?html+css文件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Whac a Mole</title>
<style>
#content{
width: 960px;
margin: 0 auto;
text-align: center;
position: relative;
}
table{
margin: 0 auto;
cursor: url('img/chuizi.png'),auto;
}
td{
width: 95px;
height: 95px;
background-color: #00ff33;
}
#start,#end{
margin: 20px 10px;
width: 50px;
height: 50px;
border-radius: 50%;
background-color: green;
font-size: 16px;
font-weight: bold;
color: white;
border: 1px solid #cccccc;
box-shadow: 2px 2px grey;
cursor: pointer;
}
#start:active{
border: none;
}
#end{
background-color: red;
}
form{
margin: 0 0 20px 0;
}
form > input{
border-radius: 50%;
border:none;
text-align: center;
font-weight: 16px;
color: red;
}
#notice{
background-color: #eee;
border-radius: 10%;
position: relative;
margin: 0 auto;
top: -400px;
box-shadow: 2px 2px #666666;
transition: all 0.2s ease-in-out;
text-align: center;
overflow: hidden;
}
.noticeshow{
width: 340px;
height: 180px;
}
.noticehide{
width: 0px;
height: 0px;
}
#notice > button{
width: 80px;
height: 25px;
position: absolute;
bottom: 18px;
left: 130px;
background-color: blue;
color: white;
font-weight: bold;
font-size: 18px;
border-radius: 10%;
border: none;
cursor: pointer;
}
#notice > .title{
padding-top: 14px;
}
img {
width: 100%;
}
</style>
<script src="https://code.jquery.com/jquery-3.0.0.min.js"></script>
<script src="main_jq.js"></script>
</head>
<body>
<div id="content">
<input type="button" value="start" id="start">
<input type="button" value="end" id="end">
<form name="form1">
<label for="">score:</label>
<input type="text" name="score" size="5" disabled>
<label for="">hit rate:</label>
<input type="text" name="success" size="10" disabled>
<label for="">countdown:</label>
<input type="text" name="remtime" size="5" disabled>
<label for="">level:</label>
<select name="level" id="level">
<option value="1">easy</option>
<option value="2">normal</option>
<option value="3">hard</option>
<option value="4">abnormal</option>
</select>
</form>
<table>
<tr>
<td></td>
<td></td>
<td></td>
<td></td>
<td></td>
</tr>
<tr>
<td></td>
<td></td>
<td></td>
<td></td>
<td></td>
</tr>
<tr>
<td></td>
<td></td>
<td></td>
<td></td>
<td></td>
</tr>
<tr>
<td></td>
<td></td>
<td></td>
<td></td>
<td></td>
</tr>
<tr>
<td></td>
<td></td>
<td></td>
<td></td>
<td></td>
</tr>
</table>
<div id="notice" class="noticehide">
<h3 class="title"></h3>
<span class="message"></span>
<button>confirm</button>
</div>
</div>
</body>
</html>
?javascript文件
$(function(){
var appertime,disappertime;
var game=new Dadishu();
$("#start").click(function(){
var level = $("#level").val();
game.setLevel(level);
});
$("#end").click(function(){
game.GameOver();
});
$("td").map(function(){
$(this).click(function(){
var elem=this;
game.hit(elem);
});
});
$("#notice > button").click(function(){
$("#notice").attr("class","noticehide");
});
});
~(function(){
window.Dadishu=dadishu;
function dadishu(){
this.playing=false;
this.intId=null;
this.timeId=null;
this.score=0;
this.beat=0;
this.knock=0;
this.success=0;
this.countdown=30;
this.appertime=500;
this.disappertime=1000;
}
dadishu.prototype.GameStart=function(appertime,disappertime){
if(this.playing){
return;
}else{
if($("#notice").attr("class")!="noticeshow"){
var that=this;
that.playing=true;
that.intId=setInterval(function(){
that.show.call(that);
},this.appertime);
document.form1.success.value=this.success;
document.form1.score.value=this.score;
that.timeShow();
}else{
return;
}
}
}
dadishu.prototype.show=function(){
if(this.playing){
var current=Math.floor(Math.random()*25);
$($("td")[current]).html("<img src='11.jpg'>");
setTimeout(function(){
$($("td")[current]).html("");
},this.disappertime);
}
}
dadishu.prototype.timeShow=function(){
if(this.playing){
document.form1.remtime.value=this.countdown;
if(this.countdown==0){
this.GameOver();
return;
}else{
this.countdown-=1;
var that=this;
this.timeId=setTimeout(function(){
that.timeShow();
},1000);
}
}
}
dadishu.prototype.GameOver=function(){
if(!this.playing){
return;
}else{
this.timeStop();
this.playing=false;
$("#notice > .title").text("Game Over!");
$("#notice > .message").html("Your score: <span style='color:red'>"+this.score+"</span></br> Your hit rate:<span style='color:red'>"+this.success+"</span>");
$("#notice").attr("class","noticeshow");
this.clearMouse();
this.success=0;
this.score=0;
this.knock=0;
this.beat=0;
this.countdown=30;
}
}
dadishu.prototype.timeStop=function(){
clearInterval(this.intId);
clearTimeout(this.timeId);
}
dadishu.prototype.clearMouse=function(){
for(var i=0;i<25;i++){
$($("td")[i]).html("");
}
}
dadishu.prototype.hit=function(elem){
if(!this.playing){
if($("#notice").attr("class")!="noticeshow"){
$("#notice > .title").text("Notice:");
$("#notice > .message").text("Please start the game!");
$("#notice").attr("class","noticeshow");
}else{
return;
}
}else{
this.beat+=1;
if($(elem).html()==""){
this.score-=1;
this.success=( Math.round(parseFloat(this.knock/this.beat)*10000)/100 )+"%";
document.form1.score.value=this.score;
document.form1.success.value=this.success;
}else{
this.knock+=1;
this.score+=1;
this.success=( Math.round(parseFloat(this.knock/this.beat)*10000)/100 )+"%";
document.form1.score.value=this.score ;
document.form1.success.value=this.success;
$(elem).html("");
$(elem).css("background-color","red");
setTimeout(() => {
$(elem).css("background-color","#00ff33");
}, 200);
}
}
}
dadishu.prototype.setLevel=function(level){
if(level==1){
this.appertime=1000;
this.disappertime=2000;
}else if(level==2){
this.appertime=500;
this.disappertime=1500;
}else if(level==3){
this.appertime=400;
this.disappertime=1000;
}else{
this.appertime=200;
this.disappertime=600;
}
this.GameStart();
}
})()
因为没有拿别人的老鼠图片,所以用的自己的图片
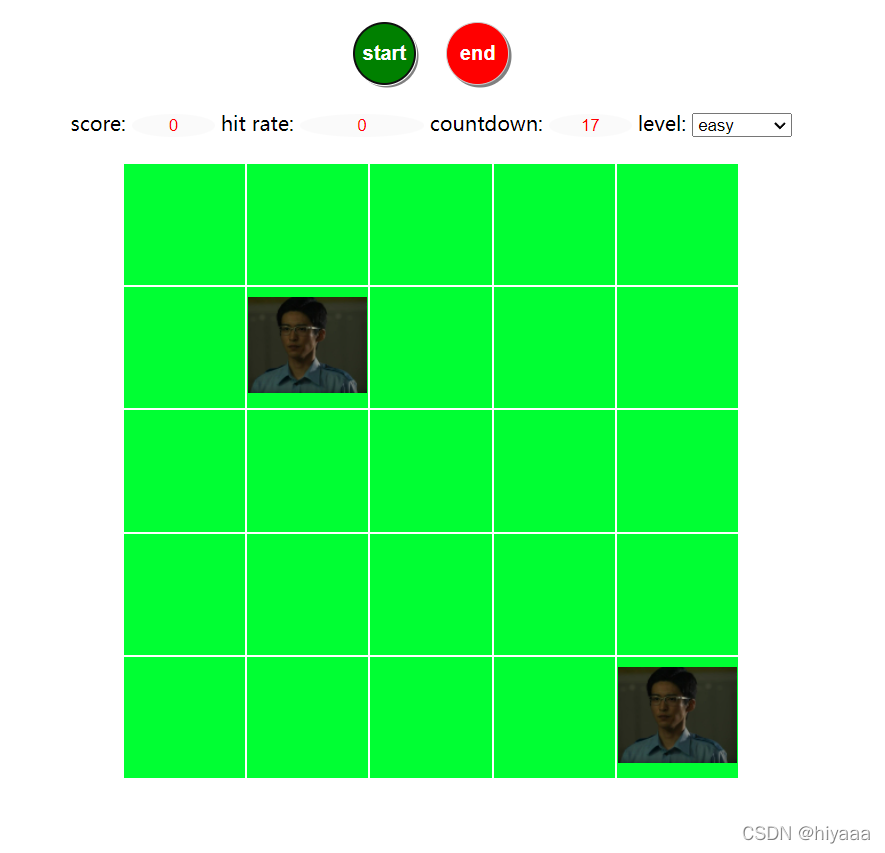
?
|