可变参数
- 在方法声明中,在指定参数类型后加一个省略号(…)
- 一个方法只能指定一个可变参数,它必须是方法的最后一个参数。任何普通的参数必须在它之前声明。
public class Demo_6 {
public static void main(String[] args) {
Demo_6 demo_6 = new Demo_6();
demo_6.test(1,2,3,4,5,6,7);
}
public void test(int x,int y,int... i){
for (int i1 = 0; i1 < i.length; i1++) {
System.out.print(""+i[i1]+"\t");
}
}
}
递归
求阶乘
package Recursion;
import java.util.Scanner;
public class Demo_2 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int x = scanner.nextInt();
System.out.println(Factorial1(x));
}
public static int Factorial1(int x){
if(x < 0){System.out.println("参数错误") return -1;}
if(x == 0)return 1;
else{return x * Factorial1(x - 1);}
}
}
或者用三目运算符偷懒。。。。。
public static int Factorial2(int x){
return x == 1 ? 1 : x * Factorial2(x - 1);
}
嗷对了,阶乘的英文是 factorial (adj.阶乘的)
由于Java是栈机制,递归的消耗会很大,尽量别用
计算器作业
import java.util.Scanner;
public class Homework {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
do{
System.out.print("请输入第一个数字:");
double a = scanner.nextDouble();
System.out.print("请输入第二个数字:");
double b = scanner.nextDouble();
System.out.print("请输入要进行的运算:");
String c = scanner.next();
switch(c){
case "+":System.out.println(""+a+" "+c+" "+b+" ="+" "+(a+b));break;
case "-":System.out.println(""+a+" "+c+" "+b+" ="+" "+(a-b));break;
case "*":System.out.println(""+a+" "+c+" "+b+" ="+" "+(a*b));break;
case "/":System.out.println(""+a+" "+c+" "+b+" ="+" "+((double)a/b));break;
case "%":System.out.println(""+a+" "+c+" "+b+" ="+" "+(a%b));break;
default: System.out.println("输入不合理!");break;
}
System.out.println("请问是否要继续(Y/N):");
String c1 = scanner.next();
if(c1.equals("Y"))continue;
else break;
}while(true);
scanner.close();
}
}
比较两个字符串是否相同:
String a = "aaa";
String b = "bbb";
a.equals(b);
有一点十分难受:
System.out.println("请问是否要继续(Y/N):");
String c1 = scanner.next();
System.out.print(c1 == "Y");
System.out.println(c1.equals("Y"));
上面false,下面true 可能的解释: 1、由于c1和"Y"地址不同,采用上面的比较法,输出肯定是false 2、通常采用equals进行比较,equals运用了Java的重载。
next() 和 nextLine() 的区别 hasNext() 和 hasNextLine() 的区别 判断字符串是否相等 Java字符串方法大全(超超超详细)
数组
数组的声明和创建
package Praparation;
public class Demo_1 {
public static void main(String[] args) {
int[] a = {1,2,3,4,5,6,7};
char[] b = {'a','b','c','d','e'};
char[] c1;
char c2[];
c1 = new char[5];
c1[0] = 'a';
c1[1] = 'b';
c1[2] = 'c';
c1[3] = 'd';
c1[4] = 'e';
int[] num = new int[10];
for (int i = 0; i < c1.length; i++) {
System.out.print(c1[i]+"\t");
}
}
}
三种初始化及内存分析
内存分析
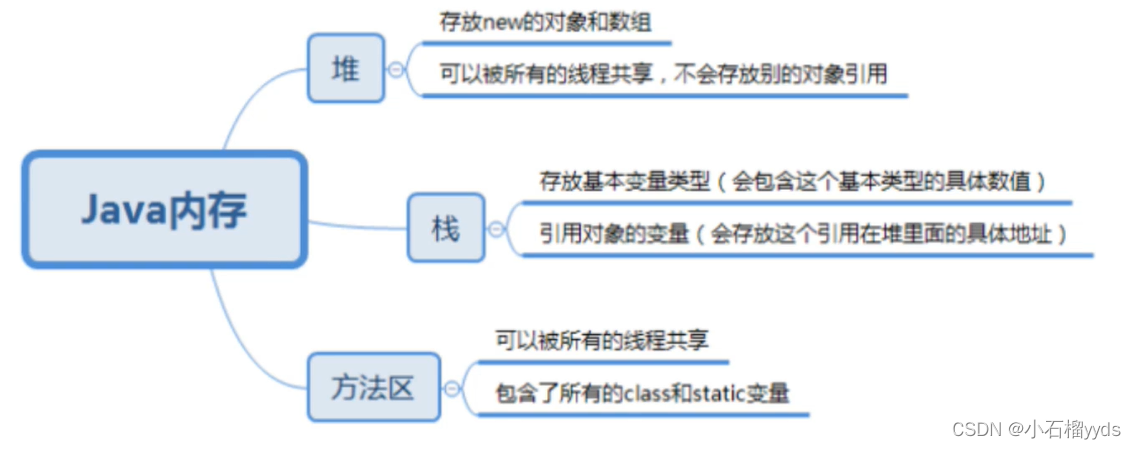 图源B站狂神 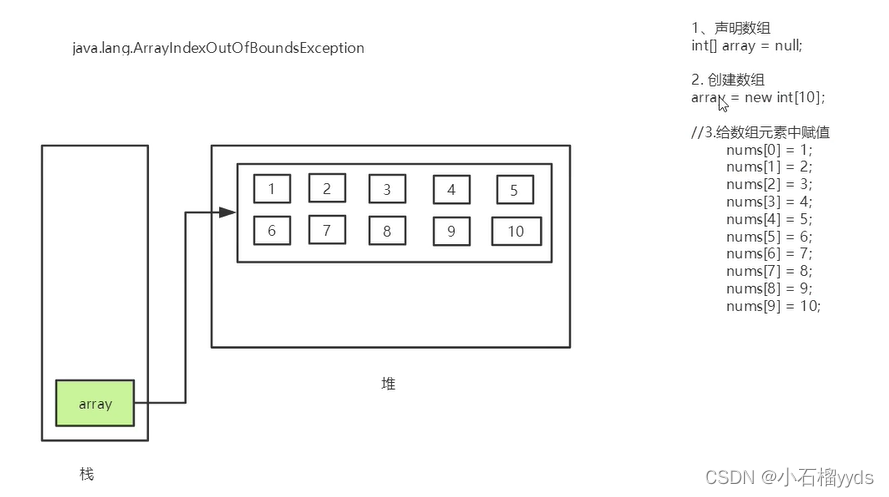
图源B站狂神
- 声明数组时,将变量类型放在栈中
- 创建数组时,在堆中开辟了数组的空间并准备储存数据
- 赋值数组时,在堆中给每一个位置赋值
三种初始化
package Praparation;
public class Initialization {
public static void main(String[] args) {
int[] a = {1,2,3};
Man[] men = {new Man(),new Man()};
int[] b = new int[10];
b[0] = 10;
}
}
默认初始化值:
- 整数类型(byte、short、int、long)的基本类型变量的默认值为0。
- 单精度浮点型(float)的基本类型变量的默认值为0.0f。
- 双精度浮点型(double)的基本类型变量的默认值为0.0d。
- 字符型(char)的基本类型变量的默认为 “/u0000”。
- 布尔性的基本类型变量的默认值为 false.
- 引用类型的变量是默认值为 null。
- 数组引用类型的变量的默认值为 null。
|