写再前面: 1,本文为Java学习第五部分第一阶段所有项目回顾(快递e站部分-对应Java体系第一部分第三阶段-10-集合) 2,整体项目系列博客(包括整体快递e站系列) 3,完整的Java体系链接(比你想的更多的Java内容)
写在前面的话
10-集合那里我没有把练习作业写上,当然不止这一个练习作业。哪些作业在网盘里都有体现。
需求介绍:
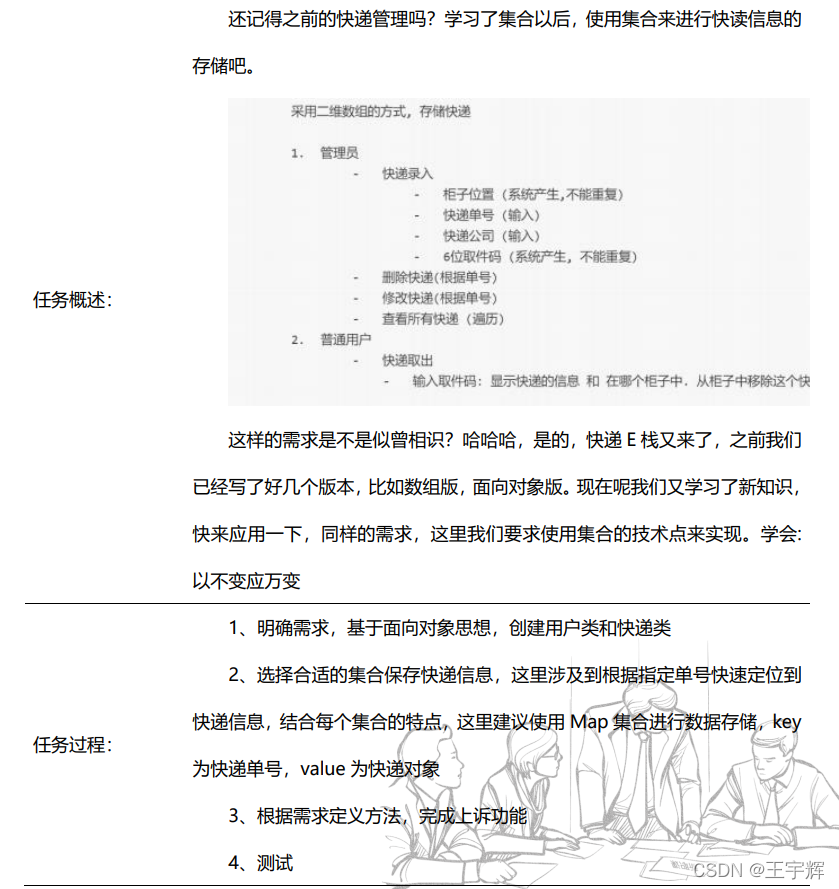
涉及到的知识点:
上一版: 面向对象+异常处理 这一版: 面向对象+集合
代码运行成功截图:
查看所有快递
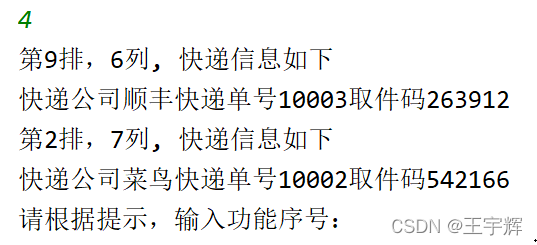
快递录入
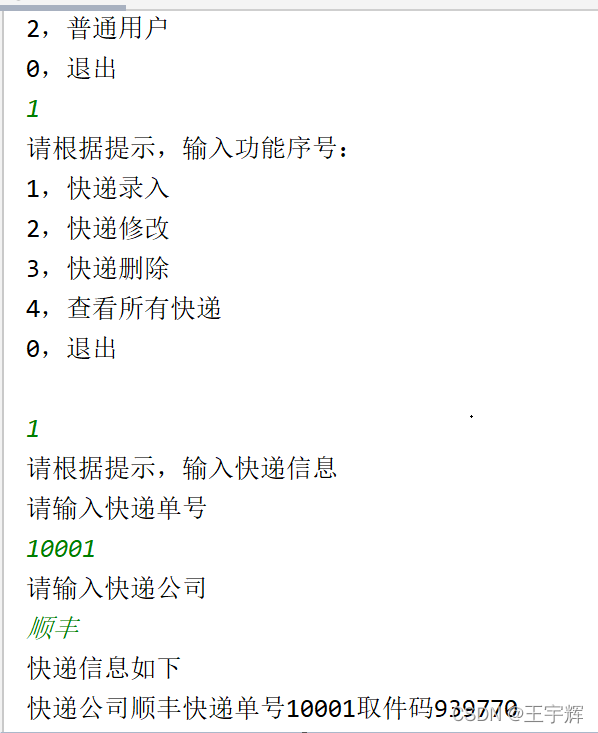
快递删除
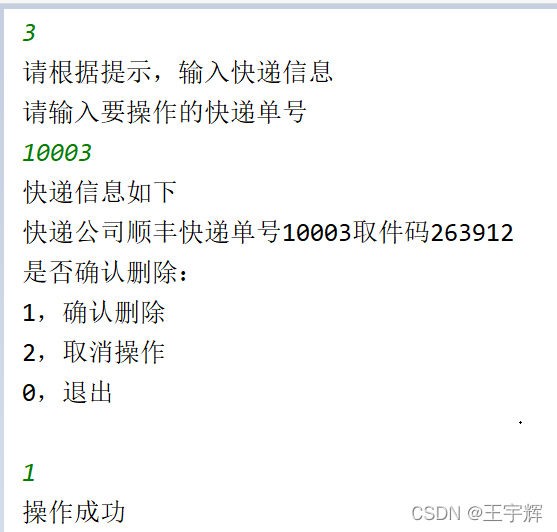
快递修改
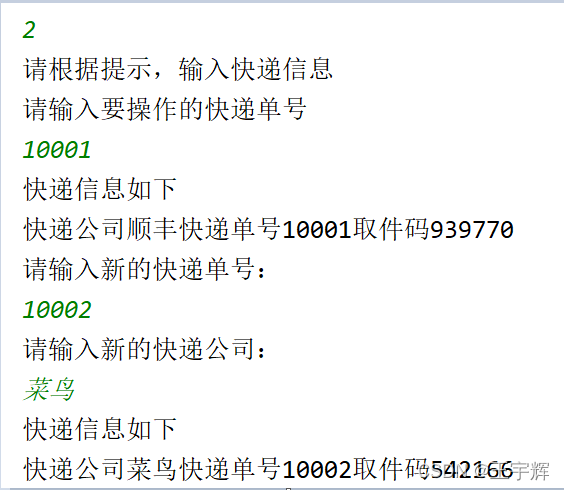
用户取快递
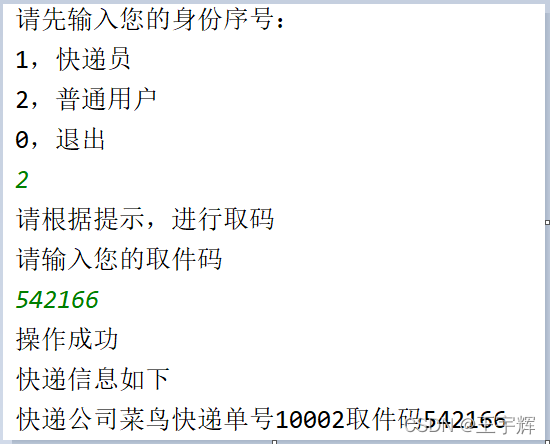
思路分析:
毕竟用自己的思路讲一遍 上一版的流程图如下:(毕竟核心的逻辑就是那么回事) 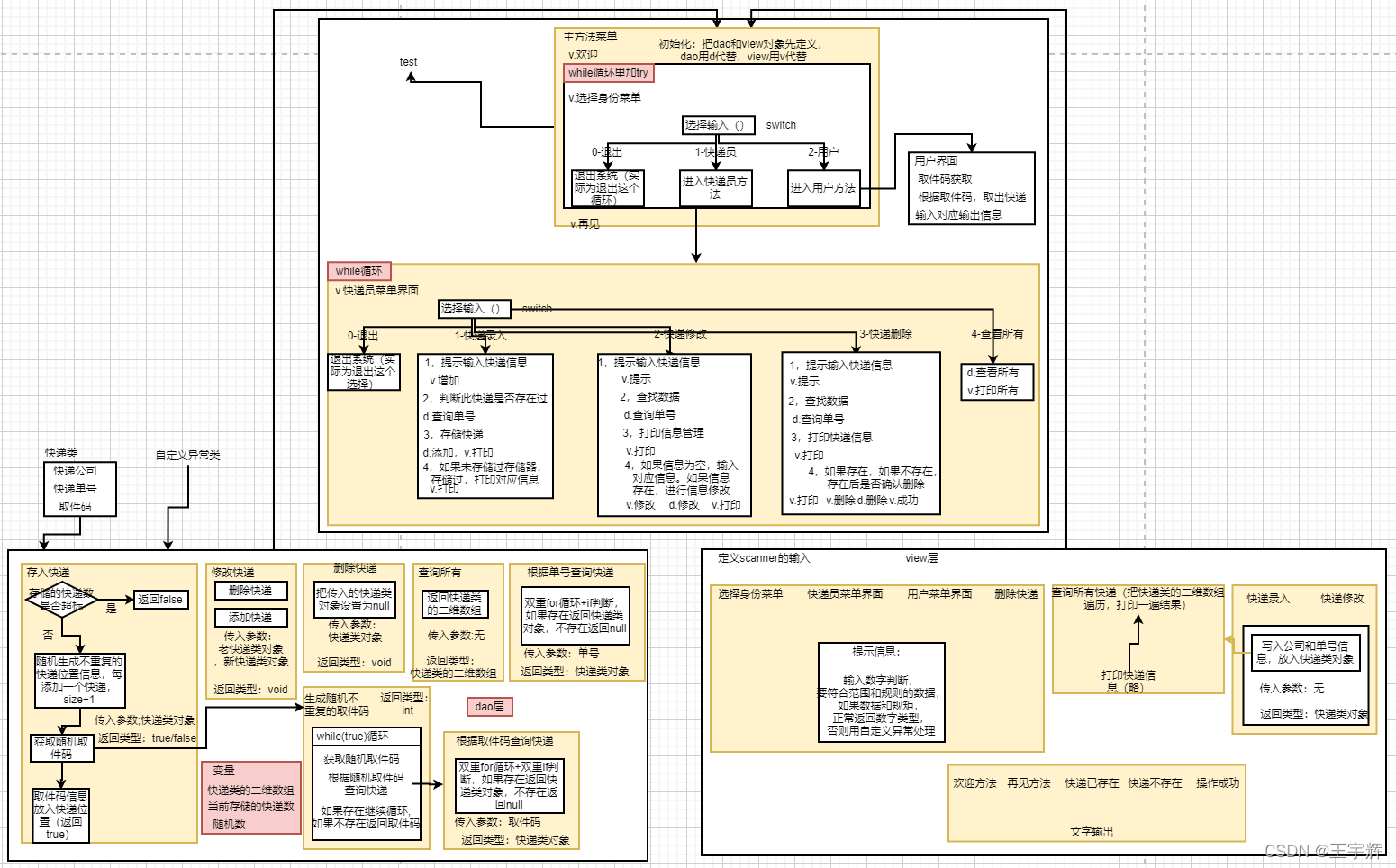 这次把集合加进去了,流程倒是没什么大的改动,代码上加了一些细节的改变。 说一下和上一版相比的改动: bean层: 快递实体类上加入了x,y坐标,意义是表示快递点里快递的位置。 dao层: 之前的二维数组分成了俩份,使用了一个布尔类型的数组表示快递点里快递的位置,HashMap代替二维数组表示快递柜(取件码和快递信息为键值对)。Collection集合存放所有的Express对象,size和random照旧。删除快递为移除键值对,坐标设置为false,size–。 view层:完善了打印快递信息方法,改善了遍历显示所有快递信息(原来是二维数组,现在是Collection集合) main层:和原来一样。 exception和test类:和原来一样
代码:
bean层
package com.wyh.rewu.bean;
import java.util.Objects;
public class Expresss {
private String number;
private String company;
private int code;
public Integer x;
public Integer y;
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
public Integer getX() {
return x;
}
public void setX(Integer x) {
this.x = x;
}
public Integer getY() {
return y;
}
public void setY(Integer y) {
this.y = y;
}
public Expresss() {
}
public Expresss(String number, String company, int code, Integer x, Integer y) {
this.number = number;
this.company = company;
this.code = code;
this.x = x;
this.y = y;
}
@Override
public String toString() {
return "Expresss{" +
"number='" + number + '\'' +
", company='" + company + '\'' +
", code=" + code +
", x=" + x +
", y=" + y +
'}';
}
}
dao层
package com.wyh.rewu.dao;
import com.wyh.rewu.bean.Expresss;
import java.util.*;
public class ExpressDao {
boolean[][] cabinet = new boolean[10][];
HashMap<Integer, Expresss> data = new HashMap<>();
Collection<Expresss> expressList = data.values();
private int size=0;
private Random random = new Random();
{
for(int i = 0; i < 10; i++){
cabinet[i] = new boolean[10];
}
}
public boolean add(Expresss e){
if(this.size >= 100){
return false;
}
size++;
int x = -1;
int y = -1;
while (true){
x = random.nextInt(10);
y= random.nextInt(10);
if (cabinet[x][y] == false){
break;
}
}
int code = radomcode();
e.setCode(code);
e.x = x;
e.y = y;
size++;
cabinet[x][y] = true;
data.put(code, e);
return true;
}
public int radomcode(){
while (true){
int code = random.nextInt(900000)+100000;
Expresss e = findBycode(code);
if (e == null){
return code;
}
}
}
public Expresss findByNumber(String number){
for(Expresss e : expressList) {
if(e.getNumber().equals(number)) {
return e;
}
}
return null;
}
public Expresss findBycode(int code){
for(Expresss e : expressList) {
if(e.getCode() == code) {
return e;
}
}
return null;
}
public void update(Expresss oldExpress,Expresss newExpress){
delete(oldExpress);
add(newExpress);
}
public void delete(Expresss e){
data.remove(e.getCode());
cabinet[e.x][e.y] = false;
size--;
}
public Collection<Expresss> findAll(){
return expressList;
}
}
view层
package com.wyh.rewu.view;
import com.wyh.rewu.bean.Expresss;
import com.wyh.rewu.exception.OutNumberBoundException;
import java.util.Collection;
import java.util.Scanner;
public class Views {
private static Scanner input = new Scanner(System.in);
public void welcome() {
System.out.println("欢迎来到开课吧快递管理系统");
}
public void bye() {
System.out.println("欢迎下次使用");
}
public static int menu() throws NumberFormatException, OutNumberBoundException {
System.out.println("请先输入您的身份序号:\n"
+ "1,快递员\n"
+ "2,普通用户\n"
+ "0,退出");
String text = input.nextLine();
int num = -1;
try {
num = Integer.valueOf(text);
if (num < 0 || num > 2) {
throw new OutNumberBoundException("数字范围0-2");
}
return num;
} catch (NumberFormatException e) {
throw new NumberFormatException("请按规则输入数字");
}
}
public int cMenu() {
System.out.println("请根据提示,输入功能序号:\n"
+ "1,快递录入\n"
+ "2,快递修改\n"
+ "3,快递删除\n"
+ "4,查看所有快递\n"
+ "0,退出\n"
);
String text = input.nextLine();
int num = -1;
try {
num = Integer.parseInt(text);
} catch (NumberFormatException e) {
System.out.println("请按规则输入数字");
return cMenu();
}
if (num < 0 || num > 4) {
System.out.println("输入错误,请重新输入~");
return cMenu();
}
return num;
}
public int uMenu() throws OutNumberBoundException {
System.out.println("请根据提示,进行取码");
System.out.println("请输入您的取件码");
String code = input.nextLine();
int num = -1;
try {
num = Integer.parseInt(code);
} catch (NumberFormatException e) {
System.out.println("请按规则输入数字");
return menu();
}
if (num < 100000 || num > 999999) {
System.out.println("输入错误,请重新输入~");
return menu();
}
return num;
}
public Expresss insert(){
System.out.println("请根据提示,输入快递信息");
System.out.println("请输入快递单号");
String number = input.nextLine();
System.out.println("请输入快递公司");
String company = input.nextLine();
Expresss e = new Expresss();
e.setCompany(company);
e.setNumber(number);
return e;
}
public Expresss update(){
System.out.println("请根据提示,输入快递信息");
System.out.println("请输入要修改的快递单号");
String number = input.nextLine();
System.out.println("请输入快递公司");
String company = input.nextLine();
Expresss e = new Expresss();
e.setCompany(company);
e.setNumber(number);
return e;
}
public String findByNumber(){
System.out.println("请根据提示,输入快递信息");
System.out.println("请输入要操作的快递单号");
String number = input.nextLine();
return number;
}
public void printExpress(Expresss e){
if(e == null){
System.out.println("快递信息不存在");
return;
}
System.out.println("快递信息如下");
System.out.println("快递公司"+e.getCompany()+"快递单号"+e.getNumber()+"取件码"+e.getCode());
}
public void update(Expresss e){
System.out.println("请输入新的快递单号:");
String number = input.nextLine();
System.out.println("请输入新的快递公司:");
String company = input.nextLine();
e.setNumber(number);
e.setCompany(company);
}
public int delete() throws OutNumberBoundException {
System.out.println("是否确认删除:\n"
+ "1,确认删除\n"
+ "2,取消操作\n"
+ "0,退出\n"
);
String text = input.nextLine();
int num = -1;
try {
num = Integer.parseInt(text);
} catch (NumberFormatException e) {
System.out.println("请按规则输入数字");
return menu();
}
if (num < 0 || num > 2) {
System.out.println("输入错误,请重新输入~");
return menu();
}
return num;
}
public void printAll(Collection<Expresss> es){
int count = 0;
for(Expresss e : es) {
count++;
System.out.print("第" + (e.x + 1) + "排," + (e.y+ 1) + "列, ");
printExpress(e);
}
if(count == 0){
System.out.println("暂无快递信息");
}
}
public void expressExit() {
System.out.println("此单号在快递中已经存在,请勿重复存储");
}
public void printNull(){
System.out.println("快递不存在,请检查您的输入");
}
public void printCode(Expresss e){
System.out.println("快递的取件码为: "+e.getCode());
}
public void success(){
System.out.println("操作成功");
}
}
main层
package com.wyh.rewu.main;
import com.wyh.rewu.bean.Expresss;
import com.wyh.rewu.dao.ExpressDao;
import com.wyh.rewu.exception.OutNumberBoundException;
import com.wyh.rewu.view.Views;
import java.util.Collection;
public class Main {
private static Views views = new Views();
private static ExpressDao expressDao = new ExpressDao();
public static void start() throws OutNumberBoundException {
views.welcome();
m:while(true){
try {
int menu = views.menu();
switch(menu){
case 0:
break m;
case 1:
cClient();
break;
case 2:
uClient();
break;
}
}catch (OutNumberBoundException | NumberFormatException e){
System.err.println(e.getMessage());
start();
}
}
views.bye();
}
private static void cClient() throws OutNumberBoundException {
while(true){
int menu = views.cMenu();
switch(menu){
case 0:
return;
case 1: {
Expresss e = views.insert();
Expresss e2 = expressDao.findByNumber(e.getNumber());
if (e2 == null) {
expressDao.add(e);
views.printExpress(e);
}else{
views.expressExit();
}
}break;
case 2: {
String number = views.findByNumber();
Expresss e = expressDao.findByNumber(number);
if (e == null) {
views.printNull();
}else{
views.printExpress(e);
views.update(e);
expressDao.update(e,e);
views.printExpress(e);
}
}break;
case 3: {
String number = views.findByNumber();
Expresss e = expressDao.findByNumber(number);
if (e == null) {
views.printNull();
}else{
views.printExpress(e);
int type = views.delete();
if (type == 1){
expressDao.delete(e);
views.success();
}
}
}break;
case 4: {
Collection<Expresss> data = expressDao.findAll();
views.printAll(data);
}break;
}
}
}
private static void uClient() throws OutNumberBoundException {
int code = views.uMenu();
Expresss e = expressDao.findBycode(code);
if (e == null) {
views.printNull();
} else {
views.success();
views.printExpress(e);
}
}
}
其他:
test启动系统
package com.wyh.rewu;
import com.wyh.rewu.exception.OutNumberBoundException;
import com.wyh.rewu.main.Main;
public class text {
public static void main(String[] args) throws OutNumberBoundException {
Main main = new Main();
main.start();
}
}
exception异常类
package com.wyh.rewu.exception;
public class OutNumberBoundException extends Throwable {
public OutNumberBoundException(String s) {
super(s);
}
}
|