java.util.Date
package com.yuzhenc.common;
import java.util.Date;
public class Test02 {
public static void main(String[] args) {
Date date = new Date();
System.out.println(date);
System.out.println(date.toGMTString());
System.out.println(date.toLocaleString());
System.out.println(date.getYear());
System.out.println(date.getMonth());
System.out.println(date.getTime());
System.out.println(System.currentTimeMillis());
long startTime = System.currentTimeMillis();
for (int i = 0; i < 10000000; i++) {
}
long endTime = System.currentTimeMillis();
System.out.println(endTime-startTime);
}
}
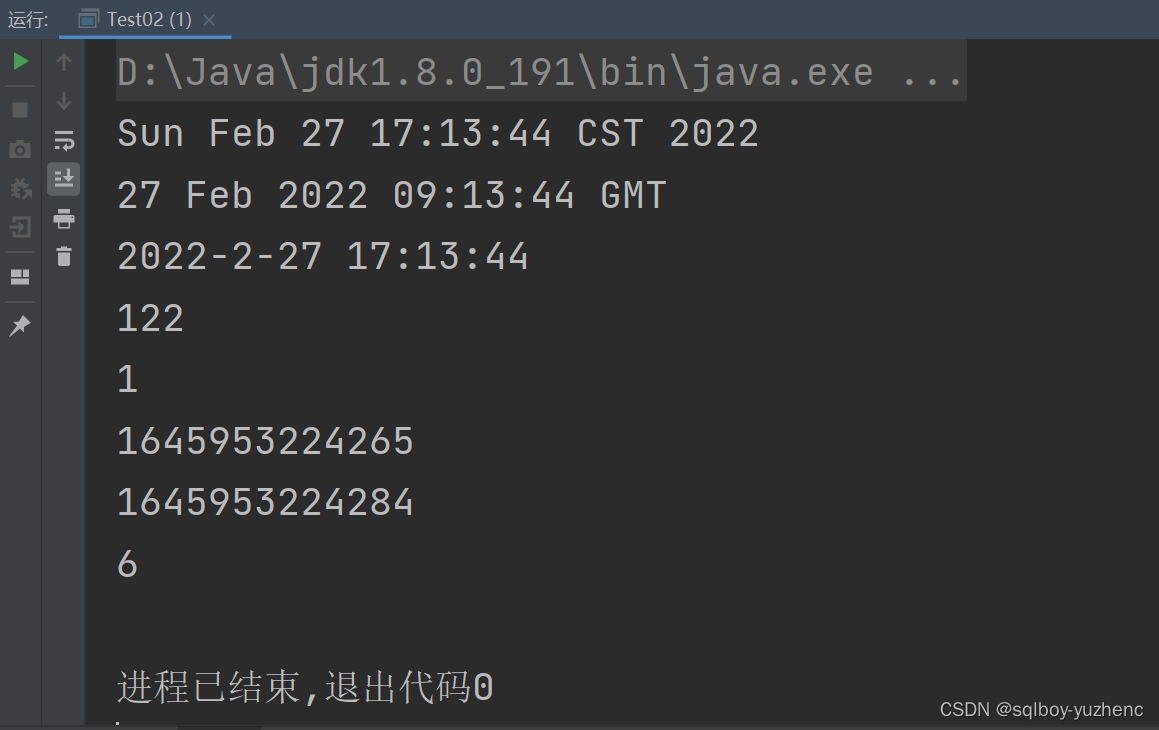
java.sql.Date
package com.yuzhenc.common;
import java.sql.Date;
public class Test03 {
public static void main(String[] args) {
java.util.Date date = new Date(System.currentTimeMillis());
Date sqlDate = (Date)date;
sqlDate = new Date(date.getTime());
date = sqlDate;
sqlDate = Date.valueOf("2022-02-27");
System.out.println(sqlDate);
System.out.println(date);
}
}
SimpleDateFormat
package com.yuzhenc.common;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Test04 {
public static void main(String[] args) {
DateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS");
String format = df.format(new Date());
System.out.println(format);
try {
Date d = df.parse("2022-02-27 17:57:20.123");
System.out.println(d);
} catch (ParseException e) {
e.printStackTrace();
}
}
}
Date and Time Pattern | Result |
---|
“yyyy.MM.dd G ‘at’ HH:mm:ss z” | 2001.07.04 AD at 12:08:56 PDT | “EEE, MMM d, ''yy” | Wed, Jul 4, '01 | “h:mm a” | 12:08 PM | “hh ‘o’‘clock’ a, zzzz” | 12 o’clock PM, Pacific Daylight Time | “K:mm a, z” | 0:08 PM, PDT | “yyyyy.MMMMM.dd GGG hh:mm aaa” | 02001.July.04 AD 12:08 PM | “EEE, d MMM yyyy HH:mm:ss Z” | Wed, 4 Jul 2001 12:08:56 -0700 | “yyMMddHHmmssZ” | 010704120856-0700 | “yyyy-MM-dd’T’HH:mm:ss.SSSZ” | 2001-07-04T12:08:56.235-0700 | “yyyy-MM-dd’T’HH:mm:ss.SSSXXX” | 2001-07-04T12:08:56.235-07:00 | “YYYY-'W’ww-u” | 2001-W27-3 |
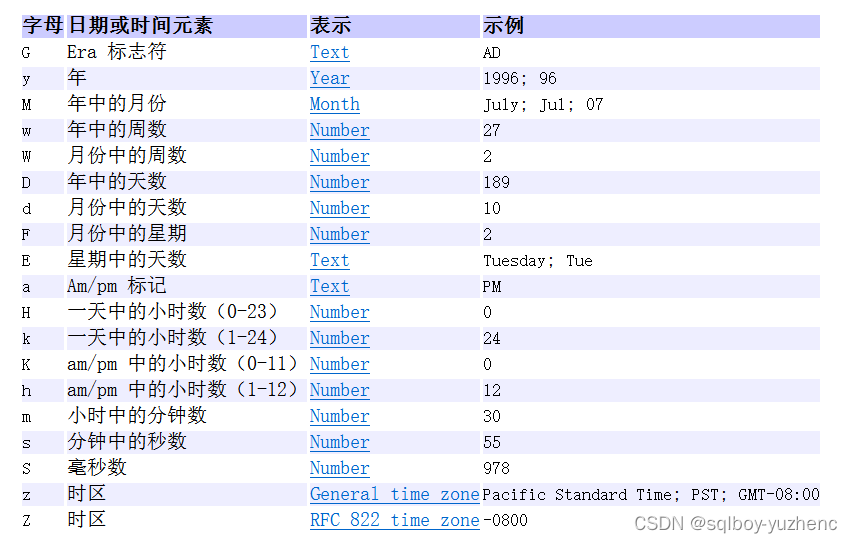
Calendar
package com.yuzhenc.common;
import java.sql.Date;
import java.util.Calendar;
public class Test05 {
public static void main(String[] args) {
String strDate = "2022-10-28";
Date date = Date.valueOf(strDate);
Calendar cal = Calendar.getInstance();
cal.setTime(date);
int year = cal.get(Calendar.YEAR);
int month = cal.get(Calendar.MONTH) + 1;
System.out.println(year + "-" + month + ":");
System.out.println("日\t一\t二\t三\t四\t五\t六\t");
int maxDay = cal.getActualMaximum(Calendar.DATE);
int nowday = cal.get(Calendar.DATE);
cal.set(Calendar.DATE,1);
int num = cal.get(Calendar.DAY_OF_WEEK);
int count = 0;
for (int i = 1; i < num; i++) {
System.out.print("\t");
}
count = count + num;
for (int i = 1; i <= maxDay; i++) {
if (i == nowday) {
System.out.print(i+"*"+"\t");
} else {
System.out.print(i+"\t");
}
if (count%7 == 0) {
System.out.println();
}
count ++;
}
}
}
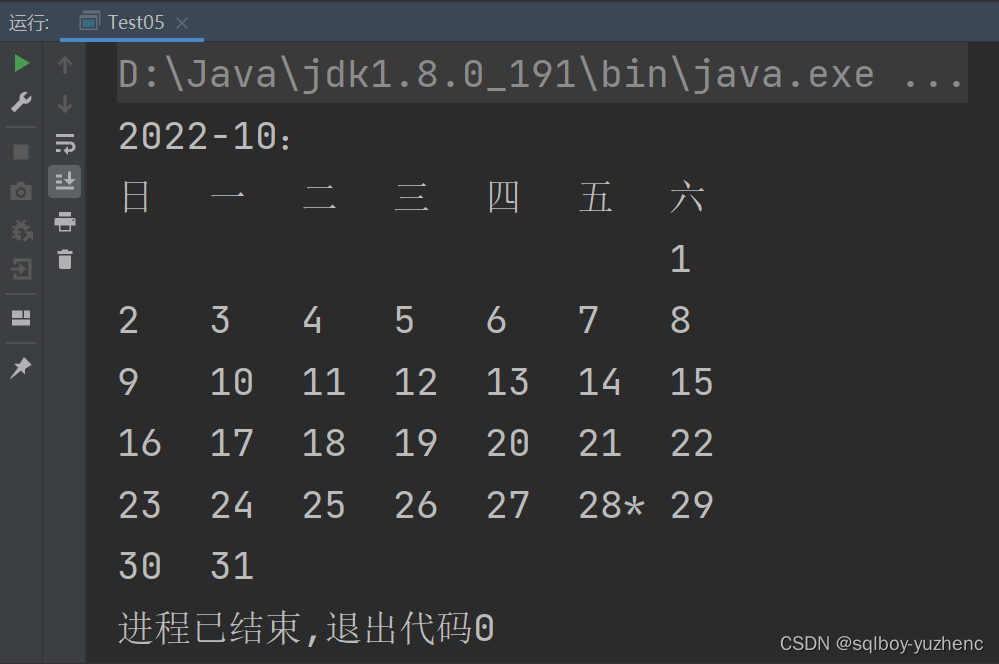
LocalDate/LocalTime/LocalDateTime
package com.yuzhenc.common;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
public class Test06 {
public static void main(String[] args) {
LocalDate localDate = LocalDate.now();
System.out.println(localDate);
LocalTime localTime = LocalTime.now();
System.out.println(localTime);
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println(localDateTime);
LocalDate localDate1 = LocalDate.of(2022,2,27);
System.out.println(localDate1);
LocalTime localTime1 = LocalTime.of(18,13,59,123);
System.out.println(localTime1);
LocalDateTime localDateTime1 = LocalDateTime.of(2022,2,27,18,16,50,959154125);
System.out.println(localDateTime1);
System.out.println(localDateTime.getYear());
System.out.println(localDateTime.getMonth());
System.out.println(localDateTime.getMonthValue());
System.out.println(localDateTime.getDayOfMonth());
System.out.println(localDateTime.getDayOfWeek());
System.out.println(localDateTime.getHour());
System.out.println(localDateTime.getMinute());
System.out.println(localDateTime.getSecond());
LocalDateTime localDateTime2 = localDateTime.withMonth(8);
System.out.println(localDateTime);
System.out.println(localDateTime2);
LocalDateTime localDateTime3 = localDateTime.plusMonths(8);
System.out.println(localDateTime);
System.out.println(localDateTime3);
LocalDateTime localDateTime4 = localDateTime.minusMinutes(8);
System.out.println(localDateTime);
System.out.println(localDateTime4);
}
}
DateTimeFormatter
package com.yuzhenc.common;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.time.format.FormatStyle;
import java.time.temporal.TemporalAccessor;
public class Test07 {
public static void main(String[] args) {
DateTimeFormatter df = DateTimeFormatter.ISO_LOCAL_DATE_TIME;
LocalDateTime localDateTime = LocalDateTime.now();
String str = df.format(localDateTime);
System.out.println(str);
TemporalAccessor parse = df.parse("2022-02-27T18:39:41.098");
System.out.println(parse);
DateTimeFormatter df1 = DateTimeFormatter.ofLocalizedDateTime(FormatStyle.LONG);
LocalDateTime localDateTime1 = LocalDateTime.now();
String str1 = df1.format(localDateTime1);
System.out.println(str1);
TemporalAccessor parse1 = df1.parse("2022年2月27日 下午06时47分19秒");
System.out.println(parse1);
DateTimeFormatter df2 = DateTimeFormatter.ofPattern("yyyy-MM-dd hh:mm:ss.SSS");
LocalDateTime localDateTime2 = LocalDateTime.now();
String str2 = df2.format(localDateTime2);
System.out.println(str2);
TemporalAccessor parse2 = df2.parse("2022-02-27 07:09:32.420");
System.out.println(parse2);
}
}
Formatter | Description | Example |
---|
BASIC_ISO_DATE | Basic ISO date | ‘20111203’ | ISO_LOCAL_DATE | ISO Local Date | ‘2011-12-03’ | ISO_OFFSET_DATE | ISO Date with offset | ‘2011-12-03+01:00’ | ISO_DATE | ISO Date with or without offset | ‘2011-12-03+01:00’; ‘2011-12-03’ | ISO_LOCAL_TIME | Time without offset | ‘10:15:30’ | ISO_OFFSET_TIME | Time with offset | ‘10:15:30+01:00’ | ISO_TIME | Time with or without offset | ‘10:15:30+01:00’; ‘10:15:30’ | ISO_LOCAL_DATE_TIME | ISO Local Date and Time | ‘2011-12-03T10:15:30’ | ISO_OFFSET_DATE_TIME | Date Time with Offset | 2011-12-03T10:15:30+01:00’ | ISO_ZONED_DATE_TIME | Zoned Date Time | ‘2011-12-03T10:15:30+01:00[Europe/Paris]’ | ISO_DATE_TIME | Date and time with ZoneId | ‘2011-12-03T10:15:30+01:00[Europe/Paris]’ | ISO_ORDINAL_DATE | Year and day of year | ‘2012-337’ | ISO_WEEK_DATE | Year and Week | 2012-W48-6’ | ISO_INSTANT | Date and Time of an Instant | ‘2011-12-03T10:15:30Z’ | RFC_1123_DATE_TIME | RFC 1123 / RFC 822 | ‘Tue, 3 Jun 2008 11:05:30 GMT’ |
Symbol | Meaning | Presentation | Examples |
---|
G | era | text | AD; Anno Domini; A | u | year | year | 2004; 04 | y | year-of-era | year | 2004; 04 | D | day-of-year | number | 189 | M/L | month-of-year | number/text | 7; 07; Jul; July; J | d | day-of-month | number | 10 | Q/q | quarter-of-year | number/text | 3; 03; Q3; 3rd quarter | Y | week-based-year | year | 1996; 96 | w | week-of-week-based-year | number | 27 | W | week-of-month | number | 4 | E | day-of-week | text | Tue; Tuesday; T | e/c | localized day-of-week | number/text | 2; 02; Tue; Tuesday; T | F | week-of-month | number | 3 | a | am-pm-of-day | text | PM | h | clock-hour-of-am-pm (1-12) | number | 12 | K | hour-of-am-pm (0-11) | number | 0 | k | clock-hour-of-am-pm (1-24) | number | 0 | H | hour-of-day (0-23) | number | 0 | m | minute-of-hour | number | 30 | s | second-of-minute | number | 55 | S | fraction-of-second | fraction | 978 | A | milli-of-day | number | 1234 | n | nano-of-second | number | 987654321 | N | nano-of-day | number | 1234000000 | V | time-zone ID | zone-id | America/Los_Angeles; Z; -08:30 | z | time-zone name | zone-name | Pacific Standard Time; PST | O | localized zone-offset | offset-O | GMT+8; GMT+08:00; UTC-08:00; | X | zone-offset ‘Z’ for zero | offset-X | Z; -08; -0830; -08:30; -083015; -08:30:15; | x | zone-offset | offset-x | +0000; -08; -0830; -08:30; -083015; -08:30:15; | Z | zone-offset | offset-Z | +0000; -0800; -08:00; | p | pad next | pad modifier | 1 | ’ | escape for text | delimiter | | ‘’ | single quote | literal | ’ | [ | optional section start | | | ] | optional section end | | | # | reserved for future use | | | { | reserved for future use | | | } | reserved for future use | | |
|