一、多线程拷贝单个文件
多核CPU上使用多线程程序来进行拷贝能提高拷贝效率,因此如何实现对一个文件使用多线程拷贝就成了问题,毕竟多线程其实是一起执行不同的任务,但是如果你直接对一个文件直接上多线程,那么其实就是每个线程都重复拷贝了这个文件,因此我使用了RandomAccessFile类来读入和写出文件,该类的优势就在于其可以从文件的某个位置开始读取而不一定要从头到尾.
@Override
public void run() {
try (RandomAccessFile rafi = new RandomAccessFile(fileInput, "r");
RandomAccessFile rafo = new RandomAccessFile(fileOutput, "rw");) {
rafi.seek(start);
rafo.seek(start);
byte[] buffer = new byte[1024];
int len = 0;
int count = 0;
while ((len = rafi.read(buffer)) != -1 && count < (end - start)) {
count += len;
rafo.write(buffer);
}
} catch (IOException e) {
e.printStackTrace();
}
}
二、遍历文件夹数据
1.思路分析
首先需要做到的是考虑到文件夹中可能还有文件夹,因此代码中一定需要有一个创建文件夹的代码,也就是mkdir/mkdirs. 并且如果当前遇到的是文件夹,那么应该继续遍历该文件夹内部的文件,而如果当前就是一个文件,那么直接使用多线程拷贝即可
2.代码
package IOStream_ch13;
import java.io.*;
public class FileCopy implements Runnable {
File fileInput;
File fileOutput;
long start;
long end;
public FileCopy() {
}
public FileCopy(File fileInput, File fileOutput, long start, long end) {
this.fileInput = fileInput;
this.fileOutput = fileOutput;
this.start = start;
this.end = end;
}
public static void main(String[] args) {
String srcFolder = "D:\\desktopfile\\.idea";
String destFolder = "D:\\dest";
copyFolder(srcFolder, destFolder);
}
private static void copyFolder(String srcFolder, String destFolder) {
File srcDir = new File(srcFolder);
if (!srcDir.exists()) {
System.out.println("待拷贝文件夹不存在");
return;
}
File destDir = new File(destFolder);
if (!destDir.exists()) {
destDir.mkdirs();
}
File[] files = srcDir.listFiles();
for (File file : files) {
File destFile = new File(destDir, file.getName());
if (file.isFile()) {
long length = file.length() / 4;
for (int i = 0; i < 4; i++) {
new Thread(new FileCopy(file,
new File(destFolder, file.getName()),
i * length, (i + 1) * length)).start();
}
} else {
copyFolder(file.getAbsolutePath(), destFile.getAbsolutePath());
}
}
}
@Override
public void run() {
try (RandomAccessFile rafi = new RandomAccessFile(fileInput, "r");
RandomAccessFile rafo = new RandomAccessFile(fileOutput, "rw");) {
rafi.seek(start);
rafo.seek(start);
byte[] buffer = new byte[1024];
int len = 0;
int count = 0;
while ((len = rafi.read(buffer)) != -1 && count < (end - start)) {
count += len;
rafo.write(buffer);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
下图是待拷贝的文件夹,这个文件夹中还有文件夹.
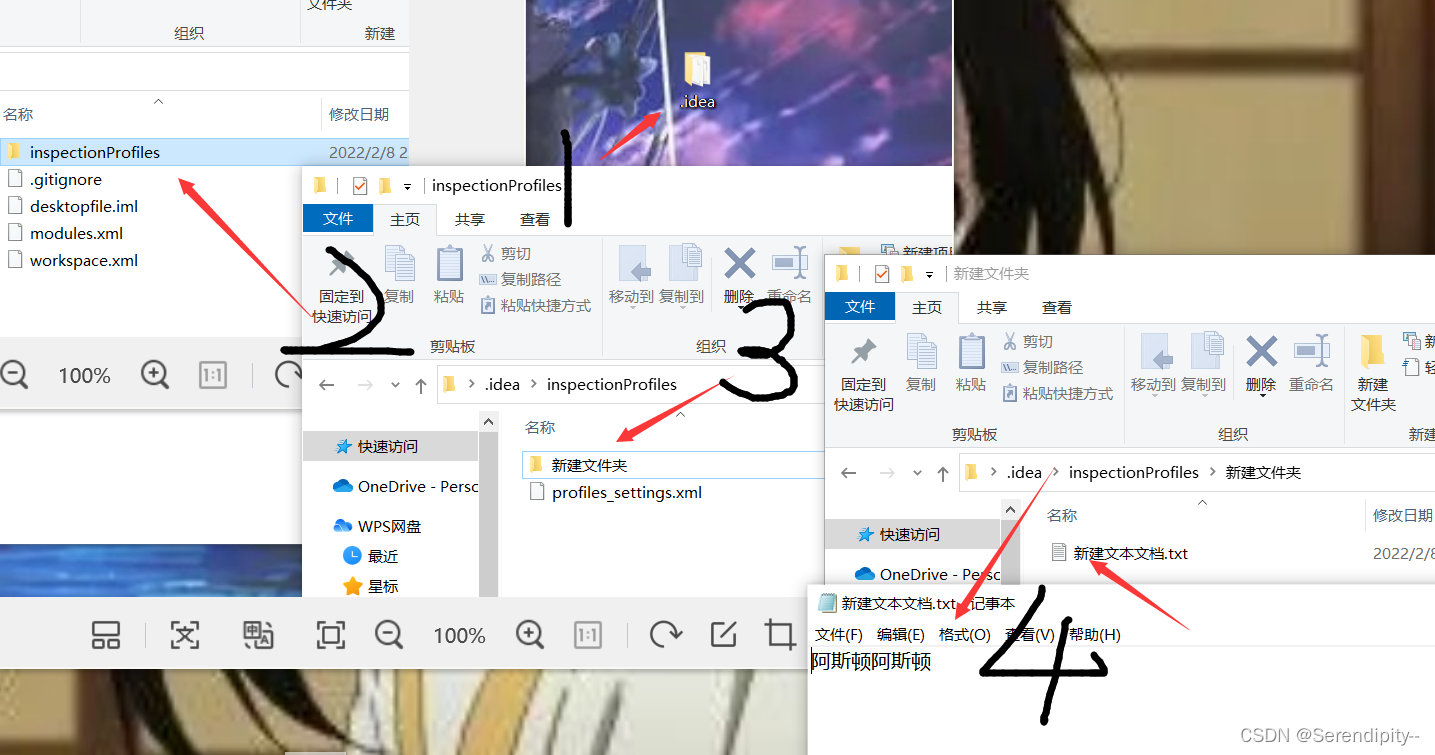 下图是拷贝效果 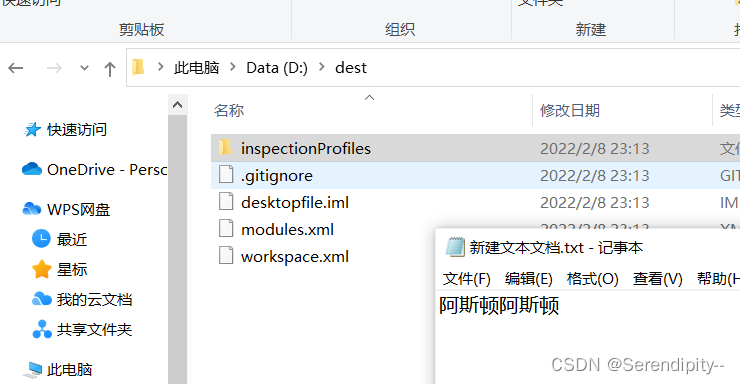
|