第四天:闰年的计算
1.if语句的嵌套
2.闰年的基本运算规律
3.布尔类型
package basic;
/**
* The complex usage of the if statement.
*
* @author 凤fff
*
*/
public class LeapYear {
/**
**************************
* The entrance of the program.
*
* @param args Not used now.
**************************
*/
public static void main(String args[]) {
// Test isLeapYear
int tempYear = 2021 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYear(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.");
tempYear = 2000 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYear(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.");
tempYear = 2100 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYear(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.");
tempYear = 2004 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYear(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.");
// Test isLeapYearV2
System.out.println("Now use the second version.") ;
tempYear = 2021 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYearV2(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.") ;
tempYear = 2000 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYearV2(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.") ;
tempYear = 2100 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYearV2(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.") ;
tempYear = 2004 ;
System.out.print(" " + tempYear + " is ");
if ( ! isLeapYearV2(tempYear)) {
System.out.print("Not ");
} // Of if
System.out.println("a leap year.") ;
} // Of main
/**
***********************
* Is the given year leap?
*
* @param paraYear The given year.
***********************
*/
public static boolean isLeapYear(int paraYear) {
if ((paraYear % 4 ==0) && (paraYear % 100 != 0) || paraYear % 400 == 0){
return true ;
} else {
return false ;
} // Of if
} // Of isLeapYear
/**
***********************
* Is the given year leap? Replace the complex condition with a number of if.
*
* @param paraYear The given Year.
***********************
*/
public static boolean isLeapYearV2(int paraYear) {
if (paraYear % 4 != 0) {
return false ;
} else if (paraYear % 400 == 0) {
return true ;
} else if (paraYear % 100 == 0) {
return false ;
} else {
return true ;
} // Of if
} // Of isLeapYearV2
} // Of class LeapYear
运行结果
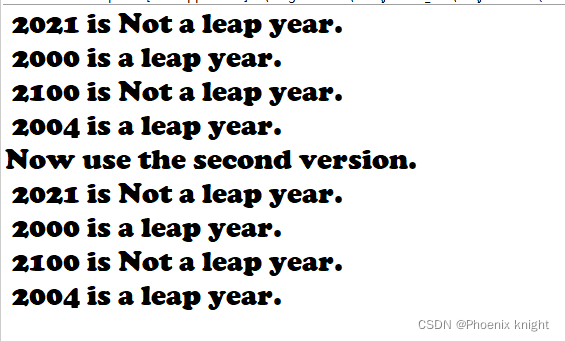
注意,在 isLeapYearV2 中 取余100 和取余 400 不能交换位置 判断是从上向下执行的如果上面的满足条件就不会向下执行
第五天:
1.Switch, case, break, default 用法
2. 单元测试单独使用一个方法,main 方法里面的代码越少越好
package basic;
/**
* This is the fifth code. Names and comments should follow my style strictly.
*
* @author 凤fff
*
*/
public class SwitchStatement {
/**
*********************
* The entrance of the program.
*
* @param args
* Not used now.
*********************
*/
public static void main(String args[]) {
scoreToLeveTest();
} // Of main
/**
*********************
* Score to level.
*
* @param paraScore
* From 0 to 100.
* @return The level form A to F.
*********************
*/
public static char scoreToLevel(int paraScore) {
// E stand for error, and F stands for fail.
char resultLevel = 'E';
// Divide by 10, the result rangs from 0 to 10
int tempDigitalLevel = paraScore / 10;
// The use of break is important.
switch (tempDigitalLevel) {
case 10:
case 9:
resultLevel = 'A';
break;
case 8:
resultLevel = 'B';
break;
case 7:
resultLevel = 'C';
break;
case 6:
resultLevel = 'D';
break;
case 5:
case 4:
case 3:
case 2:
case 1:
case 0:
resultLevel = 'F';
break;
default:
resultLevel = 'E';
} // Of switch
return resultLevel;
} // Of scoreToLevel
public static void scoreToLeveTest() {
int tempscore = 100;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
tempscore = 91;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
tempscore = 83;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
tempscore = 75;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
tempscore = 66;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
tempscore = 53;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
tempscore = 8;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
tempscore = 120;
System.out.println("Score " + tempscore + " to level is: " + scoreToLevel(tempscore));
} // Of scoreToLevelTest
} // Of class SwitchStatement
运行结果
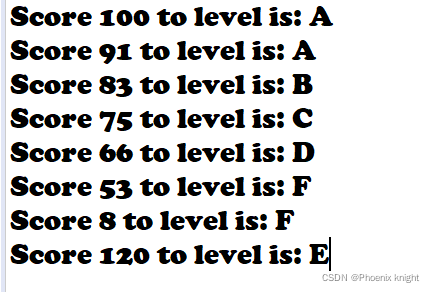
当 条件分支有多个 及 条件的值是整数或一个字符值 选用Switch更好
|