简介
swagger是为了协调前后端开发的api文档框架
- 直接运行,测试api接口
- 支持多种语言
- 支持restful风格
Swagger快速搭建
-
导入依赖 <dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
-
编写swaggerconfig @Configuration
@EnableSwagger2
public class swaggerConfig {
}
-
测试http://localhost:8080/swagger-ui.html
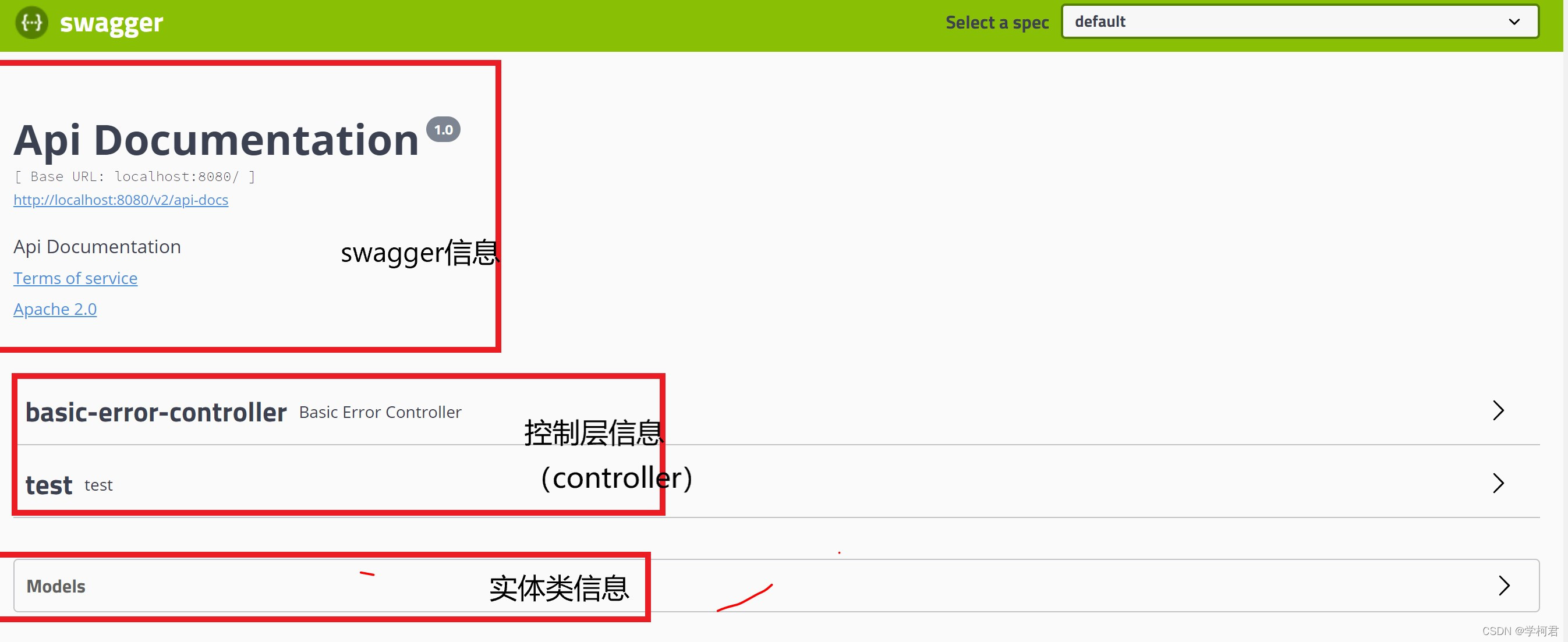
配置swagger信息
package com.ljk.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.oas.annotations.EnableOpenApi;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
import java.util.ArrayList;
@Configuration
@EnableOpenApi
public class swaggerconfig {
@Bean
public Docket docket(){
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo());
}
public ApiInfo apiInfo(){
Contact contact = new Contact("学柯君", "www.baidu.com", "sdadads");
return new ApiInfo("学习日记",
"对学习的记录",
"v1.0",
"www.baidu.com",
contact,
"Apache 2.0",
"http://www.apache.org/licenses/LICENSE-2.0",
new ArrayList());
}
}
## 启动swagger
```java
public class swaggerconfig {
@Bean
public Docket docket(){
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.enable(false)
}
如果我们只希望在开发环境中使用,在发布时不使用
@Configuration
@EnableOpenApi
public class swaggerconfig {
@Bean
public Docket docket(Environment environment){
Profiles profiles =Profiles.of("dev","test");
boolean flag = environment.acceptsProfiles(profiles);
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.enable(flag)
}
创建多个swagger日志
package com.ljk.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.env.Environment;
import org.springframework.core.env.Profiles;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.oas.annotations.EnableOpenApi;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
import java.util.ArrayList;
@Configuration
@EnableOpenApi
public class swaggerconfig {
@Bean
public Docket docket1(){
return new Docket(DocumentationType.SWAGGER_2).groupName("DDD");
}
@Bean
public Docket docket2(){
return new Docket(DocumentationType.SWAGGER_2).groupName("DD2");
}
@Bean
public Docket docket3(){
return new Docket(DocumentationType.SWAGGER_2).groupName("D");
}
@Bean
public Docket docket(Environment environment){
Profiles profiles =Profiles.of("dev","test");
boolean flag = environment.acceptsProfiles(profiles);
return new Docket(DocumentationType.SWAGGER_2).groupName("学柯君")
.apiInfo(apiInfo())
.enable(flag)
.select()
.apis(RequestHandlerSelectors.basePackage("com.ljk.controller"))
.paths(PathSelectors.ant("/ljk/**"))
.build();
}
public ApiInfo apiInfo(){
Contact contact = new Contact("学柯君", "www.baidu.com", "sdadads");
return new ApiInfo("学习日记",
"对学习的记录",
"v1.0",
"www.baidu.com",
contact,
"Apache 2.0",
"http://www.apache.org/licenses/LICENSE-2.0",
new ArrayList());
}
}
swagger配置扫描接口
实体类
package com.ljk.pojo;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
@ApiModel("用户类")
public class user {
@ApiModelProperty("用户名")
public String name;
@ApiModelProperty("用户密码")
public String pwd;
}
controller
package com.ljk.controller;
import com.ljk.pojo.user;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class helloController {
@GetMapping(value = "/hello")
public String hello(){
return "hello swagger";
}
@PostMapping("/user")
public user user(){
return new user();
}
@ApiOperation("hello控制类")
@GetMapping("/hello2")
public String hello2(@ApiParam("用户名") String name){
return "hello"+name;
}
@ApiOperation("post测试方法")
@GetMapping("/postt")
public user postt(@ApiParam("类") user user){
return user;
}
}
总结
- 我们可以通过swagger给一些比较难理解的属性和接口,增加注释
- 接口文档实时更新
- 可以在线测试
注意:发布项目是一定关闭
常用注解
@Api():
- 作用:Api该注解用在类上标记一个Controller类作为文档源
- 属性:tags表示接口说明,可以多配置
- 语法格式:
@Api(tags={"类名"})
实例:
@Api(tags = {"用户接口"})
@RestController
public class test {
@RequestMapping("/hello")
public String hello(){
return "HELLO";
}
}
结果: 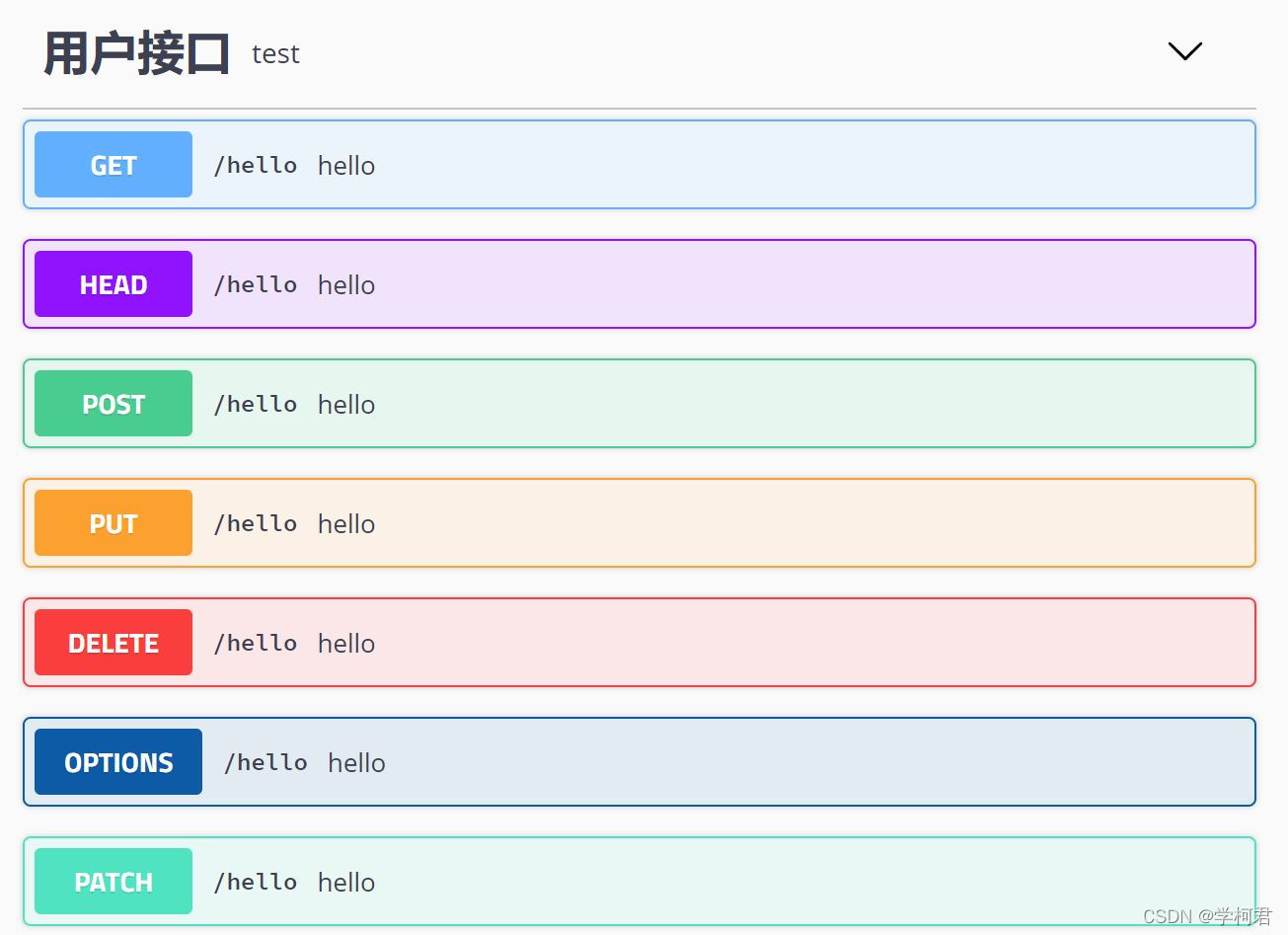
@ApiModel
-
作用:该注解用在类上,表示对类进行说明,用于实体类的参数接受说明 -
属性:
- value:类所在的包
- description :对类的作用描述
-
实例 @ApiModel(value = "com.swaggertest.entity.User",description = "用户类")
public class User {
private int id;
private String name;
private String phone;
private String address;
private String age;
}
@ApiModelProperty
-
作用:用于字段,表示对 model 属性的说明 -
实例: @ApiModel(value = "com.swaggertest.entity.User",description = "用户类")
public class User {
@ApiModelProperty(value = "ID")
private int id;
@ApiModelProperty(value = "名字")
private String name;
@ApiModelProperty(value = "手机")
private String phone;
@ApiModelProperty(value = "地址")
private String address;
@ApiModelProperty(value = "年龄")
private String age;
}
-
结果 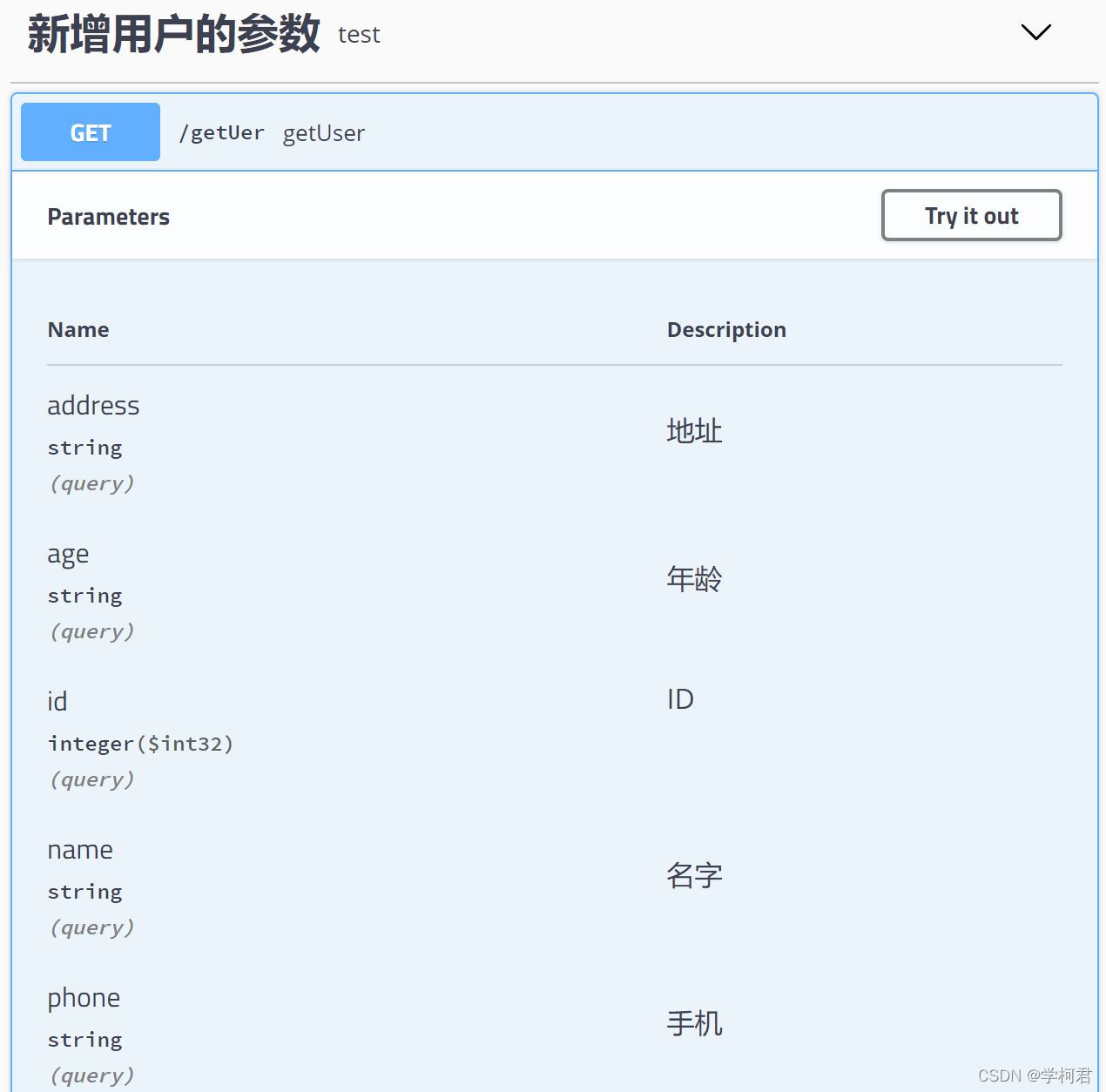
@ApiParam
-
@ApiParam用于Controller中方法的参数说明
- value:参数说明
- required:是否必填
-
实例 @RequestMapping("/getUer")
public User getUser(@ApiParam(value = "新增用户参数" ,required = true) User user){
return new User(1,"sda","sda","sda","sad");
}
@ApiOperation
-
作用:@ApiOperation,用在Controller里的方法上,说明方法的作用 -
属性:
- value接口名称
- notes:详细说明
-
实例 @RequestMapping("/getUer")
@ApiOperation(value = "得到用户",notes = "详细描述")
public User getUser(@ApiParam(value = "新增用户参数" ,required = true) User user){
return new User(1,"sda","sda","sda","sad");
}
-
结果: 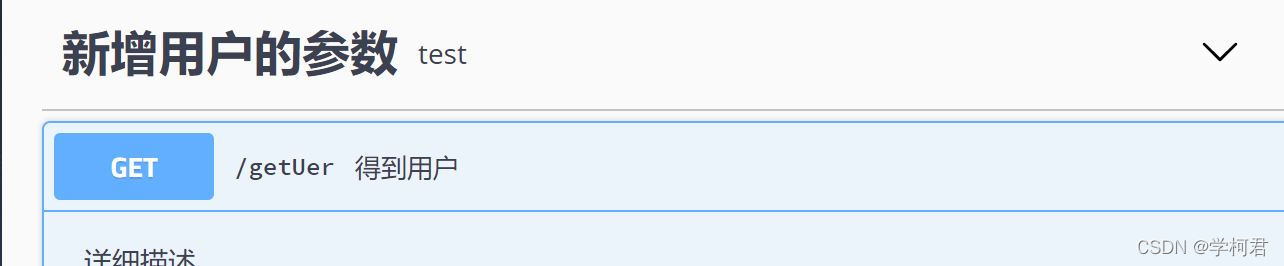
ApiResponse 和 ApiResponses
-
作用:@ApiResponse 用于方法上,说明接口响应的一些信息;@ApiResponses 组装了多个 @ApiResponse -
实例:
-
@ApiResponses({@ApiResponse(code = 200,message = "ok",response = User.class)})
@RequestMapping("/getUer")
@ApiOperation(value = "得到用户",notes = "详细描述")
public User getUser(@ApiParam(value = "新增用户参数" ,required = true) User user){
return new User(1,"sda","sda","sda","sad");
}
ApiImplicitParam 和 ApiImplicitParams
-
作用:用于方法上,为单独的请求参数进行说明 -
属性
- name:参数名,对应方法中单独的参数名称。
- value:参数中文说明。
- required:是否必填。
- paramType:参数类型,取值为 path、query、body、header、form。
- dataType:参数数据类型。
- defaultValue:默认值。
-
实例 @ApiImplicitParams({@ApiImplicitParam(name = "id",value = "用户ID",dataType = "STRING")})
|