第3章 Java的基本程序结构
3.1 一个简单的Java应用程序
public class FirstSample {
public static void main(String []args) {
System.out.println("Hello world!");
}
}
3.2 注释
3.3 数据类型
(1)整型
类型 | 存储需求 | 取值范围 |
---|
int | 4字节 | -2 147 483 648~2 147 483 647 | short | 2字节 | -32 768~32 767 | long | 8字节 | -9 223 372 036 854 775 808~9 223 372 036 854 775 807 | byte | 1字节 | -128~127 |
(2)浮点型
类型 | 存储需求 | 取值范围 | 表示 |
---|
float | 4字节 | 大约±3.402 823 47E+38F(有效数位6~7位) | 3.14F或3.14f | double | 8字节 | 大约±1.797 693 134 862 315 70E+308(有效数位15位) | 3.14或3.14D或3.14d |
注:
if(x == Double.NaN)
if(Double.isNaN(x))
(3)char类型 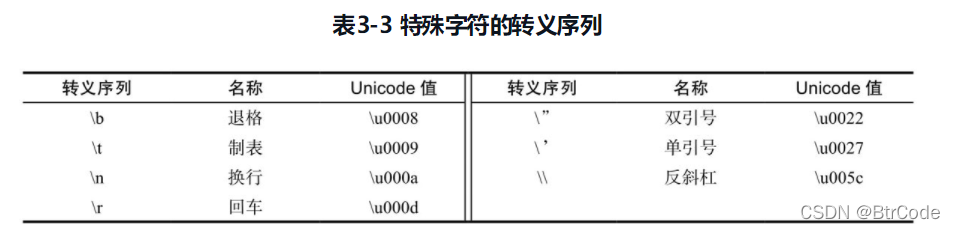
(4)boolean类型
3.4 变量
(1)常量定义
final int ABC_D_F = 2;
static final int X_Y_Z = 10;
3.5运算符
(1)/ 运算:
/ 运算 | 结果 | 说明 |
---|
15 / 2 | 7(int) | 当参与/运算的两个操作数都是整数时,表示整数除法;否则,表示浮点除法。 | 15.0 / 2 | 7.5(double) | 当参与/运算的两个操作数都是整数时,表示整数除法;否则,表示浮点除法。 |
(2)数学函数与常量
import java.lang.Math.*;
System.out.println(Math.PI);
import static java.lang.Math.*;
System.out.println(PI);
(3)数值类型之间的转换
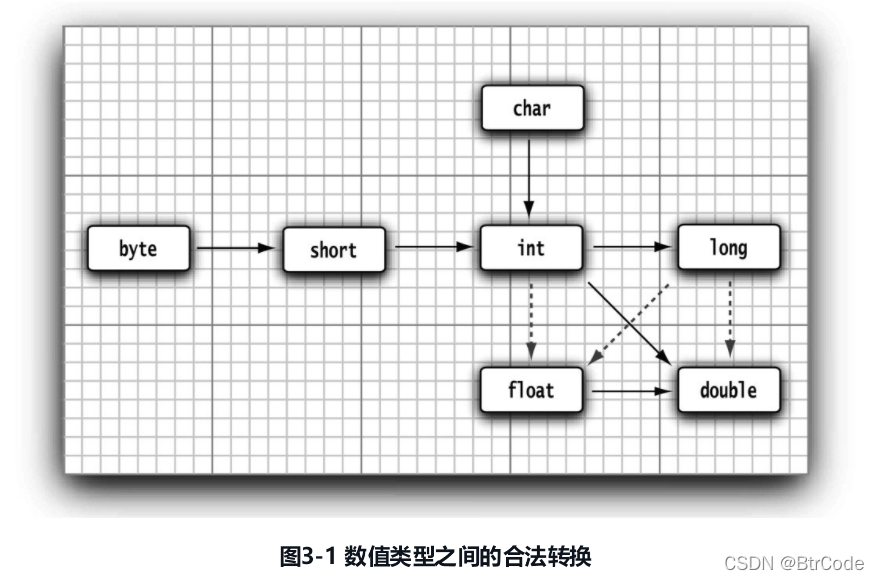
(4)强制类型转换
double x = 9.993;
int y = (int)x;
double x = 9.993;
int y = (int)Math.round(x);
(5)关系运算
- a && b 和 a|| b (短路方式运算):如果a为false,b就不计算。
(6)位运算
- a & b 和 a| b (非短路方式运算):a和b都计算。
- > > >:用0填充高位。
- > > :用符号位填充高位。
- < <
3.6 字符串
(1)子串
String str = "Hello World!";
String str1 = str.substring(0,5);
(2)拼接
String a = "Hello";
String b = "World";
String c = a + b;
int index = 987;
String d = a + index;
String str = String.join(" ", "a", "b", "c");
(3)不可变字符串
String为不可变字符串。
(4)检测字符串是否相等
== :检测两个字符串是否放在同一位置上。
equals():检测两个字符串内容是否相同。
equlsIgnoreCase():忽略大小写检测两个字符串内容是否相同。
String a = "Hello";
String b = "hello";
if(a.equals(b))
if(a.equlsIgnoreCase(b))
(5)空串和Null串
- 空串:即"",是一个Java对象,有自己的串长度(0)和内容(空)。
- Null串:即null,表示目前没有任何对象与该变量关联。
if(str == null)
if(str != null && str.length() != 0)
3.7 输入输出
(1)读取输入
Scanner in = new Scanner(System.in);
String str = in.next();
while(in.hasNext()) {
str = in.next();
}
(2)格式化输出
与C语言相比,将printf()用System.out.printf()替换即为该格式化输出方法。
Double num = 10000 / 3.0;
System.out.printf("%f\n", num);
System.out.printf("%.2f\n", num);
System.out.printf("%8.2f\n", num);
System.out.printf("%-8.2f\n", num);
System.out.printf("%,.2f\n", num);
3333.333333
3333.33
3333.33
3333.33
3,333.33
(3)文件输入与输出
try {
Scanner in = new Scanner(Paths.get("src/Test1/hello.txt"), "UTF-8");
while(in.hasNextLine()) {
System.out.println(in.nextLine());
}
} catch (IOException e) {
e.printStackTrace();
}
3.8 控制语句
(1)条件与循环
if(condition){} 和 while(condition){} 的condition 只能是 表达式 或 Boolean 对象。
(2)中断控制流程
3.9 大数值(BigInteger 和 BigDecimal)
(1)BigInteger(整数)
BigInteger a = BigInteger.valueOf(2);
(2)BigDecimal(浮点数)
BigDecimal a = BigDecimal.valueOf(2);
均不支持"+"、"-"、"*"、"/"等运算符,“加减乘除”等运算算法由其封装的方法提供。
3.10 数组
(1)二维数组foreach遍历
int[][] arr = new int[10][10];
for(int[] row : arr) {
for(int value : row) {
}
}
(2)创建不规则数组
int[][] arr = new int[10][];
for(int i=0; i<arr.length; i++) {
arr[i] = new int[i];
}
(3)Arrays方法类
``
均不支持"+"、"-"、"*"、"/"等运算符,“加减乘除”等运算算法由其封装的方法提供。
3.10 数组
(1)二维数组foreach遍历
int[][] arr = new int[10][10];
for(int[] row : arr) {
for(int value : row) {
}
}
(2)创建不规则数组
int[][] arr = new int[10][];
for(int i=0; i<arr.length; i++) {
arr[i] = new int[i];
}
(3)Arrays方法类
|