一、@ModelAttribute
- 用法
- 绑定请求参数到命令对象(入参对象):放在控制器方法的入参上时,用于将多个请求参数绑定到一个命令对象,从而简化绑定流程,而且自动暴露为模型数据用于视图页面展示时使用;当参数类型是一个bean的时候,它可以按照bean的属性一一注入。
@ModelAttribute("LoginUser") User user - 暴露表单引用对象为模型数据:
放在处理器的一般方法(非功能处理方法,也就是没有@RequestMapping标注的方法)上时 ,是为表单准备要展示的表单引用数据对象:如注册时需要选择的所在城市等静态信息。它在执行功能处理方法(@RequestMapping 注解的方法)之前,自动添加到模型对象中 ,用于视图页面展示时使用;该方式基本在某个父类Controller中包含该方法,然后子类继承它,这样所有的子类Controller就都能自动在对应的view中直接访问dicts,而无需每个都重复一遍 - @ModelAttribute 和 @RequestMapping 注解同时应用在方法上时,有以下作用:
- 方法的
返回值会存入到 Model 对象中,key 为 ModelAttribute 的 value 属性值。 - 方法的
返回值不再是方法的访问路径,访问路径会变为 @RequestMapping 的 value 值,例如:@RequestMapping(value = "/index") 跳转的页面是 index.jsp 页面 。
- 代码例子
abstract public class CommonController {
@ModelAttribute
public void MyModelAttribute(Model model){
model.addAttribute("model", "MyModel");
}
@ModelAttribute
public void newUser(Model model){
User user = new User();
user.setName("myUser");
user.setId(1);
model.addAttribute("myUser", user);
}
}
@Controller
public class MyController {
@RequestMapping("init")
@ResponseBody
public String init(){
return "init";
}
@RequestMapping("user")
@ResponseBody
public void getUser(@ModelAttribute("myUser")User user){
System.out.println(user.toString());
}
@ModelAttribute("modelAndMapping")
@RequestMapping("yes")
public String modelAttributeAndRequestMapping(){
return "yes1";
}
}
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>yes.jsp</title>
</head>
<body>
${modelAndMapping}
</body>
</html>
二、@SessionAttributes和@SessionAttribute
-
作用:作用是将指定的Model中的键值对添加至session中,方便在下一次请求中使用该注解只能作用与类上 。 -
@SessionAttributes注解设置的参数有3类方式去使用它:
- 在视图view中(比如jsp页面等)通过
request.getAttribute()或session.getAttribute 获取 - 在后面请求返回的视图view中通过session.getAttribute或者从model中获取(这个也比较常用)
- 自动将参数设置到后面请求所对应处理器的Model类型参数或者有@ModelAttribute注释的参数里面(结合@ModelAttribute一起使用应该是我们重点关注的),
注意,在别的controller中使用@ModelAttribute拿不到,只有在本控制器中使用@ModelAttribute才能拿到该属性,在别的controller中可使用@SessionAttribute来获取
@SessionAttributes(value = {"user","id","name"})
@Controller
public class UserController {
@ModelAttribute("user")
public User login(){
User user = new User();
user.setName("loginYES");
user.setId(2);
return user;
}
@RequestMapping("newUser")
public String testHandler(Model model){
model.addAttribute("id",23);
model.addAttribute("name","kk");
return "result";
}
@ResponseBody
@RequestMapping("iisLogin")
public String isLogin(@ModelAttribute("user") User user) {
if (user.getName() != null) {
return "isLogin!";
} else return "isLogin??";
}
}
@Controller
public class MyController {
@ResponseBody
@RequestMapping("isLogin")
public String isLogin(@ModelAttribute("user") User user,HttpSession httpSession){
if(user.getName()!=null){
return "isLogin!";
}
else return "isLogin??";
}
}
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>result</title>
</head>
<body>
${sessionScope.user.id}
${sessionScope.user.name}
<br/>
${sessionScope.id}
${sessionScope.name}
</body>
</html>
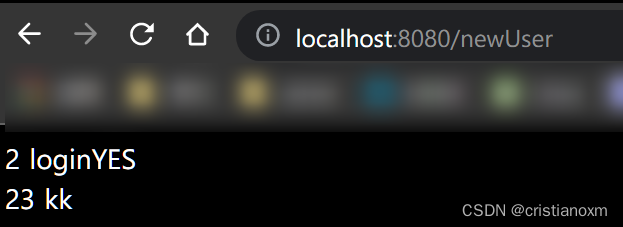
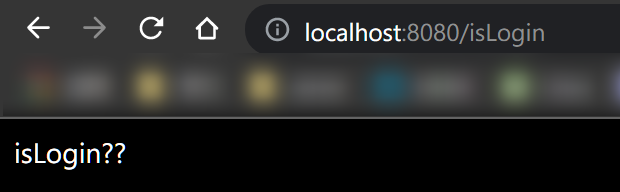 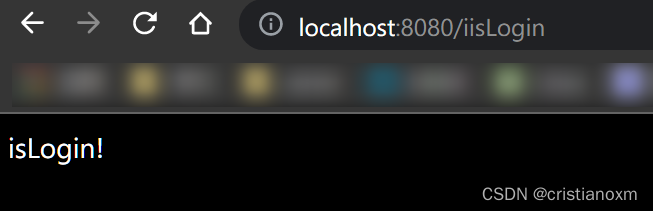
@SessionAttributes 是将model设置到session中去。 @SessionAttribute 是从session获取之前设置到session中的数据。
四、@RequestAttribute
- 作用:用在方法入参上,
从request中取对应的值,至于request中是怎么存在该属性的,方式多种多样,拦截器中预存、ModelAttribute注解预存、请求转发带过来的 。
尝试访问一个request中不存在的值时,@RequestAttribute抛出异常 :
@RequestMapping("/demo1")
public String demo1(@RequestAttribute String name){
System.out.println(name);
return "test";
}
- 请求request中预存属性的方式:
@ModelAttribute
public void storeEarly(HttpServletRequest request){
request.setAttribute("name","lvbinbin");
}
- 方式二.拦截器中request.setAttribute()
public class SimpleInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
System.out.println(" Simple Interceptor preHandle");
request.setAttribute("name",24);
return true;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception {
System.out.println(" Simple Interceptor postHandle");
}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception {
System.out.println(" Simple Interceptor afterCompletion");
}
}
- @RequestAttribute属性required
@RequestAttribute属性required默认为true, request.getAttribute获取不到参数就会抛出异常 ServletRequestBindingException ;当 required设置为false,即使没有从request中获取到就忽略跳过,赋值为null;
- @RequestAttribute和@RequestParam和@RequestBody
- @RequestAttribute注解的参数在项目里是自己解析出来的,
并不是前端传递的。 具体一点,在项目里的拦截器里会对Token信息进行解析,解析出来的参数重新放在请求里(用httpServletRequest.setAttribute(name,value)),后边接口接收参数时就用这个注解。 - @RequestParam注解则表示这个参数是通过前端传递过来的,
如果请求里没有这个参数,则会报错400 Bad Request。这个注解用来解析请求路径里的参数(get请求)或者post请求中form表单格式的请求参数 ; - @RequestBody注解
用来接收POST请求BODY里的参数,格式为JSON格式。
参考文章
|