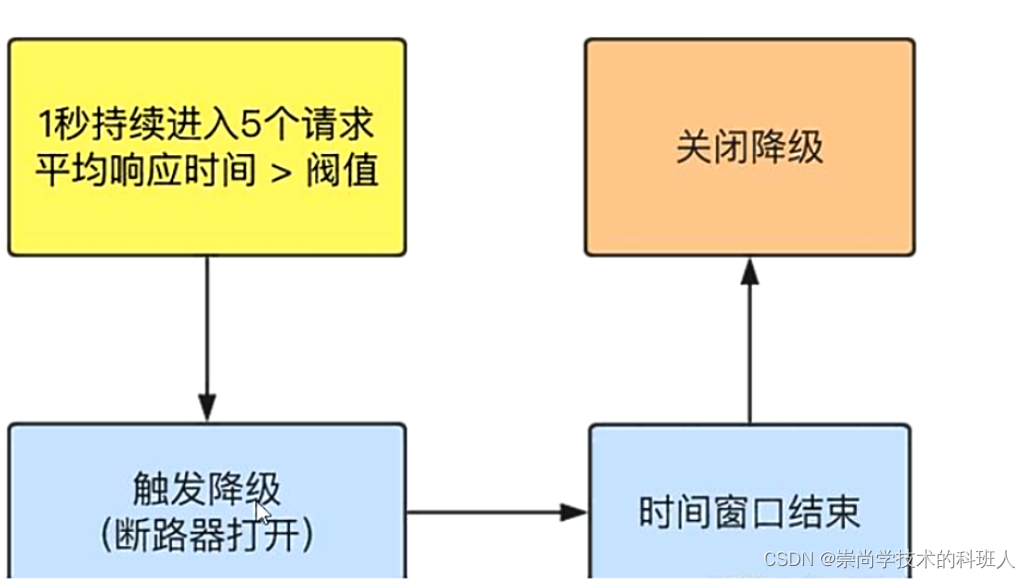
2. 实战案例
@GetMapping("/testD")
public String testD()
{
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
log.info("testD 测试RT");
return "------testD";
}
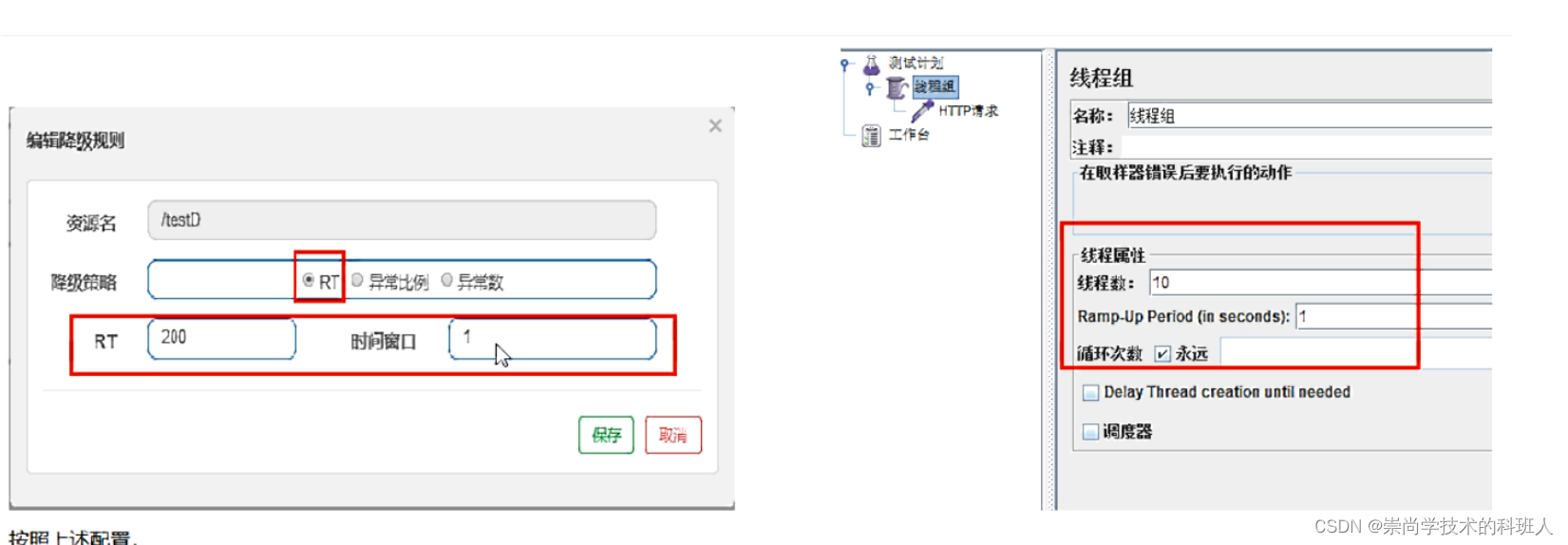
- 按照上述配置,永远一秒钟打进来
10 个线程(大于5 个了)调用testD ,我们希望200ms 处理完本次任务,如果超过200ms 还没处理完,在未来1s 的时间窗口内,断路器打开(保险丝跳闸)微服务不可用,保险丝跳闸断电了。后续我们停止线程的发送,没有这么大的访问量了,断路器关闭(保险丝回复),微服务回复OK 。
(2)、异常比例
1. 处理流程
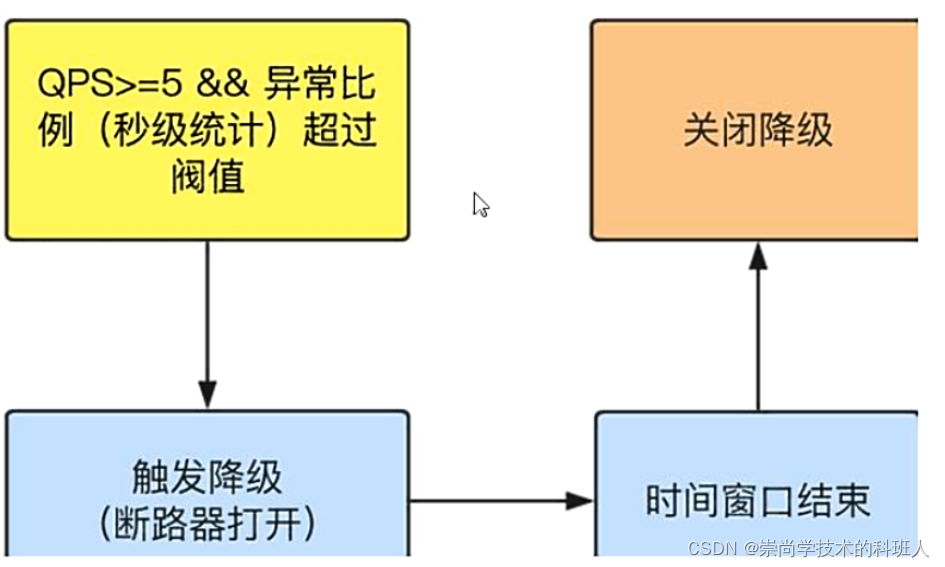
2. 实战案例
@GetMapping("/testD")
public String testD()
{
log.info("testD 测试RT");
int age = 10/0;
return "------testD";
}
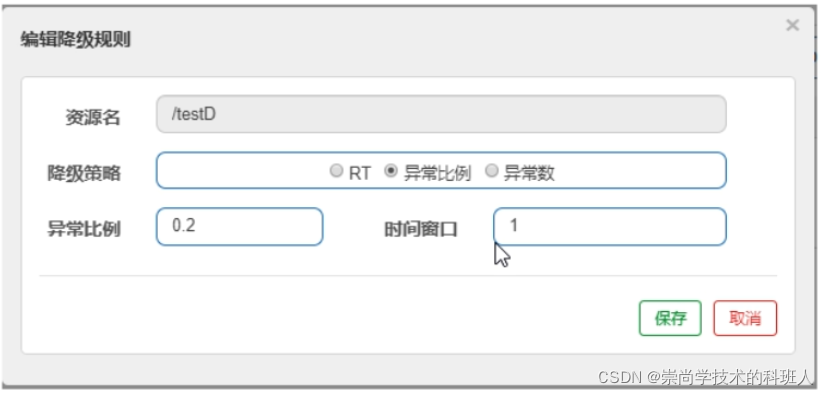
- 按照上述的配置出现服务降级的条件是
QPS >= 5 并且异常比例大于20% 的时候就会进行服务降级。 - 而如果我们虽然异常比例可能达到了
20% ,但是如果我们的QPS 如果小于5 的话,那么就会直接出现Error Page 页面(也就是直接抛出了异常而并未进行服务降级)。
(3)、异常数
1. 处理流程
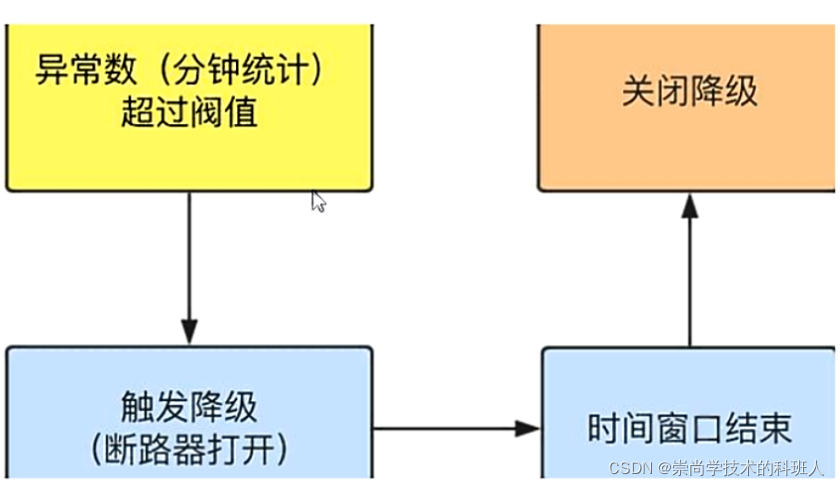
2. 实战案例
@GetMapping("/testE")
public String testE()
{
log.info("testE 测试异常数");
int age = 10/0;
return "------testE 测试异常数";
}
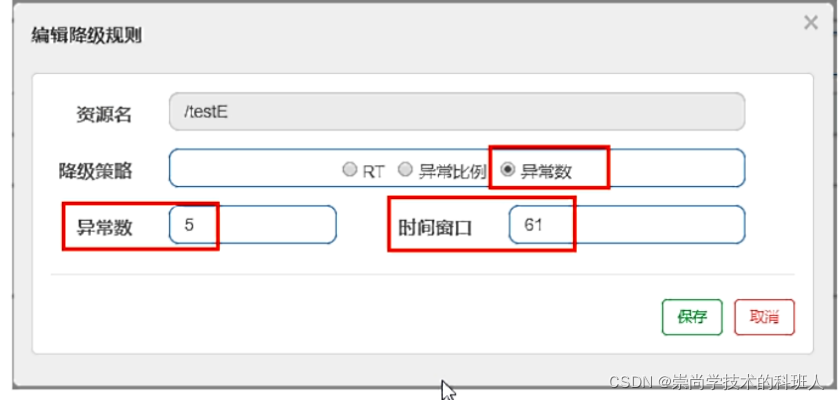
- 第一次访问绝对报错,因为除数不能为零,我们看到
Error Page 窗口,但是达到5 次报错后,进入熔断后降级。 - 注意:时间窗口一定要大于等于
60s 。
1.6、热点规则
1.6.1、基本介绍
- 就是我们通过资源名对该资源名上的参数通过参数索引进行相应的
QPS 限制。
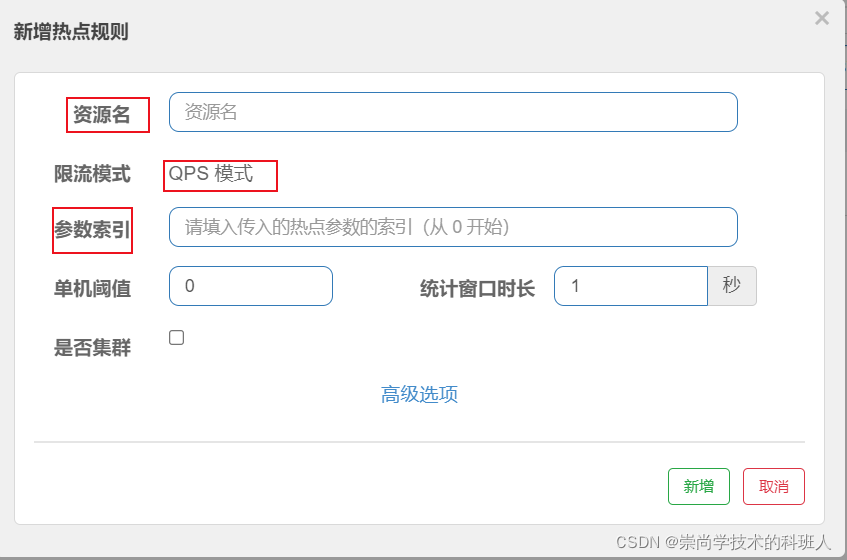
官网地址
@SentinelResource
- 分为系统默认和客户自定义两种。
- 之前的
case ,限流出问题后,都是用sentinel 系统默认的提示Blocked by Sentinel (flow limiting) 。 - 我们可以使用
@SentinelResource 进行自定义兜底方法。
1.6.2、案例演示
1. 编写的业务类代码
@GetMapping("/testHotKey")
@SentinelResource(value = "testHotKey",blockHandler = "deal_testHotKey")
public String testHotKey(@RequestParam(value = "p1",required = false) String p1,
@RequestParam(value = "p2",required = false) String p2) {
return "------testHotKey";
}
public String deal_testHotKey (String p1, String p2, BlockException exception){
return "------deal_testHotKey,o(╥﹏╥)o";
}
2. 进行配置
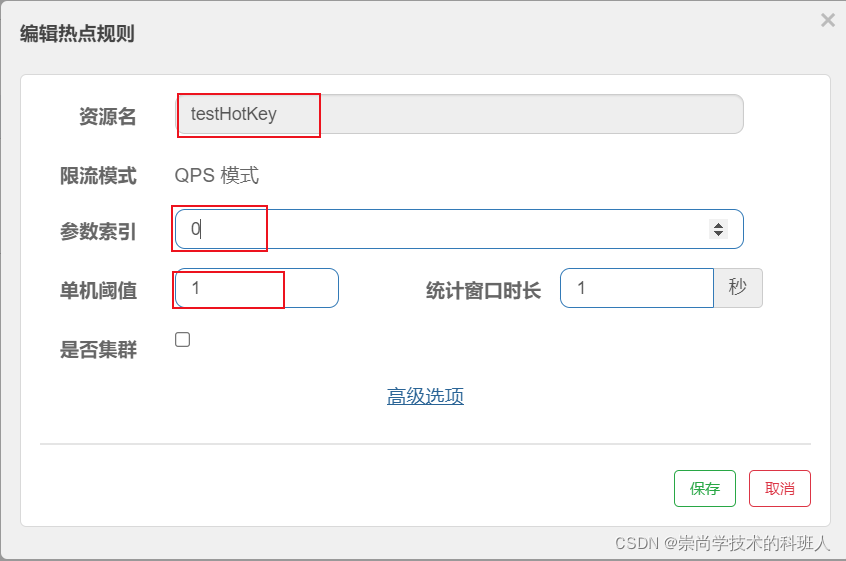
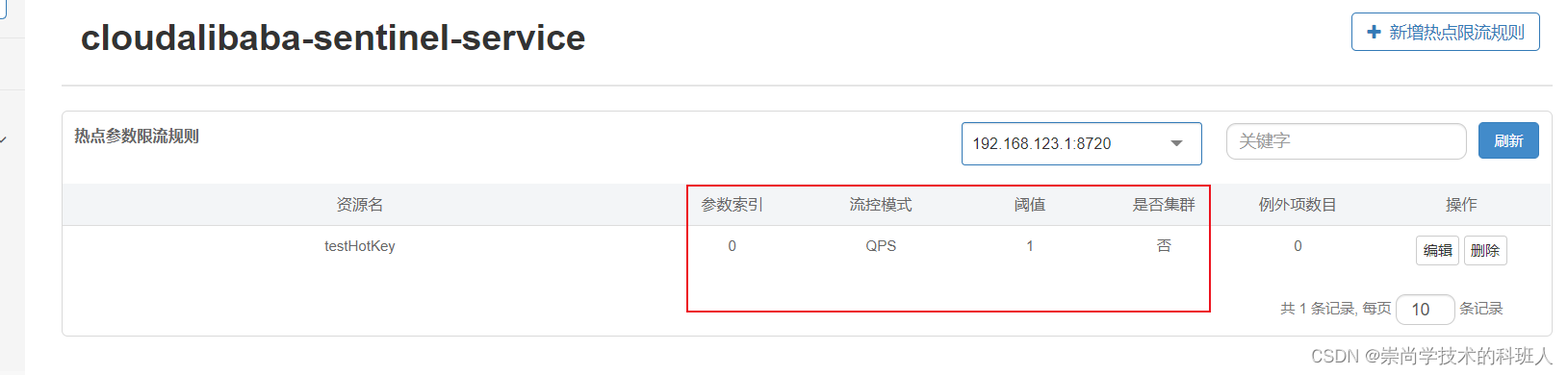
- 这里的配置表示的是如果参数索引为
0 的参数的QPS 数值超过我们预设定的1 的话,那么就会抛出相应的异常,如果我们没有自己设定相应的兜底方法,那么它就会直接跳转到Error Page 页面;否则就会执行我们自己设定的兜底方法。
3. 测试结果
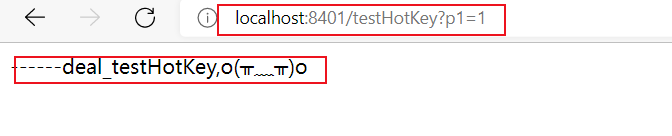
- 这里我们多次点击违反了相关配置规则,所以执行了兜底方法。
1.6.3、参数例外项
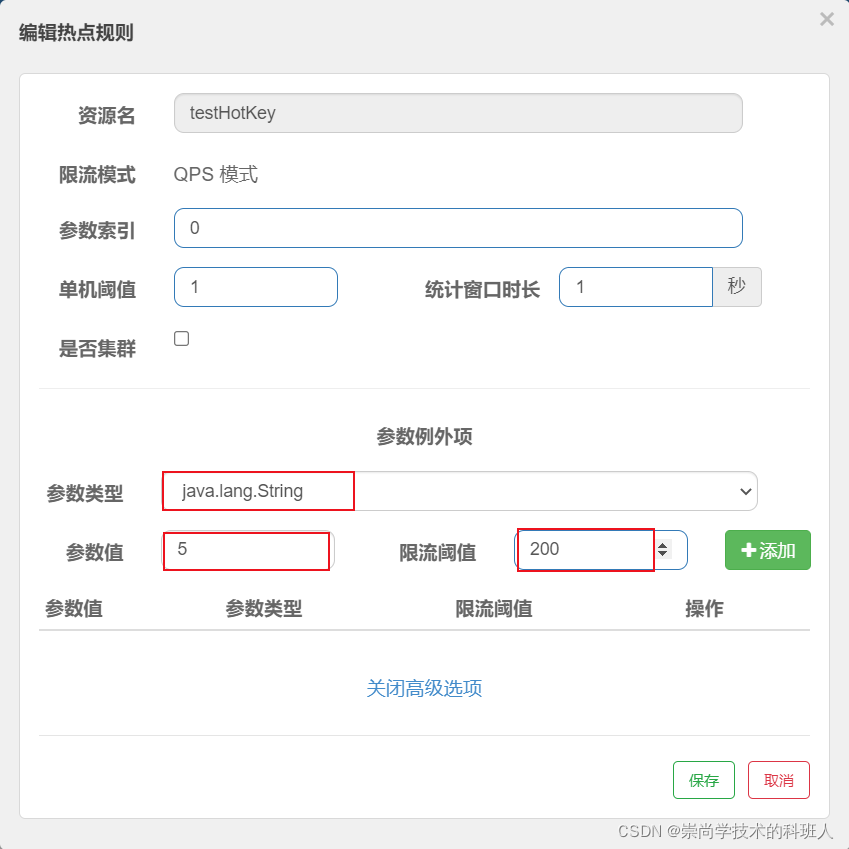
- 上述配置表示
testHotKey 资源上的参数索引为0 的QPS 数值如果超过了1 的话,那么就会执行兜底方法;而加了参数例外项之后,那么如果QPS 超过了或等于5 的话,那么它的限流阈值就会变为200 。那么此时的就还是正常的执行。如果QPS 的值不等于5 的时候,那么它的限流阈值就还是1 。 - 热点参数的注意点:参数必须是基本类型和
String 。
1.7、系统规则
1. 基本介绍
-
系统保护规则是从应用级别的入口流量进行控制,从单台机器的load 、CPU 使用率、平均RT 、入口QPS 和并发线程数等几个维度监控应用指标,让系统尽可能跑在最大吞吐量的同时保证系统整体的稳定性。 -
系统保护规则是应用整体维度的,而不是资源维度的,并且仅对入口流量生效。入口流量值的是进入应用的流量,比如Web 服务或Dubbo 服务端接受的请求,都属于入口流量。 -
系统规则就是对整个系统的应用进行相应的规则配置,而前面的规则只是更加细粒度化的配置,它是针对某个方法进行相关的规则配置。所以说,系统规则是一个总的配置。
2. 案例演示
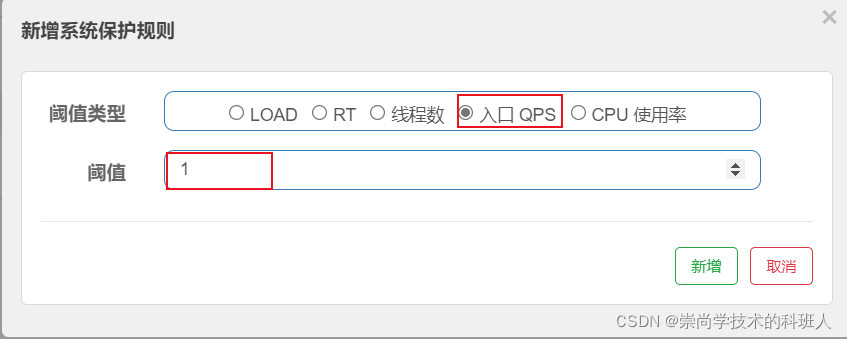
- 就是总的系统的入口
QPS 的阈值是1 ,如果超过了这个值,那么就会进行相关的服务降级,也就是抛出Blocked by Sentinel (flow limiting) 。
(1)、多次对localhost:8401/testA访问
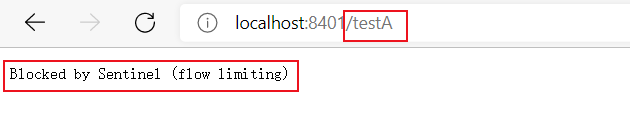
(2)、多次对localhost:8401/testB访问
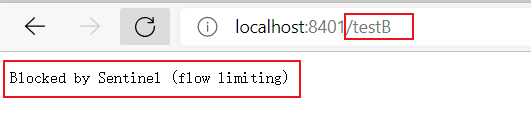
1.8、@SentinelResource
1.8.1、按资源名称限流+后续处理
1. 对cloudalibaba-sentinel-service8401进行修改
(1)、修改POM
<dependency>
<groupId>com.xiao</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>${project.version}</version>
</dependency>
(2)、添加业务类RateLimitController
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.alibaba.csp.sentinel.slots.block.BlockException;
import com.xiao.cloud.entities.CommonResult;
import com.xiao.cloud.entities.Payment;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class RateLimitController {
@GetMapping("/byResource")
@SentinelResource(value = "byResource", blockHandler = "handleException")
public CommonResult byResource() {
return new CommonResult(200, "按资源名称限流测试OK", new Payment(2020L, "serial001"));
}
public CommonResult handleException(BlockException exception) {
return new CommonResult(444, exception.getClass().getCanonicalName() + "\t 服务不可用");
}
}
2. 以资源名进行相关配置
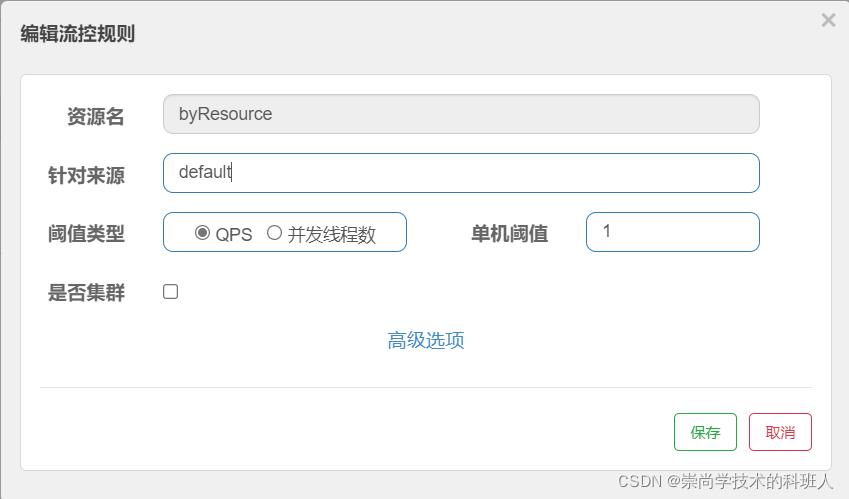
- 当
QPS 数值超过我们设置的阈值的时候,那么就会执行我们的兜底方法。
3. 测试结果

1.8.2、按照Url地址限流+后续处理
1. 在业务类上添加以下代码
@GetMapping("/rateLimit/byUrl")
@SentinelResource(value = "byUrl")
public CommonResult byUrl()
{
return new CommonResult(200,"按url限流测试OK",new Payment(2020L,"serial002"));
}
2. 以URL进行相关配置
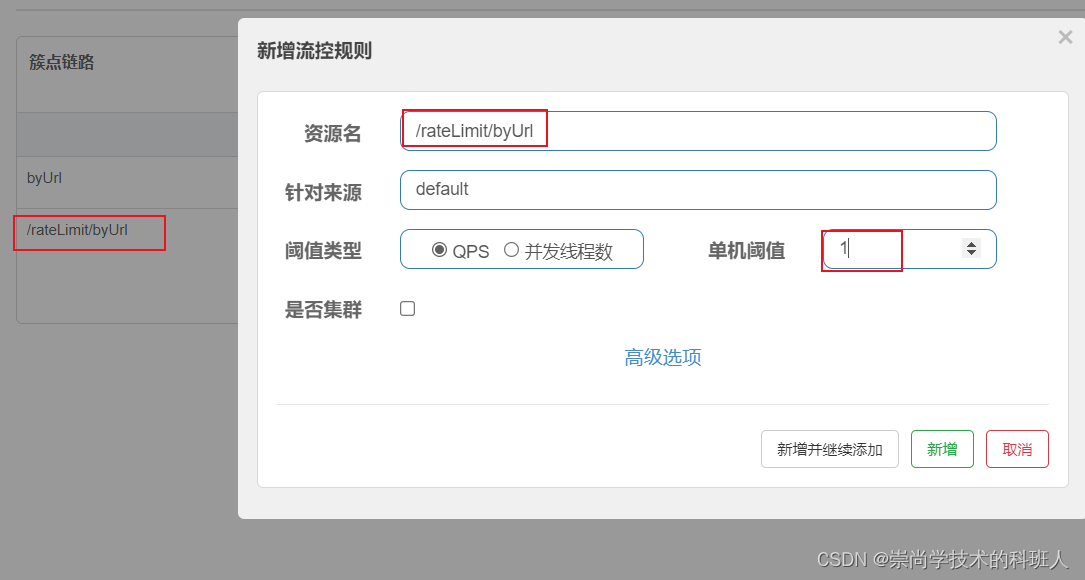
3. 测试结果
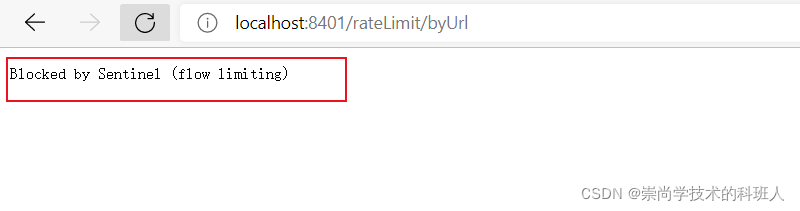
- 由我们的业务类代码也可以看出来,我们并未设置相应的兜底方法,所以它违反了系统默认的相关配置的时候执行的是系统默认自带的兜底方法。
1.8.3、面临的问题
- 系统默认的,没有体现我们自己的业务要求。
- 依照现有条件,我们自定义的处理方式又和业务代码耦合在一起,不直观。
- 每个业务方法都添加一个兜底方法,那代码膨胀加剧。
- 全局统一的处理方式没有体现。
1.8.4、客户自定义限流处理逻辑
1. 创建customerBlockHandler类用于自定义限流处理逻辑
import com.alibaba.csp.sentinel.slots.block.BlockException;
import com.xiao.cloud.entities.CommonResult;
public class CustomerBlockHandler {
public static CommonResult handleException2(BlockException exception) {
return new CommonResult(444, "自定义的全局限流处理信息..." + "\t" + " 服务不可用");
}
}
2. 在业务类上添加以下代码
@GetMapping("/rateLimit/customerBlockHandler")
@SentinelResource(value = "customerBlockHandler", blockHandlerClass = CustomerBlockHandler.class,
blockHandler = "handleException2")
public CommonResult customerBlockHandler()
{
return new CommonResult(200,"按客戶自定义",new Payment(2020L,"serial003"));
}
3. 进行相关配置
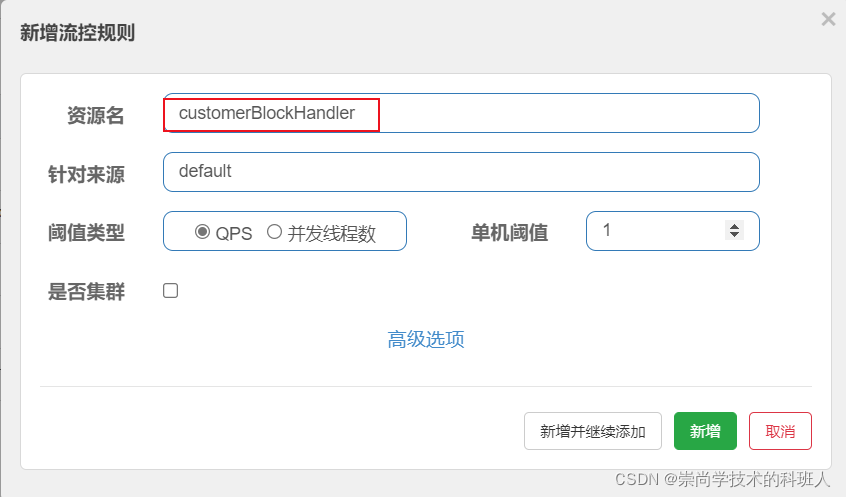
4. 测试结果

1.9、服务熔断功能
1.9.1、Ribbon作为服务调用工具
(1)、新建服务提供者
1. 建Module
Module 的名称为cloudalibaba-provider-payment9003 。
2. 改POM
<dependencies>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>com.xiao</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
3. 改YML
server:
port: 9003
spring:
application:
name: nacos-payment-provider
cloud:
nacos:
discovery:
server-addr: localhost:8848
management:
endpoints:
web:
exposure:
include: '*'
4. 主启动
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
@EnableDiscoveryClient
public class PaymentMain9003
{
public static void main(String[] args) {
SpringApplication.run(PaymentMain9003.class, args);
}
}
5. 业务类
import com.xiao.cloud.entities.CommonResult;
import com.xiao.cloud.entities.Payment;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.util.HashMap;
@RestController
public class PaymentController
{
@Value("${server.port}")
private String serverPort;
public static HashMap<Long, Payment> hashMap = new HashMap<>();
static{
hashMap.put(1L,new Payment(1L,"28a8c1e3bc2742d8848569891fb42181"));
hashMap.put(2L,new Payment(2L,"bba8c1e3bc2742d8848569891ac32182"));
hashMap.put(3L,new Payment(3L,"6ua8c1e3bc2742d8848569891xt92183"));
}
@GetMapping(value = "/paymentSQL/{id}")
public CommonResult<Payment> paymentSQL(@PathVariable("id") Long id){
Payment payment = hashMap.get(id);
CommonResult<Payment> result = new CommonResult(200,"from mysql,serverPort: "+serverPort,payment);
return result;
}
}
6. 再新建一个Module名为cloudalibaba-provider-payment9004
- 除了名称和端口号需要改为
9004 ,其它都和cloudalibaba-provider-payment9003 相同。
(2)、新建服务消费者
1. 建Module
Module 的名称为cloudalibaba-consumer-nacos-order84 。
2. 改POM
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
<dependency>
<groupId>com.xiao</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
3. 改YML
server:
port: 84
spring:
application:
name: nacos-order-consumer
cloud:
nacos:
discovery:
server-addr: localhost:8848
sentinel:
transport:
dashboard: localhost:8080
port: 8719
service-url:
nacos-user-service: http://nacos-payment-provider
4. 主启动
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.openfeign.EnableFeignClients;
@EnableDiscoveryClient
@SpringBootApplication
@EnableFeignClients
public class OrderNacosMain84
{
public static void main(String[] args) {
SpringApplication.run(OrderNacosMain84.class, args);
}
}
5. 业务类
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.alibaba.csp.sentinel.slots.block.BlockException;
import com.xiao.cloud.entities.CommonResult;
import com.xiao.cloud.entities.Payment;
import lombok.extern.slf4j.Slf4j;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
import javax.annotation.Resource;
@RestController
@Slf4j
public class CircleBreakerController {
public static final String SERVICE_URL = "http://nacos-payment-provider";
@Resource
private RestTemplate restTemplate;
@RequestMapping("/consumer/fallback/{id}")
@SentinelResource(value = "fallback",fallback = "handlerFallback",blockHandler = "blockHandler",
exceptionsToIgnore = {IllegalArgumentException.class})
public CommonResult<Payment> fallback(@PathVariable Long id) {
CommonResult<Payment> result = restTemplate.getForObject(SERVICE_URL + "/paymentSQL/"+id, CommonResult.class,id);
if (id == 4) {
throw new IllegalArgumentException ("IllegalArgumentException,非法参数异常....");
}else if (result.getData() == null) {
throw new NullPointerException ("NullPointerException,该ID没有对应记录,空指针异常");
}
return result;
}
public CommonResult handlerFallback(@PathVariable Long id,Throwable e) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(444,"兜底异常handlerFallback,exception内容 "+e.getMessage(),payment);
}
public CommonResult blockHandler(@PathVariable Long id,BlockException blockException) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(445,"blockHandler-sentinel限流,无此流水: blockException "+blockException.getMessage(),payment);
}
}
6. 配置类
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.client.RestTemplate;
@Configuration
public class ApplicationContextConfig
{
@Bean
@LoadBalanced
public RestTemplate getRestTemplate()
{
return new RestTemplate();
}
}
(3)、测试结果
1. 第一种情况
@SentinelResource(value = "fallback",fallback = "handlerFallback")

- 由于触及异常直接返回相应的
fallback 中设置的降级方法。
2. 第二种情况
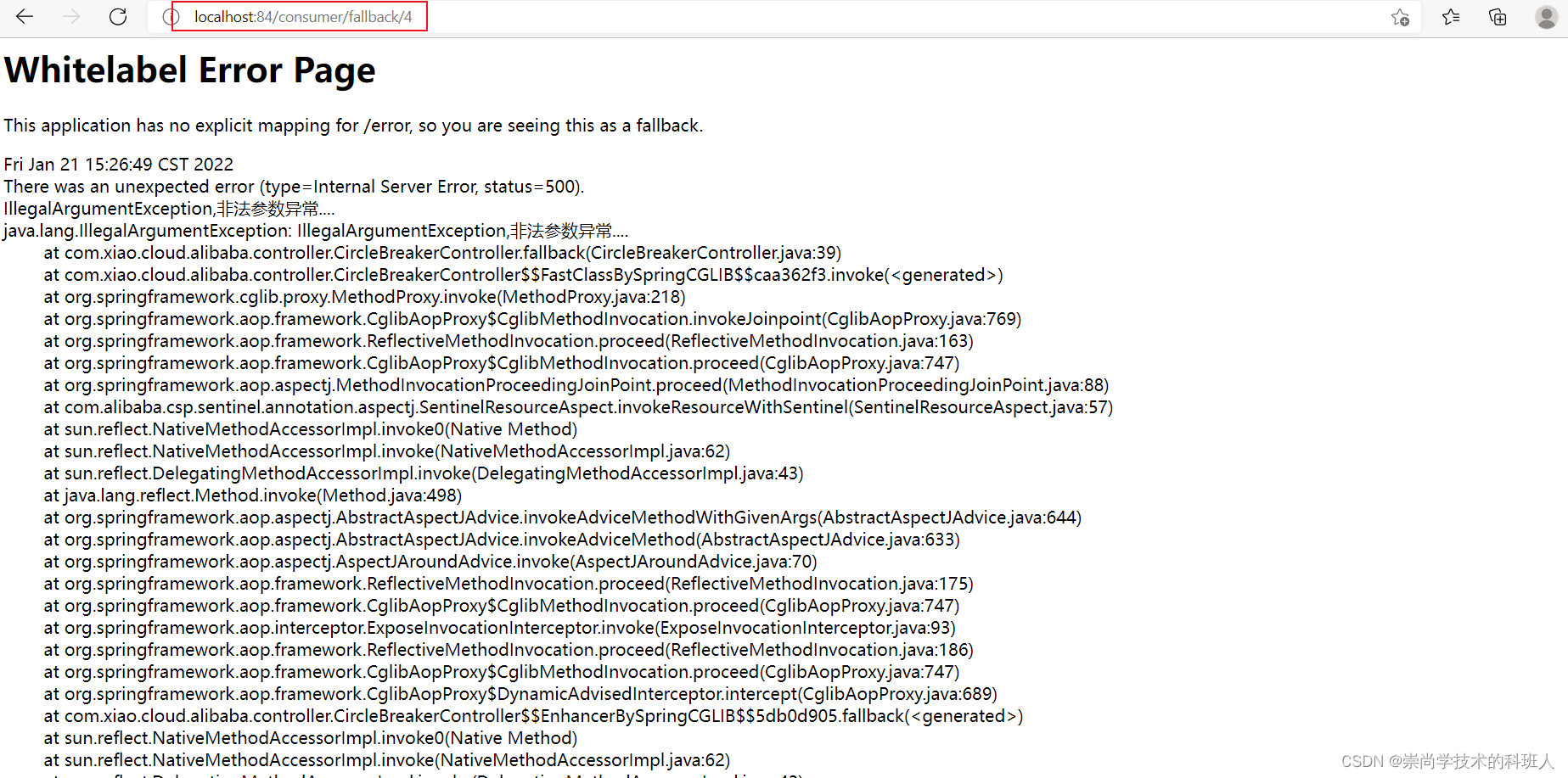
我们多次点击的时候违反了sentinel的配置规则

3. 第三种情况
@SentinelResource(value = "fallback",fallback = "handlerFallback",blockHandler = "blockHandler", exceptionsToIgnore = {IllegalArgumentException.class})
当我们携带的参数是4时
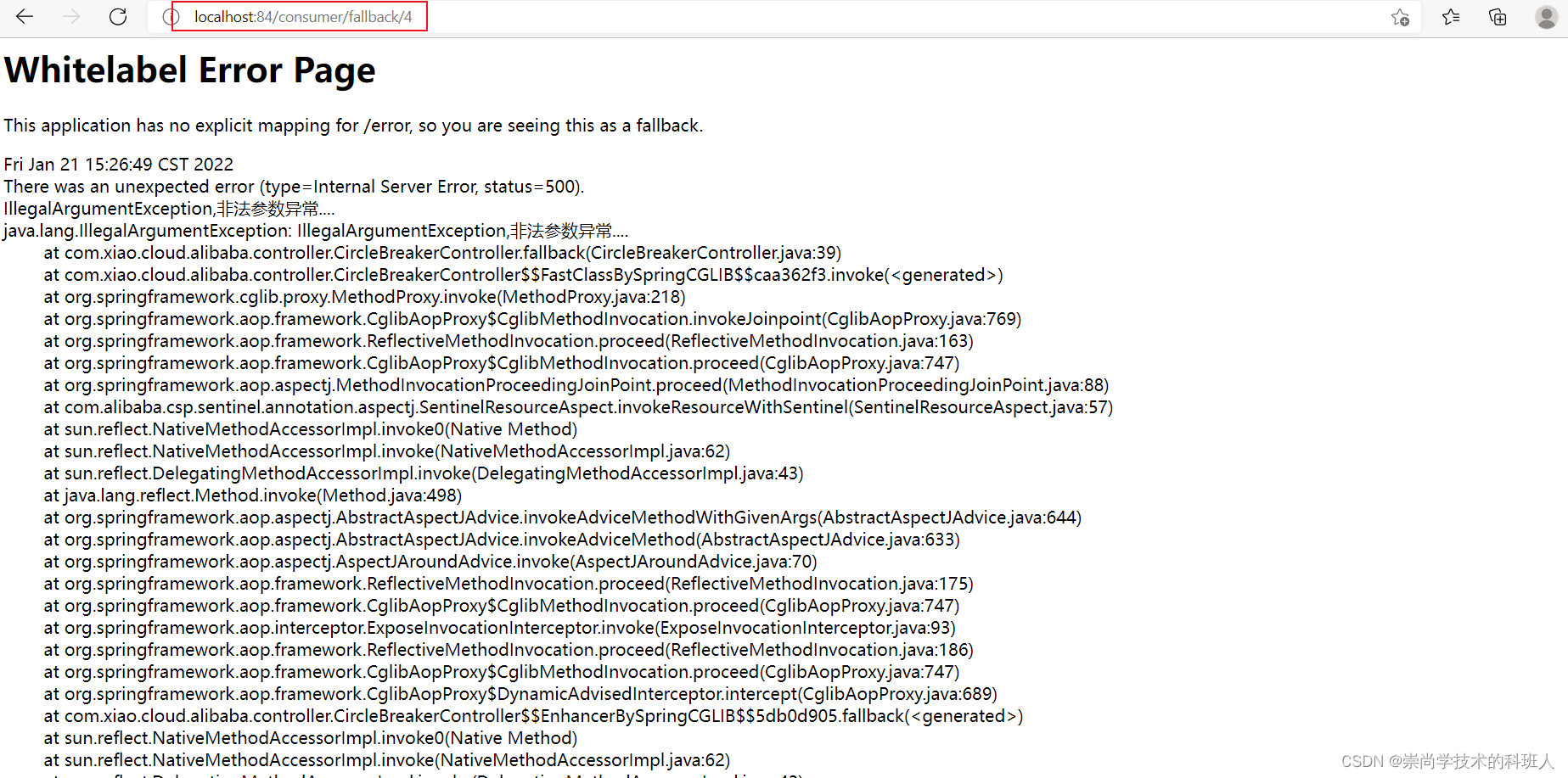
当我们携带的参数是5时且只是每秒钟一次的请求

当我们携带的参数是5时且只是每秒钟多次的请求

1.9.2、 Feign作为服务调用工具
1. 修改POM
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
2. 改YML
server:
port: 84
spring:
application:
name: nacos-order-consumer
cloud:
nacos:
discovery:
server-addr: localhost:8848
sentinel:
transport:
dashboard: localhost:8080
port: 8719
service-url:
nacos-user-service: http://nacos-payment-provider
feign:
sentinel:
enabled: true
3. PaymentService接口
import com.xiao.cloud.entities.CommonResult;
import com.xiao.cloud.entities.Payment;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
@FeignClient(value = "nacos-payment-provider",fallback = PaymentFallbackService.class)
public interface PaymentService {
@GetMapping(value = "/paymentSQL/{id}")
public CommonResult<Payment> paymentSQL(@PathVariable("id") Long id);
}
4. PaymentFallbackService实现类
import com.xiao.cloud.entities.CommonResult;
import com.xiao.cloud.entities.Payment;
import org.springframework.stereotype.Component;
@Component
public class PaymentFallbackService implements PaymentService{
@Override
public CommonResult<Payment> paymentSQL(Long id) {
return new CommonResult<Payment>(4444,"服务降级返回-------PaymentFallbackService",new Payment(1L,"errorSerial"));
}
}
5. 测试结果


1.10、持久化规则
就是我们面临的问题就是当我们项目重新启动的时候,那配置就会消失。对此我们可以将相关的配置存进Nacos ,当我们再次启动项目的时候,他会自己在sentinel 生产相应的配置(需要先进行url 访问),这就是持久化规则。
1. 改POM
<dependency>
<groupId>com.alibaba.csp</groupId>
<artifactId>sentinel-datasource-nacos</artifactId>
</dependency>
2. 改YML
server:
port: 8401
spring:
application:
name: cloudalibaba-sentinel-service
cloud:
nacos:
discovery:
server-addr: localhost:8848
sentinel:
transport:
dashboard: localhost:8080
port: 8719
datasource:
ds1:
nacos:
server-addr: localhost:8848
dataId: cloudalibaba-sentinel-service
groupId: DEFAULT_GROUP
data-type: json
rule-type: flow
management:
endpoints:
web:
exposure:
include: '*'
3. 在Nacos中进行配置
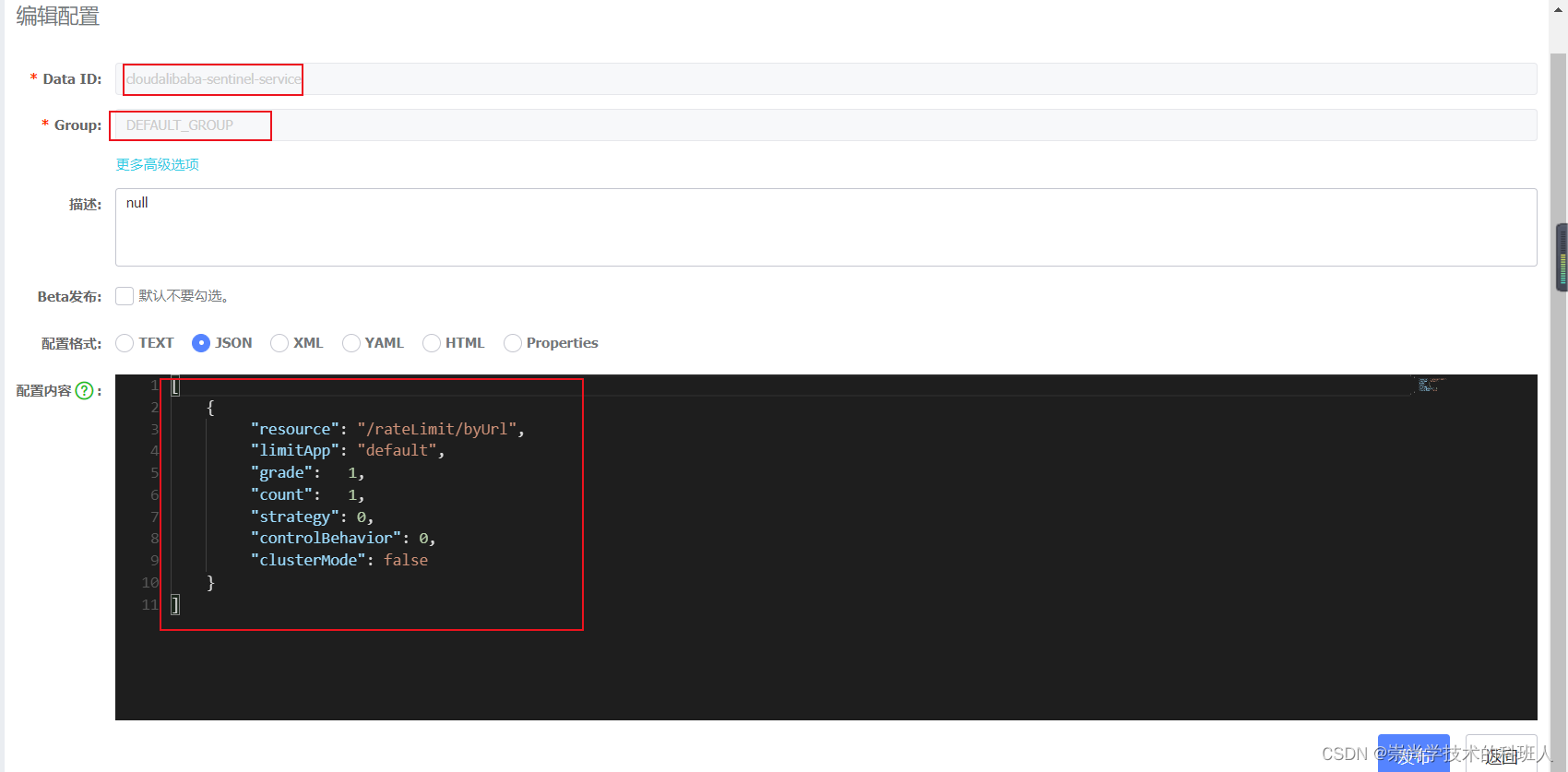
4. 启动8401刷新查看sentinel
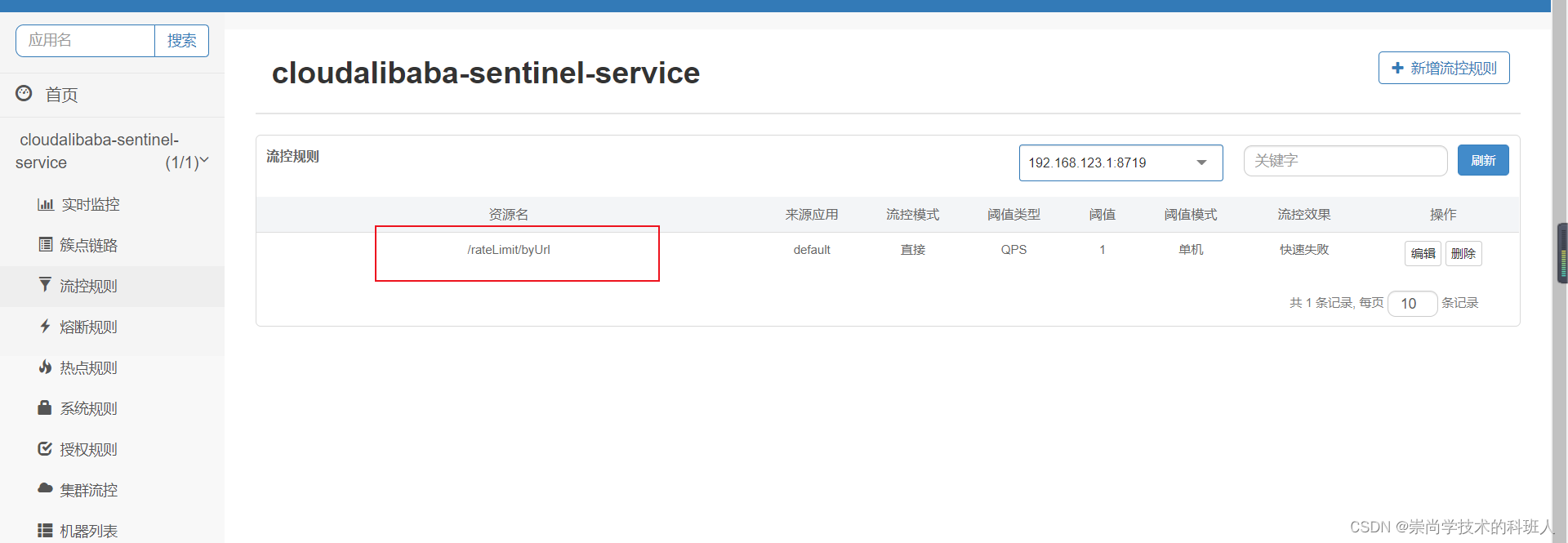
- 我们先要对
http://localhost:8401/rateLimit/byUrl 进行访问,因为sentinel 时懒加载机制的。
5. 快速访问接口
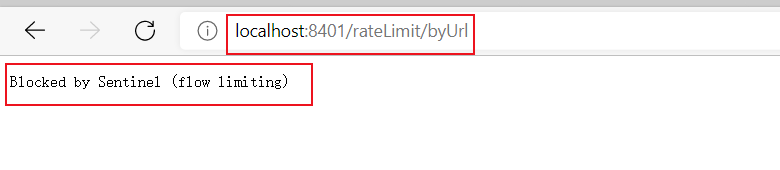
6. 停止8401再次查看sentinel
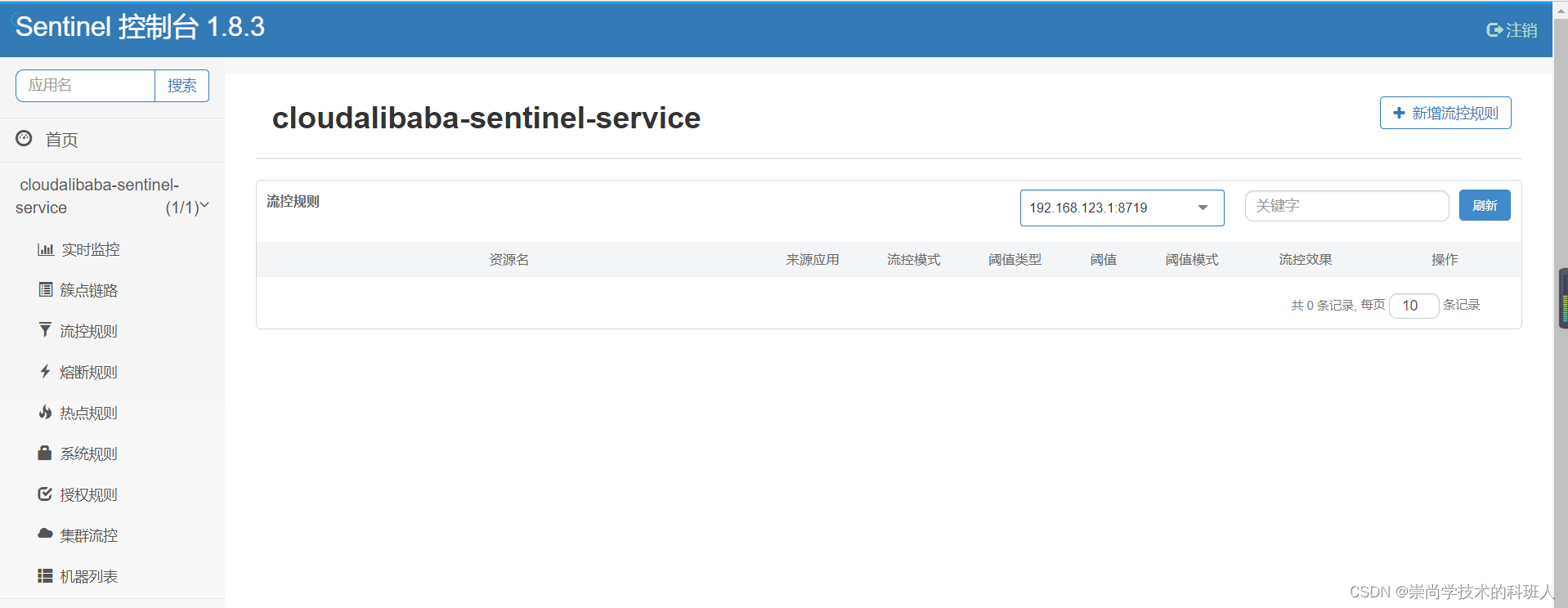
7. 启动8401再次查看sentinel
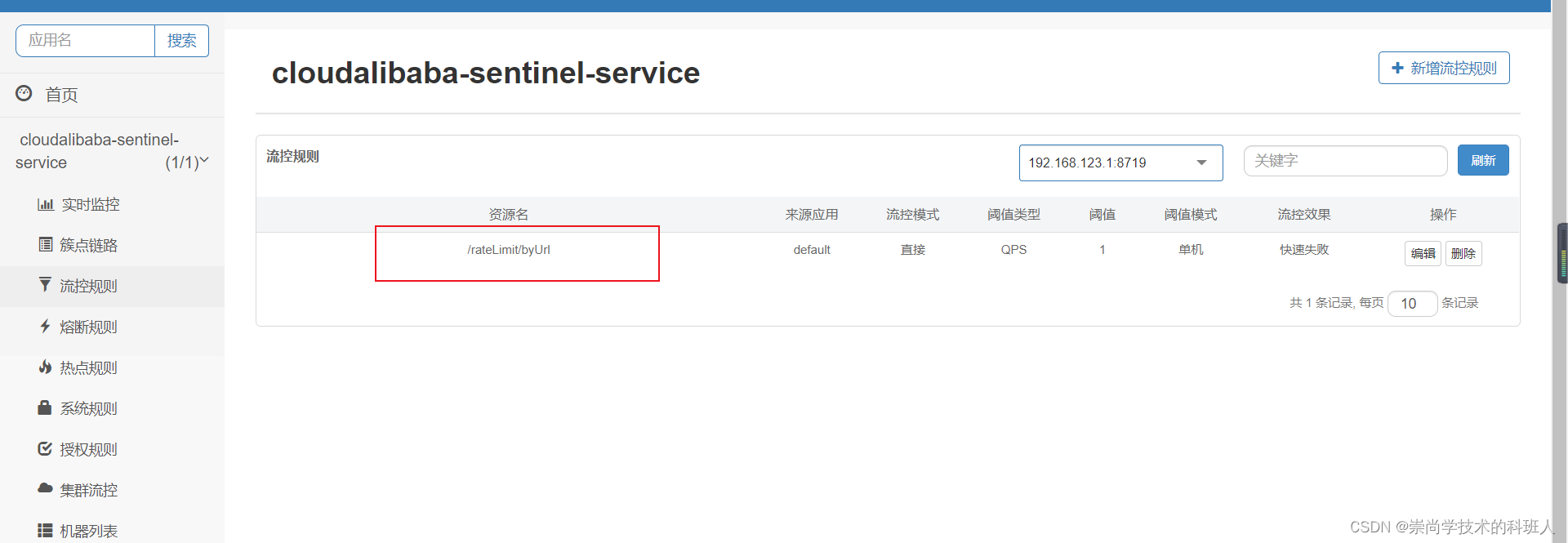
- 我们先要对
http://localhost:8401/rateLimit/byUrl 进行访问,因为sentinel 时懒加载机制的。
|