一、流程图
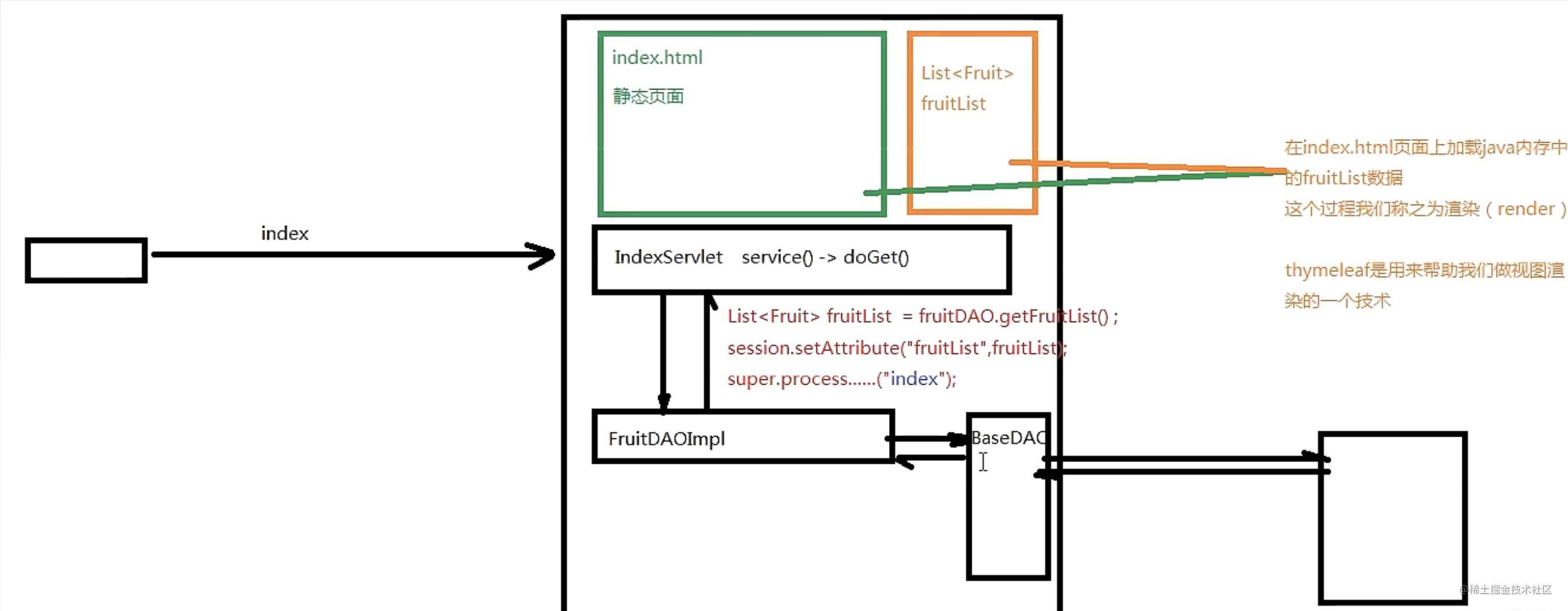
二、代码实现
- 添加thymeleaf的jar包
- 在web.xml文件中添加配置
<context-param>
<param-name>view-prefix</param-name>
<param-value>/</param-value>
</context-param>
<context-param>
<param-name>view-suffix</param-name>
<param-value>.html</param-value>
</context-param>
- 新建一个Servlet类ViewBaseServlet
public class ViewBaseServlet extends HttpServlet {
private TemplateEngine templateEngine;
@Override
public void init() throws ServletException {
ServletContext servletContext = this.getServletContext();
ServletContextTemplateResolver templateResolver = new ServletContextTemplateResolver(servletContext);
templateResolver.setTemplateMode(TemplateMode.HTML);
String viewPrefix = servletContext.getInitParameter("view-prefix");
templateResolver.setPrefix(viewPrefix);
String viewSuffix = servletContext.getInitParameter("view-suffix");
templateResolver.setSuffix(viewSuffix);
templateResolver.setCacheTTLMs(60000L);
templateResolver.setCacheable(true);
templateResolver.setCharacterEncoding("utf-8");
templateEngine = new TemplateEngine();
templateEngine.setTemplateResolver(templateResolver);
}
protected void processTemplate(String templateName, HttpServletRequest req, HttpServletResponse resp) throws IOException {
resp.setContentType("text/html;charset=UTF-8");
WebContext webContext = new WebContext(req, resp, getServletContext());
templateEngine.process(templateName, webContext, resp.getWriter());
}
}
- Servlet继承VIewBaseServlet
@WebServlet("/index")
public class IndexServlet extends ViewBaseServlet{
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
FruitDAO fruitDAO = new FruitDAOImpl();
List<Fruit> fruitList = fruitDAO.getFruitList();
fruitList.forEach(System.out::println);
HttpSession session = req.getSession();
session.setAttribute("fruitList",fruitList);
super.processTemplate("index",req,resp);
}
}
- 用thymeleaf渲染页面
- th:if 如果
- th:unless 否则
- th:each 循环
- th:text 获取文本
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="css/index.css">
</head>
<body>
<div id="div_container">
<div id="div_fruit_list">
<table id="tbl_fruit">
<tr>
<th class="w20">名称</th>
<th class="w20">单价</th>
<th class="w20">库存</th>
<th>操作</th>
</tr>
<tr th:if="${#lists.isEmpty(session.fruitList)}">
<td colspan="4">对不起,库存为空</td>
</tr>
<tr th:unless="${#lists.isEmpty(session.fruitList)}" th:each="fruit : ${session.fruitList}">
<td th:text="${fruit.fname}"></td>
<td th:text="${fruit.price}"></td>
<td th:text="${fruit.fcount}"></td>
<td><img src="/fruit1.4-thymeleaf/imgs/del.jpg" class="delImg"/></td>
</tr>
</table>
</div>
</div>
</body>
</html>
三、总结
1.编码
post提交需要设置编码,防治中文乱码
request.setCharacterEncoding("utf-8")
get提交方式,tomcat8开始,不需要设置
tomcat8之前, String fname = request.getParameter("fname");
byte[] bytes = fname.getBytes("iso-8859-1");
fname = new String(bytes,"UTF-8);
2.继承关系以及生命周期
1)Servlet接口:init、service、destroy
HttpServlet实现了service方法,通过request.getMethod判断请求方式
根据请求方式调用内部的do方法。每个do方法进行简单实现,如果请求方式不符合 报错405。
2)生命周期:实例化,初始化,服务,销毁
Tomcat负责维护Servlet实例的生命周期
每个Servlet在Tomcat容器中只有一个实例,线程不安全
Servlet启动时机--<load-on-startup>
3.HTTP协议
1)由Request和Response两部分组成
2)请求包含:请求行、请求消息头、请求主体:query String、form data、request payload
3)响应包含:响应行、响应消息头、相应主体
4.HttpSession
1)HttpSession:表示会话
2)为什么需要HttpSession:因为Http协议是无状态的
3)Session保存作用域:一次会话范围都有效
5.服务器转发和客户端重定向
1)服务器转发:request.getRequestDispatcher("index.html").forward(request,response)
2)客户端重定向:
response.sendRedirect("index.html")
|