ExecutorService的使用方法
ExecutorService介绍
ExecutorService是java.util.concurrent包下的一个接口,作为一个线程池接口,主要负责任务执行管理。 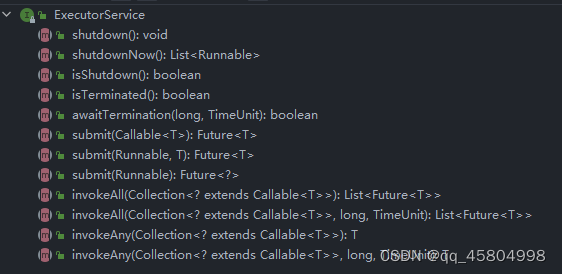 Java API对ExecutorService接口的具体实现类主要有三个,如下所示:
- ThreadPoolExecutor
- ForkJoinPool
- ScheduledThreadPoolExecutor
主要UML图如下所示 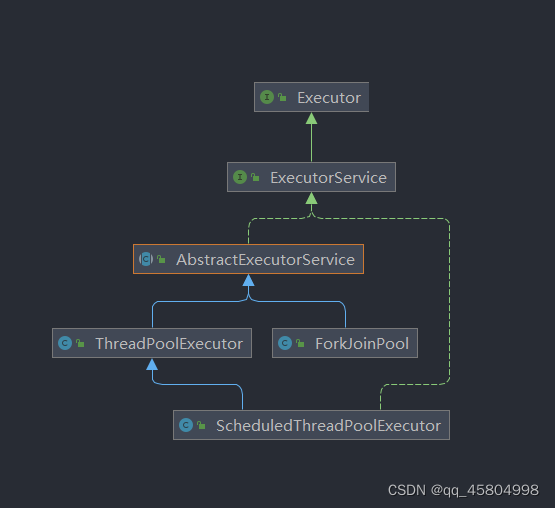
ExecutorService创建
我们创建ExecutorService时一般是直接通过java提供的工具类Executors工厂类进行创建。而Executors工厂类一共提供了下列方法区创建线程池。
1. newFixedThreadPool
2. newWorkStealingPool
3. newSingleThreadExecutor
4. newCachedThreadPool
5. newSingleThreadScheduledExecutor
6. newScheduledThreadPool
- newFixedThreadPool
创建一个线程数量固定的线程池 - newWorkStealingPool
返回一个使用所有可用处理器作为目标并行度的ForkJoinPool线程池 - newSingleThreadExecutor
创建一个单线程线程池 - newCachedThreadPool
创建一个可缓存的线程池,需要时创建线程,不需要则销毁 - newSingleThreadScheduledExecutor
创建一个单线程定时执行器(ScheduledExecutorService) - newScheduledThreadPool
创建一个定时执行器
ExecutorService的使用
ExecutorService提供任务时可以提交Runnable与Callable来执行任务并返回Future用于接受Callable中返回的数据,
submit(Callable callable)
提交Callable
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> submit = executorService.submit(() -> {
TimeUtils.sleepMilliSecond(1000);
return "abs";
});
submit.get(100, TimeUnit.MILLISECONDS);
submit.get();
submit(Runnable run)
提交Runnable
ExecutorService executorService = Executors.newSingleThreadExecutor();
executorService.submit(() -> {
LogUtils.info("执行Runnable");
});
invokeAny
执行Callable列表,当存在一个线程执行完毕并返回结果时,invokeAny执行结束并返回结果.
ExecutorService executorService = Executors.newCachedThreadPool();
List<Callable<String>> callables = new ArrayList<>();
callables.add(() -> {
TimeUtils.sleepMilliSecond(RandomUtils.nextInt(0, 1000));
return "a";
});
callables.add(() -> {
TimeUtils.sleepMilliSecond(RandomUtils.nextInt(0, 1000));
return "b";
});
callables.add(() -> {
TimeUtils.sleepMilliSecond(RandomUtils.nextInt(0, 1000));
return "c";
});
String s = executorService.invokeAny(callables);
LogUtils.info("s = {}", s);
invokeAll
执行Callable列表,并在任务执行完毕后返回future列表用于获取信息
ExecutorService executorService = Executors.newCachedThreadPool();
List<Callable<String>> callables = new ArrayList<>();
callables.add(() -> {
TimeUtils.sleepMilliSecond(RandomUtils.nextInt(0, 1000));
return "a";
});
callables.add(() -> {
TimeUtils.sleepMilliSecond(RandomUtils.nextInt(0, 1000));
return "b";
});
callables.add(() -> {
TimeUtils.sleepMilliSecond(RandomUtils.nextInt(0, 1000));
return "c";
});
List<Future<String>> futures = executorService.invokeAll(callables);
for (Future<String> future : futures) {
String s = future.get();
LogUtils.info("s = {}", s);
}
shutdown
等待线程池中所有任务结束然后停止
shutdownNow
立即停止线程池
isShutdown
判定线程池是否被停止
isTerminated
判定线程池任务是否结束
awaitTermination
当调用了awaitTermination后有一下一种状态.
1. 没有超时,并且子线程执行完,此时返回true
2. 没有超时,但子线程没有执行完,此时返回false
3. 超时,此时返回false
在执行awaitTermination前需要执行shutdown方法,否则awaitTermination一定超时并且返回false
ExecutorService executorService = Executors.newSingleThreadExecutor();
executorService.submit(() -> {
LogUtils.info(Thread.currentThread().getName());
TimeUtils.sleepMilliSecond(RandomUtils.nextInt(0, 1000));
});
executorService.shutdown();
LogUtils.info("开始等待");
boolean b = executorService.awaitTermination(3, TimeUnit.SECONDS);
LogUtils.info("等待完毕");
if(b){
LogUtils.info("分线程已经结束");
}
LogUtils.info(Thread.currentThread().getName());
|