实体类?
package com.example.demo.entity;
public class Person {
private Integer age;
private String name;
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Person(Integer age, String name) {
this.age = age;
this.name = name;
}
@Override
public String toString() {
return "Person{" +
"age=" + age +
", name='" + name + '\'' +
'}';
}
}
测试类
@Test
void contextLoads() {
List<Person> personList = new ArrayList<>();
personList.add(new Person(1,""));
personList.add(new Person(3,""));
personList.add(new Person(4,"小红"));
Map<String, List<Person>> groups = personList.stream().collect(Collectors.groupingBy(ele -> ele.getName(), Collectors.mapping(Function.identity(), Collectors.toList())));
groups.forEach((k,v)->{
if(k.isEmpty()){
System.out.println(k+"ok===" + v);
}else{
System.out.println(k+"===" + v);
}
});
System.out.println(groups);
System.out.println("============================");
List<Person> people = new ArrayList<>();
people.add(new Person(1,null));
people.add(new Person(3,""));
people.add(new Person(4,"小红"));
Map<Optional<String>, List<Person>> group = people.stream().collect(Collectors.groupingBy(ele -> Optional.ofNullable(ele.getName()), Collectors.mapping(Function.identity(), Collectors.toList())));
group.forEach((k,v)->{
if(k.filter(e->e.isEmpty()).isPresent()){
System.out.println(k+"ok===" + v);
}else{
System.out.println(k+"===" + v);
}
});
System.out.println(group);
}
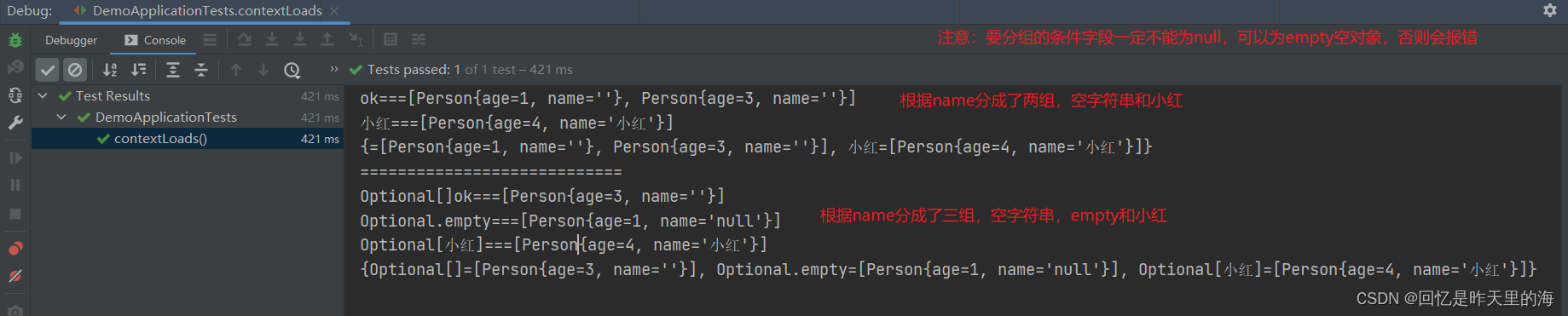
?注意:
使用groupBy的时候一定要确保分组的字段不是null的,可以是empty但是不能是null,否则会报错,如下图
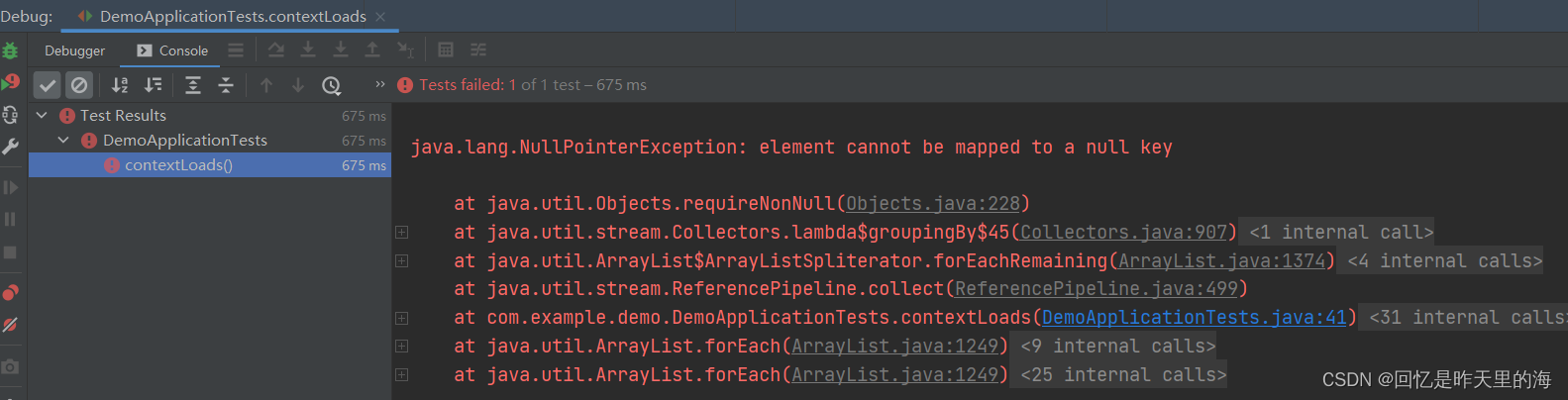
?
|